The `norm` function in MATLAB computes the norm (or length) of a vector or matrix, providing useful measures such as Euclidean distance or the Frobenius norm.
Here’s an example of how to use the `norm` function in MATLAB:
% Calculate the Euclidean norm of a vector
v = [3, 4];
vectorNorm = norm(v); % The result is 5
What is a Norm?
Definition of Norm
In mathematics, a norm is a function that assigns a strictly positive length or size to vectors in a vector space, with the exception of the zero vector, which is assigned a length of zero. Norms provide a way to measure how "far" a point is from the origin in space, enabling various applications, especially in linear algebra and optimization.
Types of Norms
There are a variety of norms utilized in mathematics, but some of the most commonly used types include:
- L1 Norm (Manhattan Norm): The sum of the absolute values of the components.
- L2 Norm (Euclidean Norm): The square root of the sum of the squares of the components.
- Infinity Norm (Maximum Norm): The maximum absolute value among the components.
Different norms can be more suitable for various applications depending on the problem at hand. For example, the L2 norm is often used in machine learning and error minimization, while the L1 norm can be preferred when dealing with sparse data.
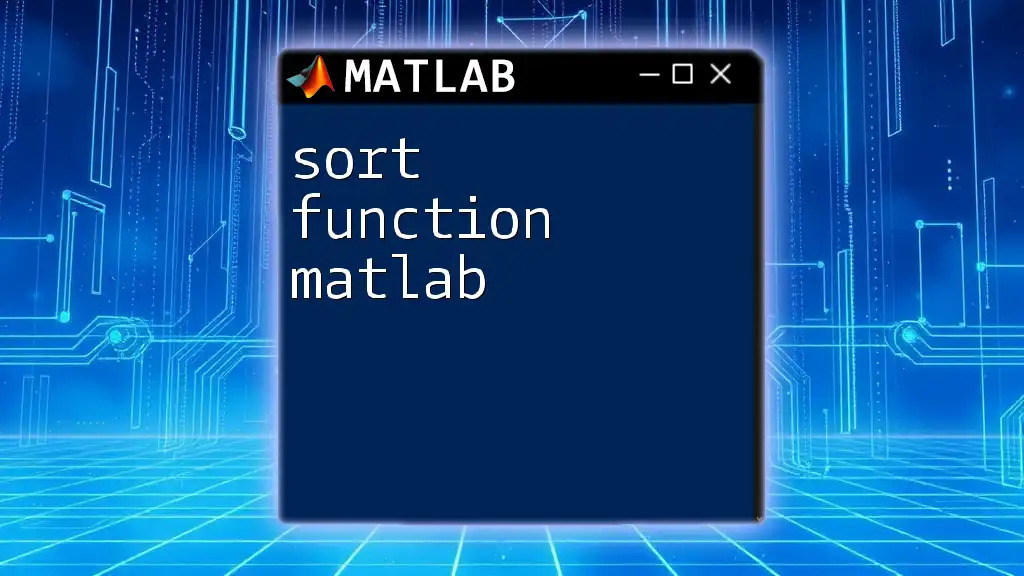
Understanding the `norm` Function in MATLAB
Syntax
The syntax for the `norm` function in MATLAB is:
N = norm(A, p)
Where:
- A is the input vector or matrix.
- p is the order of the norm you wish to calculate. If unspecified, MATLAB defaults to L2 (Euclidean) norm.
Parameters of the `norm` Function
- A: This can either be a vector or a matrix. For matrices, different norms will interpret the data in various forms—either computing the norm for columns or for the entire matrix.
- p: The parameter specifies which norm to calculate. Common values for this parameter include:
- `1` for L1 norm
- `2` for L2 norm (default)
- `'inf'` for infinity norm
- `'fro'` for Frobenius norm (used for matrices)
Return Value
The `norm` function returns a scalar value representing the computed norm based on the input provided. This value essentially gives insights into the length or size of a vector or the magnitude of a matrix.
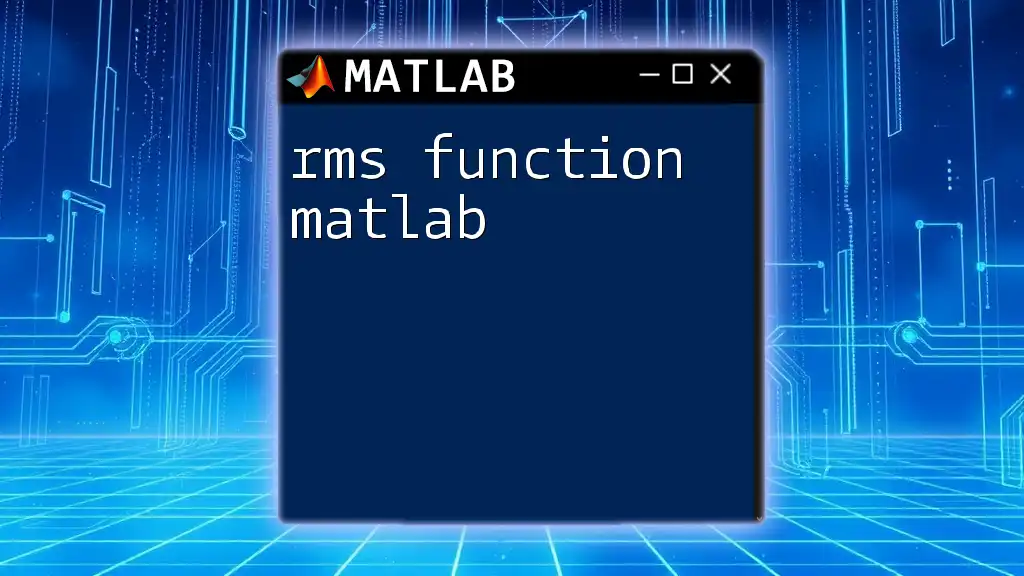
How to Use the `norm` Function
Calculating the L2 Norm (Euclidean Norm)
The L2 norm is one of the most commonly used norms. It is calculated as:
A = [3; 4];
l2_norm = norm(A);
disp(l2_norm);
Output: This calculation results in `5`, because the L2 norm can be visualized as the distance from the origin (0,0) to the point (3,4) in a 2D space.
Calculating the 1 Norm
To compute the L1 norm, you can specify `1` as the second argument:
B = [1; -2; 3];
l1_norm = norm(B, 1);
disp(l1_norm);
Output: Here, the output will be `6`, which corresponds to `|1| + |-2| + |3|`.
Calculating the Infinity Norm
The infinity norm can be calculated as follows:
C = [1, -2, 3];
inf_norm = norm(C, inf);
disp(inf_norm);
Output: The result will be `3`, as it is the maximum absolute value among the components.
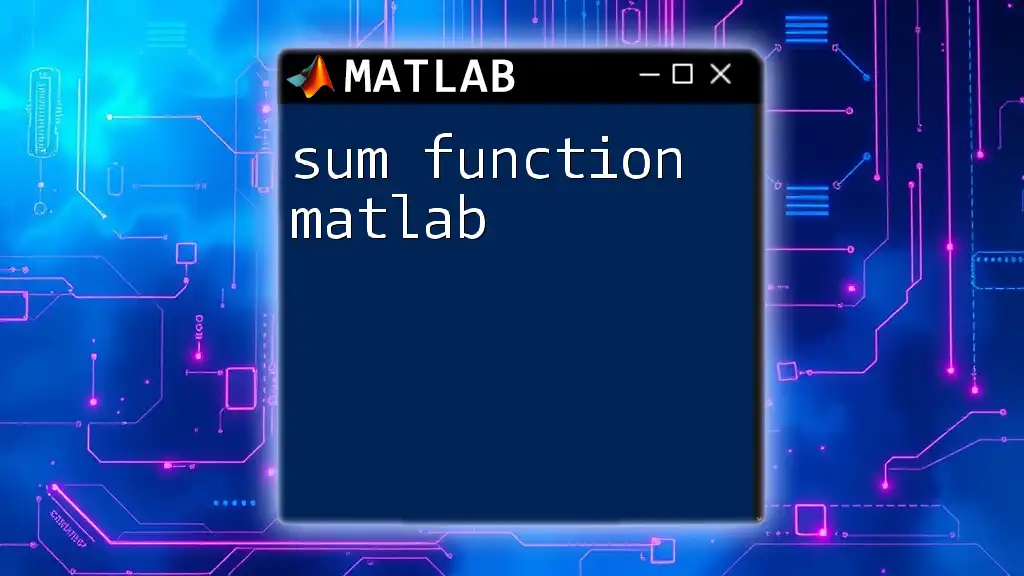
Practical Examples of the `norm` Function
Example 1: Using the Norm in Linear Algebra
Norms are particularly useful in linear algebra for measuring distances. For instance, if you want to calculate the distance between two points represented as vectors, you can do it as follows:
A = [1, 2];
B = [4, 6];
distance = norm(B - A);
disp(distance);
Output: This operation calculates the distance between the two points, resulting in approximately `4.2426`, which is the Euclidean distance between them.
Example 2: Normalizing Vectors
Normalizing a vector involves dividing each component by the vector's norm, converting it into a unit vector. Here’s how you can normalize a vector:
D = [3; 4];
normalized_vector = D / norm(D);
disp(normalized_vector);
Output: The output will yield a unit vector, approximately `[0.6; 0.8]`. Normalization is crucial, especially in machine learning, as it ensures that each feature contributes equally to the model.
Example 3: Working with Matrices
The `norm` function can also operate on matrices. A popular choice is the Frobenius norm, which is defined as the square root of the sum of the absolute squares of its elements:
E = [1, 2; 3, 4];
matrix_norm = norm(E, 'fro');
disp(matrix_norm);
Output: This returns `5.4772`, reflecting the overall "size" of the matrix in a multi-dimensional space.
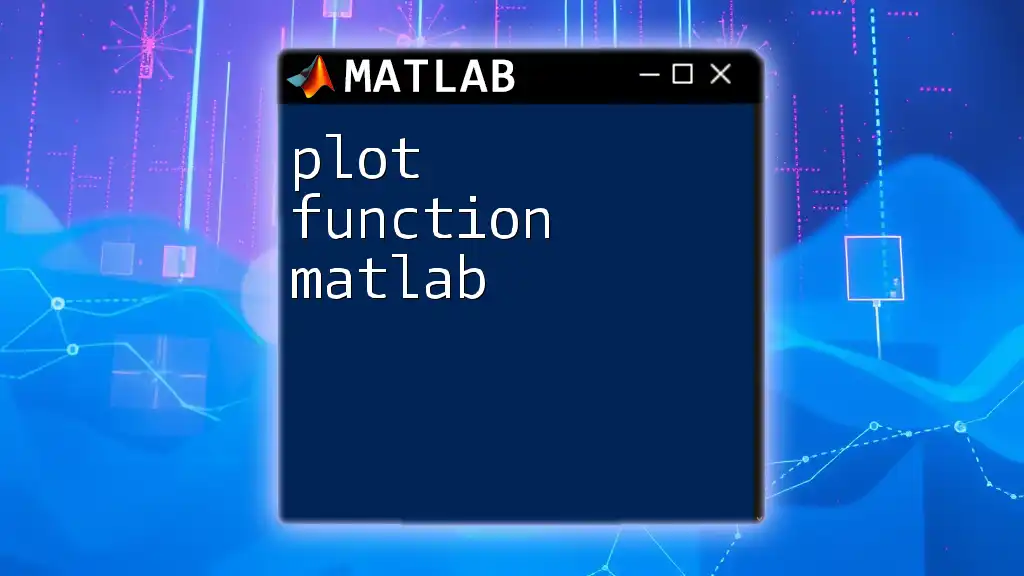
Common Errors and Troubleshooting
Error Handling
When utilizing the `norm` function in MATLAB, some common errors may arise, such as:
- Matrix Size Issues: Ensure that the dimensions of your vectors or matrices are compatible.
- Invalid Norm Order: If you input an unsupported value for the norm order, MATLAB will return an error. Always check the p parameter.
- Non-numeric Data: Ensure the input argument is numeric; otherwise, an error will occur.
If you encounter problems, carefully read error messages and review your code for potential mismatch in dimensions or unsupported operations.
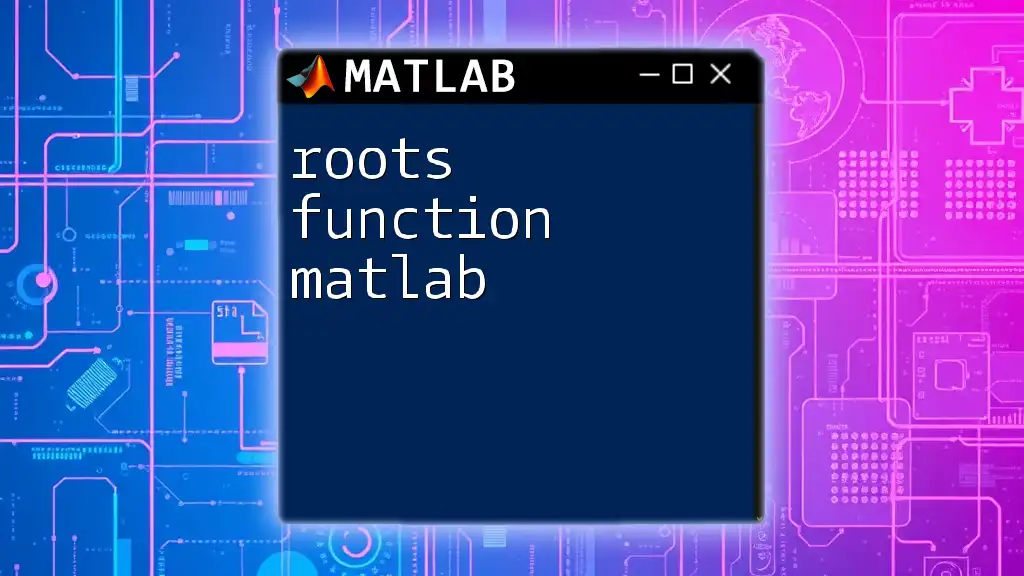
Conclusion
In this article, we've covered the essential aspects of the norm function in MATLAB, illustrating how it can be a powerful tool for various mathematical tasks, especially in linear algebra, data analysis, and machine learning applications. We encourage you to experiment with different types of norms and calculations to deepen your understanding.
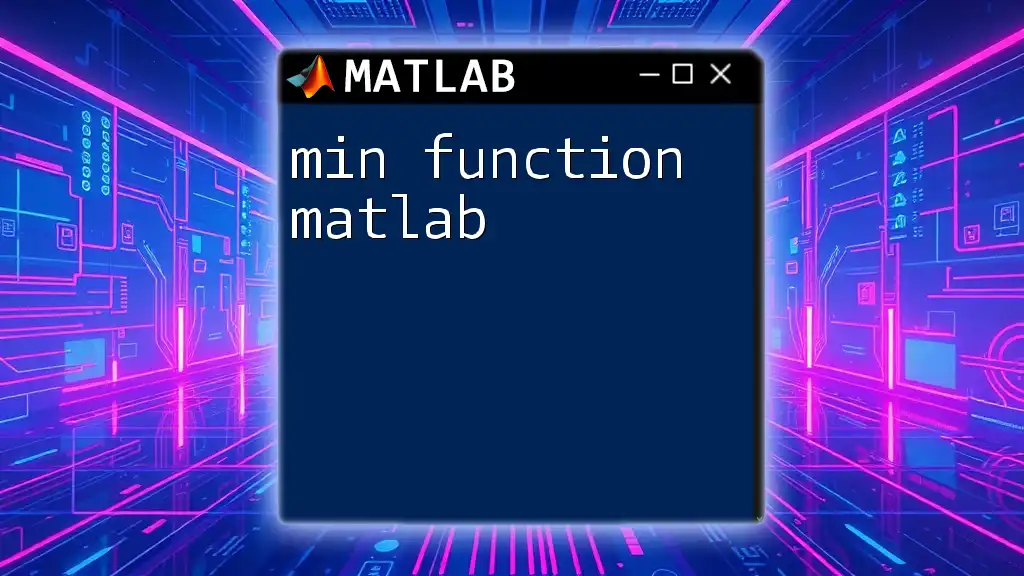
Further Resources
Official MATLAB Documentation
For more detailed information, refer to the [official MATLAB documentation](https://www.mathworks.com/help/matlab/ref/norm.html) on the norm function.
Community and Forums
Joining forums such as MATLAB Central or Stack Overflow can provide additional support and insights from fellow MATLAB users facing similar challenges.
Additional Learning Materials
To enhance your knowledge further, consider exploring books, online courses, or YouTube tutorials that focus on MATLAB programming and numerical methods.