The `cell2mat` function in MATLAB converts a cell array of numeric arrays into a single numeric matrix.
Here’s a code snippet demonstrating its usage:
% Example: Converting a cell array to a matrix
C = {1, [2; 3], 4}; % Cell array
M = cell2mat(C); % Convert to matrix
disp(M); % Display the resulting matrix
Understanding Cell Arrays
What are Cell Arrays?
Cell arrays in MATLAB are versatile structures that can hold data of varying types and sizes. Unlike regular arrays, which can only contain elements of the same data type, cell arrays can contain strings, numbers, matrices, or even other cell arrays. This flexibility makes them an excellent choice for managing a diverse set of data.
When to Use Cell Arrays?
Cell arrays are particularly useful in various scenarios, such as:
- Storing heterogeneous data: When your dataset includes strings, numbers, and matrices within the same collection.
- Dynamic-sized collections: When the size of data can change, making it impractical to use standard arrays.
- Grouping related variables: For example, if you want to store a mix of parameters related to different subjects, cell arrays can help maintain organization.
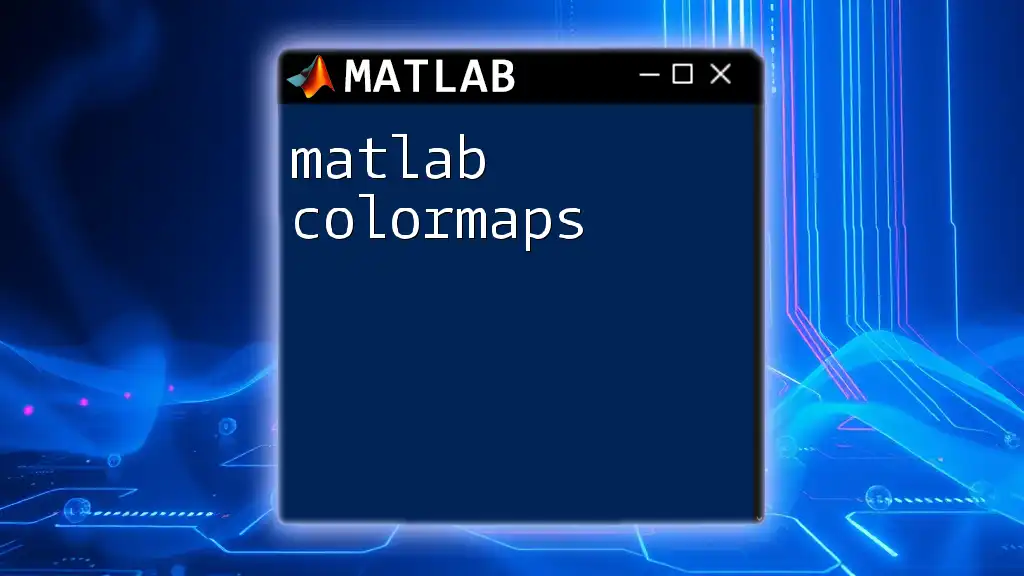
The `cell2mat` Function
What is `cell2mat`?
The `cell2mat` function is a built-in MATLAB function designed to convert a cell array into a standard matrix. This function is essential when you have a cell array that you wish to perform mathematical operations on, or when you want to manipulate the data in a more structured form.
How Does `cell2mat` Work?
`cell2mat` reads the contents of a cell array and concatenates it into a single matrix. For this function to work correctly, the elements contained within the cell array must be of compatible sizes and types. Mismatched elements will result in errors, underscoring the importance of having homogenous data in the cell.
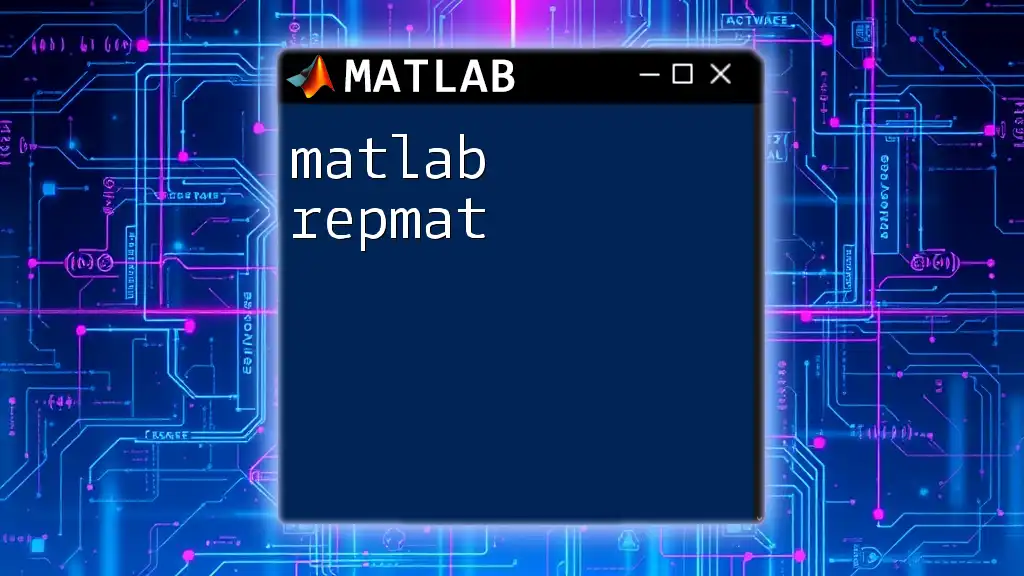
Syntax and Usage
Basic Syntax
The syntax for using `cell2mat` is straightforward. Below is the basic structure:
M = cell2mat(C)
Where:
- C is your input cell array.
- M is the resulting matrix after conversion.
Input Arguments
The primary input to `cell2mat` is the cell array C. This input should consist of elements that are compatible in size and type, e.g., all numeric or all strings that can be converted to numeric values. If the elements vary dramatically, `cell2mat` will fail and throw an error.
Output
The output M produced by `cell2mat` is a matrix formed from the concatenated elements of the cell array C. The dimensions of M will depend on the shape and size of the contents within C.
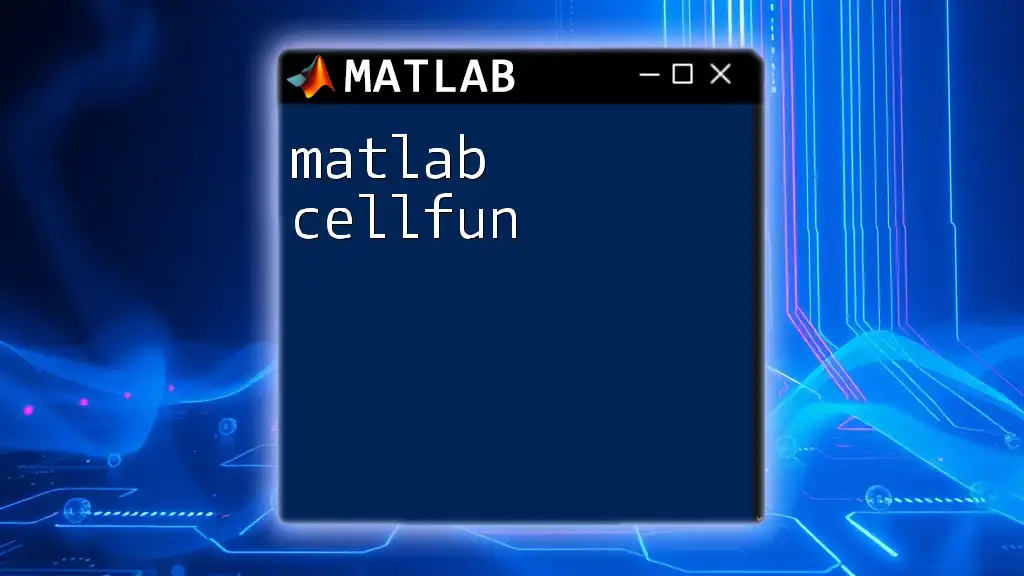
Practical Examples
Example 1: Basic Conversion
Here’s a simple demonstration of converting a cell array to a matrix:
C = {1, 2, 3; 4, 5, 6};
M = cell2mat(C);
disp(M);
In this example, the output will be:
1 2 3
4 5 6
Explanation: The elements in the cell array C are combined into a 2x3 matrix M. Each element of the cell directly corresponds to an entry in the matrix.
Example 2: Converting a Cell Array of Vectors
You can also use `cell2mat` to convert a cell array containing vectors:
C = {[1, 2, 3], [4, 5, 6]};
M = cell2mat(C);
disp(M);
The output would be:
1 2 3 4 5 6
Analysis: Here, each cell stores a row vector, and `cell2mat` concatenates them into a single row within matrix M.
Example 3: Handling Different Data Types
Sometimes, cell arrays contain mixed data types, which can lead to complications:
C = {1, 'a'; 2, 'b'};
try
M = cell2mat(C);
catch err
disp(err.message);
end
In this case, MATLAB will throw an error because you cannot convert strings into numeric form directly with `cell2mat`.
Explanation: Always ensure that the data types within the cell array are compatible to successfully execute the `cell2mat` command.
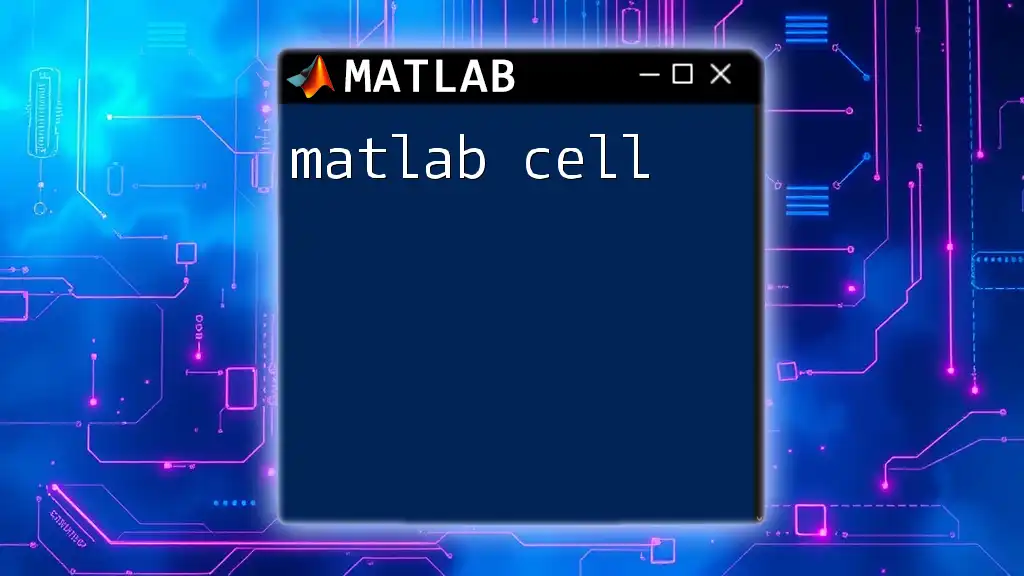
Common Errors and Troubleshooting
Incompatible Data Types
When using `cell2mat`, it is crucial to ensure that all elements of the cell array are of compatible data types. Common type compatibility issues include mixing numeric and string types. You'll encounter an error if you attempt to convert incompatible data types. To check types, you can use the `class` function for each element in your cell array.
Dimension Mismatches
Another frequent issue occurs when the sizes of cell elements don't align for concatenation. For example:
C = {1, 2; [3, 4, 5]};
M = cell2mat(C); % This will result in an error
Best Practices: Before calling `cell2mat`, always verify that all elements are of the same size. Using the `size` function can help in diagnosing dimension issues.
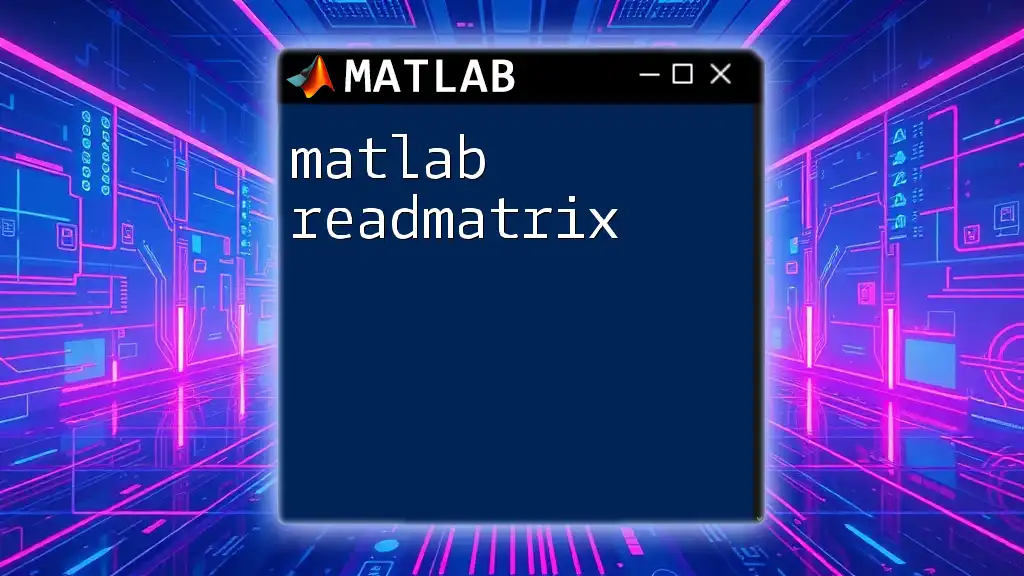
Performance Considerations
Efficiency of `cell2mat`
The `cell2mat` function is usually efficient for moderate-sized arrays. However, when working with very large cell arrays, conversion can be computationally intensive. Therefore, it’s advisable to profile your code using MATLAB’s built-in tools to determine bottlenecks if performance becomes an issue.
Alternatives to `cell2mat`
In some scenarios, other techniques may be better suited than `cell2mat`. For instance, if you're frequently adding or modifying the contents of a cell array, consider maintaining the data as a cell array until all manipulations are complete, converting it only when necessary.
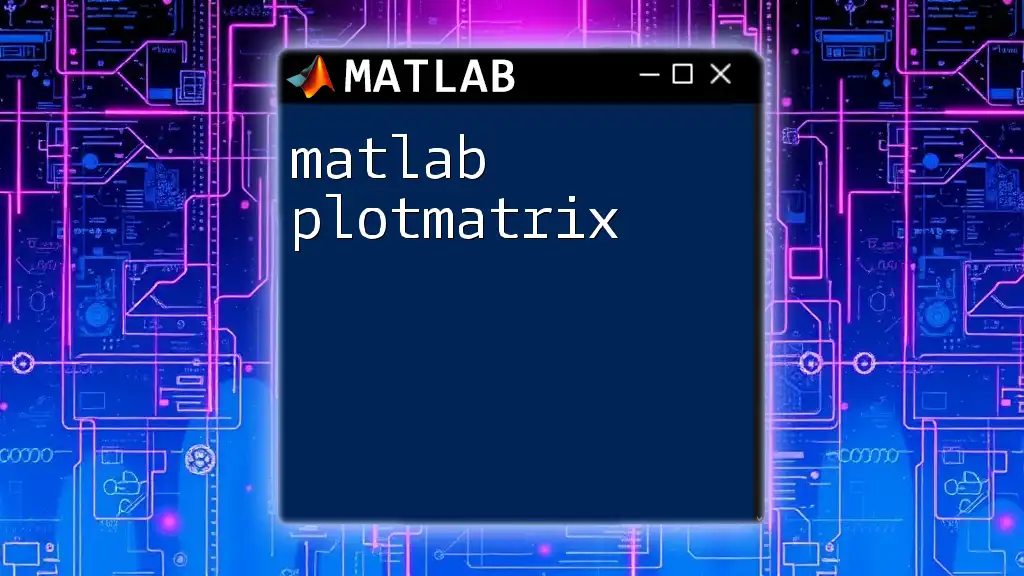
Conclusion
In summary, understanding how to effectively use MATLAB cell2mat is vital for anyone working with MATLAB, especially when dealing with diverse datasets. By harnessing the capabilities of `cell2mat`, you can convert and construct matrices seamlessly from cell arrays. Always remember to ensure data compatibility within your cell arrays to avoid pitfalls, thereby enhancing your coding prowess in MATLAB.
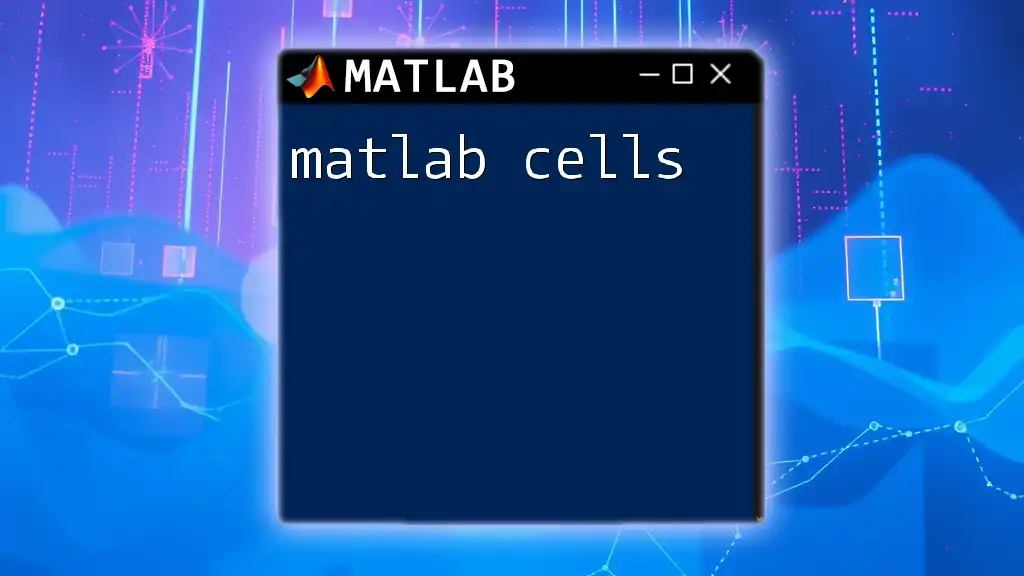
Additional Resources
For more in-depth discussions and further learning, you might want to explore the official MATLAB documentation and tutorials on cell arrays and matrix operations.