In MATLAB, eigenvalues and eigenvectors can be computed using the `eig` function, which takes a square matrix as input and returns the eigenvalues and eigenvectors.
Here's a code snippet demonstrating this:
% Define a square matrix
A = [4, -2; 1, 1];
% Calculate eigenvalues and eigenvectors
[eigVec, eigVal] = eig(A);
Understanding Eigenvalues and Eigenvectors
What Are Eigenvalues?
Eigenvalues are scalars associated with a linear transformation represented by a square matrix. They provide insights into the properties of the transformation and can help in analyzing systems across various fields such as physics, engineering, and data science. Mathematically, an eigenvalue \( \lambda \) can be defined by the equation:
\[ A \cdot v = \lambda \cdot v \]
where \( A \) is the matrix, \( v \) is the eigenvector, and \( \lambda \) is the scalar eigenvalue. Simply put, when a matrix operates on its eigenvector, the output is the same eigenvector scaled by the eigenvalue.
What Are Eigenvectors?
Eigenvectors are non-zero vectors that change at most by a scalar factor during a transformation represented by a matrix. Every eigenvalue corresponds to at least one eigenvector. This relationship is crucial: eigenvalues tell us about the magnitude of the transformation, while eigenvectors indicate the direction.
To visualize, think of a geometrical transformation where the transformation "pulls" or "stretches" vectors. Eigenvectors remain invariant in direction, while the eigenvalues indicate how much stretching occurs.
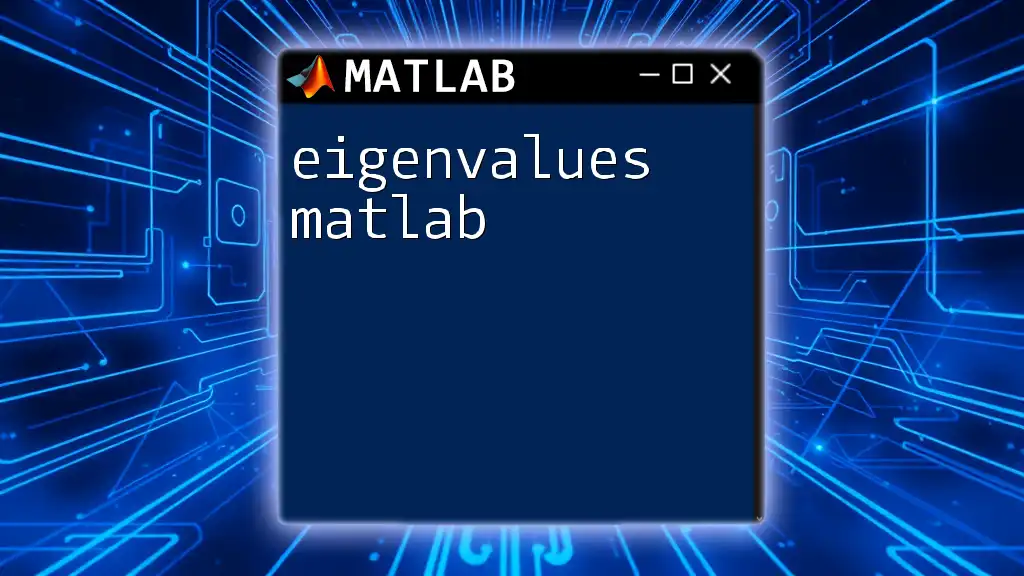
Mathematical Foundation
Linear Transformations
Linear transformations are functions that map vectors to vectors while preserving the operations of vector addition and scalar multiplication. When analyzing a linear transformation defined by a matrix, eigenvalues and eigenvectors become pivotal in understanding how the transformation behaves. They represent the "axes" along which the transformation effectively flows, simplifying complex systems into easier-to-analyze segments.
Characteristic Polynomial
The characteristic polynomial is essential for finding eigenvalues of a matrix. It is derived from the equation:
\[ \text{det}(A - \lambda I) = 0 \]
Where \( I \) is the identity matrix. This determinant forms a polynomial in \( \lambda \). The roots of this polynomial are the eigenvalues.
To find it, follow these steps:
- Construct the matrix \( A - \lambda I \).
- Compute its determinant.
- Set the determinant equal to zero to find the eigenvalues.
Here’s a practical example with a 2x2 matrix:
Consider the matrix:
A = [4, 2; 1, 3];
-
The characteristic polynomial is calculated through:
syms lambda; char_poly = det(A - lambda * eye(2));
-
Solving the equation \( \text{det}(A - \lambda I) = 0 \) yields the eigenvalues.
Calculating Eigenvalues
Eigenvalues can be calculated using various methods, including algebraic computations and numerical methods. Determinants play a crucial role in this process. If the characteristic polynomial is derived correctly, the eigenvalues correspond to the roots of this polynomial.
For example, solving the characteristic polynomial for our matrix \( A \) from earlier will yield the eigenvalues.
Calculating Eigenvectors
Once eigenvalues are known, eigenvectors can be readily computed. They are determined by substituting the eigenvalues back into the equation \( (A - \lambda I) v = 0 \). This results in a system of linear equations.
To illustrate, consider the eigenvalue \( \lambda_1 \) from earlier. The code to find its corresponding eigenvector is:
% Assuming lambda1 is calculated
eq = (A - lambda1 * eye(2)); % Adjust the size for nxn matrix
v = null(eq);
This will provide the eigenvector associated with the eigenvalue \( \lambda_1 \).
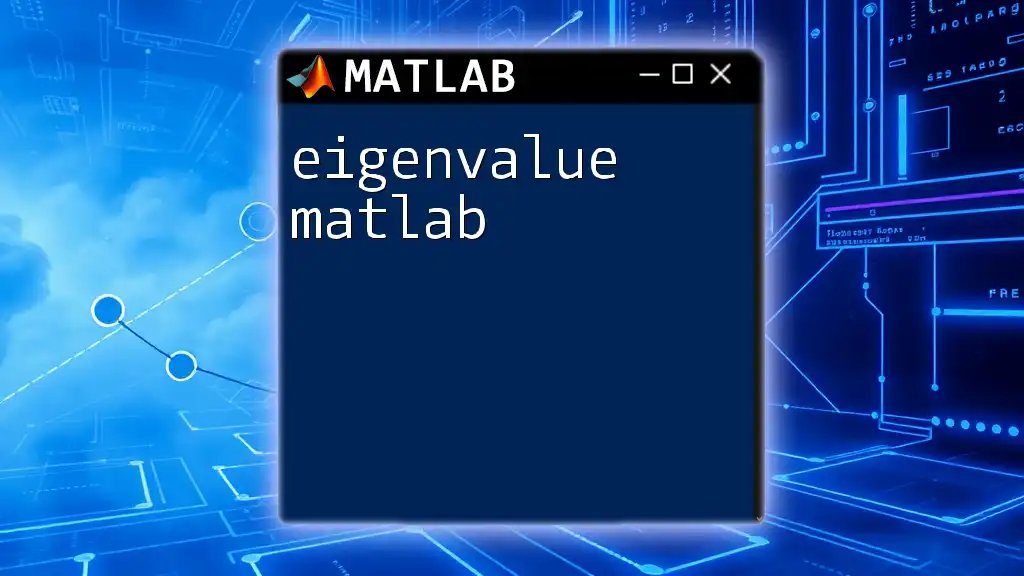
MATLAB Fundamentals for Eigenvalues and Eigenvectors
Setting Up MATLAB
Before diving into calculations, ensure MATLAB is installed and properly set up. Familiarize yourself with the interface, focusing on the Command Window and Editor, as they are essential for executing MATLAB code.
Using MATLAB for Eigenvalue and Eigenvector Computation
The `eig` Function
MATLAB simplifies the process of computing eigenvalues and eigenvectors through its built-in `eig` function. This powerful function computes the eigenvalues in a matrix form and the corresponding eigenvectors directly.
Here is the syntax and an example of using the `eig` function:
A = [4, 2; 1, 3];
[V, D] = eig(A);
In this command, \( V \) contains the eigenvectors, and \( D \) is a diagonal matrix where each diagonal element corresponds to an eigenvalue.
Example: Eigenvalues and Eigenvectors of a Matrix
Let’s execute a practical example to enhance understanding. Consider the 2x2 matrix:
A = [2, -1; 1, 0];
[V, D] = eig(A);
disp('Eigenvalues:');
disp(diag(D)); % Displays the eigenvalues
disp('Eigenvectors:');
disp(V); % Displays the eigenvectors
In this case, running the code will output the eigenvalues and eigenvectors, demonstrating a simple computation that illustrates the relationship between the two.
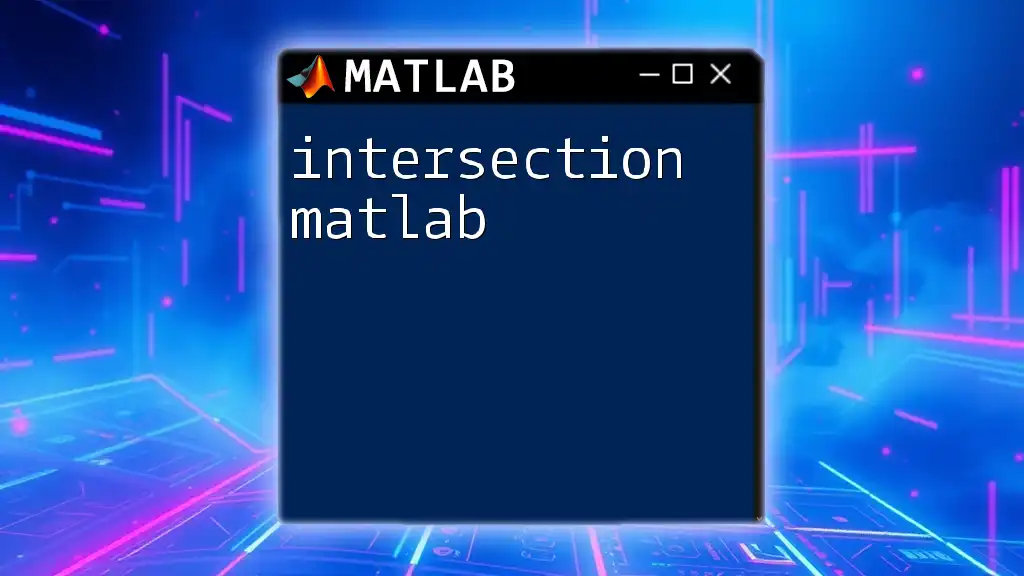
Advanced Applications
Applications in Data Science
Eigenvalues and eigenvectors play a critical role in machine learning, particularly in techniques like Principal Component Analysis (PCA). PCA relies on eigenvalues to determine the "principal components," which are essentially the eigenvectors corresponding to the largest eigenvalues. These components help reduce dimensionality in datasets while preserving variance.
A basic implementation of PCA using the eigenvalue/eigenvector approach in MATLAB can look like this:
% Assuming data is a multidimensional array
C = cov(data); % Compute covariance matrix
[V, D] = eig(C); % Eigen decomposition
% Sorting eigenvalues and eigenvectors
[~, indx] = sort(diag(D), 'descend');
V_sorted = V(:, indx);
This will give you the basis vectors for a lower-dimensional solution while retaining most of the data's variability.
Applications in Control Theory
In control theory, analyzing the stability of systems heavily relies on eigenvalues. The location of eigenvalues in the complex plane indicates system stability: if all eigenvalues have negative real parts, the system is stable. MATLAB can help analyze system dynamics through eigenvalue computation, facilitating the design of more effective control systems.
Applications in Quantum Mechanics
Eigenvalues and eigenvectors also have profound implications in quantum mechanics. They characterize observable properties of quantum systems. The eigenvalues represent possible outcomes of measuring an observable quantity, while eigenvectors represent the states associated with those outcomes.
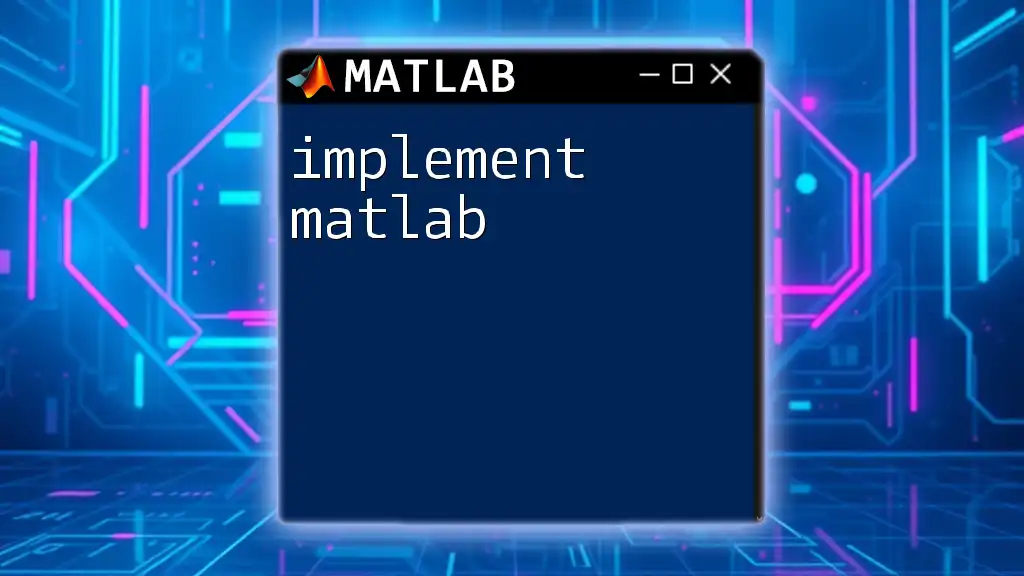
Common Issues and Troubleshooting
Numerical Stability
In computations involving eigenvalues and eigenvectors, numerical stability can be a concern. Always verify results by checking derived eigenvalues and eigenvectors meet the defined equations. Clarity on scaling and context can prevent pitfalls in high-dimensional analyses.
Interpreting Results
Understanding and validating the computed eigenvalues and eigenvectors is crucial. Confusion often arises when interpreting negative values or zero eigenvalues. It’s important to remember that negative eigenvalues indicate direction and stability, while zero eigenvalues may suggest redundancy or invariance in the system.
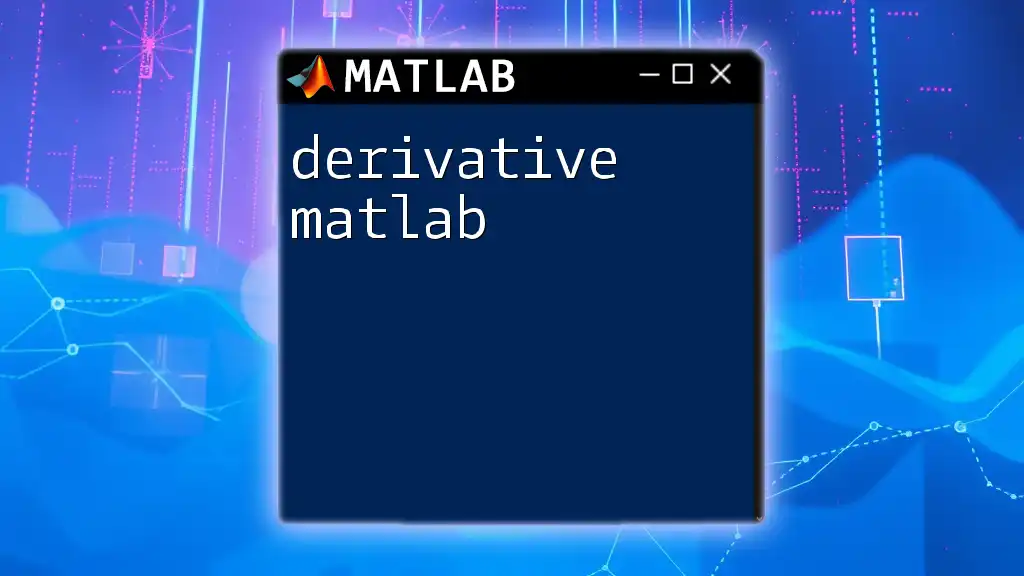
Conclusion
Understanding and utilizing eigenvalues and eigenvectors is foundational in many fields, from data science to control systems. With MATLAB, the computation of these critical mathematical concepts becomes highly efficient and accessible.
Dive into hands-on practice using the provided code snippets, and explore the vast applications of eigenvalue-eigenvector analysis in real-world scenarios. Building a solid foundation in these areas will undoubtedly enrich your analytical capabilities in MATLAB.
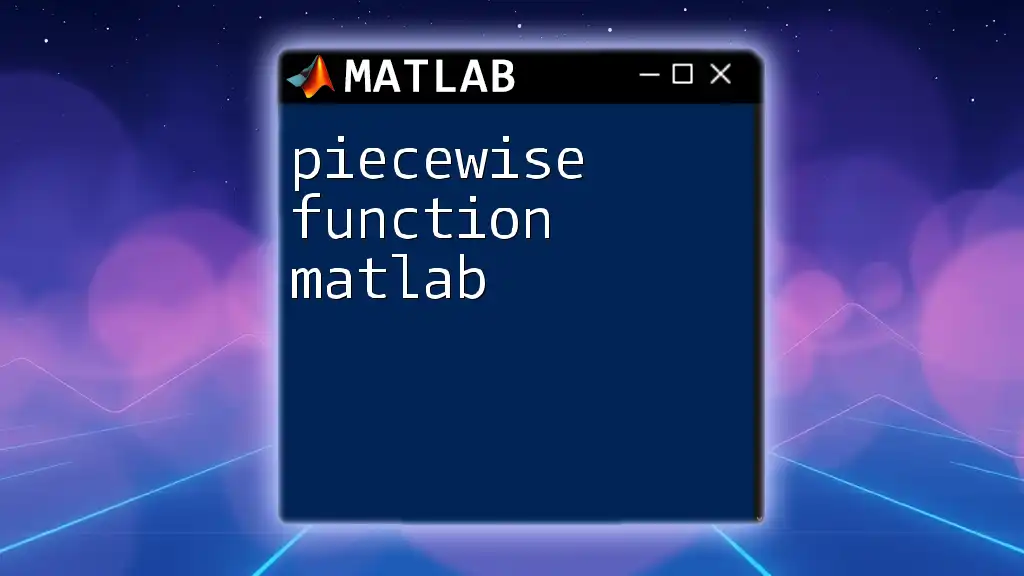
Additional Resources
To further your knowledge, consider exploring recommended readings and online courses that can deepen your understanding of eigenvalues and eigenvectors. The MATLAB documentation is also an invaluable resource for specific functions related to these concepts. Additionally, engaging with forums and communities can provide further assistance and insights from experienced users.