The `lsim` function in MATLAB simulates the time response of a linear system to arbitrary inputs, allowing you to analyze how a system reacts over time.
% Example of using lsim to simulate the response of a system
num = [1]; % Numerator coefficient of the transfer function
den = [1, 3, 2]; % Denominator coefficients of the transfer function
system = tf(num, den); % Create the transfer function
t = 0:0.01:5; % Time vector
u = sin(t); % Input signal
[y, t] = lsim(system, u, t); % Simulate the system response
plot(t, y); % Plot the output response
title('System Response using lsim');
xlabel('Time (s)');
ylabel('Output');
Understanding the Basics of Linear Systems
What is a Linear Time-Invariant (LTI) System?
A Linear Time-Invariant (LTI) system is a fundamental concept in control theory and signal processing. These systems have two primary characteristics: linearity and time-invariance.
- Linearity: This means that the output of the system is directly proportional to the input. If an input produces an output, then doubling the input leads to double the output.
- Time-Invariance: The behavior of the system remains consistent over time. If an input produces a certain output at a given time, then shifting the input in time results in the output being shifted by the same amount of time.
LTI systems are essential for analyzing and designing systems in various fields, such as control engineering, communication, and algorithm design. They simplify complex system dynamics by enabling the use of tools like the Laplace transform.
The Role of Transfer Functions
A transfer function defines the relationship between the input and output of an LTI system in the frequency domain. It is represented as a ratio of two polynomials, where:
- The numerator represents the output.
- The denominator represents the input.
Understanding how to convert differential equations into transfer functions is crucial for system analysis. A typical transfer function looks like this:
H(s) = N(s) / D(s)
where \(N(s)\) is the numerator polynomial and \(D(s)\) is the denominator polynomial.
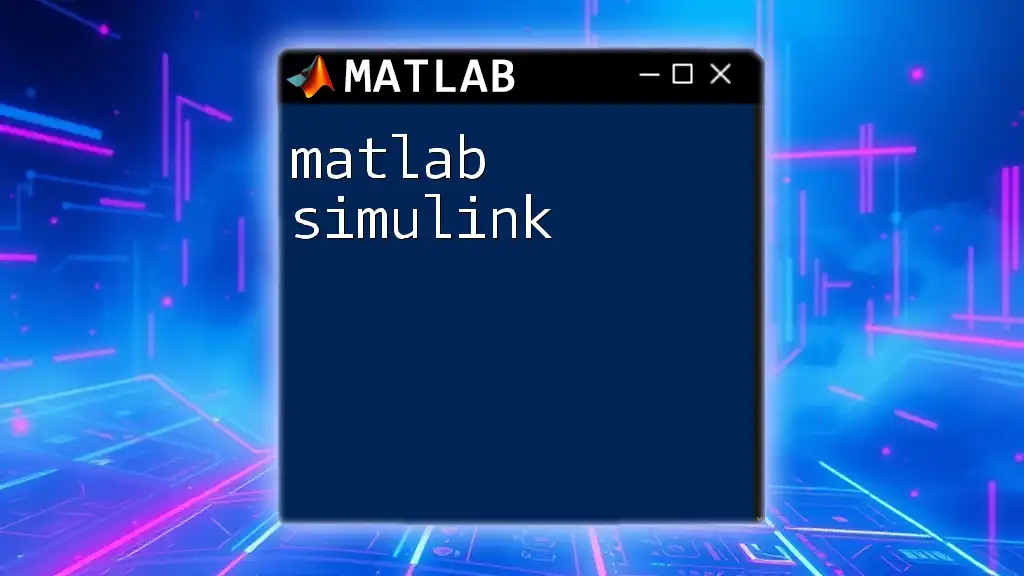
Syntax and Input Arguments for `lsim`
Basic Syntax of `lsim`
The basic syntax of the MATLAB function `lsim` is straightforward. It allows you to simulate the time response of LTI systems based on given input signals and time vectors:
lsim(sys, U, T)
In this syntax:
- `sys`: Represents the dynamic system, which can be defined as either a transfer function, state-space, or zero-pole-gain model.
- `U`: Denotes the input signal vector. This can be a continuous or discrete signal.
- `T`: This is the time vector for the simulation.
Input Argument Details
System Representation
Defining systems correctly is critical when using the `lsim` function in MATLAB. You can represent a system through various means. Here’s how to create a simple transfer function:
num = [1]; % Numerator coefficients
den = [1, 5, 6]; % Denominator coefficients
sys = tf(num, den);
In this example, we define a transfer function with a numerator of 1 and a denominator represented by the polynomial \[s^2 + 5s + 6\].
Input Signal Specifications
The input signal must be well defined for the simulation to be effective. MATLAB's `lsim` accepts various input types, such as step, impulse, and sinusoidal inputs, depending on your application.
Here's how to create a step input:
t = 0:0.1:10;
U = ones(size(t)); % Step input
In this example, we create a vector `U` that remains at 1 for the entire duration from 0 to 10 seconds, simulating a step function.
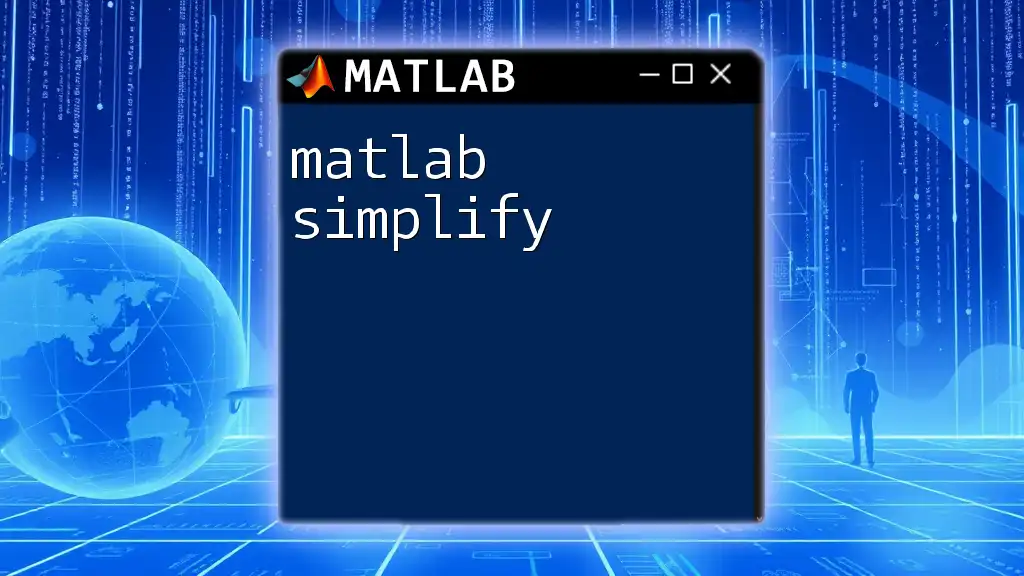
Practical Example: Using `lsim` in MATLAB
Step by Step Walkthrough
Defining the System
Let's consider a second-order system for our simulation example. Here's how to define such a system in MATLAB:
num = [2];
den = [1, 3, 2];
sys = tf(num, den);
In this scenario, our transfer function represents a system with a numerator of 2 and a denominator polynomial that can be transformed to the form \[s^2 + 3s + 2\].
Creating the Input Signal
Next, we will create a sinusoidal input signal to observe how our system responds to this type of input:
t = 0:0.1:10;
U = sin(t); % Sinusoidal input
This code snippet defines a time vector `t` and a sinusoidal input signal `U` by using the sine function.
Simulating the System
Now that we have defined our system and input signal, we can run the simulation using the `lsim` function:
lsim(sys, U, t)
title('System Response');
xlabel('Time (s)');
ylabel('Response');
This code will generate a plot showing how the second-order system responds to the sinusoidal input over the specified time duration. The title and labels enhance the interpretability of the graph.
Analyzing Output
Once the simulation is complete, analyzing the output graph is essential for understanding the system’s behavior. Key features to look for include the magnitude of the output, the phase shift, and the steady-state behavior.
The system's overshoot (how much the output exceeds its final value) and settling time (the time it takes for the output to remain within a certain percentage of its final value) are critical in determining system performance.
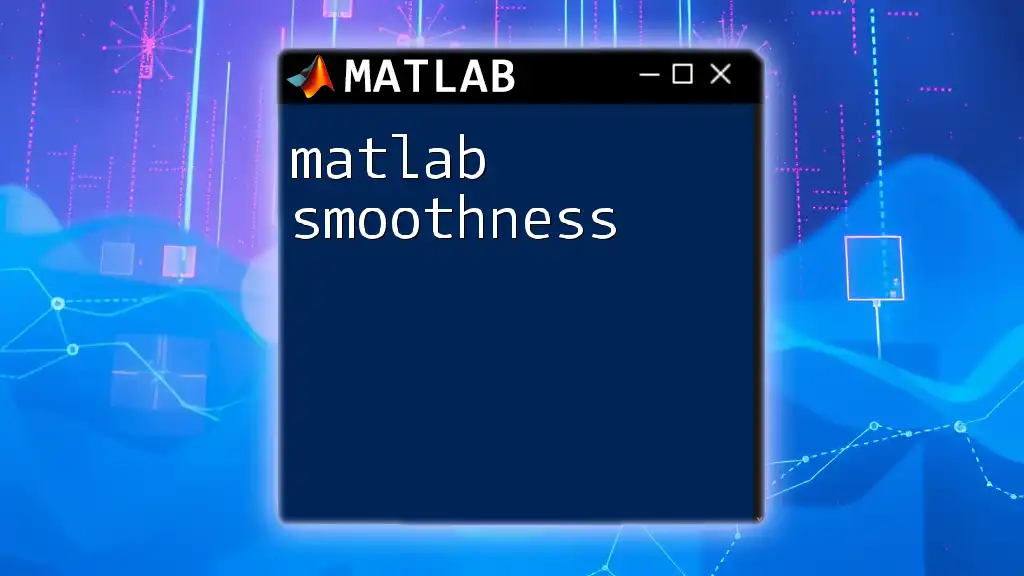
Advanced Features of `lsim`
Multiple Input Scenarios
Using `lsim` with Multiple Inputs
The `lsim` function is versatile and can handle multiple inputs. For systems with multiple inputs, it's vital to represent each input signal as a matrix, where each column corresponds to a different input signal.
Customizing Simulations
Altering Time Parameters
You can customize time parameters to better fit your simulation's requirements. Modifying the time vector will change the resolution and duration of the simulation.
For example, changing the step size or time range could significantly affect the output:
t = 0:0.01:10; % Higher resolution time vector
Adding Noise to the Input
Introducing noise makes simulations more realistic. You can add a random noise component to your input signal like this:
noise = 0.05 * randn(size(t));
U_noisy = U + noise;
By adding noise, you can test how robust your system is against real-world disturbances.
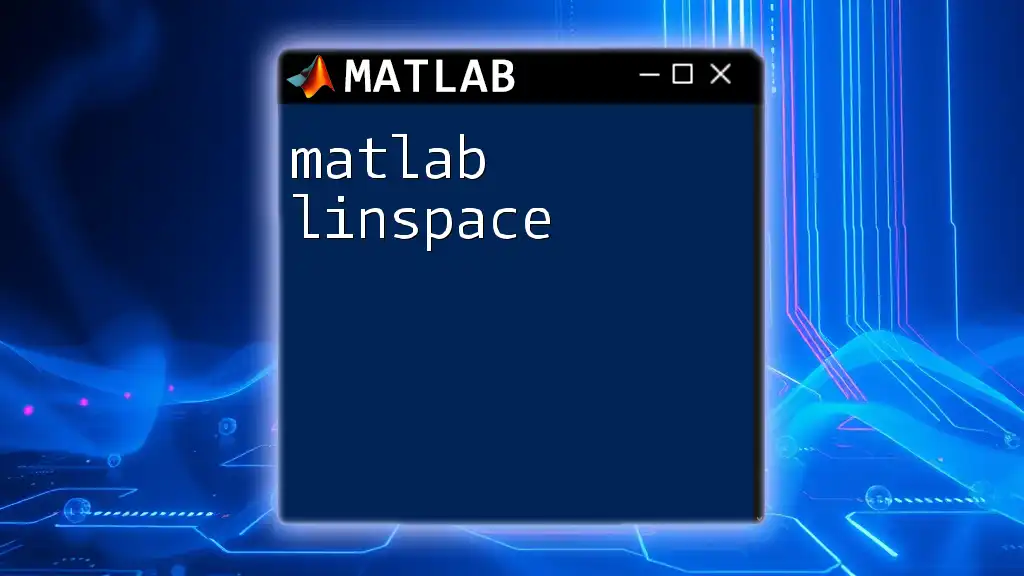
Common Issues and Troubleshooting
Frequent Errors with `lsim`
Even experienced users sometimes run into common issues when using `lsim`. Typical errors include dimension mismatches between the input signal and the time vector or an incorrect definition of the system.
Debugging Tips
When troubleshooting, always check that:
- The dimensions of the inputs are compatible.
- The `sys` object is correctly defined.
- Input signals match the system’s requirements.
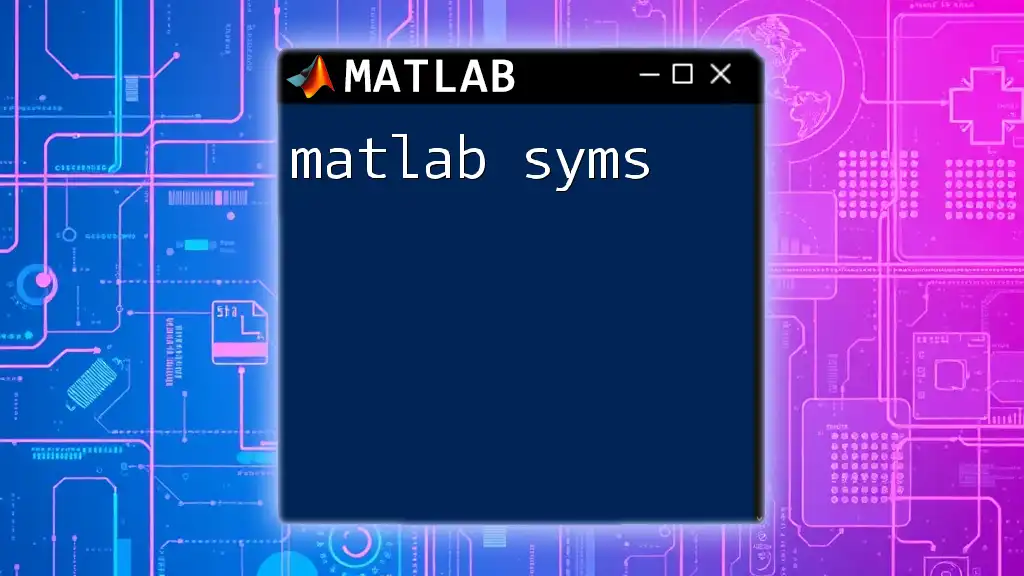
Conclusion
The MATLAB function `lsim` is an invaluable tool for simulating the response of linear time-invariant systems to various inputs in a straightforward manner. By understanding the fundamentals of linear systems, mastering the input specifications, and exploring the advanced features of `lsim`, you can effectively analyze system behavior and performance.
Experimenting with different system configurations, input signals, and simulation parameters will enhance your understanding of dynamic responses and help you become proficient in MATLAB simulations. The skills you gain from using `lsim` will undoubtedly be beneficial across multiple applications in engineering and scientific research.
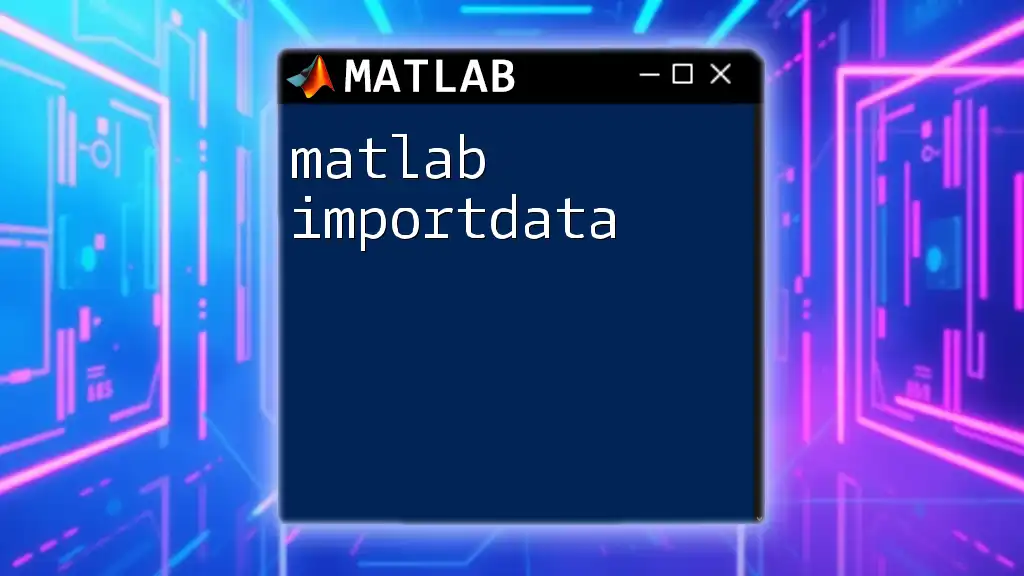
Additional Resources
You can find more information about the `lsim` function and its many applications in the official MATLAB documentation, which offers numerous examples and detailed explanations. Additionally, consider exploring further readings and tutorials on linear systems for a deeper understanding.