In MATLAB, cells are versatile data containers that can hold different types of data, including arrays, strings, and other cells, allowing for flexible storage and manipulation.
Here's a simple example of creating a cell array and accessing its elements:
% Creating a cell array
myCellArray = {1, 'Hello', [3, 4, 5]};
% Accessing the second element
secondElement = myCellArray{2}; % This will return 'Hello'
What are Cells?
Cells in MATLAB are a versatile data type that allows you to store different types of data in a single container. Unlike traditional arrays that require uniform data types, cell arrays enable you to combine various types, such as strings, numbers, and even other arrays. This flexibility makes them ideal for complex data sets where different entries may not conform to a single data type.
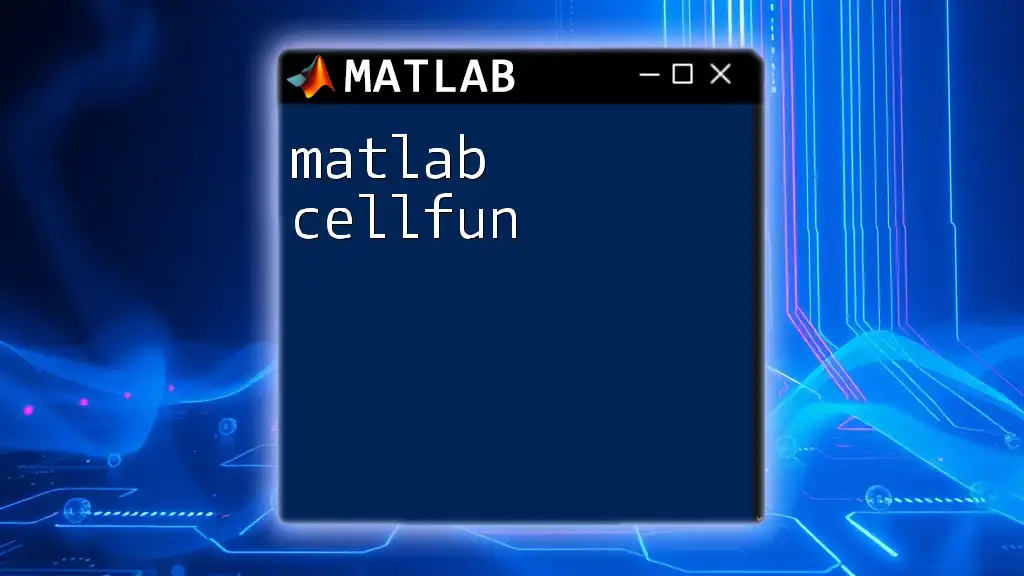
Why Use Cells?
Using cell arrays can significantly enhance your MATLAB programming, especially when dealing with heterogeneous data. Common use cases for cell arrays include:
- Storing Mixed Data Types: If you need to hold an integer, a string, and a matrix together, cell arrays are the solution.
- Handling Dynamic Data Sizes: When the size of your data changes often or is unknown, cell arrays offer a flexible way to store these variables.
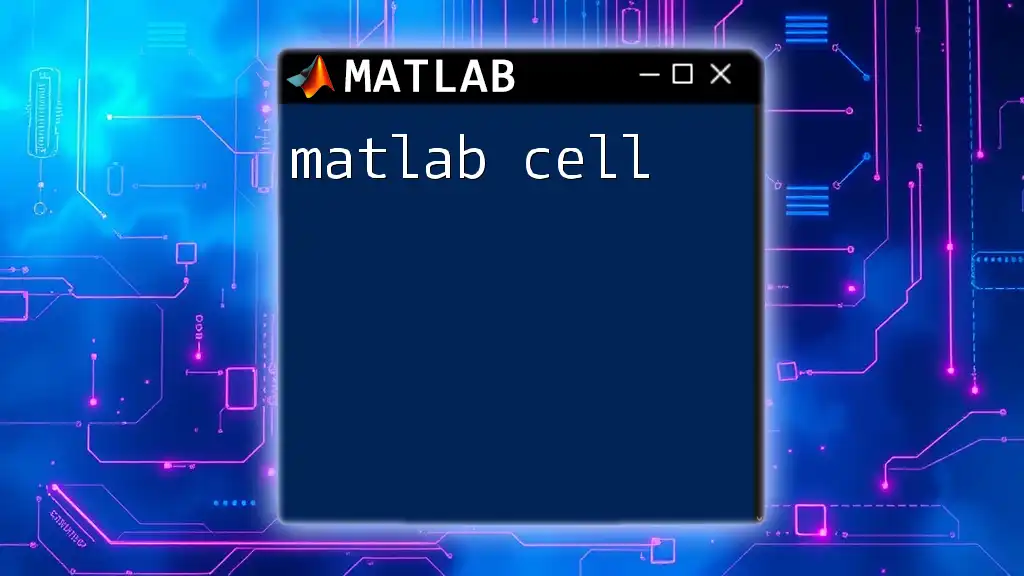
Creating Cell Arrays
Basic Syntax for Creating Cell Arrays
To create a cell array, the syntax utilizes curly braces `{}`. Here’s how to do it:
% Creating an empty cell array
myCellArray = {};
% Creating a cell array with different types
myCellArray = {1, 'MATLAB', [1, 2, 3]};
This snippet demonstrates the creation of a simple cell array containing various data types, showcasing the flexibility of cells.
Using the `cell` Function
For more complex scenarios, you might want to initialize a cell array filled with empty matrices:
% Creating a 2x3 cell array initialized with empty matrices
myCellArray = cell(2, 3);
This command defines a 2-by-3 cell array, effectively giving you a structured table that you can populate later.
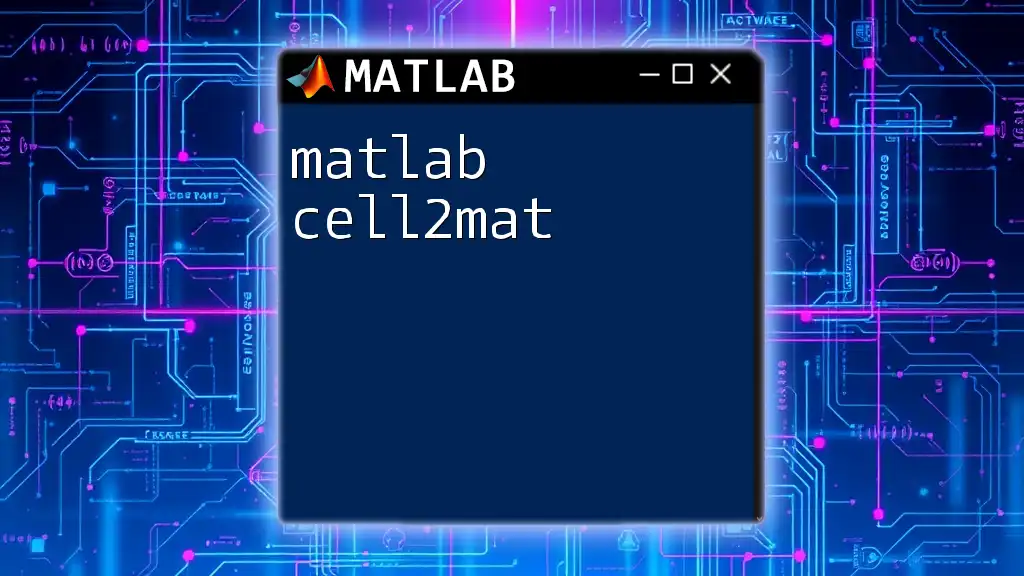
Accessing and Modifying Cell Arrays
Accessing Cell Elements
You can access elements in a cell array using curly braces `{}`, which return the content of the cell. Here’s how to perform this operation:
% Accessing the first element of a cell array
firstElement = myCellArray{1};
% Accessing the first row, second column
specificElement = myCellArray{1, 2};
The first snippet retrieves the content of the first cell, while the second accesses the cell at the intersection of the first row and the second column.
Modifying Cell Contents
Modifying elements in cell arrays is straightforward. To change the content of an existing cell, simply assign a new value:
% Changing the second cell in the array
myCellArray{1, 2} = 'Updated MATLAB';
In this case, the previous string in position (1, 2) gets replaced with “Updated MATLAB”.
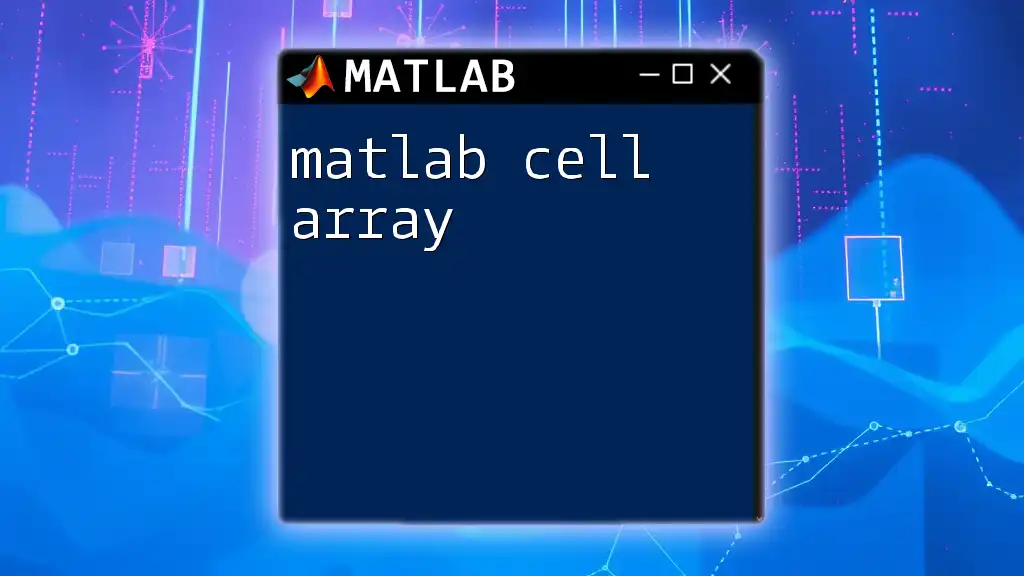
Cell Array Functions
Commonly Used Functions with Cell Arrays
Several built-in functions facilitate operations with cell arrays. A particularly powerful one is `cellfun`, which applies a specified function to each cell of a cell array.
`cellfun` Function
For instance, if you want to find the lengths of the strings stored in a cell array:
% Applying the length function to each cell
lengths = cellfun(@length, myCellArray);
This command returns an array of lengths corresponding to the contents of each cell.
`num2cell` and `cell2mat` Functions
`num2cell`: Converting Numeric Arrays to Cell Arrays
If you have a numeric array that you’d like to convert into a cell array, `num2cell` comes in handy:
% Converting a numeric array to a cell array
numericArray = [1, 2; 3, 4];
cellArrayFromNum = num2cell(numericArray);
This command transforms a numeric matrix into a cell array where each cell contains a number from the original array.
`cell2mat`: Converting Cell Arrays to Numeric Arrays
For situations when you want to revert a cell array back into a numeric array, use `cell2mat`:
% Converting a cell array to a numeric array
numericArray = cell2mat(cellArrayFromNum);
This provides a seamless way to switch the data type according to your needs.
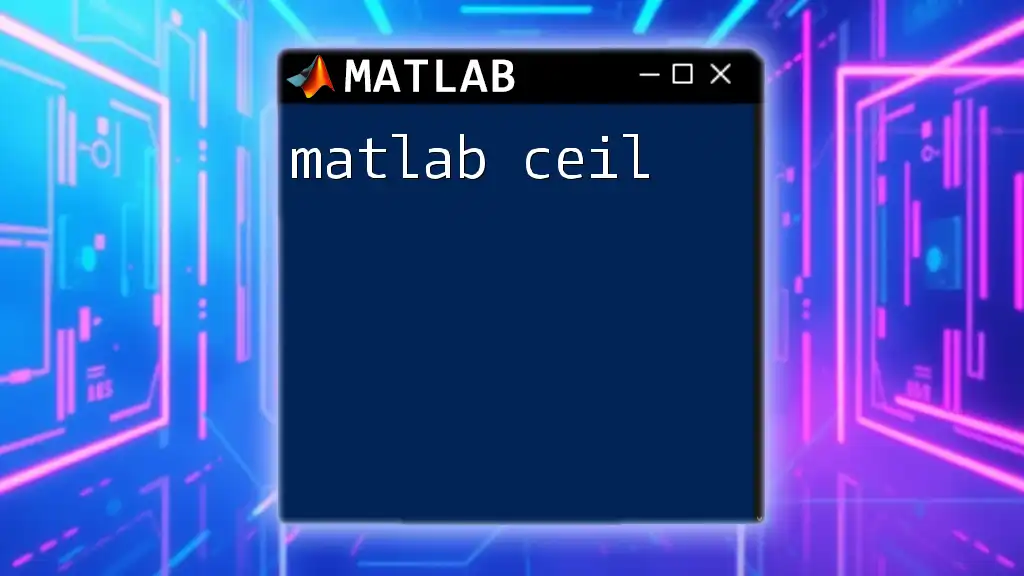
Working with Multidimensional Cell Arrays
Creating Multidimensional Cell Arrays
Cell arrays can also be multidimensional. To create a 3D cell array, you can use:
% Creating a 3D cell array
myMultiCellArray = cell(2, 2, 3);
This command creates a cell array with dimensions 2x2x3, allowing you to store structure data across three dimensions.
Accessing Multidimensional Cells
Just as with regular cell arrays, you can access elements in multi-dimensional cell arrays using a similar approach:
% Accessing a specific cell in a 3D cell array
myElement = myMultiCellArray{1, 1, 2};
This command retrieves the content of the cell located at the position (1,1,2) in the multi-dimensional array.
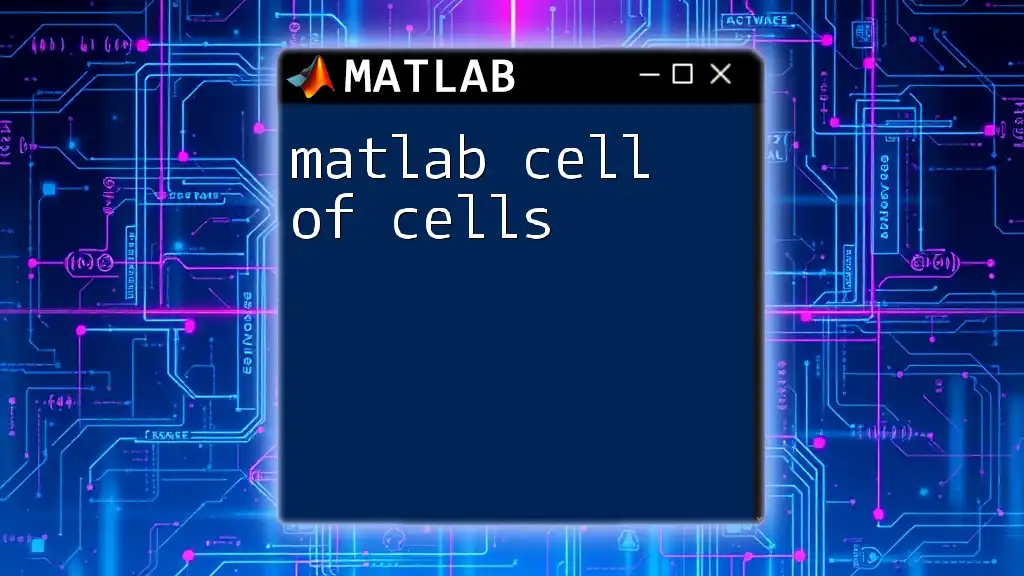
Best Practices for Using Cell Arrays
When to Use Cell Arrays vs. Matrices
Choosing between a cell array and a matrix often depends on the type of data you want to store. Use cell arrays when you need:
- Different data types stored together.
- Variable-length data entries or arrays.
Conversely, use traditional matrices when:
- All of your data is of the same type.
- You're performing mathematical operations that require uniform dimensional data.
Performance Considerations
While cell arrays offer great flexibility, they can sometimes lead to slower performance compared to numerical arrays, especially for large datasets. To optimize usage:
- Avoid resizing cell arrays frequently, as this creates overhead.
- Store similar types of data together when possible to leverage matrix operations.
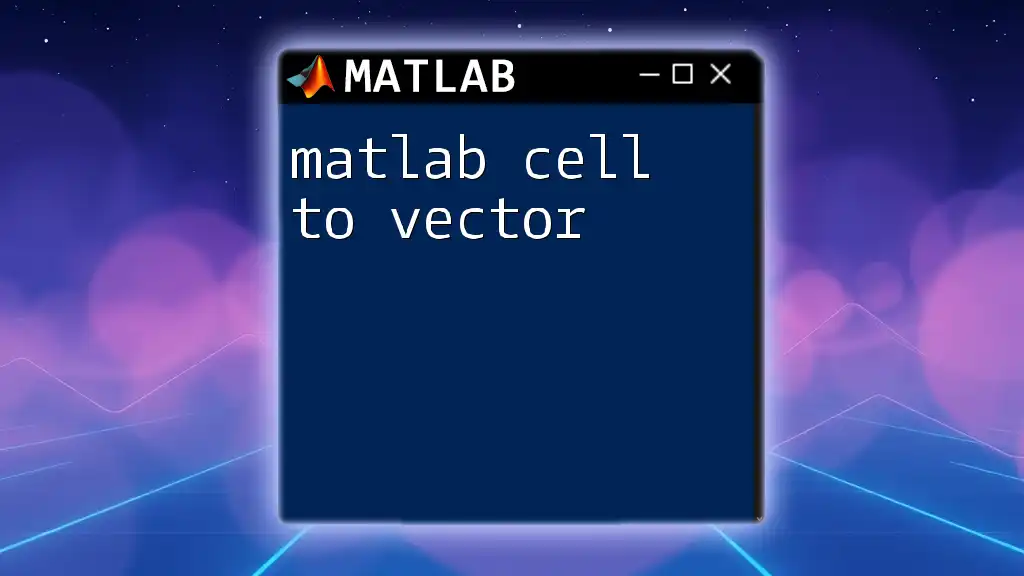
Conclusion
Mastering MATLAB cells is an essential skill for any programmer working with diverse data types in MATLAB. By understanding how to create, access, and manipulate cell arrays, you can significantly enhance your ability to handle complex datasets. As you explore, remember that cell arrays are a robust tool that can be adapted across various applications in MATLAB.
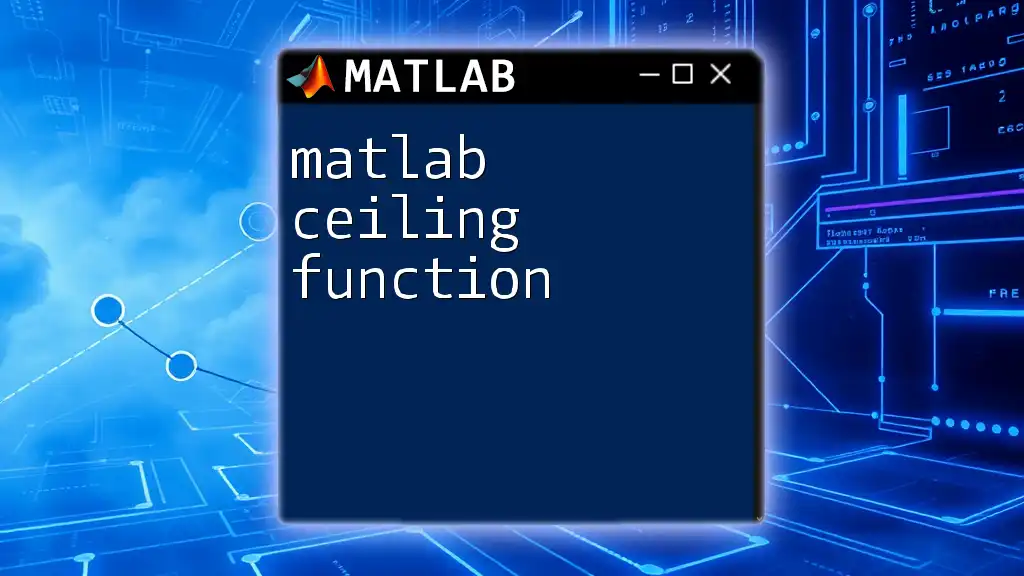
Additional Resources
For a deeper understanding, consider checking the official MATLAB documentation on cell arrays and various tutorials that delve into this versatile aspect of MATLAB programming. Exploring related resources will help you become more proficient and versatile in using MATLAB.