The `findpeaks` function in MATLAB is used to identify local maxima (peaks) in a dataset, allowing users to analyze signals or datasets for significant features easily.
Here's a simple example of using `findpeaks`:
data = [1, 3, 2, 5, 4, 6, 3, 7, 2]; % Sample data
[pks, locs] = findpeaks(data); % Find peaks and their locations
disp(pks); % Display peak values
disp(locs); % Display locations of peaks
Understanding Peaks in Data
What Are Peaks?
Peaks are significant points in a dataset where a value is higher than its immediate neighbors. In mathematical terms, a peak (or local maximum) occurs at a point where the derivative changes from positive to negative. Understanding peaks is crucial for analyzing trends, detecting events, or extracting meaningful features from data.
Importance of Peak Detection
Detecting peaks holds great value in numerous applications, such as:
- Signal Processing: Identifying significant points in sound waves or other signals.
- Data Analysis: Recognizing trends and changes in time-series data.
- Scientific Measurements: Isolating critical events in experimental data (e.g., detecting pulses in EEG signals).
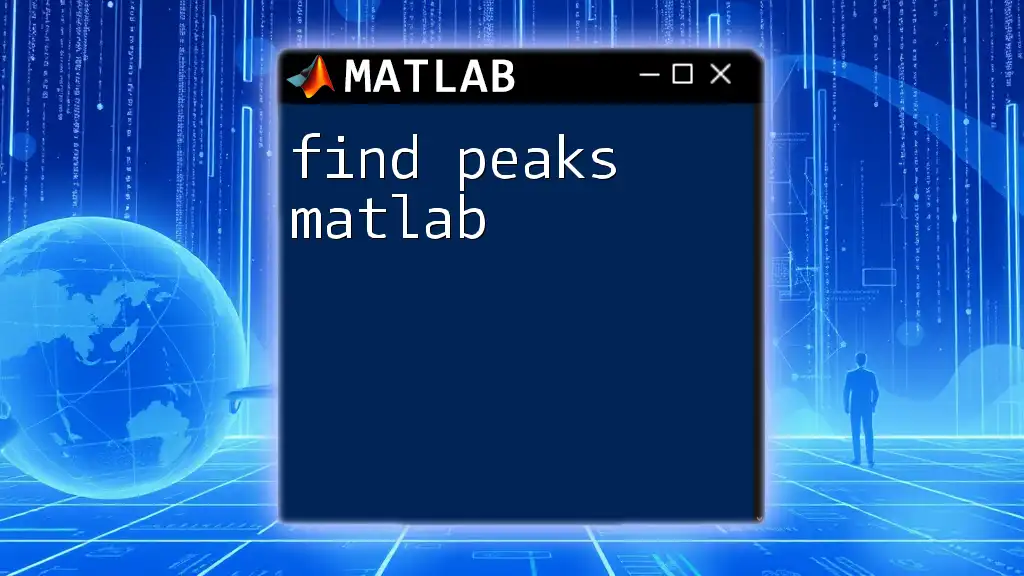
MATLAB `findpeaks` Function
Overview of `findpeaks`
The `findpeaks` function in MATLAB is a powerful tool for detecting peaks in numeric data. With a single line of code, users can easily identify local maxima, which significantly simplifies the task of data analysis.
Syntax Breakdown
The basic syntax of `findpeaks` is:
pks = findpeaks(x)
Here, `x` is the input data vector, and `pks` contains the values of the detected peaks.
For more control over the detection process, users can customize the peak-finding behavior using additional parameters:
[pks, locs] = findpeaks(x, 'PropertyName', PropertyValue...)
For example, if you want to detect peaks in a dataset:
x = [1 3 2 6 4 5 2];
[pks, locs] = findpeaks(x);
This will yield `pks` containing the peak values and `locs` specifying their indices in the original data.
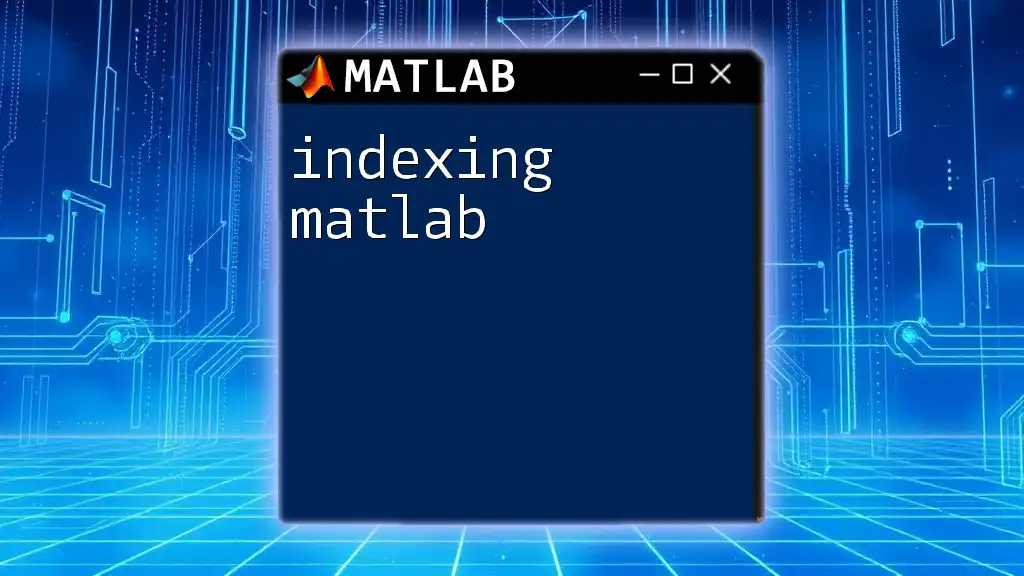
Parameters of `findpeaks`
Commonly Used Parameters
To refine the peak detection, several parameters can be specified when calling `findpeaks`:
MinPeakHeight
This parameter sets the minimum height that peaks must have to be detected. Peaks below this threshold will be ignored.
[pks, locs] = findpeaks(x, 'MinPeakHeight', 4);
MinPeakDistance
With this parameter, users can specify the minimum number of samples that must be between two peaks. This helps in avoiding the detection of closely spaced peaks.
[pks, locs] = findpeaks(x, 'MinPeakDistance', 2);
Threshold
This sets the minimum difference between a peak and its neighbors, ensuring that only the most prominent peaks are identified.
Code Examples for Parameters
Combining multiple parameters can lead to more accurate peak detection. Here’s how you might do it:
[pks, locs] = findpeaks(x, 'MinPeakHeight', 4, 'MinPeakDistance', 2);
Visualization of Peaks
Visualizing the data with detected peaks is essential for better understanding. Here is how you can plot the original signal along with the detected peaks:
plot(x);
hold on;
plot(locs, pks, 'rv', 'MarkerFaceColor', 'r'); % Red triangles for peaks
hold off;
title('Peak detection using findpeaks');
xlabel('Samples');
ylabel('Amplitude');
This visual representation will help you see exactly where the peaks are located relative to the entire dataset.
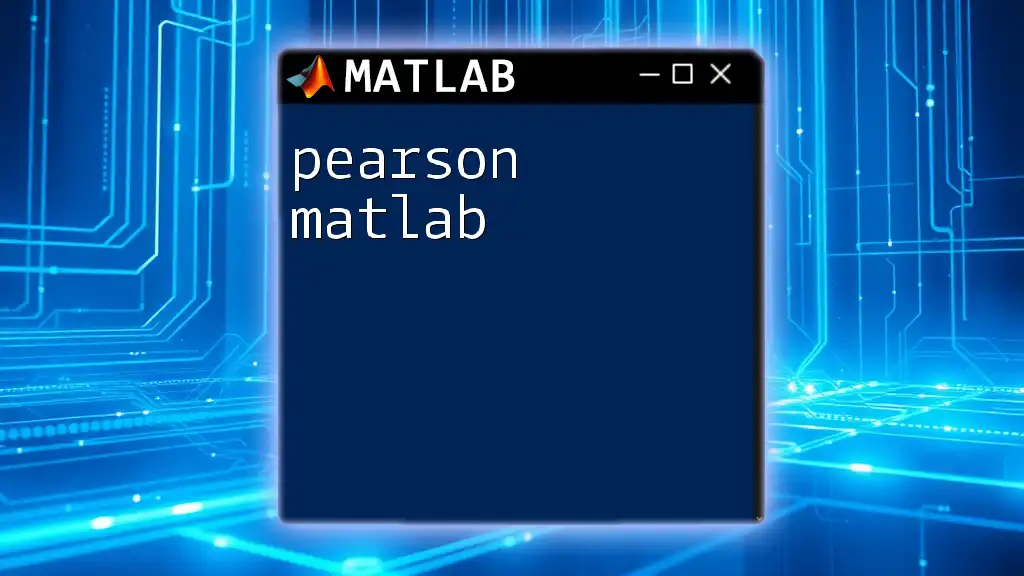
Advanced Features of `findpeaks`
Attribute Selection
`findpeaks` can return additional information about the detected peaks. Attributes like widths and prominences can provide deeper insight into the nature of each peak:
[pks, locs, widths, prominences] = findpeaks(x, 'Annotate', 'extents');
By analyzing these attributes, you can assess the strength and significance of each detected peak.
Custom Peak Finding
In some cases, users may require custom peak detection algorithms. You can create a function that implements advanced logic tailored to your specific needs:
function [pks, locs] = custom_peak_finder(data)
% Custom logic for finding peaks
end
This allows for flexibility beyond what the built-in `findpeaks` function offers.
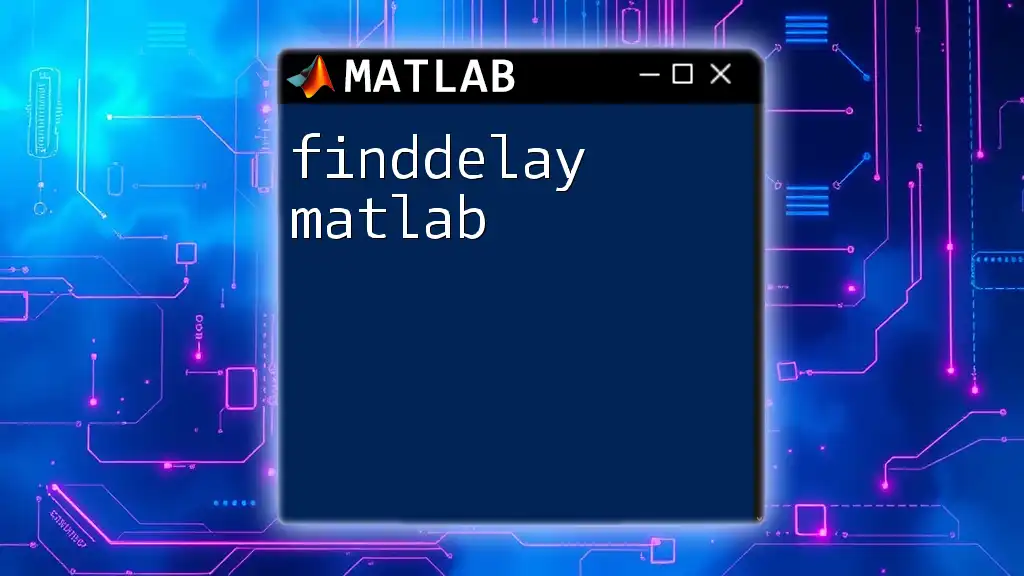
Handling Noise and Preprocessing Data
Smoothing Data Before Peak Detection
Before applying `findpeaks`, it's often beneficial to smooth your data to reduce noise. Common techniques include using a moving average. This preprocessing step can significantly enhance peak identification accuracy.
smoothed_data = smoothdata(x, 'movmean', 3);
Applying `findpeaks` to Smoothed Data
Once the data is smoothed, you can apply `findpeaks` to it:
[pks, locs] = findpeaks(smoothed_data);
This usually results in cleaner and more reliable peak detection.
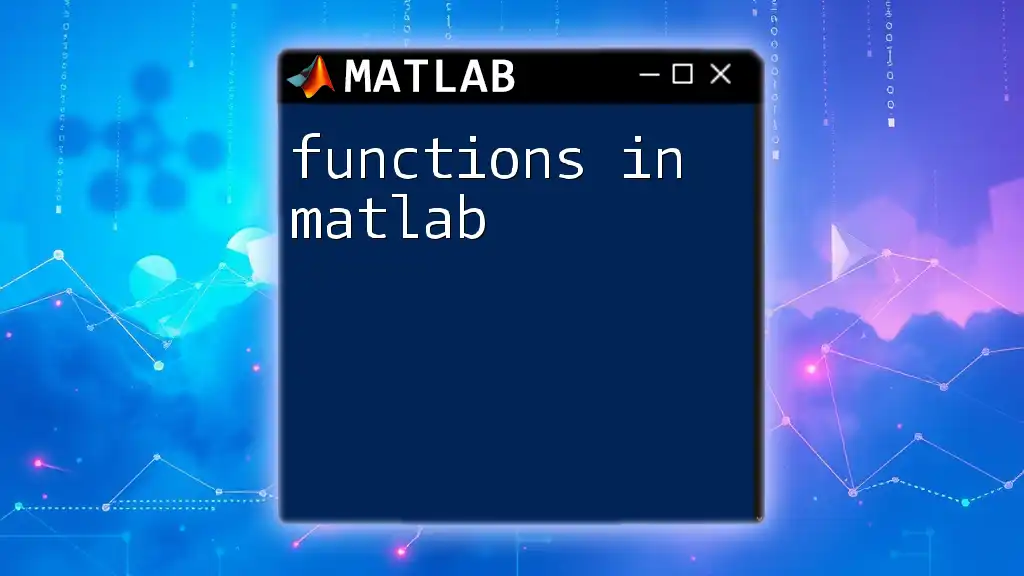
Case Studies
Practical Example 1: Signal Processing
In signal processing, noisy data can obscure significant peaks. By using `findpeaks`, you can detect important features reliably. For instance, in an audio signal, you might extract notes or beats by applying `findpeaks` after smoothing the waveform.
Practical Example 2: Time-Series Analysis
In time-series data, peaks often correspond to noteworthy events like sales spikes. By detecting these peaks with `findpeaks`, analysts can efficiently identify trends that warrant further investigation.
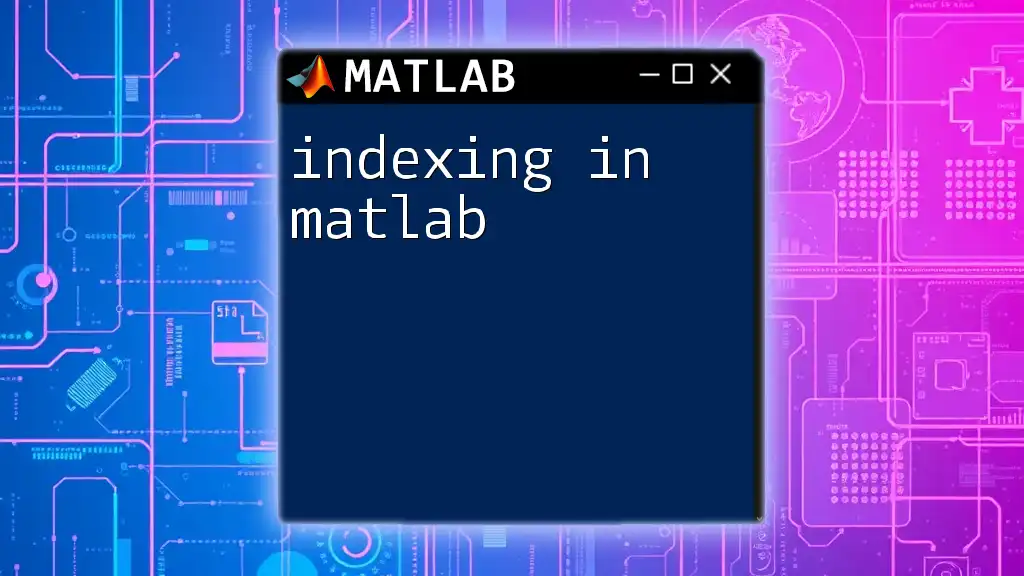
Troubleshooting Common Issues
Misidentified Peaks
Sometimes `findpeaks` may detect peaks that do not align with your expectations. A common reason for misidentification is inadequate parameter settings. Always ensure your parameters are appropriately selected based on the characteristics of your data.
Adjusting Parameters
Fine-tuning the parameters of `findpeaks` is essential for optimizing the peak detection. Experiment with different combinations of `MinPeakHeight`, `MinPeakDistance`, and `Threshold` until you achieve the desired results.
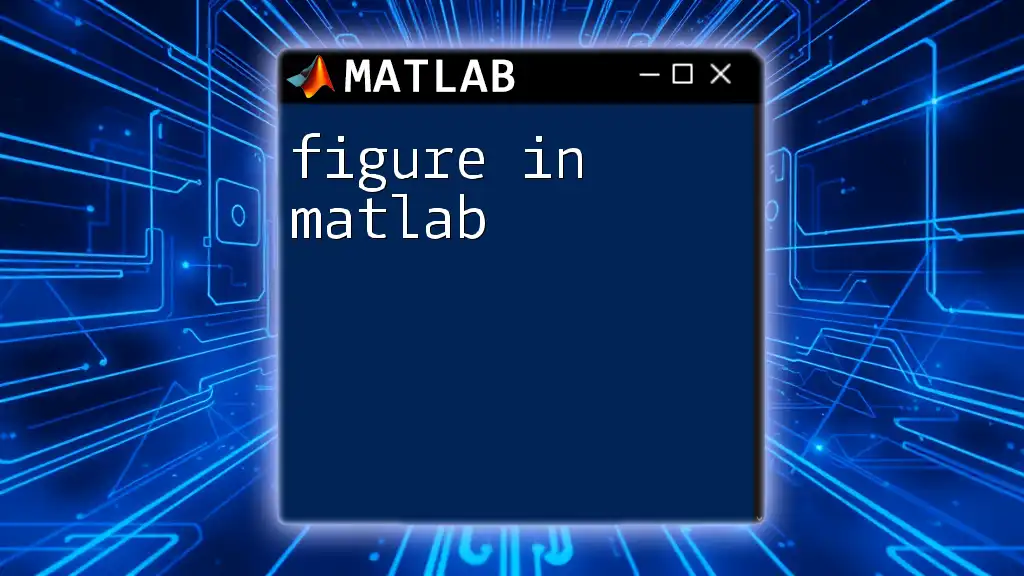
Conclusion
In conclusion, the `findpeaks` function in MATLAB is a versatile and powerful tool for peak detection. By understanding its syntax, parameters, and advanced features, users can effectively extract valuable insights from their data. Whether you're working in signal processing, data analysis, or experimental science, mastering `findpeaks` can significantly enhance your analytical capabilities. Don't hesitate to explore and experiment with this function to unlock its full potential in MATLAB.
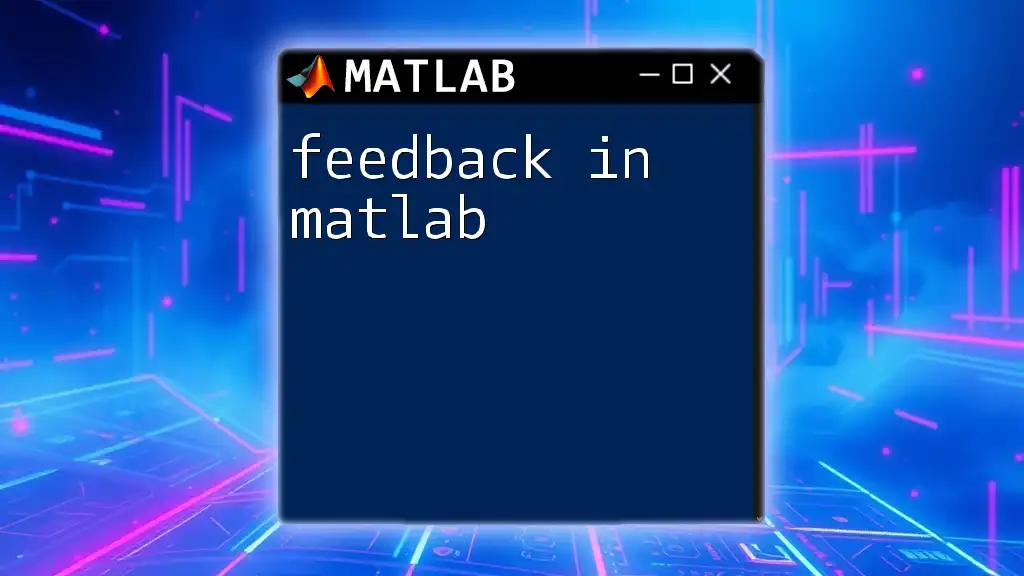
FAQs about `findpeaks` in MATLAB
What does `findpeaks` return?
The output of `findpeaks` typically includes the values of the detected peaks and their respective indices in the original data. If additional attributes are requested, it can also return information like peak widths and prominences.
Can I use `findpeaks` on multi-dimensional data?
`findpeaks` is designed for one-dimensional numeric arrays. For multi-dimensional data, consider applying `findpeaks` to each dimension separately.
How does `findpeaks` handle complex datasets?
For complex datasets with overlapping peaks, proper parameter selection is crucial. You may need to smooth the data or develop custom algorithms to cater to your specific requirements.
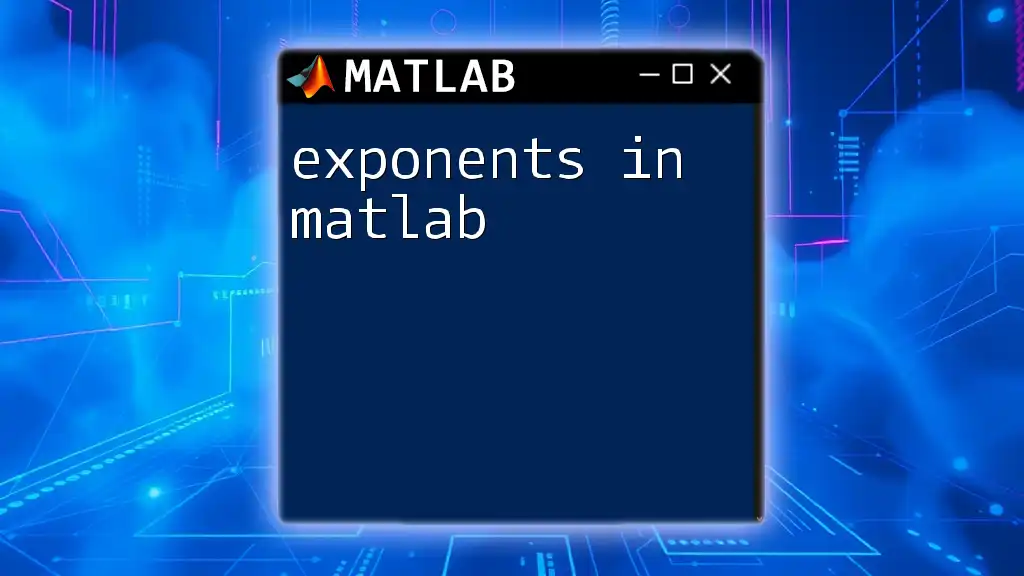
Additional Resources
For further learning, refer to MATLAB’s official documentation on the `findpeaks` function and explore community forums to connect with other MATLAB users. There are a plethora of tutorials and examples available online that can deepen your understanding and application of peak detection techniques.