The `findpeaks` function in MATLAB is used to identify and extract the local maxima (peaks) from a dataset, allowing for quick analysis of features in a signal or data series.
% Example of finding peaks in a signal
x = 0:0.1:10; % Define the x values
y = sin(x); % Define the y values as a sine wave
[pks, locs] = findpeaks(y); % Find peaks and their locations
plot(x, y, x(locs), pks, 'ro'); % Plot the signal and highlight the peaks
Understanding Peaks
Definition of Peaks
A peak in a dataset refers to a point that is higher than its surroundings. Peaks can be classified into local peaks, which are maximas within a limited range, and global peaks, the highest points in the entire dataset. Understanding these definitions is crucial for effectively analyzing your data, especially in applications like signal processing, where identifying peaks can reveal critical insights.
Why Finding Peaks is Important
Finding peaks has numerous applications across various fields:
- In engineering, analyzing signal peaks can help detect faults in systems.
- In finance, identifying peaks in stock price trends can signal the perfect entry or exit points for investors.
- In healthcare, peak detection in biological signals like ECGs can assist in diagnosing conditions.
To maximize the potential insights from your data, it’s essential to have a robust method for identifying these significant points.
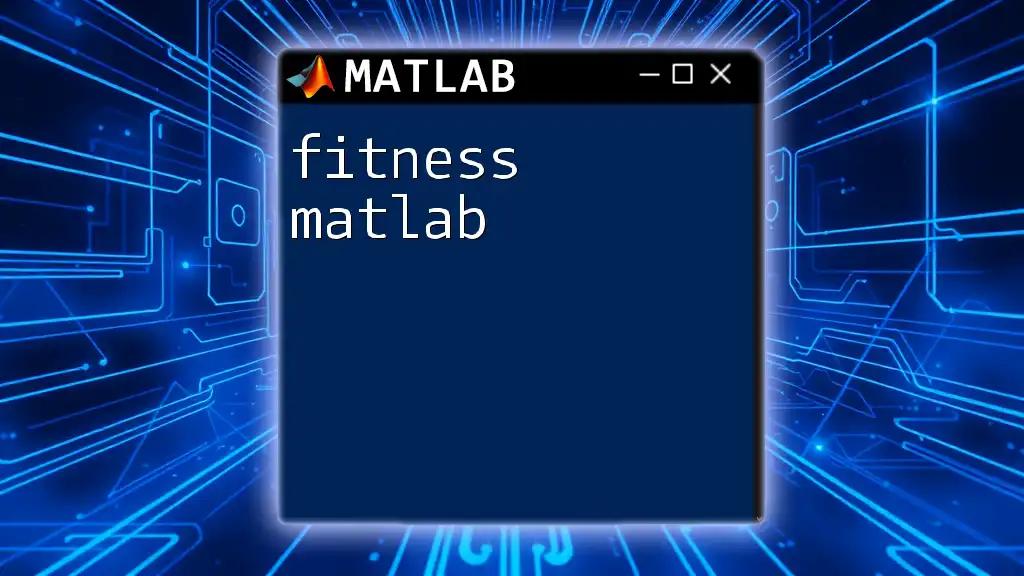
Getting Started with MATLAB
Overview of MATLAB Commands
MATLAB is a powerful tool commonly used for mathematical computations, data analysis, and visualization. Understanding the syntax and structure of commands is critical for harnessing its full potential. Commands typically consist of the function name followed by parameters enclosed in parentheses.
Setting Up Your MATLAB Environment
Before you start coding, ensure you have MATLAB installed along with necessary toolboxes, such as the Signal Processing Toolbox. This toolbox includes functions that simplify peak detection.
- Open MATLAB and create a new script in the MATLAB editor.
- Familiarize yourself with the workspace, command window, and how to run scripts.
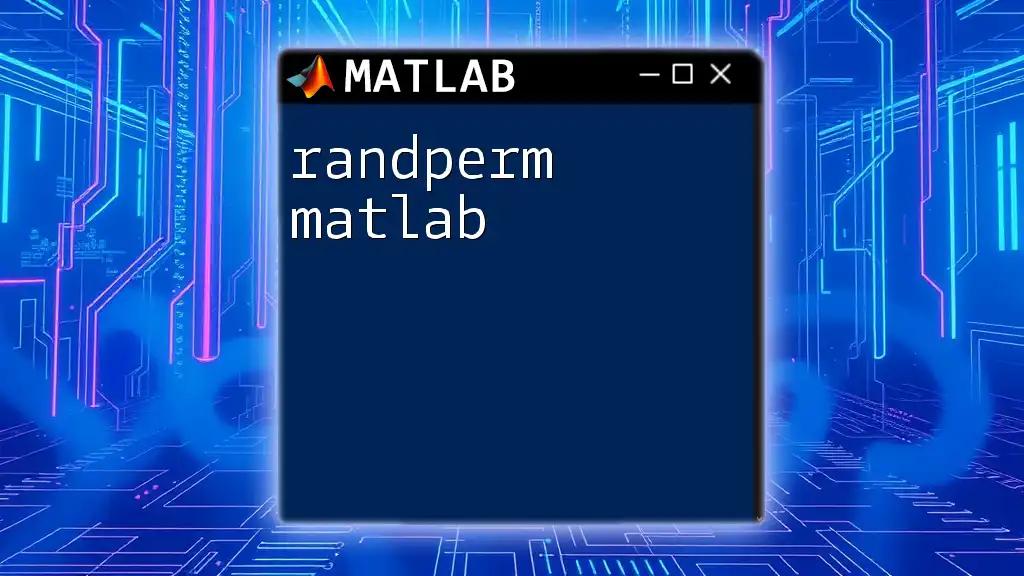
Finding Peaks using MATLAB Functions
The `findpeaks` Function
The `findpeaks` function is a go-to tool for detecting peaks in MATLAB. The basic syntax is:
[pks, locs] = findpeaks(data);
- pks: The heights of the peaks.
- locs: Indices of the peaks in the data array.
Basic Example:
x = 0:0.1:10;
y = sin(x);
[pks, locs] = findpeaks(y);
plot(x, y, x(locs), pks, 'ro');
This example generates a sine wave, detects the peaks, and plots them in red. The code above demonstrates how to visually represent the detected peaks alongside the original data.
Essential Parameters of `findpeaks`
The `findpeaks` function has several key parameters that allow you to fine-tune your peak detection:
-
MinPeakHeight: This option sets a minimum threshold for peak heights. Peaks that are below this height will not be detected.
Example:
[pks, locs] = findpeaks(y, 'MinPeakHeight', 0.5);
-
MinPeakDistance: This specifies the minimum distance between adjacent peaks. It helps to avoid detecting multiple peaks that are too close together, which can lead to confusion in your analysis.
Example:
[pks, locs] = findpeaks(y, 'MinPeakDistance', 10);
-
Threshold: This parameter is used to filter out peaks based on their height relative to neighboring points, allowing for more refined peak identification.
Handling Noise in Data: Pre-processing Techniques
Introduction to Data Smoothing
Data often contains noise, which can obscure real peaks. Smoothing techniques help reduce this noise, making it easier to detect actual peaks. Common smoothing methods include:
- Moving Average: A simple technique that averages a fixed number of neighboring points.
- Gaussian Smoothing: Applies a Gaussian kernel for smoother transitions.
Example: Smoothing Data Before Finding Peaks
Before finding peaks, it’s often helpful to smooth your data. Here’s a practical implementation:
y_smooth = smoothdata(y, 'gaussian', 5); % Gaussian smoothing
[pks_smooth, locs_smooth] = findpeaks(y_smooth);
plot(x, y_smooth, x(locs_smooth), pks_smooth, 'ro');
This code snippet demonstrates how to apply Gaussian smoothing to a dataset before peak detection, allowing for clearer identification of peaks.

Advanced Techniques for Peak Detection
Custom Peak Detection Algorithms
Creating a custom peak detection algorithm may be necessary when dealing with complex datasets where standard functions like `findpeaks` do not suffice. A tailored approach allows for unique conditions found in your specific application.
For instance, you can create a function that identifies peaks under your specific conditions:
function [pks, locs] = customPeakDetector(data)
% Custom logic for peak detection
pks = findpeaks(data);
end
Using Other Functions for Peak Detection
In addition to `findpeaks`, MATLAB offers other functions like `islocalmax` and `islocalmin` that can aid in peak detection.
Example of Using `islocalmax`
The `islocalmax` function allows you to identify local maxima without specifying parameters related to height or distance.
pks_custom = islocalmax(y);
plot(x, y, x(pks_custom), y(pks_custom), 'ro');
Utilizing `islocalmax` can be particularly beneficial for quickly scanning data for peaks without overly complex conditions.
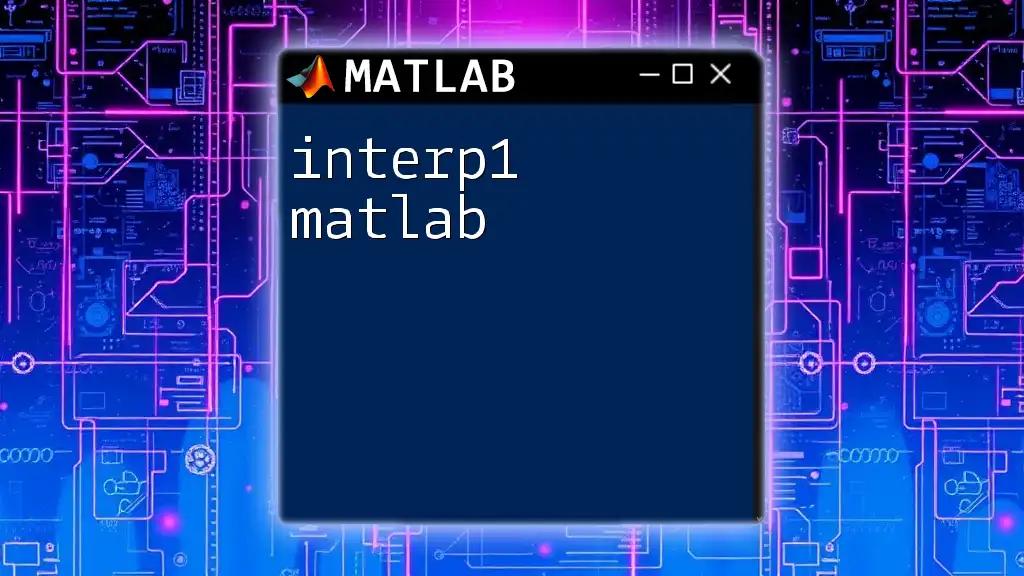
Visualizing Peaks in MATLAB
Plotting Techniques
Visualization is a key part of data analysis. When plotting detected peaks, you can enhance your MATLAB visuals using different markers, colors, and legends. Here’s a generic example of visualizing data with peaks:
figure;
plot(x, y);
hold on;
plot(x(locs), pks, 'o', 'MarkerFaceColor', 'r');
title('Peak Detection');
xlabel('X-axis');
ylabel('Y-axis');
legend('Data', 'Peaks');
Such visual clarity helps communicate your findings effectively, making your peaks stand out against the data.
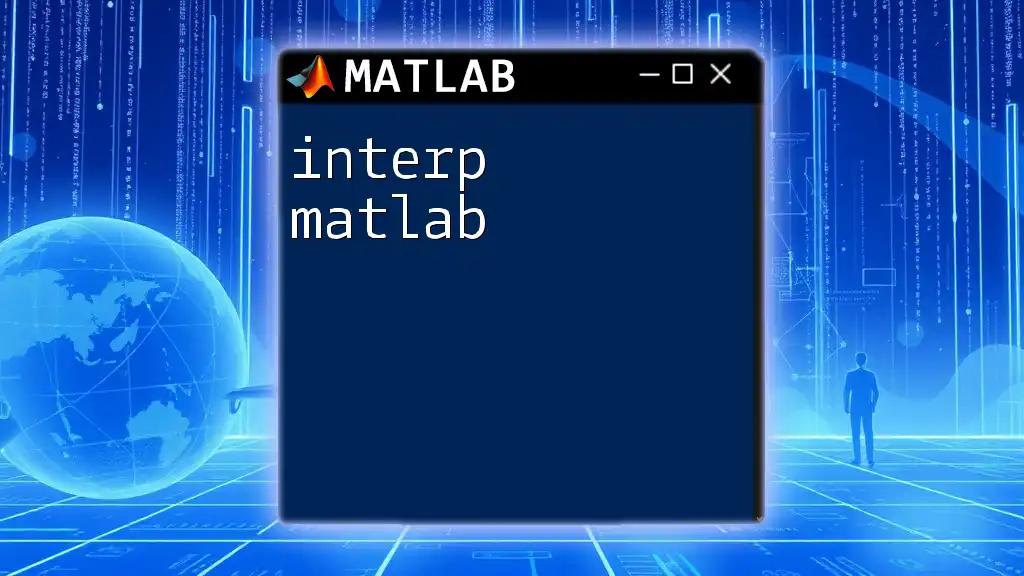
Troubleshooting Common Issues with Peak Detection
Understanding False Peaks
False peaks occur when noise or other artifacts in the data are misidentified as actual peaks. To reduce the incidence of false peaks:
- Adjust parameters: Fine-tuning `MinPeakHeight` and `Threshold` can help filter out incorrect detections.
- Pre-process data: Smoothing data before applying peak detection will minimize noise and improve accuracy.
Addressing Missing Peaks
Missing peaks can result from overly stringent parameters. Consider the following to ensure your analysis captures all relevant peaks:
- Relax parameters: Temporarily broaden the threshold or minimum height.
- Investigate the data: Visualize and examine the data to understand where peaks may exist but are currently not detected.
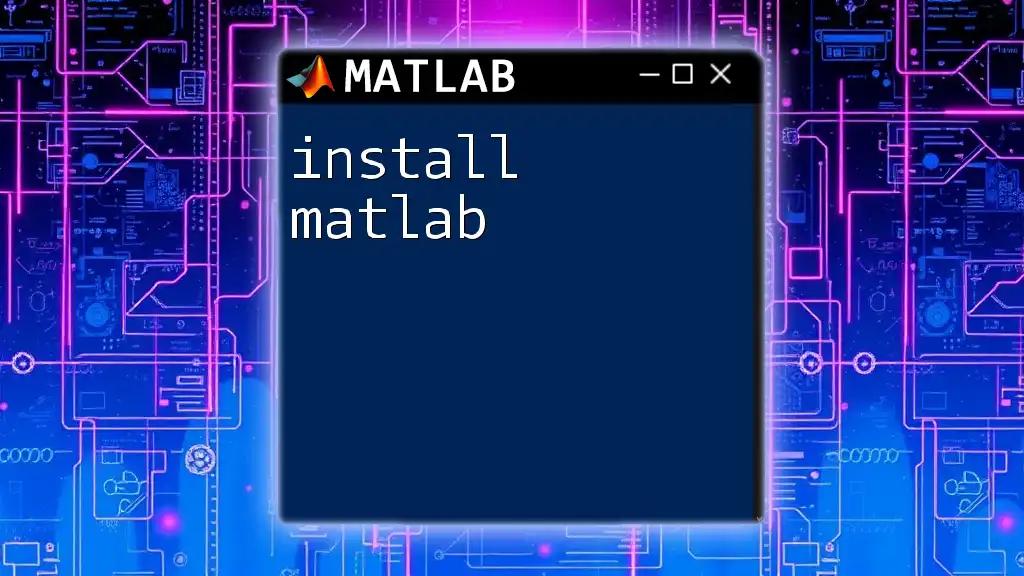
Conclusion
Effectively using MATLAB to find peaks in data sets can unlock valuable insights across various research and practical applications. By mastering the `findpeaks` function and understanding parameters such as MinPeakHeight, MinPeakDistance, and Threshold, you can enhance your data analysis skills significantly.
Experimenting with smoothing techniques and alternative functions will further improve the reliability of your peak detection. As you gain confidence in these methods, consider customizing your algorithms to fit your specific needs and explore the fascinating world of data analysis in MATLAB.
Engage with the community or reach out if you have questions, challenges, or triumphs in your peak detection journey!