The `fopen` function in MATLAB is used to open a file and returns a file identifier for reading or writing operations.
fileID = fopen('example.txt', 'r'); % Opens 'example.txt' for reading
Understanding fopen
MATLAB's `fopen` function is a critical component for file handling, allowing users to perform operations such as reading from and writing to files. Understanding how to effectively use `fopen` can greatly enhance your ability to manage data in MATLAB, which is essential for data analysis tasks, simulations, and more.
Overview of File Operations
When working with files in MATLAB, one of the most common tasks involves opening, reading, writing, and closing files. The `fopen` command provides the foundation for these operations, supporting a variety of file formats such as text and binary files.
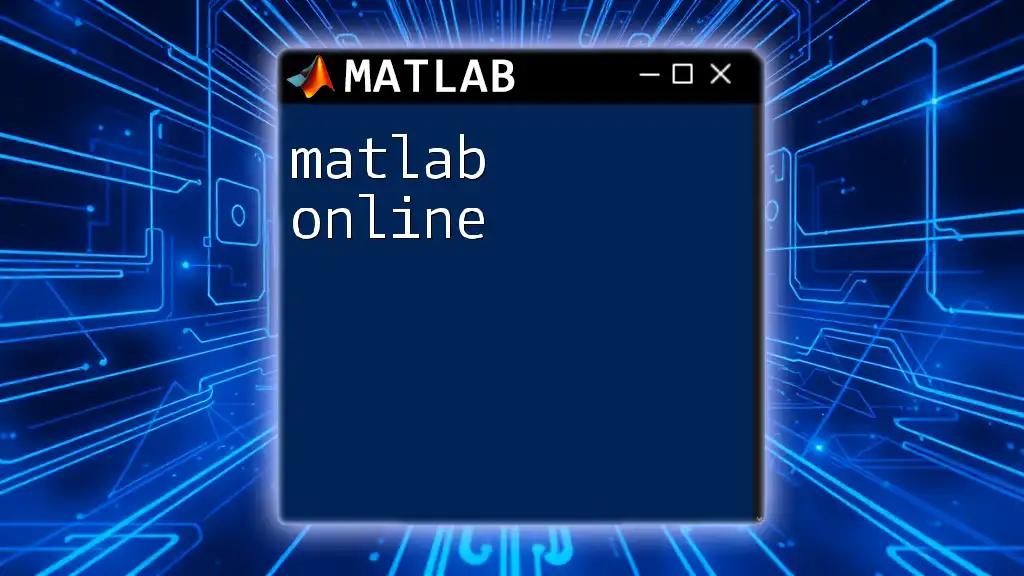
Syntax and Parameters of fopen
Basic Syntax
The basic syntax of the `fopen` function is as follows:
fid = fopen(filename, permission);
Common Parameters
-
File Identifier (fid): A unique identifier that MATLAB uses to manage the open file. This identifier is crucial for subsequent file operations, like reading or writing.
-
Permission Modes: These are the access permissions for the file you are opening:
- `'r'`: Opens the file for reading.
- `'w'`: Opens the file for writing, and if the file already exists, it overwrites it.
- `'a'`: Opens the file for appending at the end of the file.
-
File Name and Path: A string specifying the name of the file to be opened, which can be specified with its relative or absolute path. Consider specifying paths carefully to avoid errors.
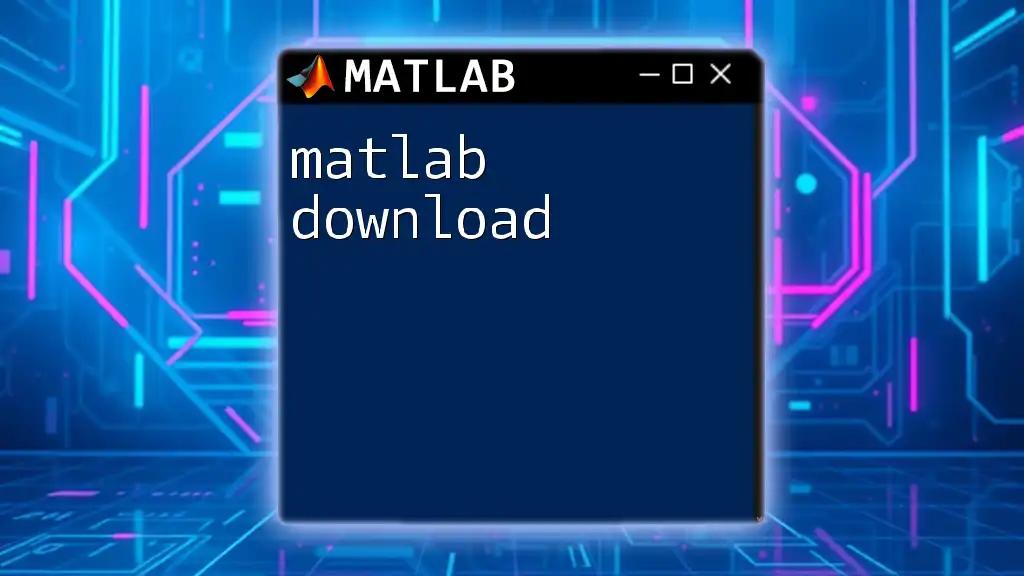
Modes of fopen
Reading Modes
When reading files, you have specific modes to consider:
- `'r'`: This mode opens the file strictly for reading. If the file does not exist, MATLAB will return `-1`, indicating an error.
Example usage:
fid = fopen('data.txt', 'r');
- `'r+'`: Opens the file for both reading and writing. The file must exist, or the function will return an error.
Writing Modes
For writing to files, you can choose from the following modes:
-
`'w'`: Opens a file for writing. If the file already exists, it will be overwritten.
-
`'a'`: This mode opens a file for writing, but new content will be appended to the end without erasing existing data.
Binary Modes
When working with binary files, you can use:
-
`'rb'`: Opens a file for reading in binary mode.
-
`'wb'`: Opens a file for writing in binary mode.
Example showing binary writing:
fid = fopen('data.bin', 'wb');
Error Modes
- `'e'`: Specifies to open the file with error handling.
- `'n'`: Opens without error handling.
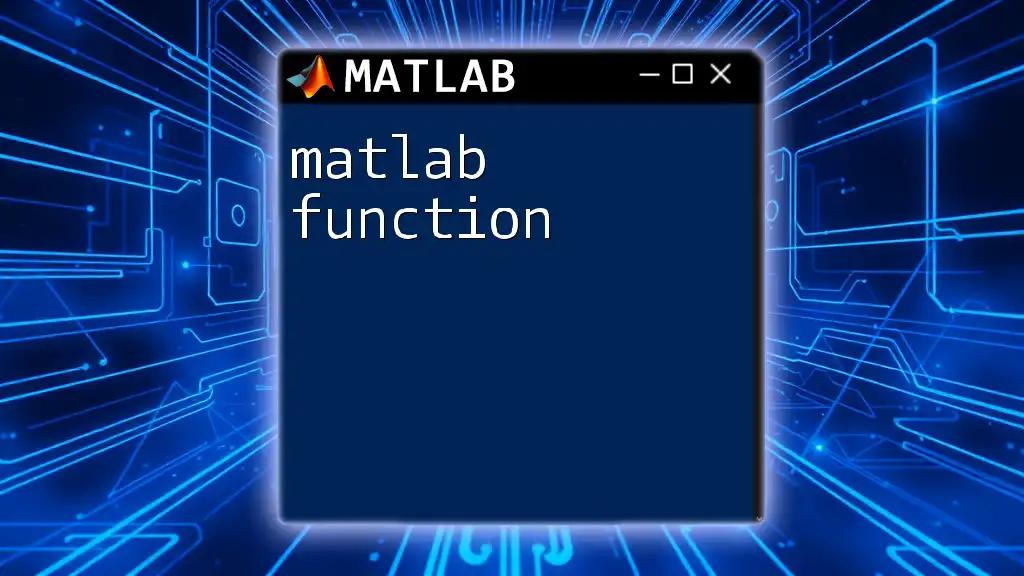
Error Handling with fopen
Checking for Errors
To ensure successful file operations, it is essential to check for errors after attempting to open a file. If the file identifier `fid` equals `-1`, it indicates that the file could not be opened.
Example:
fid = fopen('missing_file.txt', 'r');
if fid == -1
error('File could not be opened.');
end
Common Error Codes
Table of possible return values from `fopen`:
- `-1`: File could not be opened due to missing file or incorrect permissions.
- Other Numbers: Each number corresponds to a successfully opened file's identifier.
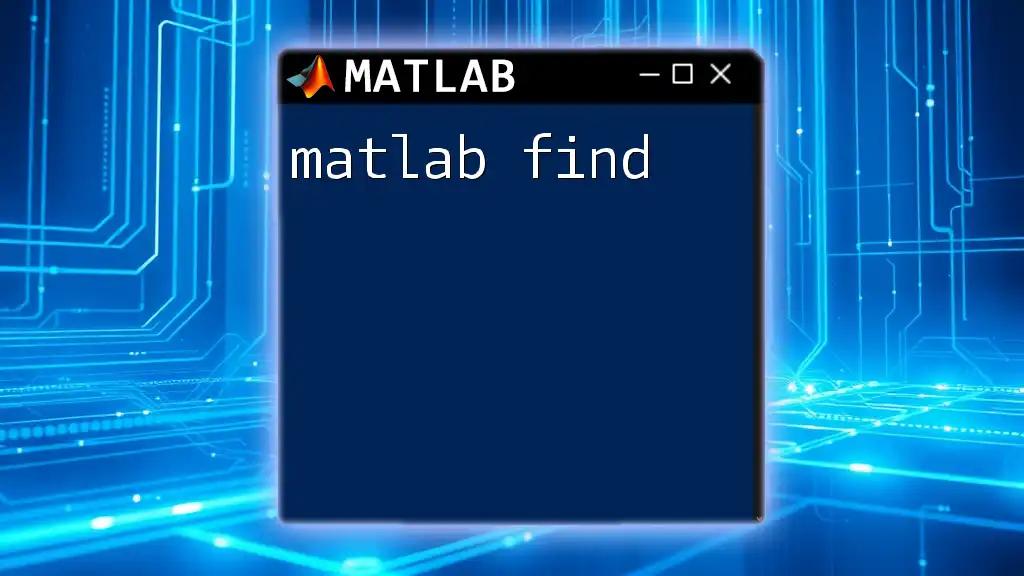
Closing Files
Importance of Closing Files
Closing files is crucial for managing system resources and avoiding data loss. Files that remain open can lead to memory leaks or prevent access to the files in the future.
Using fclose
The syntax for closing a file is simple:
fclose(fid);
It's important to check if the closing process was successful by ensuring that `fclose` returns zero, indicating success.
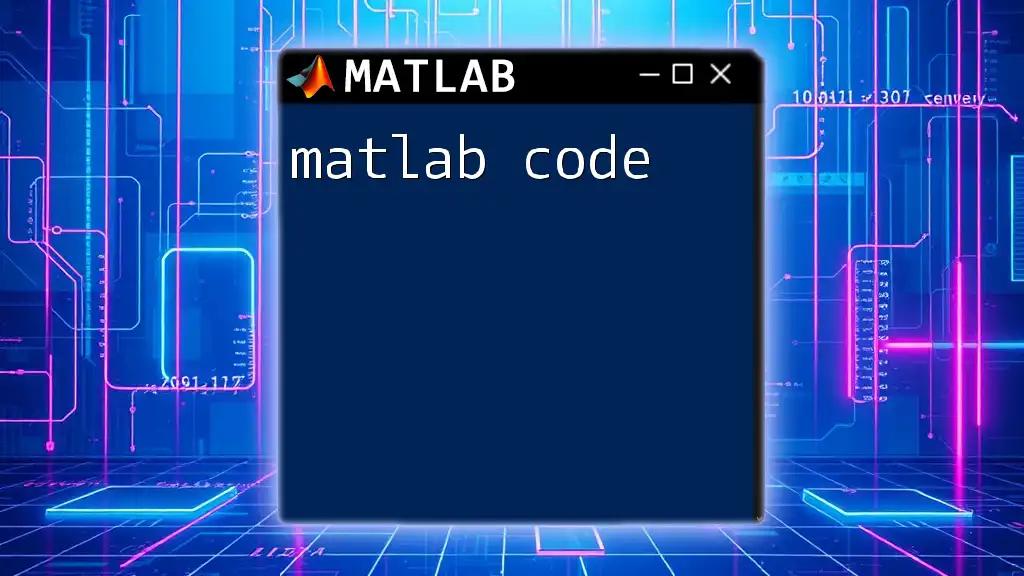
Practical Examples of fopen
Example 1: Reading from a Text File
Here's a basic example of how to read data from a text file using `fopen`:
fid = fopen('data.txt', 'r');
data = textscan(fid, '%f %f', 'Delimiter', ',');
fclose(fid);
In this example, after opening the file in read mode, we use `textscan` to read formatted data based on defined delimiters. Finally, we close the file to maintain good practice.
Example 2: Writing to a Text File
To write data to a text file, the command would look like this:
fid = fopen('output.txt', 'w');
fprintf(fid, '%f %f\n', rand(10,2)');
fclose(fid);
This code generates random numbers and writes them to `output.txt`. The `%f %f\n` format specifies how the data will be structured in the file, which is crucial for correct data organization.
Example 3: Appending to a Binary File
Appending data to an existing binary file can be done as follows:
fid = fopen('data.bin', 'ab');
fwrite(fid, rand(5,1), 'double');
fclose(fid);
In this example, we use `fwrite` to append random `double` values to `data.bin`. The `ab` mode ensures that we add to the end of the file without losing existing data.
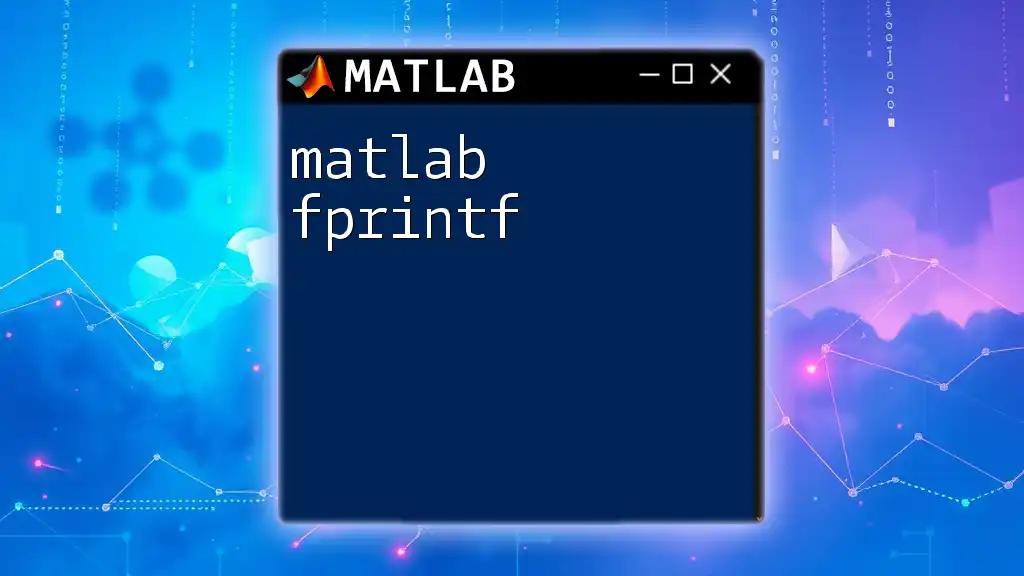
Best Practices When Using fopen
Managing File Paths
A good practice is to use the `fullfile()` function, which helps in constructing paths that are platform-independent, reducing the likelihood of errors related to path formatting.
Checking for File Existence
Before attempting to open a file, check if it exists using:
if exist('data.txt', 'file') == 2
fid = fopen('data.txt', 'r');
end
This practice prevents unnecessary errors when trying to open non-existent files.
Using Try-Catch Blocks
For robust error management, encapsulate your file handling operations in a `try-catch` block to gracefully handle exceptions.
try
fid = fopen('data.txt', 'r');
catch
disp('Error opening file');
end
Using `try-catch` will allow your program to continue running even if an error occurs during file operations.
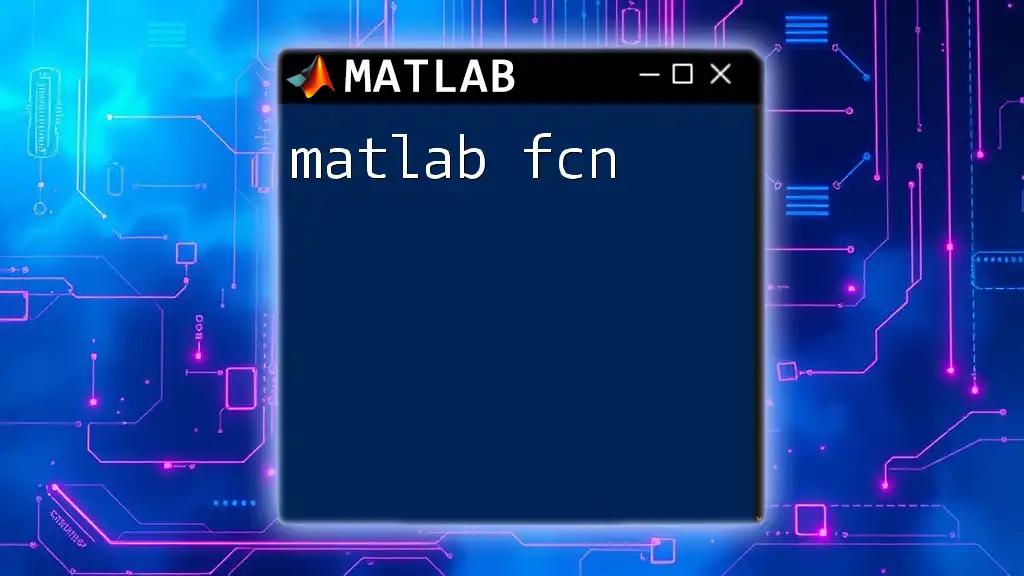
Conclusion
Understanding `matlab fopen` is essential for effective file management in your MATLAB applications. By mastering how to open and manipulate files, as well as handling errors gracefully, you can significantly improve your data analysis and processing tasks.
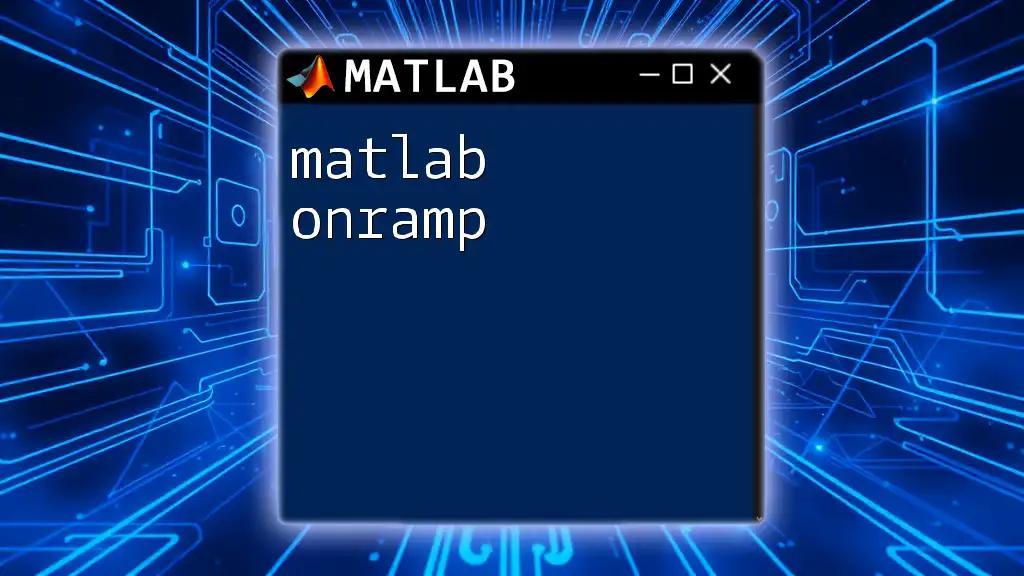
Call to Action
Join our community of MATLAB enthusiasts and explore our courses designed to help you master MATLAB commands, including file handling and more. Take the initiative to refine your skills today!