In MATLAB, you can obtain the diagonal elements of a matrix using the `diag` function, which extracts the main diagonal or creates a diagonal matrix from a vector.
% Extracting the diagonal of a matrix
A = [1 2 3; 4 5 6; 7 8 9];
d = diag(A); % This will return the vector [1; 5; 9]
Understanding Matrices in MATLAB
What is a Matrix?
A matrix is a rectangular array of numbers arranged in rows and columns. It is a fundamental structure in linear algebra, widely used in various fields, including mathematics, physics, engineering, and computer science. Matrices can be classified into several types, such as:
- Row matrices: A matrix that contains only one row.
- Column matrices: A matrix that contains only one column.
- Square matrices: A matrix that has the same number of rows and columns.
How MATLAB Handles Matrices
MATLAB is specifically designed for matrix manipulation. You can create matrices using built-in functions like `zeros`, `ones`, and `rand`. For example:
A = zeros(3, 3); % Creates a 3x3 matrix of zeros
B = ones(2, 4); % Creates a 2x4 matrix of ones
C = rand(2, 2); % Creates a 2x2 matrix with random values
By understanding how to create and manipulate matrices, you're well on your way to mastering the MATLAB diagonal of matrix operations.
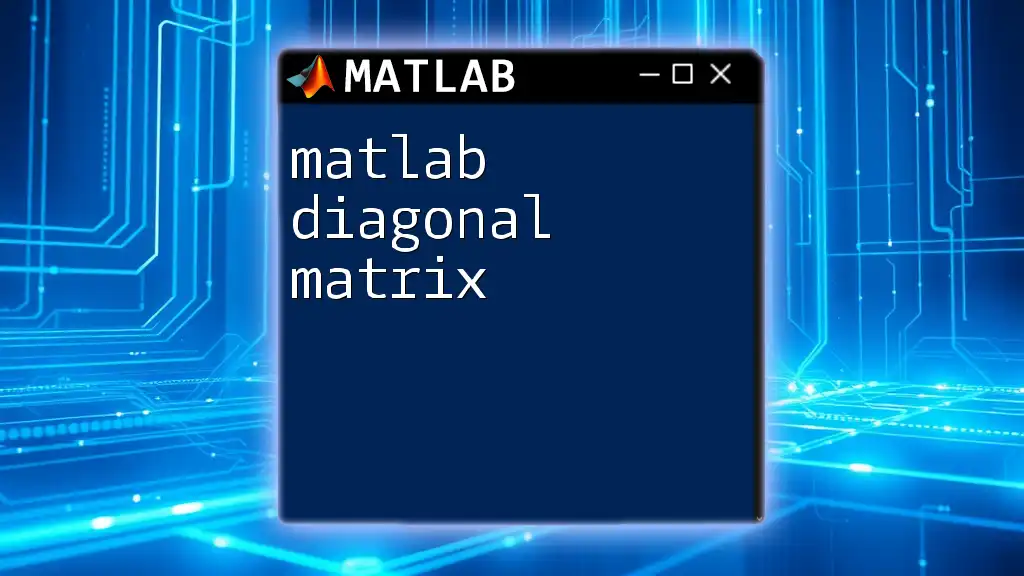
Diagonal Elements of a Matrix
What is a Diagonal?
The diagonal of a matrix refers to a set of elements that extend from one corner of the matrix to the opposite corner. In the case of a square matrix, these are known as the main diagonal and the secondary diagonal.
Extracting the Main Diagonal
The main diagonal consists of elements that span from the top left to the bottom right of a square matrix. To extract these elements in MATLAB, you can use the `diag` function. Here is an example:
A = [1 2 3; 4 5 6; 7 8 9];
mainDiagonal = diag(A);
disp(mainDiagonal); % Output: [1; 5; 9]
In this example, `mainDiagonal` retrieves the values `1`, `5`, and `9`. These elements are particularly important in various mathematical applications, including solving systems of linear equations and computing determinants.
Extracting the Secondary Diagonal
The secondary diagonal runs from the top right to the bottom left of a matrix. To extract this diagonal, you can use MATLAB's indexing capabilities. Here is how it can be done:
A = [1 2 3; 4 5 6; 7 8 9];
secondaryDiagonal = A(fliplr(1:size(A,1)), :);
disp(secondaryDiagonal); % Output: [3; 5; 7]
In this code slice, `fliplr` is used to reverse the order of the columns, allowing you to access the secondary diagonal. Understanding how to work with both main and secondary diagonals opens up a range of applications in matrix analysis.
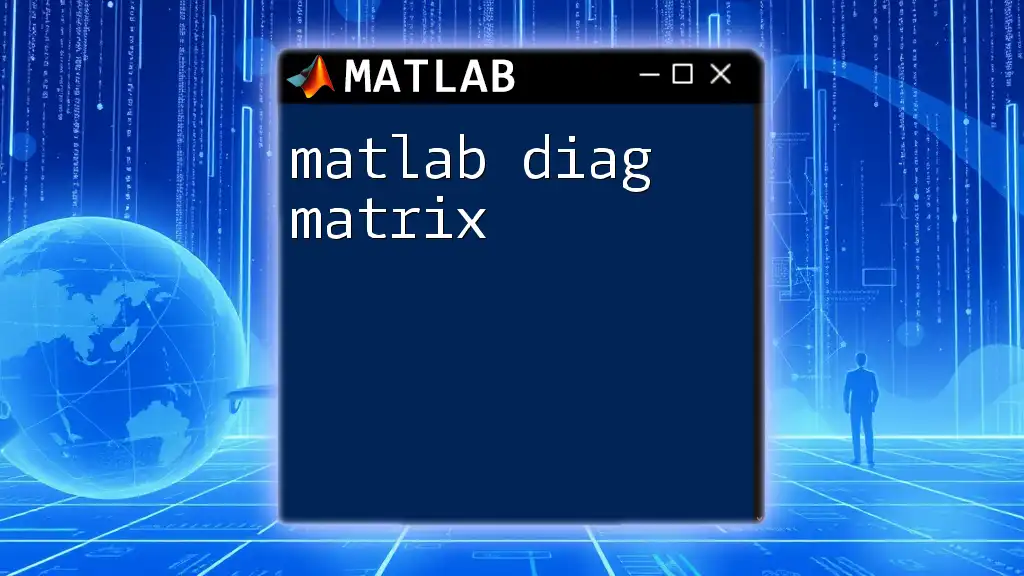
Creating Diagonal Matrices
Using the diag Function
MATLAB makes it easy to create diagonal matrices using the `diag` function. When you provide a vector, MATLAB will return a square matrix with that vector's elements on the main diagonal, and all off-diagonal elements will be zero. Here’s an example:
d = [1, 2, 3];
D = diag(d);
disp(D); % Output: [1 0 0; 0 2 0; 0 0 3]
The matrix `D` in this case is a diagonal matrix where `1`, `2`, and `3` occupy the main diagonal positions, demonstrating how easily diagonals can be constructed in MATLAB.
Modifying Existing Diagonal Elements
You can also modify the diagonal elements of a matrix directly. To do this, simply access the elements using their indices. For example:
A = [1 2 3; 4 5 6; 7 8 9];
A(1,1) = 10; % Modifying the first diagonal element
disp(A); % Output: [10 2 3; 4 5 6; 7 8 9]
This change indicates how flexible MATLAB is in terms of altering matrix structure, which can be quite powerful in computational tasks.
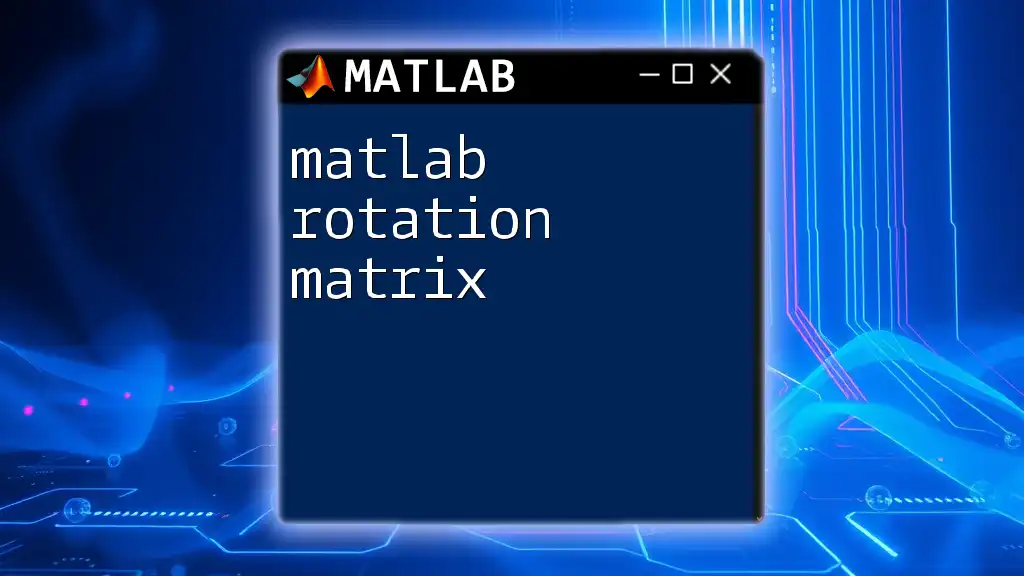
Visualizing the Diagonal of a Matrix
Using Heatmaps for Better Understanding
Visual representation can enhance comprehension, particularly when analyzing matrix elements. Using MATLAB's `heatmap` function, you can effectively visualize a matrix and highlight its diagonal elements. Here's a quick example:
heatmap(A);
title('Matrix Heatmap');
Through heatmaps, patterns in diagonal elements can become more apparent, aiding in deeper analysis and understanding.
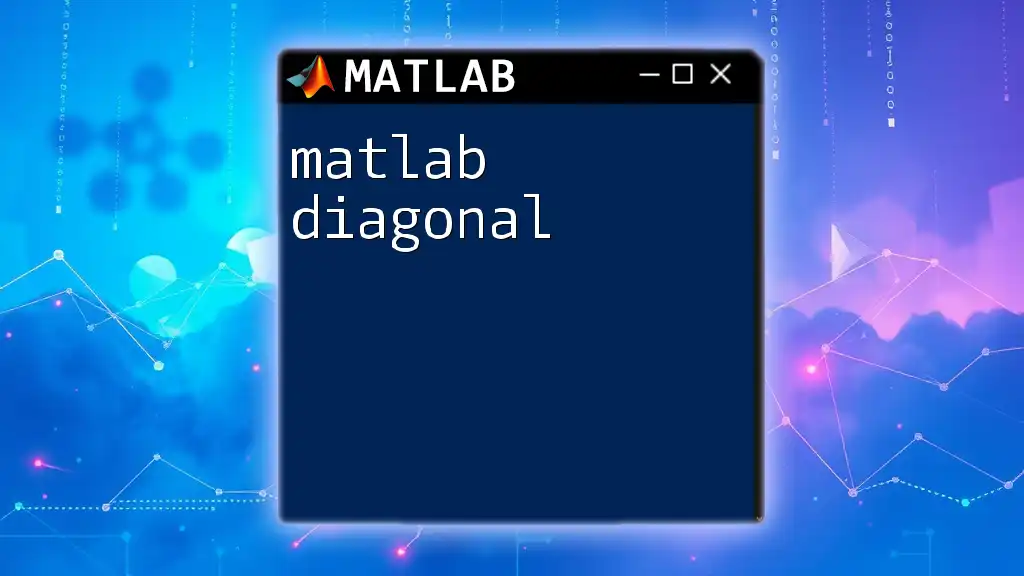
Practical Applications of Matrix Diagonals
In Linear Algebra
Matrix diagonals play a crucial role in linear algebra, particularly in solving linear equations. Diagonal matrices have unique properties that simplify complex matrix calculations. Additionally, the concepts of eigenvalues and eigenvectors often relate directly to the diagonal elements of matrix transformations.
In Data Science and Machine Learning
In fields like data science and machine learning, diagonal matrices also have important applications. For instance, creating a diagonal matrix during data normalization can help ensure that feature scales are maintained. Moreover, Principal Component Analysis (PCA) heavily relies on diagonalization, helping reduce dimensionality while preserving variance in the data.
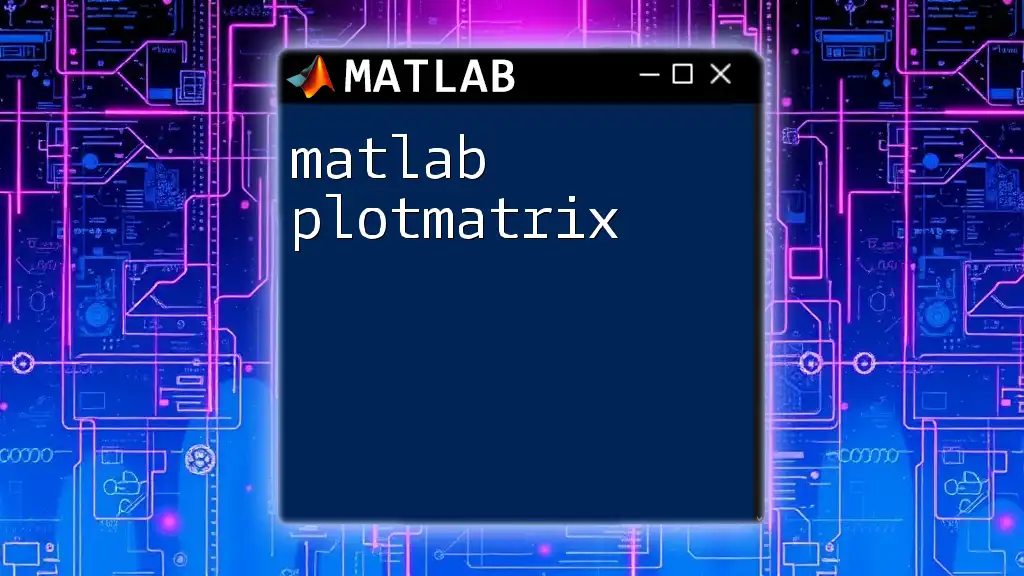
Common Mistakes to Avoid
Misunderstanding Indexing
One common pitfall when working with matrices in MATLAB is misusing indices. It's essential to always verify the dimensions of your matrix to avoid errors in accessing diagonal elements.
Ignoring Non-Square Matrices
Another frequent error occurs when working with non-square matrices. The concept of diagonals typically applies to square matrices, so understanding the context is vital.
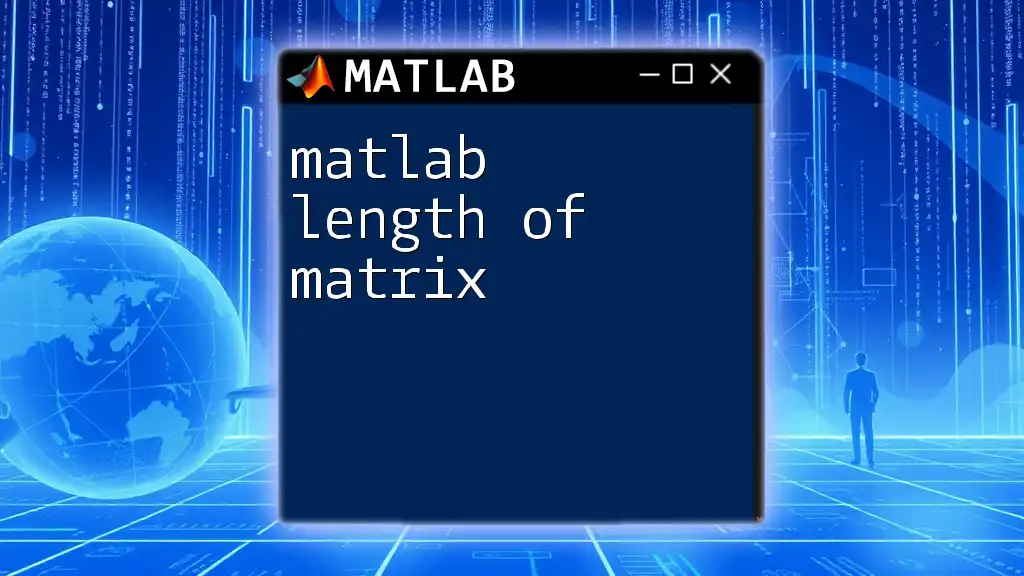
Conclusion
In summary, the MATLAB diagonal of matrix functionality is an essential aspect of matrix manipulation, offering various methods to extract and work with both main and secondary diagonal elements. Understanding how to create diagonal matrices and modify diagonal elements can significantly enhance your capabilities in MATLAB. We encourage you to practice these functions and explore additional MATLAB tutorials to deepen your knowledge.
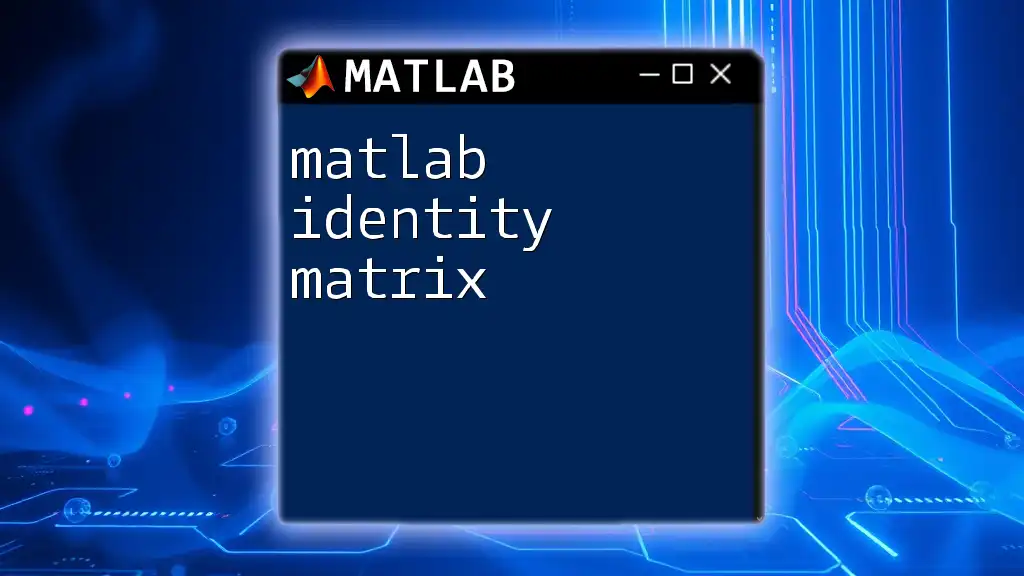
Additional Resources
For further learning, refer to the official MATLAB documentation and recommended books on linear algebra and matrix manipulation. Engaging with these resources will help round out your understanding of matrix diagonals and their applications in MATLAB.
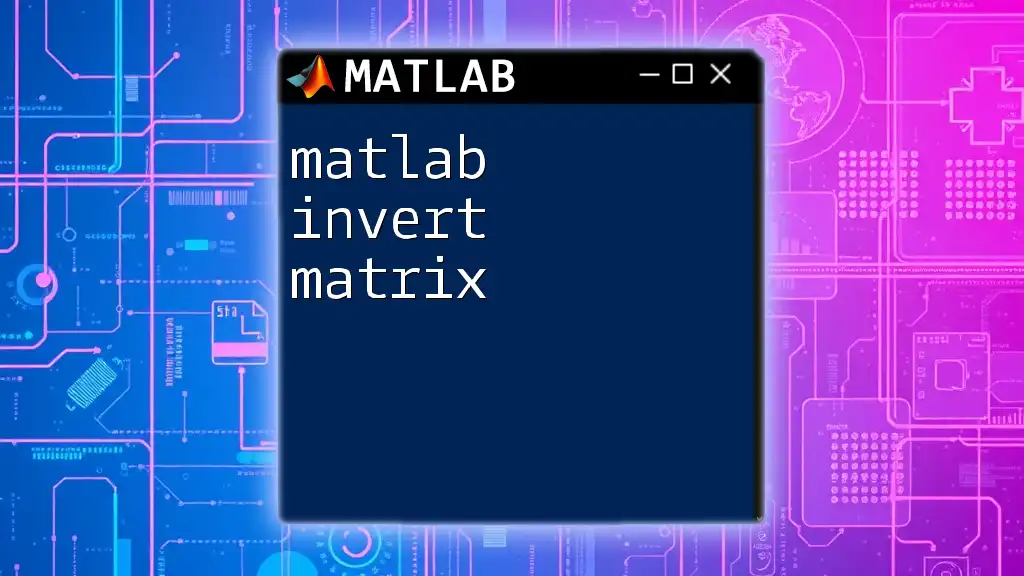
FAQs
What are the main diagonal and secondary diagonal?
The main diagonal runs from the top left to the bottom right of a matrix, while the secondary diagonal stretches from the top right to the bottom left.
How can I create a diagonal matrix in MATLAB?
You can create a diagonal matrix using the `diag` function, which takes a vector as input and outputs a square matrix with the vector's elements placed on its diagonal.
Can I manipulate diagonal elements in a matrix?
Yes, diagonal elements can be modified directly by indexing the matrix and assigning new values to the appropriate positions.
What are the implications of diagonals in theoretical math?
Diagonals play a significant role in various mathematical concepts, including eigenvalues, eigenvectors, and matrix transformations, making them essential for both theoretical exploration and practical application.