A lowpass filter in MATLAB can be used to allow signals with a frequency lower than a selected cutoff frequency to pass through while attenuating higher frequencies.
Here’s a simple example of how to design a lowpass filter using the `designfilt` function in MATLAB:
% Design a lowpass filter with a cutoff frequency of 0.1 times the Nyquist frequency
lowPassFilter = designfilt('lowpassfir', 'CutoffFrequency', 0.1, ...
'SampleRate', 1);
What is a Lowpass Filter?
A lowpass filter is a signal processing tool that allows signals with a frequency lower than a certain cutoff frequency to pass through while attenuating higher frequencies. This makes lowpass filters essential for applications in areas like audio processing, communications, and data smoothing.
The main purpose of a lowpass filter is to remove unwanted noise from a signal while preserving the essential features. For instance, in audio processing, it helps eliminate high-frequency noise while retaining the integrity of the desired audio signal.
Understanding the role of lowpass filters is crucial for anyone working with signal analysis, particularly in MATLAB, which provides extensive support for filter design and implementation.
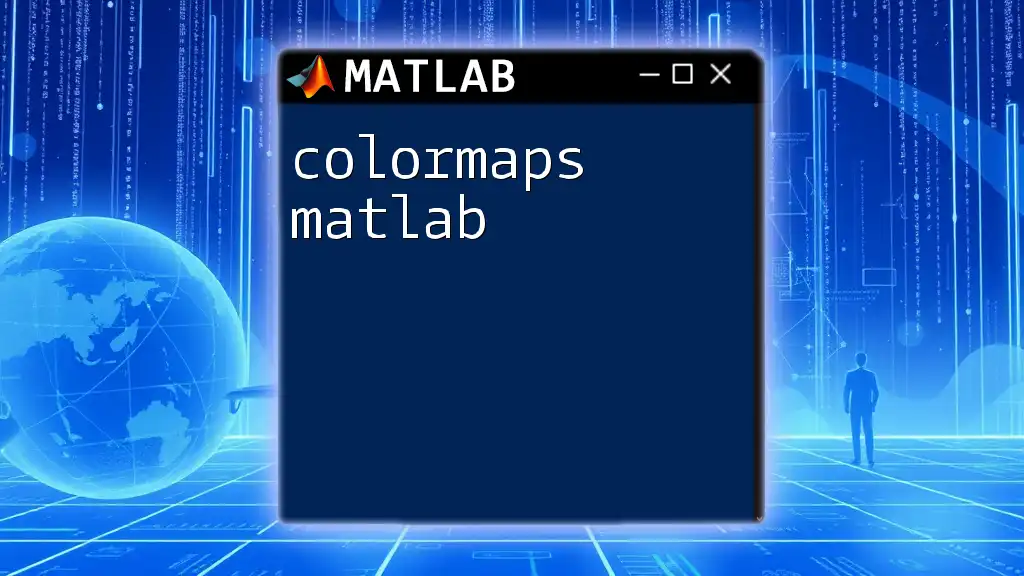
Why Use MATLAB for Lowpass Filtering?
MATLAB is a powerful tool for signal processing, offering many built-in functions and a user-friendly environment that allows for rapid prototyping and visualization. The following advantages make MATLAB an excellent choice for lowpass filtering:
- Ease of Use: With intuitive syntax, users can create and modify filters quickly.
- Visualization: MATLAB offers various plot functions to visually assess filter performance.
- Comprehensive Libraries: The Signal Processing Toolbox includes dedicated functions for filter design, analysis, and application.
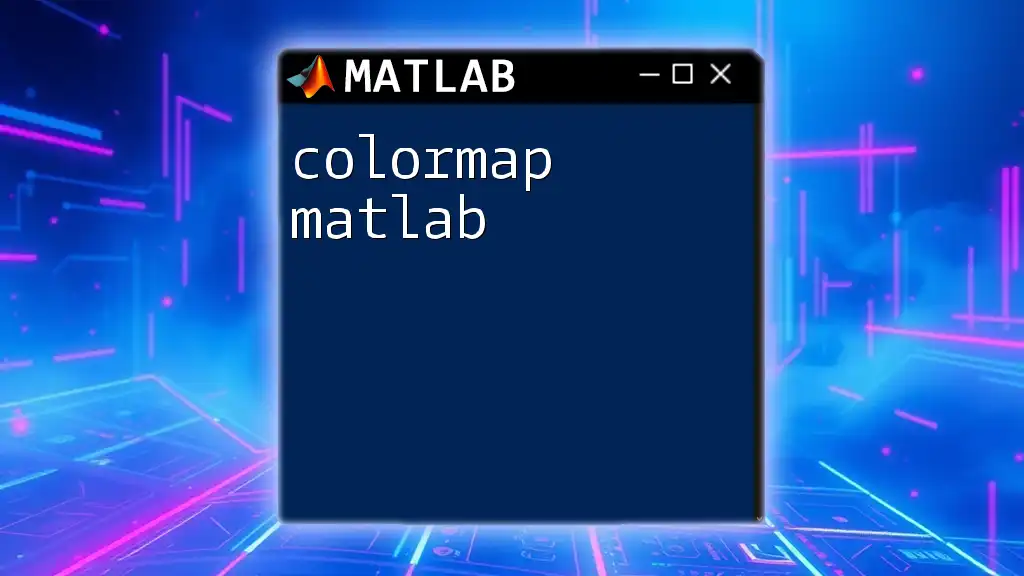
Types of Lowpass Filters in MATLAB
FIR Filters
FIR (Finite Impulse Response) filters are digital filters characterized by a finite impulse response. These filters are stable and have a linear phase, making them suitable for many applications. The primary benefits of using FIR filters include:
- No feedback: The output depends only on the current and past input values.
- Stability: FIR filters are always stable due to their finite impulse response.
Example: Designing a Simple FIR Lowpass Filter
To design a simple FIR lowpass filter in MATLAB, follow these steps:
- Define Parameters: Specify the sampling frequency, cutoff frequency, and filter order.
- Design the Filter: Use the `fir1` function to create the filter coefficients.
Code Snippet: FIR Lowpass Filter Design
Fs = 1000; % Sampling frequency
Fc = 100; % Cutoff frequency
N = 20; % Filter order
b = fir1(N, Fc/(Fs/2)); % Design FIR filter
IIR Filters
IIR (Infinite Impulse Response) filters are another class of digital filters with an impulse response that theoretically lasts forever. They can achieve a desired frequency response with fewer coefficients than FIR filters, although they may have non-linear phase characteristics.
The benefits of using IIR filters include their:
- Efficiency: IIR filters require fewer computations to achieve similar performance compared to FIR filters.
- Ease of Implementation: They can be designed using fewer parameters.
Example: Designing an IIR Lowpass Filter
Similar to FIR filters, IIR filters can be designed easily in MATLAB using the `butter` function to create Butterworth filters.
Code Snippet: IIR Lowpass Filter Design
Fs = 1000; % Sampling frequency
Fc = 100; % Cutoff frequency
[b,a] = butter(4, Fc/(Fs/2)); % Design IIR filter using Butterworth design
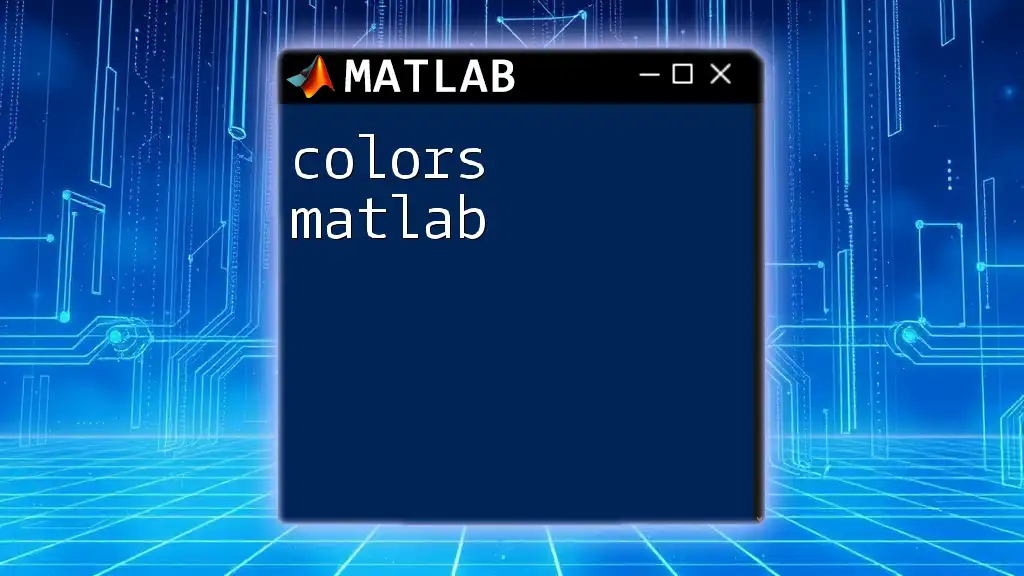
Creating a Lowpass Filter in MATLAB
Step-by-Step Process
To create a lowpass filter in MATLAB, follow this structured approach:
Define Parameters: Setting the sampling frequency and cutoff frequency is critical. The filter order will dictate the sharpness of the transition between passband and stopband.
Choose Filter Type: Decide whether to use an FIR or IIR filter based on the application requirements, either linear phase characteristics or computational efficiency.
Design the Filter: Utilize MATLAB functions like `fir1` for FIR filters or `butter` for IIR filters.
Example: Implementing a Lowpass Filter
Here’s a complete example that demonstrates how to implement a lowpass filter using a simple noisy sine wave signal.
Complete Code Example
% Sample parameters
Fs = 1000; % Sampling frequency
t = 0:1/Fs:1; % Time vector
x = sin(2*pi*50*t) + randn(size(t)); % Noisy signal
% Design FIR filter
Fc = 100; % Cutoff frequency
N = 20; % Filter order
b = fir1(N, Fc/(Fs/2)); % Design FIR filter
y = filter(b, 1, x); % Apply filter to the signal
% Plot original and filtered signal
figure;
subplot(2,1,1);
plot(t, x);
title('Original Noisy Signal');
xlabel('Time (s)');
ylabel('Amplitude');
subplot(2,1,2);
plot(t, y);
title('Filtered Signal using FIR Lowpass Filter');
xlabel('Time (s)');
ylabel('Amplitude');
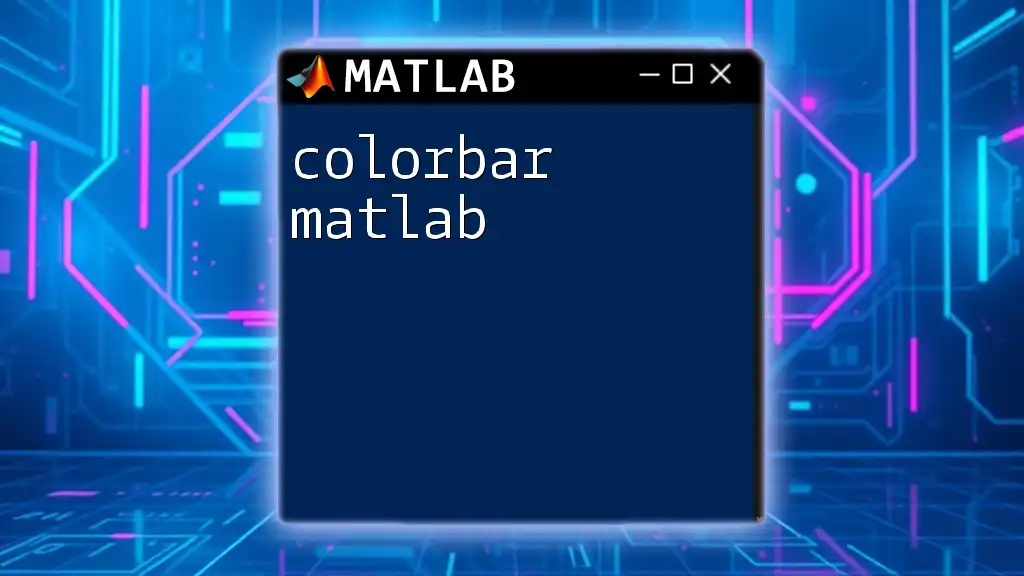
Analyzing the Filter's Performance
Frequency Response Analysis
The frequency response of a filter is a crucial measure of its effectiveness. Understanding how the filter responds to different frequencies helps users assess its performance.
You can visualize the frequency response in MATLAB using the `freqz` function, which displays the magnitude and phase response of the filter.
Code Snippet: Frequency Response
freqz(b, 1); % Display frequency response of FIR filter
title('FIR Lowpass Filter Frequency Response');
Time Domain Response
Evaluating the time-domain response gives insight into how the filter affects the original signal. By comparing the original noisy signal with the filtered output visually, one can assess the effectiveness of the lowpass filter in noise reduction.
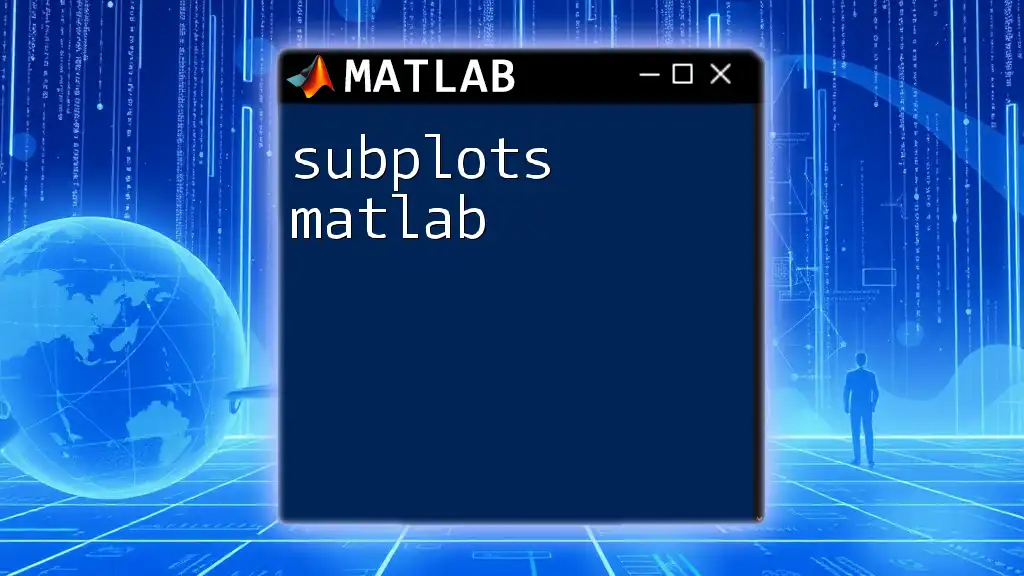
Advanced Techniques
Adaptive Lowpass Filtering
Adaptive filtering is a more advanced technique that modifies filter coefficients in real-time based on incoming signal conditions. This can be particularly useful in environments where signal characteristics fluctuate widely.
Implementing Adaptive Filters in MATLAB
MATLAB provides the `adaptfilt` function, which is great for implementing adaptive filters.
Code Snippet: Adaptive Lowpass Filter
% Adaptive filtering parameters
mu = 0.01; % Step size
N = 32; % Filter order
d = x; % Desired signal
% Adaptive filter
ha = adaptfilt.lms(N, mu);
[y_adaptive, err] = filter(ha, d, x);
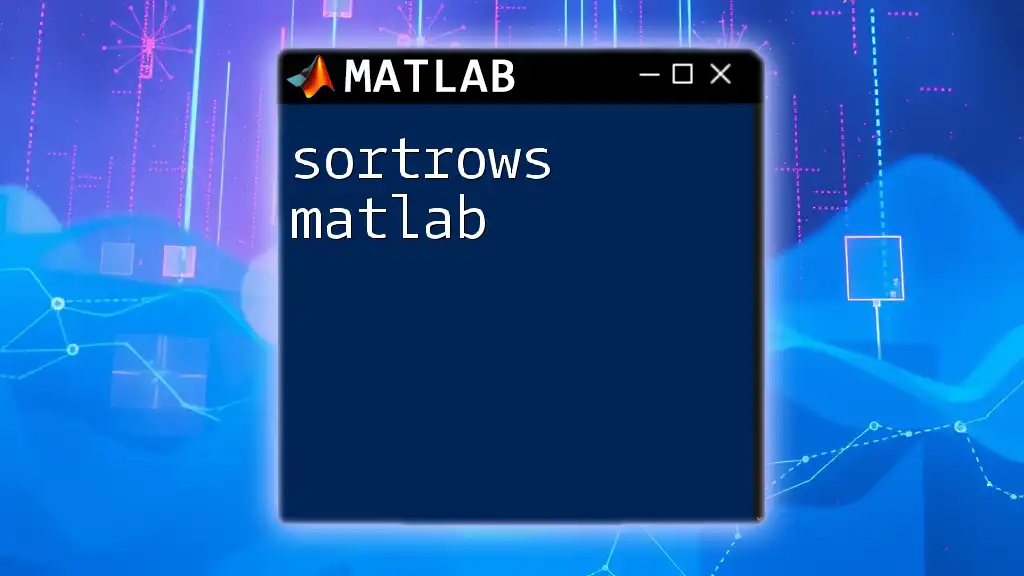
Conclusion
In summary, lowpass filters are invaluable tools in signal processing that can significantly improve the quality of your signals. By using MATLAB's rich set of functions, you can easily design, implement, and analyze lowpass filters. Whether you're working with FIR or IIR filters, MATLAB ensures flexibility and ease of use.
By exploring additional resources and practicing with real signals, you can deepen your understanding of filter design and signal processing techniques in MATLAB. Dive into the world of lowpass filters and do not hesitate to experiment with the commands and examples provided to gain practical experience.