MATLAB 2023b is the latest release of MATLAB that continues to enhance data analysis, visualization, and algorithm development with new features and improvements for users to explore.
Here’s a simple example of creating a plot in MATLAB:
x = 0:0.1:10; % Generates a vector from 0 to 10 with increments of 0.1
y = sin(x); % Calculates the sine of each x value
plot(x, y); % Plots the sine wave
title('Sine Wave'); % Adds a title to the plot
xlabel('X-axis'); % Labels the x-axis
ylabel('Y-axis'); % Labels the y-axis
Major New Features in MATLAB 2023b
Enhanced Visualizations
One of the standout features of MATLAB 2023b is the significant improvements made to the visualization capabilities. Users can now take advantage of enhanced plotting functions that allow for more intricate and visually compelling presentations of data. The introduction of new graphics options improves how we represent multiple dimensions of data effectively.
For example, creating a compelling 3D plot with enhanced aesthetics has become simpler. Here’s a code snippet that demonstrates how to do this:
x = linspace(-5, 5, 100);
y = linspace(-5, 5, 100);
[X, Y] = meshgrid(x, y);
Z = sin(sqrt(X.^2 + Y.^2));
surf(X, Y, Z, 'EdgeColor', 'none', 'FaceColor', 'interp');
colorbar;
title('Enhanced 3D Surface Plot');
In this example, we performed a 3D surface plot with interpolated face colors and no edge colors, making for a cleaner and more visually appealing display. Such enhancements are pivotal in fields that require data visualization for analysis and presentation.
Improved User Interface
The user interface has also received a noteworthy update. The MATLAB 2023b desktop environment offers greater usability and accessibility. Key enhancements streamline navigation and make it easier to manage multiple scripts and projects. The toolbar layout has been restructured for improved access to frequently used tools, improving user workflow.
With these updates, users can quickly locate features and tools, allowing for a more intuitive programming experience. Take the time to explore these new UI features—they are designed to make your coding journey smoother and more efficient.
New Functions and Toolboxes
MATLAB 2023b brings exciting new functions in the core language as well as updated toolboxes. From new command options to added functionalities that enhance existing capabilities, users will find many valuable resources at their fingertips.
An important addition is in the Statistics Toolbox, which introduces new statistical functions to facilitate data analysis. Here's an example of using a new statistical fitting function:
data = randn(1000, 1);
[mu, sigma] = normfit(data);
fprintf('Mean: %f, Standard Deviation: %f\n', mu, sigma);
In this example, we generated random data and then used the `normfit` function to estimate the mean and standard deviation. Such analytics will be beneficial for users working in research, finance, and data science.
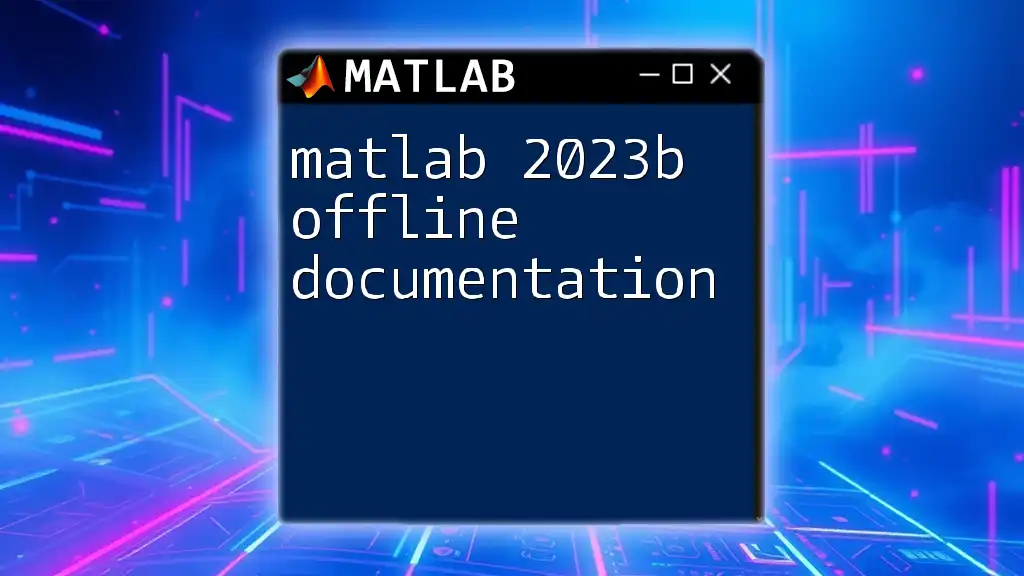
Getting Started with MATLAB 2023b
Installation and Setup
Before diving into MATLAB 2023b, ensure your system meets the necessary requirements. The installation process is straightforward and includes key steps:
- Download the installer from the MathWorks website.
- Follow the on-screen instructions to install the software.
- Ensure you activate your software license by entering your credentials.
First Steps in the MATLAB Environment
Once installed, it’s time to familiarize yourself with the environment. The Command Window allows you to enter commands directly, while the Workspace displays variables you've created.
Creating and saving scripts is crucial for organization. A simple script might look as follows:
% This script calculates the Fibonacci sequence
n = 10; % Length of Fibonacci sequence
fib = zeros(1, n);
fib(1) = 0; fib(2) = 1;
for i = 3:n
fib(i) = fib(i-1) + fib(i-2);
end
disp(fib);
This script initializes an array and computes the Fibonacci sequence for the first ten numbers, showcasing basic scripting functionalities in MATLAB.
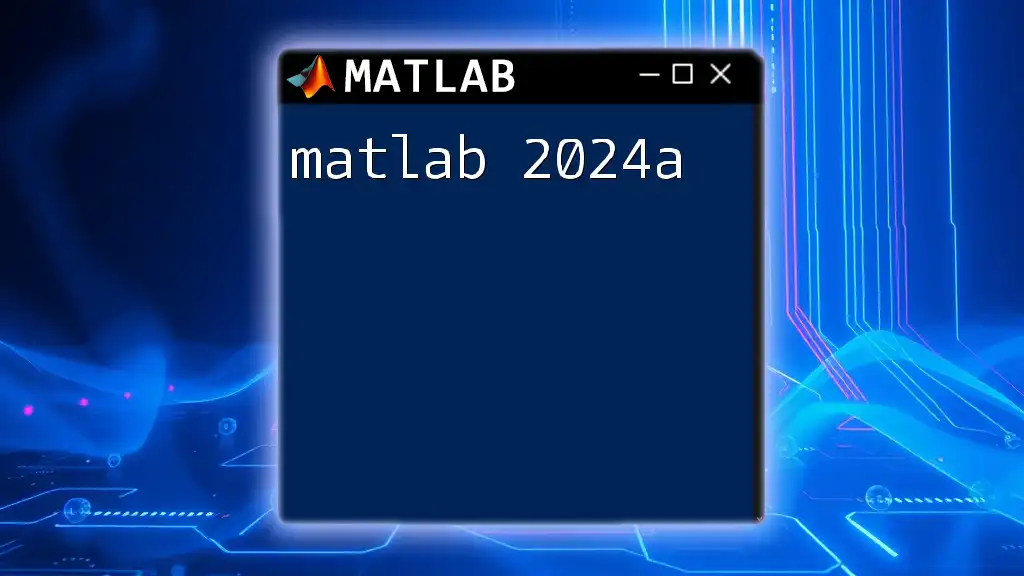
Advanced MATLAB 2023b Techniques
Custom Functions and Scripts
Creating your own functions is one of the most powerful features of MATLAB 2023b. Custom functions allow for reusable code components that can simplify complex calculations. Structuring your code properly is crucial for effective programming and collaboration.
For instance, here’s how you might write a custom function to calculate the factorial of a number:
function f = factorial(n)
if n == 0
f = 1;
else
f = n * factorial(n - 1);
end
end
This recursive function is a classic programming exercise that serves as a building block for more advanced topics in programming and mathematics.
Leveraging Built-in Functions
MATLAB is renowned for its extensive library of built-in functions, which significantly enhance the performance of operations like matrix calculations. Improving your skills in using these functions can help you write more efficient and cleaner code.
Here’s an example that demonstrates the efficiency of matrix operations in MATLAB:
A = rand(1000);
B = rand(1000);
C = A * B; % Efficient matrix multiplication
In this code snippet, we create two large matrices and multiply them with optimized underlying algorithms, highlighting MATLAB's strength in handling matrix operations.
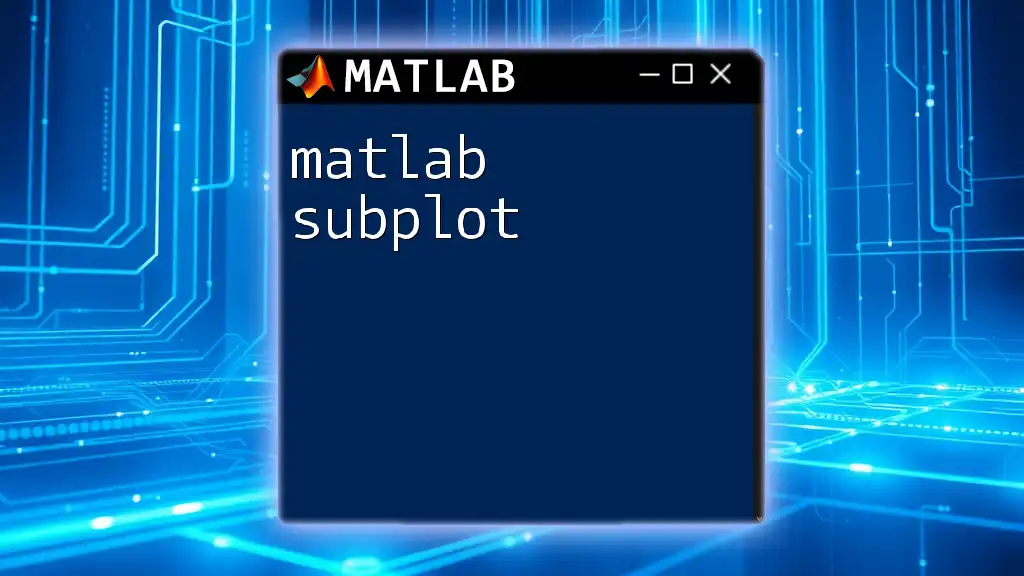
Getting Help and Resources
MATLAB Documentation
The official MATLAB documentation is an invaluable resource for all users. With detailed explanations, examples, and troubleshooting tips, it serves as a comprehensive guide to navigating any issues you may encounter while using MATLAB 2023b.
Community and Forums
Engaging with the MATLAB Central community provides not only support but also access to a wealth of user-contributed functions, files, and tutorials. It’s an excellent way to expand your knowledge and learn from others’ experiences.
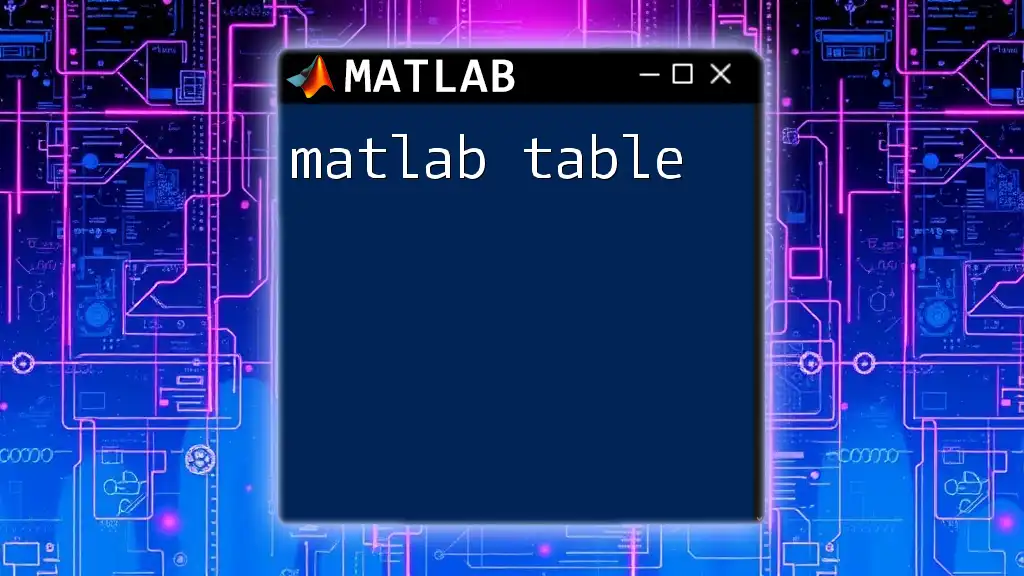
Real-world Applications of MATLAB 2023b
Engineering Applications
In various engineering fields, MATLAB 2023b finds applications ranging from signal processing to control systems. For instance, engineers often use MATLAB for modeling and simulating complex systems, allowing for predictive analysis and optimization of designs.
Data Science and Machine Learning
The role of MATLAB in data science and machine learning has grown significantly. With its robust toolboxes, users can easily implement data preprocessing, analysis, and modeling. Here’s an example of fitting a simple linear model using MATLAB:
% Load the Fisher Iris dataset and fit a linear model
load fisheriris;
mdl = fitlm(meas(:,1), species);
This example demonstrates how easily MATLAB can be used for predictive modeling and classification. Such capabilities make it a go-to choice for those working in data-heavy fields.
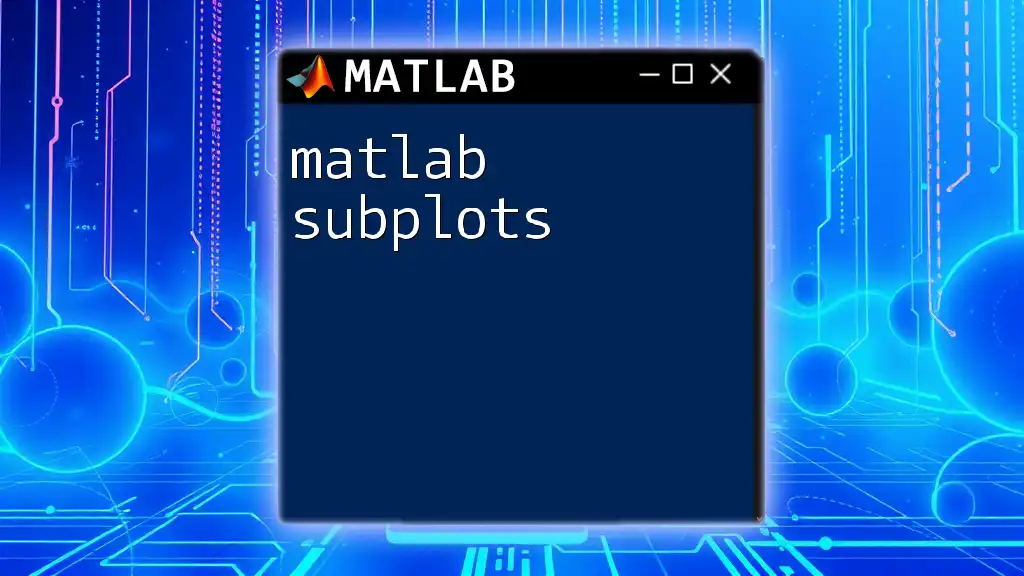
Conclusion
With its plethora of new features and enhancements, MATLAB 2023b continues to offer powerful tools for both novice and experienced users. Whether you're focusing on visualizations, custom functions, or navigating the user-friendly interface, this latest version is designed to facilitate advanced coding and data analysis.
Explore the exciting updates and vast functionalities it brings to your programming toolkit, and engage with the vibrant MATLAB community to enhance your learning experience. Whether you are an engineer, scientist, or data analyst, mastering MATLAB can profoundly impact your work and efficiency.
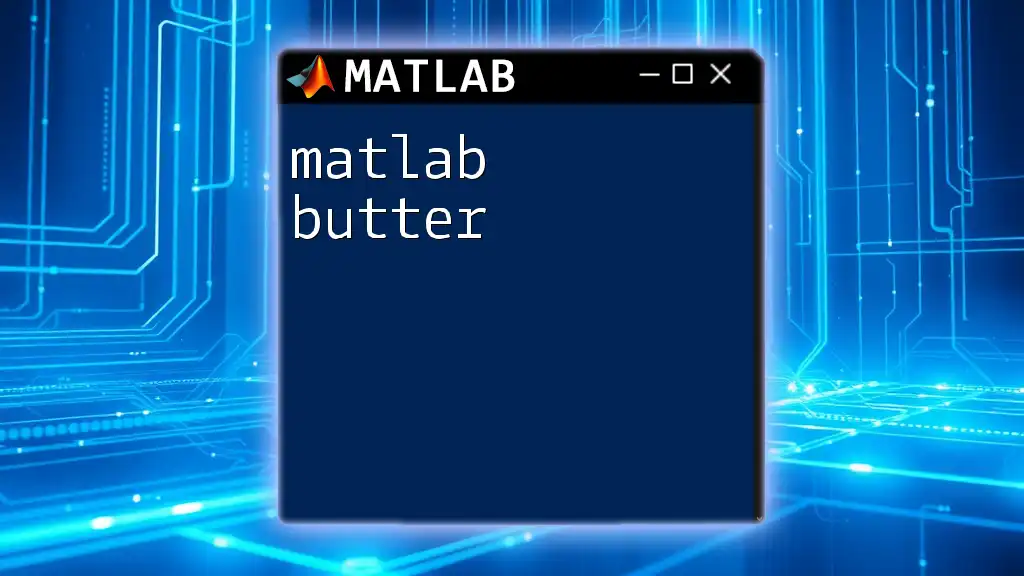
Call-to-Action
If you’re eager to dive deeper into the capabilities of MATLAB 2023b, consider signing up for our hands-on courses where you can gain practical experience with the software. Join us to unlock your potential in programming and data analysis with MATLAB!