A MATLAB contour plot is a graphical representation that displays the levels of a variable on a two-dimensional plane, using contour lines to connect points of equal value.
[X, Y] = meshgrid(-5:0.1:5, -5:0.1:5); % Create a grid of points
Z = sin(sqrt(X.^2 + Y.^2)); % Compute values based on a function
contour(X, Y, Z); % Generate the contour plot
What is a Contour Plot?
A contour plot is a graphical representation of a three-dimensional surface, where the value of a given function is represented by contour lines. These lines represent the levels of a variable in a particular range, similar to how a topographic map shows elevation. Contour plots are crucial in various fields, providing insights into the relationship between variables, such as in engineering, physics, and environmental sciences.
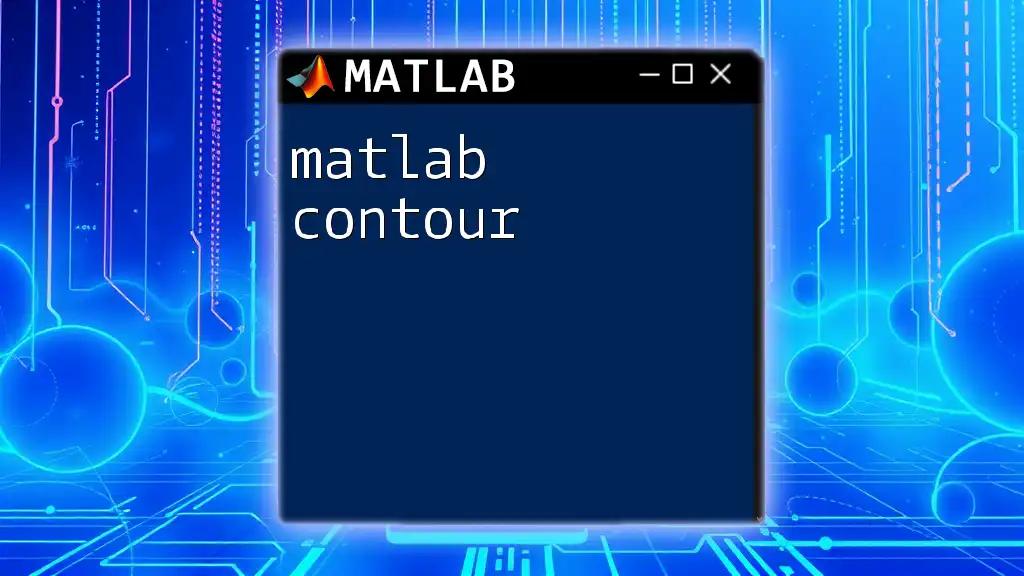
Why Use Contour Plots in MATLAB?
MATLAB stands out as a powerful tool for generating contour plots due to its extensive functionality and user-friendly interface. By using MATLAB contour plot capabilities, users can quickly visualize complex data in an interpretable way, making it easier to analyze relationships and patterns.

Basic Concepts of Contour Plots
Explanation of Contour Lines and Levels
Contour lines represent constant values of a function in a two-dimensional plane. For instance, in a mathematical model representing temperature across a geographical area, each contour line could indicate areas with the same temperature. The space between the lines illustrates the gradient—the closer the lines, the steeper the gradient.
Relationship Between Contour Plots and 3D Surfaces
Contour plots serve as a bridge between two-dimensional and three-dimensional visualizations. While a 3D plot provides a rounded surface view, contour plots allow you to see the hidden details of this surface on a flat plane, simplifying the comprehension of complex data.
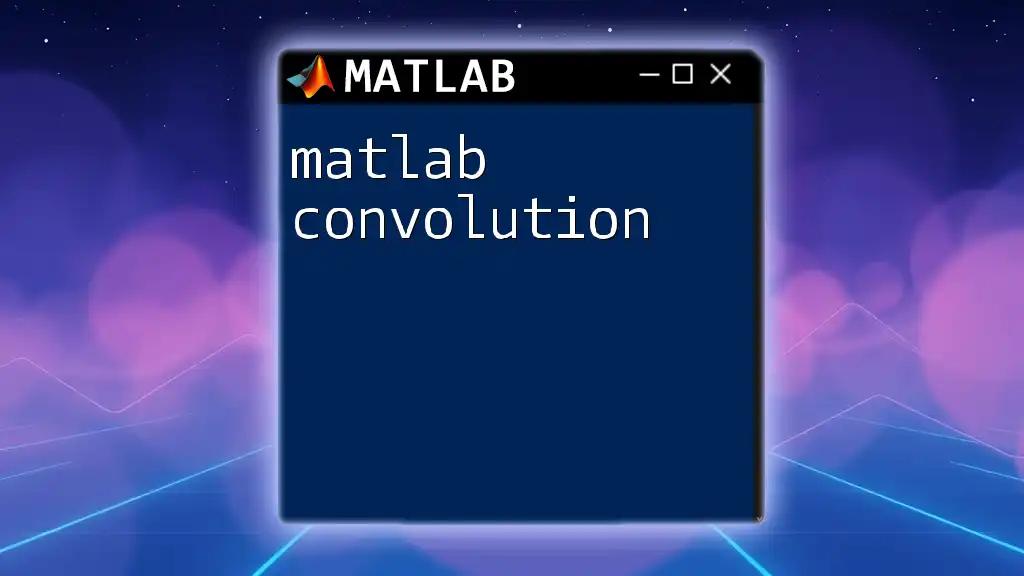
Creating Contour Plots in MATLAB
Basic Syntax for Contour Plotting
In MATLAB, the contour function allows you to create contour plots from a grid of data. The basic syntax is straightforward:
contour(X, Y, Z);
Here, `X` and `Y` are matrices that define the grid points, and `Z` contains the function values at those grid points.
Code Snippet: Basic Contour Plot
Here’s how you can create a basic contour plot in MATLAB:
[X, Y] = meshgrid(-5:0.1:5, -5:0.1:5);
Z = sin(sqrt(X.^2 + Y.^2));
contour(X, Y, Z);
title('Basic Contour Plot');
xlabel('X-axis');
ylabel('Y-axis');
This code creates a 2D contour plot of a sine function over a specified grid. The `meshgrid` function generates a coordinate matrix, while the `contour` function plots the contour lines.
Customizing Your Contour Plot
Adding Labels and Titles
It’s essential to enhance the clarity of your plots by adding relevant titles and labels. The `title`, `xlabel`, and `ylabel` functions in MATLAB allow you to annotate your plots effectively.
Adjusting Colormap
You can customize the appearance of your contour plots by changing the colormap. The `colormap` function adjusts the color scheme used for visual representation:
colormap(jet);
Using the `jet` colormap, for instance, will display a colorful gradient that often highlights variations in your data more vividly.
Enhancing Plot Appearance
Using `clabel` to Label Contours
To make your contour plots more informative, you can label specific contours using the `clabel` function. This can help users instantly grasp the significance of certain levels:
[C, h] = contour(X, Y, Z);
clabel(C, h);
This code snippet will add numeric labels to the contour lines, providing an immediate understanding of the function values at those contours.
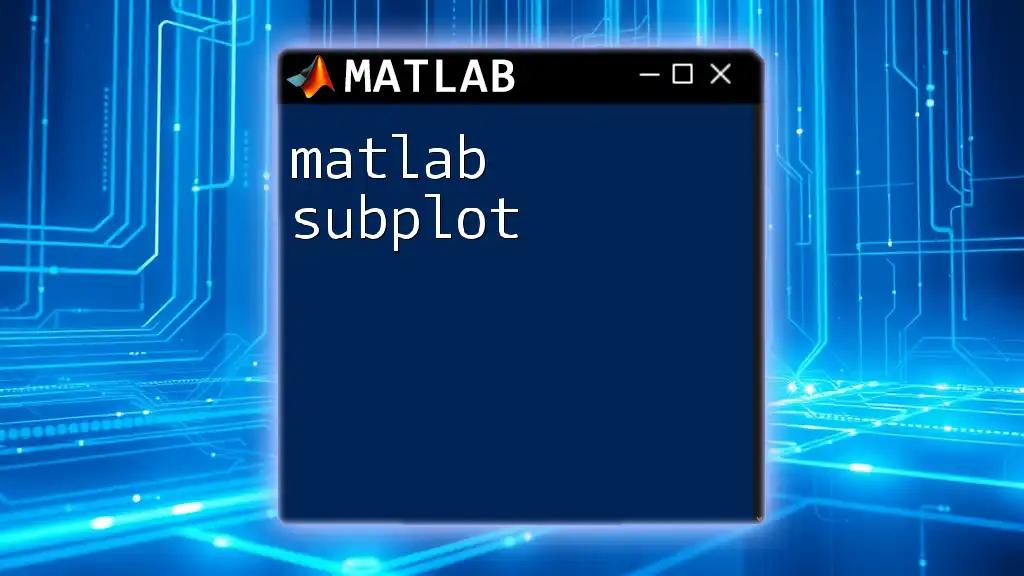
Advanced Contour Plot Techniques
Contour Filled Plots
A filled contour plot, created with the `contourf` function, displays filled areas between contour lines, providing a smoother appearance:
contourf(X, Y, Z);
title('Filled Contour Plot');
colorbar;
This technique enhances the visualization of data clusters while allowing for more straightforward interpretation of how values change across the surface.
Working with Multiple Contour Levels
You can specify contour levels to highlight particular values within a range. This can be done by providing a vector of levels as an additional argument to the `contour` function:
contour(X, Y, Z, [-1, 0, 1], 'ShowText', 'on');
The above snippet creates contour lines at the specified levels of -1, 0, and 1, while displaying the corresponding level values directly on the plot.
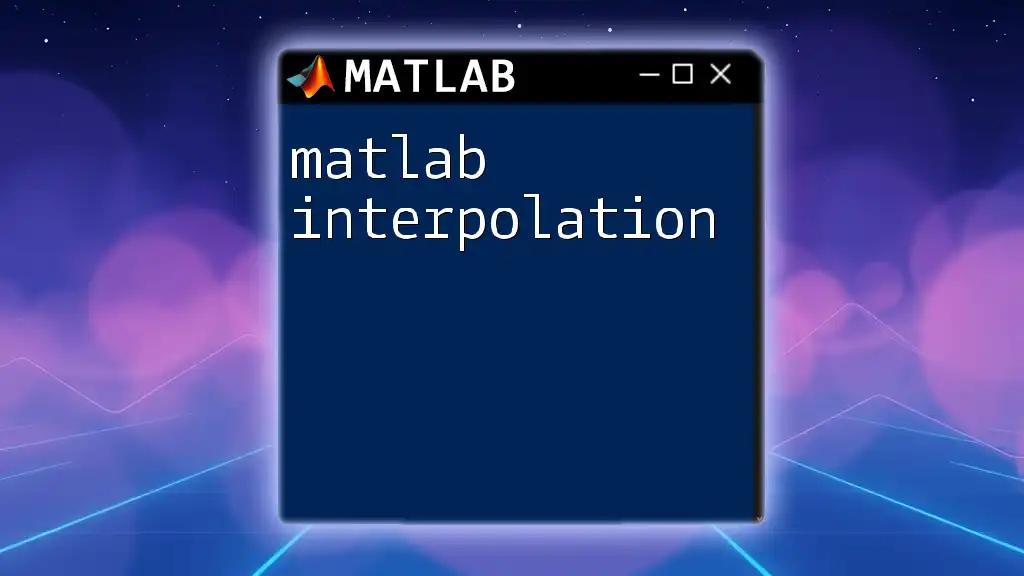
Tips and Tricks for Effective Contour Plots
Choosing the Right Data Resolution
The accuracy and clarity of a contour plot greatly depend on data resolution. Higher resolution data can yield smoother contours but may require additional computational resources. Be mindful of finding a balance that provides detailed information without overwhelming the visualization.
Interpreting Contour Plots
Understanding how to read a contour plot is critical. Contour lines that are tightly spaced indicate a steep change in value, while widely spaced lines suggest a gradual change. Interpreting these aspects can lead to significant insights into the underlying data and its relationships.
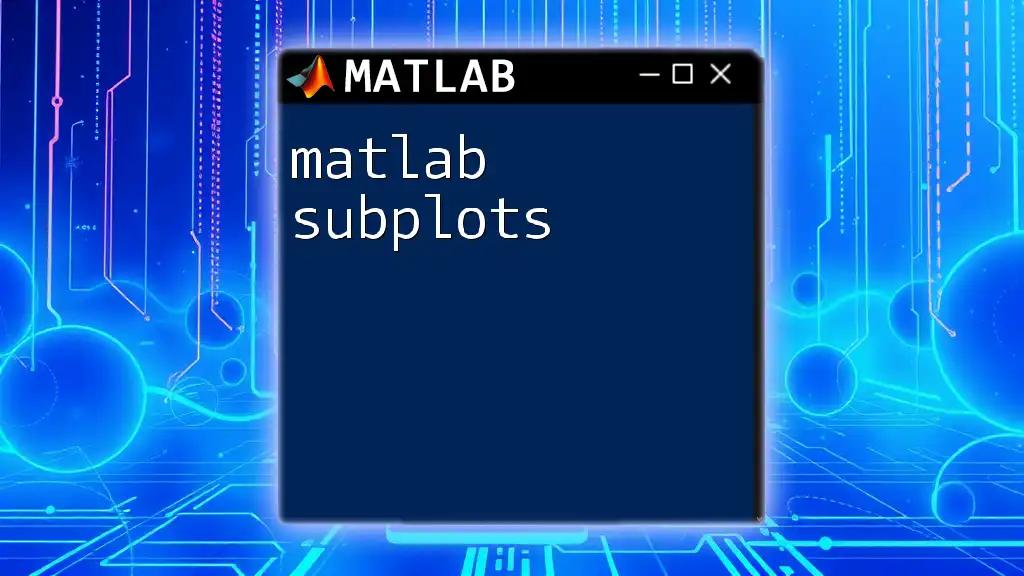
Real-World Applications of Contour Plots
Case Study: Geological Surveys
Geologists often use contour plots to represent features like elevation or mineral distribution. These plots aid in visualizing areas of interest and making predictions about geological formations.
Case Study: Weather Forecasting
Meteorologists utilize contour plots to depict isotherms, which represent varying temperatures across geographical areas. Such visualizations are essential in forecasting weather patterns and understanding climate changes.
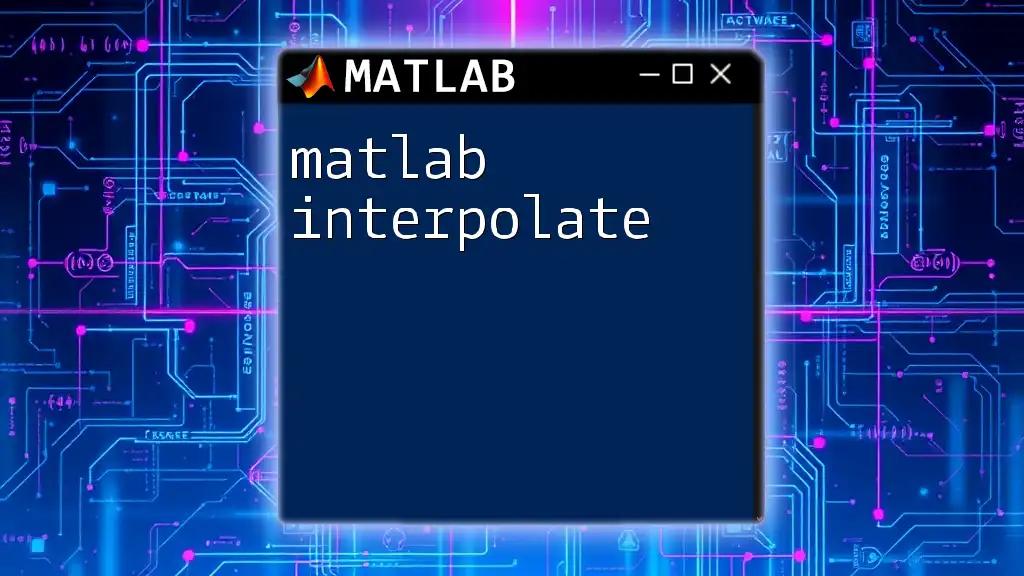
Troubleshooting Common Issues
Common Errors in Contour Plotting
Users can occasionally make mistakes, such as providing mismatched dimensions for the `X`, `Y`, and `Z` matrices. Always ensure your data matrices are of the same size, as this is crucial for a valid contour plot.
How to Debug Your Contour Plots
If your contour plots don't display as expected, consider simplifying your data. Try plotting a subset or use the `mesh` function to check the underlying grid points and values. This can often reveal discrepancies in your data setup.

Conclusion
In summary, the MATLAB contour plot function is a powerful tool for visualizing the relationships between variables in multidimensional data. From creating simple contour plots to customizing them for enhanced clarity and insight, MATLAB provides a comprehensive platform for effective data visualization. I encourage you to practice these techniques to deepen your understanding and improve your data presentation skills.
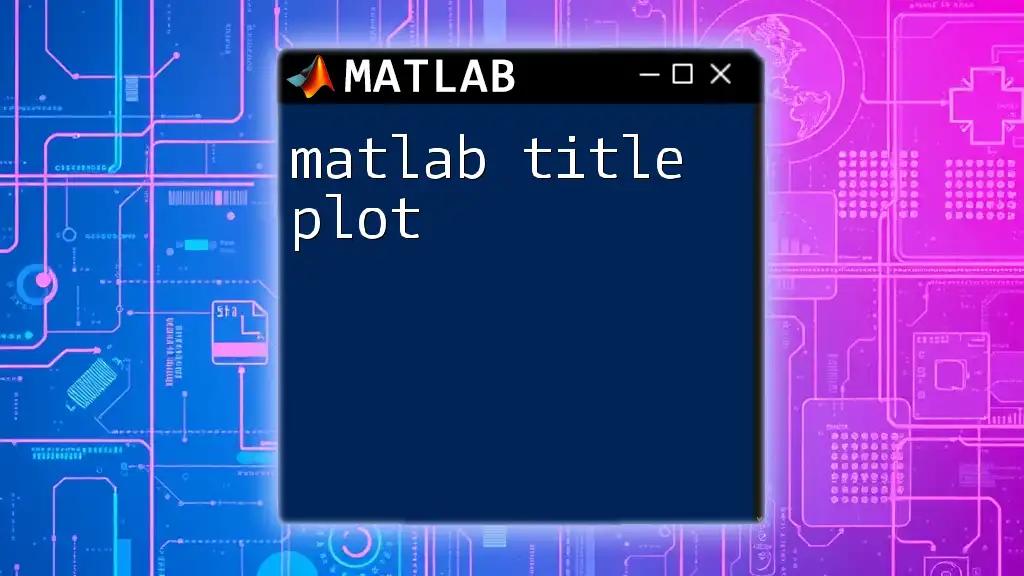
Additional Resources
For those eager to learn more, consider exploring additional literature on MATLAB plotting techniques and data visualization principles. Engage with community forums for real-time discussions and tips from fellow MATLAB users.
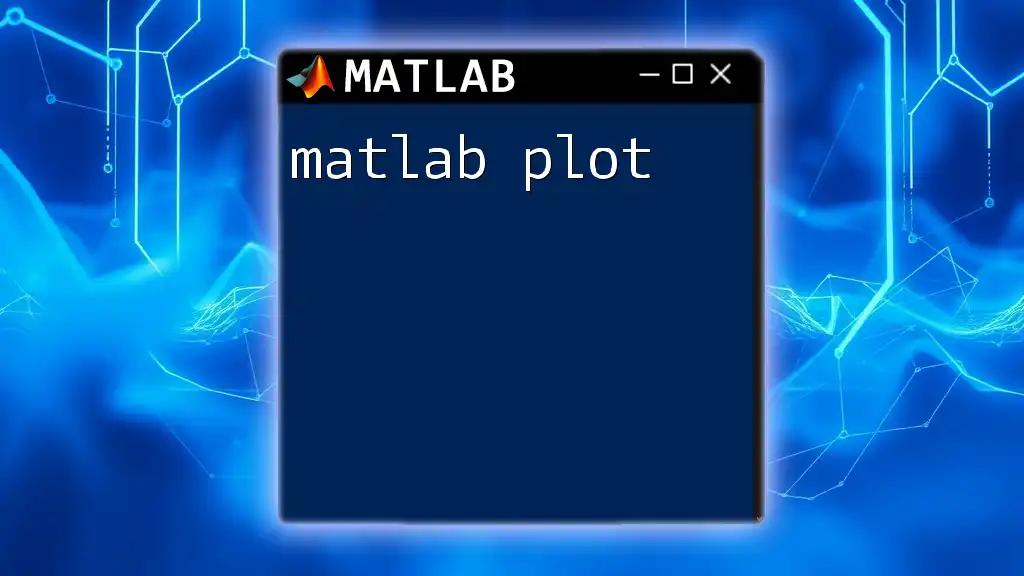
Call to Action
If you're interested in mastering MATLAB and enhancing your data visualization skills, I invite you to join our MATLAB course. Our teaching program is designed to offer in-depth training and practical applications, ensuring you gain confidence in using MATLAB for your projects.