The MATLAB cross product is used to compute the vector that is perpendicular to two input vectors in three-dimensional space, and it can be easily performed using the `cross` function.
A = [1, 2, 3];
B = [4, 5, 6];
C = cross(A, B);
Understanding the Cross Product
Definition and Formula
The cross product is a mathematical operation that takes two vectors in three-dimensional space and produces a third vector that is orthogonal (perpendicular) to both input vectors. The formula for the cross product is:
\[ \mathbf{a} \times \mathbf{b} = |\mathbf{a}||\mathbf{b}|\sin(\theta)\mathbf{n} \]
Where:
- \(\mathbf{a}\) and \(\mathbf{b}\) are the input vectors.
- \(\theta\) is the angle between the two vectors.
- \(|\mathbf{a}|\) and \(|\mathbf{b}|\) refer to the magnitudes of the vectors.
- \(\mathbf{n}\) is the unit vector in the direction of the resultant vector.
Geometric Interpretation
The resulting vector from the cross product has a magnitude equal to the area of the parallelogram that the two vectors span. The right-hand rule can be used to determine the direction of the cross product vector: if you point the fingers of your right hand in the direction of the first vector and curl them towards the second vector, your thumb will point in the direction of the resultant vector.
Properties of the Cross Product
Understanding the properties of the cross product is essential for correctly applying it in various contexts. Key properties include:
-
Anti-commutativity: The cross product is anti-commutative, meaning that switching the order of the vectors reverses the direction of the resultant vector: \[ \mathbf{a} \times \mathbf{b} = -(\mathbf{b} \times \mathbf{a}) \]
-
Distributive Property: The cross product is distributive over vector addition: \[ \mathbf{a} \times (\mathbf{b} + \mathbf{c}) = \mathbf{a} \times \mathbf{b} + \mathbf{a} \times \mathbf{c} \]
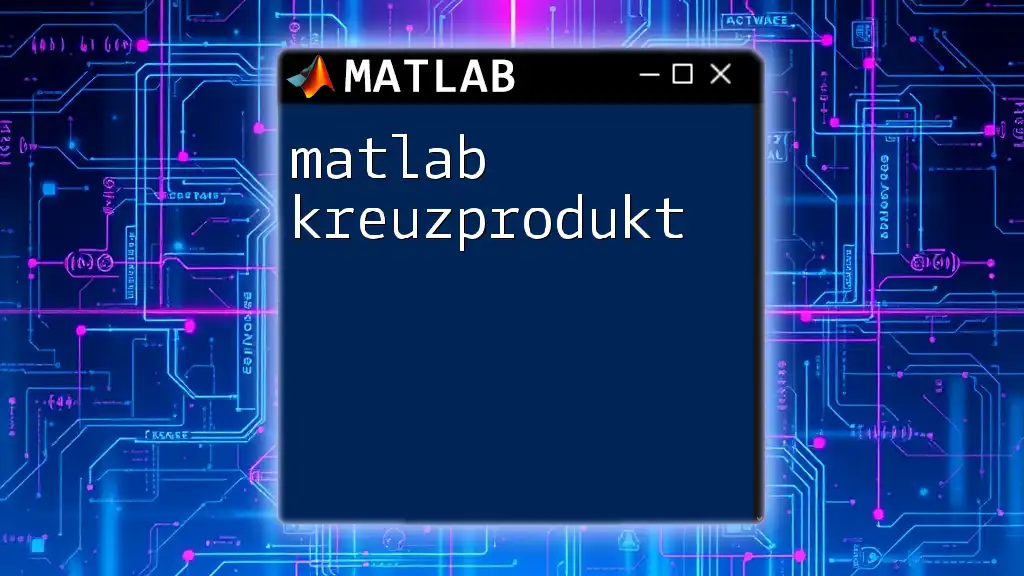
How to Compute the Cross Product in MATLAB
Using Built-in Functions
MATLAB simplifies the computation of the cross product through its built-in `cross()` function. The basic syntax for using this function is straightforward and intuitive. Here is a simple example:
a = [1, 2, 3];
b = [4, 5, 6];
result = cross(a, b);
disp(result); % Output will be [-3, 6, -3]
In this code snippet, we define two vectors `a` and `b` and then compute their cross product, storing the result in the variable `result`.
Cross Product of Two Vectors
To calculate the cross product of two vectors step-by-step, first create two vectors in MATLAB, then use the `cross()` function to perform the calculation. Here's how you do it:
vector1 = [2, 3, 4];
vector2 = [5, 6, 7];
crossProduct = cross(vector1, vector2);
disp(crossProduct); % Displays the resulting vector
This operation yields a vector that is orthogonal to both `vector1` and `vector2`.
Cross Product for Multiple Vectors
MATLAB also allows for the computation of cross products involving multiple vectors using matrices. This can enhance performance significantly. For example:
A = [1 2 3; 4 5 6; 7 8 9]; % 3x3 matrix representing 3 vectors
B = [9 8 7; 6 5 4; 3 2 1];
C = cross(A, B); % Cross product of each row vector in A and B
disp(C); % Display the result
In this case, the `cross()` function computes the cross product for the corresponding rows of the two matrices `A` and `B`.
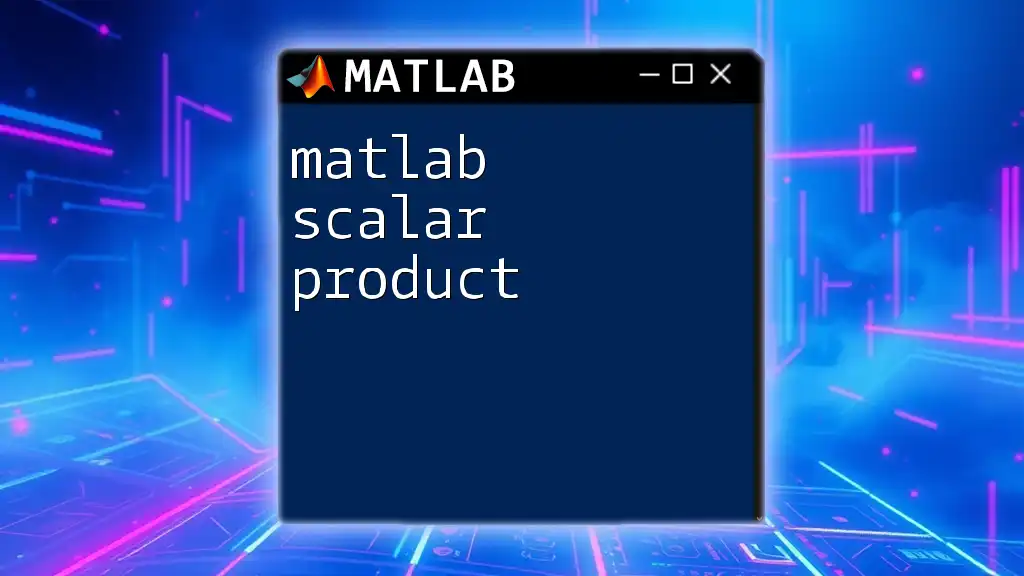
Practical Applications of Cross Product in MATLAB
Physics: Torque Calculation
In physics, the cross product is crucial for calculating torque, which is defined as the rotational equivalent of linear force. The formula for torque is given by:
\[ \mathbf{\tau} = \mathbf{r} \times \mathbf{F} \]
Where:
- \(\tau\) is the torque vector.
- \(\mathbf{r}\) is the distance vector from the pivot point to the point of force application.
- \(\mathbf{F}\) is the applied force vector.
Here's how you can calculate torque in MATLAB:
force = [10, 0, 0]; % Force in Newtons
distanceVector = [0, 5, 0]; % Distance in meters
torque = cross(distanceVector, force);
disp(torque); % Producing the torque vector
This command will yield the torque vector resulting from the specified force applied at a distance.
Engineering: Finding Normal Vectors
In engineering, calculating normal vectors to surfaces is essential, particularly in 3D modeling and computer graphics. The normal vector is perpendicular to the plane defined by points in 3D space. You can find a normal vector using the cross product of two non-parallel vectors that lie in the plane.
For example, consider two vectors:
p1 = [1, 0, 0];
p2 = [0, 1, 0];
normalVector = cross(p1, p2); % Find normal using cross product
disp(normalVector); % Resulting normal vector
The output will represent the normal vector to the plane defined by `p1` and `p2`.
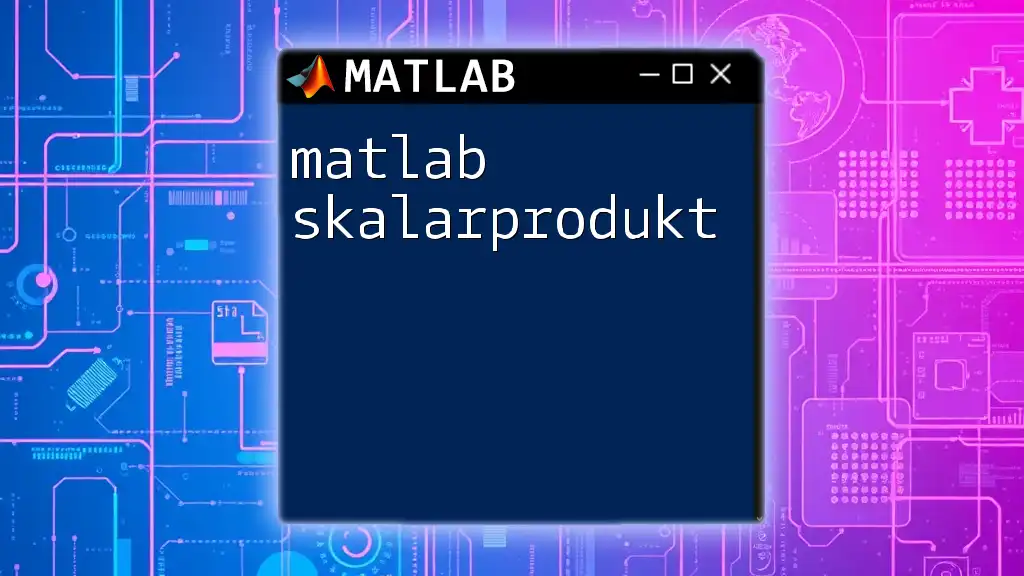
Visualizing the Cross Product
3D Vector Plots in MATLAB
MATLAB provides visualization tools to better understand the relationships between vectors and their cross products. One such function is `quiver3`, which can be used to plot vectors in a 3D space, effectively illustrating the result of a cross product.
Here's an example of how to visualize the cross product:
figure;
quiver3(0, 0, 0, a(1), a(2), a(3); % Plotting vector a
hold on;
quiver3(0, 0, 0, b(1), b(2), b(3); % Plotting vector b
quiver3(0, 0, 0, result(1), result(2), result(3); % Plotting the cross product
axis equal;
grid on;
title('Visualization of Cross Product');
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
This code creates a 3D plot showing the vectors and their cross product, enhancing the understanding of their geometric relationships.
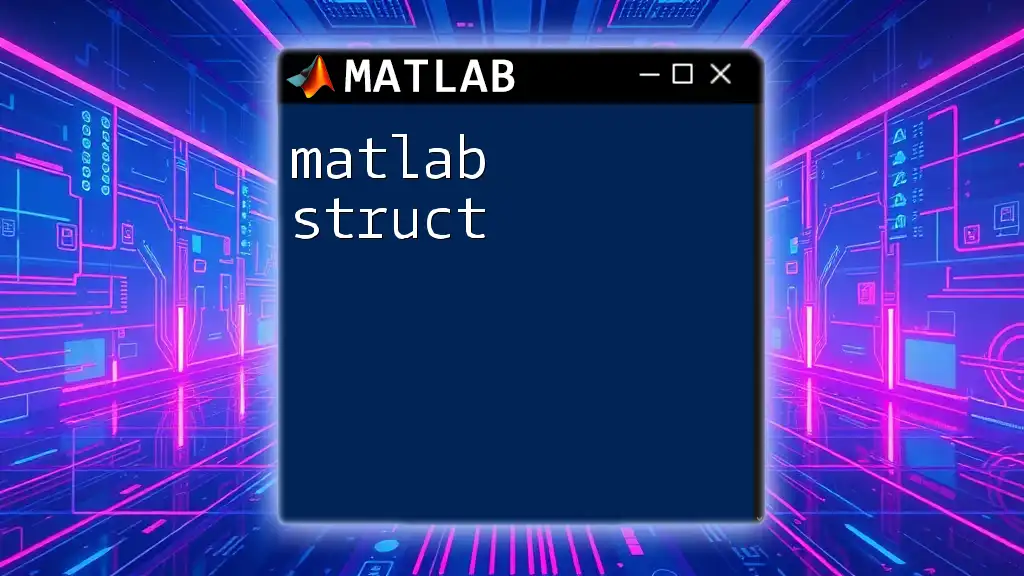
Common Errors and Troubleshooting
Common Mistakes When Using Cross Product
While working with the `matlab cross product`, users often encounter common pitfalls. One of the most prevalent issues is dimension mismatch. The vectors used in a cross product should always be in a specific format, typically 1x3 arrays for three-dimensional vectors. Failing to follow this can lead to errors that disrupt your computations.
Another frequent error is using the wrong functions. To ensure correct usage, be certain that you're calling the `cross()` function when you intend to calculate the cross product.
Debugging Tips
When debugging, always ensure that your vector dimensions are appropriate for the cross product operation. You can use the `isvector()` function to confirm that your inputs are valid vectors. For example:
if isvector(a) && isvector(b)
result = cross(a, b);
else
disp('One or both inputs are not vectors.');
end
This simple check can help minimize common errors and improve the robustness of your code.
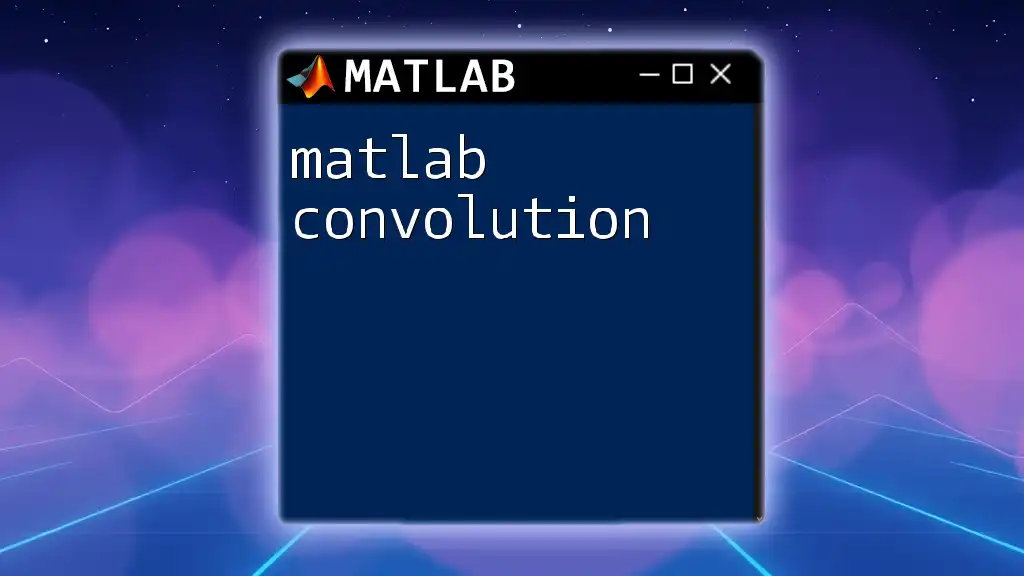
Conclusion
In summary, the MATLAB cross product is a powerful tool for performing vector calculations in three-dimensional space. Understanding its definition and properties, coupled with practical examples and applications, can significantly enhance your capabilities in MATLAB. Whether calculating torque in physics or finding normal vectors in engineering, mastering the cross product is essential for anyone working with vectors in technical fields.
Additional Resources
To further your grasp of the cross product and MATLAB commands, consider exploring additional reading materials, video tutorials, and the official MATLAB documentation. Engaging with these resources will deepen your knowledge and proficiency, enabling you to employ MATLAB effectively in various applications.