The `conv2` function in MATLAB computes the 2D convolution of two matrices, which is commonly used in image processing and signal analysis.
Here's a basic usage example in MATLAB:
A = [1 2; 3 4];
B = [0 1; 1 0];
C = conv2(A, B);
What is conv2?
The `conv2` function in MATLAB is a powerful tool for performing 2D convolution on matrices. It is widely used in image processing, signal processing, and various mathematical computations, allowing users to apply filters to images and signals efficiently. Convolution helps in manipulating the data by emphasizing certain aspects, such as edges or textures, which can be crucial for analysis.
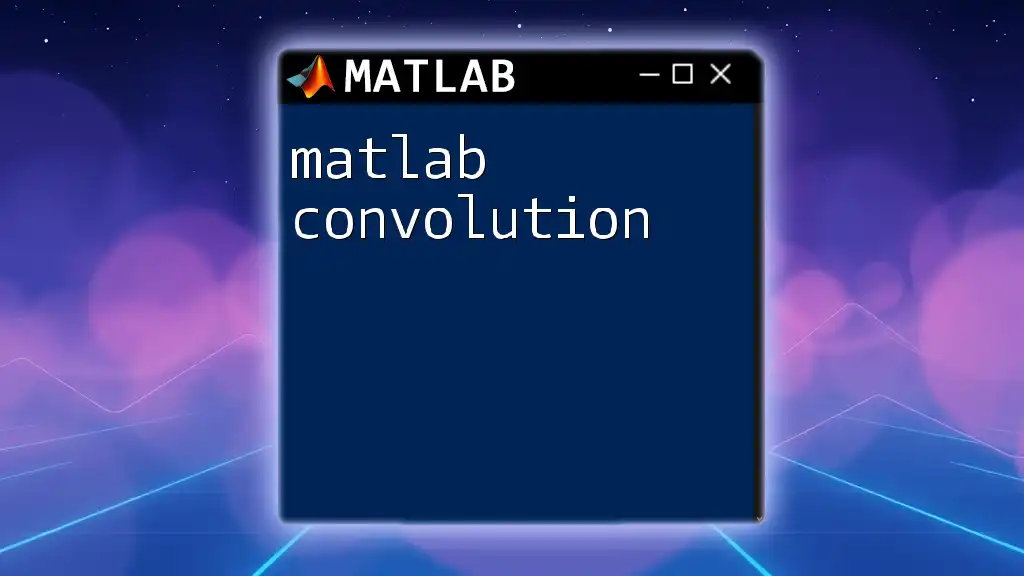
Prerequisites
Before diving into `conv2`, it's essential to have a basic understanding of MATLAB and the concept of convolution in mathematical terms. In simple terms, convolution combines two sets of information, often represented as matrices or signals.
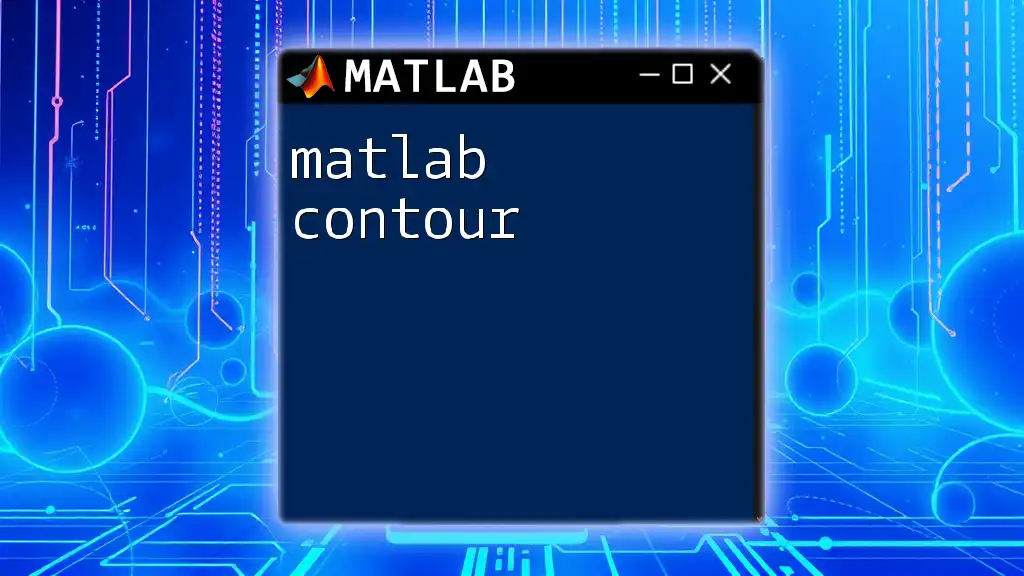
Understanding Convolution
The Concept of Convolution
Convolution can be defined as a mathematical operation that blends two functions into a third function. In the context of matrices, convolution involves sliding one matrix (the kernel) over another matrix (typically an image) to produce a new matrix. This operation is crucial for various applications such as blurring, sharpening, and pixel manipulation.
When visualizing convolution, think of the kernel entering the image at each pixel, performing a weighted sum based on its neighboring pixels.
Types of Convolution
There are two types of convolution:
- Linear Convolution: This is the direct application of convolution, where each output pixel is computed as a weighted sum of its neighbors.
- Non-linear Convolution: In this case, output pixels can be derived from operations other than simple addition, such as taking the maximum value.
In MATLAB, `conv2` primarily deals with linear convolution.
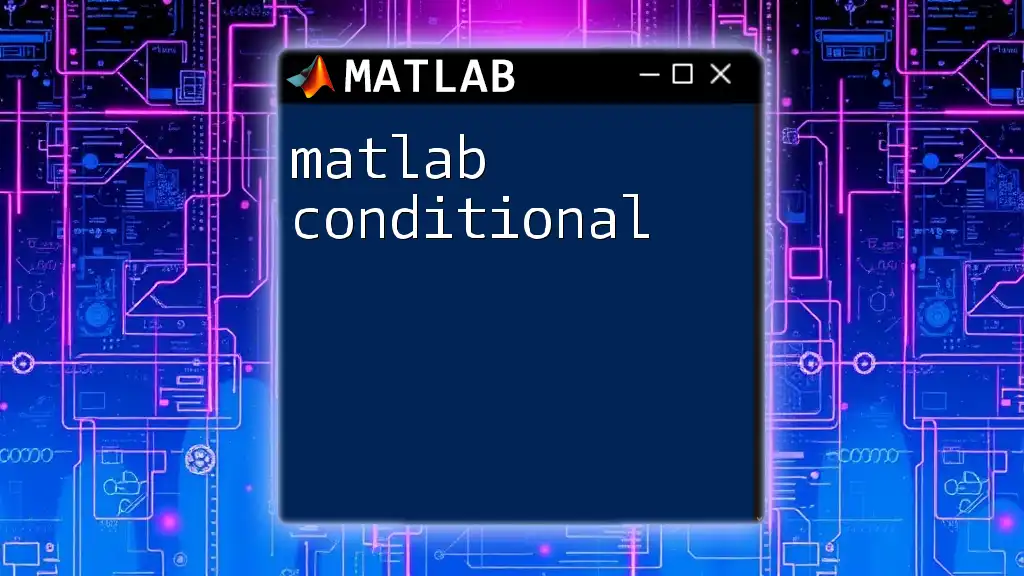
The Syntax of conv2
Basic Syntax
The basic syntax of the `conv2` function is as follows:
C = conv2(A, B)
Here, `C` is the resulting matrix obtained after convolution.
- `A`: This is the input matrix (e.g., an image or a 2D signal).
- `B`: This refers to the convolution kernel (or filter) that you apply.
Output Explanation
The output variable `C` represents the convolved result. The dimensions of `C` can vary based on the options specified (like `'full'`, `'same'`, or `'valid'`) when using `conv2`. Understanding the output size is crucial for accurate processing.
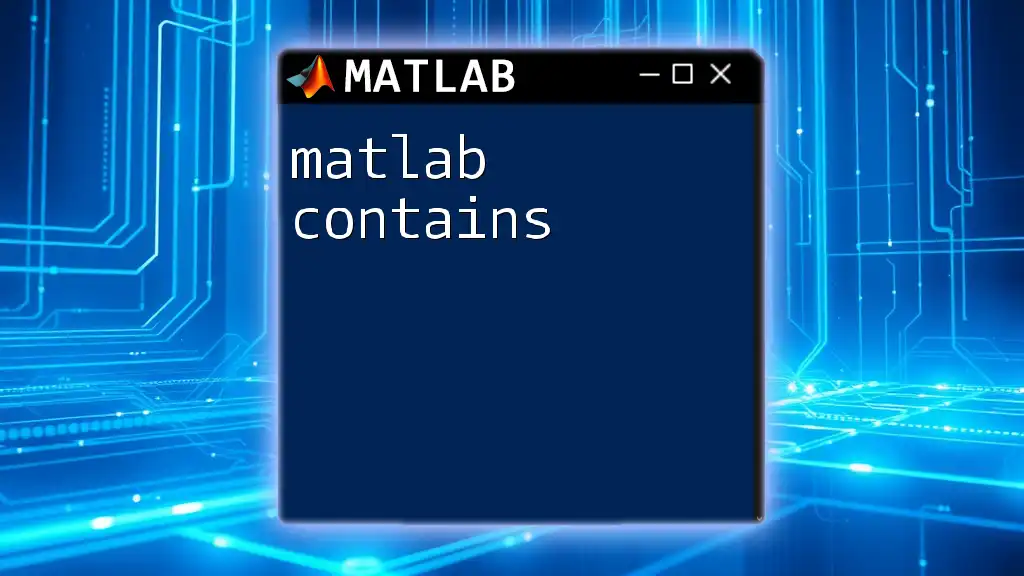
Practical Applications of conv2
Image Filtering
One of the main applications of `conv2` is image filtering. Libraries and functions in image processing that help in adjusting image characteristics use convolution as the backbone.
Example on Image Filtering: Applying a Gaussian Filter
To apply a Gaussian filter for smoothing an image:
A = imread('image.jpg');
B = fspecial('gaussian', [5 5], 2);
C = conv2(A, B, 'same');
In this example:
- `fspecial('gaussian', [5 5], 2)` generates a Gaussian filter of size 5x5 with a standard deviation of 2.
- The option `'same'` returns an output matrix `C` that is the same size as the input `A`.
Edge Detection
Another important application of `conv2` is in edge detection, which highlights transitions in intensity, allowing for the extraction of edges from images.
Example: Using Sobel Filter for Edge Detection
B = fspecial('sobel');
C = conv2(double(rgb2gray(A)), B, 'same');
This example demonstrates how:
- The Sobel filter, available via `fspecial`, is applied to the grayscale representation of an image for edge detection.
Transition Effects in Signal Processing
Convolution is also used for analyzing signals, especially in understanding how systems respond to inputs.
Example: Convolution of a Signal
t = 0:0.01:1;
x = sin(2*pi*5*t);
h = [1 2 1]/4;
y = conv2(x, h, 'same');
In this scenario:
- A sine wave is created and convolution is performed with a smoothing filter to reduce noise in the signal.
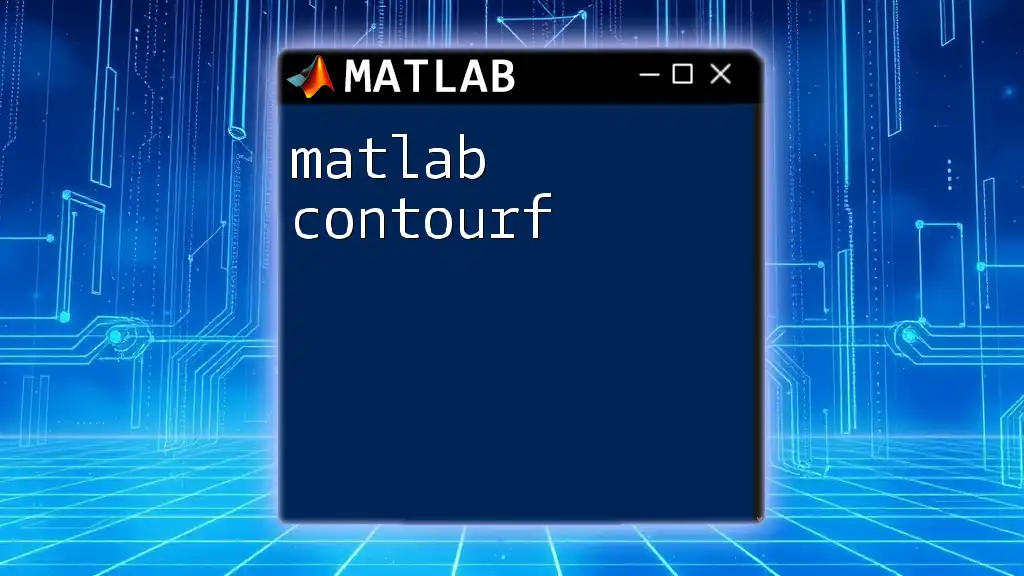
Advanced Usage of conv2
Handling Different Modes
`conv2` allows for three different modes of convolution that affect the output size:
- `'full'`: Returns the full convolution result, which is larger than both input matrices.
- `'same'`: Returns the central part of the convolution output that is the same size as the first input.
- `'valid'`: Returns only those parts of the convolution that produce valid outputs, essentially reducing the output size.
Custom Kernels
You can also create custom kernels to suit specific filtering needs.
Example: Custom Filter for Sharpening Images
kernel = [0 -1 0; -1 5 -1; 0 -1 0];
sharpened_image = conv2(A, kernel, 'same');
In this example, a sharpening filter emphasizes the edges and increases the definition of the image.
Performance Optimization
To optimize performance when dealing with larger matrices and convolution operations, consider:
- Using `imfilter` as an alternative to `conv2`. It can perform similar tasks, often with better speed for image processing applications.
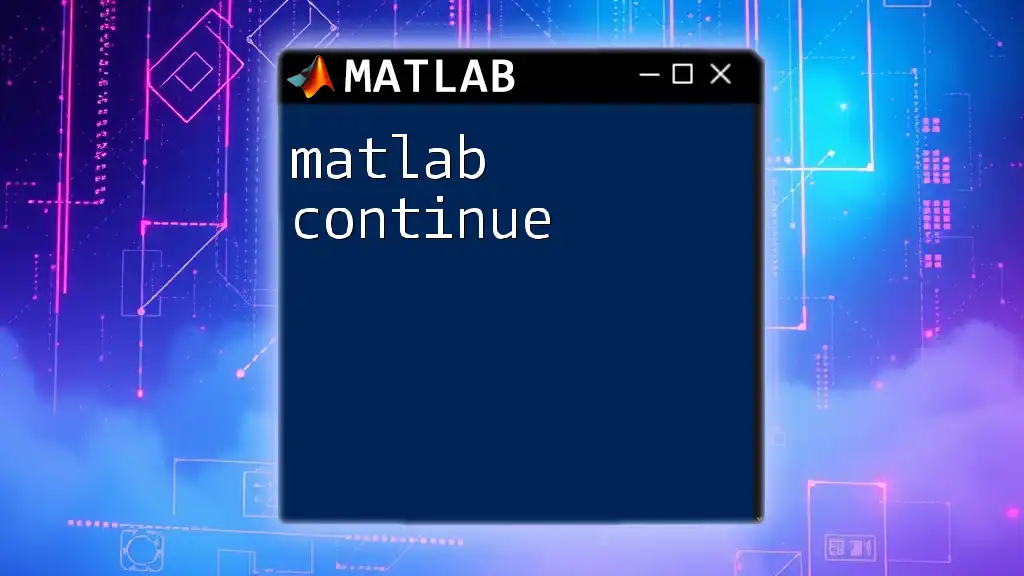
Common Pitfalls and Troubleshooting
Dimension Mismatch
It’s easy to run into dimension mismatch errors, especially if the kernel and input matrix sizes don’t align.
Example: Adjusting Matrix Sizes Ensure that matrix dimensions are compatible when performing operations. If necessary, you can pad your matrices or resize appropriately for successful convolution.
Understanding Padding
Padding can significantly influence the output of convolution operations. The choice of mode in `conv2` determines how boundaries are handled, which is vital for maintaining image properties.
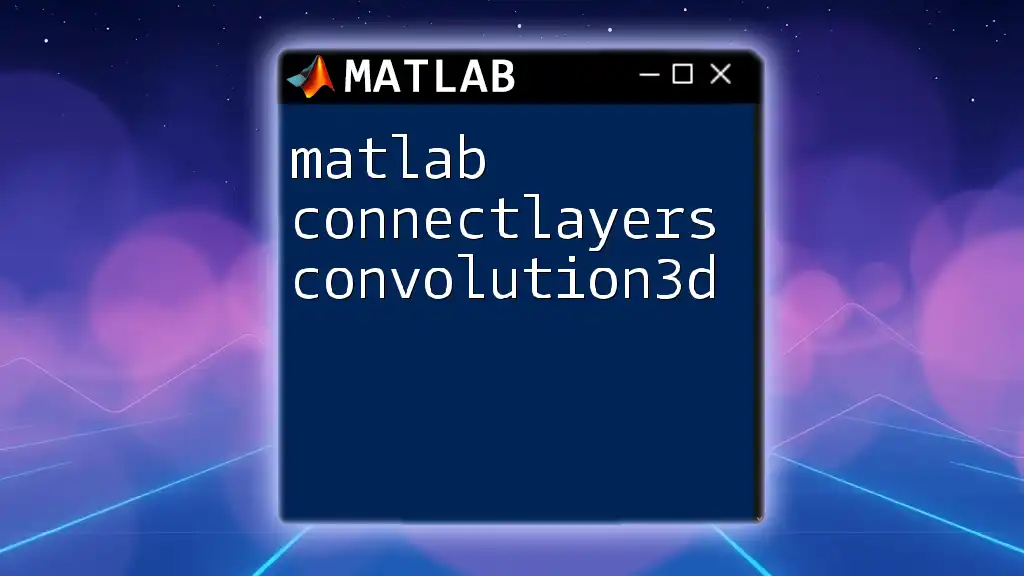
Conclusion
In summary, the `matlab conv2` function is an essential tool for conducting 2D convolution. It has extensive applications in fields like image processing, where it serves for filtering and edge detection. Mastering `conv2` allows users to harness the power of convolution to carry out sophisticated analyses in both images and signals. For further learning, exploring online resources and tutorials on MATLAB will enhance your command over this potent tool. Don’t hesitate to experiment with the examples provided and share your experiences as you delve into the world of convolution!