To plot a vector in MATLAB, you can simply use the `plot` function to visualize the elements of the vector against their indices.
v = [1, 3, 2, 5, 4]; % Define a vector
plot(v); % Plot the vector
xlabel('Index'); % Label for x-axis
ylabel('Value'); % Label for y-axis
title('Vector Plot'); % Title of the plot
grid on; % Enable grid
Understanding Vectors in MATLAB
Definition of Vectors
In MATLAB, a vector is a one-dimensional array that can hold multiple values in a single variable. Vectors are fundamental in MATLAB because they are used extensively in mathematical computations, data analysis, and plotting. There are two primary types of vectors: row vectors and column vectors.
- Row vectors are horizontal arrays (1 x n dimensions), e.g., `[1 2 3 4 5]`.
- Column vectors are vertical arrays (n x 1 dimensions), e.g., `[1; 2; 3; 4; 5]`.
Creating Vectors
Using the Colon Operator
The colon operator is a simple and efficient way to create row vectors. It uses the syntax `start:step:end`. For example, if you want to create a row vector from 1 to 5, you could write:
row_vector = 1:5; % Result: [1 2 3 4 5]
Using the Linspace Function
The `linspace` function creates vectors by specifying the start value, end value, and the number of points you want. This is particularly useful when you need evenly spaced values. For example:
linspace_vector = linspace(0, 10, 5); % Result: [0 2.5 5 7.5 10]
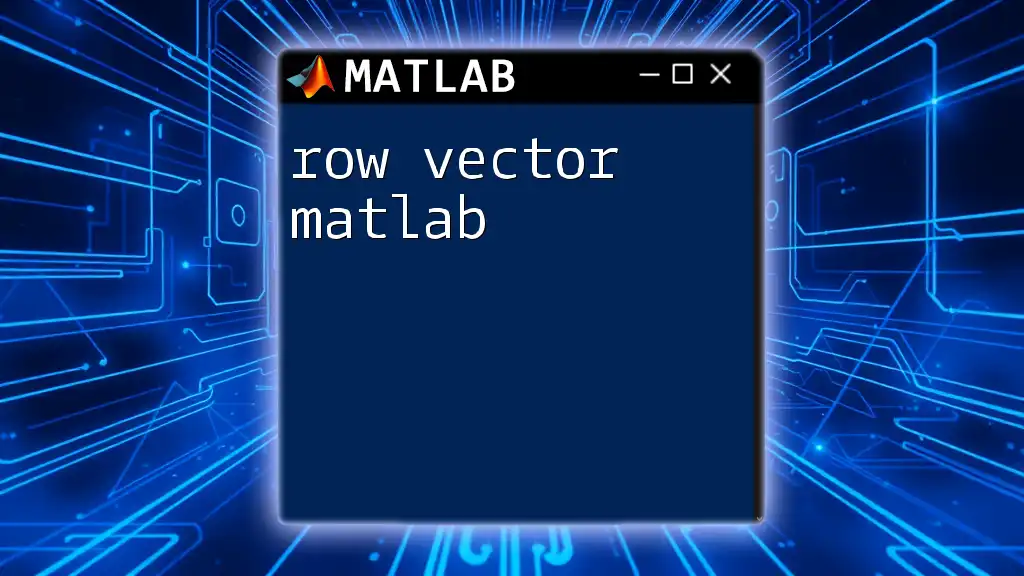
Basic Plotting of Vectors
The Plot Function
One of the most powerful features of MATLAB is its ability to visualize data through plots. The `plot` function is used to create 2D plots of vectors. The basic syntax is:
plot(x, y)
Here, `x` represents the independent variable, and `y` is the dependent variable being plotted against `x`.
Plotting Row Vectors
To plot a row vector, you simply pass it to the `plot` function. For example, creating a row vector of random numbers and plotting it can be done as follows:
x = 1:10; % Create a row vector from 1 to 10
y = rand(1,10); % Create random values corresponding to x
plot(x, y); % Plot the row vectors
title('Plot of Random Values'); % Adding title
xlabel('X-axis'); % X-axis label
ylabel('Y-axis'); % Y-axis label
Plotting Column Vectors
You can also plot column vectors in a similar manner. Here’s how you can create and plot a column vector:
x_col = (1:10)'; % Create a column vector
y_col = rand(10,1); % Random values as a column vector
plot(x_col, y_col); % Plot the column vectors
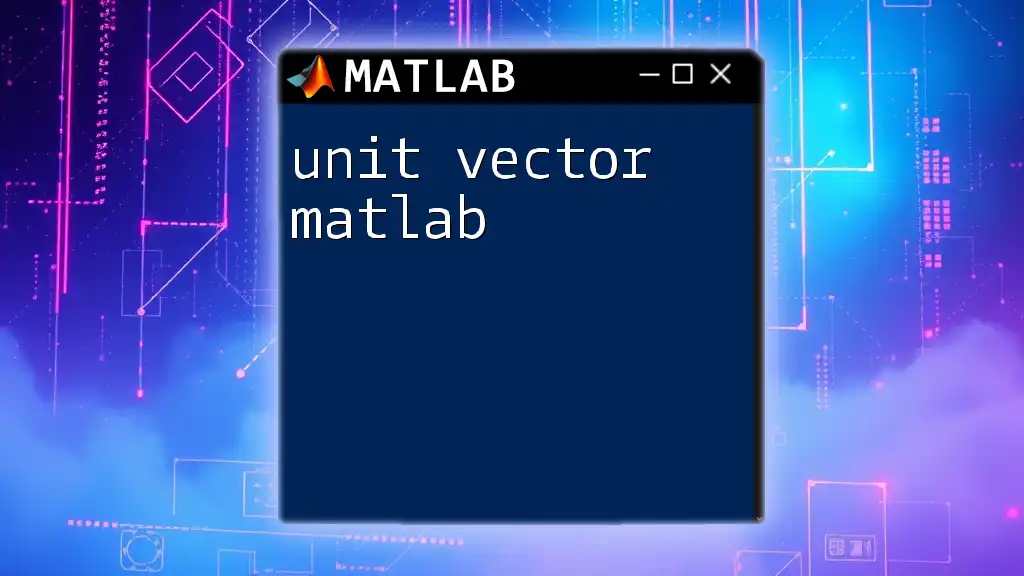
Enhancing Vector Plots
Adding Titles and Labels
Adding titles and labels to your plots is essential for clarity. By annotating your graphs, you help viewers understand what they are looking at. Use `title`, `xlabel`, and `ylabel` functions to do this:
title('Column Vector Plot');
xlabel('Time (s)');
ylabel('Amplitude');
Customizing Line Styles and Colors
MATLAB allows you to customize the appearance of your plots. You can change line styles, colors, and markers. For instance, if you want to create a red dashed line, use:
plot(x, y, 'r--', 'LineWidth', 2); % Red dashed line with a specified width
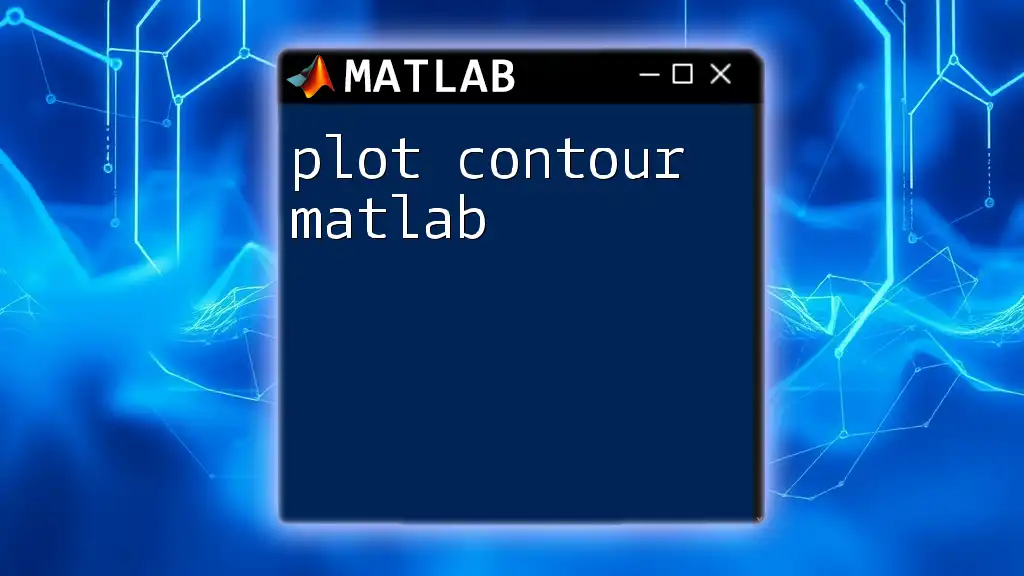
Advanced Plotting Techniques
Multiple Vectors on One Plot
If you want to compare multiple vectors visually, you can plot them using the `hold on` command. This command allows multiple datasets to be plotted on top of each other in the same figure:
hold on; % Hold the current plot
plot(x, y1, 'b-'); % Plot first vector as blue solid line
plot(x, y2, 'g:'); % Plot second vector as green dotted line
hold off; % Release the current plot
Subplots
When dealing with multiple plots, subplots can be a great way to organize them neatly within the same figure, especially when comparing related datasets. You can create subplots in a grid format:
subplot(2,1,1); % First subplot in a 2-row layout
plot(x, y1); % Plotting first vector
subplot(2,1,2); % Second subplot
plot(x, y2); % Plotting second vector
Customizing Axes
Customizing the axes is crucial for making your plots more informative. You can set axis limits and add grid lines to enhance readability:
xlim([0 10]); % Set x-axis limits
ylim([0 1]); % Set y-axis limits
grid on; % Add grid lines for better visibility
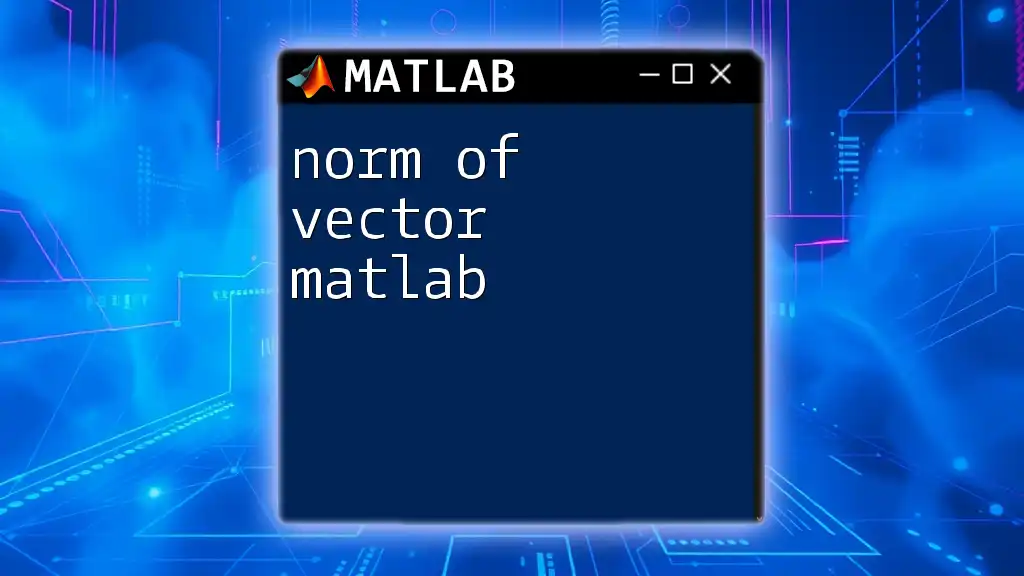
Troubleshooting Common Issues
Error Messages Related to Plotting
Frequently encountered error messages while plotting often stem from a mismatch in vector dimensions. For instance, if you attempt to plot vectors `x` and `y` of different lengths, MATLAB will alert you with an error. Always ensure that your vectors are of compatible sizes before plotting.
Tips for Effective Plotting
To improve your plotting capabilities, consider the following best practices:
- Keep your visuals simple and uncluttered.
- Check for consistent vector sizes, especially when plotting multiple datasets.
- Use clear labels and legends to help convey your data's meaning.
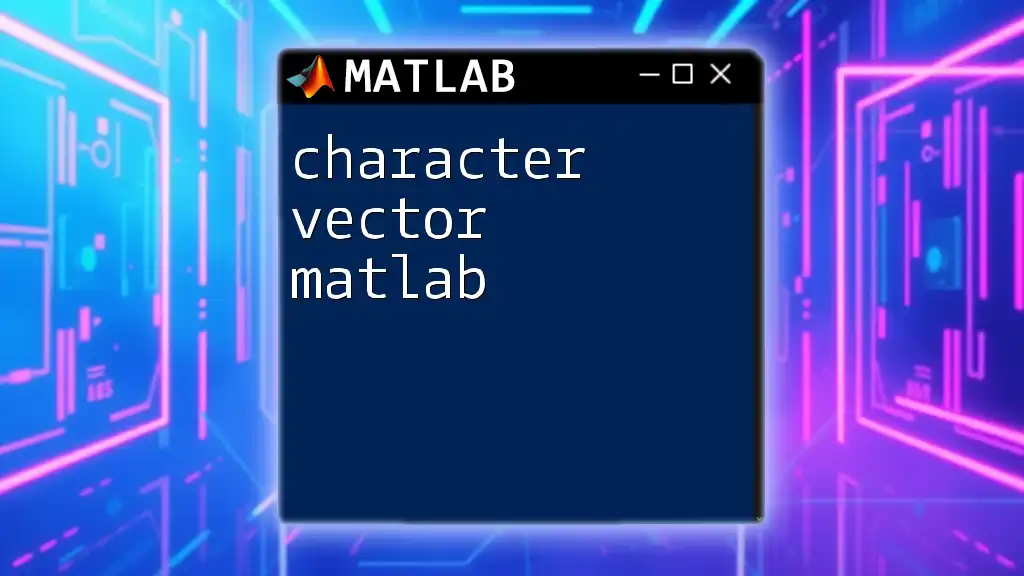
Conclusion
This guide has provided a detailed overview of how to effectively plot vectors in MATLAB. From creating vectors to customizing your plots, you should now feel more confident in harnessing the power of MATLAB for data visualization. Remember, practice is key; don’t hesitate to experiment with different datasets and plotting techniques to enhance your understanding.
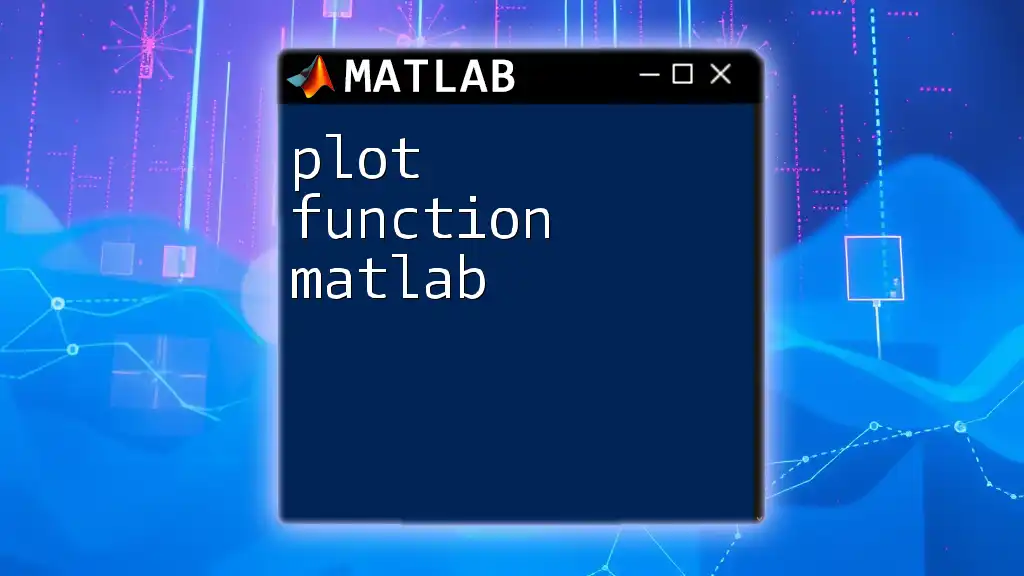
Additional Resources
For further exploration, refer to the official MATLAB documentation, which offers extensive resources on vector manipulation and plotting functions. Consider joining community forums where you can engage with other MATLAB users for collaborative learning and troubleshooting assistance.