Fitting with MATLAB allows users to model data using various mathematical techniques, enabling the discovery of relationships between variables through functions like polynomial, linear, or nonlinear fitting.
Here’s a simple example of polynomial fitting in MATLAB:
% Sample data points
x = [1, 2, 3, 4, 5];
y = [1.2, 2.9, 3.5, 4.8, 5.1];
% Fit a polynomial of degree 2
p = polyfit(x, y, 2);
% Generate fitted values
y_fit = polyval(p, x);
% Plot data and fitting curve
plot(x, y, 'ro', x, y_fit, 'b-');
title('Polynomial Fitting with MATLAB');
xlabel('X');
ylabel('Y');
legend('Data Points', 'Fitted Curve');
Understanding Fitting
What is Fitting?
Data fitting refers to the process of constructing a curve or mathematical function that best aligns with a series of data points. It is essential in modeling relationships between variables and predicting future data points. Unlike interpolation, which connects data points exactly, fitting allows the model to approximate data trends, providing a smooth model that can be used for prediction.
Fitting plays a crucial role in various fields, such as science (e.g., growth models in biology), engineering (e.g., stress-strain relationships in materials), and finance (e.g., forecasting stock prices).
Types of Fitting
Linear Fitting
Linear fitting involves modeling data with a straight line. This is useful when the relationship between the independent variable \(x\) and dependent variable \(y\) can be approximated with a linear equation of the form \(y = mx + b\), where \(m\) is the slope and \(b\) is the y-intercept. Linear fitting is commonly applied in trend analysis, where a simple summary of data behavior is required.
Non-Linear Fitting
Non-linear fitting is required when data do not follow a straight line. Examples include quadratic curves, exponential growth, and logarithmic decay. The model is defined by a more complex equation, accommodating varying rates of change, making it suitable for more intricate relationships found in real-world applications.
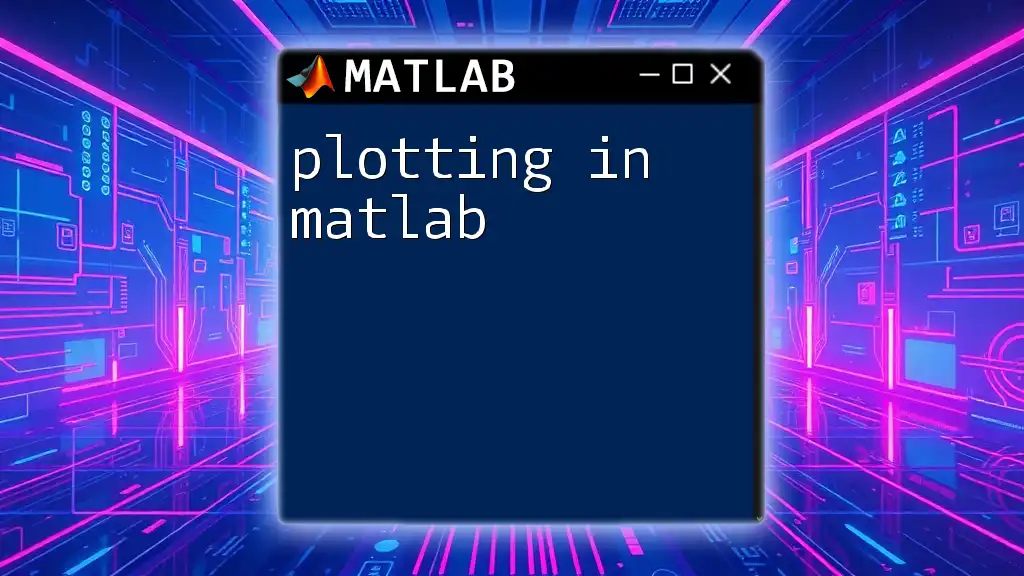
Getting Started with MATLAB
Setting Up MATLAB
To commence fitting with MATLAB, you first need to set up your environment. MATLAB can be downloaded from the MathWorks website. After installation, familiarize yourself with the interface, which includes command windows, the workspace, and the editor.
Key MATLAB Functions for Fitting
To achieve effective data fitting in MATLAB, there are several commands to familiarize yourself with:
- `polyfit()`: This function is used for polynomial data fitting and is ideal for linear and polynomial relationships.
- `fit()`: Provides a flexible way to fit custom models to data.
- `lsqcurvefit()`: This function employs the least squares method to fit non-linear models.
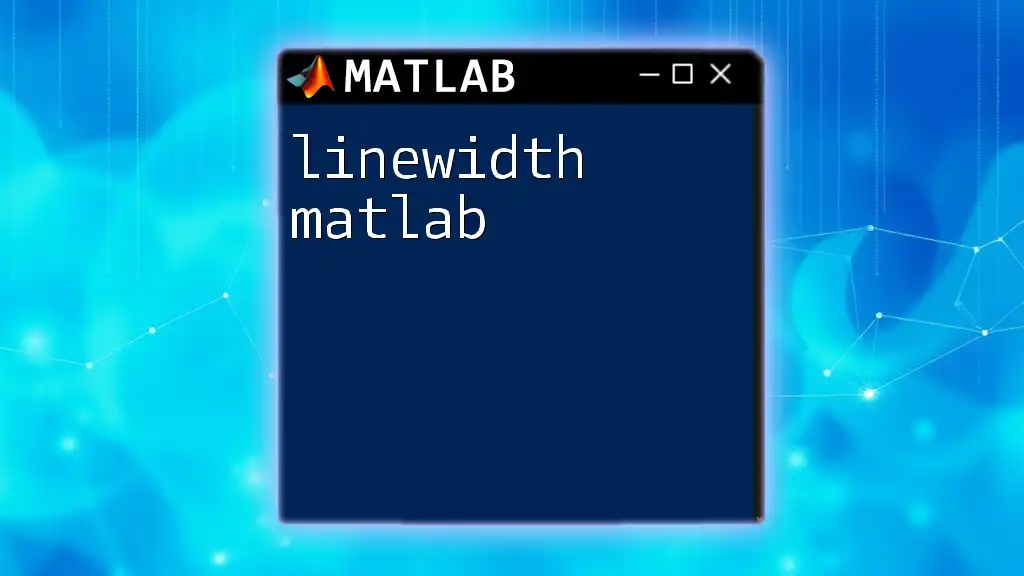
Data Preparation
Importing Data into MATLAB
The first step in any analysis is data importation. MATLAB supports various file formats like CSV, Excel, and text files. Here’s how you can import data from a CSV file:
data = readtable('data.csv'); % Importing data from a CSV file
Cleaning and Preprocessing Data
Before fitting, it's crucial to ensure your data is clean. This involves removing any missing or erroneous values that might skew your results. MATLAB has built-in functions to facilitate this:
data = rmmissing(data); % Remove missing values
Outliers can also affect your fitting models significantly. It can be beneficial to identify and optionally exclude these outliers in data preprocessing.
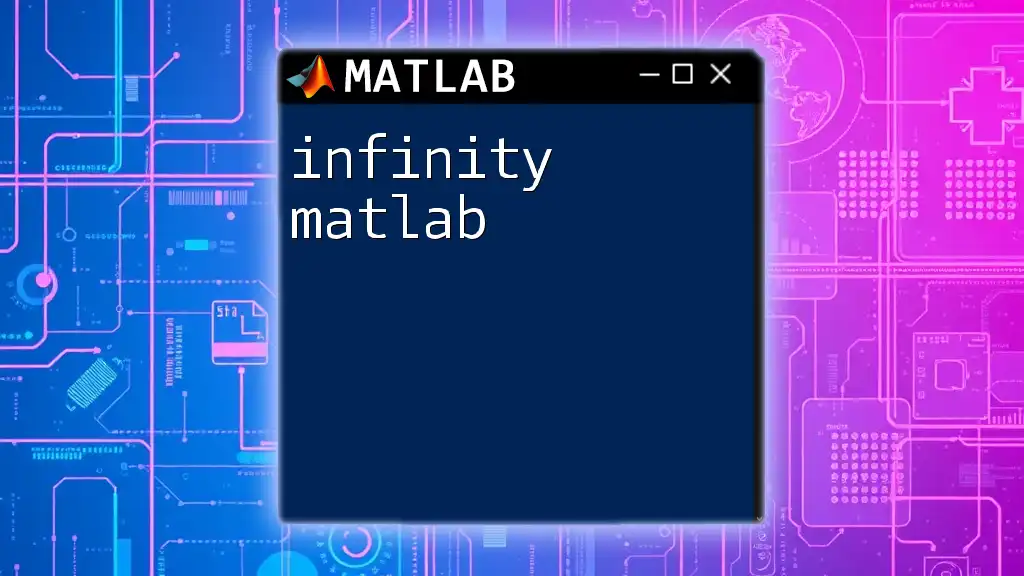
Performing Linear Fitting
Using `polyfit()`
Once your data is ready, you can start fitting. For linear data fitting, the `polyfit()` function is straightforward to use. Here’s an example:
x = data.x; % Independent variable
y = data.y; % Dependent variable
p = polyfit(x, y, 1); % Linear fit (1st degree polynomial)
In this code, the output `p` contains the coefficients of the linear fit.
Visualizing Linear Fit
Visual representation of data and the fitted line is essential for understanding how well the model fits. You can create plots using the following code:
y_fit = polyval(p, x); % Calculate fitted values
plot(x, y, 'o', x, y_fit, '-'); % Plot original data and fit
In this snippet, `y_fit` calculates the predicted values based on the linear coefficients, and the `plot()` function allows you to see both the data points and the fitted line.
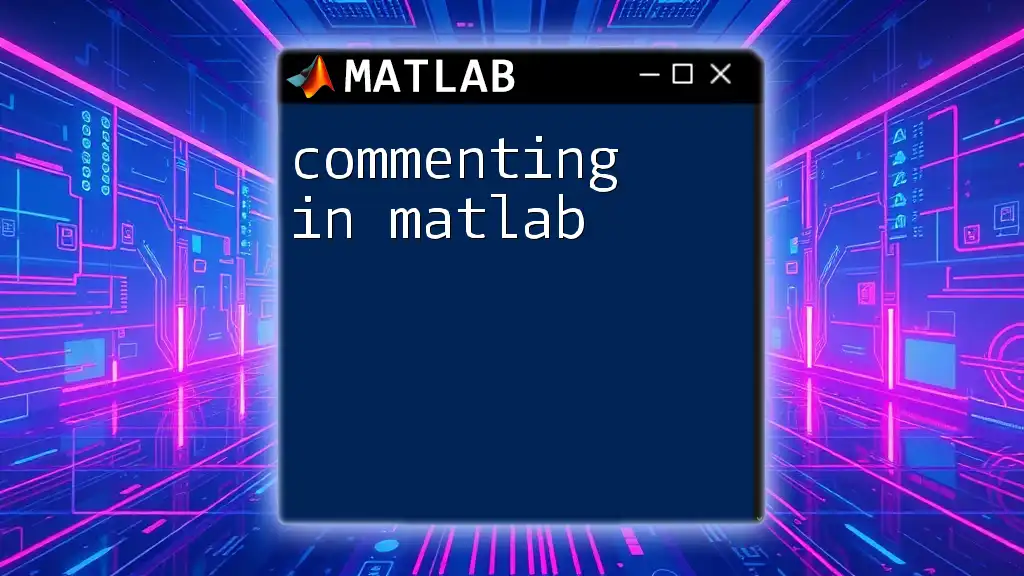
Performing Non-Linear Fitting
Using `fit()`
For more complex datasets that require non-linear fitting, the `fit()` function is powerful. Here’s how you can define and apply a non-linear fitting model:
ft = fittype('a*exp(b*x)', 'independent', 'x'); % Define the fit type
fitted_model = fit(x, y, ft, 'StartPoint', [1, 1]); % Execute fit
In this example, you define a fitting type, specifying the model (in this case, an exponential). The `StartPoint` parameter provides initial guesses for the fitting parameters.
Goodness of Fit
Once you have your fit, it’s imperative to assess its quality. The R-squared statistic is commonly used to evaluate the goodness of fit. Here's how you might calculate R-squared:
y_pred = feval(fitted_model, x); % Predicted values
R2 = 1 - (sum((y - y_pred).^2) / sum((y - mean(y)).^2)); % Calculate R-squared
A higher R-squared value (closer to 1) indicates a better fit, while values significantly below this suggest the model may not represent the data well.
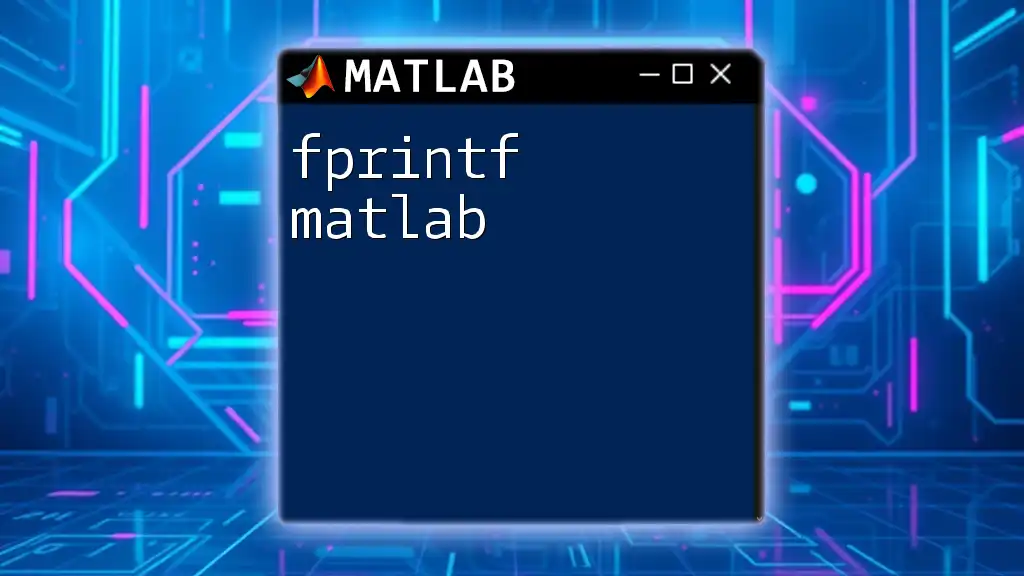
Advanced Fitting Techniques
Using `lsqcurvefit()`
For those interested in more sophisticated fitting, `lsqcurvefit()` allows you to define custom non-linear models while minimizing residuals squared. Here’s an example of how to implement this:
model_func = @(b, x) b(1) * exp(b(2) * x); % Define model
beta0 = [1, 0.1]; % Initial parameters
beta_fit = lsqcurvefit(model_func, beta0, x, y); % Fit the model
In this example, you define the model function and initial parameter estimates, allowing MATLAB to refine those parameters to best fit the data.
Handling Outliers
Outliers can distort fitting results significantly. You can utilize the `isoutlier()` function to detect these data points and decide whether to exclude or adjust them.
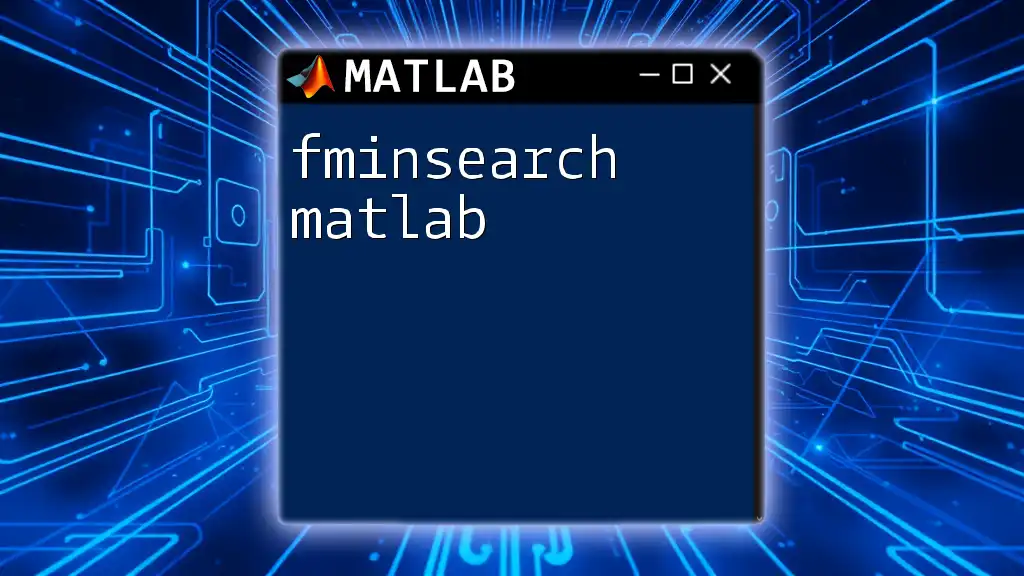
Conclusion
Fitting with MATLAB is an essential skill for data analysis across various fields. Understanding how to prepare your data, perform both linear and non-linear fitting, and assess the quality of your models empowers you to analyze data effectively. As you grow more comfortable with the provided commands and techniques, explore MATLAB's extensive documentation and resources to expand your skills further and tackle more complex datasets.
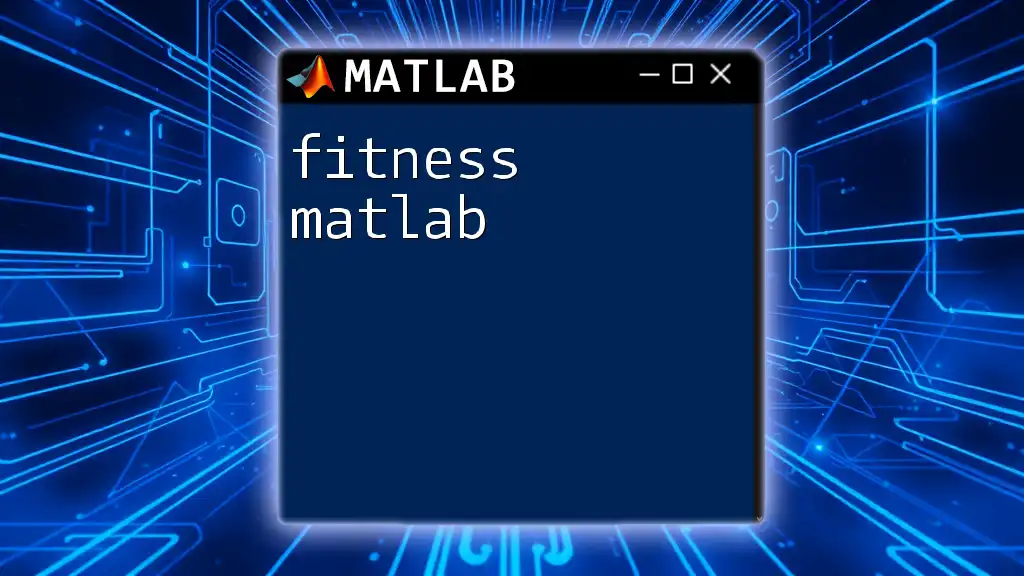
Additional Resources
For further learning, refer to the MATLAB documentation on fitting functions. Comprehensive books and online courses can deepen your understanding, while community forums provide support and networking opportunities with fellow MATLAB users.
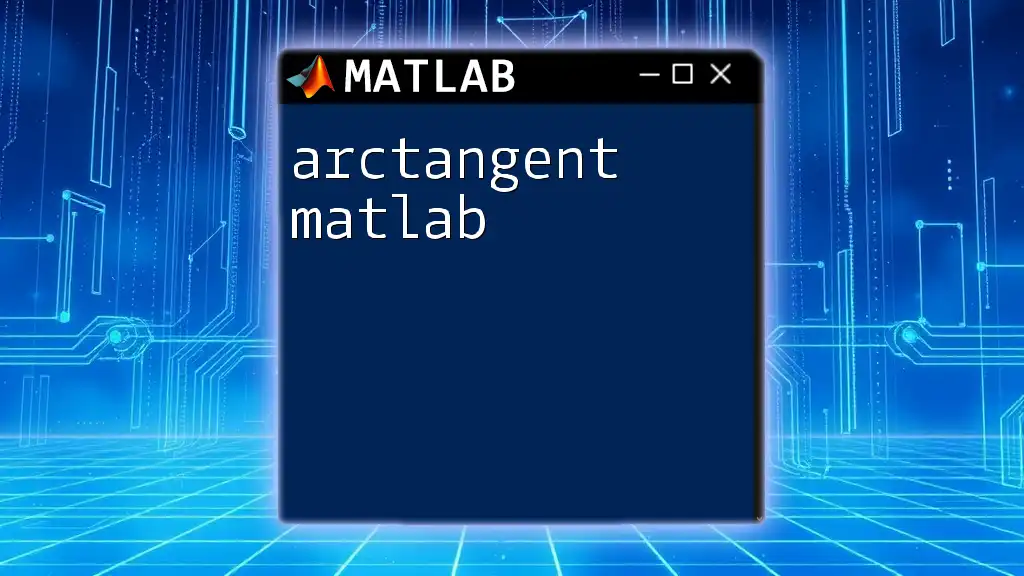
Call to Action
Consider enrolling in our courses focused on fitting with MATLAB, where you will gain hands-on experience and expert guidance in mastering these techniques. Experiment with your own datasets, and don’t hesitate to share your findings—collaboration enhances learning!