The delta function in MATLAB, represented by `dirac`, is used to model an ideal impulse response, returning infinity at zero and zero elsewhere, and can be utilized to generate a symbolically defined impulse signal.
t = -5:0.01:5; % Time vector
delta_function = dirac(t); % Delta function
plot(t, delta_function); % Plotting the delta function
xlabel('Time');
ylabel('Amplitude');
title('Delta Function using dirac in MATLAB');
grid on;
What is the Delta Function?
The delta function is an essential mathematical concept widely used in various fields, particularly in engineering, physics, and signal processing. It is fundamentally characterized as a distribution, often represented mathematically as:
\[ \delta(t) = \begin{cases} +\infty & \text{if } t = 0 \\ 0 & \text{if } t \neq 0 \end{cases} \]
The attributes of the delta function are crucial for understanding impulse signals and the behavior of systems under the influence of such signals. It is often called the impulse function due to its ability to represent idealized impulses in time.
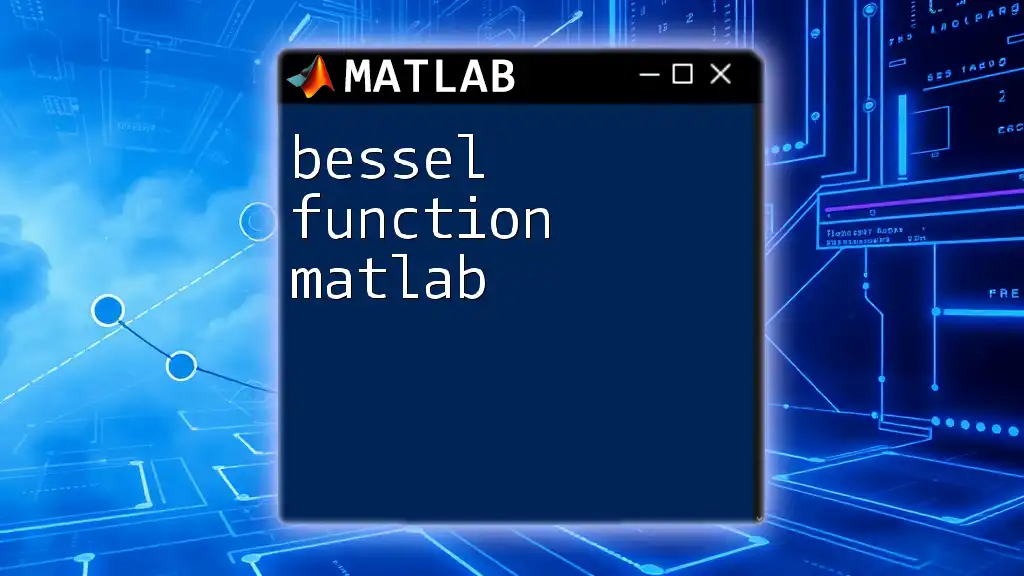
Applications of the Delta Function
The delta function appears in a variety of applications, playing a key role in:
- Impulse Responses: Where it acts as an input to a linear time-invariant (LTI) system, allowing the examination of the system's output response.
- Convolution: The delta function is used to simplify convolution integrals, providing insights into system behaviors based on inputs and impulse responses.
- Sampling Theory: Used in signal processing, the delta function serves as a mathematical tool to understand the reconstruction of signals.
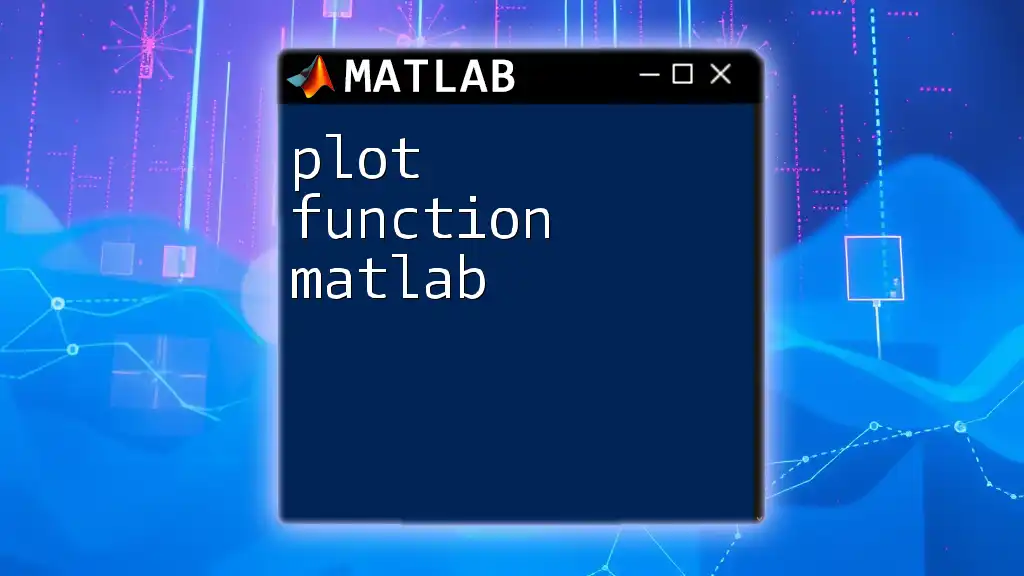
Understanding Delta Function in MATLAB
Overview of MATLAB’s Capabilities
MATLAB provides robust capabilities to work with mathematical concepts like the delta function. The built-in functions available in MATLAB allow users to define and manipulate delta functions efficiently. The `dirac` function is particularly important in this context as it represents the delta function in MATLAB's symbolic toolbox.
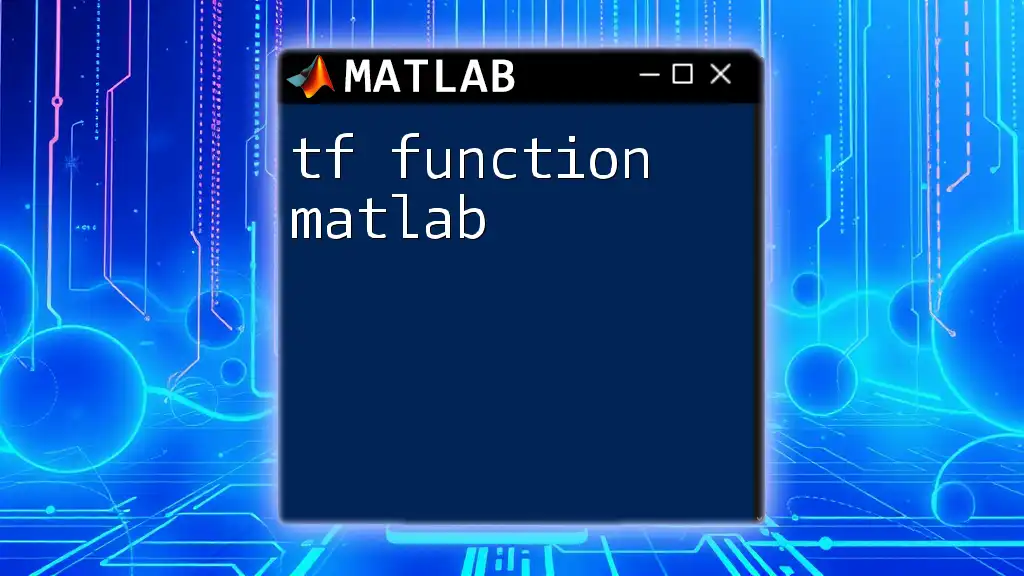
Creating a Delta Function in MATLAB
Using the `dirac` Function
In MATLAB, you can create a delta function using the `dirac` function. This function allows users to define a symbolic delta function easily.
To visualize the delta function, you can plot it over a range using the following code snippet:
syms t;
deltaFunc = dirac(t);
fplot(deltaFunc, [-5, 5]);
title('Dirac Delta Function');
xlabel('Time (t)');
ylabel('Amplitude');
grid on;
This snippet utilizes the symbolic toolbox's `dirac` function for creating a delta function representation. The output will display a peak at zero, indicating the delta function's behavior.
Discrete Delta Function
Definition and Importance
The discrete delta function, often referred to as the Kronecker delta, represents a sequence of values, primarily 1 at zero and 0 elsewhere. Understanding the discrete delta function is essential in the context of digital signal processing, where systems operate on discrete samples.
Code Example for Discrete Delta Function
You can define a discrete delta function in MATLAB as follows:
n = -5:5;
deltaDiscrete = n == 0;
stem(n, deltaDiscrete);
title('Discrete Delta Function');
xlabel('n');
ylabel('Amplitude');
grid on;
In this code, the discrete delta function is generated using a logical condition where deltaDiscrete equals 1 when n is 0 and 0 otherwise. This visual representation showcases the core traits of the discrete delta function and its role in digital systems.
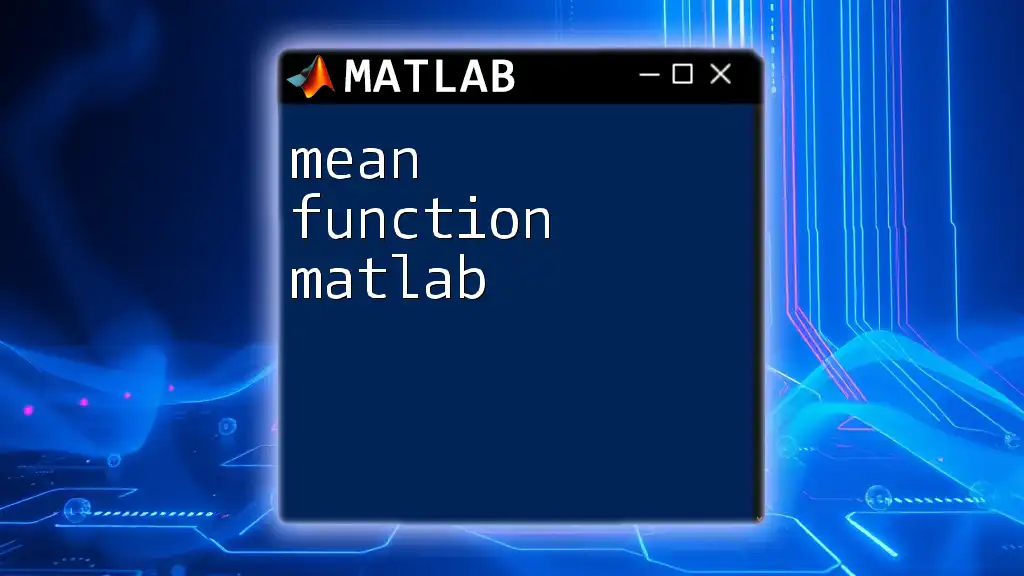
Properties of the Delta Function
Sifting Property
One of the most significant properties of the delta function is the sifting property. This property states that:
\[ \int_{-\infty}^{\infty} f(t) \delta(t - t_0) \, dt = f(t_0) \]
This means that the integral of a function multiplied by the delta function extracts the value of the function at the point where the delta function is centered.
Code Example Demonstrating Sifting Property
Here’s a MATLAB example that illustrates the sifting property:
f = @(x) x.^2;
integralValue = int(f(t) * dirac(t - 1), t);
disp(integralValue);
When this code is executed, it calculates the integral of the function \( f(t) = t^2 \) multiplied by the delta function centered at \( t = 1 \). The output reveals the value of the function at that specific point.
Linearity
The delta function possesses a linearity property, which means that if you take a linear combination of delta functions, the result is the same. This property allows us to superimpose several delta functions, making it easier to model complex systems.
Time Shifting
The time shifting property of the delta function indicates that shifting the delta function in time will not affect its integral property. Formally, for a shift \( t_0 \):
\[ \delta(t - t_0) \]
This shifted delta function still behaves as a unit impulse centered at \( t = t_0 \).
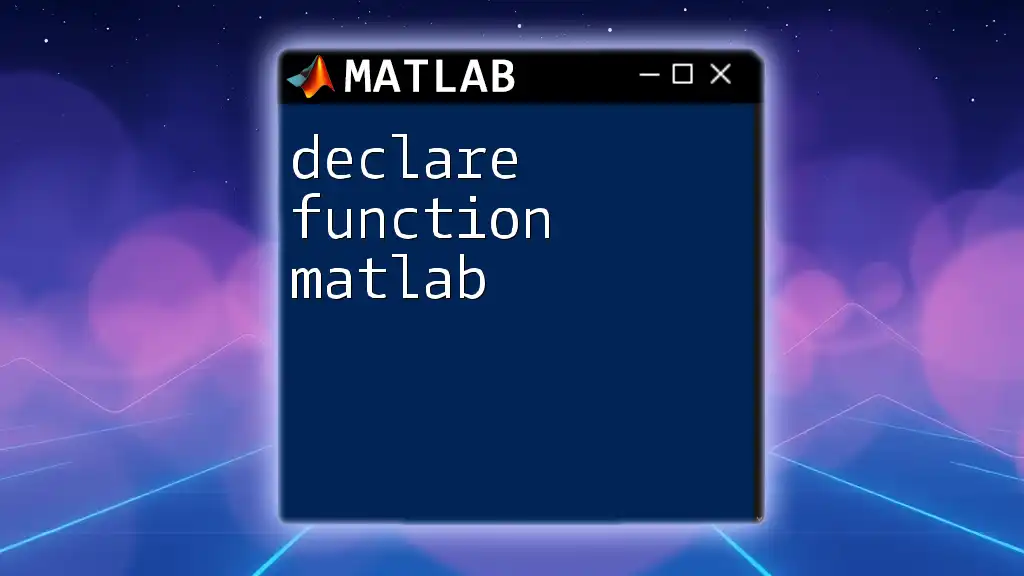
Working with Delta Functions in Signal Processing
Impulse Response
In systems theory, the impulse response is a critical concept that allows engineers to analyze linear time-invariant systems. The impulse response of a system can be obtained by applying the delta function as an input.
Code Example of Impulse Response
Here’s how you can simulate the impulse response in MATLAB:
t = 0:0.01:5;
h = [0, 0, 1, 0, 0];
y = conv(h, deltaDiscrete, 'same');
stem(t, y);
title('Impulse Response');
xlabel('Time (s)');
ylabel('Amplitude');
grid on;
In this example, a simple impulse response is simulated using the convolution of the impulse response h with the delta function. The `conv` function calculates the convolution, providing insight into how the system responds to an impulse.
Fourier Transform and Delta Function
In signal analysis, the Fourier transform of a delta function is particularly noteworthy. The Fourier transform reveals the frequency representation of the signal, and for the delta function, this representation encompasses all frequencies equally.
Code Example for Fourier Transform
To illustrate this concept further, the following MATLAB code demonstrates the Fourier transform of a delta function:
dt = 0.01;
t = -5:dt:5;
delta = dirac(t);
F = fftshift(fft(delta, 512));
f = linspace(-1/(2*dt), 1/(2*dt), 512);
plot(f, abs(F));
title('Fourier Transform of Delta Function');
xlabel('Frequency (Hz)');
ylabel('Magnitude');
grid on;
This code uses the Fast Fourier Transform (FFT) to compute the frequency response of the delta function. The plot shows a uniform response across the frequency spectrum, validating the delta function's significance in representing signals across all frequencies.
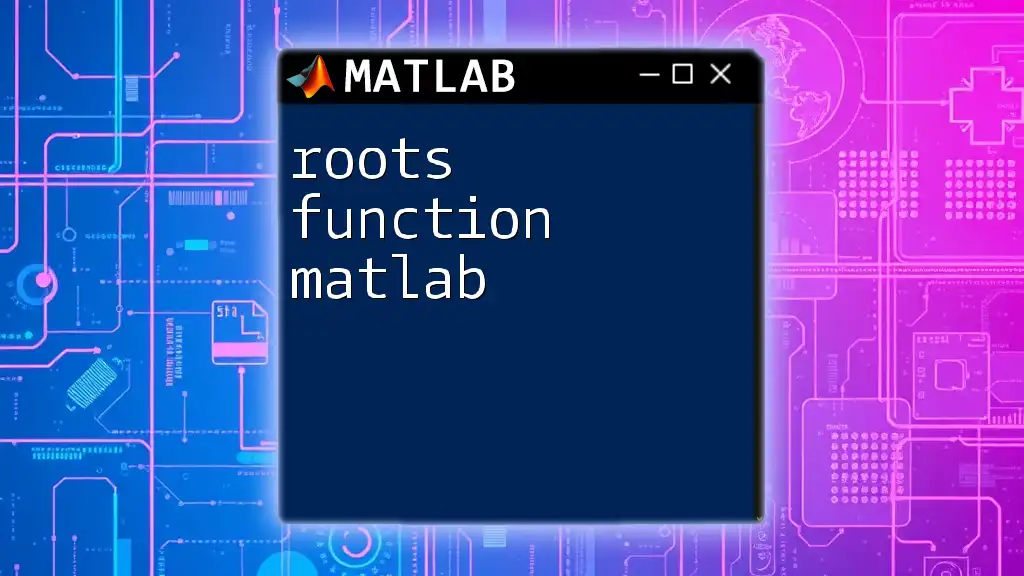
Challenges and Common Misconceptions
Understanding Delta Function Behavior
Many learners struggle with the abstract concept of the delta function, often confusing it with a typical function. Key points to remember include:
- The delta function is not a function in the traditional sense; rather, it is a generalized function or a distribution.
- It represents idealized impulses and does not have a defined value outside the context of an integral or convolution.
Conclusion
In this comprehensive guide on the delta function in MATLAB, we have explored its definition, properties, and applications in different contexts. MATLAB provides an excellent platform for working with the delta function, enabling users to apply it effectively across multiple domains. Understanding and mastering the delta function will enhance your ability to analyze and design complex systems in both theoretical and practical scenarios.
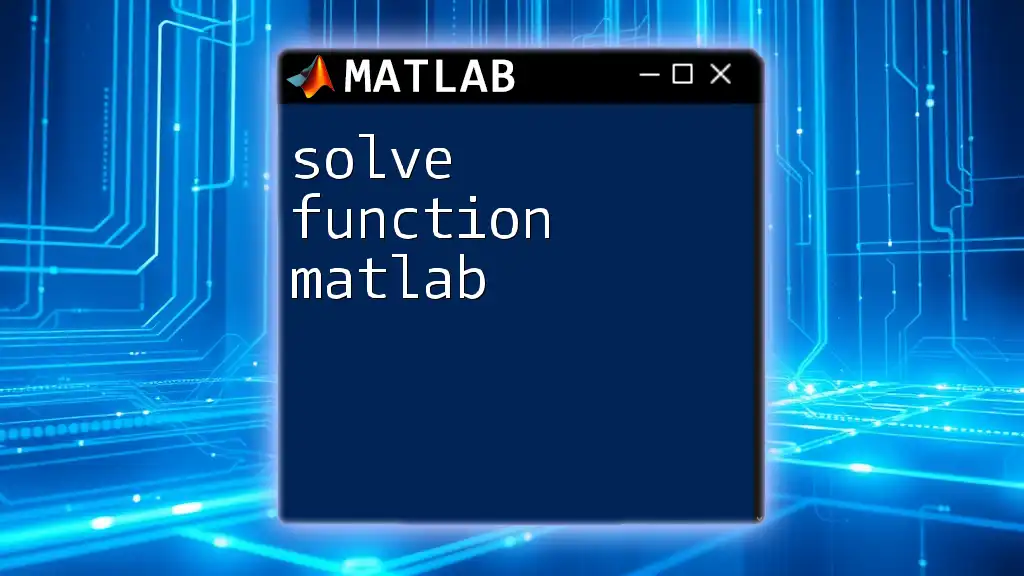
Additional Resources
Recommended Books and Articles
To deepen your understanding of the delta function and its applications, consider referring to:
- Advanced Engineering Mathematics by Erwin Kreyszig
- Signals and Systems by Alan V. Oppenheim
Online Tutorials and MATLAB Documentation
For further insights into MATLAB functionalities, explore the official MATLAB documentation and other online tutorials that focus on signal processing and mathematical modeling techniques.
By practicing the examples provided and experimenting with various scenarios, you will solidify your grasp of the delta function and its utility in MATLAB.