MATLAB markers are specific symbols used to denote data points in plots, enabling users to visually distinguish between different datasets or categories.
Here’s an example of how to use markers in a MATLAB plot:
x = 1:10; % X data
y = rand(1, 10); % Y data with random values
plot(x, y, 'o-r', 'MarkerSize', 10, 'LineWidth', 2); % Plot with circle markers and red line
title('Example of MATLAB Markers');
xlabel('X-axis');
ylabel('Y-axis');
grid on;
Understanding MATLAB Markers
What are Markers?
In MATLAB, markers are essential visual symbols or shapes that represent data points in plots. They serve a dual purpose: not only do they help visualize individual data points, but they also enhance the overall clarity of plots by differentiating between various datasets. Utilizing markers effectively can significantly improve the interpretability of your visualizations, making it easier to convey insights from the data.
Types of Markers Available in MATLAB
MATLAB provides a rich variety of marker types to choose from, allowing for creative and informative visualizations. Some of the commonly used markers include:
- Circle: Represented by `'o'`
- Square: Represented by `'s'`
- Diamond: Represented by `'*'`
- Upward Triangle: Represented by `'^'`
- Downward Triangle: Represented by `'v'`
- Pentagram: Represented by `'p'`
- Hexagram: Represented by `'h'`
You can create a variable to see these markers in action:
markers = {'o', 's', '*', '^', 'd', 'p', 'h'};
disp(markers);
Marker Properties
Markers can be customized extensively through various properties:
- MarkerSize: Controls the size of the markers. A larger size can make markers more prominent, whereas a smaller size may be appropriate for dense data.
- MarkerEdgeColor: Changes the outline color of the marker, which can help distinguish between different data series.
- MarkerFaceColor: Fills the marker with a specific color, enhancing visibility and aesthetics in your plot.
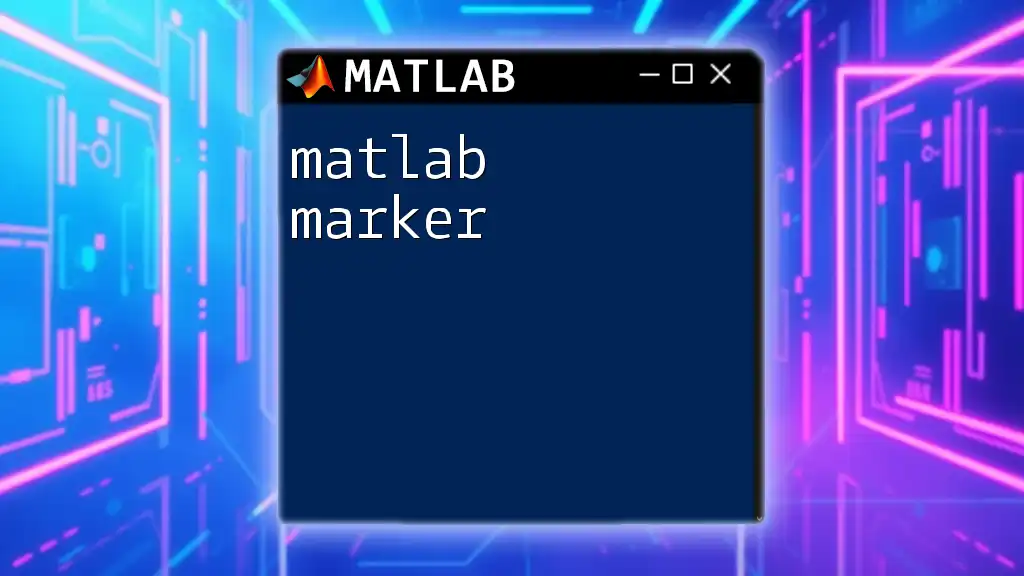
Creating Basic Plots with Markers
Basic Plotting with Markers
Creating a simple plot with markers is straightforward. Here’s an example of how to plot data points using circle markers:
x = 1:10; % Define the x-data
y = rand(1, 10); % Generate 10 random y data points
plot(x, y, 'o'); % Plot with circle markers
title('Basic Plot with Circle Markers');
xlabel('X-axis');
ylabel('Y-axis');
This code will generate a scatter plot where each point is represented by a circle, showcasing the random data.
Customizing Marker Appearance
Adjusting Marker Size
To highlight or minimize certain data points, you can adjust marker size using the `MarkerSize` property. For example:
plot(x, y, 'o', 'MarkerSize', 10);
This will plot the same data with larger circle markers, making them more noticeable.
Changing Marker Color
Markers can also be stylized by changing their colors. You can use predefined colors or custom RGB values. Here’s how to do it:
plot(x, y, 'o', 'MarkerEdgeColor', 'r', 'MarkerFaceColor', 'b');
In this code, the edge of the marker will be red and the face will be blue, creating a striking visual contrast.
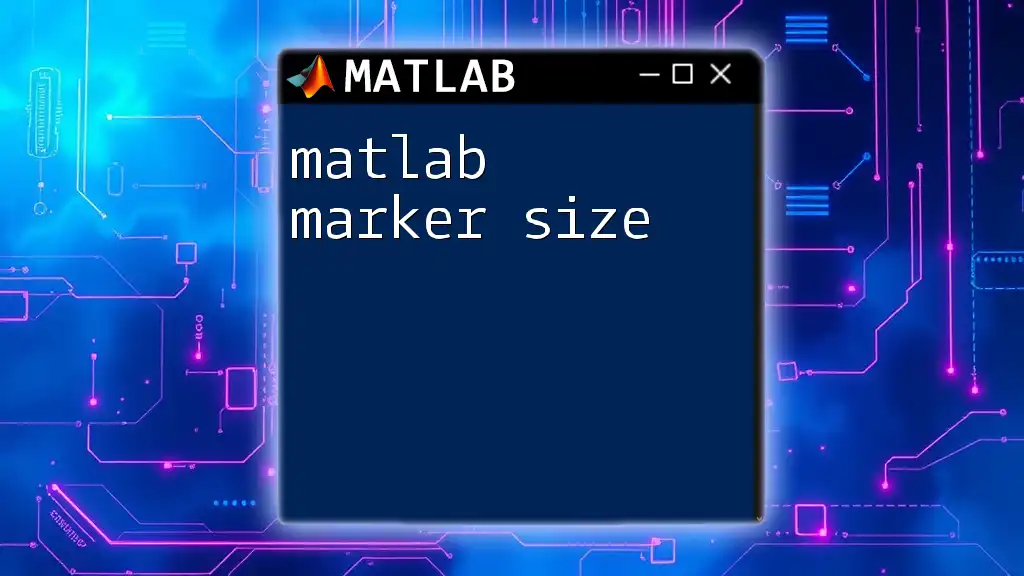
Advanced Marker Usage
Using Multiple Markers in One Plot
When dealing with multiple datasets, it’s essential to differentiate them. You can plot multiple datasets using different markers:
y2 = rand(1, 10) * 0.5; % Generate a second dataset with different range
plot(x, y, 'o', x, y2, 's');
legend('Dataset 1', 'Dataset 2');
In this example, the first dataset is represented with circular markers while the second uses square markers, making it easy for viewers to distinguish between the two.
Marker Visibility
Transparent Markers
For plots with overlapping data points, using transparent markers can help improve visibility. You can emulate this effect using:
plot(x, y, 'o', 'MarkerFaceAlpha', 0.5);
Here, the markers will be semi-transparent, allowing overlapping points to remain visible and enhance the viewer's understanding of data density.
Overlapping Markers
When markers overlap significantly, consider adjusting marker size, color, or transparency as mentioned above. Each of these techniques aids in preventing the loss of data clarity.
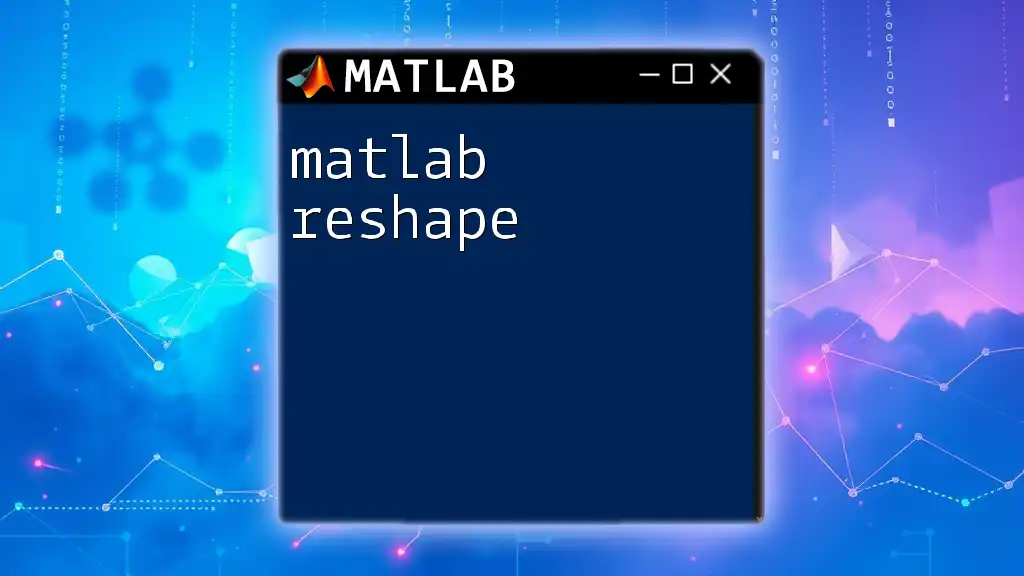
Combining Markers with Lines
Adding Lines to Marker Plots
To provide a clearer visual connection between data points, we can combine markers with lines. Here’s a code example:
plot(x, y, '-o', 'MarkerFaceColor', 'r');
This will plot the data points as red circles connected by lines, enhancing the understanding of trends in the data.
Customizing Line Styles and Colors
MATLAB allows for further customization of lines accompanying markers. You can specify line styles and colors alongside your markers, like this:
plot(x, y, 'r--o', 'MarkerFaceColor', 'g'); % Dashed red line with green filled markers
In this case, the connection between points is made with a dashed line, providing additional context without overwhelming the plot visually.
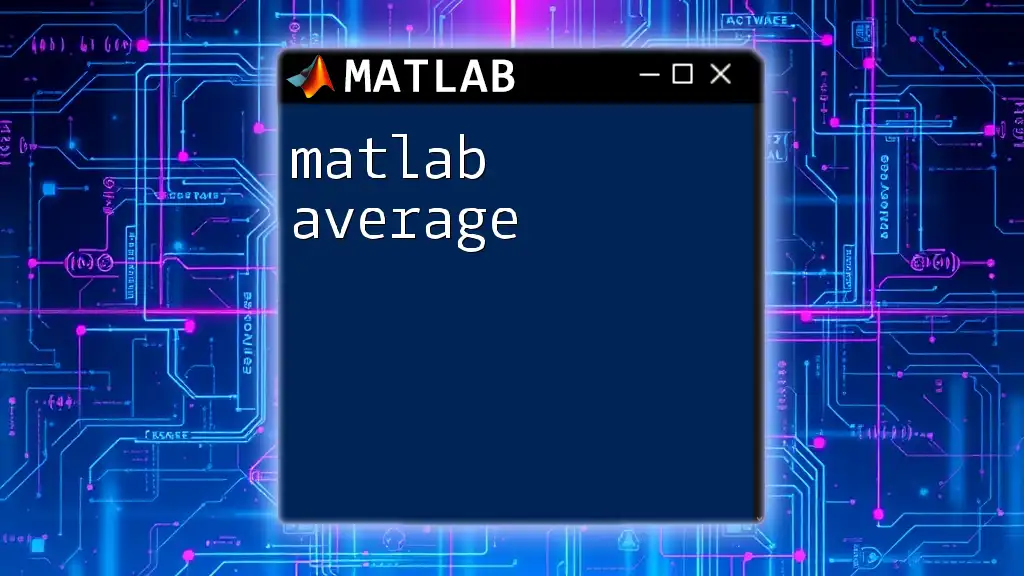
Special Marker Types
Using Custom Markers
MATLAB allows you to create custom markers. You can use images or different shapes as markers by specifying them in the plot command.
Marker Grouping and Legends
For clarity in complex plots, using legends is crucial. Ensure each set of markers corresponds to its appropriate label, facilitating better data interpretation. Use the `legend` function strategically to enhance clarity:
legend('Data Set 1', 'Data Set 2');
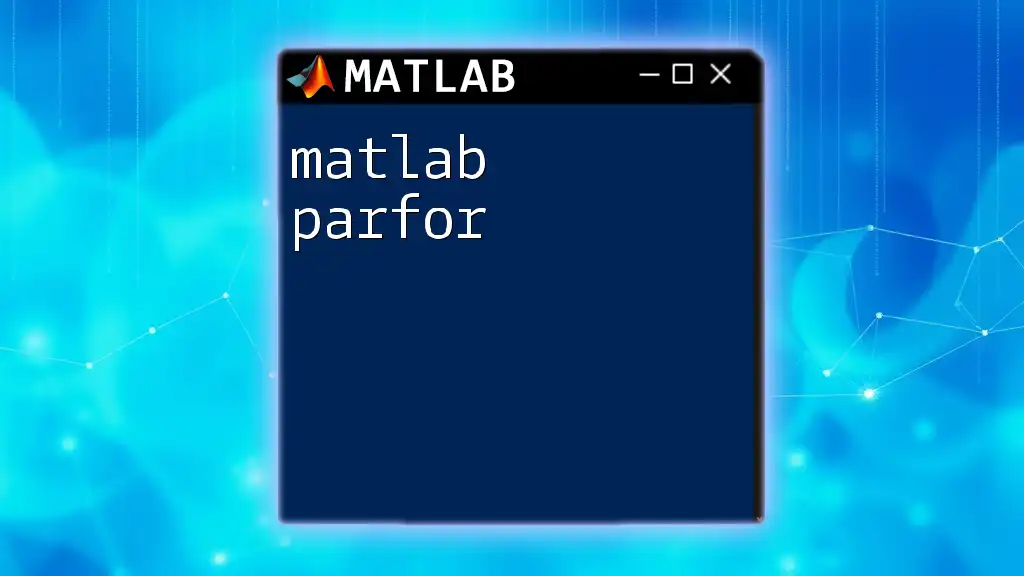
Conclusion
In summary, MATLAB markers are powerful tools for enhancing data visualization. By mastering the various types of markers, adjusting their properties, and creatively combining them with lines, you can create compelling visual narratives from your data. Whether you're a beginner or looking to refine your data visualization skills, experimenting with markers will greatly improve the effectiveness of your mathematical plots. Consider applying the guidance provided in this article to elevate your MATLAB plotting skills to the next level.
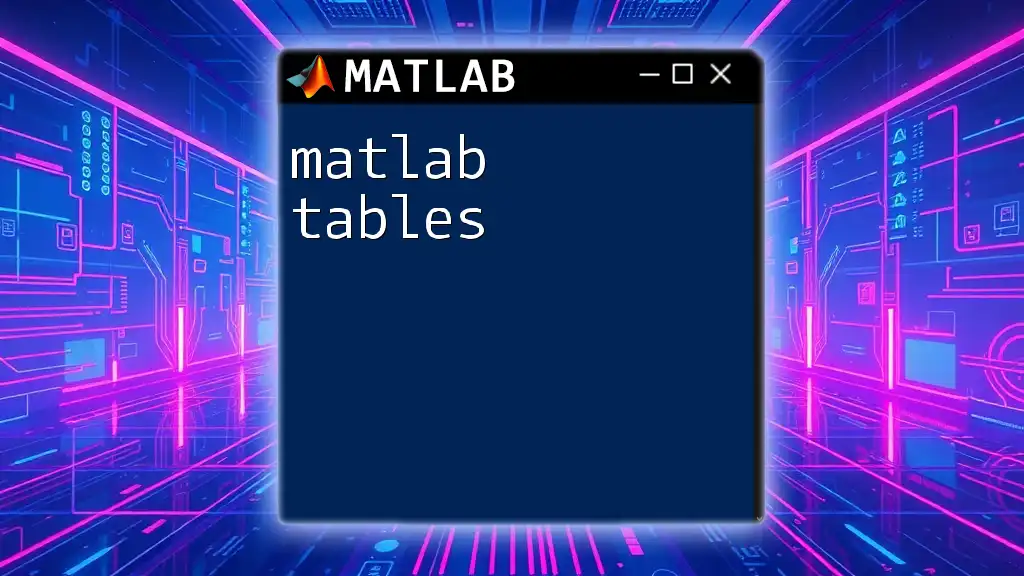
Additional Resources
For further exploration of MATLAB markers, consider visiting the official MATLAB documentation for in-depth examples and command references. This is invaluable for anyone looking to dive deeper into MATLAB’s rich graphical capabilities.