In Python, the equivalent of MATLAB's `interp3d` for 3D interpolation can be achieved using the `scipy.interpolate.interp3d` function.
Here's a code snippet illustrating its usage:
import numpy as np
from scipy.interpolate import RegularGridInterpolator
# Define the grid points
x = np.array([0, 1, 2])
y = np.array([0, 1, 2])
z = np.array([0, 1, 2])
# Define the function values on the grid
values = np.array([[[0, 1, 2], [3, 4, 5], [6, 7, 8]],
[[9, 10, 11], [12, 13, 14], [15, 16, 17]],
[[18, 19, 20], [21, 22, 23], [24, 25, 26]]])
# Create the interpolating function
interp_func = RegularGridInterpolator((x, y, z), values)
# Interpolate at a given point
point = [0.5, 0.5, 0.5]
result = interp_func(point)
print(result)
Understanding Interpolation
What is Interpolation?
Interpolation is a mathematical technique used to estimate unknown values that fall between known data points. It is essential in various fields, especially in engineering, data analysis, and scientific computing. The process involves constructing new data points within the range of a discrete set of known data points, allowing for smooth transitions and predictions in datasets.
Why Use Interpolation?
Interpolation plays a vital role in many applications, such as creating smoother curves from discrete data points, filling in missing data, and optimally rendering visual graphics. For example, in computer graphics, interpolation is used to generate realistic images by estimating pixel colors based on surrounding pixel values. In simulation studies, it is often necessary to interpolate values to forecast behaviors of dynamic systems.
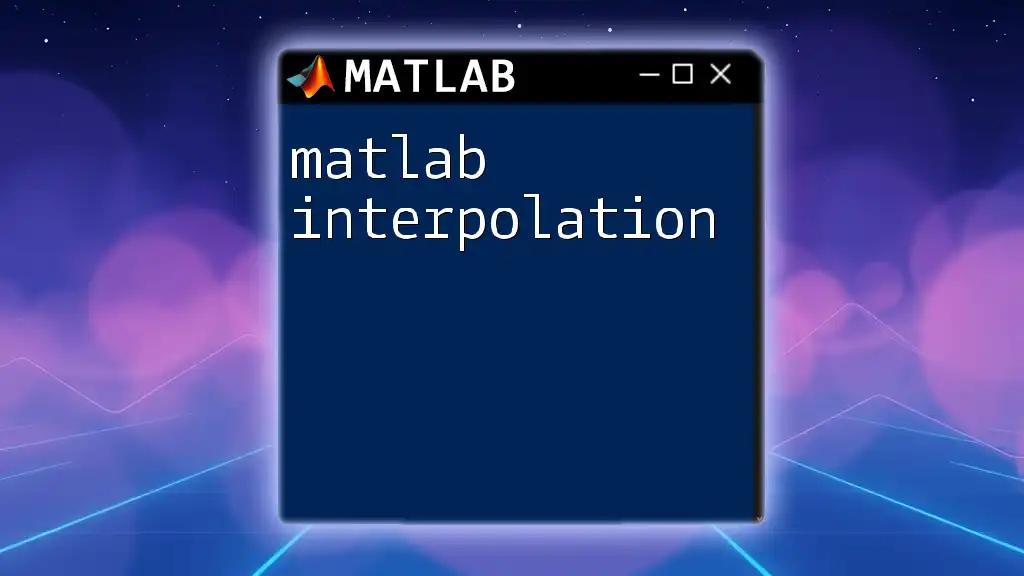
MATLAB's interp3d Function
Overview of interp3d
In MATLAB, the `interp3d` function is designed for performing three-dimensional interpolation. It takes a grid of values and allows users to query interpolated results at specific points. The function can handle various types of grid arrangements, making it versatile for a range of applications in 3D spatial data analysis.
Example of interp3d in MATLAB
Consider the following MATLAB code that demonstrates the use of `interp3d`:
% Example data
[X,Y,Z] = meshgrid(1:10, 1:10, 1:10);
V = X.^2 + Y.^2 + Z.^2;
% Interpolation query points
xi = 5.5; yi = 5.5; zi = 5.5;
% Using interp3d
Vq = interp3(X, Y, Z, V, xi, yi, zi);
disp(Vq);
In this example, we create a meshgrid of points and compute a scalar field `V` which is a function of those points. When we query at the point `(5.5, 5.5, 5.5)`, `interp3d` returns the interpolated value within the defined volume. The output of this operation illustrates how the interpolation smoothly estimates values between the defined points, providing insights into the field.
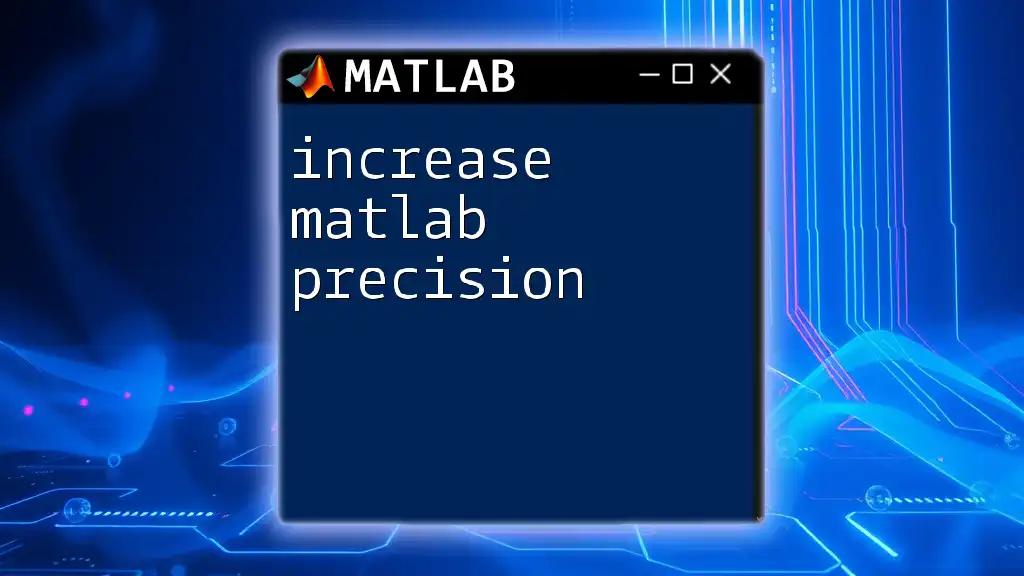
Python's Equivalent for interp3d
Introduction to SciPy
When it comes to finding an equivalent of MATLAB's `interp3d` in Python, the SciPy library is the go-to solution. SciPy offers a comprehensive collection of numerical algorithms, including several functions for multidimensional interpolation, making it suitable for scientific computing tasks.
The `griddata` Function
Overview
The `griddata` function from the `scipy.interpolate` module is a core tool for performing 3D interpolation in Python. This function can take irregularly spaced data and interpolate it onto a grid, offering functionality similar to MATLAB's `interp3d`.
Example of griddata in Python
Here's a Python code snippet that mirrors the functionalities of MATLAB's `interp3d`:
import numpy as np
from scipy.interpolate import griddata
# Example data
x = np.linspace(0, 10, 10)
y = np.linspace(0, 10, 10)
z = np.linspace(0, 10, 10)
X, Y, Z = np.meshgrid(x, y, z)
V = X**2 + Y**2 + Z**2
# Query points
query_points = np.array([[5.5, 5.5, 5.5]])
# Using griddata for interpolation
Vq = griddata((X.flatten(), Y.flatten(), Z.flatten()), V.flatten(), query_points, method='linear')
print(Vq)
In this Python example, we generate a meshgrid and calculate a scalar field similar to the MATLAB code. By querying the point `(5.5, 5.5, 5.5)`, `griddata` provides the interpolated value, effectively matching the behavior of `interp3d`.
Using Other Interpolation Functions in SciPy
LinearNDInterpolator
If your data points are unstructured, the `LinearNDInterpolator` is an excellent alternative. This class allows you to perform linear interpolation on arbitrarily sampled data.
Example usage:
from scipy.interpolate import LinearNDInterpolator
# Create a linear interpolator
interp = LinearNDInterpolator(np.array([X.flatten(), Y.flatten(), Z.flatten()]).T, V.flatten())
# Query interpolation
Vq_lin = interp(5.5, 5.5, 5.5)
print(Vq_lin)
This snippet demonstrates how to create a linear interpolator and query the interpolated value at a specific point. The process is intuitive and provides a smooth transition based on the surrounding data.
RegularGridInterpolator
For regularly spaced grid data, `RegularGridInterpolator` is the ideal choice. It efficiently performs interpolation by taking advantage of the grid structure.
Example usage:
from scipy.interpolate import RegularGridInterpolator
# Create a regular grid interpolator
interp_reg = RegularGridInterpolator((x, y, z), V)
# Query value
Vq_reg = interp_reg((5.5, 5.5, 5.5))
print(Vq_reg)
Using `RegularGridInterpolator`, you can quickly perform interpolations, maintaining high performance for large datasets.

Conclusion
In summary, the equivalent of MATLAB's `interp3d` in Python can be comfortably achieved using the SciPy library, specifically with functions like `griddata`, `LinearNDInterpolator`, and `RegularGridInterpolator`. Each method has its strengths and is suited for different types of data and applications, enabling seamless interpolation in three dimensions. Both MATLAB and Python offer powerful tools to facilitate effective and efficient visualizations and data analyses, making them both essential skills for professionals and researchers alike.
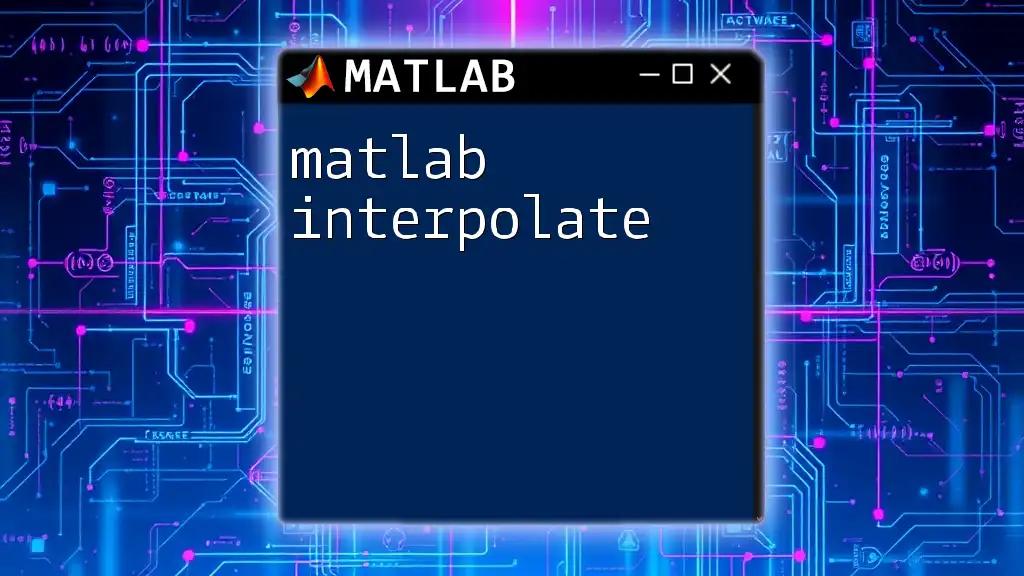
Additional Resources
To further enhance your understanding of interpolation techniques, you can refer to the following documentation:
- [MATLAB Interpolation Documentation](https://www.mathworks.com/help/matlab/ref/interp3.html)
- [SciPy Interpolation Documentation](https://docs.scipy.org/doc/scipy/reference/interpolate.html)
- Explore further readings on advanced interpolation techniques and their application in various fields.
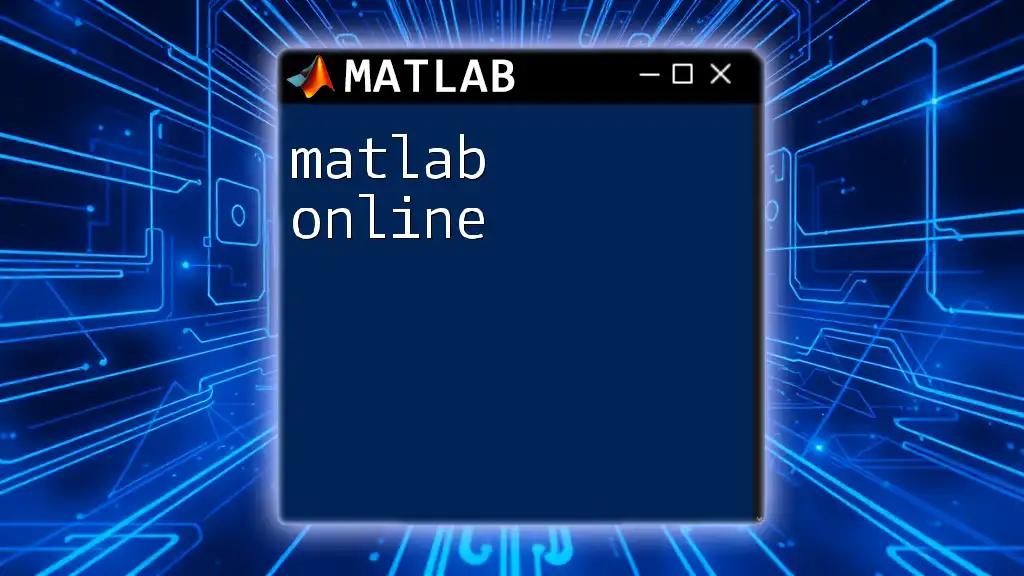
FAQs
What are the performance differences between MATLAB and Python for interpolation?
While MATLAB is highly optimized for matrix operations and can offer faster execution times in some cases, Python's flexibility and extensive libraries allow for broader applications. Performance may vary depending on the specific dataset and task complexity.
Can interpolation be performed in higher dimensions?
Yes, interpolation can be extended to higher dimensions. Both MATLAB and Python's SciPy provide functions that can manage multidimensional data, allowing you to handle complex datasets beyond three dimensions.
Is there an alternative to `griddata` in Python?
Yes, there are other libraries, such as `numpy` and `pandas`, that can perform interpolation, but for specialized multidimensional tasks, SciPy remains the most robust option.
With these insights and practical examples, you are now better equipped to utilize interpolation techniques across both MATLAB and Python, enhancing your capabilities in data analysis and visualization.