A boxplot in MATLAB visually summarizes the distribution of a dataset through its quartiles and highlights potential outliers.
Here’s a simple code snippet to create a boxplot:
data = randn(100,1); % Generating random data
boxplot(data); % Creating a boxplot of the data
What is a Boxplot?
Boxplots are graphical representations that provide insights into the distribution of a dataset. They summarize data through their five-number summary: minimum, first quartile (Q1), median (Q2), third quartile (Q3), and maximum. Boxplots visually depict the central tendency, variability, and potential outliers of your data.
When to Use a Boxplot
Boxplots are particularly valuable in scenarios where you need to compare distributions across multiple groups or identify outliers. They are beneficial when visualizing:
- Continuous data segmented into categories.
- Multiple datasets in a concise format.
When compared to histograms or scatter plots, boxplots offer a clearer summary of data properties without losing significant information.
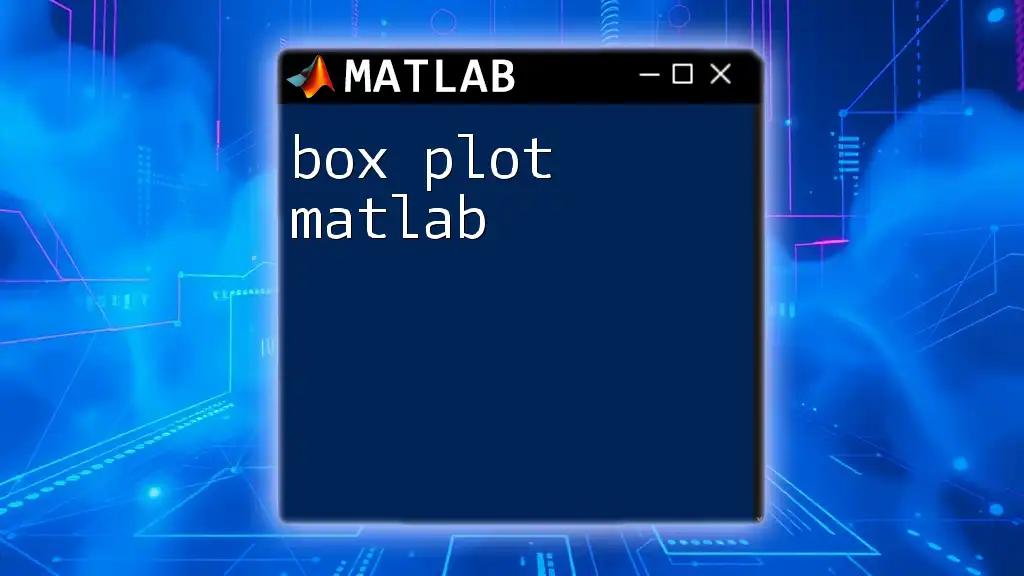
How to Create a Boxplot in MATLAB
Basic Syntax of the boxplot Function
To create a boxplot in MATLAB, you will primarily use the `boxplot` function. The most basic syntax looks like this:
boxplot(data)
Example 1: Simple Boxplot
Here's a straightforward example using randomly generated data.
data = randn(100, 1); % Generate random data
boxplot(data)
title('Simple Boxplot Example')
ylabel('Values')
In this example, we generate 100 random data points from a standard normal distribution. The resulting boxplot will visually summarize the data, indicating the median, quartiles, and any potential outliers present within the dataset.
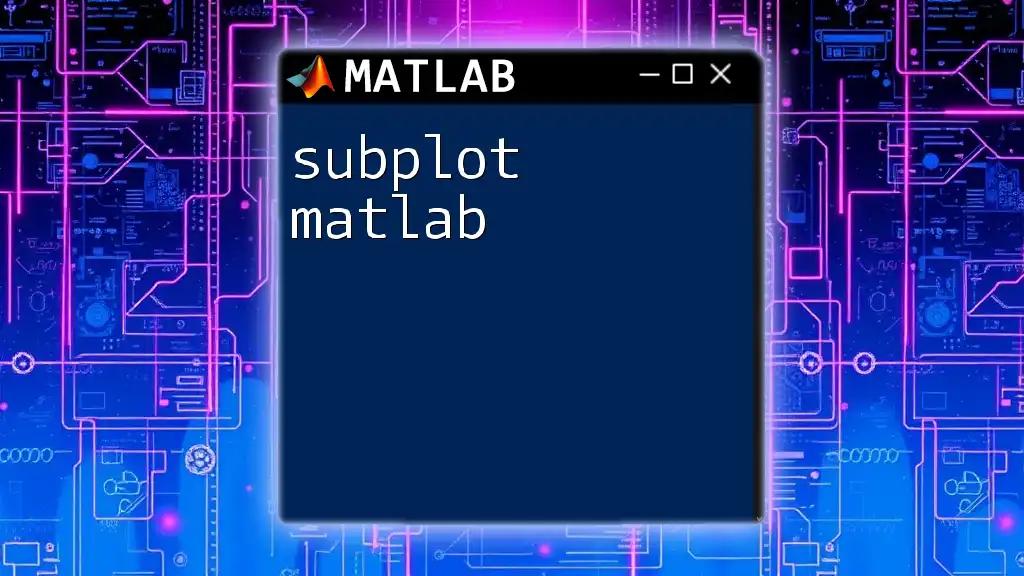
Customizing Boxplots
Adding Titles and Labels
To enhance the interpretability of your boxplot, it's important to add titles and axis labels.
boxplot(data)
title('Customized Boxplot')
xlabel('Groups')
ylabel('Data Values')
These enhancements help provide context to the viewer, making it easier to understand the data being presented.
Color Customization
You can also change the appearance of your boxplot by customizing the colors of the boxplot elements.
boxplot(data, 'Colors', 'r')
In this example, we set the color of the boxplot to red, which can be particularly useful when presenting data grouped by categories or when emphasizing specific datasets.
Grouping Data
Creating grouped boxplots allows for comparison across different categories.
group = [1 1 1 2 2 2]; % Example groups
boxplot(data, group)
This code snippet enables us to visualize how different groups behave compared to one another. The resulting boxplot will have separate boxes for each group in the data.
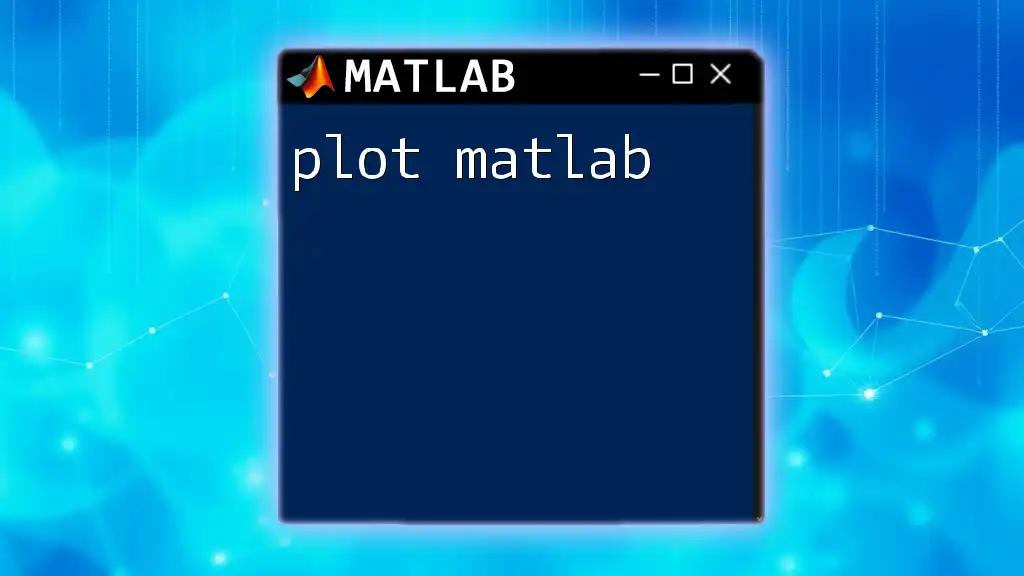
Advanced Features of Boxplots in MATLAB
Adding Data Points to Boxplots
To provide additional context, you can overlay individual data points on the boxplot. This can help highlight specific trends or patterns.
boxplot(data)
hold on
scatter(ones(size(data)), data, 'r.')
hold off
In this example, we use the `scatter` function to plot the individual data points on top of the boxplot, using red dots for visibility.
Handling Outliers
Outliers are typically represented as distinct points outside the whiskers of the boxplot. Understanding how MATLAB defines and visualizes outliers can significantly aid your analysis. You can customize threshold values to define what counts as an outlier, providing flexibility in your analysis.
Creating Boxplots for Multiple Datasets
To visualize multiple datasets together with boxplots, you can concatenate your data arrays.
data1 = randn(100, 1);
data2 = randn(100, 1) + 1; % Offset
boxplot([data1 data2], 'Labels', {'Dataset 1', 'Dataset 2'})
This code snippet creates a side-by-side boxplot comparison, allowing you to easily see differences in the distributions of the two datasets.
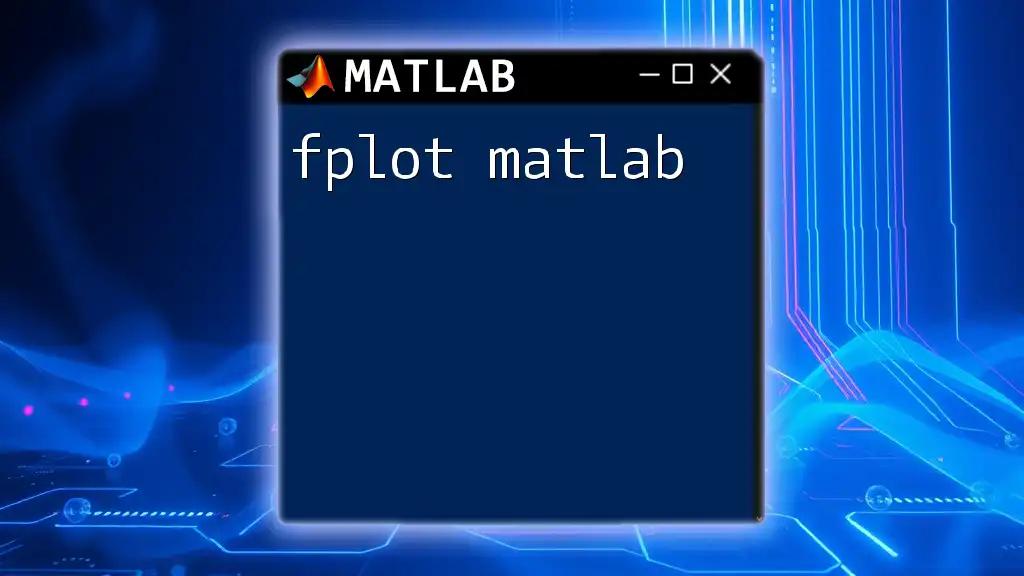
Interpreting Boxplots
Reading the Boxplot Components
Understanding how to read the various components of a boxplot is crucial.
- The line in the center of the box represents the median.
- The edges of the box represent the first and third quartiles (Q1 and Q3).
- The whiskers extend to show the range of the data, excluding outliers, while any points outside the whiskers are considered outliers.
Common Mistakes to Avoid
While interpreting boxplots, avoid common pitfalls such as:
- Misinterpreting outliers, which can skew the representation of your data.
- Neglecting to consider the spread of the data when comparing multiple boxplots.
Understanding the context and the data’s nature is essential for accurate analysis and interpretation.
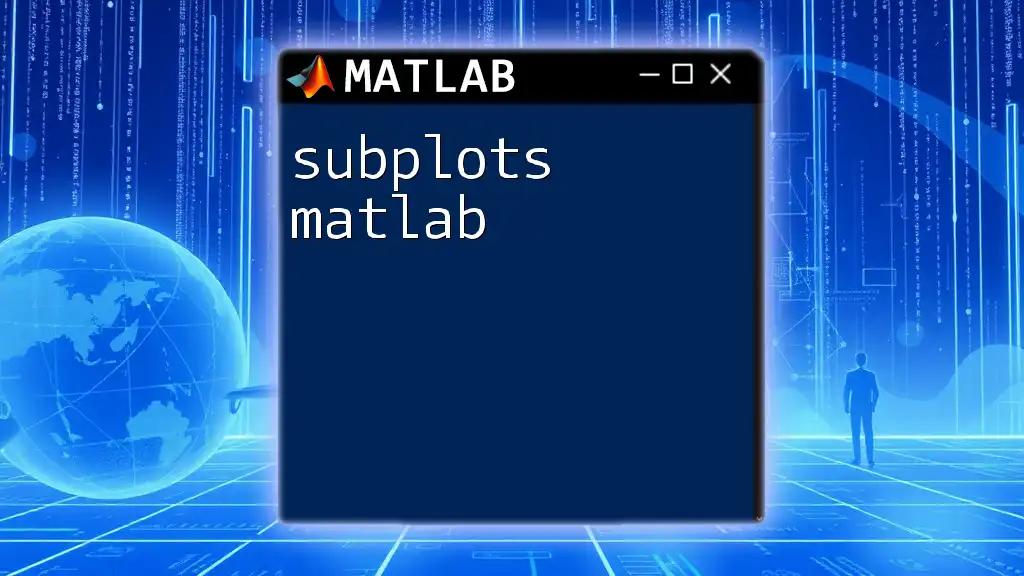
Conclusion
In this guide, we explored the boxplot in MATLAB, covering everything from basic creation to advanced features. Boxplots provide an effective means to visually summarize and compare datasets, making them an invaluable tool in data analysis. We encourage you to use the examples and customization options outlined here to experiment with your own datasets.
Explore how boxplots can enhance your data presentation and improve your analysis outcomes!
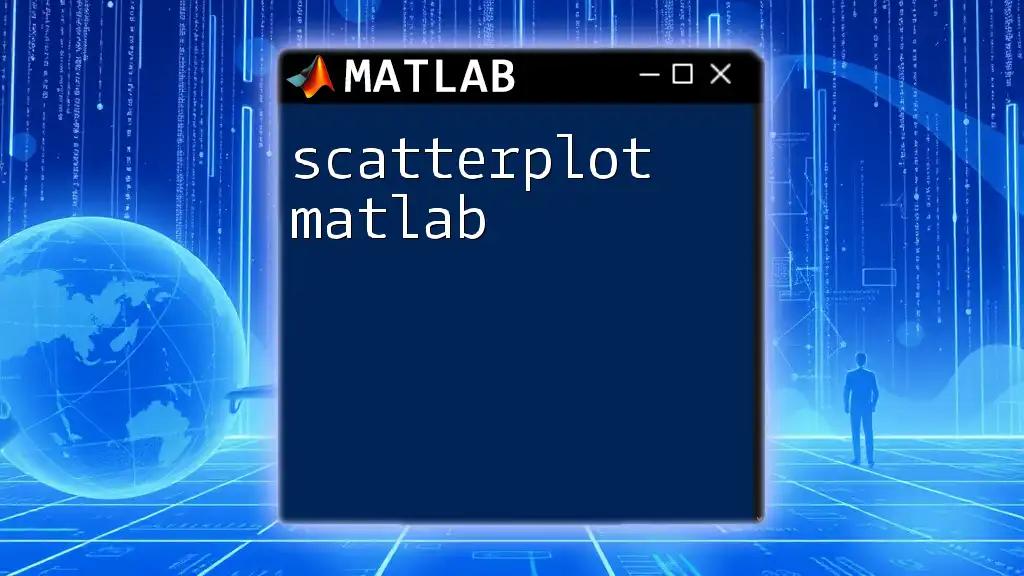
Additional Resources
Further Learning
For more in-depth knowledge, refer to the official [MATLAB documentation on `boxplot`](https://www.mathworks.com/help/stats/boxplot.html). Consider engaging with online courses or tutorials to deepen your MATLAB skills.
Community and Support
Join MATLAB user forums and online communities for additional support and shared learning experiences. These platforms can provide valuable insights and assistance as you navigate your data analysis journey.