Certainly! Here's your post with a concise explanation and a code snippet:
When seeking MATLAB help, it's essential to understand fundamental commands that can enhance your productivity; for instance, here's how to create a simple plot:
x = 0:0.1:10; % Create a vector from 0 to 10 in increments of 0.1
y = sin(x); % Calculate the sine of each value in x
plot(x, y); % Plot y versus x
title('Sine Wave'); % Add a title to the plot
xlabel('x values'); % Label x-axis
ylabel('sin(x) values'); % Label y-axis
Let me know if you need further assistance!
Getting Started with MATLAB
What is MATLAB?
MATLAB, short for “Matrix Laboratory,” is a high-level programming language and interactive environment designed for numerical computation, visualization, and programming. It's widely used across various domains, including engineering, finance, data analysis, and academia, making it a crucial tool for solving complex mathematical problems.
Installation and Setup
System Requirements
Before installing MATLAB, it’s important to ensure that your system meets the minimum requirements. MATLAB typically requires a compatible operating system (Windows, macOS, or Linux), sufficient RAM (at least 4GB is recommended), and ample disk space for installation and operations.
Downloading and Installing MATLAB
To get started, follow these steps to download and install MATLAB:
- Visit the official MathWorks website.
- Create an account or log in to your existing account.
- Navigate to the download section after purchasing a license or accessing a trial version.
- Follow the installation prompts specific to your operating system. Typically, the installation process involves running an installer file and selecting components to install.

Navigating the MATLAB Environment
MATLAB Interface Overview
Command Window
The Command Window is the heart of MATLAB where you can enter commands and see results in real-time. It allows users to perform calculations, execute scripts, and access variable values instantly.
Editor and Debugger
The Editor is where you can write and save your MATLAB scripts. When you're debugging your code, the Debugger is an invaluable tool that allows you to set breakpoints, step through the code line by line, and examine variable values to diagnose issues effectively.
Key Features of MATLAB
Variables and Data Types
In MATLAB, variables are easy to create and can store various data types – scalars, arrays, and matrices are the most common. Here's how to create a simple variable:
a = 5; % Example of creating a variable
Variables in MATLAB are dynamically typed, meaning you don’t need to explicitly declare their types. However, being familiar with data types will improve your coding efficiency.
Built-in Functions
MATLAB comes with a plethora of built-in functions designed to simplify mathematical operations and algorithms. You can access these functions easily by using the command syntax or through the user interface. Some commonly used functions include `sum()`, `mean()`, `max()`, and many more.
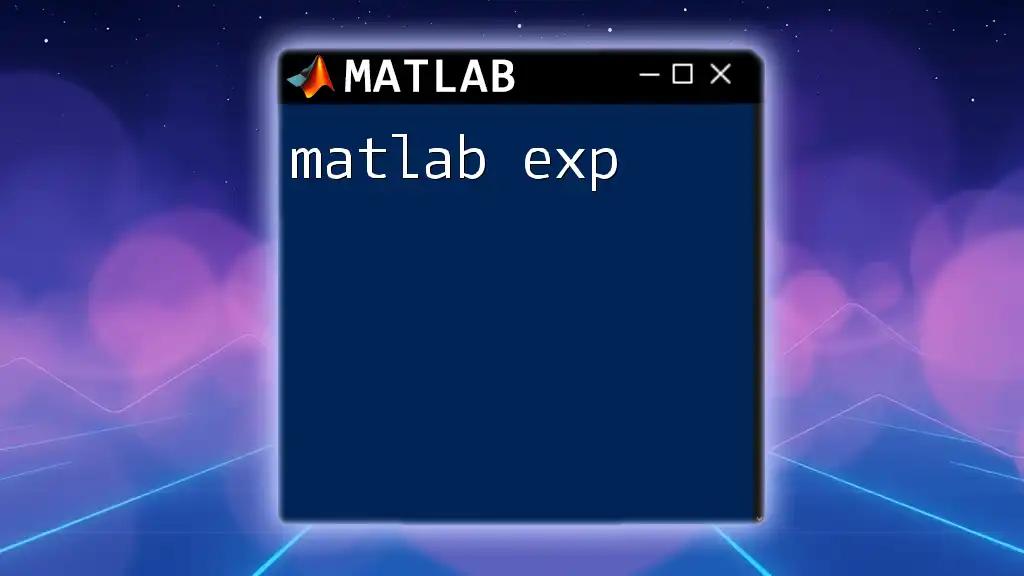
Basic MATLAB Commands
Working with Variables and Arrays
Creating and Modifying Arrays
Creating arrays and matrices is at the core of MATLAB. Here's an example:
A = [1, 2, 3; 4, 5, 6]; % Creating a 2x3 matrix
You can modify these arrays with simple indexing:
A(1,2) = 10; % Changes the element at the first row and second column to 10
Control Flow
Conditional Statements
Control flow allows your program to take different paths based on conditions. Here’s how to use an `if` statement:
if a > 0
disp('Positive number');
elseif a < 0
disp('Negative number');
else
disp('Zero');
end
This code checks the value of `a` and displays corresponding messages.
Loops: For and While
Loops are fundamental for executing code repetitively. Here's an example of a `for` loop:
for i = 1:5
disp(i);
end
This loop will display the numbers 1 through 5. Similarly, a `while` loop can structure your code to run as long as a specified condition is true:
count = 1;
while count <= 5
disp(count);
count = count + 1;
end
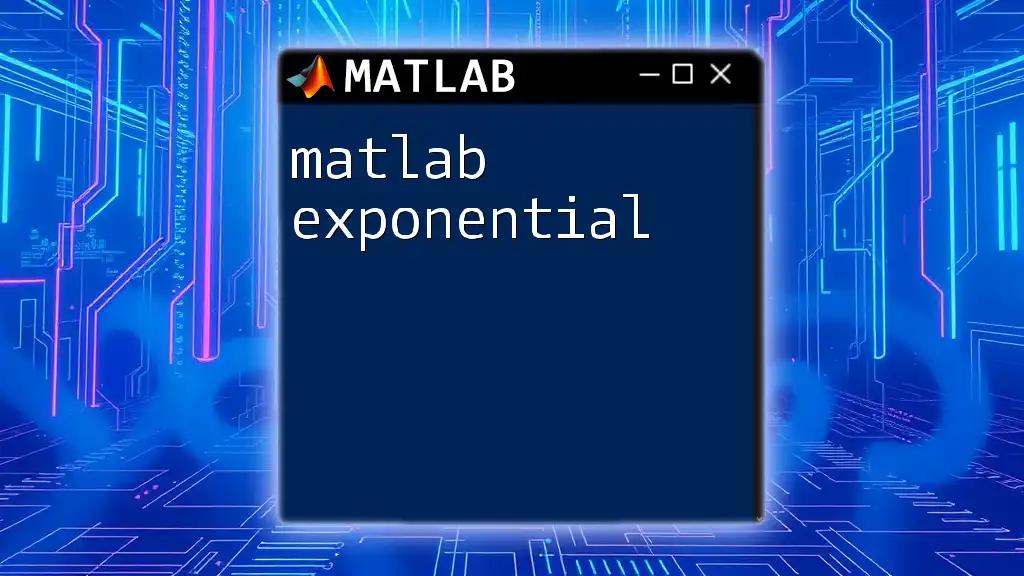
Advanced MATLAB Functions
Custom Functions
Creating and Calling Functions
MATLAB allows users to create their own functions, which can make your code more organized and reusable. Here's a simple example of a function that squares a number:
function y = square(x)
y = x^2;
end
To call this function, simply use:
result = square(4); % result will be 16
Visualization and Plotting
Basic Plotting Techniques
Visualizing data is an integral part of data analysis. Creating a simple plot using the `plot` function is straightforward:
x = 0:0.1:10;
y = sin(x);
plot(x, y); % Simple 2D plot of the sine function
Enhancing Plots with Annotations
To make your plots clearer, you can add titles, axis labels, and legends:
title('Sine Wave');
xlabel('X-axis');
ylabel('Y-axis');
legend('y = sin(x)');
These elements enhance the comprehensibility of your plots and provide context for your data.
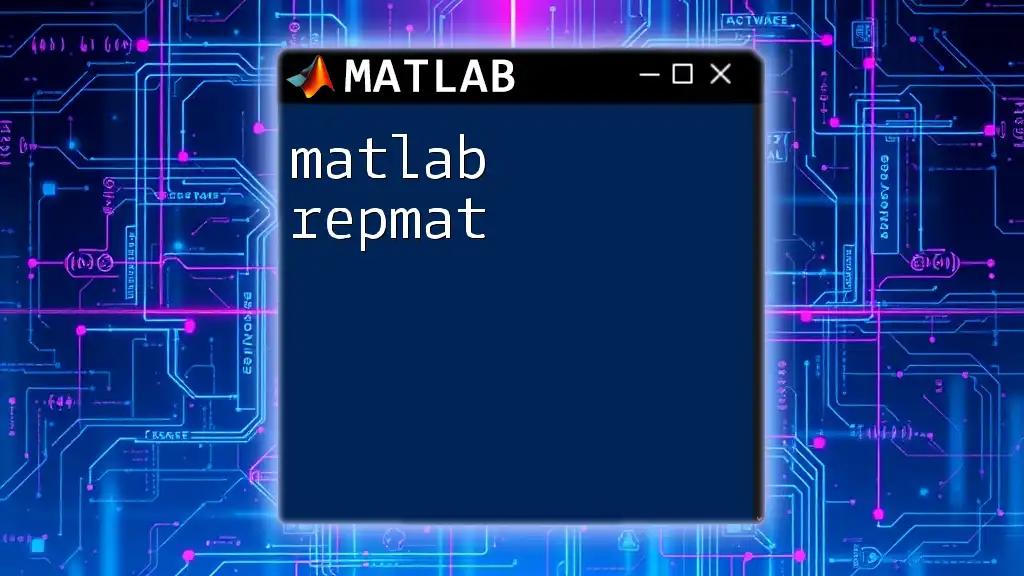
Debugging and Error Handling
Common Errors in MATLAB
Syntax Errors
Syntax errors occur when there's a mistake in the code structure, such as forgetting a semicolon or misspelling a function name. These errors will stop the execution of your code and need to be fixed before proceeding.
Runtime Errors
Runtime errors occur during code execution, often due to issues like invalid indexing or division by zero. It's crucial to thoroughly test your code to minimize such errors.
Debugging Tools in MATLAB
MATLAB's debugging features help identify and resolve issues. Setting breakpoints allows you to pause execution at specific points in the code, making it easier to inspect variable states. Use the `dbstop` command to set breakpoints before running your script.
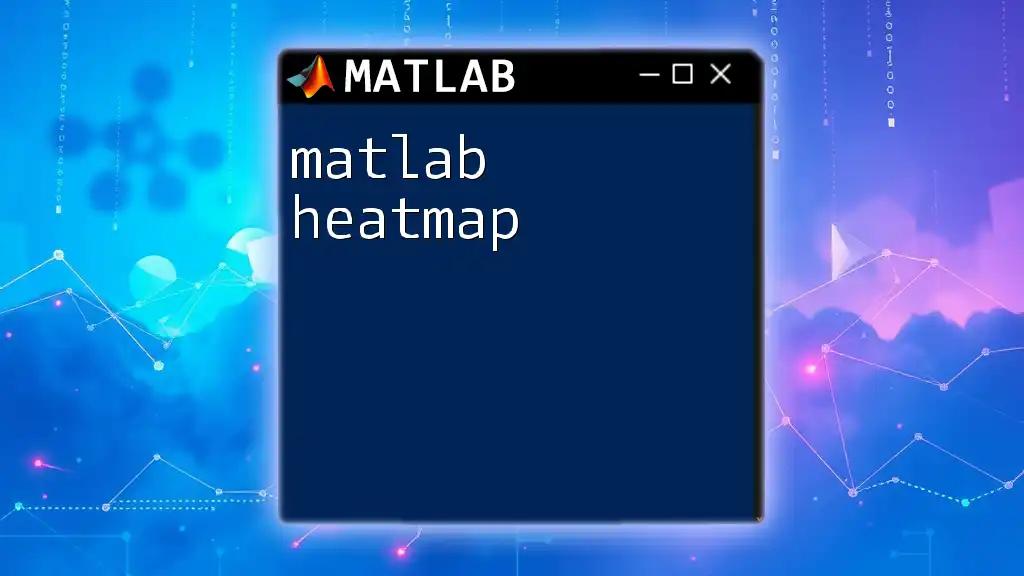
Resources for MATLAB Help
Online Communities and Forums
Engaging with online communities like MATLAB Central and Stack Overflow can provide valuable insights and support. These platforms allow you to ask questions, share knowledge, and learn from experienced users.
Documentation and Tutorials
The official MATLAB documentation is an extensive resource that covers all aspects of MATLAB, including function definitions, usage examples, and tutorials. Familiarize yourself with the search functionality to quickly find the information you need.
Online Courses and Tutorials
For structured learning, consider enrolling in online courses available on platforms like Coursera, Udemy, and edX. These platforms offer both free and paid courses, catering to all skill levels, from beginners to advanced users.

Conclusion
Becoming proficient in MATLAB requires continuous learning and practice. The vast capabilities of MATLAB make it an indispensable tool across various fields, and understanding the essential commands can significantly enhance your analytical skills.
Call to Action
Start your MATLAB journey today! Don’t hesitate to explore the resources mentioned and consider our tailored services for comprehensive MATLAB help, designed to meet your specific learning needs.

FAQs about MATLAB Help
What is the best way to learn MATLAB?
The best way to learn MATLAB is by combining hands-on practice with official documentation and community support. Consider following tutorials and experimenting with your projects.
How do I troubleshoot errors in my MATLAB code?
Use the debugging features available in MATLAB, such as breakpoints and step execution, to investigate issues. Additionally, referring to online resources and forums can provide solutions to common problems.
Are there any free resources available to learn MATLAB?
Yes, several free resources are available, such as MATLAB's official documentation, introductory courses on platforms like Coursera or edX, and community forums.
How do I optimize my code performance in MATLAB?
Optimize your MATLAB code by vectorizing operations, preallocating memory for arrays, and utilizing built-in functions that are designed for performance. Regularly profiling your code can also highlight bottlenecks that need attention.