The LU method in MATLAB is an algorithm used for factorizing a given matrix into a lower triangular matrix (L) and an upper triangular matrix (U), which can then be used to solve linear equations more efficiently.
% Example of LU decomposition using MATLAB
A = [4, 3; 6, 3];
[L, U] = lu(A);
disp('Lower triangular matrix L:');
disp(L);
disp('Upper triangular matrix U:');
disp(U);
Understanding LU Decomposition
Definition and Purpose
LU decomposition is a method used in linear algebra to factor a matrix into the product of two simpler matrices: a lower triangular matrix \( L \) and an upper triangular matrix \( U \). The decomposed form is often denoted as:
\[ A = LU \]
This decomposition is valuable because it simplifies solving systems of linear equations, calculating determinants, and finding inverses of matrices.
Applications of LU Decomposition
LU decomposition is applicable in numerous fields, such as:
- Engineering: Used for structural analysis and fluid dynamics.
- Computer Science: Key in algorithms for numerical computations.
- Data Analysis: Leveraged for solving large systems of equations that arise during modeling and data fitting.
The Mathematics Behind LU Decomposition
Mathematical Representation
In its simplest form, the LU decomposition expresses any square matrix \( A \) as the product of a lower triangular matrix \( L \) (where all elements above the main diagonal are zero) and an upper triangular matrix \( U \) (where all elements below the main diagonal are zero). The factors \( L \) and \( U \) can be computed using various methods, including Gaussian elimination.
Conditions for LU Decomposition
Not all matrices can be decomposed in this manner. Key requirements include:
- Square Matrix: LU decomposition is typically performed on square matrices. For non-square matrices, you might explore other factorizations like QR decomposition.
- Non-Singular Matrix: The matrix \( A \) must be non-singular (i.e., it must have a non-zero determinant) for decomposition without pivoting.
- Presence of Zeros: Matrices with strategically placed zeros may require partial pivoting to ensure stability and accuracy.
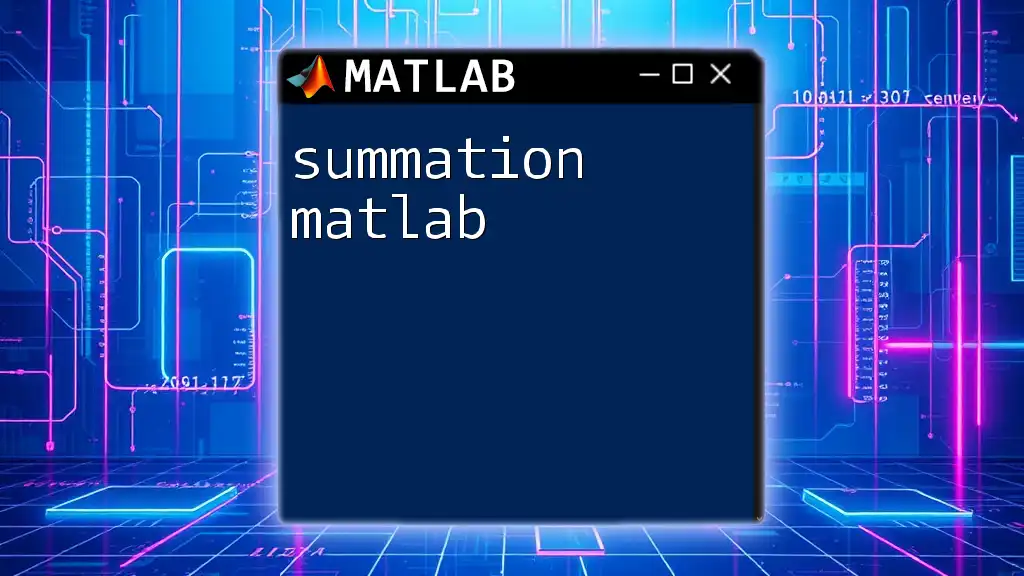
Implementing LU Decomposition in MATLAB
Using the `lu` Function
MATLAB offers built-in functionality for LU decomposition through its `lu` function. The basic syntax to decompose a matrix \( A \) is as follows:
A = [4, 3; 6, 3];
[L, U] = lu(A);
Understanding the Outputs
After executing this command, MATLAB returns two matrices:
- \( L \): The lower triangular matrix with elements below the main diagonal filled and diagonal elements typically set to one.
- \( U \): The upper triangular matrix with all elements below the main diagonal equal to zero.
Additional Options of the `lu` Function
Pivoting
To enhance the stability and performance of the decomposition, MATLAB supports pivoting, which rearranges the rows of the matrix. This can be particularly beneficial in avoiding numerical issues.
Using pivoting with the `lu` function is straightforward:
[L, U, P] = lu(A);
Here, \( P \) is the permutation matrix that indicates the row swaps made during decomposition. This is crucial in maintaining numeric stability during the computation.
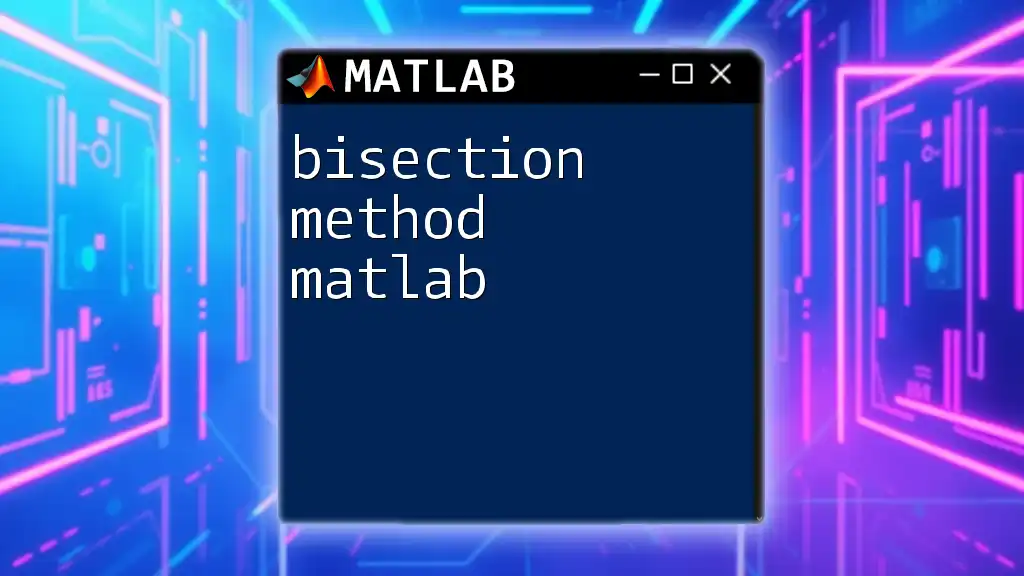
Practical Examples
Example 1: Solving a Linear System
Consider a linear system represented in matrix form \( Ax = b \), where \( A \) is the coefficient matrix and \( b \) is the result vector. To solve such systems using LU decomposition, follow these steps:
- Define the Matrix and Vector:
A = [3, 2; 1, 2];
b = [5; 4];
- Perform LU Decomposition:
[L, U] = lu(A);
- Solve for \( y \) (Intermediate Step):
You will first solve the equation \( Ly = b \) using forward substitution. In MATLAB, this can be done simply by:
y = L\b;
- Solve for \( x \):
Next, substitute \( y \) into the equation \( Ux = y \) using back substitution:
x = U\y;
At this point, \( x \) contains the solutions to the linear equations.
Example 2: Performance Comparison
When deciding between various numerical techniques for solving linear systems, it's beneficial to consider performance. LU decomposition often outperforms direct methods like Gaussian elimination, particularly for systems requiring multiple solves with the same coefficient matrix.
To measure execution time, use the `tic` and `toc` functions:
A = rand(1000); % Generate a large random matrix
b = rand(1000, 1);
tic;
[L, U] = lu(A);
toc;
This will provide insight into how efficient the LU method is compared to directly solving the system.
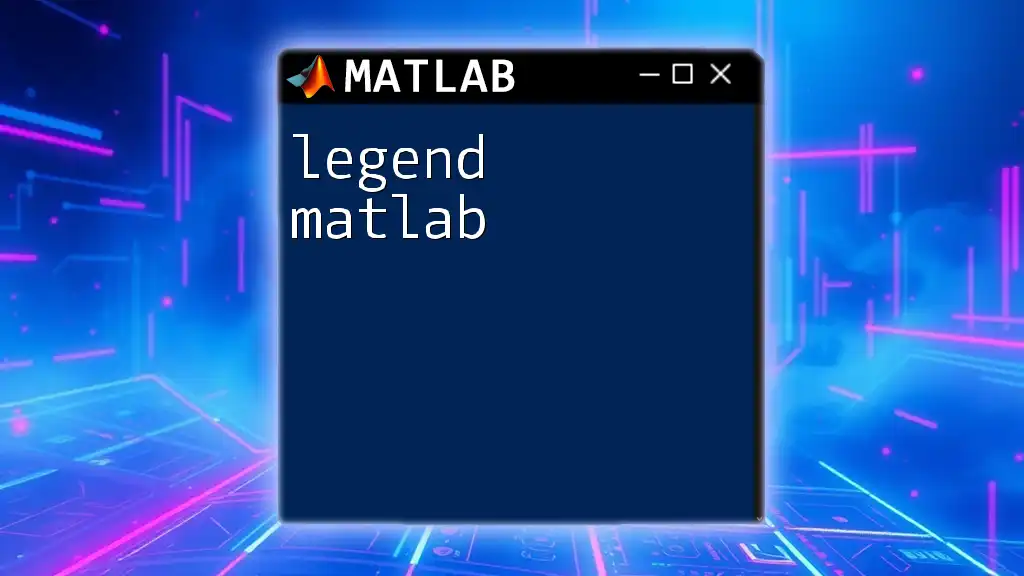
Troubleshooting Common Issues
Non-Decomposable Matrices
If you attempt to perform LU decomposition on a matrix that is not suitable, such as a singular matrix, MATLAB may either return an error or provide a warning. Always check the condition of your matrix before decomposition to avoid any pitfalls.
Using Try-Catch for Robustness
To handle potential errors gracefully, implement a try-catch statement in your MATLAB code. This not only helps in debugging but also ensures your program does not crash:
try
[L, U] = lu(A);
catch ME
disp('LU decomposition failed:');
disp(ME.message);
end
This adds robustness to your code, allowing you to manage unexpected behavior effectively.
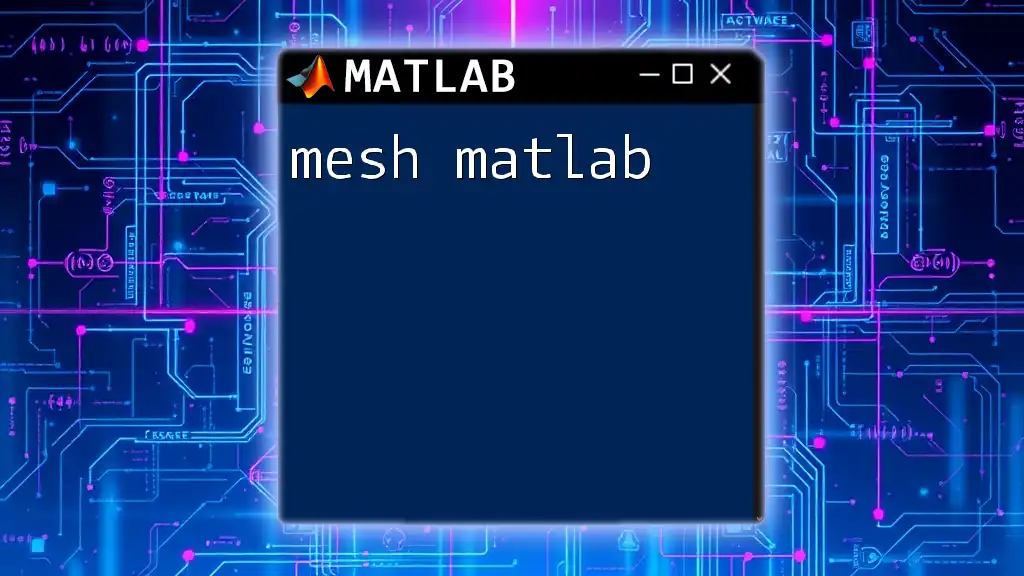
Conclusion
In summary, the LU method in MATLAB provides an efficient and reliable way to decompose matrices for various applications in linear algebra. By understanding the basic syntax, practical applications, and troubleshooting techniques, you can harness the power of LU decomposition to solve linear systems and analyze numerical data effectively. The hands-on examples presented not only demonstrate the implementation but also encourage further practice, ensuring a solid foundation in using MATLAB for mathematical computations.
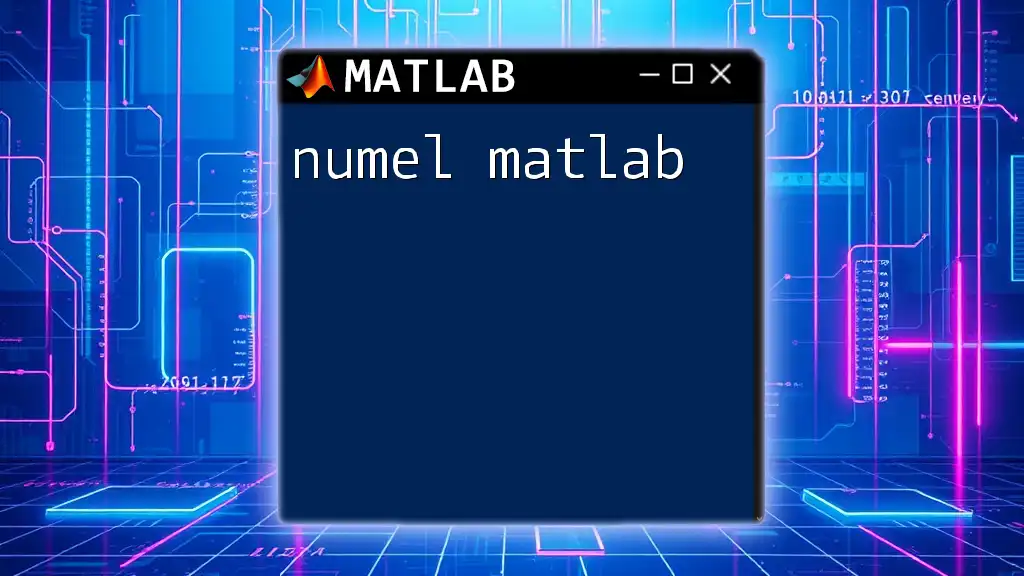
Additional Resources
For further reading and exploration, consider visiting the following MATLAB documentation and tutorials that delve deeper into the `lu` function and linear algebra principles. This will provide you with more extensive insights and practical applications to enrich your understanding and skills.