In MATLAB, the `writetable` function allows you to easily export a table to a file, such as a CSV, making it convenient to share data.
Here’s a simple example:
% Create a sample table
T = table([1; 2; 3], {'A'; 'B'; 'C'}, 'VariableNames', {'Numbers', 'Letters'});
% Write the table to a CSV file
writetable(T, 'myTable.csv');
Understanding MATLAB Tables
What is a Table in MATLAB?
In MATLAB, a table is a data type designed for storing column-oriented or mixed-type data. Tables can contain different variable types, such as numeric, character, or cell arrays. This versatility makes them ideal for representing datasets, especially when working with data that has different properties.
Using tables over other data types like matrices or cell arrays has several advantages:
- Easier Data Management: Each column can have a different data type, allowing you to manage and manipulate heterogeneous data more intuitively.
- Descriptive Variable Names: You can label the columns with meaningful names, which enhances readability and interpretability.
- Automatic Handling of Row Names: Tables can effectively manage row identifiers, enhancing the organization of your data.
Creating a Table
Creating a table in MATLAB is straightforward. You can create a table from a variety of sources such as arrays, cell arrays, or even structures. Here’s how you can create a simple table containing names, ages, and scores:
% Sample data
Names = {'Alice'; 'Bob'; 'Charlie'};
Ages = [23; 34; 29];
Scores = [85.5; 91.0; 78.0];
% Creating a table
T = table(Names, Ages, Scores);
In this example, the variables `Names`, `Ages`, and `Scores` contain the data we want to organize. The function `table()` generates a table called `T` with three columns corresponding to the input variables.
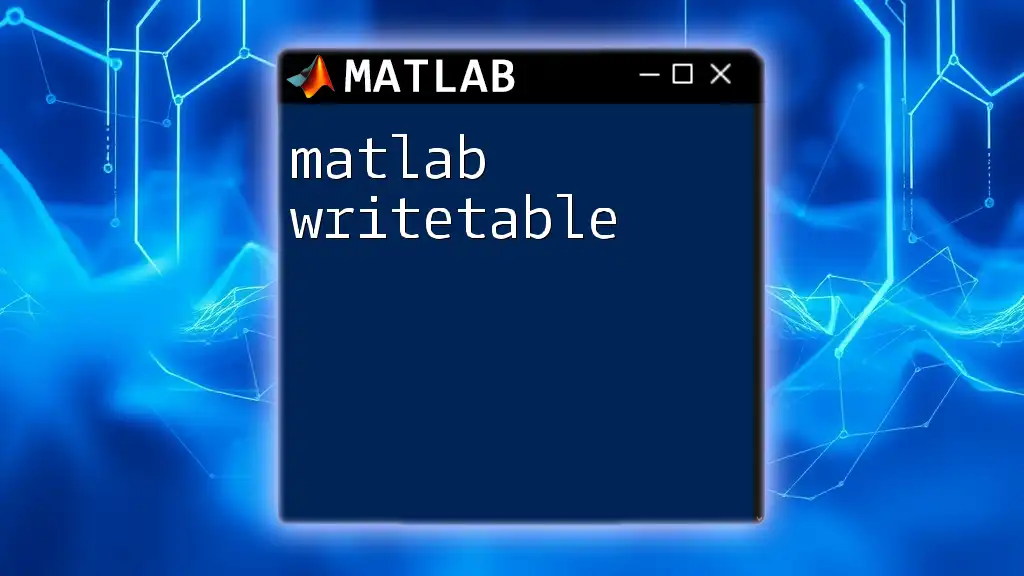
Overview of the `writetable` Function
What is `writetable`?
The `writetable` function is a powerful tool in MATLAB that allows you to write a table to a external file, such as a CSV or Excel file. This utility is indispensable when you need to export your processed data for reporting or sharing purposes.
Basic Syntax
The basic syntax for `writetable` is straightforward. You begin by specifying the table variable and the filename to which you want to write the table:
writetable(T, 'output.csv');
In this example, the table `T` will be saved as a CSV file named `output.csv` in the current working directory.
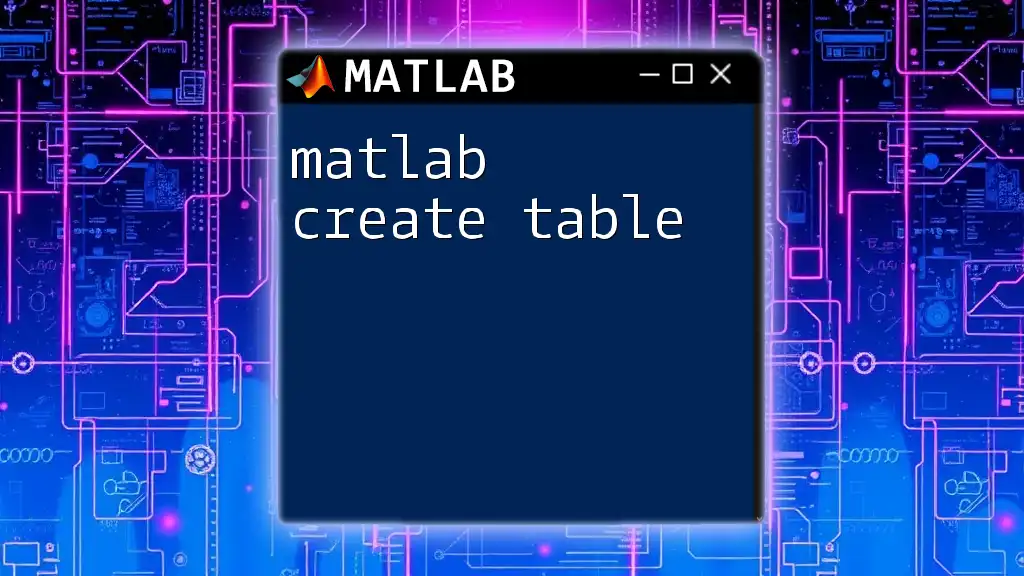
Writing Tables to Different Formats
Writing to CSV Files
Writing tables to CSV files is commonly performed for data exports, especially since CSV files are easily readable by countless software applications. Here’s how you can do it:
writetable(T, 'output.csv');
This command saves the contents of `T` to a file named `output.csv`. The default behavior includes variable names as the first row in the CSV file and uses commas as delimiters. It's efficient and effective for a variety of applications.
Writing to Excel Files
MATLAB also provides the ability to write tables to Excel files. This is particularly useful for users who require formatted spreadsheets or easy data manipulation in Excel. To save the table `T` as an Excel file, you can use the following command:
writetable(T, 'output.xlsx');
Exporting to `.xlsx` format not only maintains the structure of the table but also supports advanced options like formatting and formulas, which can be beneficial for further data analysis in Excel.
Writing to Text Files
If a plain text format is more suited for your needs, MATLAB allows you to write tables to text files as well. You can specify a delimiter such as tab or space to format the output. Here is an example:
writetable(T, 'output.txt', 'Delimiter', '\t');
In this scenario, the table will be saved as a tab-separated values file. The `Delimiter` parameter specifies how the columns are separated in the output file.
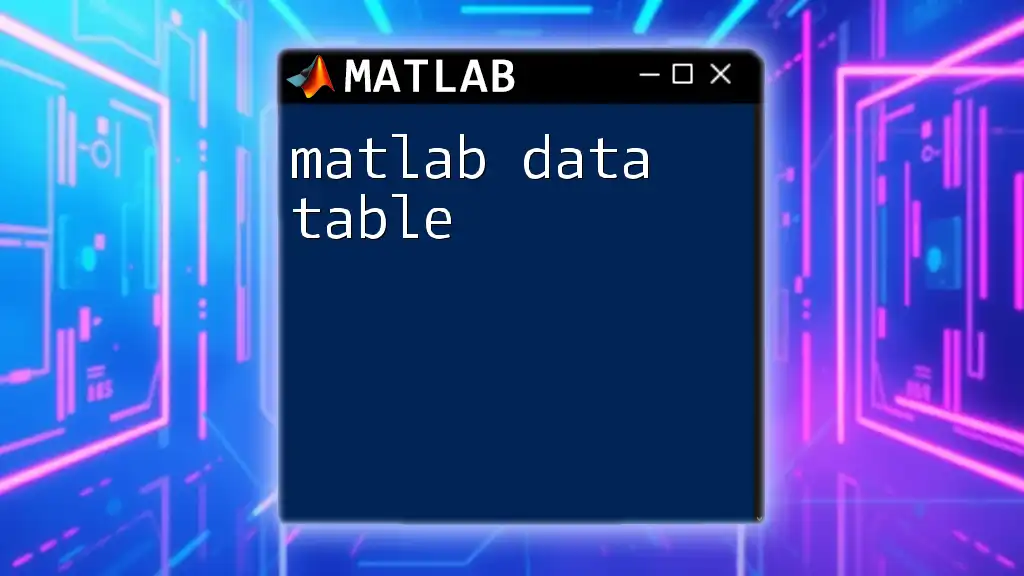
Customizing the Output
Specifying Variable Names
One of the strengths of MATLAB tables is the ability to customize column names before writing them to a file. Changing variable names can make your data more interpretable for end-users. Here's how:
T.Properties.VariableNames = {'Name', 'Age', 'Score'};
writetable(T, 'output.csv');
In this example, the variable names in the table `T` have been changed to more descriptive labels. Upon exporting, these new names will replace the default variable names in the CSV file.
Behavior with Row Names
You can also control whether row names are included in your output file. By default, row names are not written to the file, but you can opt to include them as follows:
writetable(T, 'output.csv', 'WriteRowNames', true);
This command configures `writetable` to include row names in the written CSV file, which can be useful when row identifiers carry specific meanings within your dataset.
Specifying Data Formats
When exporting tables, sometimes you may want to control how specific variables are formatted, particularly numeric data. You can achieve this with the `NumberStyle` parameter. For example:
writetable(T, 'output.csv', 'NumberStyle', '%.2f');
In this case, all numerical values in the table will be formatted to two decimal places, ensuring consistency and clarity in how numbers are represented in the file.
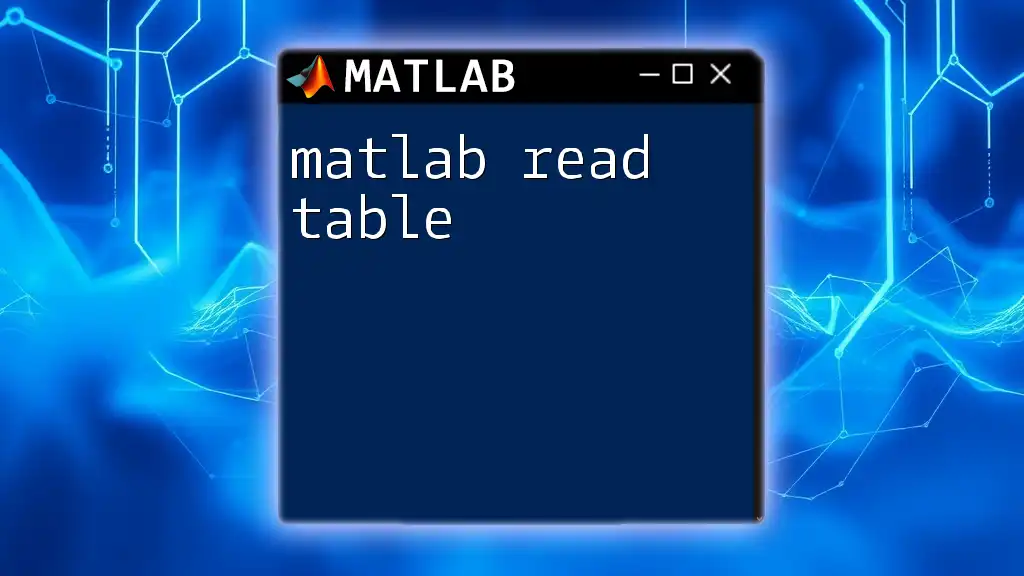
Handling Large Tables
Performance Considerations
When working with large datasets, performance becomes crucial. Here are some tips for optimizing writing times:
- Chunking the Table: Write the data in smaller chunks instead of trying to process the entire dataset at once, especially when dealing with large files.
- Adjusting Memory Settings: Ensure MATLAB has sufficient memory available to handle large tables effectively.
One method to manage chunking is to loop over segments of the table and write them iteratively. This is particularly useful when memory limits might be exceeded.
Troubleshooting Common Errors
Despite its user-friendliness, you may encounter common issues when using `writetable`. Here are some typical pitfalls and their solutions:
- File Already Open: If you receive an error stating the file cannot be opened, it could be because the file is currently open in another program. Make sure to close it before attempting to write.
- Invalid File Formats: Ensure that the filename extension matches the file type you intend to write. For example, using `.csv` with a command that specifies Excel features may lead to errors.
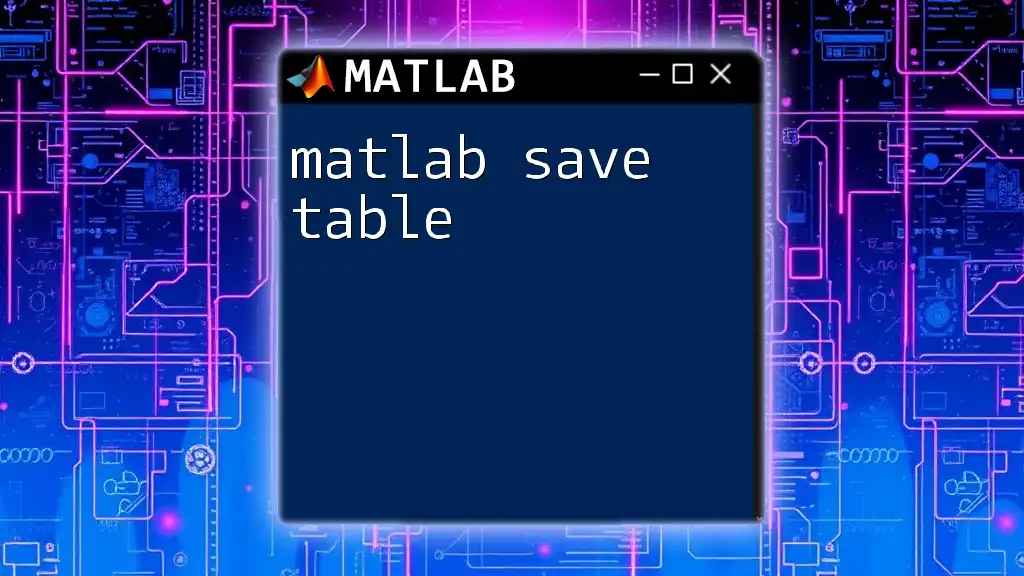
Best Practices
Organizing Your Code
Organizing your MATLAB code is crucial for enhancing readability and maintainability. Use clear variable names and include comments to explain complex sections of your code. For instance:
% Creating a table of student data
Names = {'Alice'; 'Bob'; 'Charlie'};
Ages = [23; 34; 29];
Scores = [85.5; 91.0; 78.0];
T = table(Names, Ages, Scores);
By commenting your code, future users (or even yourself) can more easily understand the logic and purpose of your code blocks.
Version Control and Output Management
Version control is essential when working with output files. Establish a consistent naming convention, like including timestamps or version numbers in your file names. For example, use:
writetable(T, ['output_' datestr(now, 'yyyy-mm-dd_HH-MM-SS') '.csv']);
This adds a timestamp to your filename, helping you keep track of different file versions and preventing overwriting important data.
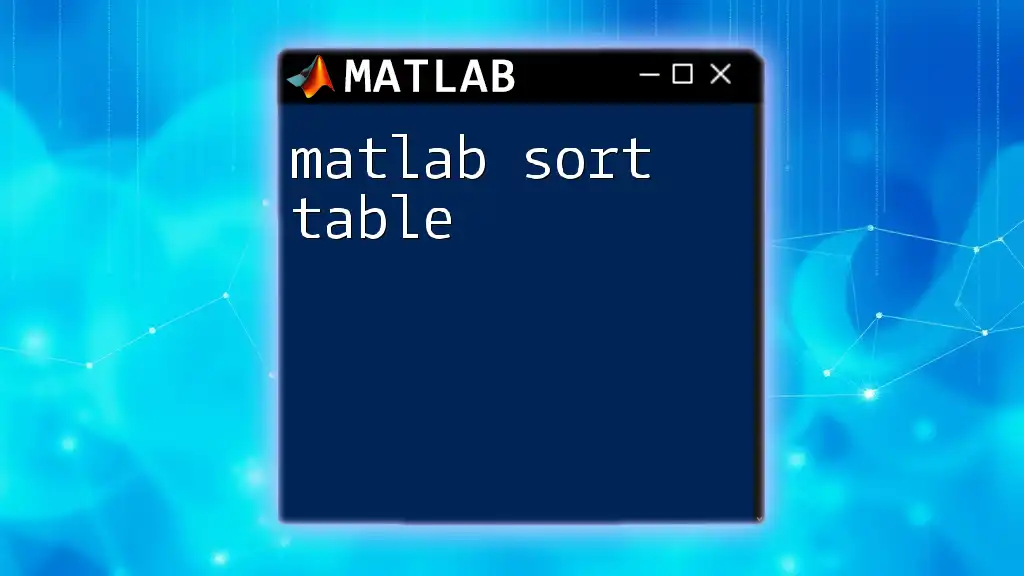
Conclusion
In this guide, we've covered everything you need to know about using the `matlab write table` functionality. From understanding tables to exporting them in various formats, customizing output, and handling large datasets, you now have a comprehensive toolkit for effective data management in MATLAB.
We encourage you to put these commands into practice and share your experiences or any questions you might have in the comments below. Happy coding!
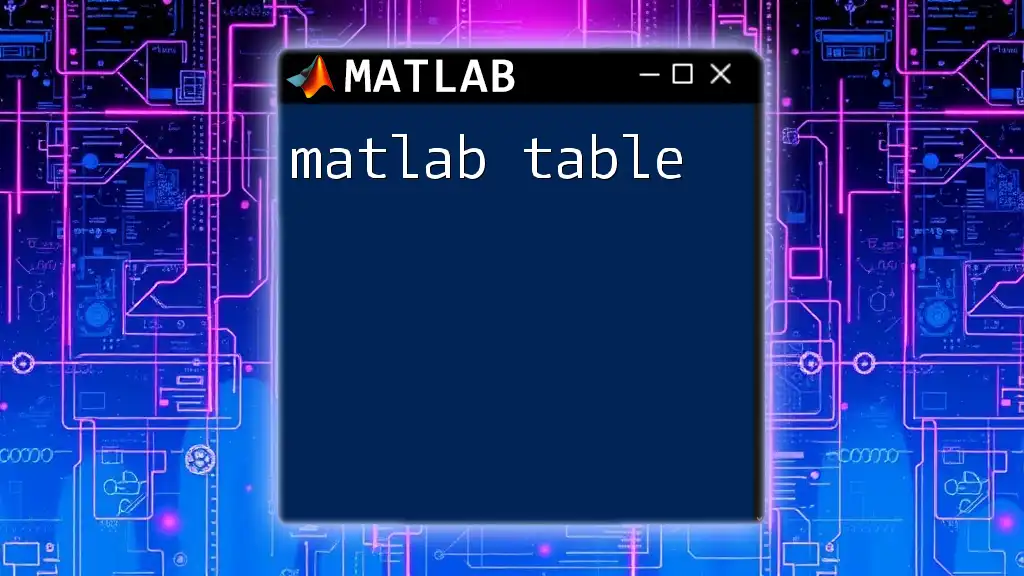
Additional Resources
For further learning, consider accessing MATLAB’s official documentation on tables and the `writetable` function. Additionally, you may find tutorials and textbooks covering MATLAB data handling particularly helpful as you deepen your understanding and skills.
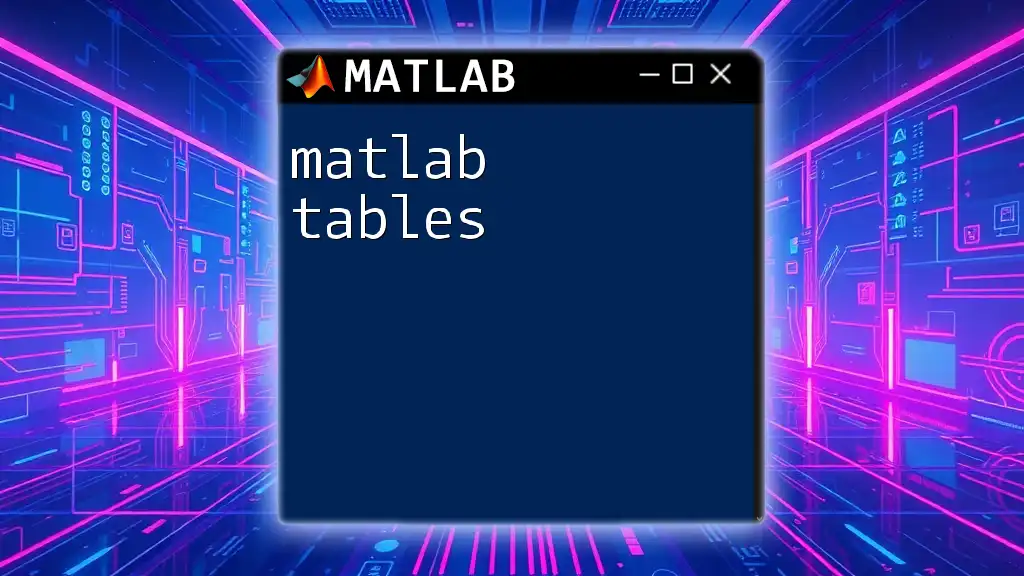
Frequently Asked Questions
You might find answers to common inquiries regarding the `writetable` function and its applications within tables in MATLAB. If you have more specific questions, feel free to ask for clarification or additional details!