The `fill` function in MATLAB is used to create filled polygons or fill areas between curves, enhancing the visualization of data by shading between specified boundaries.
Here's a code snippet demonstrating how to use the `fill` function:
x = [1 2 3 4];
y = [1 3 2 4];
fill(x, y, 'r') % This creates a red filled polygon defined by the vertices in x and y.
What is the `fill` Function?
The `fill` function in MATLAB is a powerful tool designed to create filled polygons or areas in your plots. It allows users to add clarity and emphasis to their visual data presentations by filling shapes with specified colors. Whether you want to enhance a plot or visualize the area between two curves, `fill` helps you achieve a visually appealing outcome.
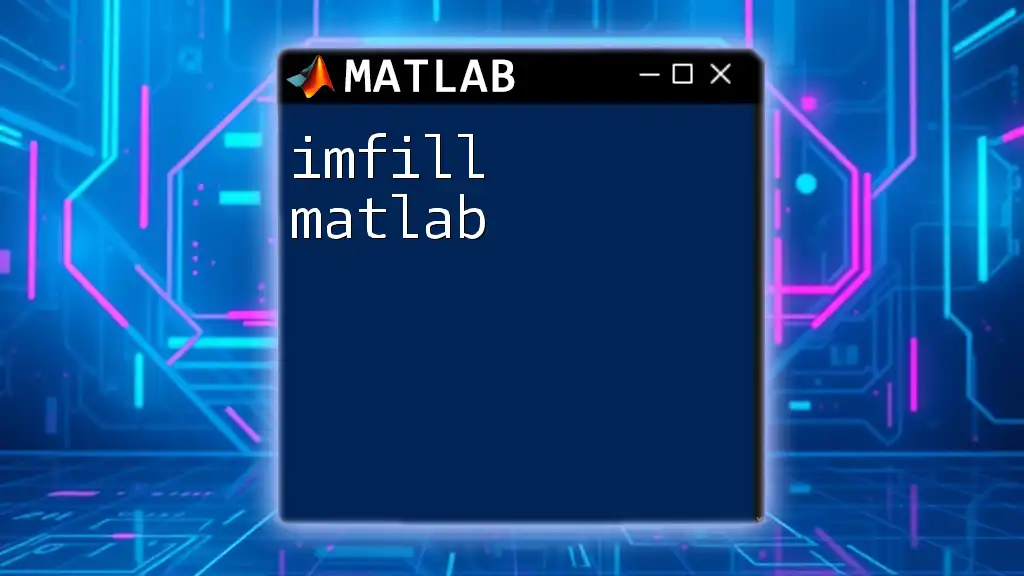
Why Use Filling?
The primary purpose of filling in plots is to enhance visual clarity. By filling areas, you can better represent data trends or highlight specific values. This is especially useful in presentations and reports where making the data stand out can significantly impact understanding and engagement.
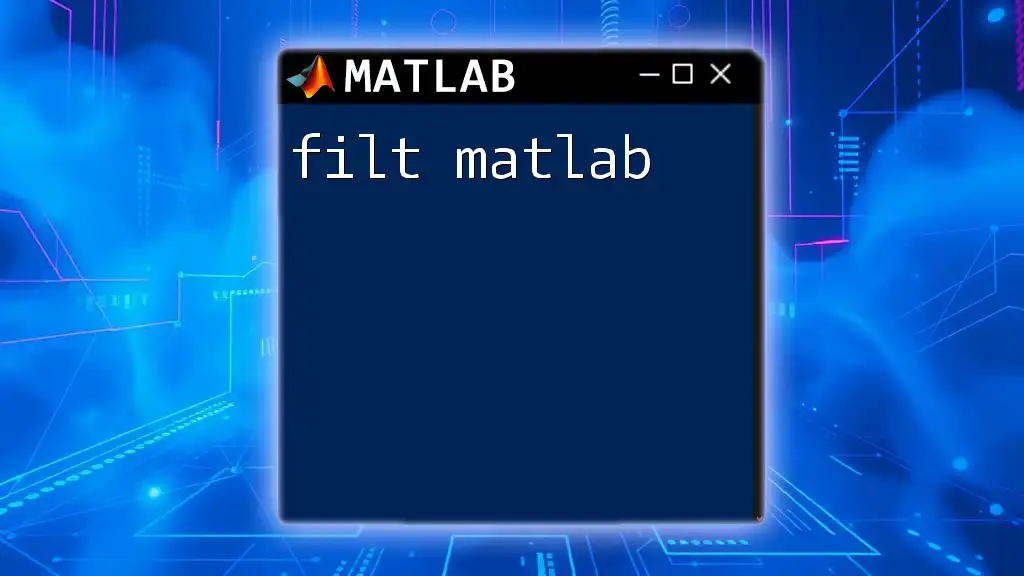
Types of Filling with the `fill` Function
Filling Polygons
One of the fundamental uses of the `fill` function is to create filled polygons. This is accomplished by specifying the x and y coordinates of the polygon's vertices along with a color.
Here's an example to illustrate:
x = [1 2 3];
y = [1 3 1];
fill(x, y, 'r'); % Fill a red triangle
In this example, a triangle defined by the vertices (1,1), (2,3), and (3,1) is filled with red color.
Filling Between Plot Lines
Another common application of `fill` is to fill between two plot lines, which aids in visualizing the area encompassed by them effectively.
Consider the following code snippet:
x = 0:0.1:10;
y1 = sin(x);
y2 = cos(x);
fill([x fliplr(x)], [y1 fliplr(y2)], 'g', 'FaceAlpha', 0.5);
Here, the area between the sine and cosine curves is filled with green, and `fliplr` is used to flip the x-coordinates, allowing the shape to close correctly.
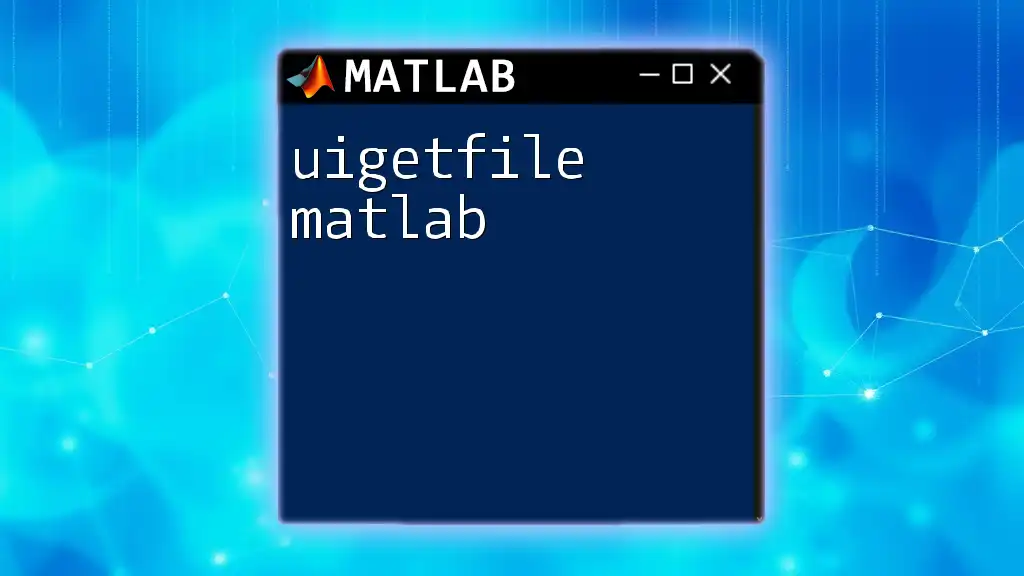
Advanced Features of the `fill` Function
Color and Transparency
To make your plots visually appealing, customizing colors is essential. You can use both RGB triplets and hexadecimal color codes. Furthermore, you can control the transparency of the fill using the `FaceAlpha` property. This allows underlying plots or grids to remain visible, which is beneficial for complex data visualizations.
Edge Properties
The `fill` function also allows customization of edge properties, such as color and line style. Here’s how you can specify these options:
fill(x, y, 'b', 'EdgeColor', 'k', 'LineStyle', '--');
In this example, the polygon is filled blue with a black dashed edge, enhancing its visibility.
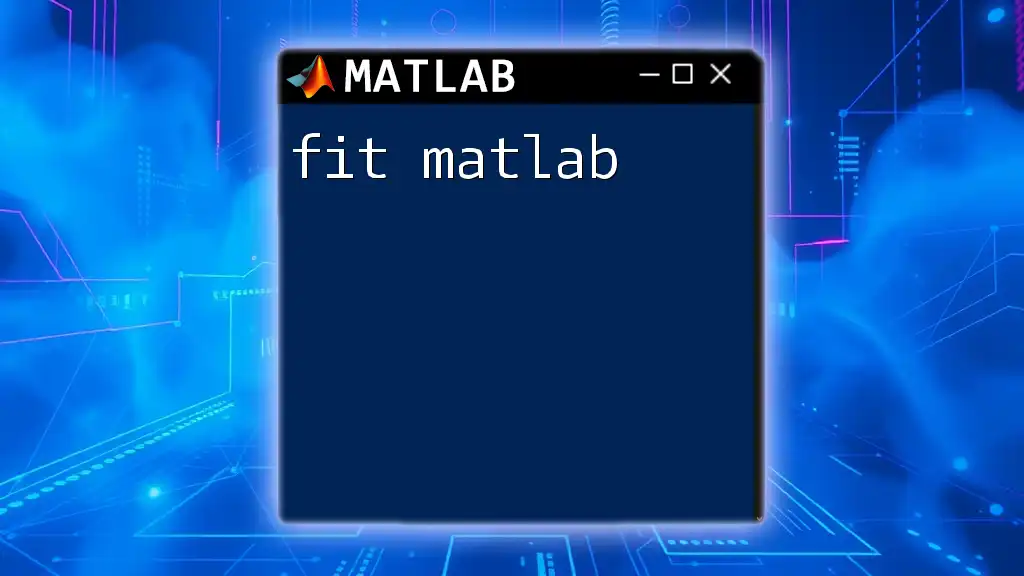
Practical Applications of the `fill` Function
Creating Filled Area Charts
Filled area charts are an effective way to visualize trends over time or among different groups. Here’s a detailed example that illustrates creating a filled area chart:
- Generate Data: First, create your data points, such as a sine and cosine wave.
- Plot Using `fill`: Use the `fill` function to visualize the area between the two datasets:
x = linspace(0, 2*pi, 100);
y1 = sin(x);
y2 = cos(x);
fill([x fliplr(x)], [zeros(size(y1)) fliplr(y1)], 'b', 'FaceAlpha', 0.2);
hold on;
fill([x fliplr(x)], [zeros(size(y2)) fliplr(y2)], 'r', 'FaceAlpha', 0.2);
hold off;
In this example, you are creating a filled area chart to compare sine and cosine functions, significantly enhancing visual comparison.
Highlighting Data Ranges
Filling specific areas in your plot can effectively highlight significant ranges or thresholds in your data. For instance, if you have a dataset representing temperature through a year, you could fill the range that signifies a cold season, visually emphasizing that period.
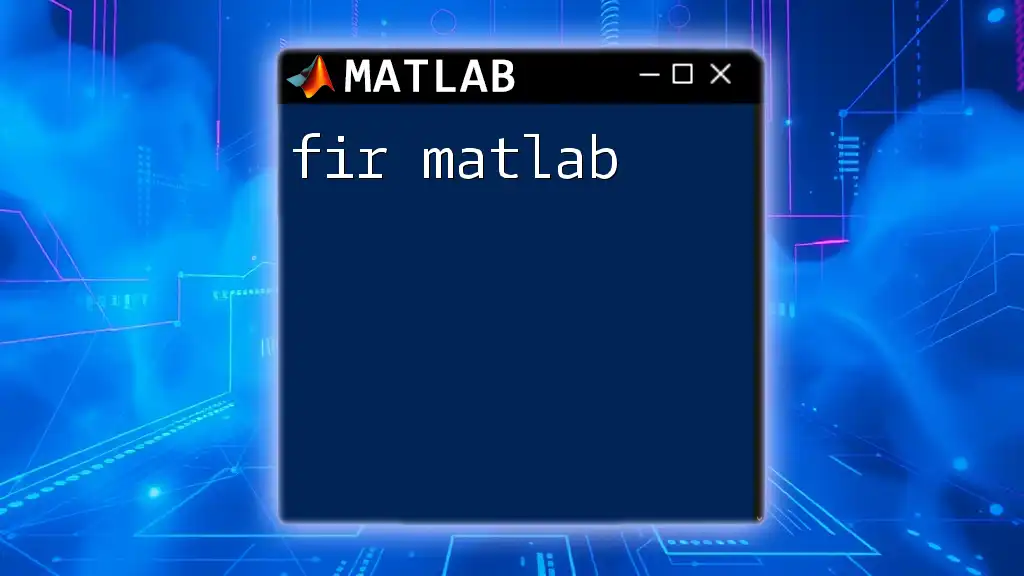
Customizing the `fill` Function
Specifying Axes and Limits
Specifying axis limits is crucial so that the fill extends only to the desired sections. This can be done with the `xlim` and `ylim` functions in MATLAB, and it allows for better control over the appearance of your plots.
Combining `fill` with Other Plotting Functions
The combination of `fill` with other MATLAB plotting functions like `plot` offers greater flexibility. You can layer plots effectively by using `hold on` and `hold off`. For example:
plot(x, y1, 'k--');
hold on;
plot(x, y2, 'k--');
fill([x fliplr(x)], [y1 fliplr(y2)], 'g', 'FaceAlpha', 0.3);
hold off;
This allows you to create a richer visualization composed of both lines and filled areas.
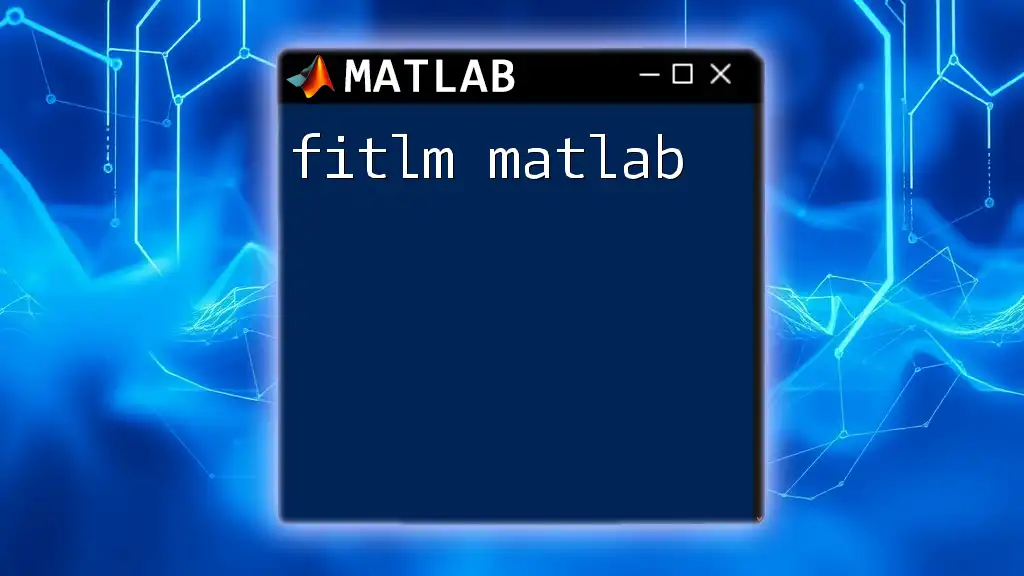
Common Mistakes and Tips
Common Errors When Using `fill`
One frequent error is attempting to fill shapes without correctly closing them, which can result in incomplete visualizations. Always ensure your x and y vectors close the polygon properly.
Best Practices for Effective Use
To optimize your fills, ensure your colors have sufficient contrast from the background and other plot elements. Additionally, don’t overuse filling; it should enhance understanding, not clutter it.
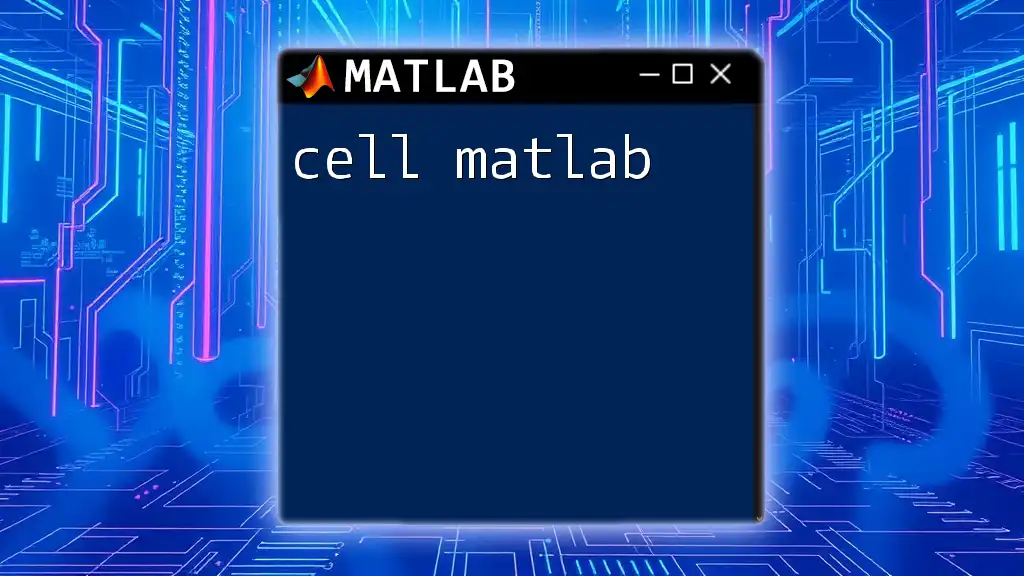
Recap of the `fill` Function’s Importance in MATLAB
The `fill` function is a versatile tool that significantly enriches data visualization in MATLAB. By mastering its usage, you can create informative, aesthetically pleasing plots that can effectively communicate your data’s story.
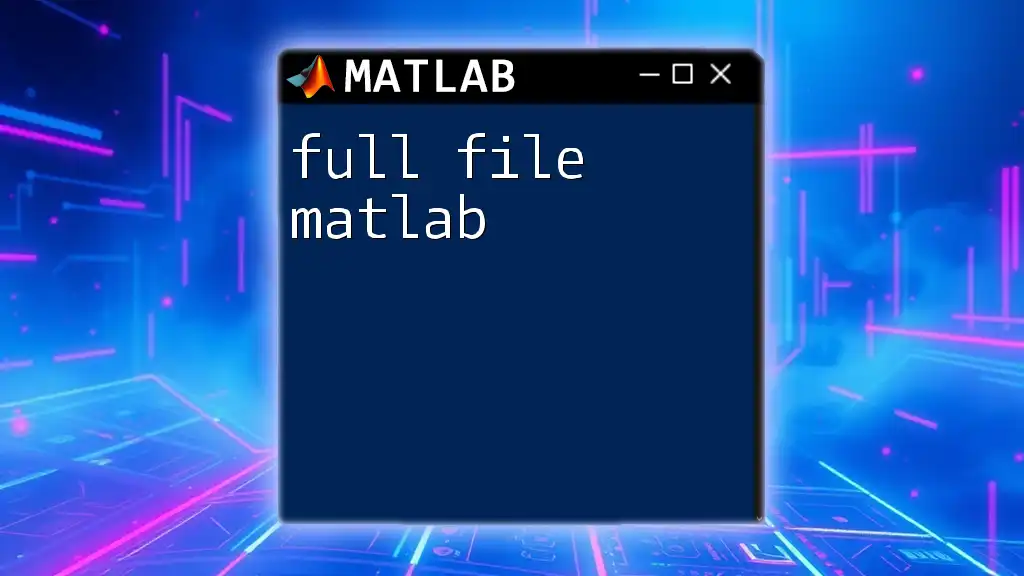
Further Resources
For those looking to delve deeper into the `fill` function and its applications in MATLAB, consider checking the official [MATLAB documentation](https://www.mathworks.com/help/matlab/ref/fill.html) or engaging with community forums and tutorials that provide additional insights and examples for mastering MATLAB visualization.