The bisection method in MATLAB is a simple root-finding algorithm that repeatedly narrows down an interval containing a root of a continuous function by dividing it in half.
Here's a code snippet demonstrating the bisection method in MATLAB:
function root = bisection(f, a, b, tol)
if f(a) * f(b) >= 0
error('f(a) and f(b) must have different signs');
end
while (b - a) / 2 > tol
midpoint = (a + b) / 2;
if f(midpoint) == 0
root = midpoint; % Root found
return;
elseif f(a) * f(midpoint) < 0
b = midpoint; % Update upper bound
else
a = midpoint; % Update lower bound
end
end
root = (a + b) / 2; % Approximate root
end
Understanding the Bisection Method
What is the Bisection Method?
The bisection method is a root-finding technique that continuously divides an interval in half to locate a root of a function. It relies on the Intermediate Value Theorem, which states that if a function is continuous on an interval and takes on different signs at the endpoints, then it must cross the x-axis within that interval. This method is particularly valuable in numerical analysis as it guarantees convergence under the right conditions, making it a reliable choice for approximating roots of nonlinear equations.
How Does the Bisection Method Work?
To implement the bisection method, consider an interval \([a, b]\) such that \(f(a) \cdot f(b) < 0\). The algorithm operates in the following manner:
- Compute the midpoint \(c = \frac{a + b}{2}\).
- Evaluate the function at the midpoint \(f(c)\).
- Determine the subinterval by checking the sign of \(f(c)\):
- If \(f(c) = 0\), then \(c\) is the root.
- If \(f(a) \cdot f(c) < 0\), the root lies within \([a, c]\); hence, set \(b = c\).
- Otherwise, the root lies within \([c, b]\); thus, set \(a = c\).
- Repeat the process until the width of the interval is smaller than a specified tolerance level.
The convergence of the bisection method is linear, which means it takes a predictable number of steps to move closer to the root, making it a robust choice for finding solutions when other methods might fail.
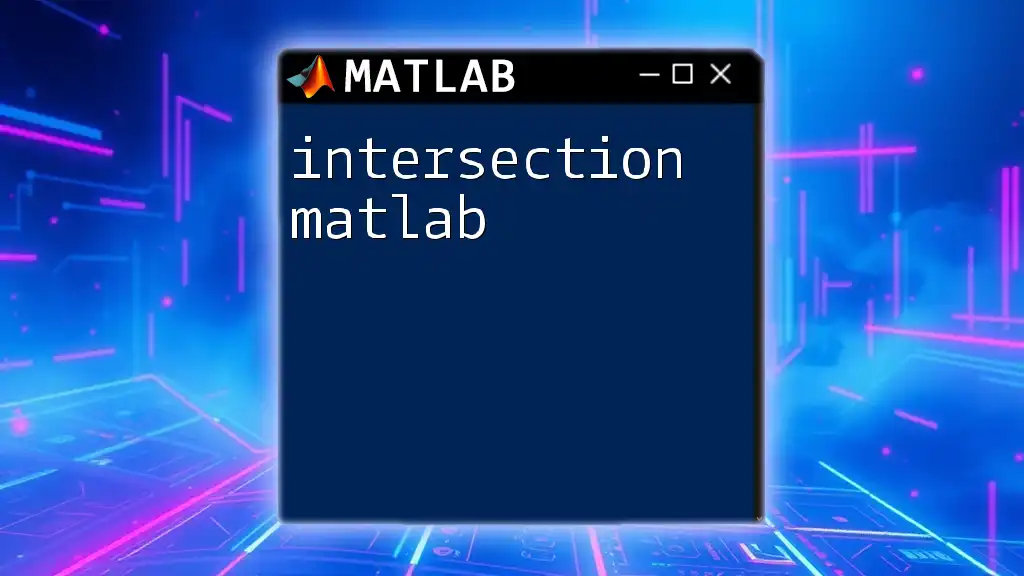
Setting Up MATLAB for Bisection Method
Installing MATLAB
Before diving into the algorithm, ensure you have MATLAB installed on your computer. You can download it from the official MathWorks website. MATLAB offers a 30-day free trial for new users, which is a great way to familiarize yourself with the software before committing to a purchase.
Opening and Using the MATLAB Environment
Once installed, open MATLAB to access its development environment. Familiarize yourself with key components:
- Command Window: This is where you can execute commands and see output instantly.
- Editor: Use this for writing and saving longer scripts and functions.
- Workspace: This shows all the variables currently defined in your session.
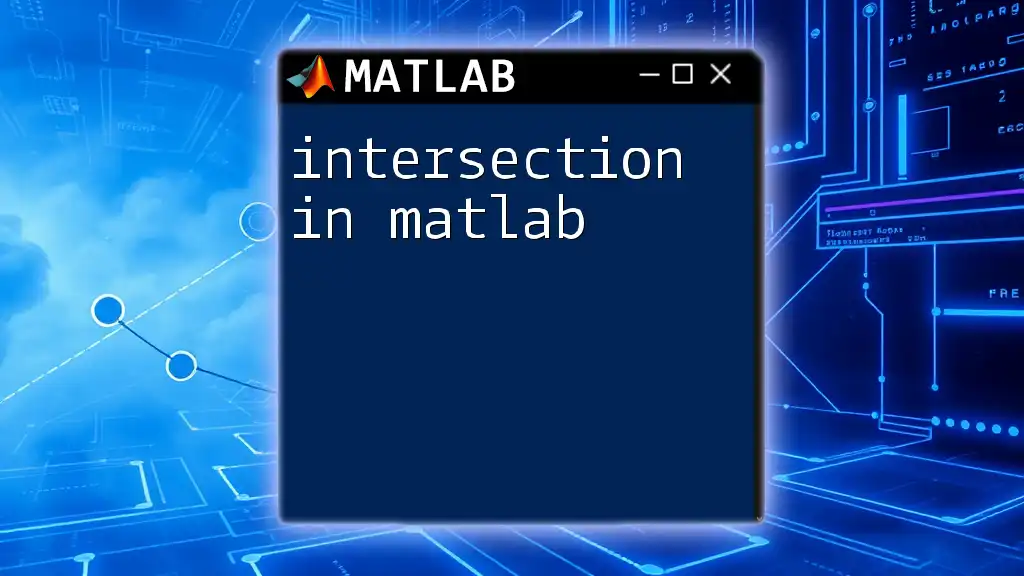
Implementing the Bisection Method in MATLAB
Writing the Bisection Function
You can easily implement the bisection method in MATLAB. Below is a simple function that encapsulates the algorithm:
function [root, iterations] = bisection(func, a, b, tol)
if func(a) * func(b) >= 0
error('Function must have different signs at ends a and b.');
end
iterations = 0;
while (b - a)/2 > tol
c = (a + b)/2; % Midpoint
if func(c) == 0 % c is the root
break;
elseif func(c) * func(a) < 0
b = c; % Root is in the left half
else
a = c; % Root is in the right half
end
iterations = iterations + 1;
end
root = (a + b)/2;
end
This function takes four inputs: the function to evaluate, the lower bound \(a\), the upper bound \(b\), and the tolerance \(tol\) that dictates how precise the root should be.
Example: Finding a Root
Problem Statement
Let's apply the bisection method to find a root of the function \(f(x) = x^2 - 4\). This function has roots at \(x = -2\) and \(x = 2\). We will search for the root in the interval \([0, 3]\).
Applying the Bisection Method
To utilize our `bisection` function, we can do so by executing the following commands:
func = @(x) x^2 - 4; % Define the function
[root, iterations] = bisection(func, 0, 3, 0.01); % Find root
fprintf('Root is: %f found in %d iterations\n', root, iterations);
This snippet will execute the bisection process and print out the root found, along with the number of iterations taken.
Visualizing the Bisection Method
Visual representation can greatly enhance understanding. To visualize the function and the root-finding process, use the following code snippet:
fplot(func, [0, 3]); % Plot the function
hold on;
plot(root, func(root), 'ro'); % Highlight the root point
grid on;
title('Bisection Method Visualization');
xlabel('x');
ylabel('f(x)');
legend('f(x)', 'Root');
hold off;
This code will plot the curve of the function and mark the identified root on the graph, making it easier to see how the bisection method narrows down the solution.
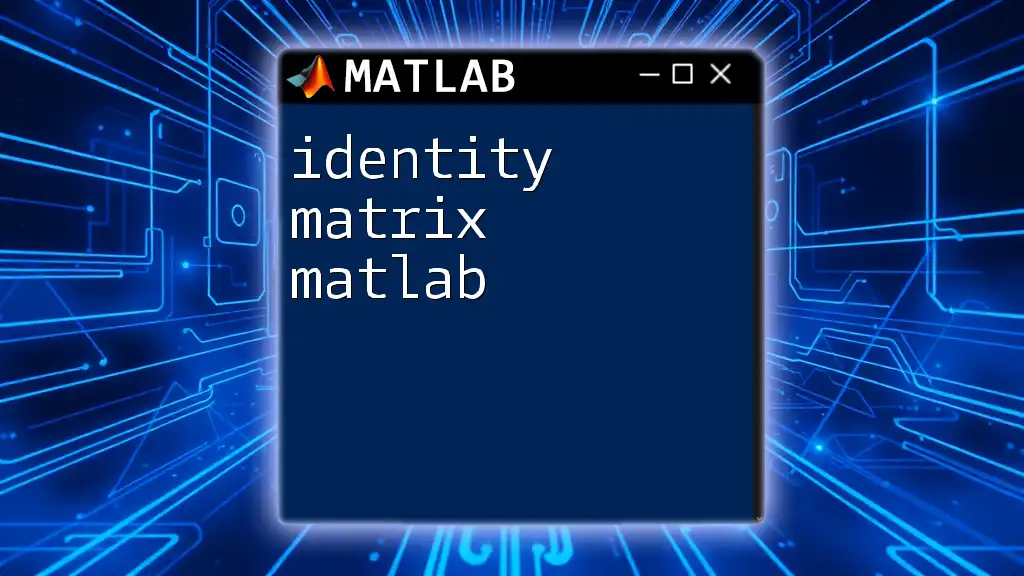
Analyzing Convergence and Error
Understanding Error Tolerance
The tolerance level you set in the bisection method directly affects the accuracy of the root you find. A smaller tolerance results in a more precise root but requires more iterations, possibly increasing computation time. Conversely, a larger tolerance may yield a result faster but at the cost of precision. It is essential to find a balance depending on your specific application.
Convergence Rate of the Bisection Method
The convergence rate of the bisection method is linear, which means each iteration effectively halves the interval containing the root. It is important to note that while this method is guaranteed to converge if the initial interval is selected correctly, it may not be the fastest approach when compared to methods such as Newton-Raphson or the Secant method, which can offer quadratic convergence under appropriate conditions.
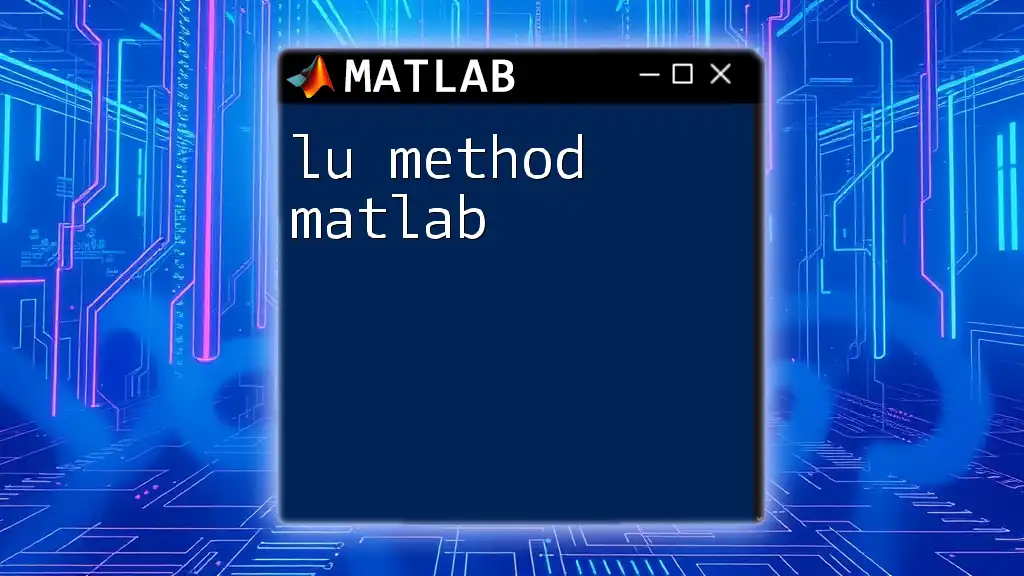
Troubleshooting Common Issues in MATLAB
Handling Function Sign Stability
One common issue when using the bisection method is ensuring that the function values at the endpoints of the interval have opposite signs. If \(f(a) \cdot f(b) \geq 0\), the method cannot proceed. In such cases, re-evaluate your initial guesses \(a\) and \(b\) or adjust your interval. Always ensure the signs differ in the selected interval to apply the bisection method effectively.
Performance Optimization
Although the bisection method is straightforward, there are ways to enhance performance. Avoid using excessively small tolerance levels that lead to unnecessary iterations. An additional way to optimize performance is by vectorizing your functions where possible to take full advantage of MATLAB’s capabilities. Moreover, if the function evaluation is computationally expensive, consider caching the results instead of recalculating them for the same values.
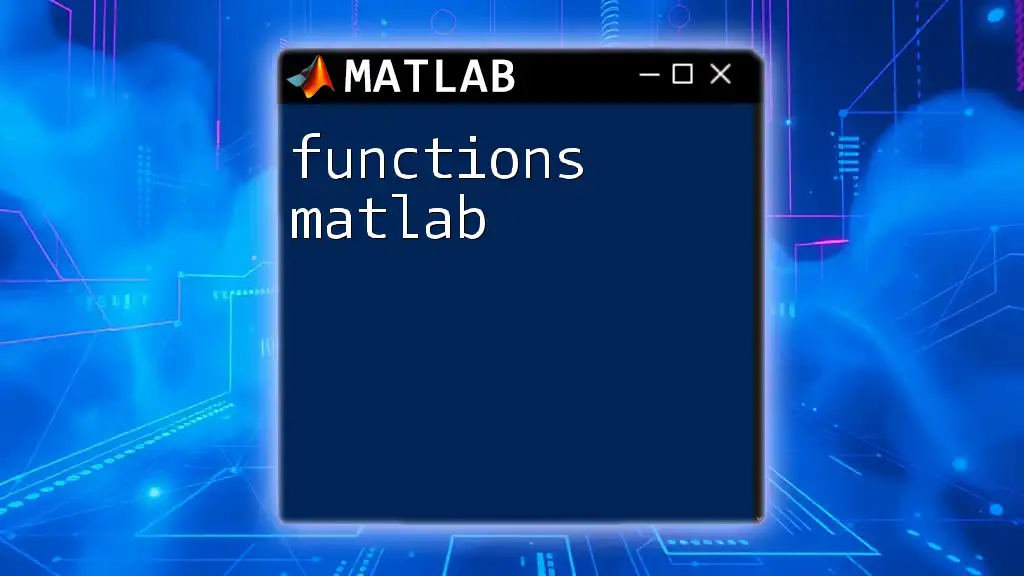
Conclusion
Summary of Key Takeaways
The bisection method is a powerful and reliable numerical technique for root-finding in MATLAB. By correctly implementing the algorithm, you can ensure a systematic approach to approximating roots. Be mindful of the necessary intervals and understand the importance of setting appropriate tolerance levels to balance accuracy and computation time.
Additional Resources
For further reading, consider exploring community forums and MATLAB's own documentation. You might also find valuable insights in textbooks dedicated to numerical analysis, which cover bisection along with a variety of other methods available for root-finding and numerical computations.
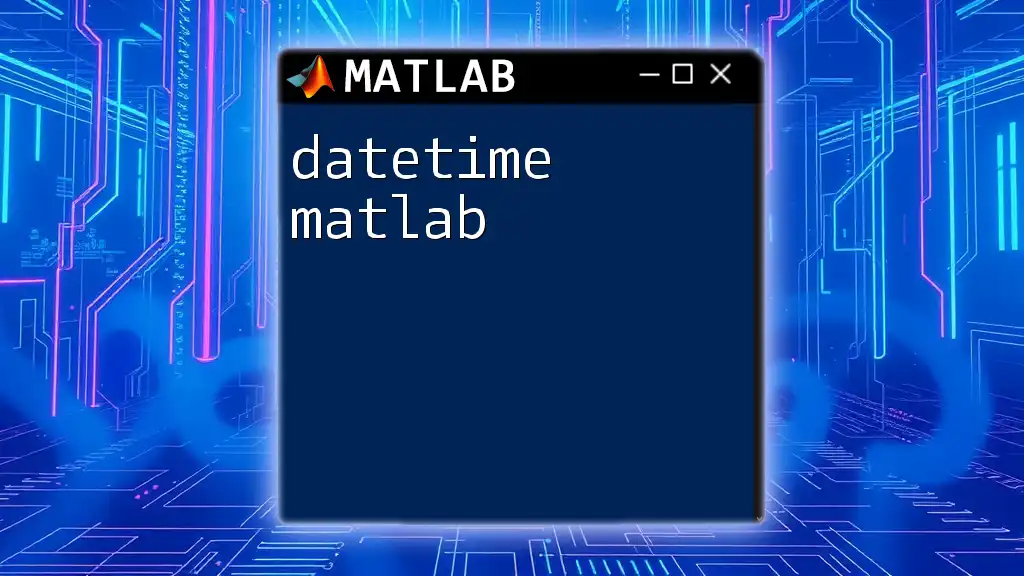
Call to Action
Embrace the power of MATLAB to enhance your numerical analysis skills! Subscribe for more concise tips and tricks, and feel free to share your experiences or questions in the comments section below. Your journey into mastering the bisection method in MATLAB begins now!