LQR (Linear-Quadratic Regulator) in MATLAB is a method used to design a controller that regulates the state of a dynamic system to minimize a cost function defined by quadratic expressions of state and control variables.
Here's a simple code snippet to demonstrate how to implement LQR in MATLAB:
% Define system matrices
A = [0 1; -1 -1];
B = [0; 1];
C = [1 0];
D = 0;
% Define weighting matrices
Q = [1 0; 0 1]; % State weights
R = 1; % Control weight
% Calculate the LQR controller gain
K = lqr(A, B, Q, R);
% Display the gain
disp('LQR Gain Matrix K:');
disp(K);
What is LQR?
The Linear Quadratic Regulator (LQR) is a crucial controller design technique in control theory. At its core, LQR is designed to operate linear systems optimally while minimizing a predefined quadratic cost function. This method is widely adopted because it provides a systematic approach to designing controllers that influence the performance and stability of dynamic systems.
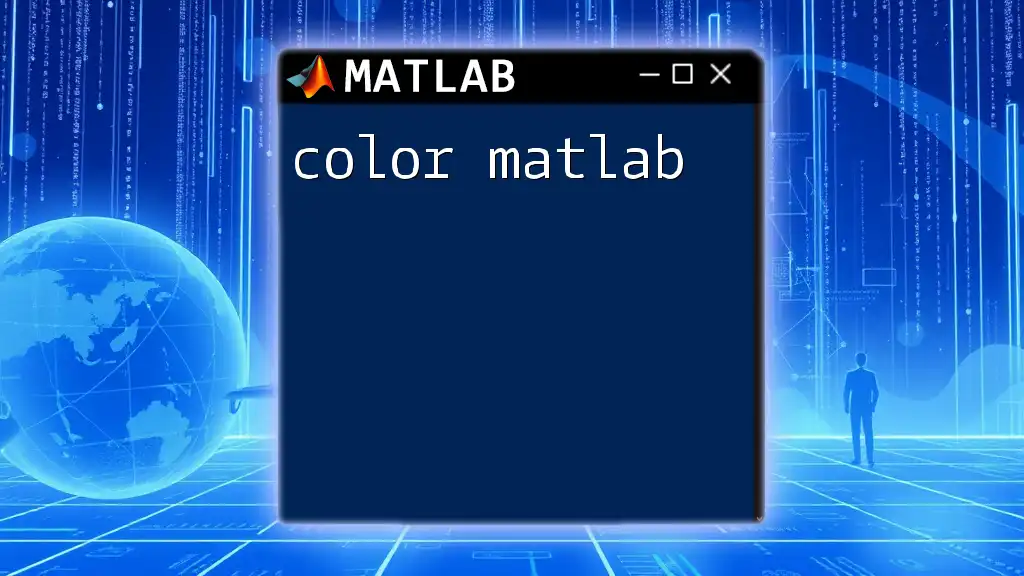
Applications of LQR
LQR has found applications in numerous fields:
- Robotics: LQR is instrumental in the mobile robots' motion planning and trajectory tracking tasks, where precise control is essential.
- Aerospace: It aids in autopilot systems for aircraft, ensuring stability and optimal path following during flights.
- Automotive Systems: LQR is applied in vehicle dynamics control, such as anti-lock braking systems and ensuring stability during maneuvering.
- Manufacturing and Process Control: In these fields, LQR is used for optimizing processes and improving the efficiency of operations.
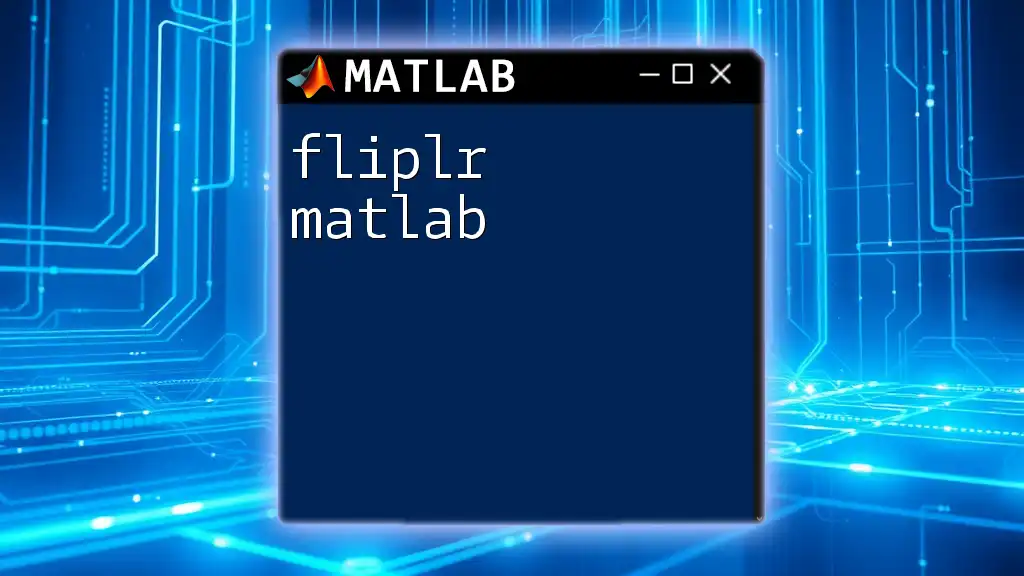
Mathematical Background
State-Space Representation
Before diving into LQR, it’s essential to understand state-space representation. A linear system can be expressed in the state-space form, represented as:
\dot{x} = Ax + Bu
where \( x \) is the state vector, \( u \) is the control input, and \( A \) and \( B \) are system matrices. Using state-space representation for LQR simplifies the controller design, making it tractable for analysis and implementation.
Quadratic Cost Function
The LQR method revolves around minimizing a quadratic cost function, denoted as \( J \):
J = \int_0^\infty (x^T Q x + u^T R u) dt
Here, \( Q \) and \( R \) are weighting matrices that dictate how much emphasis is placed on state errors versus control input efforts. Choosing these matrices wisely is critical for optimal performance.
Weighting Matrices \( Q \) and \( R \)
- Matrix \( Q \): This defines the significance of the state variables. A larger value in \( Q \) gives more weight to controlling specific states, encouraging the controller to prioritize those states.
- Matrix \( R \): This reflects the cost associated with using control inputs. Higher values in \( R \) lead to lower control effort, promoting more conservative control actions.
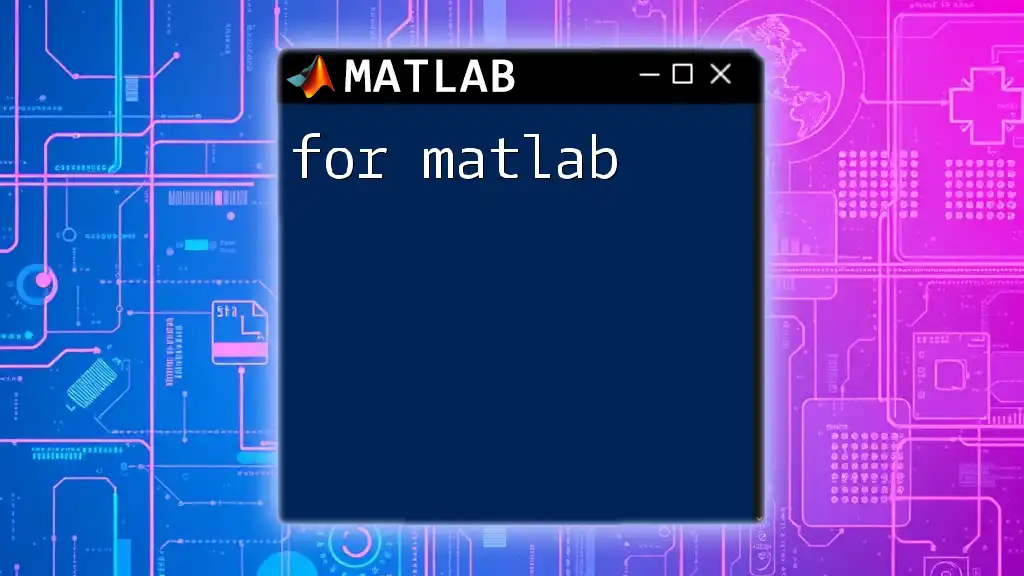
Setting Up LQR in MATLAB
MATLAB Environment Setup
Before you implement LQR in MATLAB, ensure you have the appropriate toolkits installed. The Control System Toolbox is essential for robust performance.
Basic Syntax of LQR Command
The main function for implementing LQR in MATLAB is `lqr`. Below is a simple command structure using a hypothetical system:
A = [0 1; -2 -3];
B = [0; 1];
Q = [1 0; 0 1]; % State weighting
R = 1; % Control weighting
[K, S, e] = lqr(A, B, Q, R);
Explaining Output Variables
- \( K \): The optimal feedback gain matrix generated by LQR.
- \( S \): The solution of the Riccati equation that is critical for the stability of the system.
- \( e \): Eigenvalues of the closed-loop system. These determine the stability characteristics.
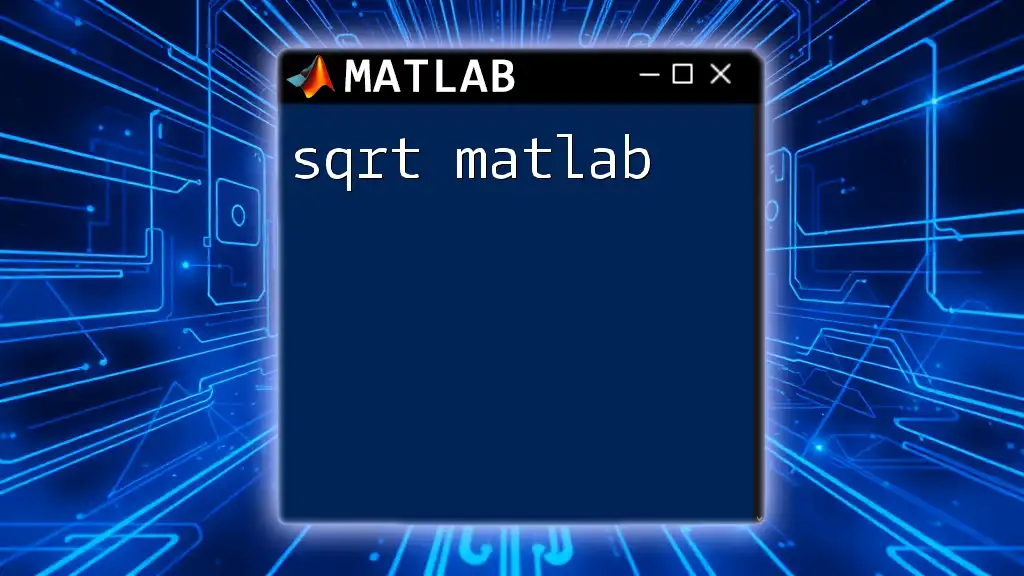
Designing an LQR Controller
Step-by-Step Approach
- Defining System Dynamics: Start with defining your \( A \) and \( B \) matrices according to the dynamics of your system.
- Choosing \( Q \) and \( R \): Select appropriate weighting matrices reflecting your control priorities.
- Implementing the LQR Controller: Use the MATLAB command provided above to compute the gains.
- Simulating the System Response: Validate the controller performance using MATLAB's built-in simulation functions.
Code Example and Explanation
Here's an example of setting up an LQR controller for a simple dynamic system:
A = [0 1; -2 -3];
B = [0; 1];
C = [1 0];
D = 0;
sys = ss(A,B,C,D);
[K, S, e] = lqr(A, B, Q, R);
% Closed-loop system
Ac = A - B*K;
sys_cl = ss(Ac, B, C, D);
In this example:
- `sys` creates a state-space model of the original system.
- Using `lqr`, we acquire the gain matrix \( K \).
- The closed-loop system matrix \( Ac \) is computed, setting the stage for further analysis.
Simulating the System Response
You can simulate the system's response using MATLAB's `lsim` or `step` functions. For example:
t = 0:0.1:10;
u = zeros(size(t)); % Assuming zero-input response
y0 = [1; 0]; % Initial conditions
[t, y] = lsim(sys_cl, u, t, y0);
plot(t, y);
title('LQR Controlled System Response');
xlabel('Time (s)');
ylabel('Response');
This code generates a time response plot showing how the system behaves under LQR control.
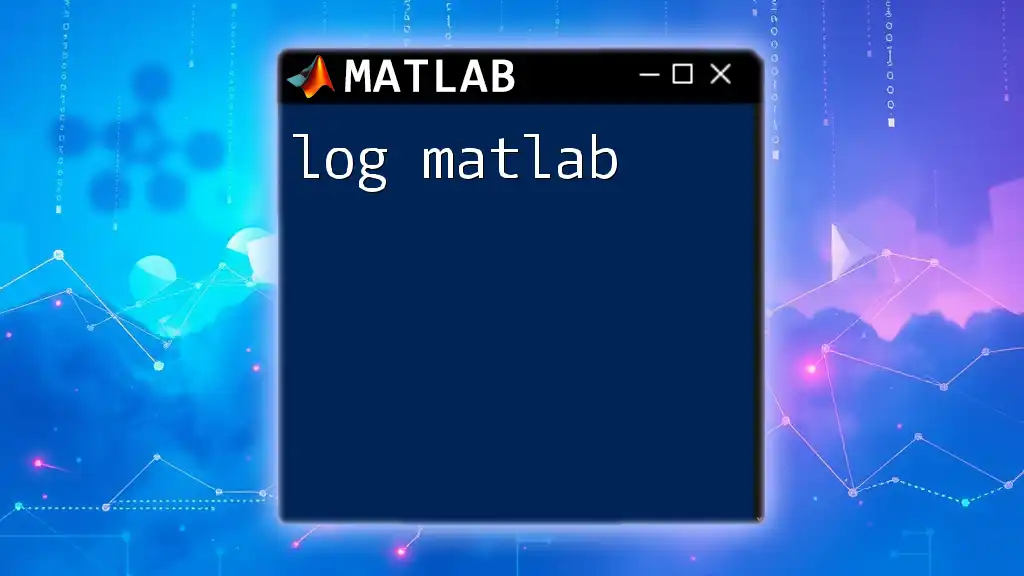
Tuning LQR Parameters
Adjusting Weighting Matrices
Tuning your \( Q \) and \( R \) matrices is a subtle art. A systematic approach should be adopted:
- Start with Initial Guesses: Choose values based on the importance of states and input effort that suits your system.
- Run Simulations: Evaluate the system's response and iteratively adjust these values.
- Observe Performance Metrics: Analyze response speed, overshoot, and settling time, adjusting \( Q \) for performance and \( R \) to limit control action.
Discussion on Trade-offs
It’s pivotal to understand the trade-offs. Increasing weights in \( Q \) can enhance the performance speed, but at the cost of potentially leading to overshoot or instability. Conversely, boosting \( R \) results in smoother control but may slow down the response, creating a balance that must be achieved based on system requirements.
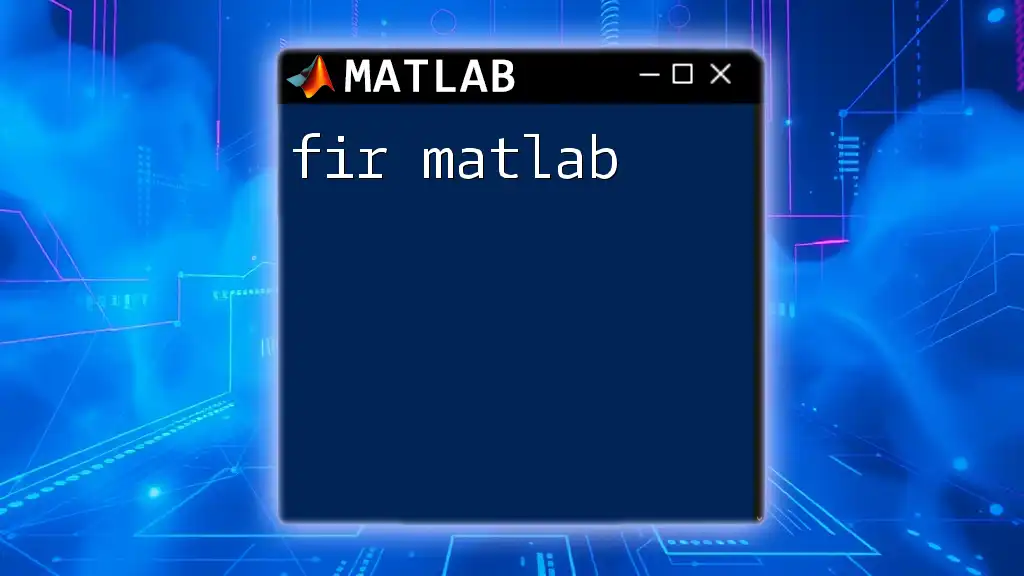
Advanced Topics in LQR
Full-State Feedback vs. Output Feedback
LQR mainly focuses on full-state feedback, but it’s essential to recognize that in some scenarios where not all states are measurable, output feedback is necessary. Modifications may include observer-based designs.
LQR for Nonlinear Systems
For nonlinear systems, LQR can still be utilized through linearization approaches or gain scheduling techniques, enhancing performance in operating regions.
Time-Varying LQR
When dealing with systems where dynamics change over time, time-varying LQR can be implemented. Advanced design can accommodate system changes dynamically, ensuring optimal performance amidst variability.
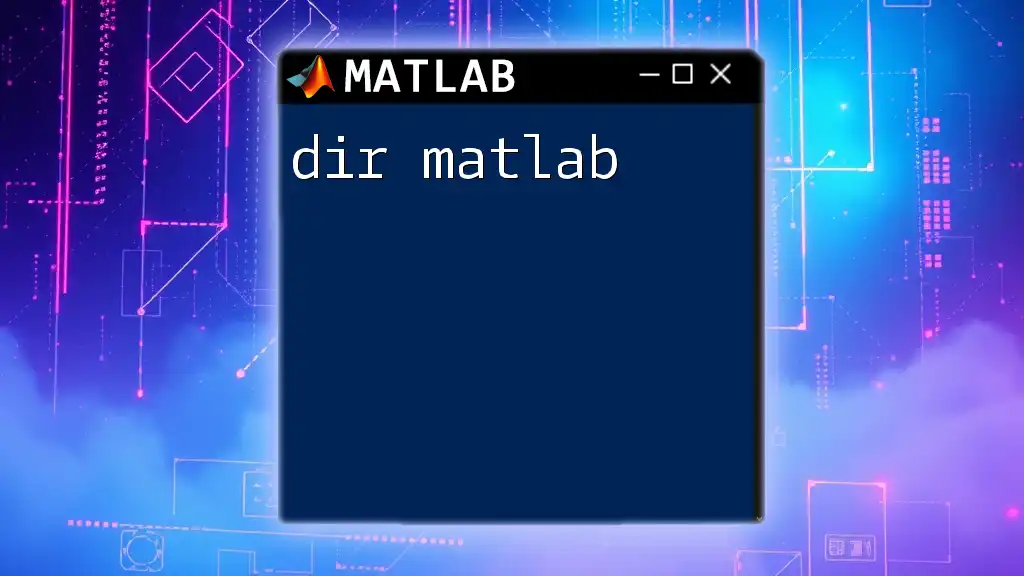
Visualizing LQR Controller Performance
Control System Analysis
Performance analysis becomes paramount. Use MATLAB to evaluate system behavior with frequency response tools such as Bode plots, Nyquist plots, and root locus to shine a light on stability margins, bandwidth, and performance richness.
MATLAB Visualization Tools
Utilizing the following commands can provide insight into your system’s control characteristics:
bode(sys_cl); % Frequency response analysis
nyquist(sys_cl); % Stability analysis
rlocus(sys); % Root locus plot for understanding poles
These visualizations help to identify how the controller behaves in various frequency ranges and to assess stability during system operation.
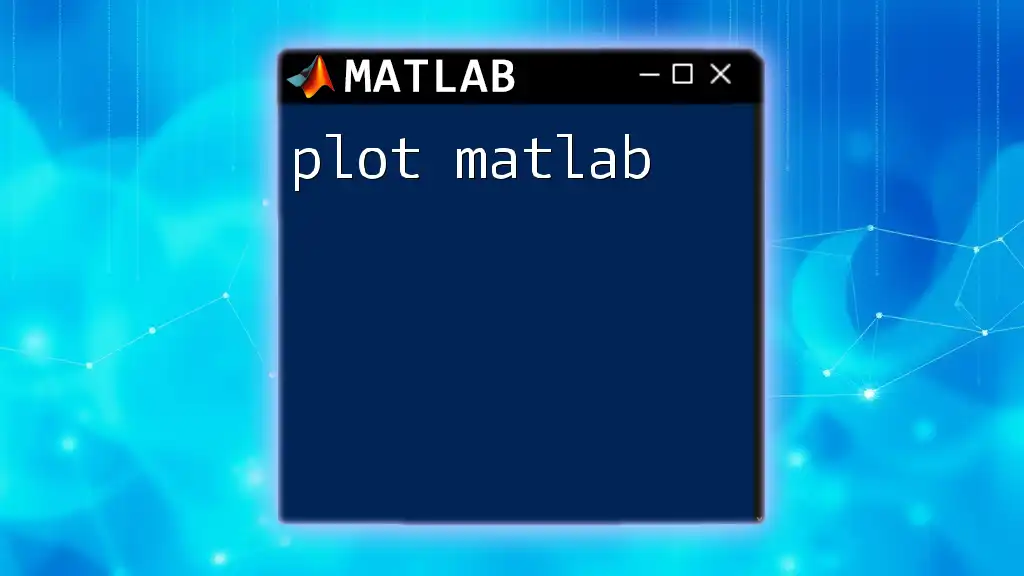
Conclusion
In summary, LQR in MATLAB is an invaluable process for developing effective control systems. Familiarity with the underlying mathematical principles, coupled with practical implementation strategies, forms the backbone of success when applying LQR methodologies. Experimentation with various parameters will deepen your understanding and refine your skills. As the field evolves, continuously seeking resources and studying advanced applications will expand your capabilities in this domain.
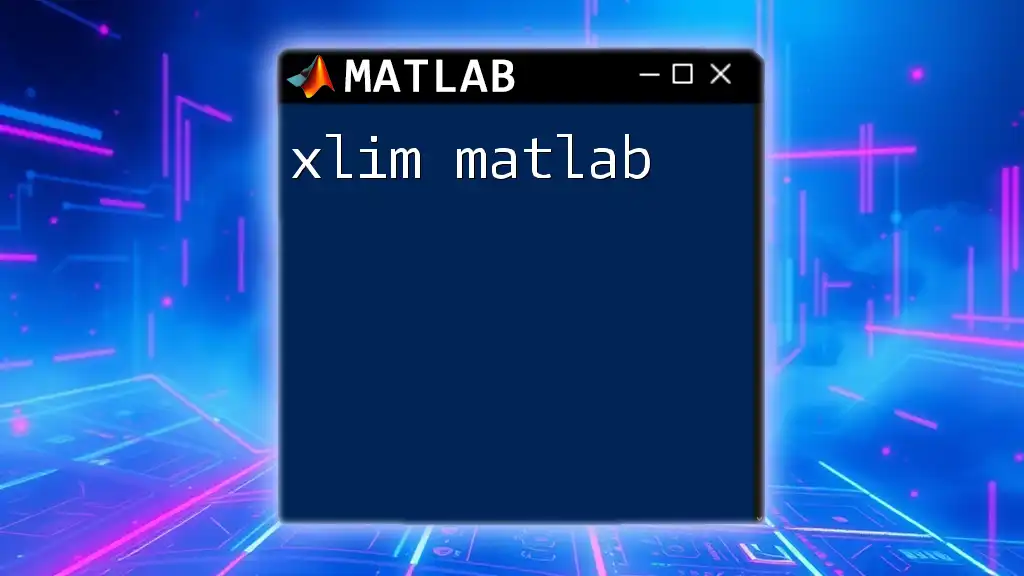
FAQs about LQR in MATLAB
- What are the typical pitfalls when using LQR?: Common mistakes include improper selection of \( Q \) and \( R \) matrices, which can lead to suboptimal performance or instability.
- How does LQR compare to PID controllers?: LQR is generally more effective for multi-variable systems and provides optimal control, whereas PID is better suited for simpler or less dynamic applications.
- When to prefer LQR over other control strategies?: Choosing LQR is advantageous when system dynamics can be accurately described by linear models, or when performance optimization in a multi-variable context is critical.