In MATLAB, boolean values represent logical true or false states, often used for conditional statements and indexing within arrays.
Here's a simple code snippet demonstrating boolean operations in MATLAB:
% Example of boolean operations in MATLAB
a = true; % Assigning boolean true to variable a
b = false; % Assigning boolean false to variable b
% Logical AND operation
result_and = a && b; % This will yield false
% Logical OR operation
result_or = a || b; % This will yield true
% Display results
disp(['AND Result: ', num2str(result_and)]);
disp(['OR Result: ', num2str(result_or)]);
Understanding Boolean Logic
Definition of Boolean Logic
Boolean logic operates on two distinct values: true and false. These values, fundamental to computer programming and logic circuits, can utilize various operations to manipulate and evaluate expressions. At its core lies Boolean algebra, a mathematical structure that describes the relationship between these true and false values, often using operators such as AND, OR, and NOT.
Significance of Boolean Logic in Programming
In programming, including MATLAB, Boolean logic is crucial for guiding the flow of control within scripts and functions. It determines conditions under which code segments run, directing the program's decisions like a set of traffic lights, efficiently guiding the flow of operations based on conditions. Applications such as logical conditions for filtering data, managing program states, and steering applications through user inputs are a few examples where Boolean logic becomes essential.
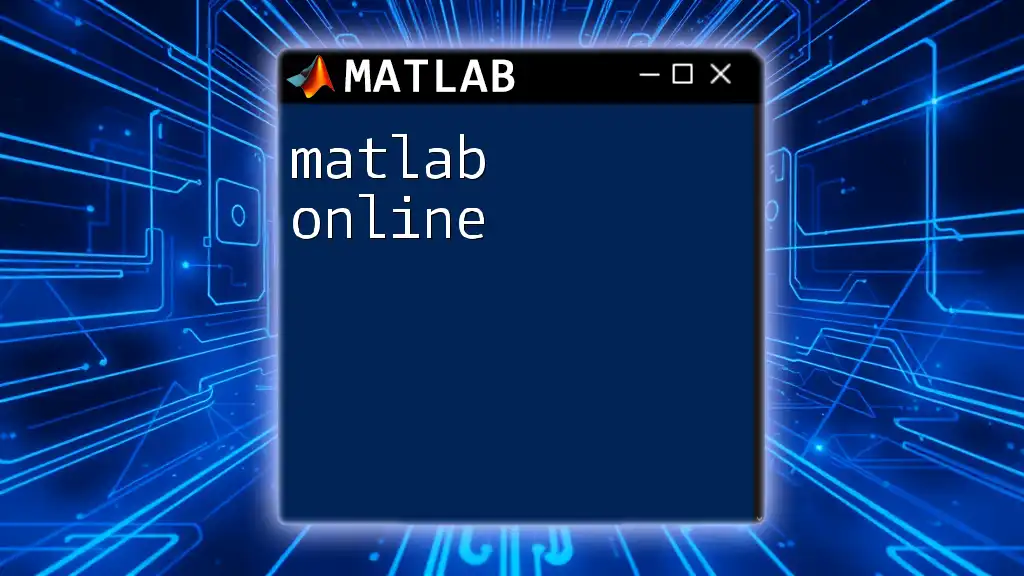
Boolean Data Types in MATLAB
Creating Boolean Values
In MATLAB, the logical data type serves as the backbone for representing Boolean values. You can easily create Boolean variables like so:
a = true;
b = false;
In this example, `a` is assigned `true`, whereas `b` represents `false`. Understanding how MATLAB stores these values is vital, as the logical data type is distinct from numerical or character types, optimizing memory usage and performance when working with Boolean expressions.
Converting Data Types to Boolean
MATLAB provides a straightforward way to convert non-Boolean data types into Boolean values using the `logical` function. This conversion adheres to simple rules, where zero is considered false and any non-zero number is regarded as true.
For example:
c = logical(7); % Converts non-zero integer to true
This line converts the integer 7 into a Boolean `true`. Thus, knowing the conversion rules can assist you when integrating logical conditions into your code.
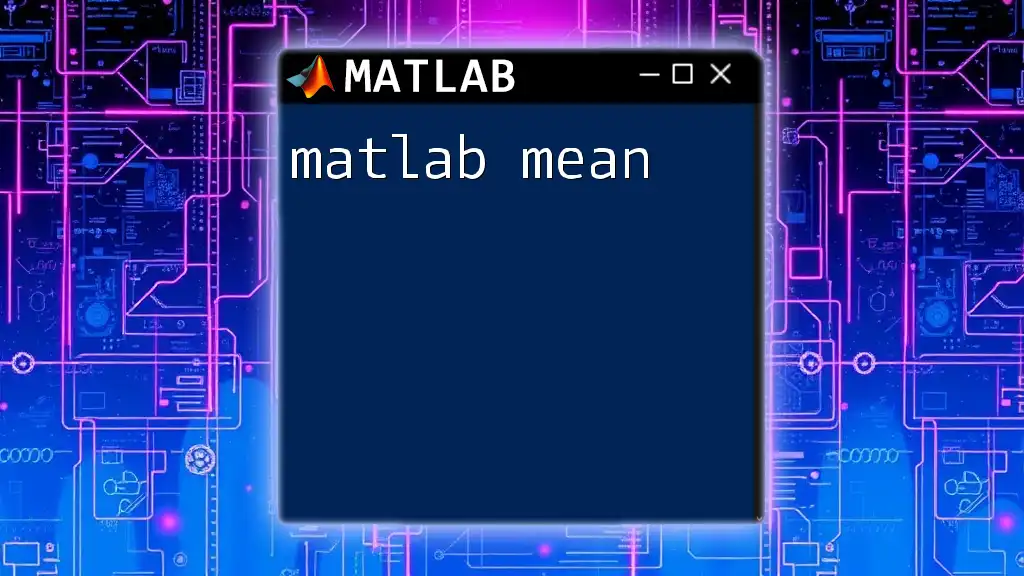
Boolean Operations in MATLAB
Basic Boolean Operators
MATLAB supports several fundamental Boolean operators: AND (`&&`), OR (`||`), and NOT (`~`). These operators leverage Boolean values to produce a new Boolean result.
Here’s how you can use these operators:
x = true;
y = false;
and_result = x && y; % Logical AND
or_result = x || y; % Logical OR
not_result = ~x; % Logical NOT
In this snippet:
- `and_result` evaluates to false because both variables must equal true for the AND condition.
- `or_result` evaluates to true as at least one of the variables is true.
- `not_result` negates the value of `x`, resulting in false.
Comparison Operations
Comparison operators in MATLAB also return Boolean values. The following operators are most commonly used: == (equal), ~= (not equal), > (greater than), and < (less than).
For instance:
a = 5;
b = 3;
isEqual = (a == b); % Returns false
isGreater = (a > b); % Returns true
Here, `isEqual` will evaluate to `false` because `a` is not equal to `b`, while `isGreater` will yield `true`. The capability to perform these comparisons and manage the results with Boolean values is fundamental for condition checking in any MATLAB application.
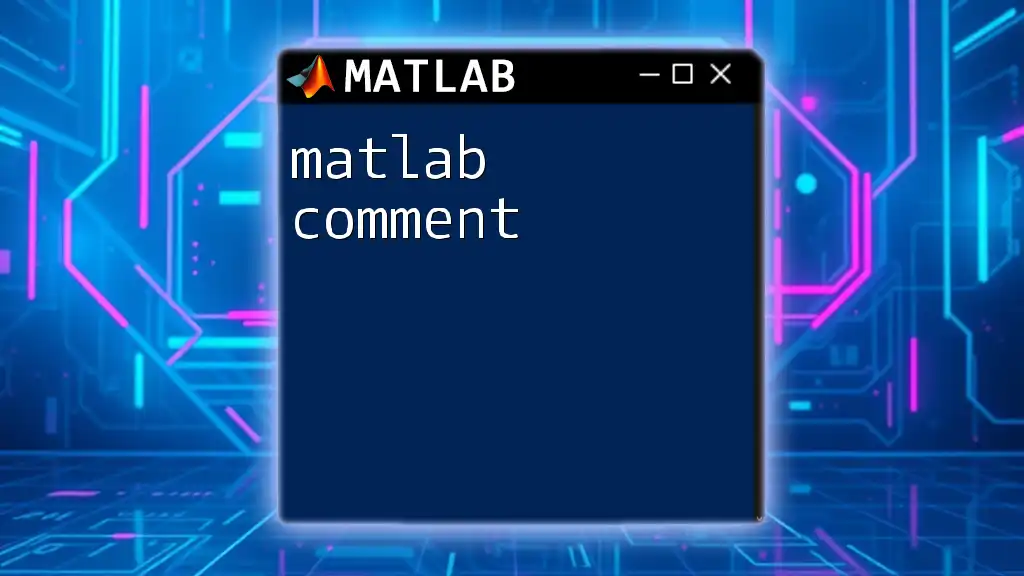
Utilizing Boolean Logic in Conditional Statements
If Statements
Conditional statements like if leverage Boolean logic for flow control. By evaluating conditions, you can direct the execution path of your program based on whether expressions are true or false.
Consider the following example:
if (a > b)
disp('a is greater than b');
else
disp('a is not greater than b');
end
In this scenario, if `a` is indeed greater than `b`, it will display the first message; otherwise, it will show the alternative. This structure allows for dynamic decision-making depending on the conditions evaluated.
Switch Statements
When you have multiple conditions to evaluate, a switch statement might be more efficient than several if-else conditions. The switch evaluates variables against several cases seamlessly.
Example:
switch a
case 5
disp('a is five');
case 6
disp('a is six');
otherwise
disp('a is neither five nor six');
end
This allows for a clean and organized means of decision-making. Using a switch instead of multiple if-else branches enhances code clarity, especially when working with numerous conditions.
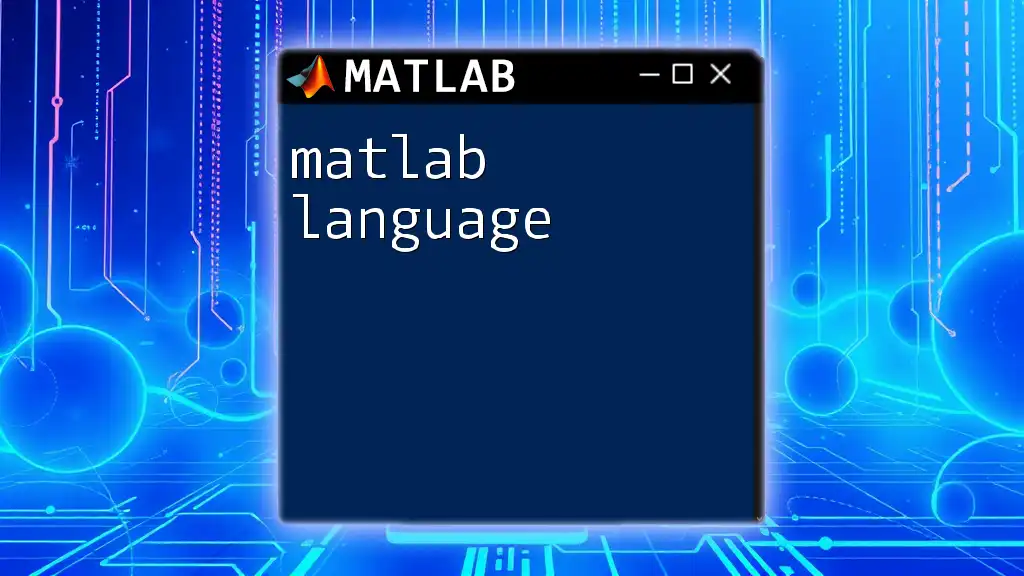
Boolean Indexing in MATLAB
What is Boolean Indexing?
Boolean indexing is a powerful feature in MATLAB that allows you to use Boolean arrays to index and manipulate data. A logical array can serve as a mask, enabling selective access to elements based on specified conditions.
Examples of Boolean Indexing
Using Boolean indexing can simplify your data manipulation tasks. For instance, if you want to select elements from an array based on certain conditions, you can do this efficiently:
array = [1, 2, 3, 4, 5];
condition = array > 3;
result = array(condition); % Returns [4, 5]
Here, `condition` is a logical array where each position corresponds to whether elements of `array` are greater than 3. Consequently, `result` contains only those elements that meet the condition. This is particularly useful for filtering and extracting data that satisfies specific criteria.
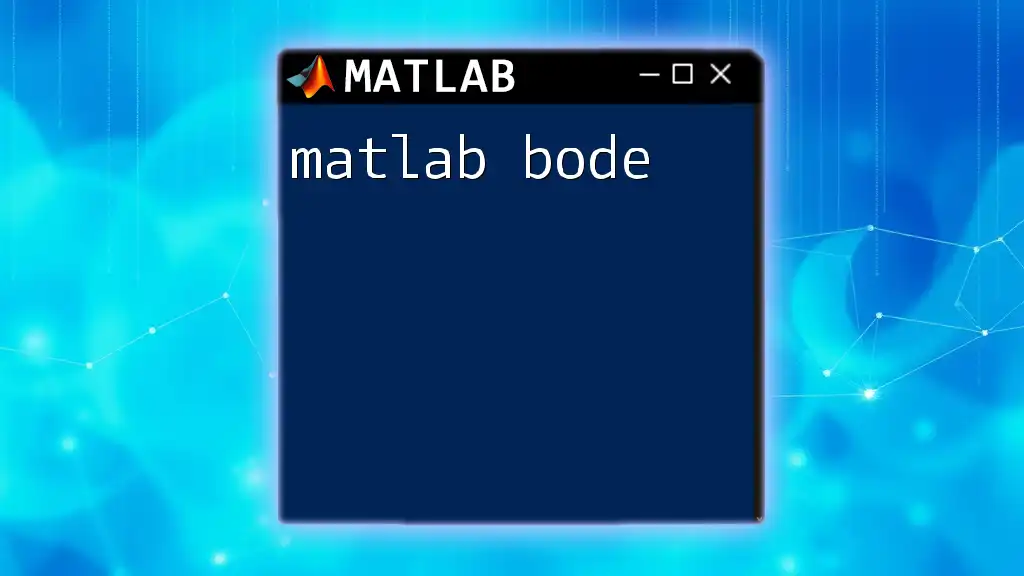
Best Practices for Using Boolean Logic in MATLAB
Writing Readable Code
While writing code with Boolean logic, prioritize clarity in your expressions. Use meaningful variable names and break complex logical expressions into smaller, readable segments. This not only enhances code maintainability but also reduces the likelihood of errors.
Common Pitfalls to Avoid
One of the frequent mistakes programmers encounter is misunderstanding operator precedence. Always use parentheses to clarify the order of operations, especially when multiple operators are used in conditions:
if (a > b) && (c < d)
disp('Both conditions are true');
end
In this snippet, parentheses explicitly define which conditions are grouped together, ensuring accurate evaluations.
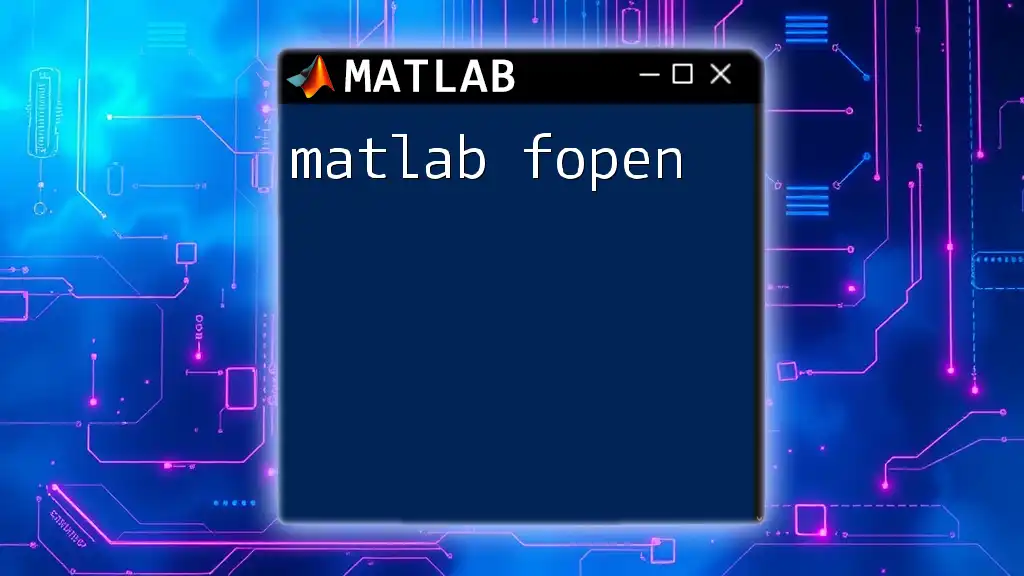
Conclusion
Understanding and effectively utilizing MATLAB Boolean logic is crucial for creating efficient and powerful programs. From simple true/false evaluations to intricate data manipulations with Boolean indexing, mastering these concepts will significantly enhance your programming skills. Continual practice with the examples provided will deepen your insight into logical operations and elevate your capabilities in using MATLAB.
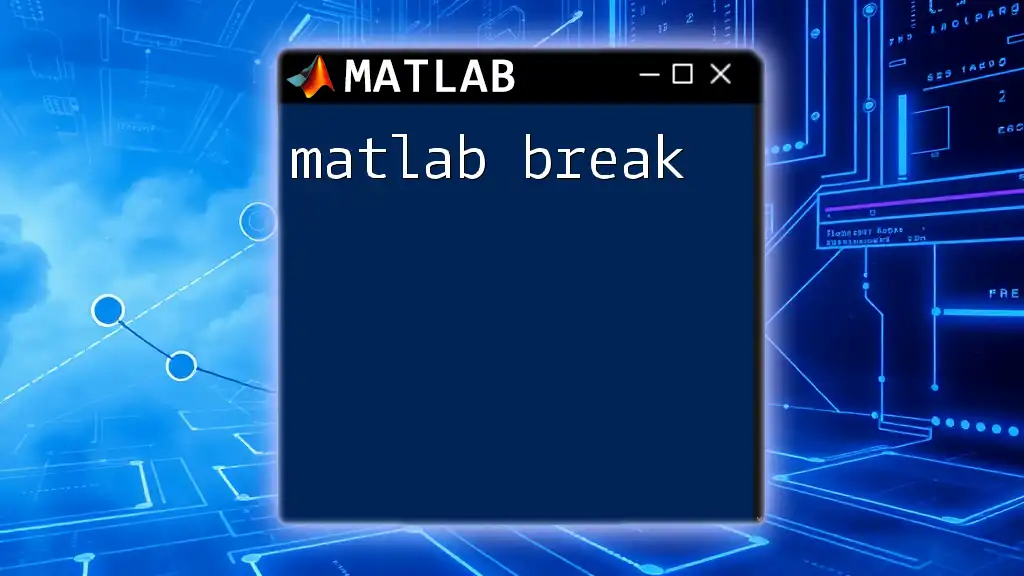
Additional Resources
For further exploration, consider diving into MATLAB's official documentation, which offers in-depth descriptions of logical operations. Additionally, various MATLAB courses and tutorials can enhance your learning experience and solidify your understanding of programming practices.