In MATLAB, complex numbers are created using the `real` and `imag` functions or simply by defining them with the imaginary unit `i` or `j`, allowing for easy manipulation of both real and imaginary parts.
Here's a code snippet that demonstrates how to create and manipulate complex numbers in MATLAB:
% Creating complex numbers
z1 = 3 + 4i; % 3 is the real part, 4 is the imaginary part
z2 = 1 - 2j; % 1 is the real part, -2 is the imaginary part
% Performing operations
sum = z1 + z2; % Complex addition
product = z1 * z2; % Complex multiplication
magnitude = abs(z1); % Magnitude of z1
Understanding Complex Numbers
Definition of Complex Numbers
Complex numbers are fundamental to many areas of mathematics and engineering. A complex number is generally expressed in the form \( z = a + bi \), where \( a \) represents the real part and \( b \) is the imaginary part. The imaginary unit \( i \) is defined by the property that \( i^2 = -1 \). This unique property allows for the extension of basic arithmetic operations among real numbers, thereby introducing a new dimension to numerical computation.
Visual Representation
Understanding complex numbers graphically can enhance comprehension. Complex numbers can be represented in a two-dimensional plane known as the complex plane. In this representation, the x-axis corresponds to the real part, while the y-axis corresponds to the imaginary part.
To visualize complex numbers in MATLAB, you can use the following code snippet:
z = [1+2i, 3+4i, 5-1i];
plot(real(z), imag(z), 'o');
xlim([-5 5]);
ylim([-5 5]);
xlabel('Real Part');
ylabel('Imaginary Part');
title('Complex Numbers in the Complex Plane');
grid on;
This graphical representation not only aids in visual learning but also helps in grasping concepts such as addition and subtraction, where the geometric interpretation involves straightforward vector addition.
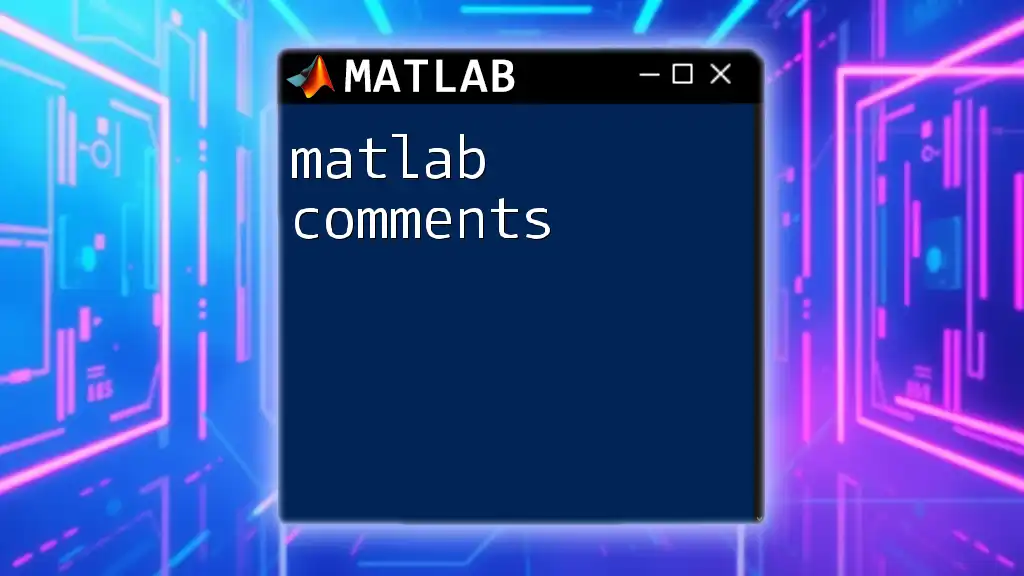
Working with Complex Numbers in MATLAB
Creating Complex Numbers
Creating complex numbers in MATLAB is achieved either through the direct use of mathematical expressions or the `complex` function.
For example:
z1 = complex(3, 4); % Creates 3 + 4i
z2 = 2 + 5i; % Creates 2 + 5i
Both methods are equally valid, but some programmers prefer `complex` for readability, especially in scripts involving numerous complex number generations.
Basic Operations
Addition and Subtraction
In MATLAB, you can easily add and subtract complex numbers just as you would with real numbers. Understanding these operations conceptually and algebraically helps in applying them efficiently.
Consider the following code example:
sum = z1 + z2; % (3 + 4i) + (2 + 5i)
difference = z1 - z2; % (3 + 4i) - (2 + 5i)
In this example, the resulting `sum` would be \( 5 + 9i \), while the `difference` results in \( 1 - 1i \).
Multiplication and Division
Multiplication and division of complex numbers follow specific rules. The multiplication of two complex numbers \( z_1 = a + bi \) and \( z_2 = c + di \) can be expressed as:
\[ z_1 \cdot z_2 = (ac - bd) + (ad + bc)i \]
Similarly, for division, the conjugate of the denominator is often employed for simplification. Here’s how you could perform these operations in MATLAB:
product = z1 * z2; % (3 + 4i) * (2 + 5i)
quotient = z1 / z2; % (3 + 4i) / (2 + 5i)
Understanding these operations is crucial as they often appear in applications requiring calculations of impedance in electrical engineering and signal processing.
Advanced Operations
Conjugate
The complex conjugate of a complex number \( z = a + bi \) is defined as \( \overline{z} = a - bi \). It holds significance in many mathematical applications, particularly in calculations involving division and finding magnitudes.
To obtain the conjugate in MATLAB, you can use:
conj_z1 = conj(z1); % Conjugate of 3 + 4i
Magnitude and Angle
The magnitude (or modulus) of a complex number \( z \) can be calculated using the formula:
\[ |z| = \sqrt{a^2 + b^2} \]
The angle (or argument) provides information about the direction of the vector representing the complex number in the complex plane. To compute these values in MATLAB, use:
magnitude = abs(z1); % Magnitude of 3 + 4i
angle = angle(z1); % Angle in radians
These calculations provide insight into the properties of complex numbers, which are critical in fields such as control systems and signal processing.
Polar Representation of Complex Numbers
Complex numbers can also be expressed in polar form, which includes the magnitude and angle. The polar form is given by:
\[ z = r(\cos(\theta) + i\sin(\theta)) \]
where \( r \) is the magnitude and \( \theta \) is the angle. Converting between rectangular and polar forms can be accomplished using the following MATLAB commands:
[theta, rho] = cart2pol(real(z1), imag(z1)); % Converts to polar
Understanding this representation allows for simplified computation in many mathematical and engineering applications.
Using Built-in Functions
MATLAB provides a suite of built-in functions for handling various operations related to complex numbers. Key functions include:
- `real()`: Extracts the real part of a complex number
- `imag()`: Extracts the imaginary part
- `abs()`: Calculates the magnitude
- `angle()`: Computes the argument
- `conj()`: Finds the conjugate
These can be easily applied as shown below:
real_part = real(z1); % Returns the real part
imag_part = imag(z1); % Returns the imaginary part
Utilizing these functions allows for efficient coding, reducing the need for manual calculations.
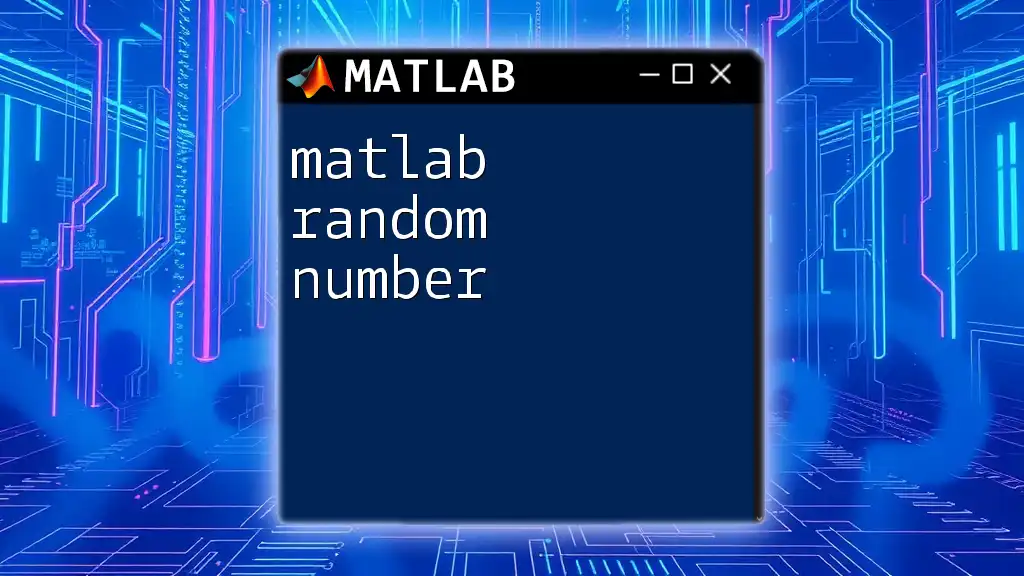
Practical Applications
Applications in Engineering
Complex numbers play a pivotal role in electrical engineering, particularly in analyzing AC circuits. For example, impedance calculations in circuits often involve complex numbers to account for resistance and reactance.
When analyzing a simple RLC circuit, one might use the following MATLAB snippet to calculate the total impedance:
R = 10; % Resistance
L = 0.1; % Inductance in Henries
C = 0.001; % Capacitance in Farads
f = 60; % Frequency in Hertz
omega = 2 * pi * f; % Angular frequency
Z_L = omega * L * 1i; % Inductive reactance
Z_C = -1 / (omega * C * 1i); % Capacitive reactance
Z_total = R + Z_L + Z_C; % Total impedance
This example illustrates the necessity of proficiently working with MATLAB complex numbers to perform such calculations.
Applications in Physics
In physics, complex numbers are essential for representing wave functions and phenomena in quantum mechanics. For instance, the amplitude and phase of periodic waves can be easily expressed using complex notation.
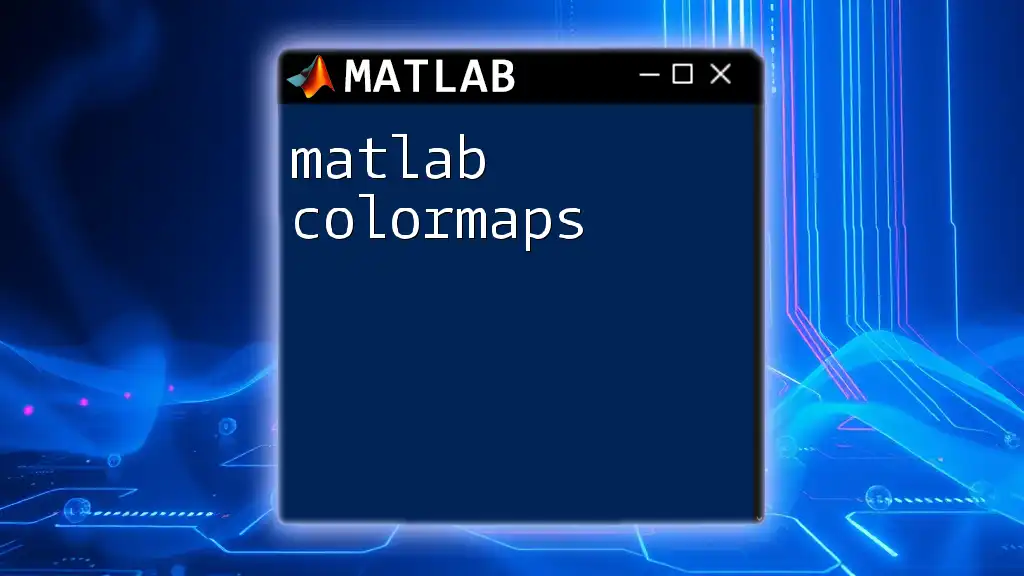
Troubleshooting Common Issues
Error Checking
When working with complex numbers, MATLAB users may encounter misunderstandings, particularly regarding data types. Attempting arithmetic operations with mixed data types can lead to unexpected errors. Familiarizing yourself with common errors, such as trying to take the real part of a non-complex number, will help in avoiding these pitfalls.
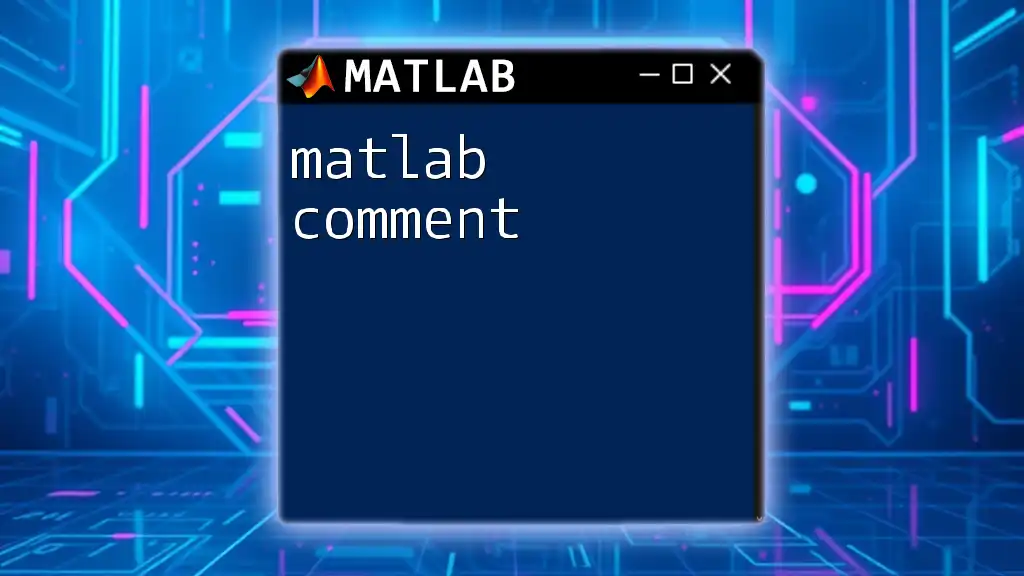
Conclusion
Throughout this guide, we have delved into the foundational elements of MATLAB complex numbers, their operations, and their applications across several fields. Understanding complex numbers not only enhances your MATLAB coding capabilities but also strengthens your problem-solving skills in practical scenarios.
As you continue to explore and apply these concepts, consider experimenting with complex numbers in your projects. Engaging with them will solidify your understanding and open new avenues in your studies or professional pursuits.
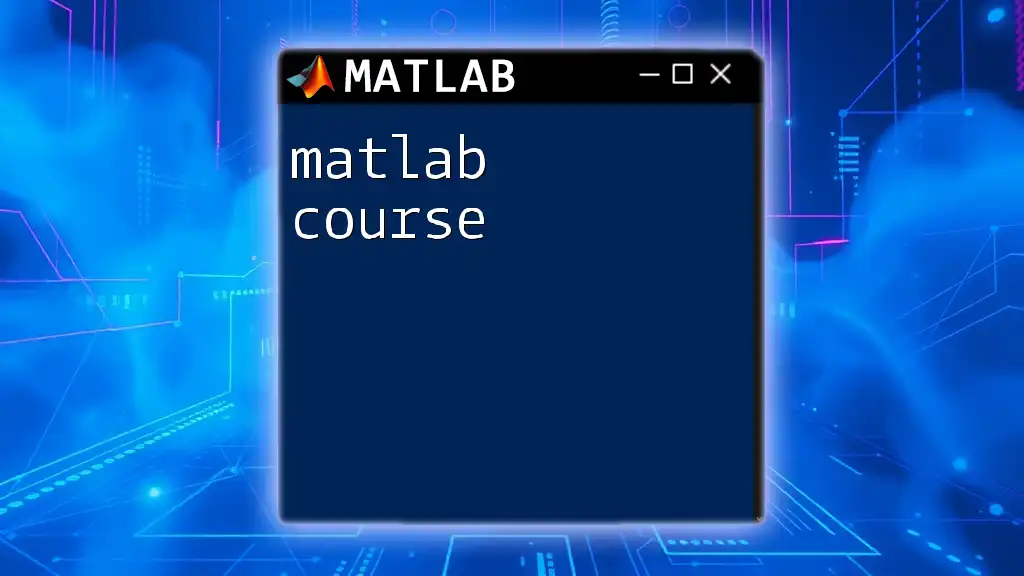
Additional Resources
For those looking to delve deeper into the fascinating world of complex numbers in MATLAB, visit the official MATLAB documentation for complex numbers. Recommended books and online courses are also excellent resources for further learning.
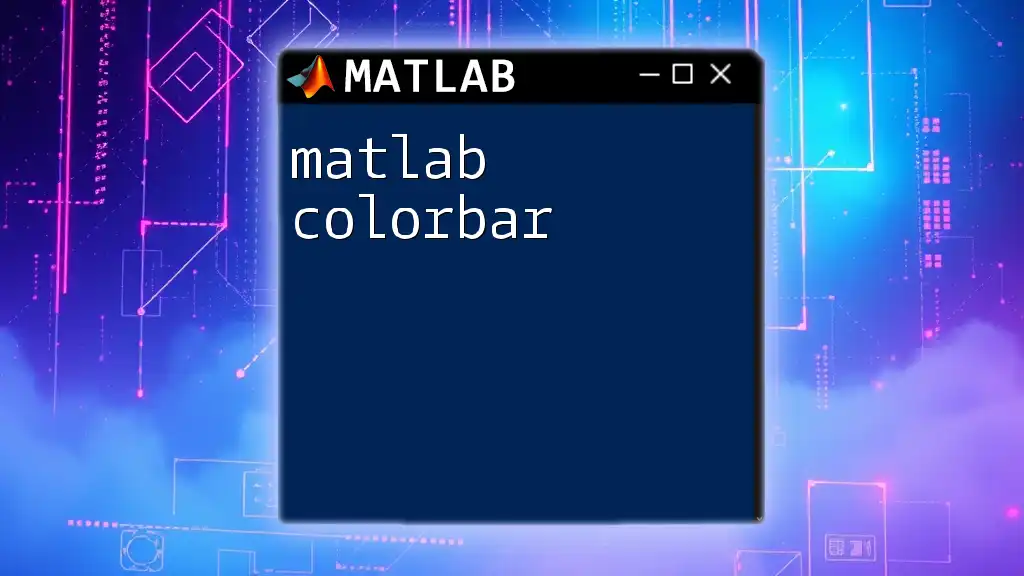
Call to Action
Begin your journey with complex numbers by applying your knowledge in projects and simulations. Our upcoming classes and tutorials will provide you with more insights and practical experience. Stay tuned!