An augmented matrix is a matrix that combines the coefficients and the constant terms of a system of linear equations, facilitating the use of matrix methods to solve the system.
Here’s a basic example of creating an augmented matrix in MATLAB:
A = [2 3 5; 4 1 -2; -1 2 1]; % Coefficients of the equations
B = [7; -1; 3]; % Constant terms
augmentedMatrix = [A B]; % Constructing the augmented matrix
Understanding Augmented Matrices
Definition of an Augmented Matrix
An augmented matrix is a matrix that combines the coefficient matrix of a system of linear equations and the constants from the equations into a single, comprehensive structure. This matrix is crucial for solving linear systems using techniques like Gaussian elimination or the Reduced Row Echelon Form (RREF). An augmented matrix is depicted as:
\[ \begin{bmatrix} a_{11} & a_{12} & \dots & a_{1n} & | & b_1 \\ a_{21} & a_{22} & \dots & a_{2n} & | & b_2 \\ \vdots & \vdots & \ddots & \vdots & | & \vdots \\ a_{m1} & a_{m2} & \dots & a_{mn} & | & b_m \\ \end{bmatrix} \]
Components of Augmented Matrices
The augmented matrix is made up of two main parts:
-
Coefficient Matrix: This matrix contains all the coefficients of the variables involved in the system of equations. For example, in the equations:
- \(2x + 3y = 5\)
- \(4x - y = 1\)
The coefficient matrix will be:
A = [2 3; 4 -1];
-
Constant Matrix: This matrix consists of the constant terms on the right-hand side of the equations. For the previous equations:
B = [5; 1];
Combining both, the augmented matrix will appear as:
augmentedMatrix = [A B];
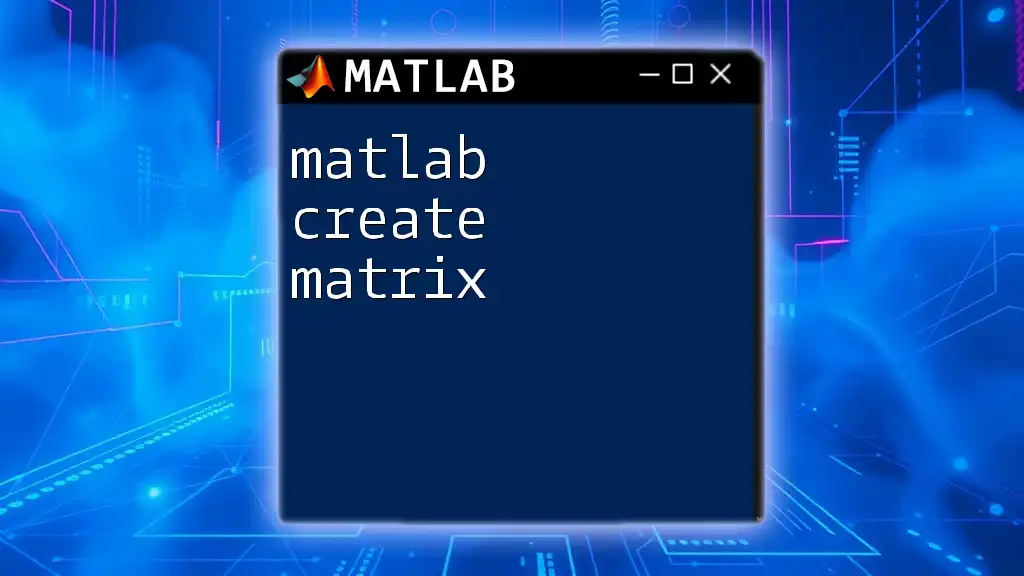
Creating Augmented Matrices in MATLAB
Basic Syntax for Creating Augmented Matrices
In MATLAB, creating an augmented matrix is straightforward. You can construct the coefficient and constant matrices separately and then concatenate them.
To demonstrate, here’s how you can create an augmented matrix from the previously discussed matrices:
A = [2 3; 4 -1]; % Coefficient matrix
B = [5; 1]; % Constant matrix
augmentedMatrix = [A B]; % Creating augmented matrix
disp(augmentedMatrix);
This code will display the augmented matrix as:
2 3 5
4 -1 1
Displaying Augmented Matrices
MATLAB provides several functions to display matrices. The `disp` function is commonly used for this purpose, but you can also format the output by using `fprintf` for more control:
fprintf('Augmented Matrix:\n');
disp(augmentedMatrix);
This can make your output more readable and professional, especially when working with multiple matrices.
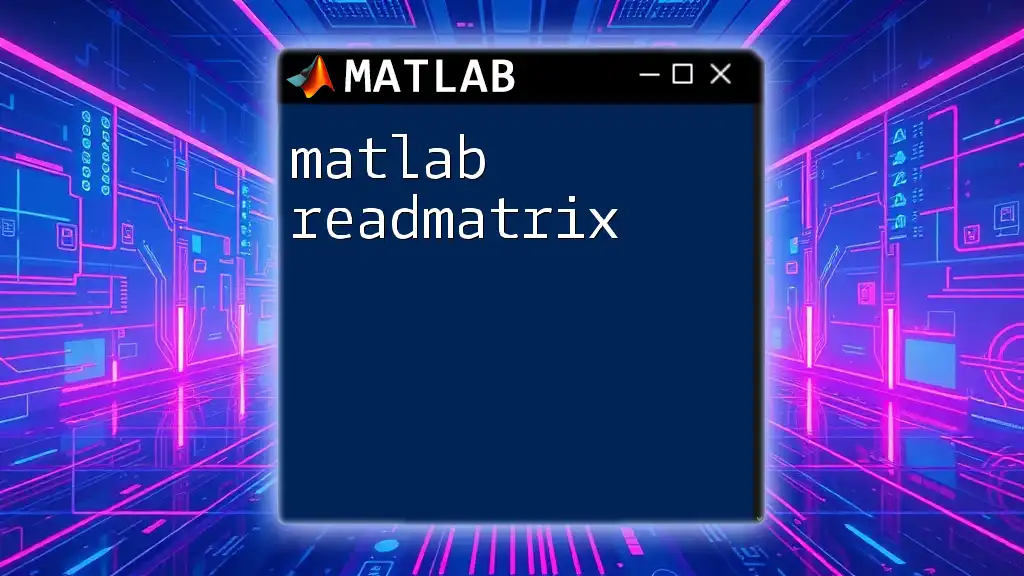
Operations with Augmented Matrices
Row Operations
Row operations are fundamental techniques that manipulate rows of an augmented matrix to bring it to a simpler form. The three types of row operations are:
- Swap: Changing the position of two rows.
- Multiply: Multiplying a row by a non-zero scalar.
- Add: Adding a multiple of one row to another row.
In MATLAB, you can perform these operations directly on the augmented matrix. For example:
% Swap rows
augmentedMatrix([1, 2], :) = augmentedMatrix([2, 1], :);
% Multiply the first row by 2
augmentedMatrix(1, :) = 2 * augmentedMatrix(1, :);
% Add the first row to the second row
augmentedMatrix(2, :) = augmentedMatrix(2, :) + augmentedMatrix(1, :);
Row Echelon Form and Reduced Row Echelon Form
The transformation of an augmented matrix into Row Echelon Form (REF) or Reduced Row Echelon Form (RREF) is crucial for simplifying systems of equations.
-
Row Echelon Form requires that all leading coefficients (the leftmost non-zero entry in each row) are to the right of the leading coefficients in the rows above. Additionally, all entries below a leading coefficient must be zero.
-
Reduced Row Echelon Form goes further, requiring that all leading coefficients are 1 and the only non-zero entries in their columns.
In MATLAB, you can easily achieve RREF using the built-in function `rref`:
rrefMatrix = rref(augmentedMatrix); % Converts to Reduced Row Echelon Form
disp(rrefMatrix);
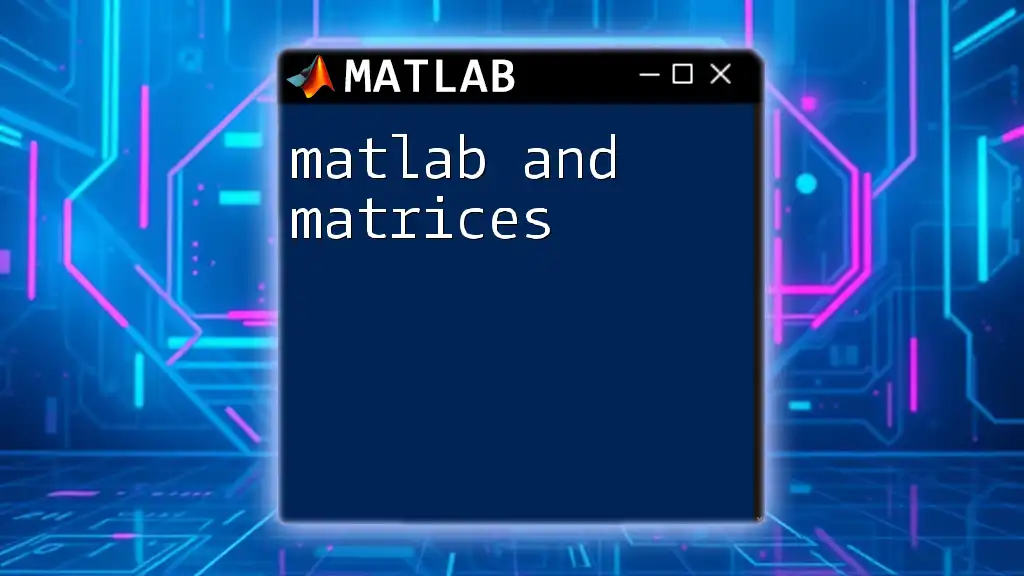
Solving Systems of Equations with Augmented Matrices
Setting Up a System of Equations
When you have a system of equations, you can express it as an augmented matrix. For instance, consider the following system:
- \(2x + 3y = 5\)
- \(4x - y = 1\)
The corresponding augmented matrix will look like this:
augmentedMatrix = [2 3 5; 4 -1 1];
Using MATLAB to Solve the System
To find solutions to the system, you can utilize the `rref` function directly on the augmented matrix:
solvedMatrix = rref(augmentedMatrix); % Find solutions
disp('Solutions:');
disp(solvedMatrix);
The output tells you the values of the variables. If the last column contains any leading variables, those represent the values of \(x\) and \(y\).
Interpretation of Solutions
When analyzing the results from `rref`, we often encounter three scenarios:
- Unique Solution: If every variable can be determined from the matrix.
- Infinite Solutions: If there are free variables present in the results.
- No Solution: If a row forms a contradiction, such as \(0 = 1\).
Understanding this is critical in interpreting the solution of a linear system.
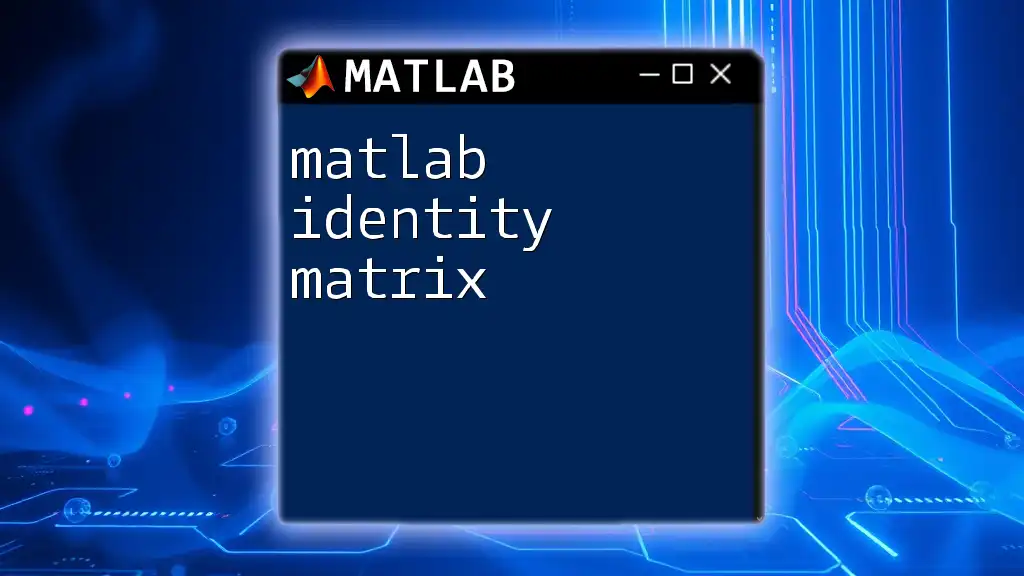
Applications of Augmented Matrices
Engineering and Physics Applications
Augmented matrices find extensive usage in engineering fields, particularly in solving circuit problems, structural analyses, and other applications involving systems of linear equations. For instance, Kirchhoff’s laws can be represented and solved using augmented matrices that model Voltage and Current equations in electrical circuits.
Data Science and Machine Learning
In data science, augmented matrices help in regression analysis where multiple variables need to be analyzed simultaneously. When you fit a regression model, the augmented matrix helps efficiently organize the coefficient and constant terms for computation, facilitating better data handling and decision-making.
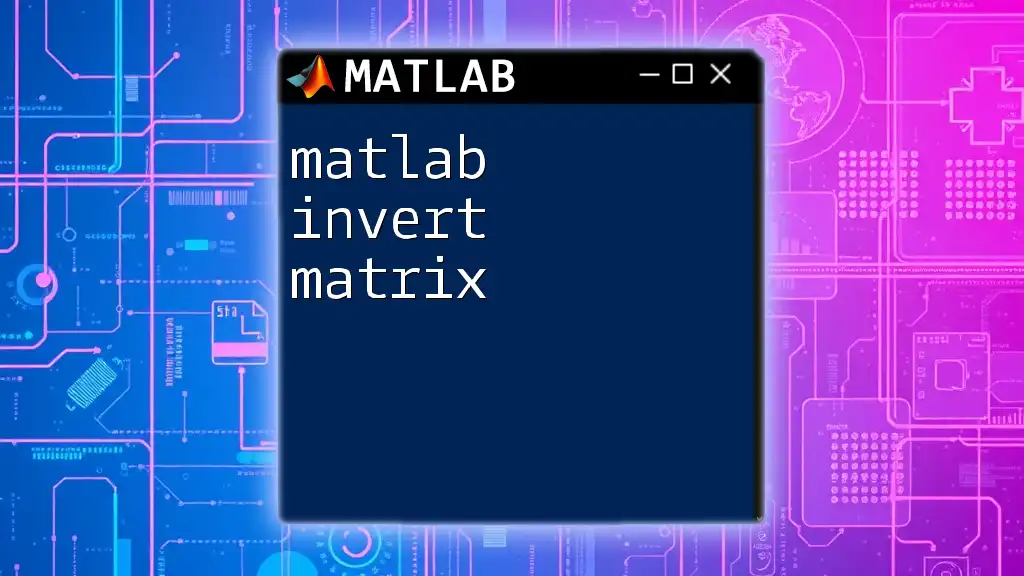
Troubleshooting Common Issues
Common Errors in MATLAB with Augmented Matrices
While using MATLAB to work with augmented matrices, you may encounter several common errors. These include dimension mismatches where the number of rows or columns in your matrices may not align. Always ensure that the dimensions are consistent when performing operations.
Additionally, misusing functions like `rref` without validating the input matrix type can also lead to runtime errors.
Performance Considerations
Efficiency can be a concern particularly with larger matrices. Direct manipulation of large matrices can lead to performance issues. To optimize, prefer using built-in functions and avoiding loops for matrix manipulations.
It's also advisable to understand the underlying operations of MATLAB. Using matrix operations effectively can dramatically improve speed and efficiency in solving complex problems.
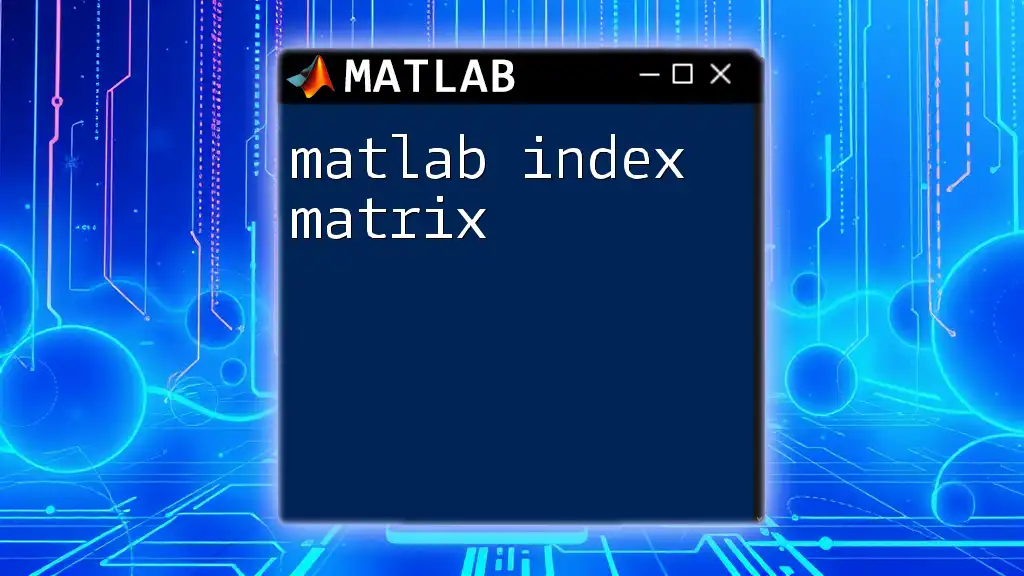
Conclusion
Mastering the concept of MATLAB augmented matrices is essential for anyone interested in linear algebra and its applications. These matrices simplify the process of solving systems of linear equations and can be applied across various fields including engineering, physics, and data science. Practice creating and manipulating augmented matrices in MATLAB to enhance your understanding and skills.
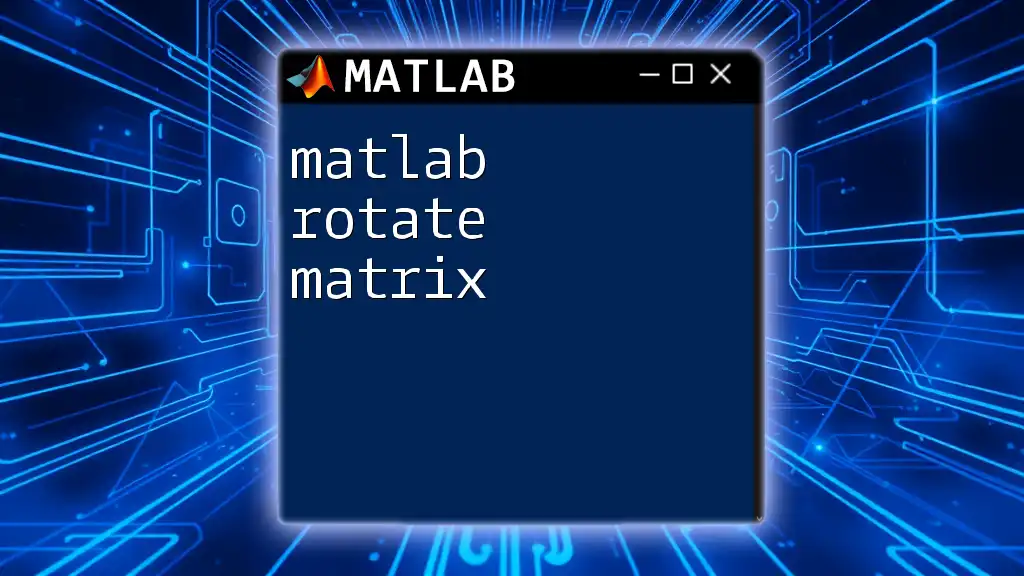
Additional Resources
For those interested in deepening their knowledge, consider exploring MATLAB’s extensive documentation and tutorials. Additionally, there are numerous books and courses available that focus on linear algebra, specifically emphasizing the practical use of augmented matrices in MATLAB.
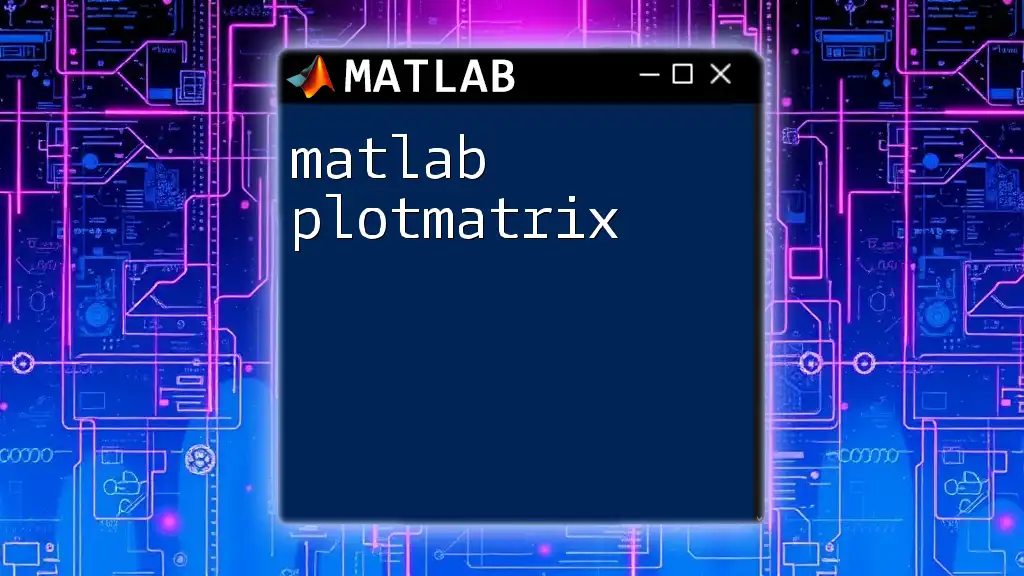
Call to Action
Join our MATLAB training programs today to gain more hands-on experience with augmented matrices and other powerful MATLAB features. Discover easy-to-follow techniques to enhance your MATLAB skills and boost your learning curve!