The `plot3d` command in MATLAB is used to create three-dimensional plots of functions or data points using Cartesian coordinates.
[X, Y] = meshgrid(-5:0.5:5, -5:0.5:5); % Create a grid of points
Z = sin(sqrt(X.^2 + Y.^2)); % Define a function for Z values
plot3(X, Y, Z); % Create a 3D plot
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
title('3D Surface Plot');
Understanding the Basics of 3D Plotting
What is 3D Plotting?
3D plotting is an essential method of visualizing data that extends beyond the traditional two dimensions. In various fields such as engineering, physics, and data science, being able to represent complex relationships in three dimensions allows for a more comprehensive understanding of the underlying phenomena. While 2D plots are limited to displaying relationships using only two axes (x and y), 3D plots add a third axis (z), which creates a spatial relationship and helps convey additional data insights.
Key Concepts and Terminology
Understanding a few key concepts is fundamental when working with `plot3d` in MATLAB:
- 3D Axes: The three dimensions in a 3D plot are represented by the x, y, and z axes. Each axis corresponds to a different variable in your dataset, allowing for multidimensional visualization.
- Data Points: In 3D space, each data point is represented by a triplet of coordinates (x, y, z), which collectively provide the plot's spatial position.
- Coordinate Systems: The Cartesian coordinate system is most commonly used in 3D plots. It organizes points in a space defined by the three axes.
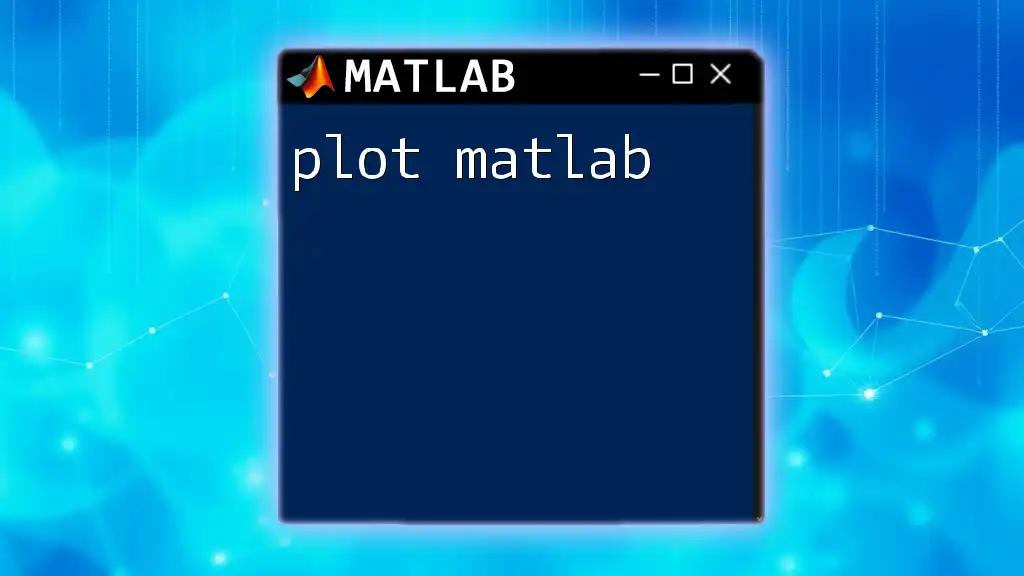
MATLAB Commands for 3D Plotting
Overview of Basic Plotting Functions
In MATLAB, the `plot3` function is the cornerstone of 3D plotting. However, it interacts effectively with several other commands that extend its capabilities:
- `scatter3`: Produces a 3D scatter plot, emphasizing the configuration of data points.
- `surf`: Creates surface plots that visualize the surface defined by z-values over a grid of x and y values.
- `mesh`: Similar to `surf`, but displays a wireframe representation of the surface.
Syntax of `plot3`
The syntax of `plot3` is straightforward, making it accessible for users at all skill levels. The basic format is:
plot3(X, Y, Z, LineSpec)
Here:
- X, Y, Z are vectors representing coordinates in the three-dimensional space.
- LineSpec specifies the appearance of the line, such as color, marker, and line style.
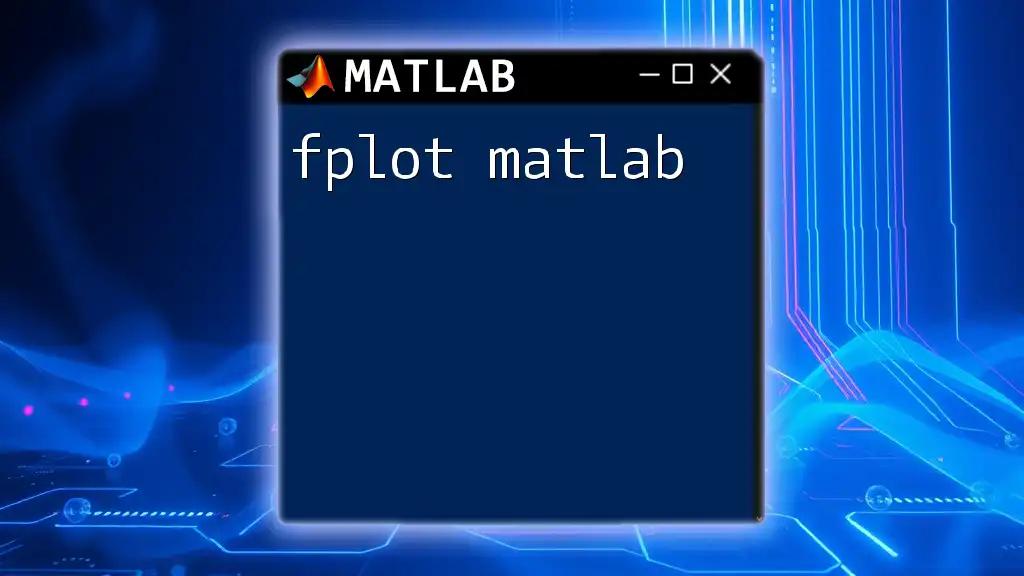
Creating Your First 3D Plot
Sample Data Creation
Before plotting, it is essential to have suitable data. Here’s how to create a simple dataset:
t = 0:0.1:10;
x = sin(t);
y = cos(t);
z = t;
In this example:
- `t` spans from 0 to 10 with increments of 0.1, acting as our time variable.
- `x` and `y` represent sine and cosine functions, respectively, while `z` is simply t.
Plotting the Data
Once the data has been defined, plotting is straightforward. Use the following code:
plot3(x, y, z, 'r-o'); % Plot with red circles and lines
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
title('3D Plot of Sine and Cosine Waves');
grid on;
This code enables the following features:
- Red circles with connecting lines for visual distinction.
- Axes labels and a title to help interpret the plot.
- A grid to enhance visual clarity and facilitate data reading.
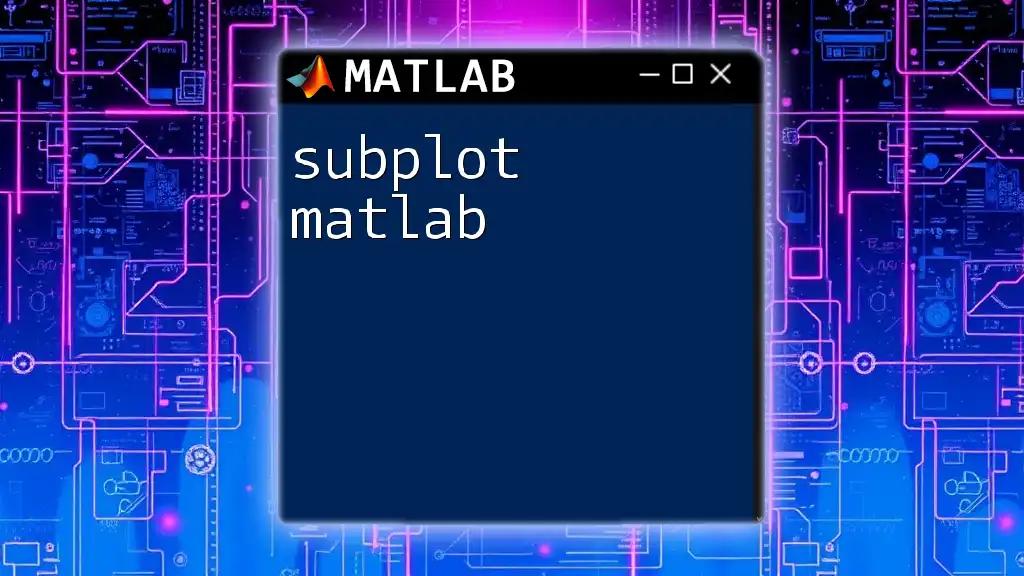
Customizing Your 3D Plot
Adding Labels and Titles
Effective communication through visualizations is crucial. Proper labeling aids in understanding the data being represented. Here’s how to implement it:
xlabel('Sine Value');
ylabel('Cosine Value');
zlabel('Time');
title('3D Representation of Sine and Cosine Over Time');
By using these commands, you provide necessary context for anyone interpreting the graph.
Styling the Plot
To further enhance the visual appeal and effectiveness of a plot, you can modify its style. Here’s how to adjust color, line style, and markers:
plot3(x, y, z, 'b--', 'LineWidth', 2); % Blue dashed line
This example sets the plot to display a thicker blue dashed line, effectively improving clarity.
Adding Grid and View Adjustments
To make the data easier to read, including a grid and adjusting the viewing angle can be beneficial:
grid on;
view(45, 30); % Change viewing angle
This command enables the grid for reference and modifies the angle of view to capture a better perspective of the data.
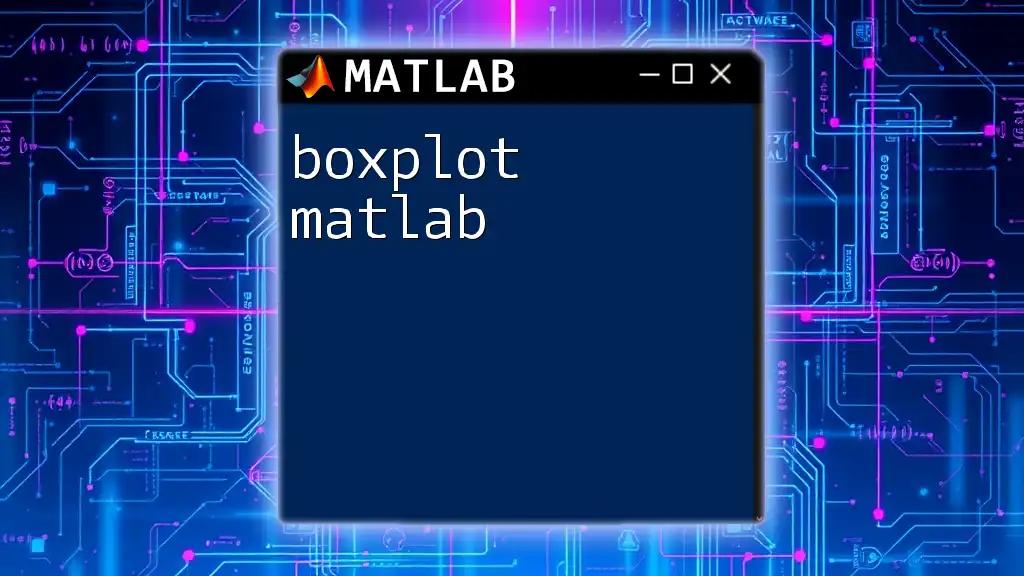
Advanced 3D Plotting Techniques
Creating 3D Mesh and Surface Plots
For more intricate visualizations, the `mesh` and `surf` functions come into play. These functions are particularly useful for representing mathematical surfaces. Here’s how to create a basic surface plot:
[X, Y] = meshgrid(-5:0.5:5, -5:0.5:5);
Z = sin(sqrt(X.^2 + Y.^2));
surf(X, Y, Z);
In this code:
- `meshgrid` generates a grid of x-y coordinates over the specified range, and `Z` computes values based on a function of `X` and `Y`.
- The `surf` function then plots these values in a visually impactful way, allowing for a deeper analysis of relationships between the three dimensions.
Animating 3D Plots
Animating plots can dramatically enhance visual storytelling. Here’s a simple animation example:
for k = 1:100
z = sin(x + k/10); % Update Z data for animation
plot3(x, y, z); % Re-plot with updated Z
pause(0.1); % Pause for illustration
end
In this loop, the z-values are iteratively updated, creating a dynamic movement effect that could be valuable for demonstrating time-varying phenomena or trends.
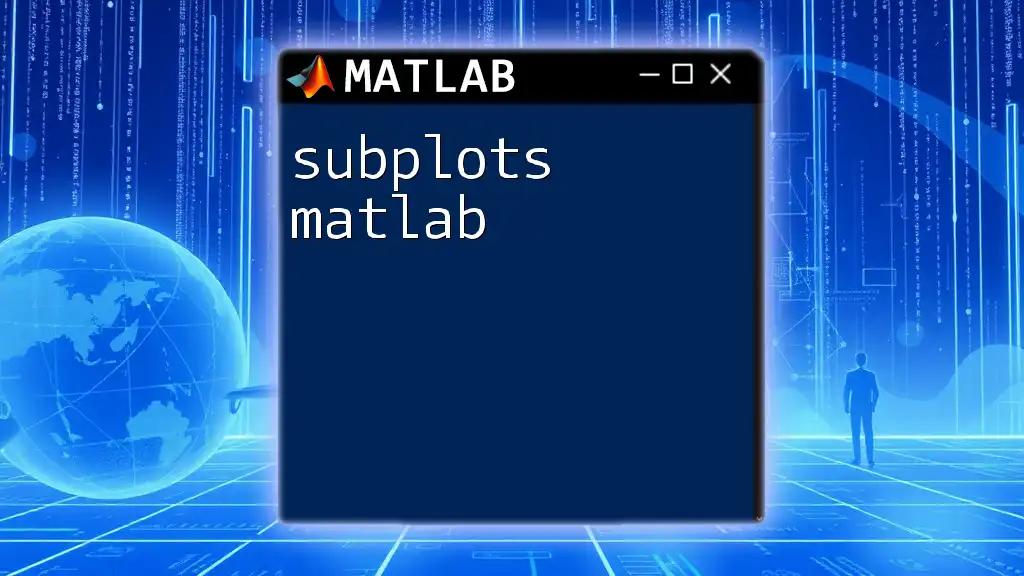
Tips and Best Practices for Effective 3D Plotting
Choosing the Right Visualization
Selecting the appropriate type of 3D plot is vital. Consider the nature of your data and the insights you wish to convey. For instance, a surface plot suitable for representing continuous data variation may not be ideal for discrete data, where a scatter plot might be more effective.
Avoiding Common Pitfalls
When it comes to 3D plotting, less can often be more. Overly complex plots can clutter the presentation and be challenging to interpret. Aim for clarity by avoiding unnecessary elements that do not contribute to the understanding of the data.
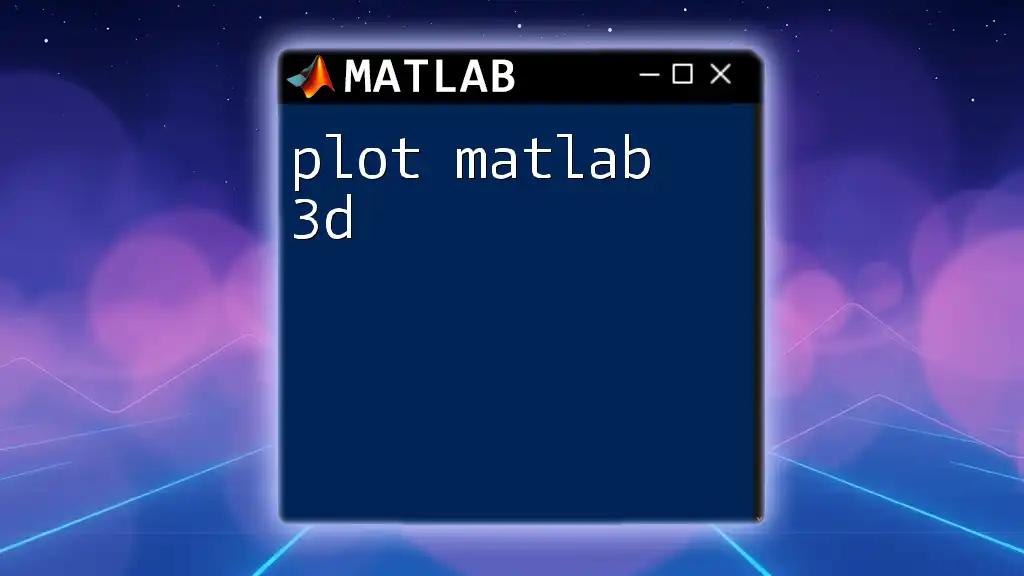
Conclusion
Mastering the `plot3d matlab` commands is crucial for anyone aiming to leverage MATLAB’s powerful capabilities in data visualization. Understanding the basics of 3D plotting, utilizing various commands, and customizing plots will not only enhance your presentations but also provide valuable insights into your data. Practice using these techniques will undoubtedly lead to improved data communication.
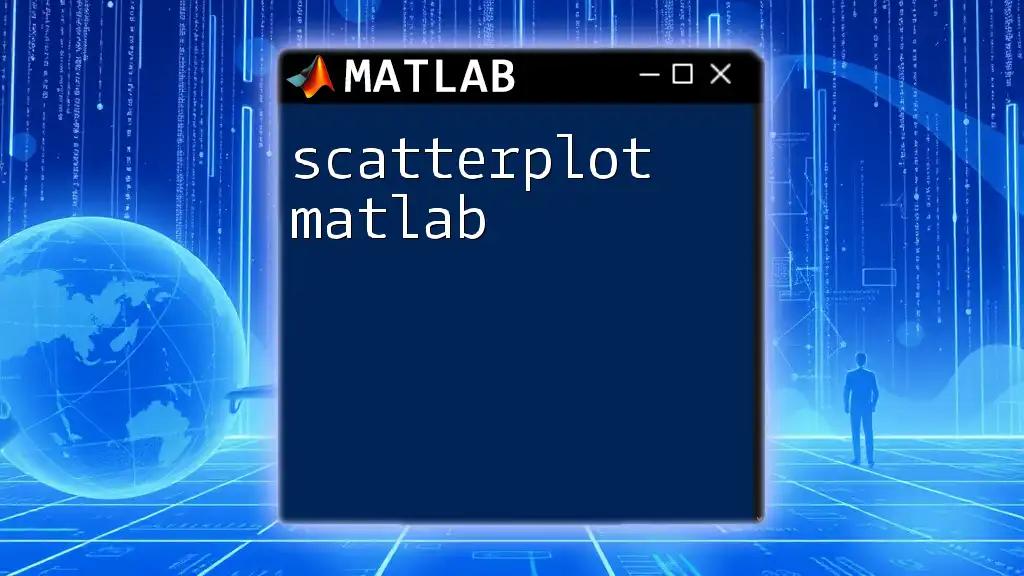
Further Resources
Explore the official MATLAB documentation for additional functions and detailed explanations of plotting techniques. Consider joining MATLAB community forums for further learning and to share preferences and insights with fellow enthusiasts.