Linear regression in MATLAB is a statistical method used to model the relationship between a dependent variable and one or more independent variables using the least squares technique. Here's a simple code snippet to perform linear regression:
% Example data
x = [1 2 3 4 5]'; % Independent variable
y = [2.2 2.8 3.6 4.5 5.1]'; % Dependent variable
% Perform linear regression
mdl = fitlm(x, y);
% Display the results
disp(mdl);
Understanding Linear Regression
Definition of Linear Regression
Linear regression is a statistical method that models the relationship between a dependent variable and one or more independent variables. In simple terms, it helps us understand how the dependent variable changes as we alter the independent variables. The basic equation for a linear model can be expressed as:
\[ Y = b_0 + b_1X + \epsilon \]
Where:
- Y is the dependent variable.
- X is the independent variable.
- b0 is the intercept of the line.
- b1 is the slope of the line.
- ε is the error term.
Types of Linear Regression
Simple Linear Regression
In simple linear regression, we have only one independent variable. This method is particularly useful when we want to predict a single outcome based on a single factor. For instance, if we want to predict sales based on advertising spend, we can utilize this method efficiently by fitting a straight line to the observed data.
A real-world example might include the relationship between study hours and exam scores. As one increases, the other tends to increase as well.
Multiple Linear Regression
Multiple linear regression, on the other hand, involves two or more independent variables. This approach is used when a single predictor isn't sufficient to explain the variations in the dependent variable. For example, predicting house prices might require multiple factors, like location, size, and age as independent variables.
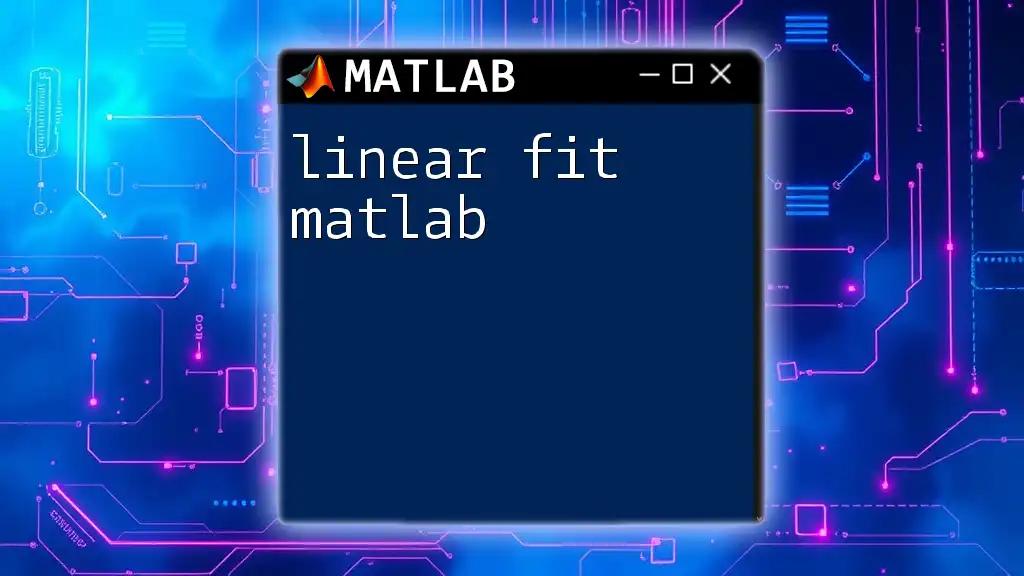
Getting Started with MATLAB
Setting Up MATLAB for Linear Regression
Before diving into linear regression techniques in MATLAB, ensure MATLAB is properly installed on your system. Visit the MathWorks website to download and install the latest version if you haven't already. Familiarize yourself with the MATLAB interface and ensure access to relevant toolboxes like Statistics and Machine Learning Toolbox.
Useful MATLAB Commands for Linear Regression
MATLAB offers several commands that simplify the linear regression process:
- polyfit(): Useful for polynomial least squares fitting.
- regress(): A straightforward function for multiple regression analysis.
- fit(): Provides robust options for fitting various types of models.
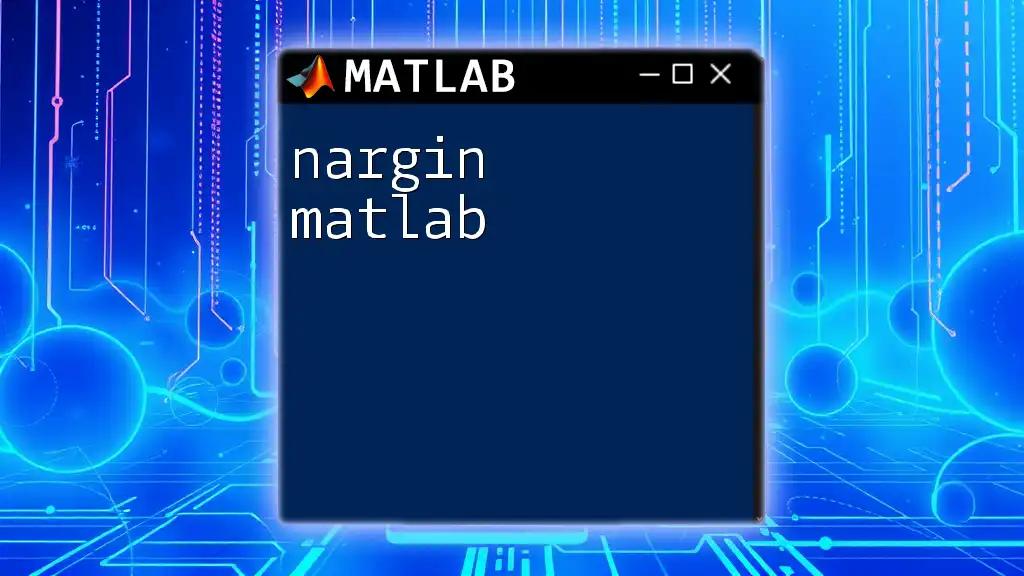
Performing Simple Linear Regression in MATLAB
Step-by-Step Guide
To demonstrate simple linear regression, let’s create a synthetic dataset.
% Example Data
X = [1; 2; 3; 4; 5]; % Independent variable
Y = [2.2; 2.8; 3.6; 4.5; 5.1]; % Dependent variable
Fitting the Model
With the data ready, we can fit the simple linear regression model using `polyfit()`:
% Performing Simple Linear Regression
p = polyfit(X, Y, 1);
The `p` vector will contain the coefficients of the linear polynomial, where `p(1)` is the slope and `p(2)` is the intercept of the fitted line.
Plotting the Results
Visualizing the fitted model is crucial to understand the relationship between variables. Here's how to plot the original data and the regression line:
% Plot
plot(X, Y, 'o'); % Original data points
hold on;
x_fit = linspace(1, 5, 100);
y_fit = polyval(p, x_fit);
plot(x_fit, y_fit, '-r'); % Fitted line
hold off;
This produces a scatter plot of the original data points with a red line representing the fitted model.
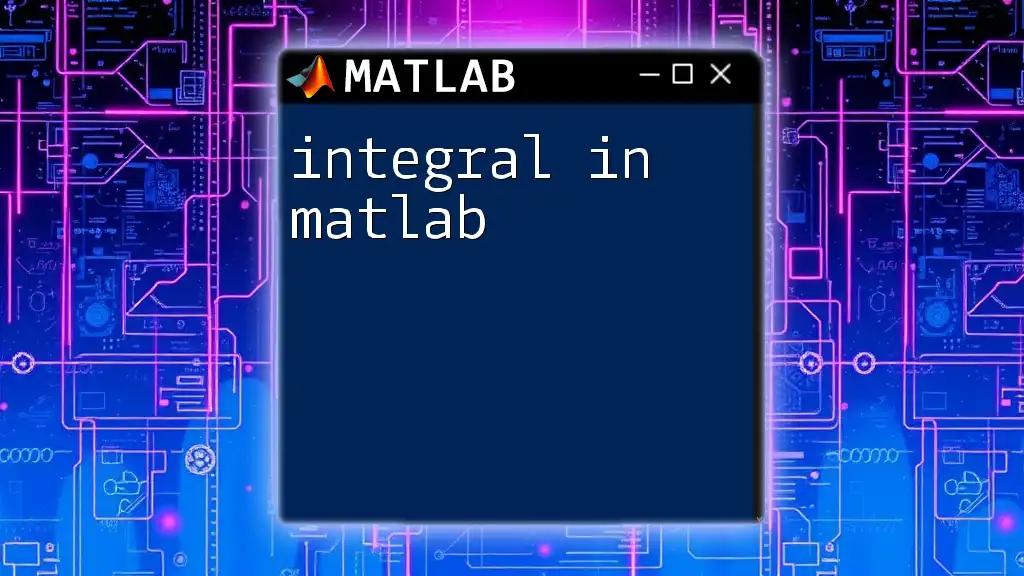
Performing Multiple Linear Regression in MATLAB
Understanding the Data Format
For multiple linear regression, the dataset should be structured appropriately, where X contains multiple columns representing various independent variables.
Step-by-Step Guide for Multiple Linear Regression
Let’s create a synthetic dataset with two independent variables:
% Example Data
X = [1, 1; 2, 2; 3, 3; 4, 4; 5, 5]; % Multiple independent variables
Y = [2.2; 2.8; 3.6; 4.5; 5.1]; % Dependent variable
Fitting the Model with `regress()`
We can now fit the multiple linear regression model using the `regress()` function:
% Performing Multiple Linear Regression
b = regress(Y, [ones(size(X, 1), 1), X]); % Including the intercept
In this code, we first prepend a column of ones to the independent variables to account for the intercept term.
Interpreting the Results
After fitting the model, the `b` vector will contain the coefficients for all independent variables, providing insights into how each variable affects the dependent variable.
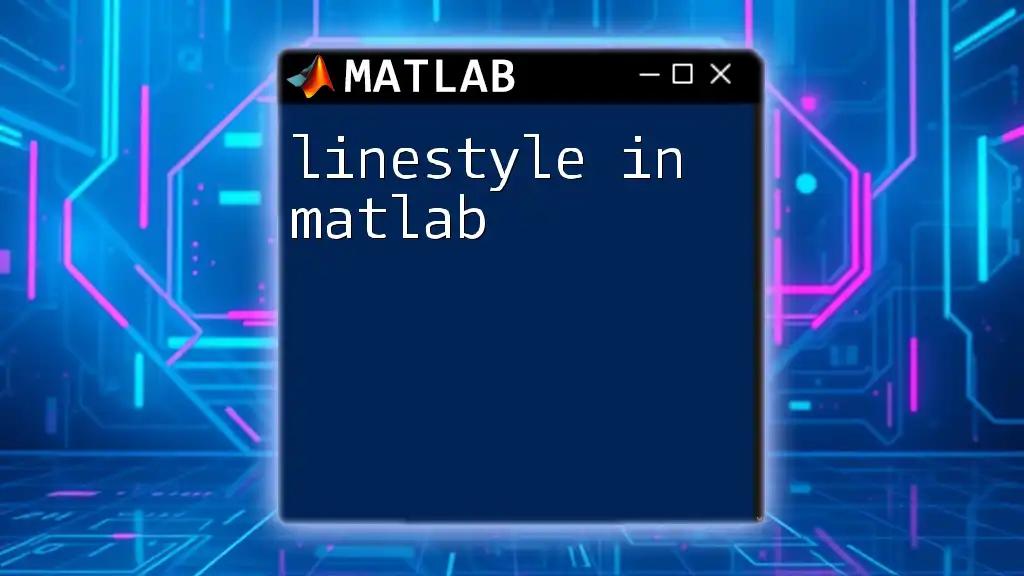
Evaluating the Model
Assessing the Goodness of Fit
To evaluate how well your model fits the data, you can calculate the R-squared value. A higher R-squared indicates a better fit. You can also use MATLAB’s `fitlm()` function for a detailed analysis of your regression model.
% Fit and Analyze
mdl = fitlm(X, Y);
This command will return a linear model object `mdl`, allowing you to inspect statistics, residual plots, and more.
Residual Analysis
Residuals can reveal patterns not captured by the model. Plotting these residuals can help identify issues like non-linearity or heteroscedasticity.
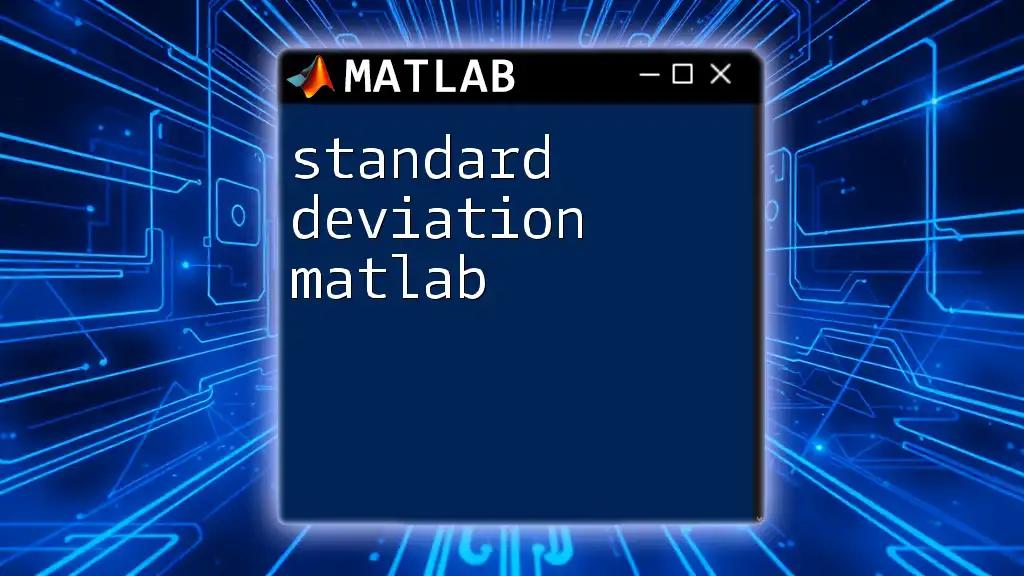
Advanced Techniques in Linear Regression
Polynomial Regression
To model non-linear relationships, you can perform polynomial regression using `polyfit()` with higher-degree polynomials. For example, to fit a quadratic model, use:
% Polynomial Regression
p = polyfit(X, Y, 2); % Quadratic fit
This allows for curved relationships rather than just straight lines.
Regularization Techniques
Regularization techniques like Lasso and Ridge regression are valuable for handling multicollinearity and improving model performance. MATLAB offers the `lasso()` and `ridge()` functions, which can be used to implement these techniques effectively.
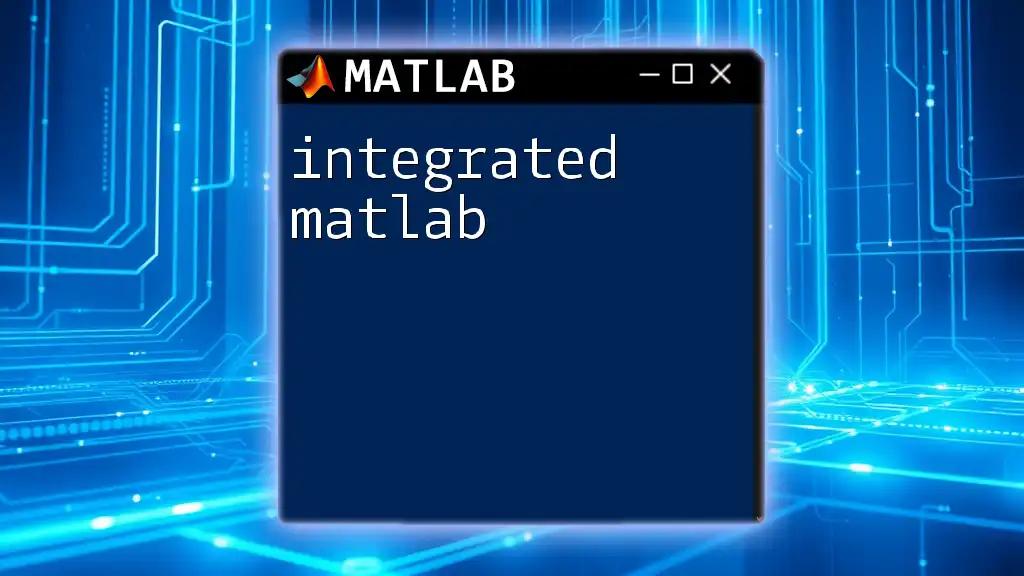
Conclusion
In summary, linear regression is a powerful statistical technique used for modeling relationships between variables and predicting outcomes. In MATLAB, you can easily implement simple and multiple linear regression using various commands and functions, allowing you to analyze, visualize, and evaluate your models effectively.
By understanding both types of linear regression and their implementation in MATLAB, you're well on your way to mastering data analysis techniques that are impactful in numerous real-world applications. Continue your learning journey to enhance your MATLAB skills and explore advanced topics in regression analysis.