Convolution in MATLAB is a mathematical operation used to combine two sequences or functions, typically represented as vectors, to produce a third vector that expresses how the shape of one is modified by the other.
Here’s a simple example of how to perform convolution in MATLAB:
% Define two input signals
x = [1, 2, 3];
h = [0, 1, 0.5];
% Perform convolution
y = conv(x, h);
% Display the result
disp(y);
Understanding Convolution
What is Convolution?
Convolution is a mathematical operation that combines two functions to produce a third function, expressing how the shape of one is modified by the other. This operation is fundamental in various fields, such as signal processing, systems analysis, and image processing. In essence, convolution helps to filter and analyze signals or data, revealing features or trends that may not be readily apparent.
Mathematical Representation
Mathematically, convolution is defined as the integral of the product of two functions, with one of the functions being reversed and shifted. In the discrete-time domain, it can be expressed as:
\[ y[n] = (x * h)[n] = \sum_{k=-\infty}^{\infty} x[k] h[n-k] \]
Here, \( x[n] \) represents the input signal, \( h[n] \) denotes the impulse response (or kernel), and \( y[n] \) is the resulting output signal after convolution. Visualizing this operation enhances comprehension, as it allows one to see the interaction between the input signal and the kernel.
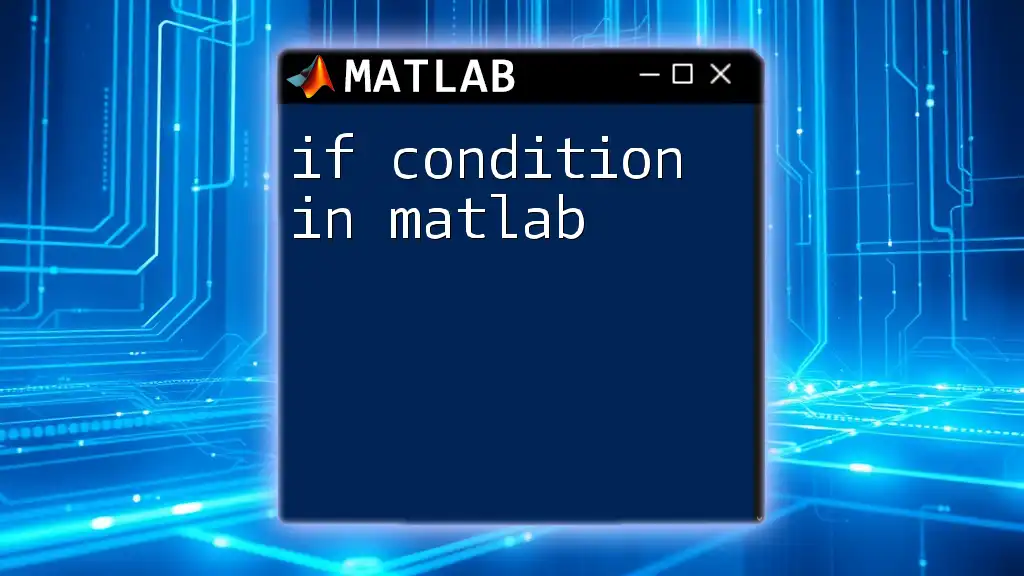
Convolution in MATLAB
Overview of MATLAB's Convolution Functions
MATLAB offers several built-in functions to perform convolution, each suited to specific types of data:
- `conv`: Used primarily for one-dimensional convolution.
- `conv2`: Suitable for two-dimensional convolution, particularly with matrices.
- `filter`: Ideal for real-time applications, such as implementing FIR filters.
Understanding when to use each function is crucial for efficient programming in MATLAB.
Using the `conv` Function
Syntax
The syntax for the `conv` function is straightforward:
c = conv(a, b)
Where \( a \) is the first vector (input signal), and \( b \) is the second vector (impulse response), and \( c \) will be the output vector.
Example
Consider an example where we perform convolution on two discrete signals:
% Define two signals
x = [1, 2, 1]; % Input signal
h = [1, 0, -1]; % Impulse response
% Perform convolution
y = conv(x, h);
disp(y);
This operation yields a new signal, which can be analyzed to determine how the input has been modified by the impulse response.
Explanation
The output of this convolution operation reveals important features regarding the interactions between the input signal and the kernel. Specifically, it highlights reactions to impulses in the input signal due to the structure of the impulse response, offering insights into filtering effects or signal transformations.
Convolution in 2D Using `conv2`
Syntax
The `conv2` function is specifically designed for two-dimensional convolution. Its syntax is as follows:
C = conv2(A, B)
Where \( A \) is a matrix (image data) and \( B \) is a kernel (filter).
Example
Let’s consider an example involving a 2D matrix and a kernel:
% Define a matrix and a kernel
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
B = [1, 0; 0, -1];
% Perform convolution
C = conv2(A, B);
disp(C);
In this case, the `conv2` function applies the kernel to the matrix, generating a new matrix that reflects the convolution operation.
Explanation
The resulting matrix from this convolution operation often highlights edges within the original matrix, emphasizing features such as gradients and transitions. This property makes it quite valuable in image processing applications, like edge detection.
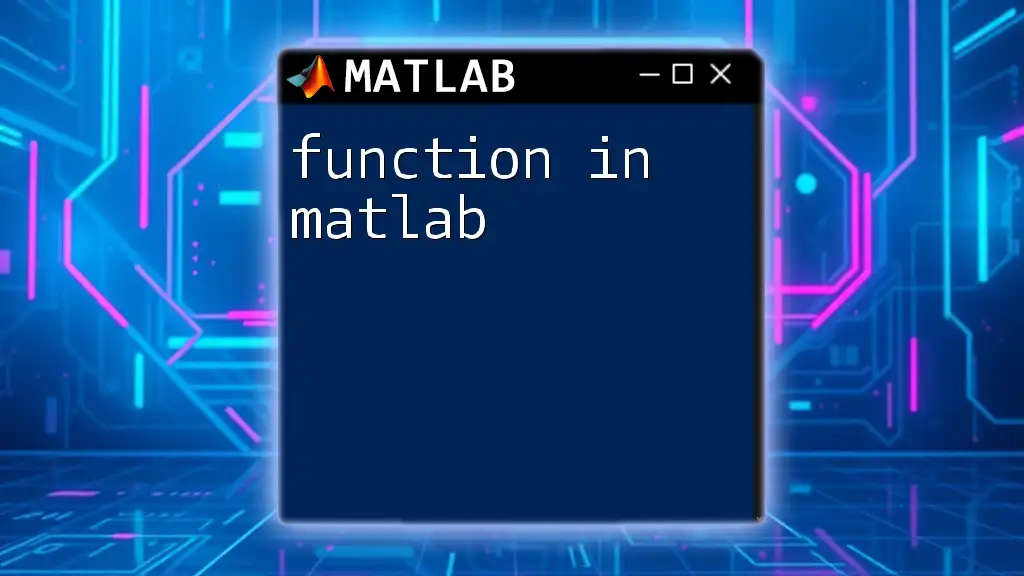
Advanced Techniques in Convolution
Using `filter` for Real-time Applications
Syntax and Use Case
The `filter` function is adept at implementing Finite Impulse Response (FIR) filters, which can smooth, enhance, or manipulate signals. Its syntax is as follows:
y = filter(b, a, x)
Where \( b \) represents the numerator coefficients, \( a \) the denominator coefficients, and \( x \) the input signal.
Example
Here’s an example where we apply a simple filter to a signal:
b = [1, -1]; % Difference filter
x = [3, 5, 2, 6, 3];
y = filter(b, 1, x); % Filter application
disp(y);
Explanation
This operation effectively computes the difference between consecutive elements in the signal, which is useful in applications such as change detection or smoothing by removing rapid fluctuations, thus revealing longer-term trends.
Performance Considerations
Computational Efficiency
When performing convolution, it is crucial to consider computational efficiency. Direct convolution can be computationally expensive, especially for large datasets. Using the Fast Fourier Transform (FFT) can speed up the process significantly, rendering it more efficient for larger signals.
Here's a comparison between direct convolution and convolution using FFT:
% Direct convolution
c_direct = conv(x, h);
% Convolution using FFT
c_fft = ifft(fft(x) .* fft(h));
The FFT method leverages the convolution theorem, which states that multiplication in the frequency domain is equivalent to convolution in the time domain.
Use Cases in Signal Processing and Image Analysis
Convolution finds myriad applications in both signal processing and image analysis:
- Filtering audio signals: For applications such as noise reduction, where specific frequency components must be amplified or attenuated.
- Image blurring or sharpening: By applying appropriate filters, you can manipulate image quality to improve visual features or perform edge detection.
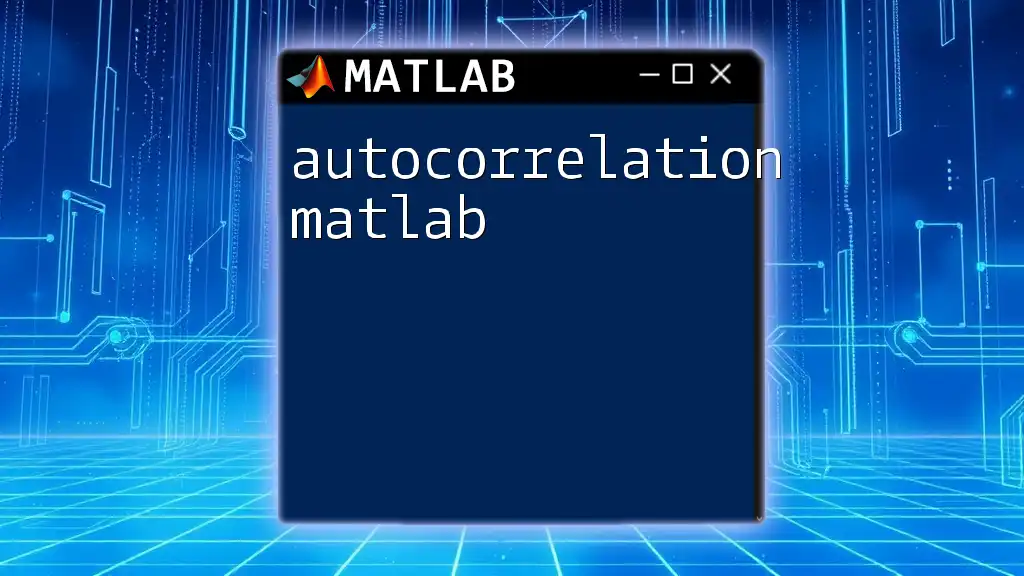
Common Mistakes and Troubleshooting
Misunderstanding Dimensions
One common issue users encounter when performing convolution is mismatched dimensions between the input vectors or matrices. It is vital to ensure compatibility by confirming that the sizes of the data structures align with the requirements of the convolution functions in MATLAB.
Performance Bottlenecks
Inappropriately choosing convolution methods can lead to performance bottlenecks, particularly with larger datasets. Understanding which convolution method to use, based on data size and dimensionality, is key to achieving optimal execution speed.
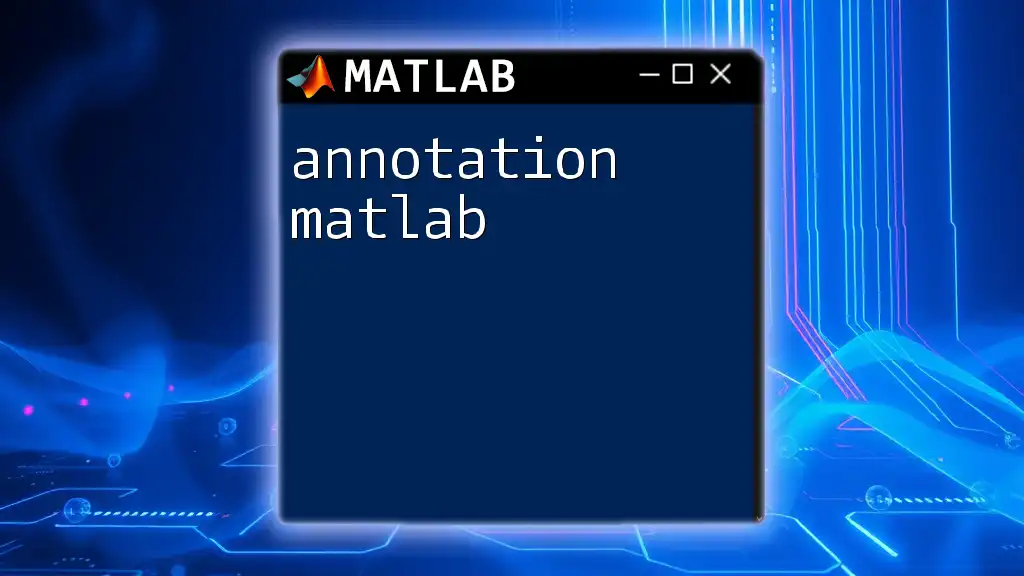
Conclusion
In conclusion, understanding convolution in MATLAB is crucial for anyone looking to analyze or manipulate signals and images effectively. By mastering built-in functions like `conv`, `conv2`, and `filter`, users can capitalize on the powerful capabilities of MATLAB in various applications. To fully harness these techniques, practice by implementing diverse convolution tasks tailored to your specific projects. The ability to filter and analyze complex datasets can illuminate features and insights previously obscured, opening pathways to innovative solutions across multiple disciplines.
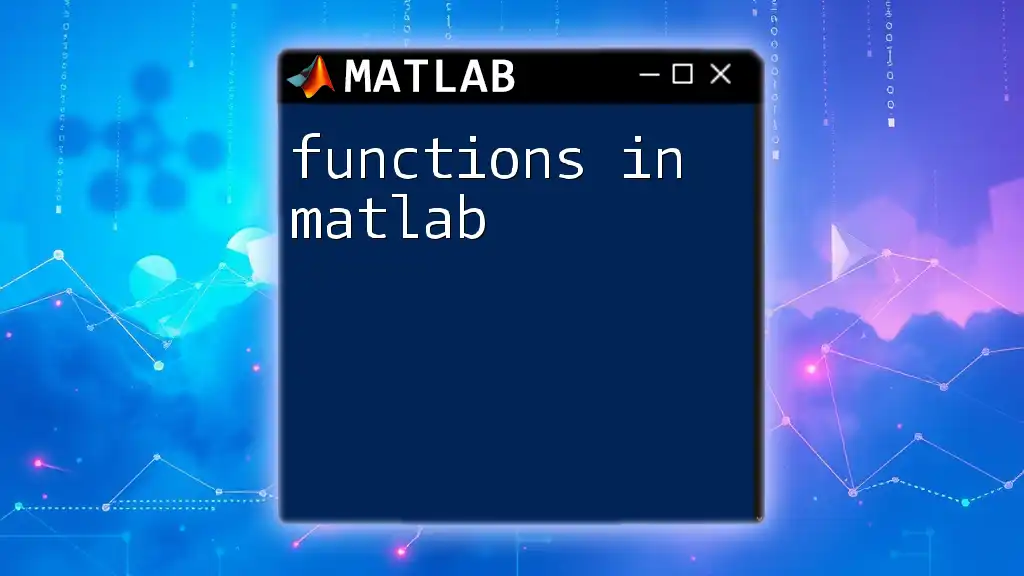
Additional Resources
For more detailed learning, refer to the official MATLAB documentation and community forums, where you can access a wealth of knowledge and support on convolution and other MATLAB operations.
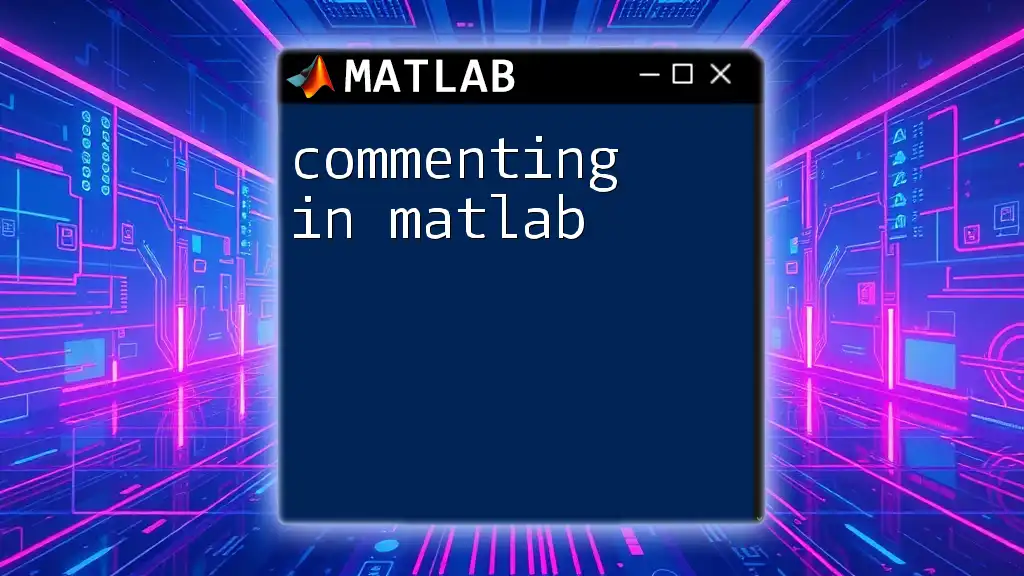
Call to Action
To further enhance your MATLAB skills and explore the depth of convolution and beyond, consider signing up for our courses or tutorials designed to elevate your understanding and proficiency in MATLAB programming.