The `max` function in MATLAB is used to find the maximum value in an array or matrix, optionally returning the index of that value as well. Here's a simple code snippet demonstrating its usage:
data = [3, 5, 1, 7, 2]; % Define an array
[maxValue, index] = max(data); % Find the maximum value and its index
fprintf('Maximum value: %d at index: %d\n', maxValue, index); % Display the result
Understanding Maximum Values in MATLAB
What Does it Mean to Find a Maximum Value?
In the context of arrays and matrices, finding a maximum value refers to determining the largest number within a set of data. This is crucial across various domains, such as engineering, data analysis, and scientific computing. In practical scenarios, you might need to extract the highest temperature from a dataset, determine the peak signal in a transmission, or optimize a function in machine learning.
When to Use the `max` Function
The `max` function becomes essential when dealing with numerical data that requires understanding extreme values. For example, in signal processing, you may want to find the maximum amplitude of a recorded signal. In optimization problems, knowing the maximum value can help identify the best solution among multiple candidates.
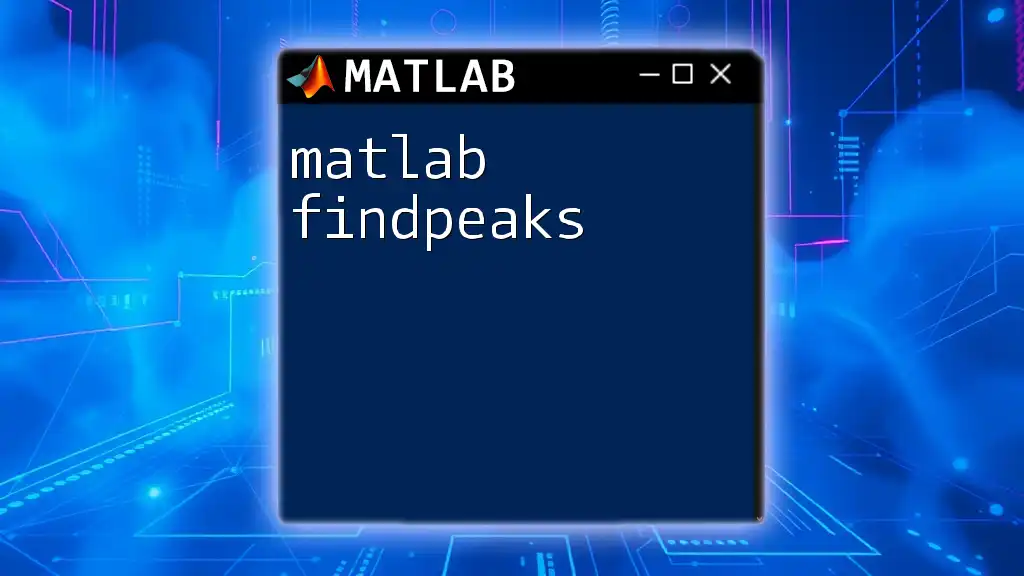
The Basics of the `max` Function
Syntax of the `max` Function
The basic syntax of the `max` function is:
M = max(A)
In this syntax:
- A represents the input array or matrix from which you want to find the maximum value.
- M is the resulting maximum value returned by the function.
Simple Examples
Example 1: Finding Maximum in a Vector
To illustrate how to find the maximum in a vector, consider the following code:
A = [3, 5, 2, 8, 1];
maxValue = max(A);
Here, `maxValue` will return 8, which is the highest number in the array. This is a straightforward way to quickly ascertain the maximum of a dataset without needing complex calculations or loops.
Example 2: Finding Maximum in a Matrix
For matrices, the `max` function can also be applied effectively. Consider this example:
B = [1, 2, 3; 4, 5, 6; 7, 8, 9];
maxValueMatrix = max(B(:)); % For the entire matrix
Using `B(:)` flattens the matrix into a single column vector. The output here will be 9, the maximum value contained within the entire matrix. This method is particularly useful when analyzing multidimensional datasets.
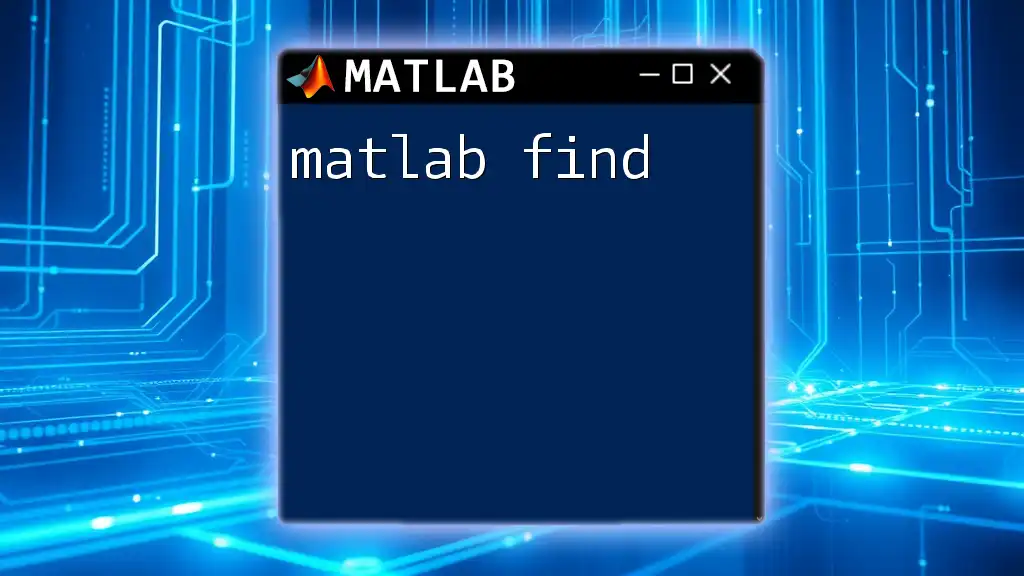
Advanced Usage of the `max` Function
Finding Row-wise and Column-wise Maximums
Row-wise Maximums
When you need the largest value from each row, the `max` function can be used with an additional argument:
rowMax = max(B, [], 2); % Maximum of each row
This will return a column vector containing the maximum value from each row:
rowMax =
3
6
9
This function can be useful in various applications such as calculating the highest score in a series of tests or determining the maximum temperature recorded in each month of a year.
Column-wise Maximums
Conversely, to find the maximum values for each column, modify the function slightly:
colMax = max(B, [], 1); % Maximum of each column
This returns a row vector of the maximums from each column:
colMax =
7 8 9
Such column-wise analyses are beneficial in experimental data where you might need to find peak outputs across different trials.
Comparing Multiple Arrays
Using `max` to Compare Two Arrays
The `max` function can also perform element-wise comparisons across multiple arrays. For instance:
C = [2, 3, 5];
D = [4, 1, 6];
elementWiseMax = max(C, D);
The result here is:
elementWiseMax =
4 3 6
This feature is helpful when analyzing two datasets to find out which dataset provides better results in each category.
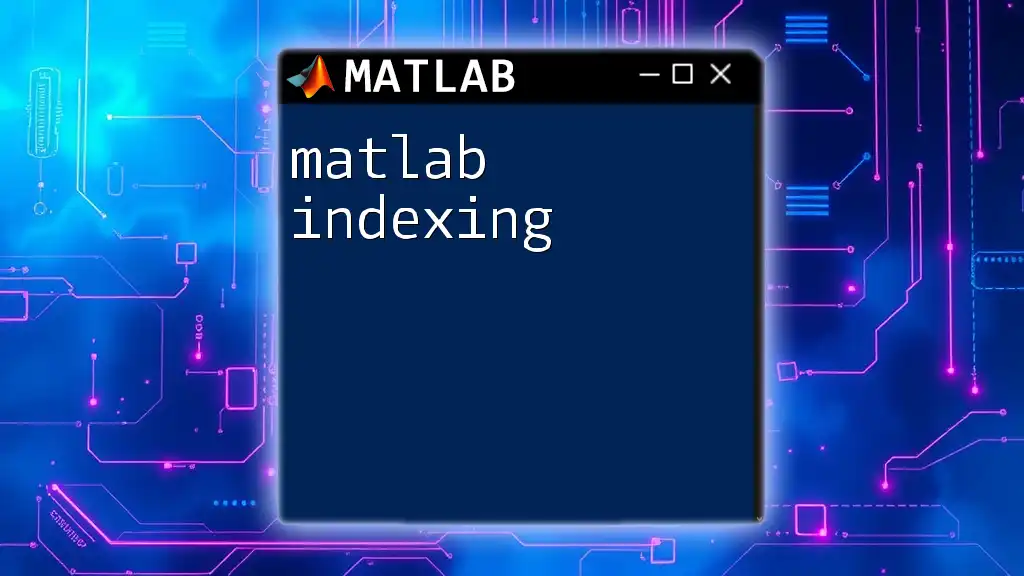
Dealing with NaN Values
Finding Maximum Values When NaN Exists
In many data analysis scenarios, datasets may contain NaN (Not a Number) values which can skew the results of the `max` function. To appropriately handle these cases, include an additional option to omit NaNs:
E = [NaN, 2, 3, NaN, 4];
maxWithNaN = max(E, [], 'omitnan'); % Omitting NaN values
With this code, `maxWithNaN` returns 4, which is the highest value ignoring the NaNs. Effectively managing NaN values ensures that your analyses remain accurate and reliable.
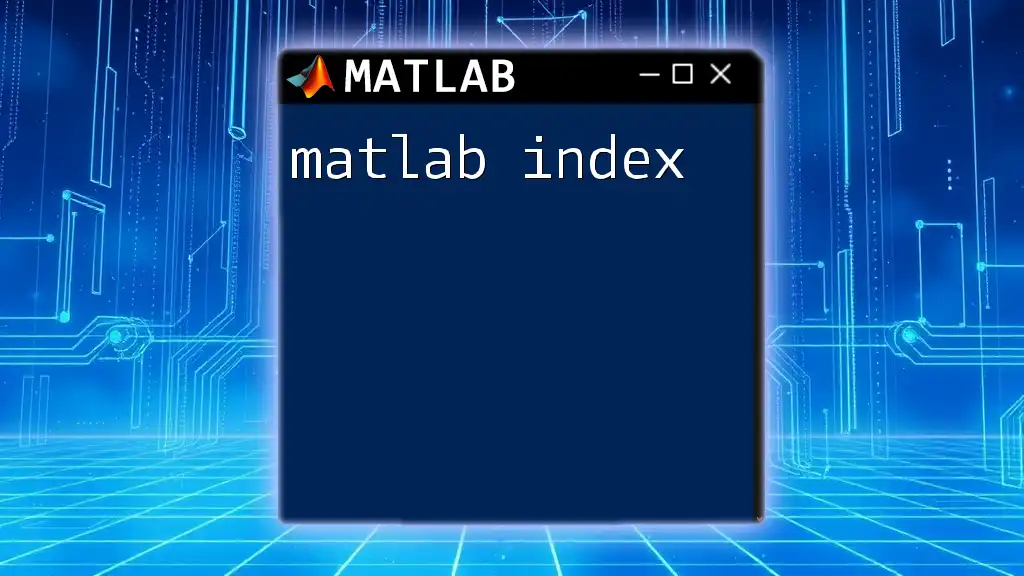
Additional Tips and Best Practices
Tips for Efficiently Using `max` in MATLAB
When working with the `max` function, it's essential to understand the data structures involved. Take time to become familiar with how MATLAB handles different data types, as this can influence the results significantly.
Common Mistakes to Avoid
Be cautious when referencing dimensions. Mixing up rows and columns can lead to incorrect results; always check the output structure of the function. Also, neglecting NaN values can produce misleading results, emphasizing the need for data cleaning before analysis.
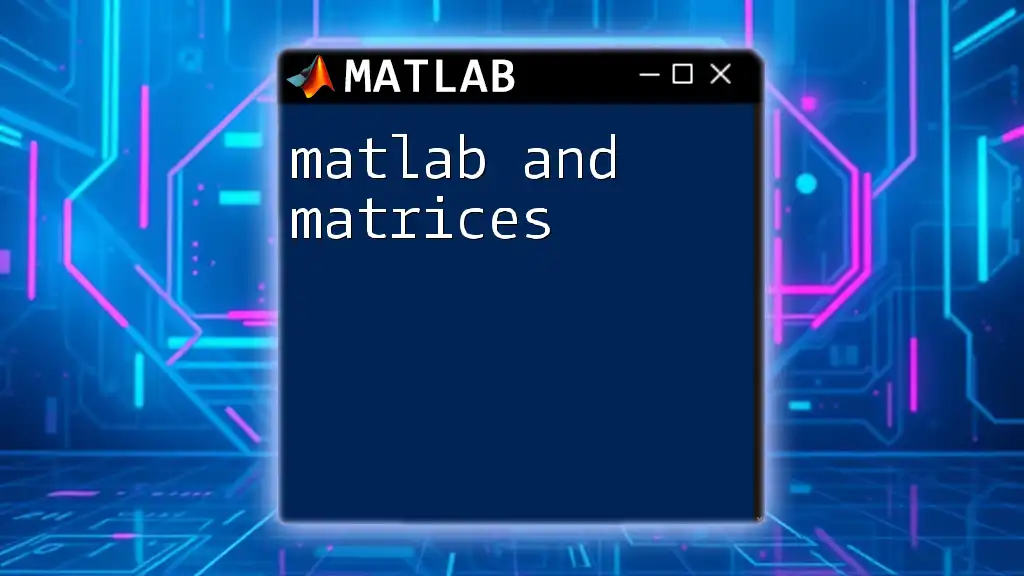
Conclusion
Mastering the `max` function empowers users to effectively analyze data and extract meaningful insights quickly. Through various examples and applications, you have learned how to leverage this powerful tool in MATLAB. Encourage practice with real datasets to build proficiency and confidence in using MATLAB for data-centric tasks.
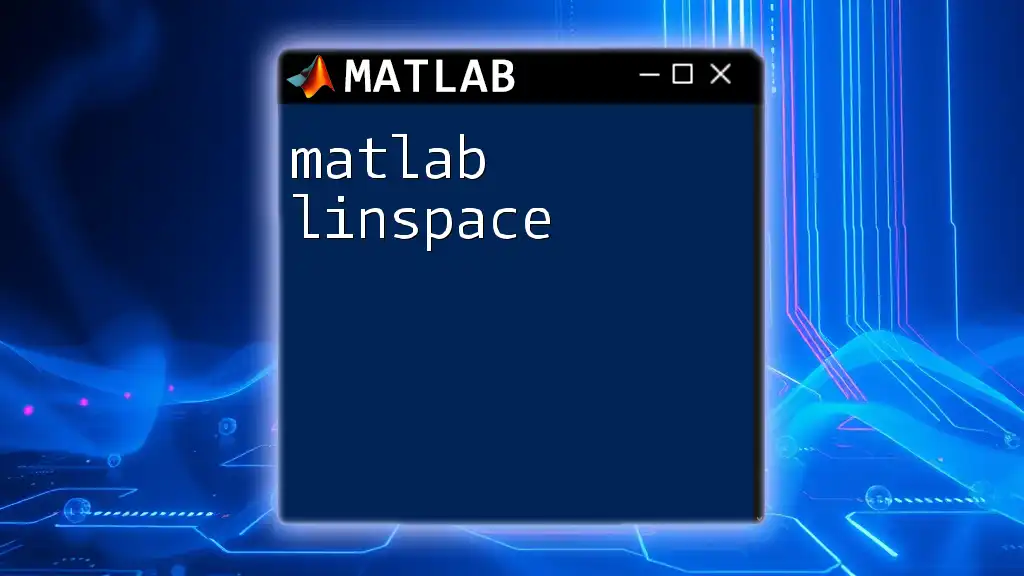
Further Reading and Resources
For those looking to expand their knowledge about the `max` function in MATLAB, consider visiting the official MATLAB documentation. Engaging with additional resources, tutorials, and hands-on projects will deepen your understanding and proficiency.
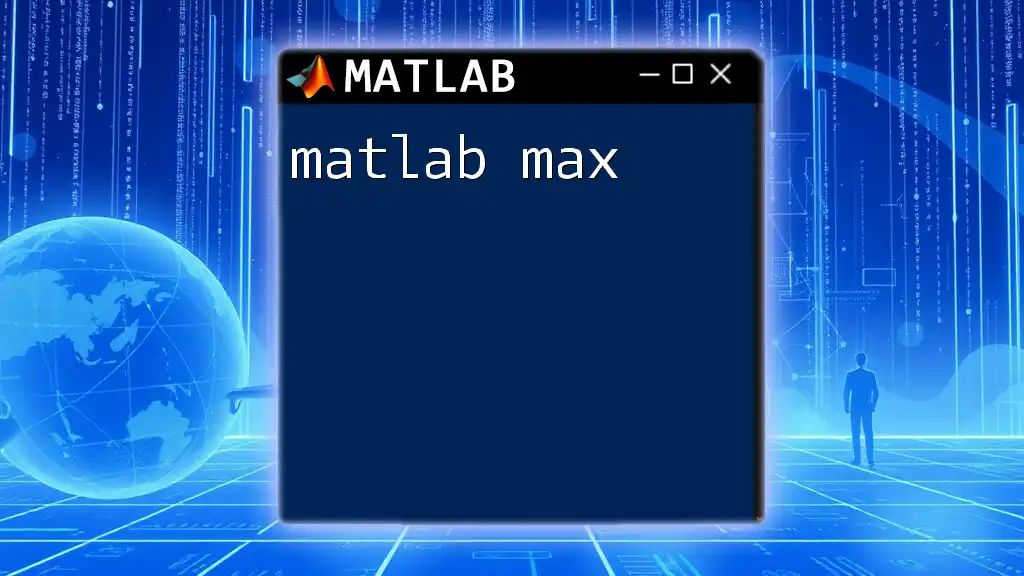
Call to Action
For more MATLAB insights and to stay updated with the best practices in using MATLAB commands, subscribe to our newsletter. Enhance your learning with our free eBook on getting started with MATLAB today!