In MATLAB, imaginary numbers can be represented using the imaginary unit `i` or `j`, allowing users to perform complex calculations effortlessly. Here’s a simple example of creating a complex number and performing operations with it:
% Create a complex number and perform operations
z = 3 + 4i; % Define a complex number (3 + 4i)
magnitude = abs(z); % Compute the magnitude
realPart = real(z); % Extract the real part
imaginaryPart = imag(z); % Extract the imaginary part
% Display results
disp(['Magnitude: ', num2str(magnitude)]);
disp(['Real Part: ', num2str(realPart)]);
disp(['Imaginary Part: ', num2str(imaginaryPart)]);
Understanding Imaginary Numbers
Definition of Imaginary Numbers
Imaginary numbers are a critical concept in mathematics, defined by the use of the imaginary unit \( i \), where \( i^2 = -1 \). In this context, a complex number can be expressed in the form \( a + bi \), where \( a \) is the real part and \( b \) is the imaginary part. Understanding how to manipulate and apply these numbers is fundamental for many areas, including engineering, physics, and even computer science.
Visual Representation
Imaginary numbers can be visualized on a complex plane, where the horizontal axis represents real numbers and the vertical axis represents imaginary numbers. This visual perspective allows for a better understanding of how complex numbers function and interact. In MATLAB, plotting these numbers can help illustrate their relationships effectively.
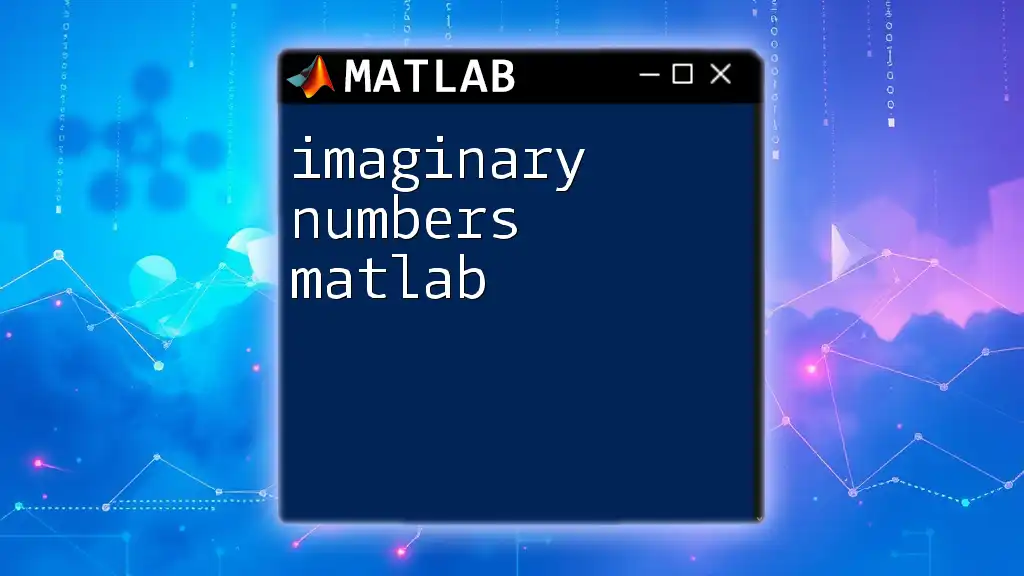
MATLAB Basics with Imaginary Numbers
Creating Imaginary Numbers
In MATLAB, creating imaginary numbers is straightforward. You can define them using either the `i` or `j` notation. For example, to create a simple imaginary number:
z1 = 3 + 4i;
Alternatively, MATLAB also provides the `complex` function, which allows you to create a complex number by specifying the real and imaginary parts separately:
z2 = complex(3, 4); % Real = 3, Imaginary = 4
Both methods are valid, and the choice depends on your code readability and personal preference.
Basic Operations with Imaginary Numbers
Once you've created imaginary numbers, you'll often need to perform operations such as addition, subtraction, multiplication, and division.
Addition and Subtraction
To add or subtract complex numbers in MATLAB, you can use the standard arithmetic operators. For example:
z3 = z1 + z2; % Addition
z4 = z1 - z2; % Subtraction
These operations yield complex results while maintaining the real and imaginary parts distinctly.
Multiplication and Division
Multiplication and division of complex numbers can also be performed using standard operators. For instance:
z5 = z1 * z2; % Multiplication
z6 = z1 / z2; % Division
MATLAB handles the necessary calculations behind the scenes, allowing you to work seamlessly with both the real and imaginary components.
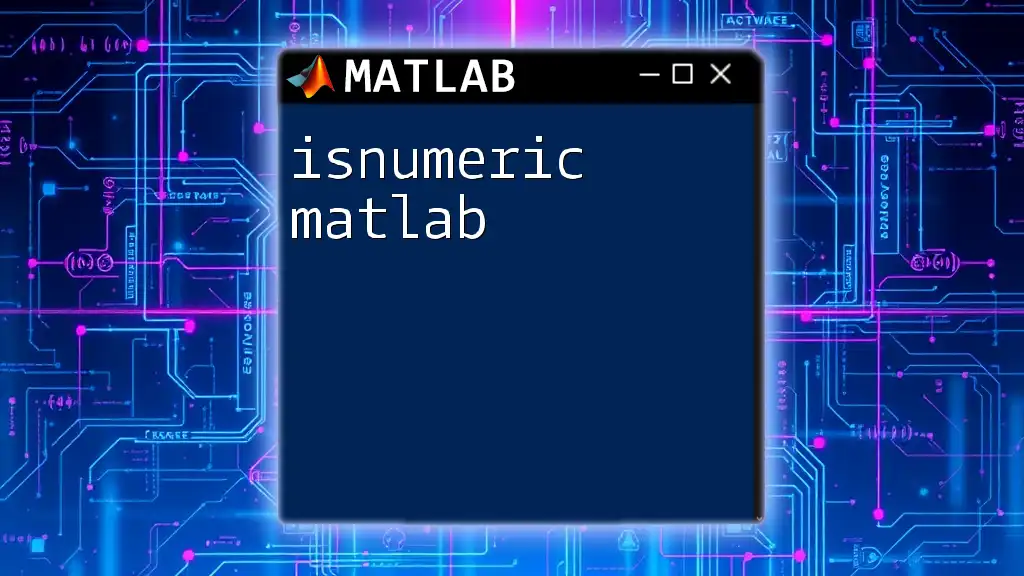
Advanced Operations with Imaginary Numbers
Magnitude and Angle
Understanding the magnitude and angle of complex numbers is vital in various applications. The magnitude (or modulus) measures the distance of the complex number from the origin in the complex plane.
To calculate the magnitude of a complex number in MATLAB, you can use the `abs` function:
magnitude = abs(z1);
The phase angle (or argument) indicates the direction of the complex number relative to the positive real axis. To find the phase angle, you can use the `angle` function:
angle = angle(z1);
Complex Conjugates
The complex conjugate of a complex number is obtained by changing the sign of its imaginary part. This concept is crucial in various calculations, especially in simplifying expressions.
In MATLAB, you can easily obtain the complex conjugate using the `conj` function:
z_conj = conj(z1);
This operation returns the conjugate of the complex number, which is essential in certain mathematical analyses.
Exponentiation of Complex Numbers
Euler’s formula relates complex exponentials to trigonometric functions. The formula states that \( e^{ix} = \cos(x) + i\sin(x) \). This relation is pivotal for applications in electrical engineering and physics.
You can explore this concept in MATLAB through exponentiation. Here’s an example:
z_exp = exp(1i * pi); % Returns -1
This operation demonstrates Euler’s identity and how it can be practically applied within MATLAB.
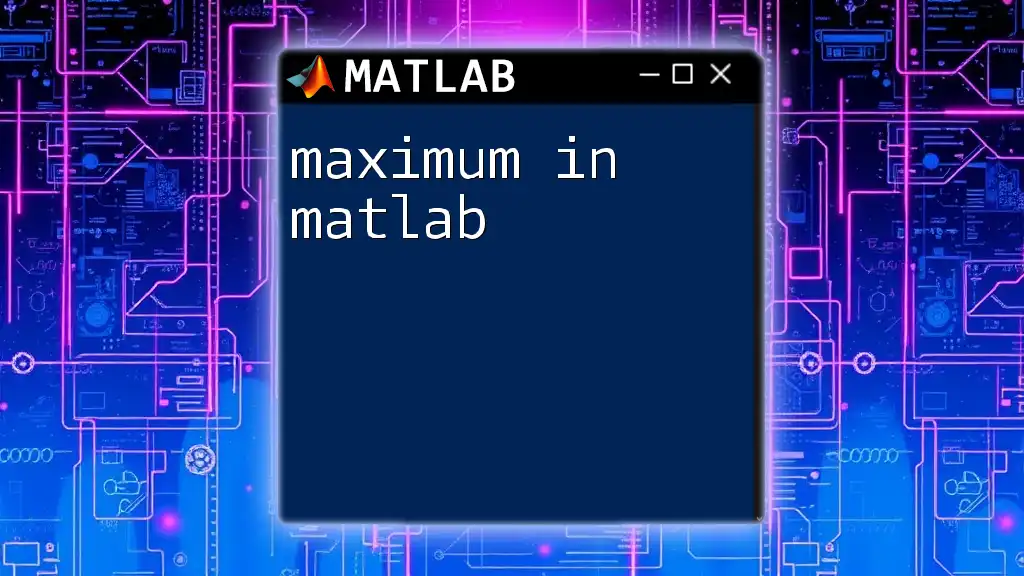
Practical Applications of Imaginary Numbers in MATLAB
Engineering Applications
Imaginary numbers are extensively used in signal processing. They allow engineers to analyze signals that vary sinusoidally in time. Complex numbers facilitate easier manipulation of these signals, making calculations like Fourier transforms more intuitive.
In control systems, imaginary numbers help analyze system stability through poles and zeros in the complex plane. Techniques such as Bode plots heavily rely on complex-number analysis to represent system behavior effectively.
Physics Applications
In quantum mechanics, imaginary numbers play a pivotal role. They are often used to represent quantum states, where the complex probability amplitudes describe potential outcomes. Handling these states necessitates a solid understanding of imaginary numbers in MATLAB for simulations and calculations.
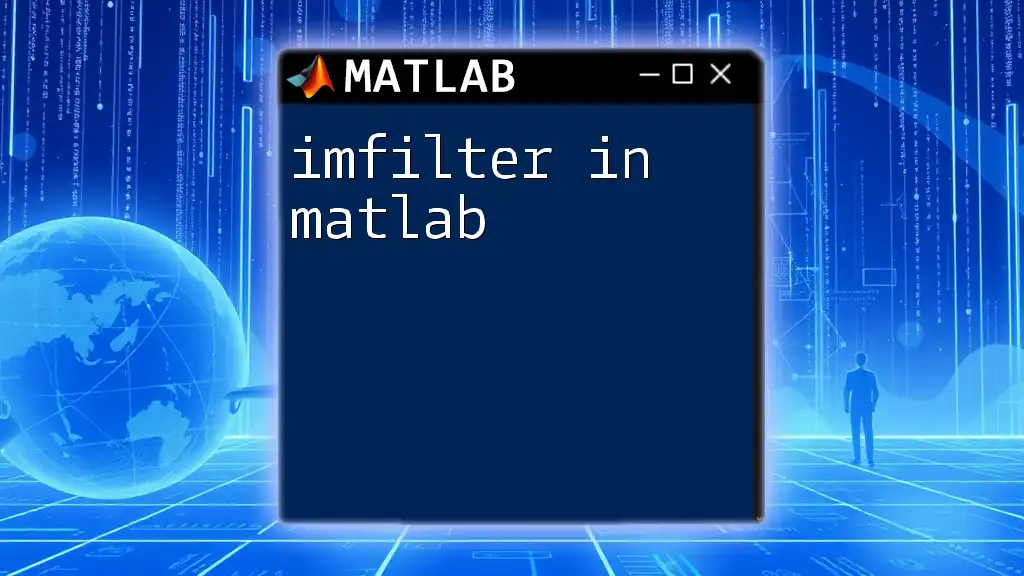
Troubleshooting Common Issues
Common Errors with Imaginary Numbers
When working with imaginary numbers in MATLAB, one common error is attempting to perform operations without recognizing that the imaginary part must be treated as a component of a whole. For example, using a single variable where parts are mixed can lead to confusion in results.
Tips for Effective Use
To write efficient MATLAB code for handling imaginary numbers, always ensure clarity in defining your complex variables. Consider using clear variable names and comments to describe what each part represents. Using functions like `abs()`, `angle()`, and `conj()` can simplify your workflow when analyzing complex data.
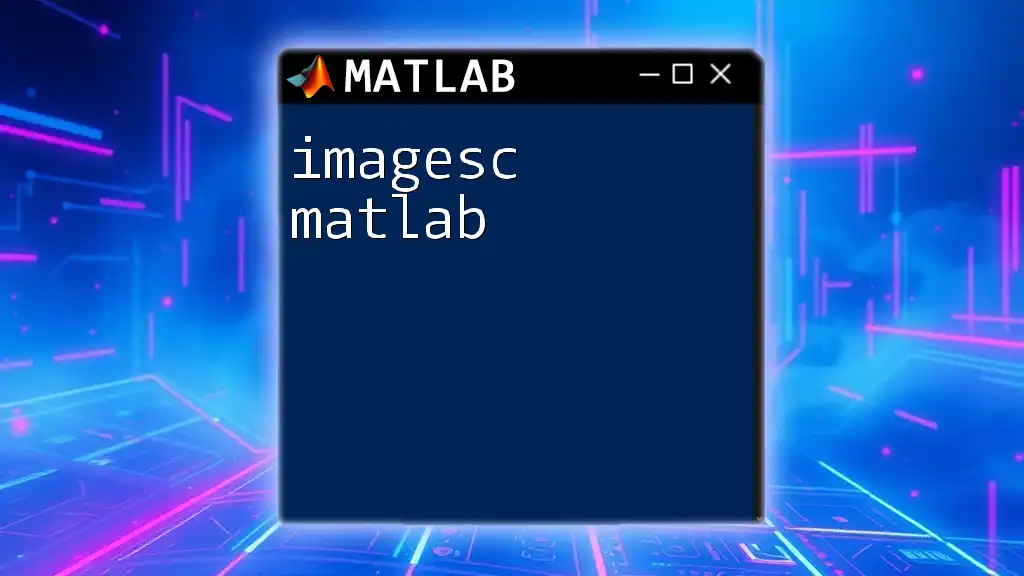
Conclusion
Imaginary numbers are not only mathematically intriguing but are also indispensable tools in practical applications across various fields. MATLAB, with its intuitive syntax and robust functions for handling complex numbers, makes it an excellent environment for both learning and applying concepts related to imaginary numbers. By practicing the operations discussed and exploring additional MATLAB resources, you can master this critical mathematical concept.
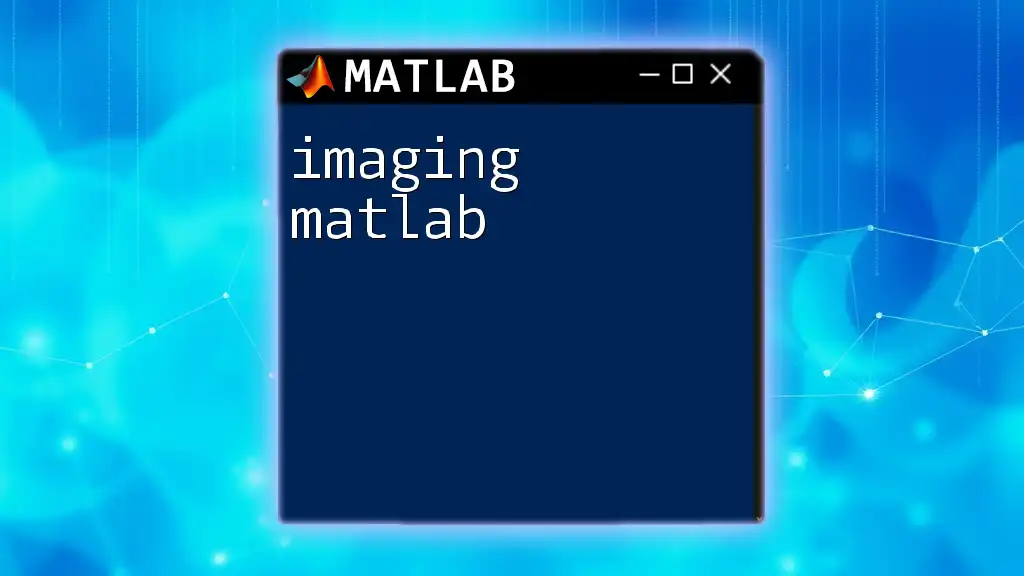
Additional Resources
To further your understanding of imaginary numbers in MATLAB, consider exploring online tutorials, textbooks, and community forums. Engaging with others who are also learning can significantly enhance your grasp of the material and provide additional insights into complex number applications.