Integration in MATLAB can be easily performed using the `int` function for symbolic computations or the `integral` function for numerical integration, allowing users to quickly calculate definite or indefinite integrals.
% Symbolic integration example
syms x
f = x^2;
indefinite_integral = int(f, x)
% Numerical integration example
numerical_integral = integral(@(x) x.^2, 0, 1)
What is Integration?
Integration is a fundamental concept in mathematics, representing the process of finding the integral of a function. It serves as a powerful tool for accumulating quantities such as area, volume, and displacement, making it essential in fields like engineering, physics, and economics.
There are two primary types of integration: definite and indefinite integrals. An indefinite integral represents a family of functions, while a definite integral produces a numerical value that represents the area under a curve over a specific interval. Understanding these concepts is crucial when employing integration using MATLAB.
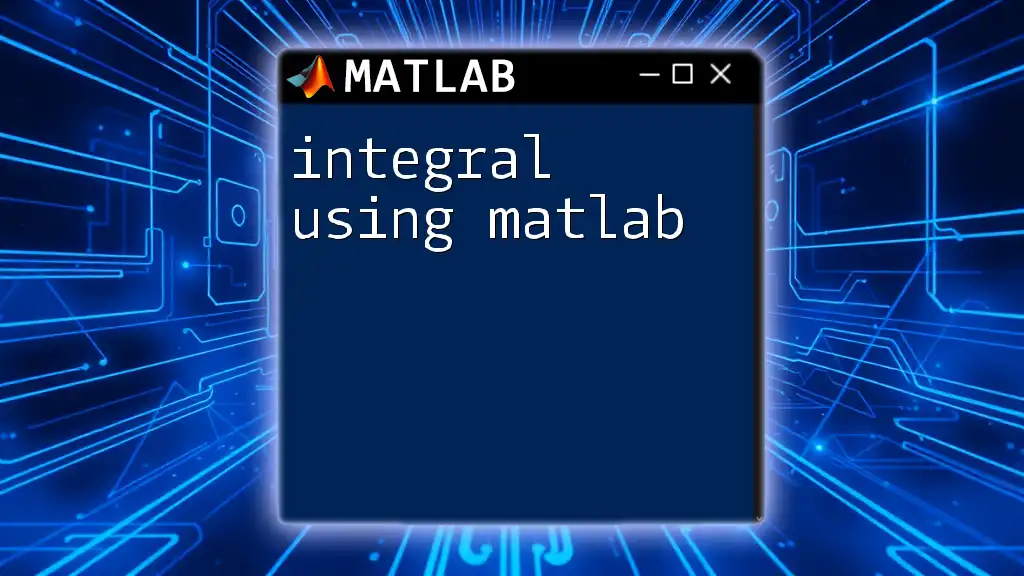
Getting Started with MATLAB Integration
Setting Up MATLAB for Integration
Before diving into integration techniques, ensure you have MATLAB installed and set up. Opening MATLAB gives you access to its interactive environment, where you can execute commands and visualize results effectively.
Basic Syntax for Integration in MATLAB
MATLAB provides several commands for performing integration, such as `int` for symbolic integration, `quad`, `integral`, and `trapz` for numerical integration. Familiarizing yourself with these commands will allow you to tackle various integration problems efficiently.
Here is a simple example of indefinite integration using MATLAB:
syms x
f = x^2 + 3*x + 2;
indefinite_integral = int(f, x)
In this code, the variable `f` is defined as a polynomial, and the `int` command computes its indefinite integral.
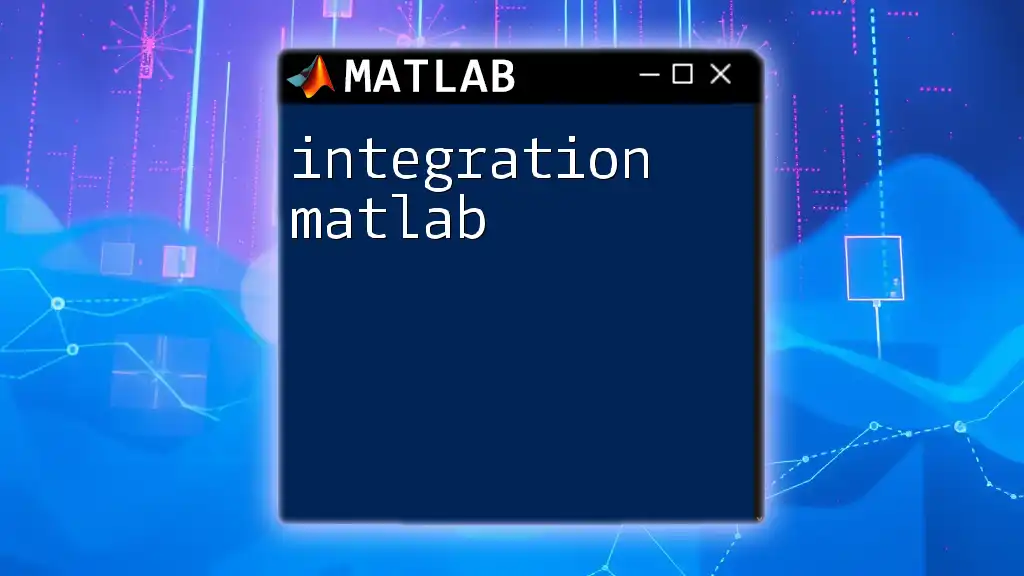
Types of Integration Techniques in MATLAB
Indefinite Integration
Indefinite integrals, which yield a function rather than a specific numeric value, can be easily computed using the `int` command in MATLAB. This command enables symbolic computation, allowing for the integration of functions represented as symbolic expressions.
For instance, to perform the indefinite integration of a trigonometric function like sine, you can use the following code:
syms x
f = sin(x);
indefinite_integral = int(f, x)
This results in the antiderivative of \( \sin(x) \), which is \( -\cos(x) + C \).
Definite Integration
Definite integrals, which calculate the area under a curve between two specified limits, are also achievable using the `int` function but with added parameters to define the limits. Understanding how to correctly set these limits is crucial when applying integration using MATLAB.
Here is an example calculating the definite integral of \( \sin(x) \) from \( 0 \) to \( \pi \):
syms x
f = sin(x);
definite_integral = int(f, x, 0, pi)
The output of this code will provide the numerical result of the area under the sine curve from \( 0 \) to \( \pi \), which equates to \( 2 \).
Numerical Integration
When dealing with complex functions or when an analytic solution is hard to obtain, numerical methods become indispensable. MATLAB offers functions like `quad` and `integral` for evaluating integrals numerically.
Here’s how you can compute a numerical integral of a simple quadratic function:
f = @(x) x.^2; % function defined as an anonymous function
numerical_integral = integral(f, 0, 1)
This example calculates the area under the curve \( x^2 \) from \( 0 \) to \( 1 \) using the `integral` function, returning a numeric value.
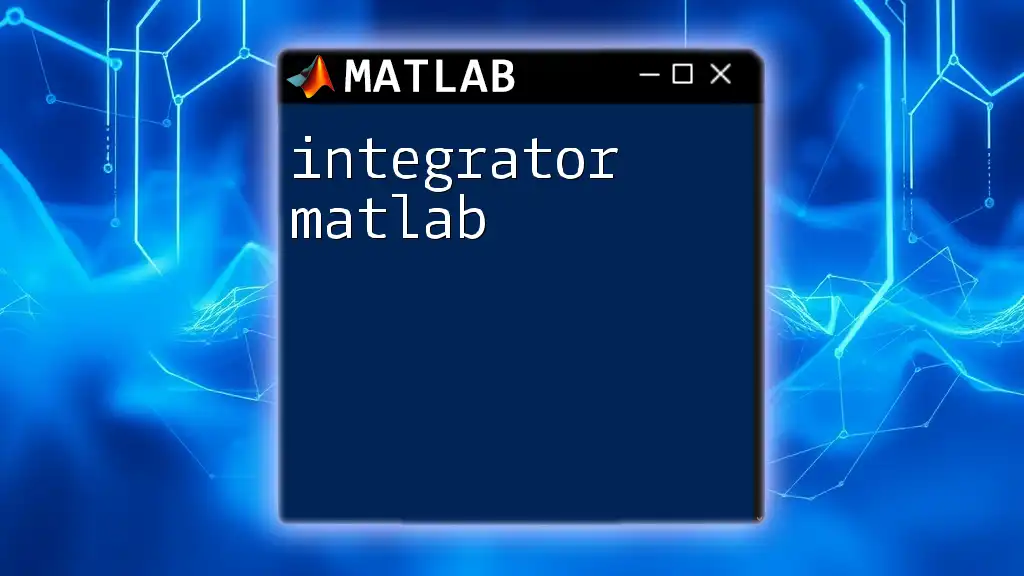
Advanced Integration Techniques
Multiple Integrals
In addition to single-variable integration, MATLAB allows for the calculation of multiple integrals. These are often necessary in applications involving multivariable functions. For double integrals, use the `integral2` function.
For example, consider the following code that computes the double integral of a function defined by \( f(x,y) = xy \):
f = @(x,y) x.*y; % Function of two variables
double_integral = integral2(f, 0, 1, 0, 1)
This code calculates the integral of \( xy \) over the square region where both \( x \) and \( y \) range from \( 0 \) to \( 1 \).
Integration over Non-standard Domains
When integrating over irregular domains, a change of variables may be necessary. MATLAB allows you to utilize different coordinate systems, such as polar coordinates, to simplify the integration process.
Consider this example where we integrate a function using polar coordinates:
f = @(r,theta) r.^2; % Function in polar coordinates
polar_integral = integral2(@(r,theta) f(r,theta).*r, 0, 1, 0, pi)
This code computes the integral of \( r^2 \) in the polar coordinate system.
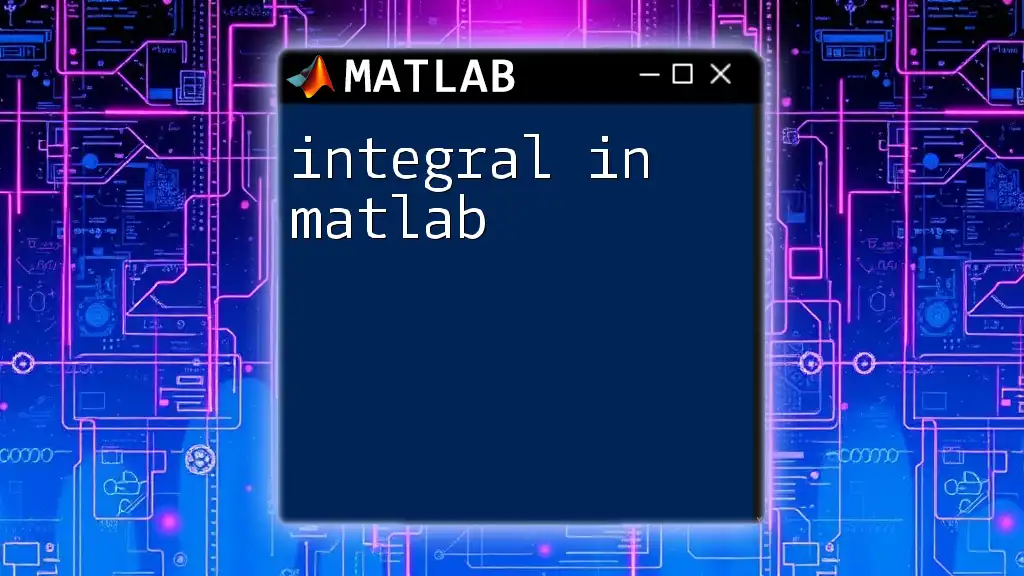
Visualizing Integrals in MATLAB
Plotting Functions and Their Integrals
Visualization enhances understanding and helps verify results. MATLAB's plotting capabilities enable you to graph functions and their integrals effortlessly.
Using `fplot`, you can visualize a function alongside its integral. Here’s a piece of code that achieves this:
f = @(x) x.^2;
fplot(f, [0, 1]);
hold on;
x = linspace(0, 1, 100);
y = integral(f, 0, x);
plot(x, y);
hold off;
title('Function and its Integral');
legend('Function','Integral');
In this code, both the function \( x^2 \) and its integral are plotted, providing a visual representation that makes it easier to understand the relationship between the two.
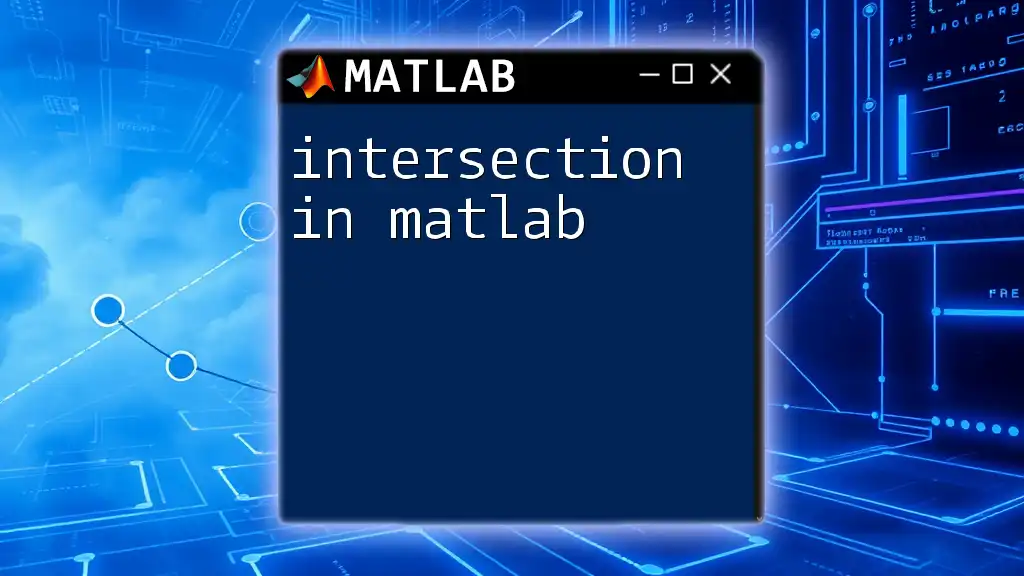
Troubleshooting Common Issues
FAQs about MATLAB Integration
As you embark on your journey with integration using MATLAB, you may encounter common errors. Common issues include improper function definitions and limits, as well as choosing inappropriate integration methods.
Tips for Efficient Integration in MATLAB
To enhance your integration efficiency in MATLAB, it’s vital to:
- Verify your functions: Ensure that the function you intend to integrate is correctly defined.
- Select the right method: Depending on the function complexity, choose symbolic or numerical integration wisely.
- Utilize MATLAB’s robust documentation: Familiarize yourself with built-in functions highlighted in MATLAB’s help resources.
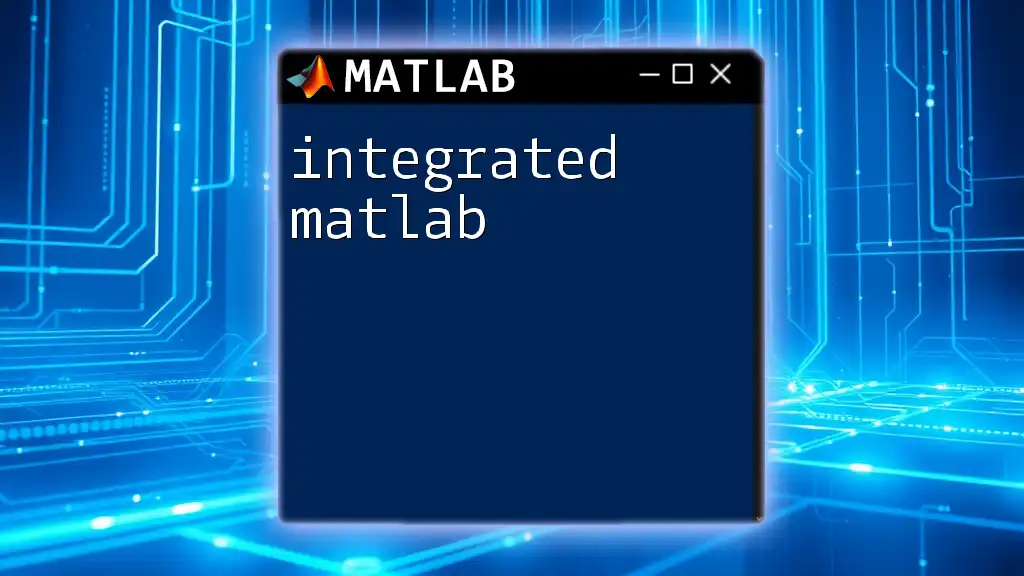
Conclusion
Mastering integration using MATLAB is a pivotal skill in both academic and professional settings. This guide provides you with foundational knowledge alongside practical examples, helping you perform various integration tasks efficiently.
As you continue exploring MATLAB’s capabilities, don’t hesitate to dive deeper into advanced mathematical techniques. Joining our courses opens the door to a wealth of resources aimed at enhancing your MATLAB knowledge and skills.
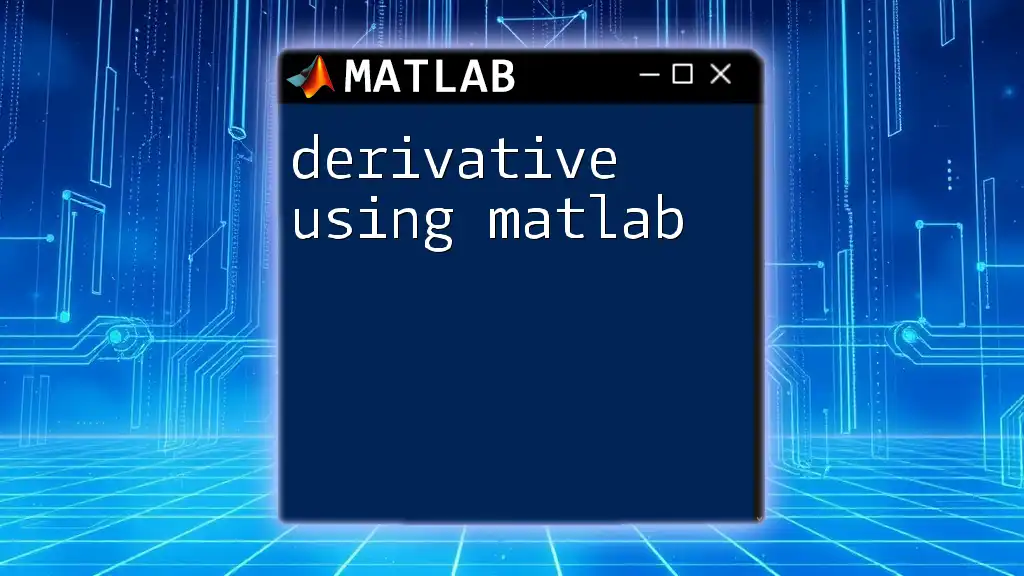
Additional Resources
For further reading, exploring the official MATLAB documentation can enhance your understanding of the commands and features available for integration. Additionally, consider books and online courses focused on advanced MATLAB practices to develop your expertise in mathematical modeling and analysis.