The `finddelay` function in MATLAB is used to determine the delay between two signals, enabling you to identify how much one signal is shifted relative to another.
Here's a simple example of how to use the `finddelay` function:
% Define two signals
x = [1, 2, 3, 4, 5];
y = [0, 1, 2, 3, 4]; % y is x delayed by 1 time unit
% Find the delay between the signals
delay = finddelay(x, y);
disp(['The delay is: ', num2str(delay)]);
Understanding the Concept of Delay
What is Delay in Signals?
In signal processing, delay refers to the time shift that occurs when one signal is altered in time relative to another. This phenomenon is significant in various applications, including telecommunications, audio processing, and control systems. Understanding the delay between two signals can help engineers and scientists synchronize data, adjust timing in communications, and enhance audio effects.
Types of Delays
There are primarily two types of delays that one might encounter in signal processing:
-
Fixed Delay: A constant delay that remains the same throughout the duration of the signal. For instance, if a signal is consistently delayed by 2 seconds, this is a fixed delay.
-
Variable Delay: A delay that can fluctuate over time or across different segments of the signal. For example, in real-time communications, the delay may change due to network congestion.
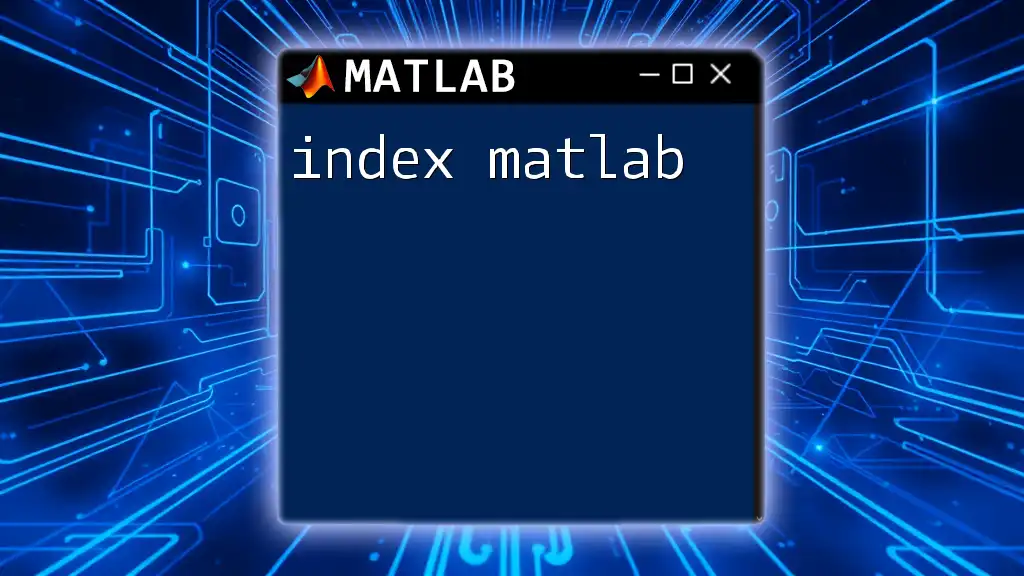
Introduction to `finddelay`
What is `finddelay`?
The `finddelay` function in MATLAB serves to identify the time delay between two signals. Primarily used in applications such as signal processing and data synchronization, this function is integral for tasks where precise timing is crucial.
Syntax of `finddelay`
The basic syntax of the `finddelay` function is:
delay = finddelay(x, y)
Here, `x` and `y` are the two signals you wish to compare. The function returns the number of samples by which the first signal `x` is delayed relative to the second signal `y`.
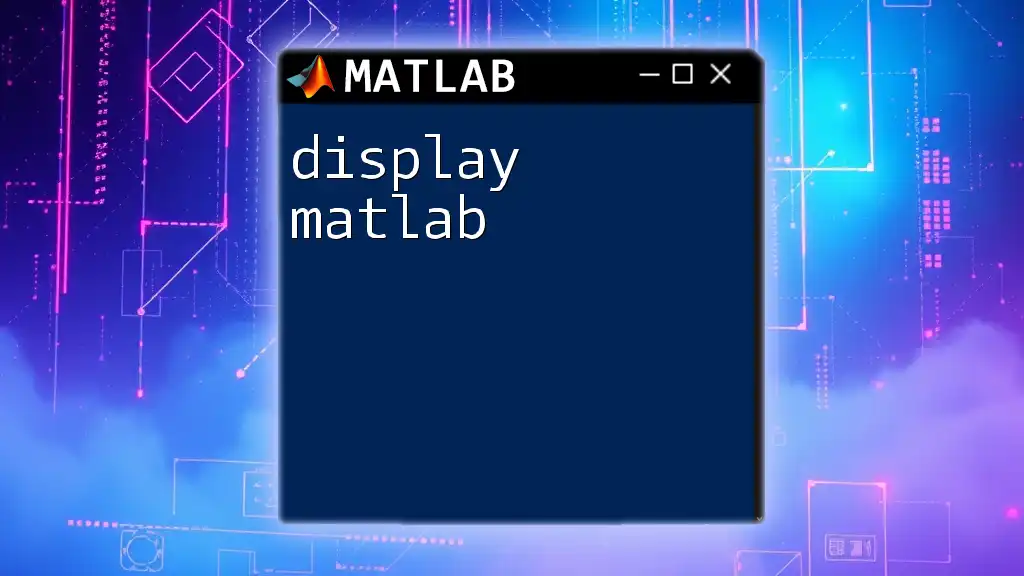
How to Use `finddelay` in MATLAB
Prerequisites
Before using `finddelay`, ensure that you have the required MATLAB toolboxes, specifically the Signal Processing Toolbox. Additionally, it's essential to prepare the signals correctly by ensuring they have the same sampling frequency and are appropriately aligned for analysis.
Step-by-step Tutorial
Preparing Your Signals
To demonstrate how to use `finddelay`, you first need to create two example signals in MATLAB. Below is an example of how to create a sine wave and a delayed version of it.
t = 0:0.01:1; % Time vector
signal1 = sin(2*pi*5*t); % Original signal
signal2 = [zeros(1, 30), signal1(1:end-30)]; % Delayed signal
In this code:
- `t` defines the time vector over which our signal will be evaluated.
- `signal1` is a sine wave generated at 5 Hz, while `signal2` is created by delaying `signal1` by 0.3 seconds (30 samples, considering a 100 Hz sampling frequency).
Implementing `finddelay`
With the signals prepared, you can now use the `finddelay` function to calculate the delay. Here's how:
delay = finddelay(signal1, signal2);
disp(['The delay between the two signals is: ', num2str(delay)]);
This snippet executes the `finddelay` function, calculates the delay, and outputs the result. The output will provide the number of samples that correspond to the time delay between the signals. For a sampled signal at 100 Hz, a delay of 30 samples would indicate that `signal2` is delayed by 0.3 seconds.
Interpreting the Results
Once you obtain the delay value, it’s crucial to analyze what this means in the context of your project. A positive value implies that `signal1` leads `signal2`, while a negative value indicates that `signal2` leads `signal1`.
Understanding these dynamics can prove vital when designing systems where timing and synchronization are pivotal.
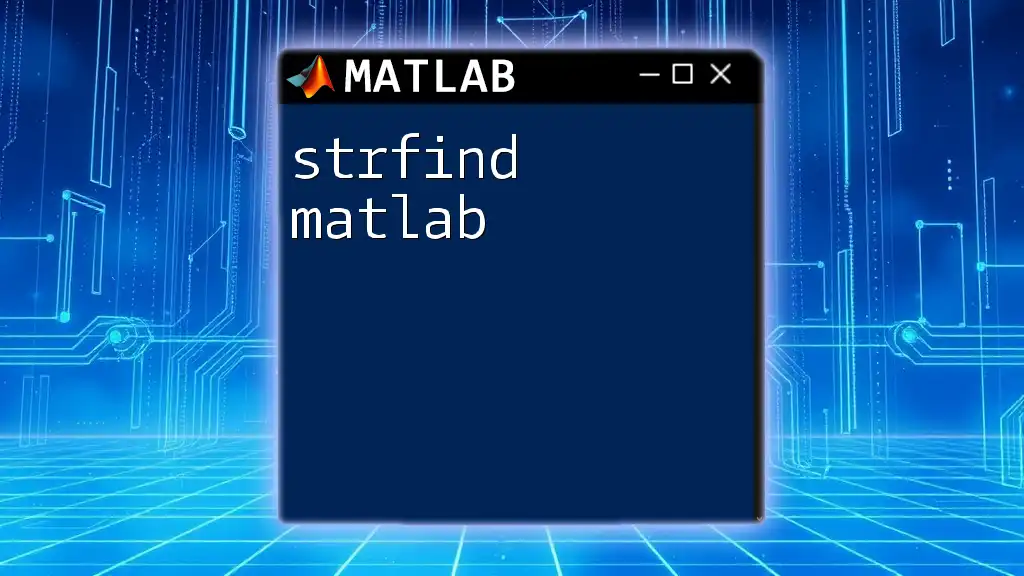
Common Mistakes and Troubleshooting
How to Avoid Errors
A common issue when using `finddelay` is providing incompatible signal lengths. Always ensure that `x` and `y` are of the same length or that the relevant parts of the signals intended for comparison are being analyzed.
Debugging `finddelay` Results
If the results from `finddelay` are unexpected, consider validating your input signals. Ensure they are both properly scaled, have the same sampling rates, and are free from noise or distortion. You can also visualize the signals using the `plot` function to see their alignment before applying the delay function.
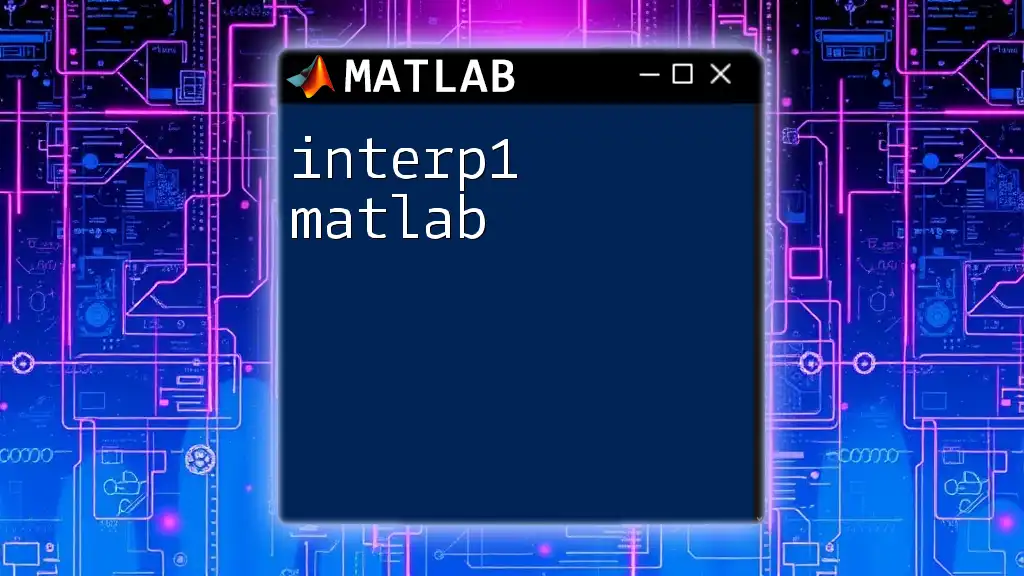
Practical Applications of `finddelay`
Audio Signal Processing
In audio signal processing, the `finddelay` function can be crucial for synchronizing multiple audio tracks. For instance, if you have two audio signals captured at different times, `finddelay` can help you align them for coherent playback. Here is a practical example:
[audio1, fs1] = audioread('track1.wav');
[audio2, fs2] = audioread('track2.wav');
delay = finddelay(audio1, audio2);
% Correct the delay in audio2 using the signal processing toolbox functions
This code reads two audio files and finds the delay between them, which could then be used to adjust the timing of playback.
Communications Systems
In telecommunications, accurately understanding delay can inform network design, especially in systems where time-sensitive data is essential. If two signals represent messages sent through a network, identifying the time delay can help optimize data processing and delivery.
Example Code Snippet:
message1 = randn(1, 1000); % Simulated signal
message2 = circshift(message1, 5); % Simulated delayed message
delay = finddelay(message1, message2);
disp(['Measured delay in communications: ', num2str(delay), ' samples']);
Other Use Cases
- Robotics and Control Systems: Delays are common in feedback control loops where sensor data could be delayed due to processing time. `finddelay` can help tune control systems by identifying these delays and adjusting responses accordingly.
- Biological Data Analysis: In areas such as healthcare, monitoring time delays in physiological signals (e.g., heartbeats) is crucial for accurate diagnostics.
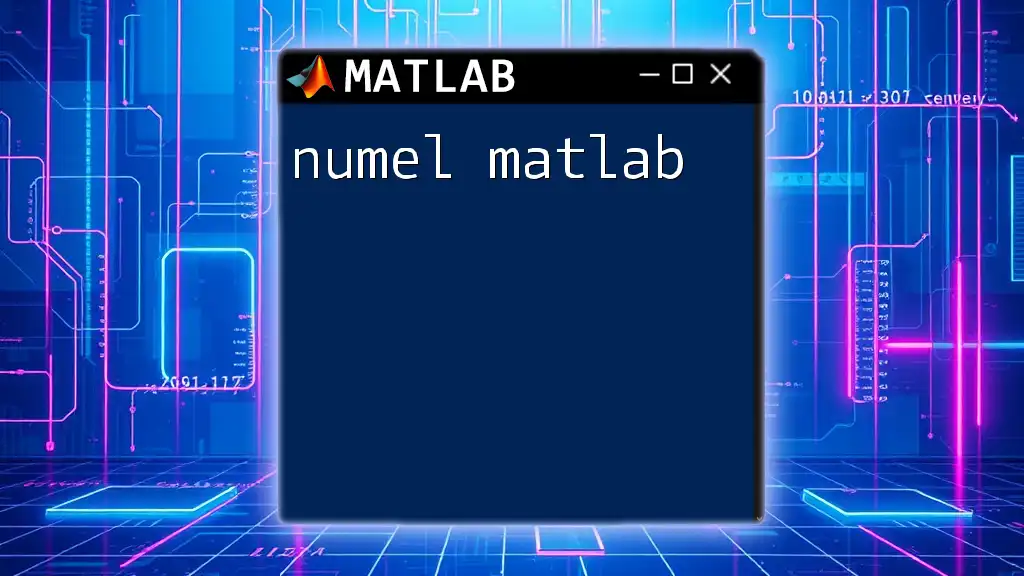
Conclusion
Using the `finddelay` function in MATLAB can significantly enhance your signal processing capabilities. From synchronizing audio tracks to optimizing communication systems, understanding how to accurately measure delays empowers users to address real-world problems effectively. By experimenting with examples, you can deepen your understanding of time delays in signals and their practical applications across various fields.
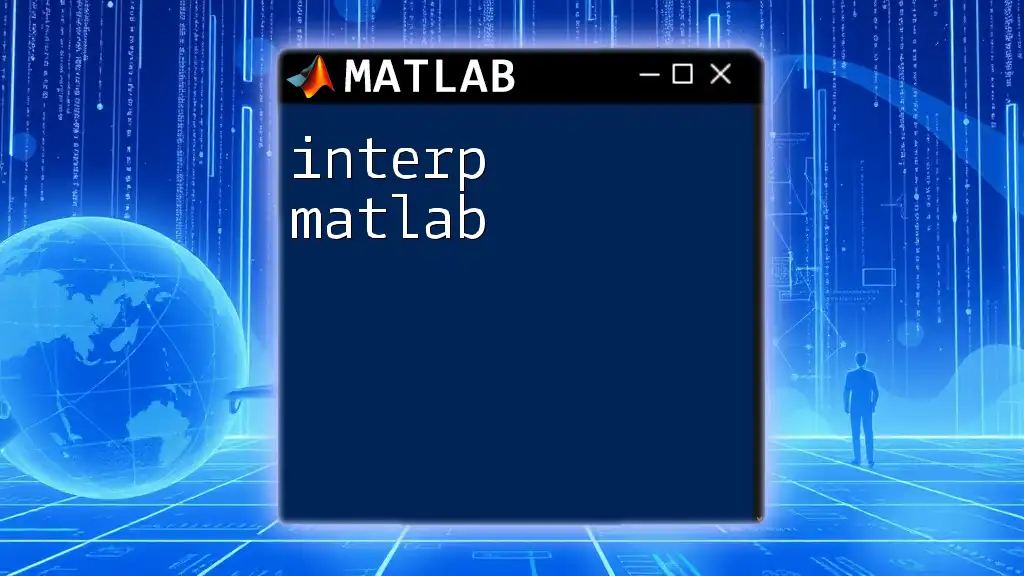
Additional Resources
Recommended MATLAB Documentation
For further learning, MATLAB provides extensive documentation on `finddelay` and related functions. Explore [MATLAB’s Official Documentation](https://www.mathworks.com/help/signal/ref/finddelay.html) for detailed information.
Online Communities and Forums
Engage with communities like MATLAB Central, Stack Overflow, and specific Signal Processing forums for additional support, where you can ask questions, share insights, and collaborate with other enthusiasts.
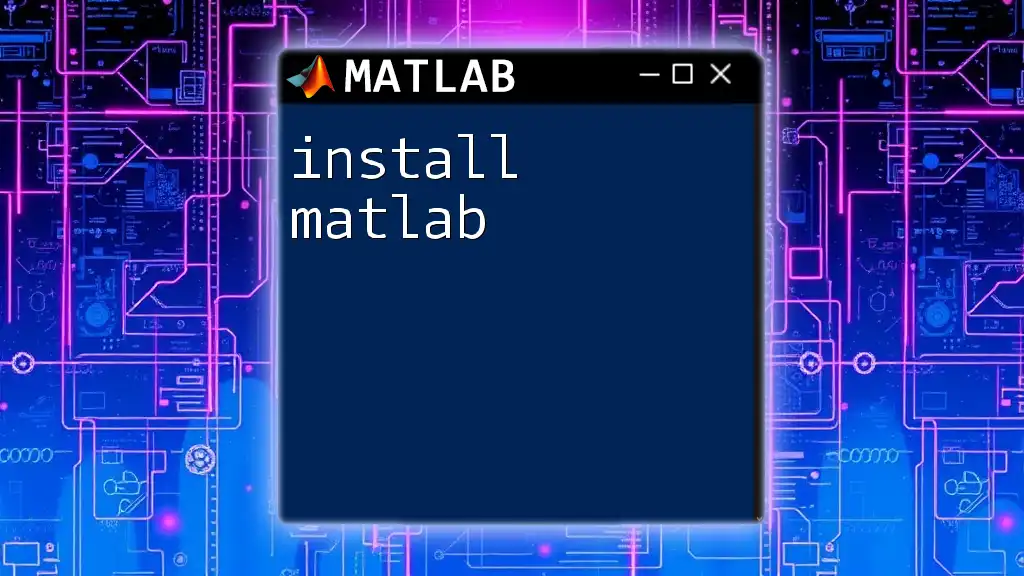
Call to Action
If you are eager to unlock the full potential of MATLAB commands, consider signing up for our courses tailored to teach MATLAB efficiently and effectively. Visit our website for more articles and tutorials that resonate with your learning style!