A line plot in MATLAB is used to visualize data points connected by straight lines, and can be easily created using the `plot` function.
Here’s a simple code snippet to create a line plot:
x = 0:0.1:10; % Generate x values from 0 to 10 with 0.1 intervals
y = sin(x); % Compute the sine of each x value
plot(x, y); % Create a line plot of y versus x
title('Sine Wave'); % Add a title to the plot
xlabel('X-axis'); % Label for the x-axis
ylabel('Y-axis'); % Label for the y-axis
grid on; % Enable grid for better readability
Understanding Line Plots
What is a Line Plot?
A line plot is a type of graph that displays information as a series of data points connected by straight line segments. It is a fundamental visualization technique used in data analysis, especially to represent trends and relationships between two continuous variables. Line plots are ideal for showing changes over time or any other ordered sequence.
Why Use Line Plots?
Line plots are favored for their simplicity and clarity. They can effectively showcase trends, fluctuations, and patterns. Unlike scatter plots, which emphasize individual data points, line plots highlight the connection between them, making it easier to observe overall trends. This is particularly useful in fields like finance, engineering, and scientific research where time series data is common.
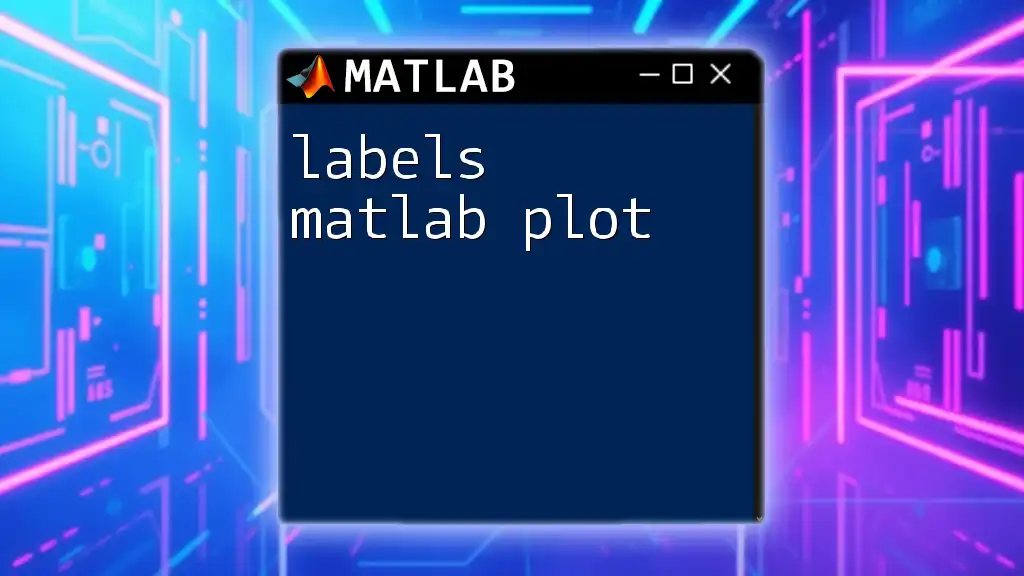
Getting Started with MATLAB Line Plots
Basic Syntax for Line Plot
To create a line plot in MATLAB, you utilize the `plot` function. The syntax is straightforward, typically written as `plot(X, Y)`, where `X` contains your x-coordinates and `Y` contains the corresponding y-coordinates.
Example: Creating Your First Line Plot
To illustrate, let's plot a simple sine wave. The following code creates an array of x values, computes the sine for each x, and generates the plot:
x = 0:0.1:10; % Create an array of x values
y = sin(x); % Calculate y values based on a sine function
plot(x, y); % Create the line plot
title('Sine Wave'); % Add a title
xlabel('X-axis'); % Label the x-axis
ylabel('Y-axis'); % Label the y-axis
Upon executing this code, you will see a continuous wave pattern, which is representative of the variable changes over the specified range.
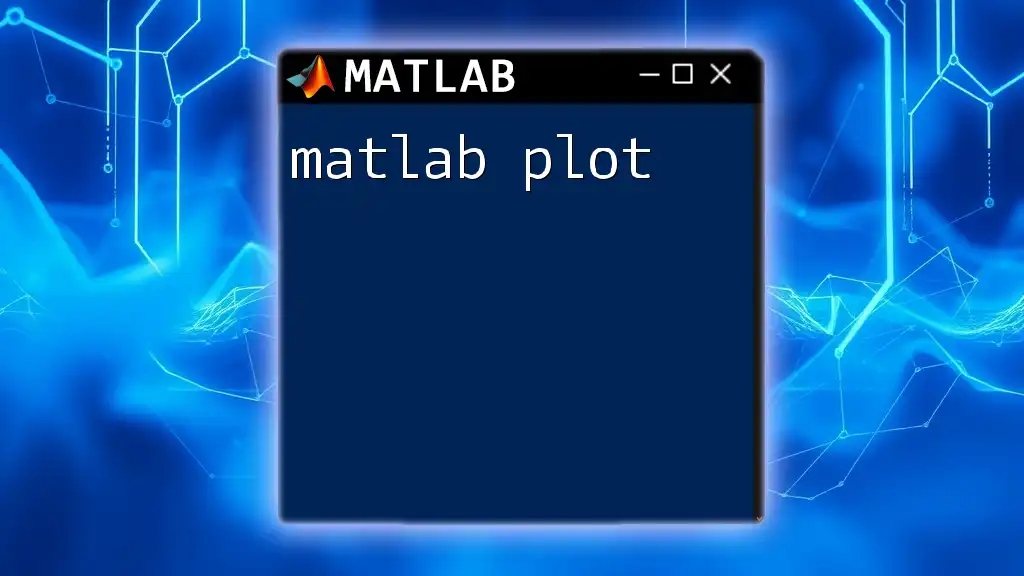
Customizing Line Plots
Title and Axis Labels
Proper labeling of your plot is crucial. The `title`, `xlabel`, and `ylabel` functions enable you to add descriptive headings and axis markers, enhancing readability and understanding of the data being presented. Good practices include avoiding overly technical jargon in titles and making them descriptive yet concise.
Line Styles and Colors
MATLAB allows you to customize the appearance of your lines using various styles and colors. Different line styles such as solid, dashed, or dotted can provide visual cues about the nature of the data. For example, using a red dashed line can differentiate a specified dataset.
plot(x, y, 'r--'); % Red dashed line
Marker Styles
In addition to line styles, markers can greatly improve the visibility of data points in your line plot. You can add different markers (like circles, squares, or stars) to the line plot to indicate specific points of interest.
plot(x, y, 'o'); % Circle markers at each data point
Example: Customizing Your Plot
Here’s how to create a more visually enriched plot with custom styles and markers:
plot(x, y, 'g-.o'); % Green dash-dot line with circle markers
title('Customized Sine Wave');
xlabel('X values');
ylabel('Sine values');
grid on; % Add a grid for better readability
This code snippet not only plots the sine wave but also provides it a distinct style, enhancing its clarity.
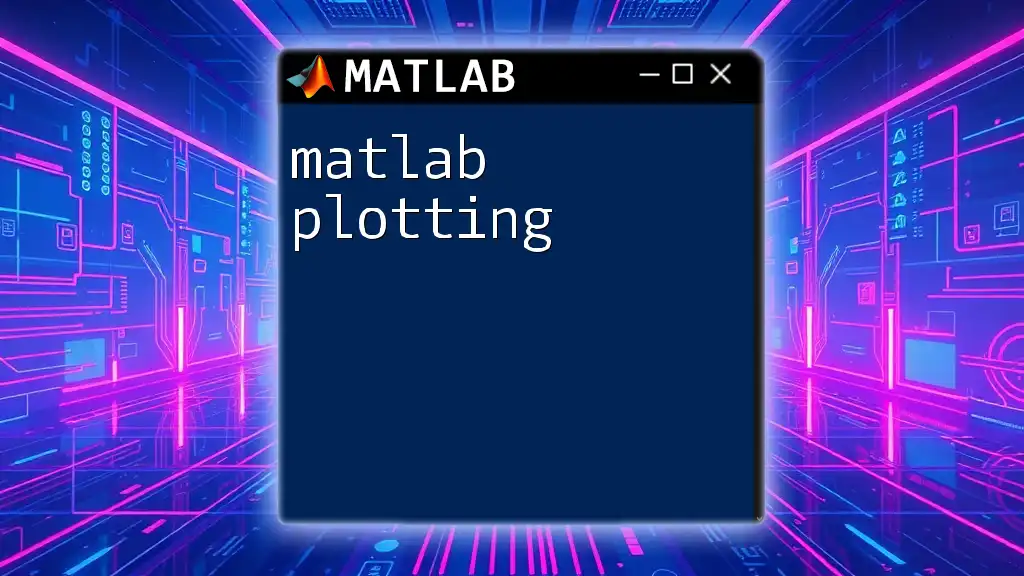
Advanced Features of MATLAB Line Plots
Multiple Line Plots on Same Graph
Often, it’s useful to compare multiple datasets within the same figure. MATLAB makes this easy by using the `hold on` command, which allows you to overlay multiple plots.
y2 = cos(x);
plot(x, y, 'r--'); % Plot sine
hold on; % Hold the current plot
plot(x, y2, 'b-'); % Plot cosine
hold off; % Release the hold
In this code, both sine and cosine functions are plotted on the same graph, helping to visualize their relationship simultaneously.
Legends and Annotations
Legends are vital for explaining what each line on the graph represents, especially when multiple datasets are present. You can easily add these using the `legend` function. Additionally, text annotations can provide helpful clarifications at specific points.
legend('Sine', 'Cosine');
text(5, 0.5, 'Intersection Point'); % Adding text annotation to the plot
These features enhance the narrative of your data visualization and assist viewers in interpreting the plots without confusion.
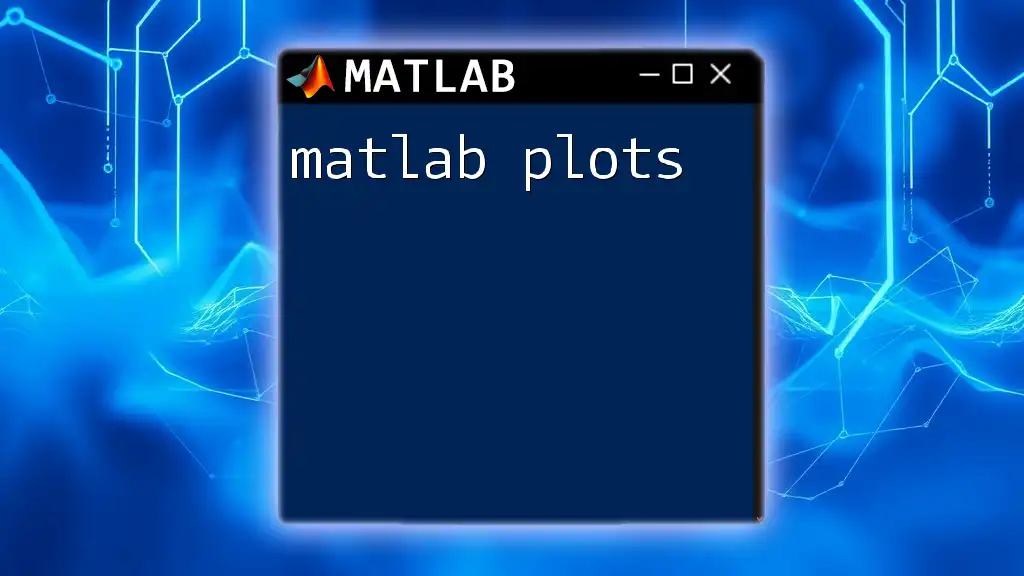
Troubleshooting Common Issues
Errors When Plotting
When working with MATLAB plots, you may encounter common errors, such as mismatched dimensions between your x and y vectors. Always ensure that both have the same number of elements before attempting to plot. A helpful way to diagnose is to check the size of each variable using the `size()` function.
Improving Plot Readability
To enhance the readability of your plots, consider adjusting properties such as font size, line width, and the background color. These tweaks can make your plot more accessible and visually appealing, particularly for presentations.
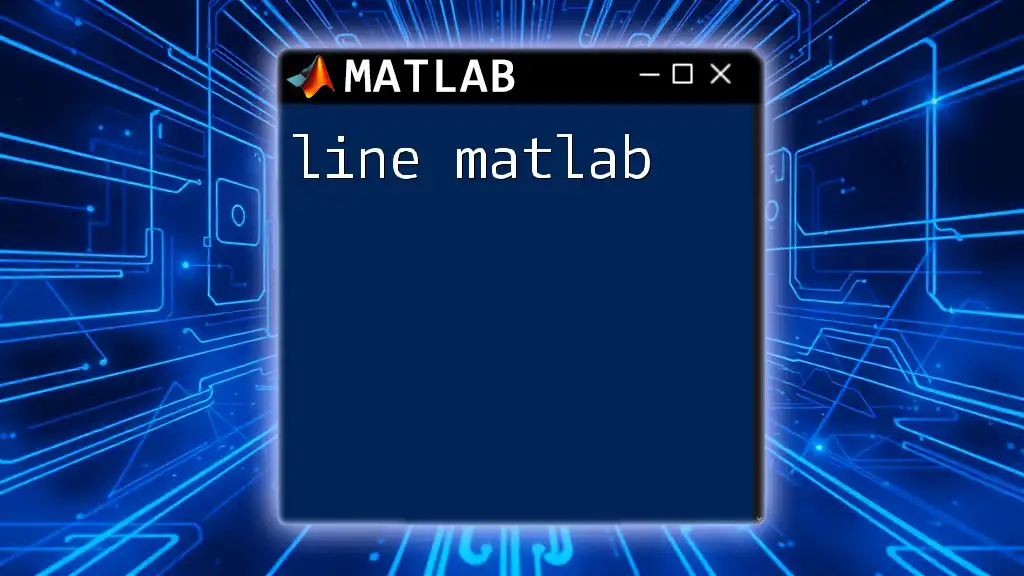
Conclusion
In summary, line MATLAB plots are an essential tool in data visualization, offering a clear and concise way to observe trends and relationships in datasets. Learning how to effectively create and customize these plots can significantly enhance your analysis and communication of results.
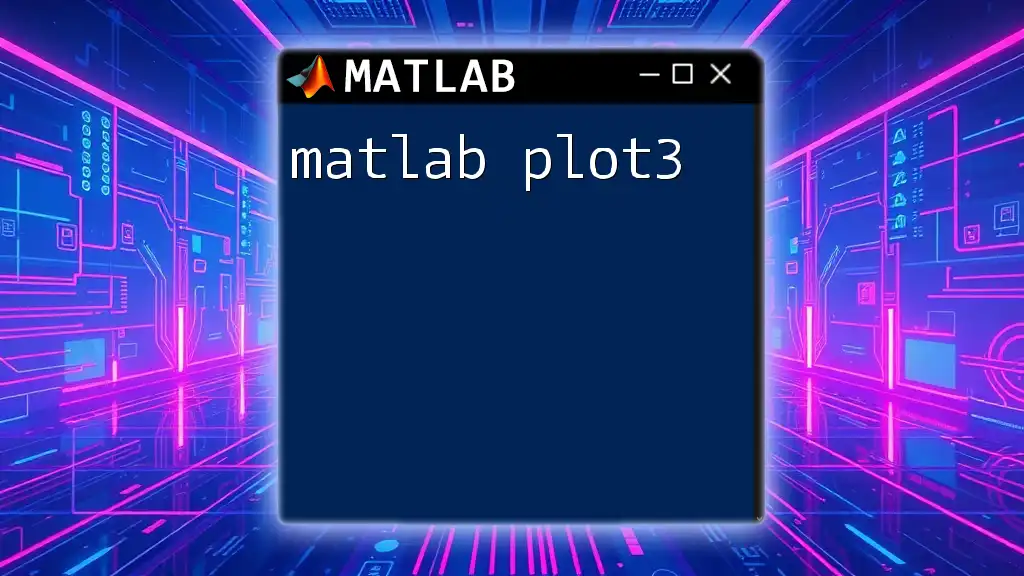
Additional Resources
For further exploration of MATLAB’s plotting capabilities, consider diving into official documentation or engaging with online courses that cover MATLAB graphics and visualization techniques. These resources can expand your knowledge and improve your plotting skills.
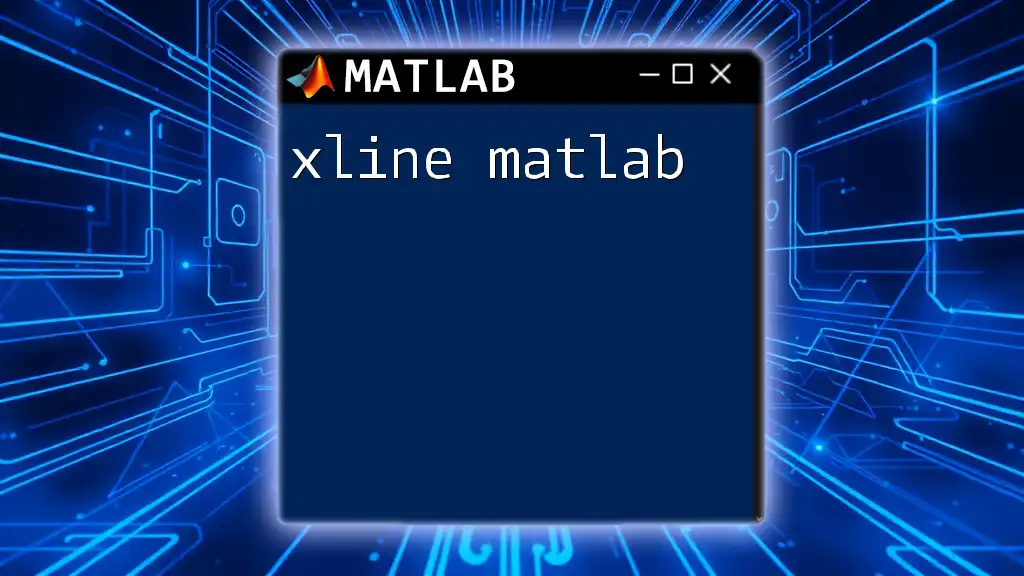
FAQs About Line MATLAB Plots
Common Questions Related to Line Plots
If you find that your plot doesn’t display, check if the figure has been created using the `figure()` command and inspect for any errors in your code. If you want to save your plot, use the `saveas()` or `print()` functions to export your figure in different formats.
By putting these guidelines into practice, you can develop a deeper understanding of creating effective line MATLAB plots and enhance your data analysis capabilities.