The `mat2cell` function in MATLAB is used to divide a matrix into cell arrays of smaller matrices, allowing for better organization and manipulation of data.
Here’s a code snippet demonstrating how to use `mat2cell`:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
C = mat2cell(A, [1, 2], [2, 1]); % Divides A into a 1x2 cell array
What is `mat2cell`?
`mat2cell` is a powerful MATLAB function that enables users to convert a matrix into a cell array. This transformation is particularly useful when you need to segment your matrix into smaller parts, allowing for more flexible data manipulation and access. By converting matrices into cell arrays, you can manage collections of arrays of varying sizes, types, and dimensions efficiently.
When to Use `mat2cell`
You should consider using `mat2cell` in scenarios where:
- You need to separate a large matrix into smaller, more manageable pieces.
- You are working with heterogeneous data types that cannot be stored in a standard numeric array.
- You require an efficient way to handle submatrices for applications such as machine learning, data analysis, or simulations.
Using `mat2cell` is a critical skill in MATLAB programming, especially when working with complex datasets.
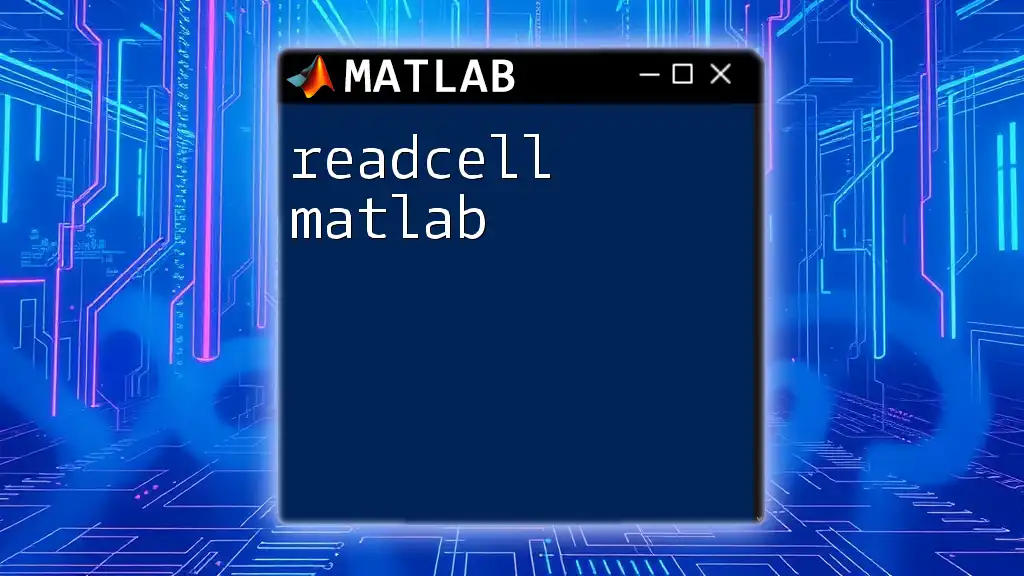
Understanding Cell Arrays in MATLAB
What are Cell Arrays?
Cell arrays are a unique data type in MATLAB designed to hold data of varying types and sizes. Unlike regular numeric arrays, which can only contain elements of a single type, cell arrays can contain numbers, strings, other arrays, or even other cell arrays. This flexibility allows users to manage diverse datasets efficiently.
Advantages of Using Cell Arrays
The primary advantages of cell arrays include:
- Flexibility: You can store different types of data in a single container, allowing for more complex data structures.
- Variable Sizes: Sub-arrays can have disparate sizes, making cell arrays excellent for irregular datasets.
These features make cell arrays essential for many MATLAB applications, enabling efficient data manipulation.
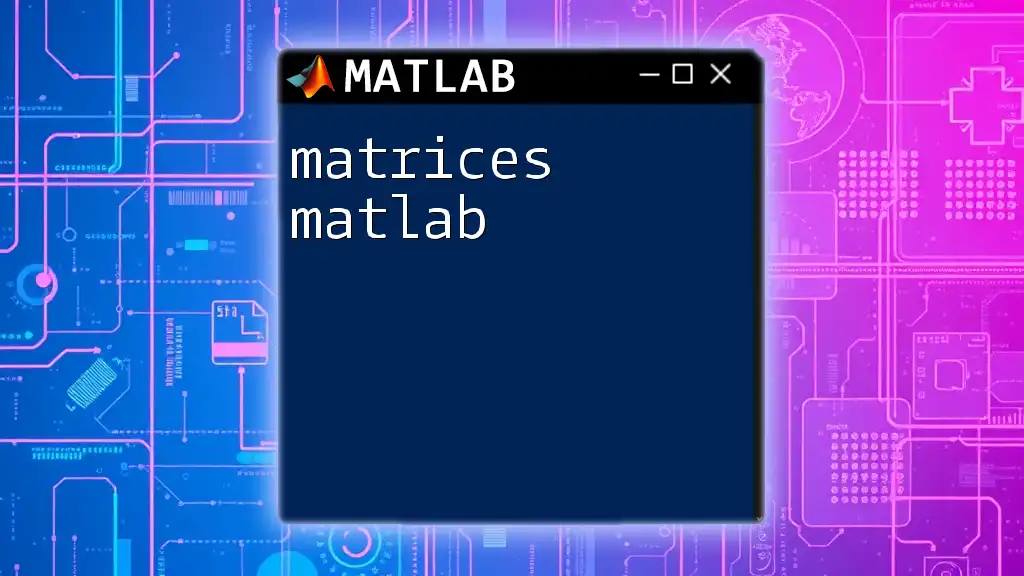
Syntax of `mat2cell`
Breakdown of the Syntax
The syntax for the `mat2cell` function is straightforward:
C = mat2cell(A, rowSize, colSize)
- `A` is the matrix you wish to convert into a cell array.
- `rowSize` specifies the desired sizes for each row in the cell array.
- `colSize` denotes the sizes for each column in the resulting cell array.
Default Behavior
When the `rowSize` and `colSize` arguments are omitted, MATLAB will automatically divide the matrix into cell arrays where each subarray corresponds to a single element of `A`. This default behavior allows for quick conversions without the need to specify sizes.
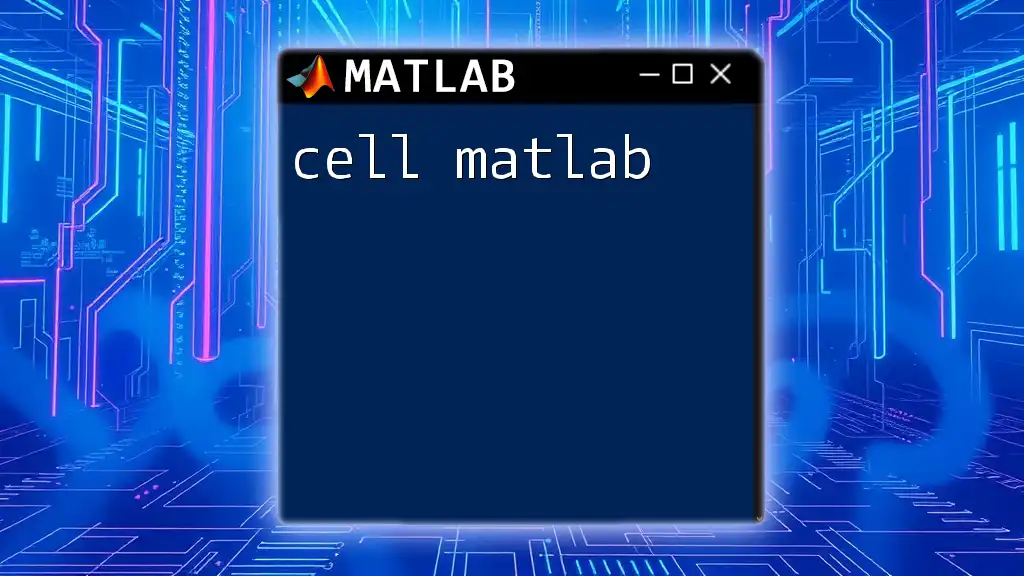
Creating Cell Arrays with `mat2cell`
Specifying Row and Column Sizes
To demonstrate how to create a cell array using specified sizes, consider the following example:
A = [1 2 3; 4 5 6; 7 8 9];
C = mat2cell(A, [2, 1], [1, 2]);
disp(C);
In this code snippet, the matrix `A` is being divided into subarrays. The row sizes `[2, 1]` indicate that the first cell will contain 2 rows and the second cell will contain 1 row. The column sizes `[1, 2]` specify that the first cell will contain 1 column and the second cell will contain 2 columns. The output will be a cell array with specific partitioning based on these sizes.
Handling Different Sized Subarrays
For cases where you want to create uneven partitions, you can use the following approach:
B = rand(4, 8);
C = mat2cell(B, [2, 1, 1], [3, 2, 3]);
disp(C);
In this example, matrix `B` is split into three rows with sizes of 2, 1, and 1, and three columns with sizes of 3, 2, and 3. This flexibility allows you to tailor the division of your matrix based on your specific needs.
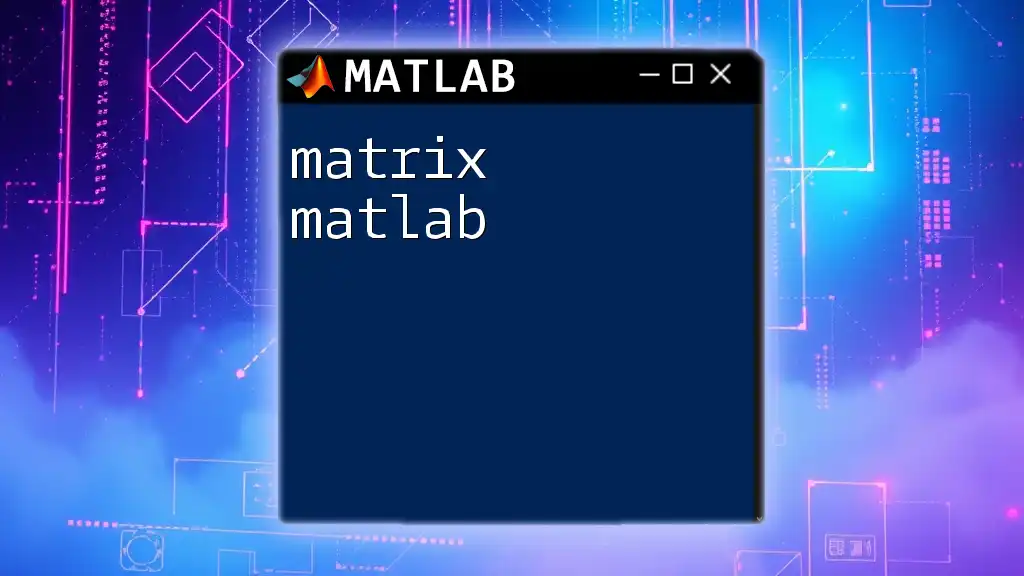
Advanced Usage of `mat2cell`
Nested Cell Arrays
Creating nested cell arrays can be beneficial in complex applications, such as structuring hierarchical data. Here’s an example:
D = magic(4);
C = mat2cell(D, [2, 2], [2, 2]);
disp(C);
The output will be a cell array of cell arrays, enabling nested data organization.
Combining with Other Functions
`mat2cell` can be seamlessly integrated with other MATLAB functions, such as `cell` and `num2cell`, to fulfill specific needs. For instance, if you have a numeric array and want to convert it while also manipulating the dimensions, see this example:
E = [1 2 3; 4 5 6];
F = num2cell(E);
G = mat2cell(F, [1, 1], [2, 1]);
disp(G);
Here, a conversion occurs from a numeric matrix into a cell array, demonstrating versatility in data handling.
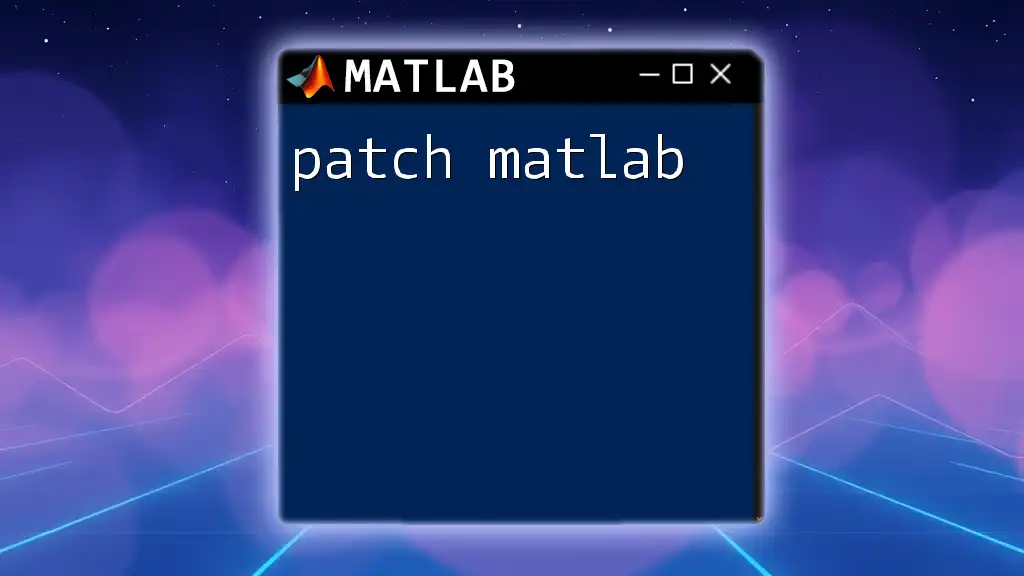
Practical Applications of `mat2cell`
Data Segmentation
In data analysis, you may often need to segment your dataset for training and testing subsets in machine learning. For example:
X = rand(100, 5); % Features
Y = randi([0, 1], 100, 1); % Labels
trainFeatures = mat2cell(X(1:70,:), [70], [5]);
trainLabels = mat2cell(Y(1:70,:), [70], [1]);
This example demonstrates creating training features and labels for model training.
Dynamic Array Management
Dynamic data needs often call for various array dimensions. By using `mat2cell`, you can manage this effectively.
data = rand(10, 10);
C = mat2cell(data, [4, 3, 3], [3, 4, 3]);
In this scenario, you create a structure that dynamizes your matrix handling.
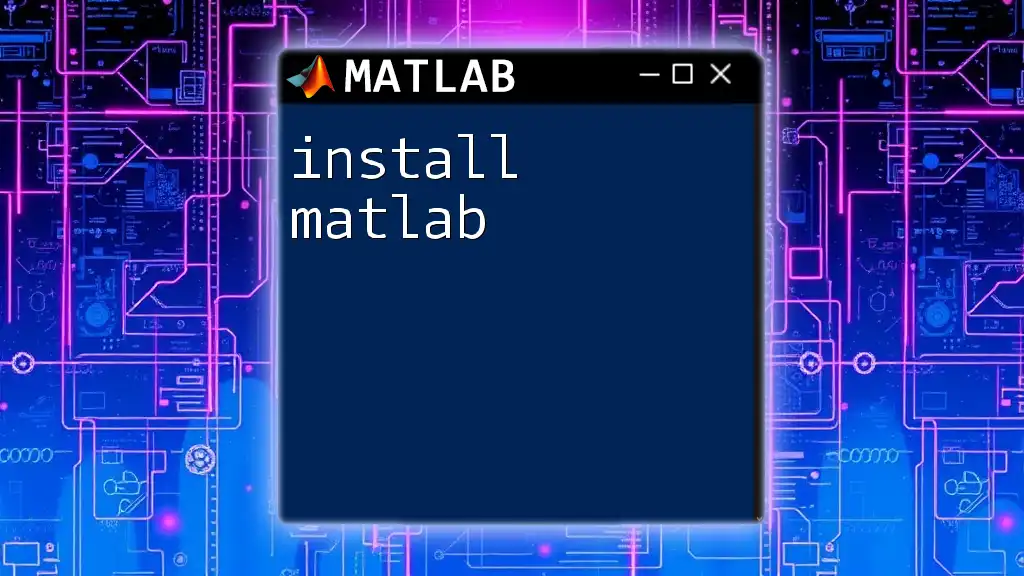
Performance Considerations
Memory Management
While cell arrays provide flexibility, it's vital to consider memory usage. Cell arrays can consume more memory than standard arrays due to their dynamic nature. Use `mat2cell` judiciously and ensure you're not unnecessarily increasing memory overhead.
Alternatives to `mat2cell`
Sometimes, a simpler function like `reshape` or the use of `arrayfun` might be more suitable. Evaluate your application needs carefully before opting for `mat2cell`, especially in performance-critical applications.
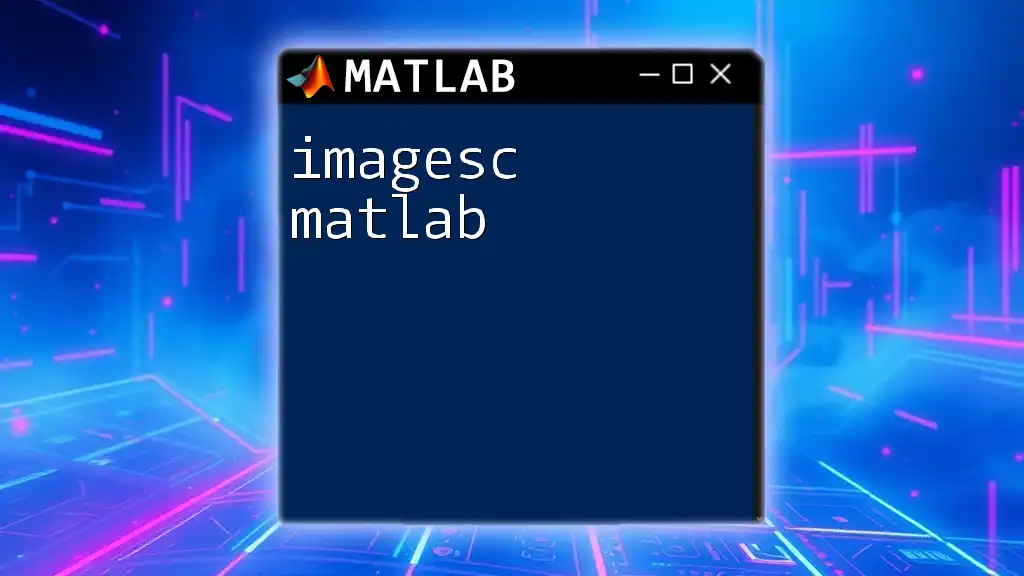
Common Errors and Troubleshooting
Common Mistakes with `mat2cell`
Mismatched dimensions between specified sizes and the original matrix will frequently lead to runtime errors. Additionally, overlooking the need for proper array partitioning is a common error leading to inefficient data handling.
Solutions and Tips
To avoid errors, always ensure that the total sizes specified in `rowSize` and `colSize` sum appropriately to the dimensions of your original matrix. Familiarize yourself with the structure of cell arrays to enhance understanding and proficiency.
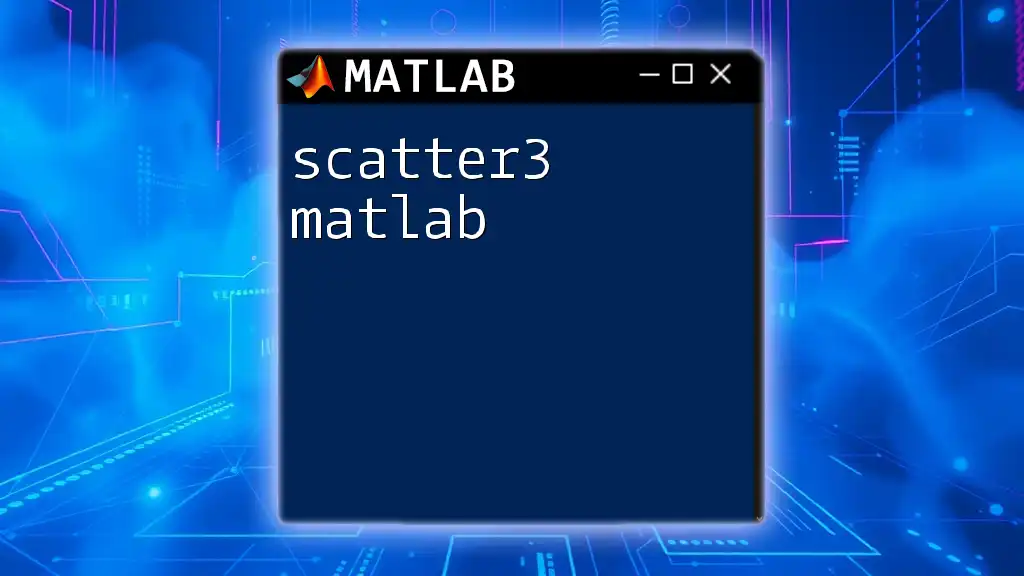
Conclusion
The `mat2cell` function in MATLAB is an indispensable tool for anyone dealing with complex datasets. By learning to efficiently convert matrices into cell arrays, you enhance your programming capabilities, making it easier to manipulate, analyze, and visualize data. With practice and application, the use of `mat2cell` can significantly streamline your MATLAB workflow.
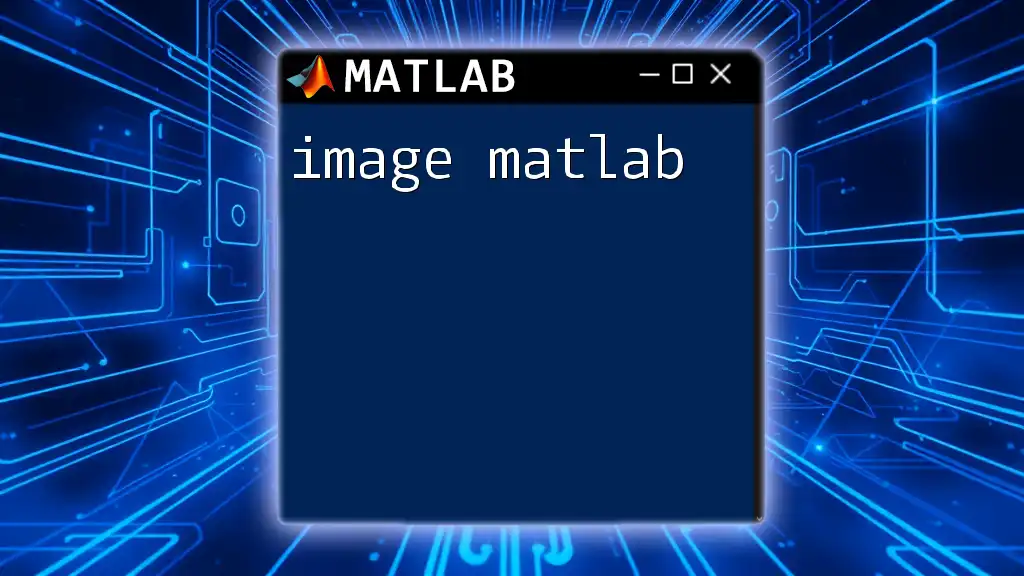
Additional Examples and Exercises
Example Problems
-
Task: Convert a 3x4 matrix of random integers into a 2x2 cell array with specific subarray sizes.
-
Task: Use `mat2cell` to segment a dataset into training and validation sets.
Solutions and Explanations
Carefully work through these exercises by applying the techniques outlined throughout this guide, reinforcing your understanding of how to use `mat2cell` effectively in MATLAB.