The `round` function in MATLAB rounds the elements of an array to the nearest integers, with ties rounding away from zero.
Here's a code snippet demonstrating its use:
% Example of using the round function in MATLAB
values = [1.2, 2.5, 3.7, -4.5, -5.1];
roundedValues = round(values);
disp(roundedValues); % Output will be: 1 3 4 -5 -5
Understanding the `round` Function
The `round` function in MATLAB is used to round a number to the nearest integer. This function is particularly useful when precise whole numbers are needed from floating-point numbers that might arise during calculations.
The syntax for the `round` function is quite straightforward:
y = round(x)
In this syntax, `x` is the input value, which can be a single number, vector, or matrix, and `y` is the result after rounding.
In addition to the basic usage, the `round` function can also take a second optional argument:
y = round(x, n)
Here, `n` indicates the number of decimal places to which you want to round `x`. This versatility makes the `round` function a powerful tool in MATLAB programming and data analysis.
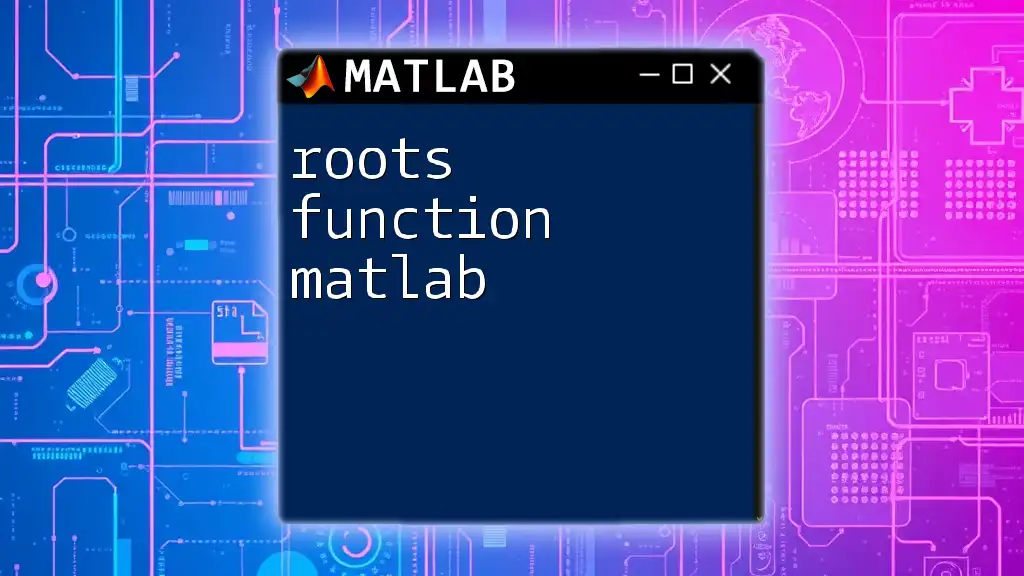
How the `round` Function Works
Basic Rounding
When you use the `round` function without any additional parameters, MATLAB applies standard rounding rules. Specifically:
- If the fractional part of the number is exactly 0.5, MATLAB rounds away from zero.
- If the fractional part is less than 0.5, it rounds down.
- If it is greater than 0.5, it rounds up.
For example:
x = 3.6;
y = round(x); % y will be 4
By rounding `3.6`, we get `4`, demonstrating how the function works with numbers greater than 0.
Rounding to Specific Decimal Places
Using the optional second parameter, you can specify how many decimals you want to include in the rounded output. This is beneficial in cases where accuracy is needed only up to a certain precision level. For instance:
x = 3.6789;
y = round(x, 2); % y will be 3.68
In this example, `3.6789` is rounded to two decimal places, resulting in `3.68`. This flexibility makes it easier to manage numerical data in various applications.
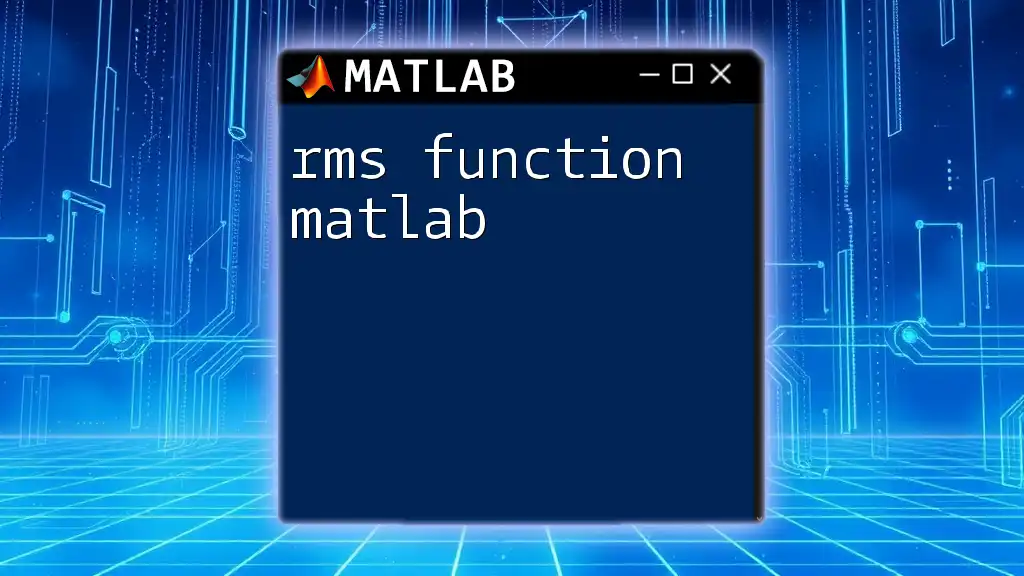
Different Rounding Behaviors
Rounding Positive and Negative Numbers
The `round` function handles positive and negative values differently, which can be an important point to consider in programming. Positive numbers behave as expected, while negative numbers might have unexpected results if not handled correctly.
For example:
pos_num = 2.3;
neg_num = -2.3;
round_pos = round(pos_num); % round_pos will be 2
round_neg = round(neg_num); % round_neg will be -2
In this code, `2.3` rounds to `2`, while `-2.3` rounds to `-2`. This illustrates how the function conforms to standard rounding practices.
Rounding with Halfway Cases
MATLAB's rounding method for halfway cases (like `2.5` or `3.5`) is particularly important. Depending on your application, this could be significant.
For example:
halfway_up = round(2.5); % Outputs 3
halfway_down = round(3.5); % Outputs 4
In these cases, both `2.5` and `3.5` round away from zero, thus demonstrating how MATLAB determines the nearest integer.
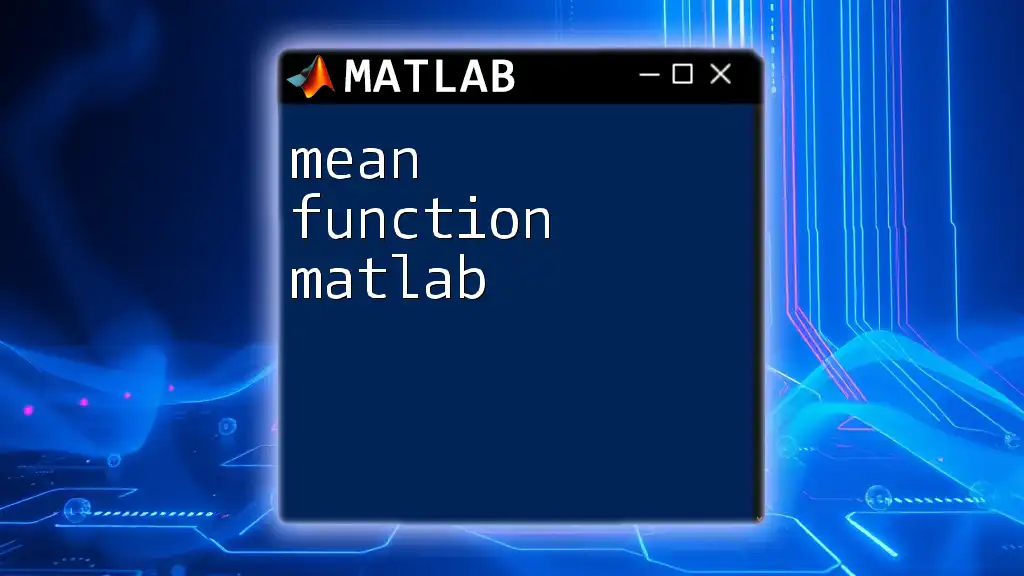
Using the `round` Function with Arrays
Rounding Elements in a Vector
One of the strengths of the `round` function is its ability to operate on arrays and matrices. When applied to a vector, it rounds each element individually, making it particularly useful for dataset adjustments.
Here’s an example:
arr = [1.2, 2.5, 3.7];
rounded_arr = round(arr); % Outputs [1, 3, 4]
The array is rounded element-wise, yielding `[1, 3, 4]`, showcasing how easily the function scales to collections of values.
Rounding in Matrices
The `round` function also works seamlessly with matrices, making it applicable in various scientific and engineering computations.
Consider the following code:
matrix = [1.6, 2.3; 2.7, 3.0];
rounded_matrix = round(matrix); % Outputs [2, 2; 3, 3]
In this instance, all elements in the matrix are rounded, demonstrating the function's versatility and capability of handling multidimensional data structures.
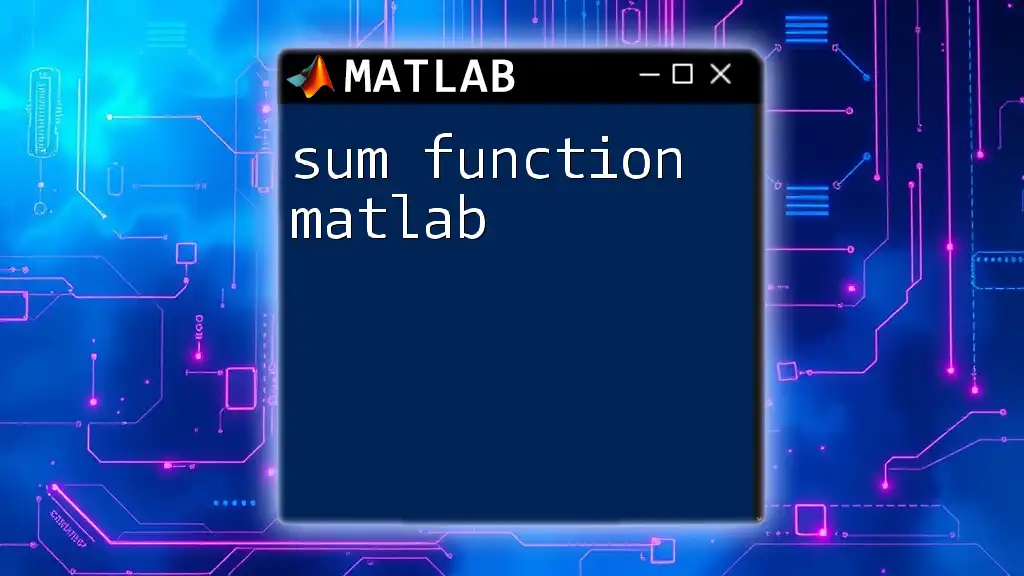
Common Use Cases for the `round` Function
Data Preparation and Cleaning
The `round` function is invaluable when processing datasets. Often, data analytics require rounding to eliminate noise in numerical data or prepare it for visualization. This ensures clean and comprehensible results.
Financial Calculations
In financial scenarios, rounding can mean the difference between a profit and a loss. Costs and revenues usually need to be rounded to two decimal places, which is where the `round` function shines. For instance:
price = 19.99;
rounded_price = round(price, 2); % Outputs 20.00
This not only maintains accuracy but also conforms to typical financial reporting standards.
Scientific Computations
In scientific fields, precision can be critical, especially when working with significant figures. The `round` function helps ensure that values conform to acceptable tolerances for various calculations, such as in physical experiments or simulations.
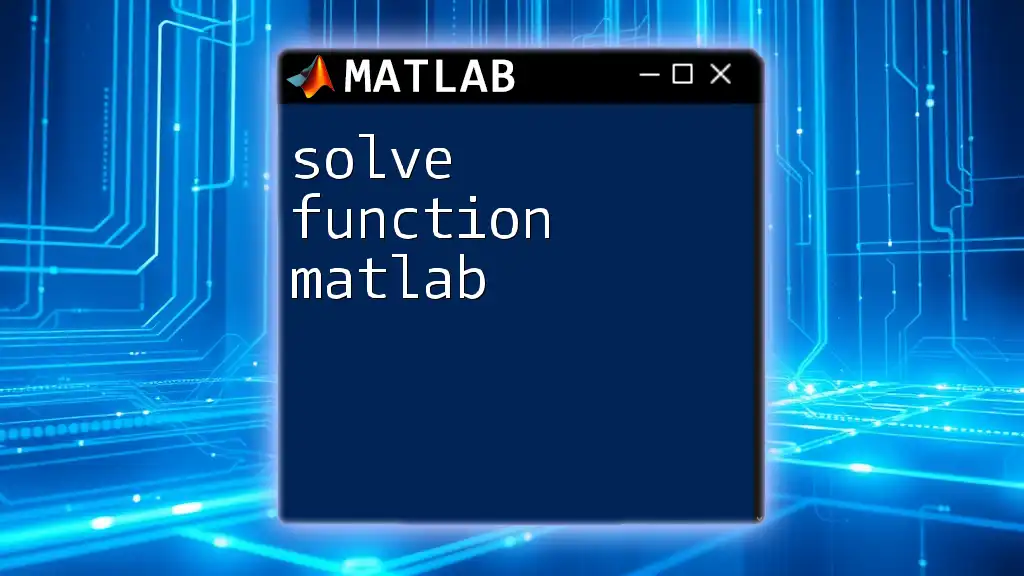
Errors and Troubleshooting
Common Errors with `round`
Although very straightforward, users may face certain errors when using the `round` function, such as forgetting the syntax or misinterpreting the output. If you receive unexpected results, check to make sure your input values meet the expected format and type.
Performance Considerations
While the `round` function is efficient, understanding its performance impact is essential in large datasets. If you are processing extensive arrays, testing your functions for speed and efficiency might be beneficial.
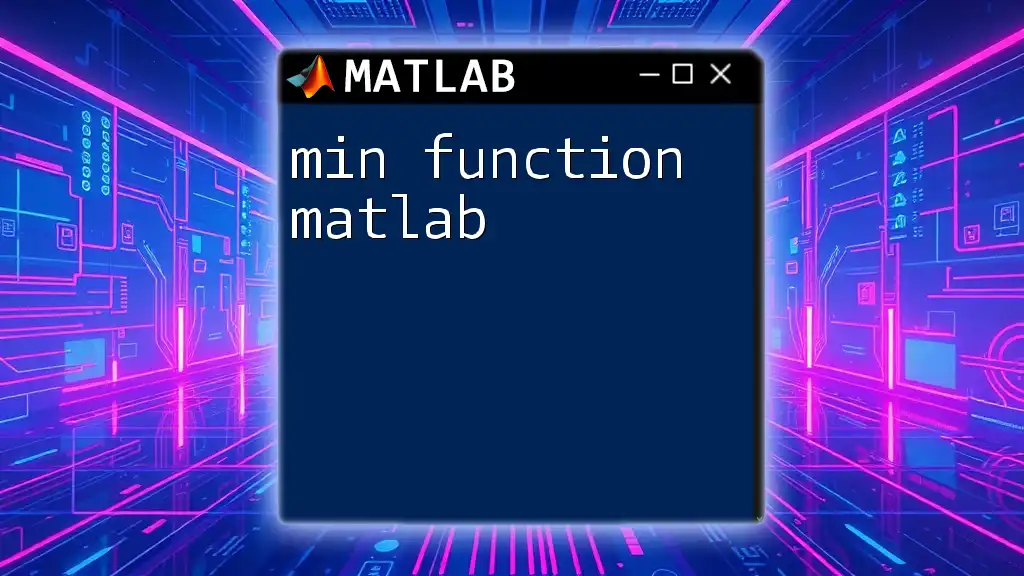
Conclusion
The `round function in MATLAB` is a fundamental tool that simplifies the handling of numerical data, allowing for rounding to the nearest integer or specific decimal places. Whether you are preparing datasets, performing financial calculations, or working in scientific fields, mastering this function is key. Practice applying the `round` function in your projects to become more proficient in MATLAB programming.
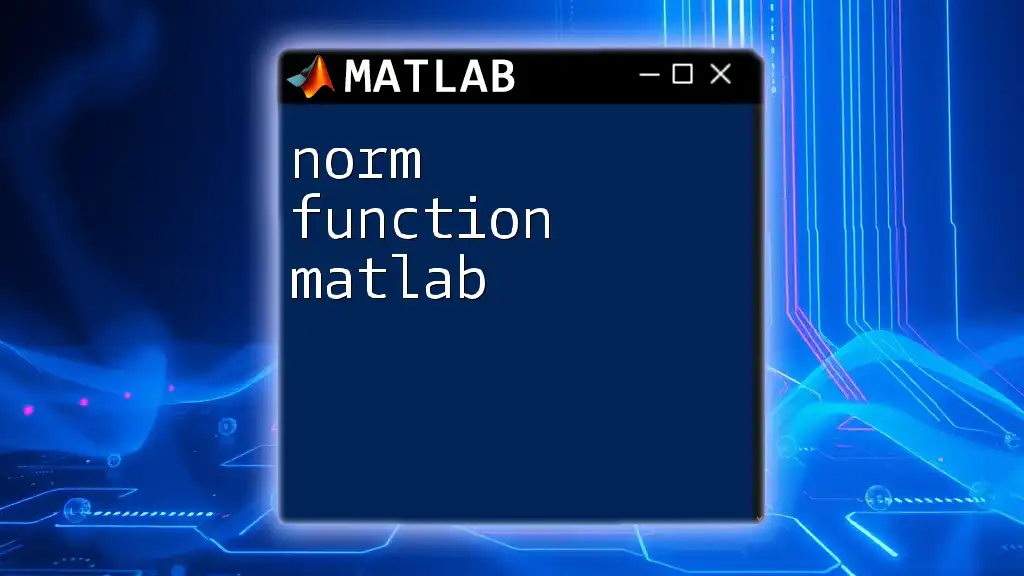
Additional Resources
To deepen your understanding of the `round` function and MATLAB in general, consider exploring the official MATLAB documentation, along with books and tutorial videos that specifically cover numerical computing.
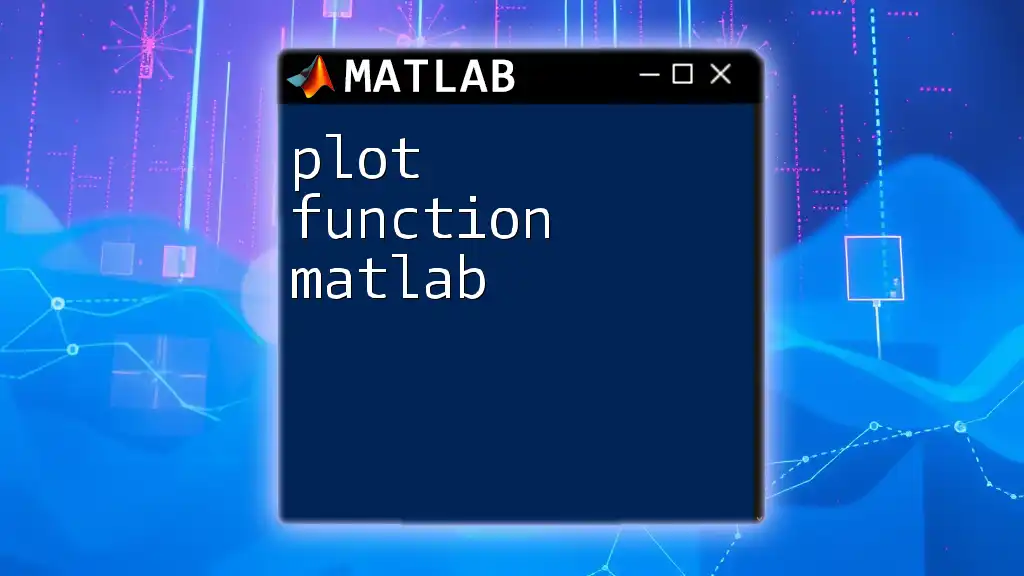
Call to Action
Try out the `round` function today in different contexts, and explore how it can enhance your numerical analyses. Feel free to share your experiences and questions as you navigate through MATLAB!