The `ceil` function in MATLAB rounds each element of an array to the nearest integer greater than or equal to that element. Here's a simple code snippet demonstrating its use:
% Example of using ceil in MATLAB
x = [1.2, 2.5, 3.8, -1.3, -2.7];
y = ceil(x);
disp(y); % Output will be [2, 3, 4, -1, -2]
What is the `ceil` Function?
The `ceil` function is a built-in MATLAB function that rounds each element of an array to the nearest integer greater than or equal to that element. This function is especially useful in various mathematical and engineering applications where rounding down could lead to miscalculations or invalid results.
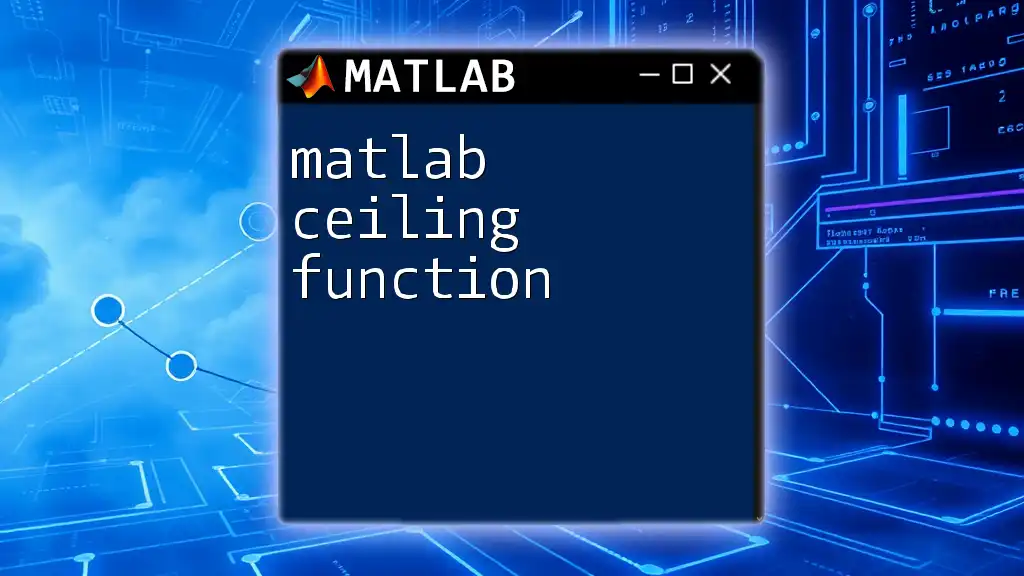
Syntax of the `ceil` Function
Understanding the syntax is crucial for effectively using the `ceil` function. The basic syntax in MATLAB is as follows:
Y = ceil(X)
Here, `X` is the input numeric array, which can be a scalar, vector, or matrix. It can consist of various data types, primarily floating-point numbers. The output, `Y`, will be an array of the same size, with each element rounded up to the nearest integer.
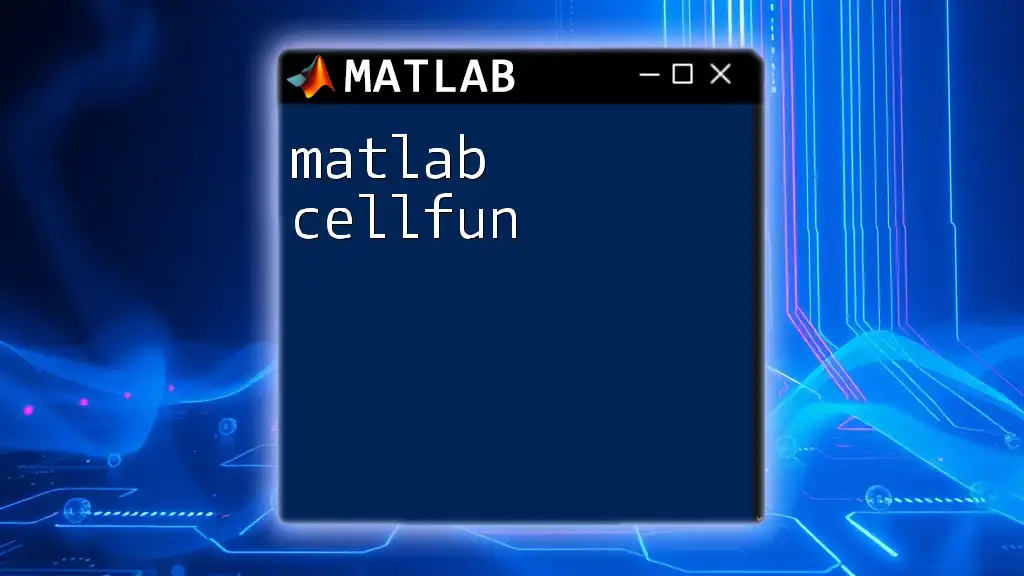
How Does `ceil` Work?
The behavior of the `ceil` function can be best understood through its mathematical definition. The ceiling function effectively captures the smallest integer that is greater than or equal to a given number.
- Positive Numbers: For example, if you input `3.7`, the function `ceil(3.7)` will yield `4` because `4` is the smallest integer greater than `3.7`.
- Negative Numbers: If you input `-2.1`, calling `ceil(-2.1)` will round to `-2`. This is crucial because rounding for negative numbers operates differently; the ceiling function rounds up toward zero rather than down.
- Zero: Inputting `0` provides a straightforward case as `ceil(0)` will return `0`.
Understanding these basic behaviors ensures that you can effectively apply the `ceil` function in your numerical computations.
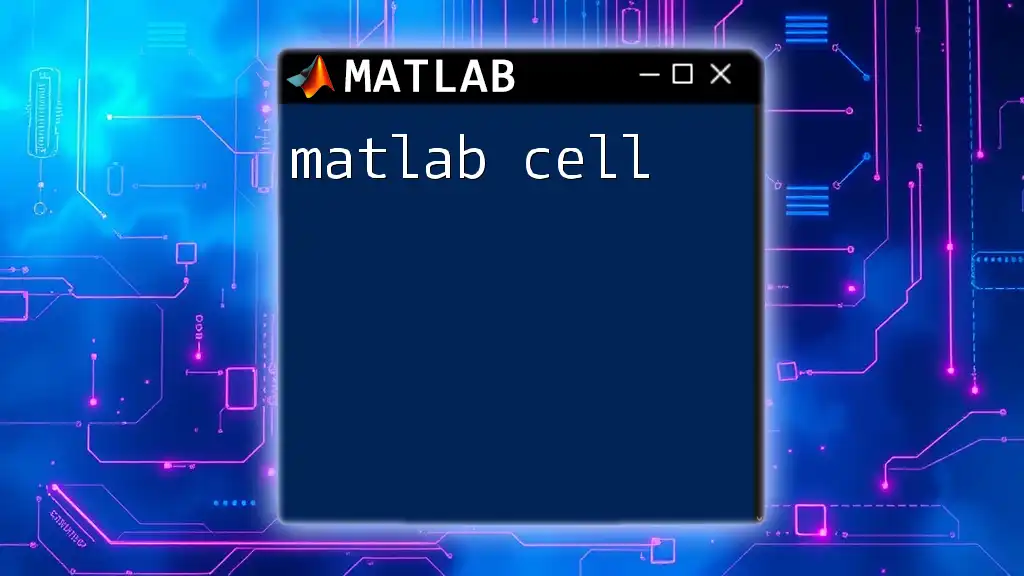
Practical Examples
Example 1: Basic Usage with a Scalar Value
Let's examine a simple usage with a scalar value:
x = 3.7;
y = ceil(x);
% Output: y = 4
In this instance, the function rounds `3.7` to `4`.
Example 2: Working with Vectors
The `ceil` function also operates effectively on vectors. Consider this example:
x = [1.2, 3.8, -2.1, -4.9];
y = ceil(x);
% Output: y = [2, 4, -2, -4]
In this case, you observe how `ceil` adjusts each value in the vector individually, with positive numbers rounding up and negative numbers rounding towards zero.
Example 3: Applying `ceil` to Matrices
The function works similarly on matrices:
x = [1.5, 2.3; -3.1, 4.6];
y = ceil(x);
% Output: y = [2, 3; -3, 5]
Each element within the matrix is processed, demonstrating the versatility of the `ceil` function across various array structures in MATLAB.
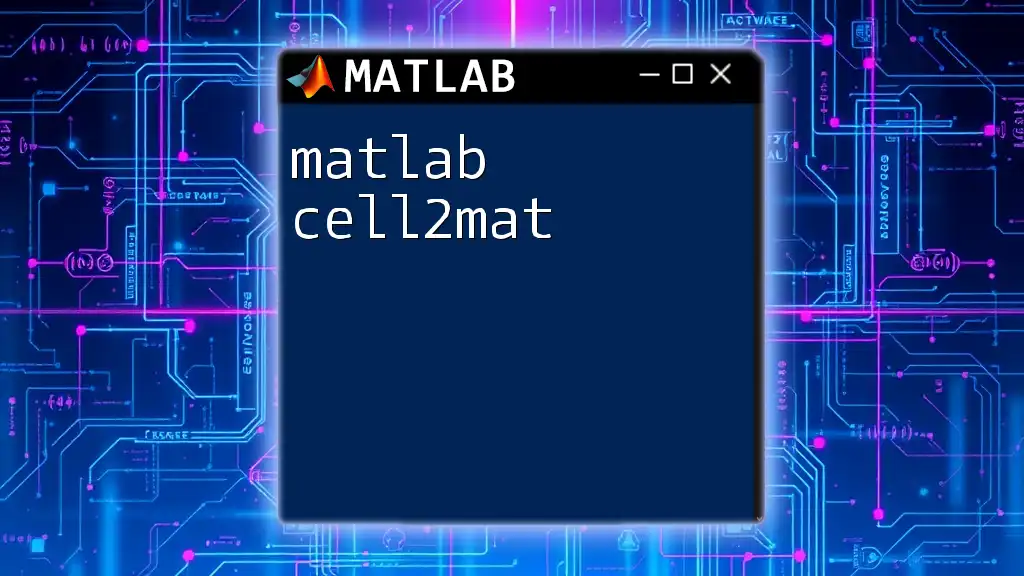
Performance Considerations
When using `ceil`, it is essential to consider performance, particularly with large arrays. The execution speed of the `ceil` function is optimized within MATLAB. Nonetheless, if operating on extensive datasets, always conduct a performance review to ensure that the function is being applied effectively without introducing bottlenecks.
Also, keep in mind the memory usage involved when working with large arrays. MATLAB internally manages memory allocation efficiently, but the type of array and its size can affect performance; choosing the right data structure is vital for optimal resource management.
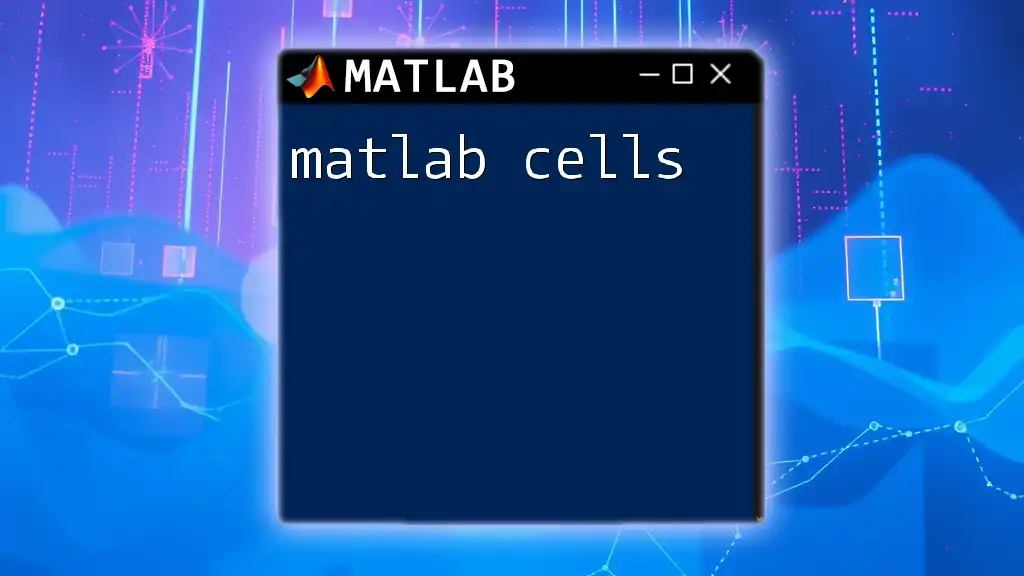
Common Use Cases for `ceil`
The `ceil` function finds its application in various settings:
- Data Analysis: Rounding up can help standardize data points for further analysis, thus avoiding skewed results.
- Graphing and Plotting: Using `ceil` ensures that data points display correctly on graphs, particularly when creating axes limits.
- Engineering Applications: In fields such as control systems, accurately rounded numbers can be critical in simulations and modeling scenarios where tolerances are tight.
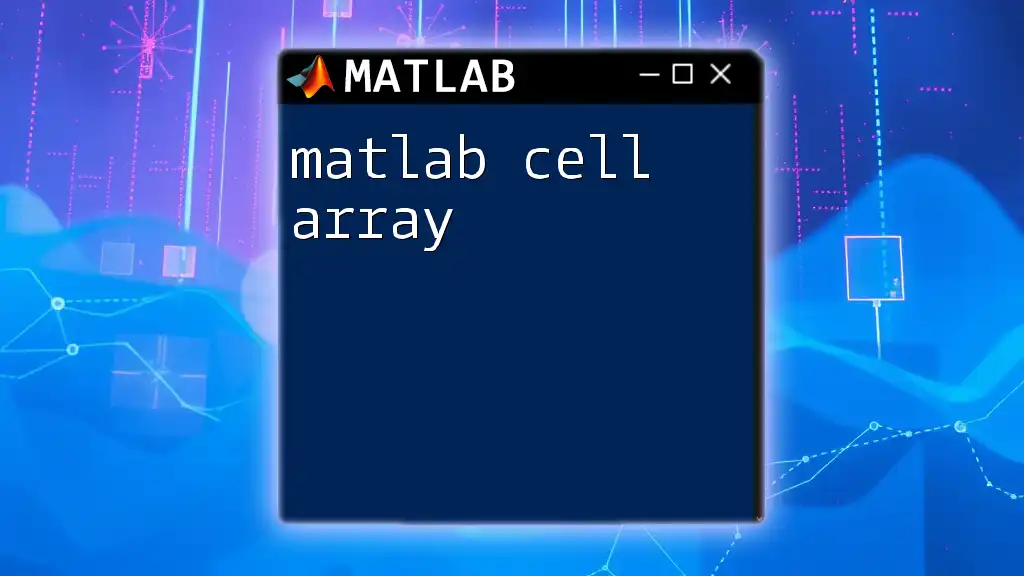
Alternatives to `ceil`
While `ceil` is indispensable, it’s beneficial to know its alternatives for different contexts:
- Comparison with `floor` Function: While `ceil` rounds up, `floor` rounds down to the nearest integer. Use `floor` when you need to round down instead.
- The `round` Function: This function rounds to the nearest integer based on the halfway point. It provides a balance between rounding up and down based on the decimal value.
- `fix` Function: The `fix` function rounds toward zero—positive values round down, while negative values round up toward zero. This can offer an alternative approach for different scenarios.
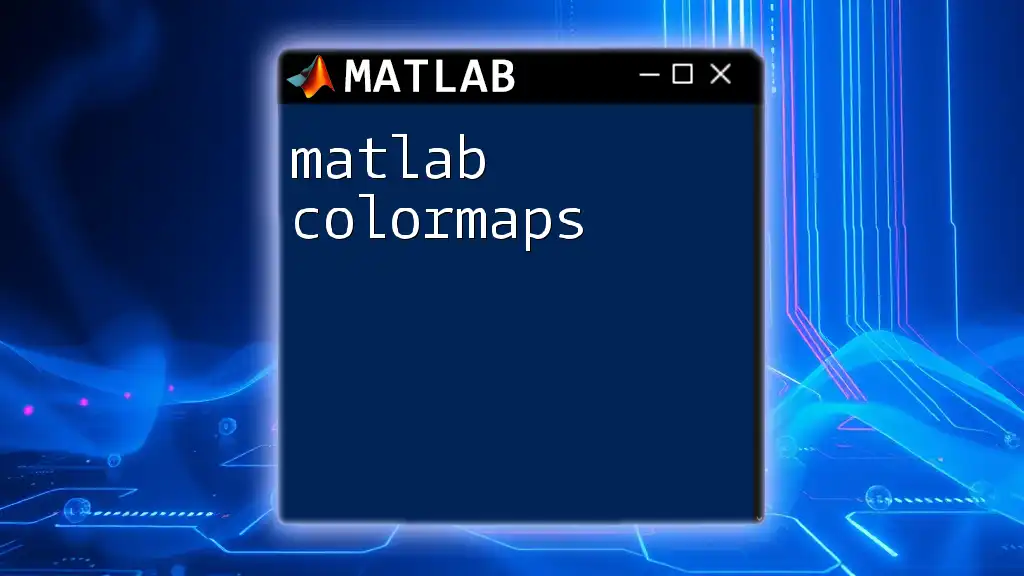
Troubleshooting and FAQs
When working with the `ceil` function, some common issues might arise:
- Common Errors: Misunderstanding the behavior of `ceil` on negative numbers can lead to errors. Ensure you remember that rounding for negatives moves toward zero.
- How to Diagnose Issues: If an unexpected outcome arises, try breaking down your calculations step-by-step or inspecting the individual elements of your array before applying `ceil`.
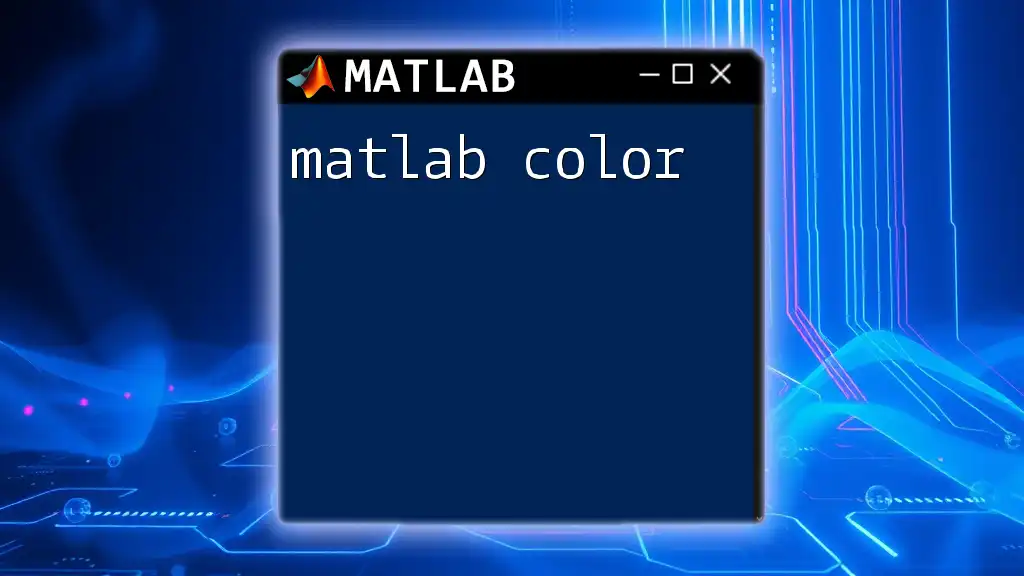
Conclusion
The MATLAB `ceil` function is a powerful tool for rounding numbers up. By understanding its syntax, behavior across different numerical types, and use cases, you can effectively incorporate it into your programming.
Remember to practice with various datasets to gain proficiency in utilizing `ceil`. Mastering this function will not only enhance your mathematical computations but also provide the foundation for more advanced analysis and engineering applications.
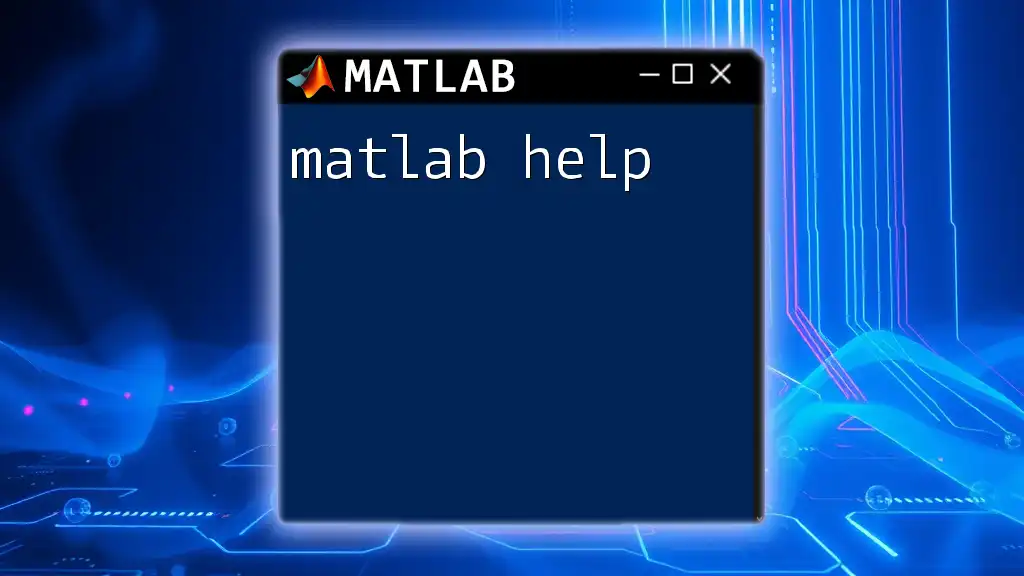
Additional Resources
To delve deeper into the `ceil` function and explore other aspects of MATLAB, consider checking the following:
- Official MATLAB Documentation: A great source for comprehensive and up-to-date information about MATLAB functionalities and its extensive capabilities.
- Further Reading: Look for tutorials and articles that enhance your understanding of MATLAB programming and numerical methods.
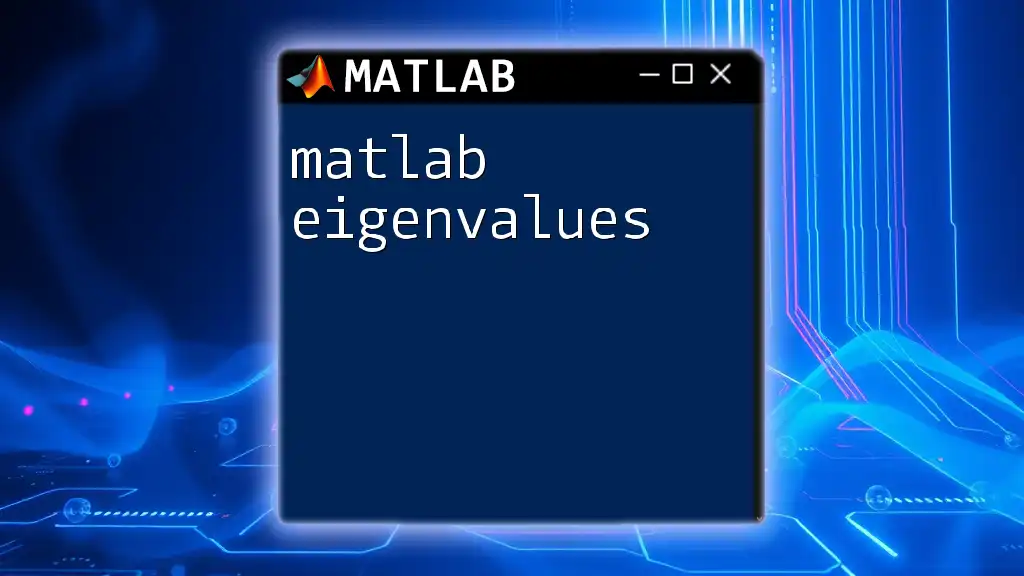
Call to Action
Now that you are equipped with insights on the `ceil` function, experiment by applying it to various data sets in MATLAB! If you want to enhance your skills further, check out additional MATLAB courses and sessions offered by our company.