The `floor` function rounds a number down to the nearest integer, while the `ceil` function rounds it up to the nearest integer in MATLAB.
Here is a code snippet illustrating both functions:
% Example of using floor and ceil in MATLAB
value = 5.7;
flooredValue = floor(value); % Result: 5
ceiledValue = ceil(value); % Result: 6
Understanding the Basics of Floor and Ceiling Functions
What are Floor and Ceiling Functions?
The floor and ceiling functions are essential mathematical operations used in many programming applications, including MATLAB. The floor function rounds a number down to the nearest integer, while the ceiling function rounds a number up to the nearest integer.
Why Use Floor and Ceiling Functions in MATLAB?
Utilizing floor and ceiling functions in MATLAB is crucial for a variety of reasons. They are particularly useful in data analysis when one needs to round numbers for discrete computations. Such functions serve importance in algorithm development and numerical methods, where precision and accurate data representation are vital. Whether you are working in engineering simulations, statistical analysis, or financial calculations, understanding these functions will refine your approach to numerical data.
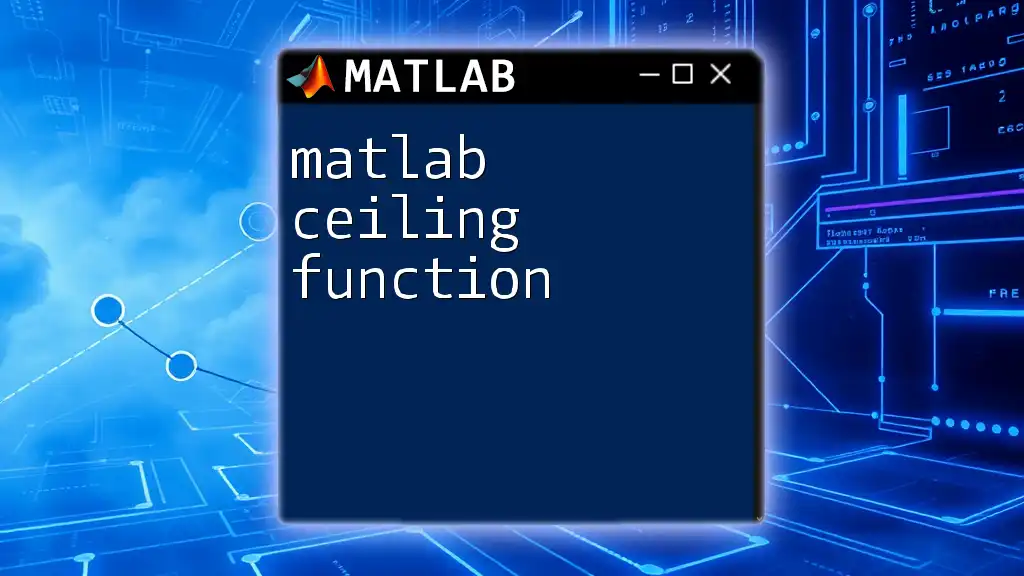
Using the Floor Function in MATLAB
Syntax of the Floor Function
The syntax for the floor function in MATLAB is straightforward:
Y = floor(X)
In this syntax:
- `X` can be a scalar or an array.
- `Y` is the resulting scalar or array after applying the floor operation.
Examples of the Floor Function
Example 1: Single Value
Consider the following code snippet:
x = 3.7;
y = floor(x);
disp(y); % Output: 3
In this example, the number 3.7 gets rounded down to 3. This showcases the basic functionality of the floor command.
Example 2: Array of Values
Now let’s look at how the floor function operates on an array:
x = [2.3, 3.9, -1.1, -4.5];
y = floor(x);
disp(y); % Output: [2, 3, -2, -5]
Here, we see that:
- Positive numbers are rounded down towards zero.
- For negative values, the rounding goes away from zero, demonstrating how floor functions behave differently near zero.
Practical Applications of the Floor Function
In practical applications, the floor function can be employed in various scenarios:
- In finance, when calculating discounts or tax calculations, using floor prevents unexpected fractional results.
- In engineering, floor might be crucial for deciding the number of components required when specific quantities cannot be fractional, for instance, the number of sensors needed.
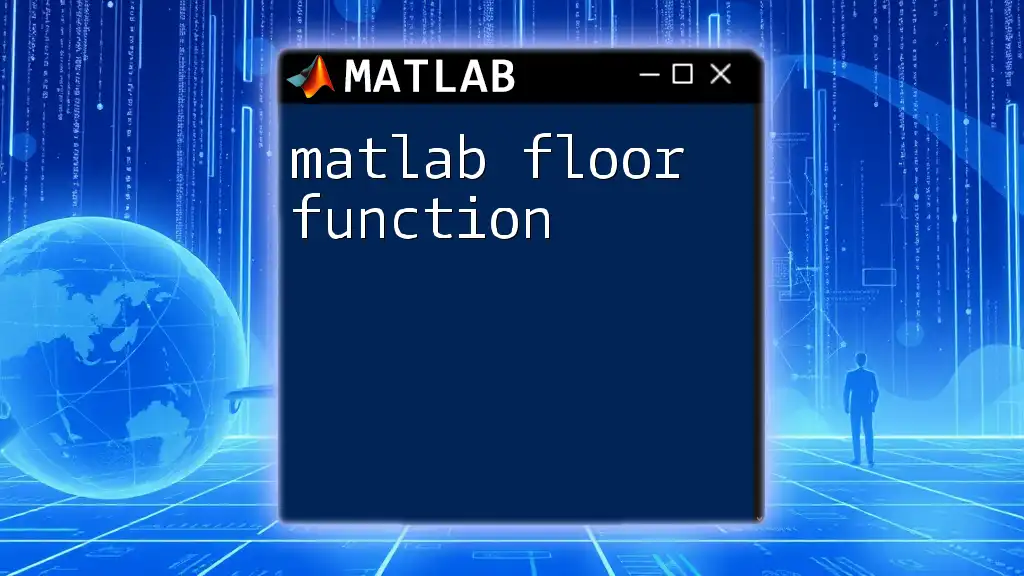
Using the Ceiling Function in MATLAB
Syntax of the Ceiling Function
The ceiling function in MATLAB follows a similar syntax:
Y = ceil(X)
Here, just like in the floor function, `X` represents the input array or scalar, and `Y` is the corresponding output.
Examples of the Ceiling Function
Example 1: Single Value
Let’s see how the ceiling function works with a single value:
x = 3.2;
y = ceil(x);
disp(y); % Output: 4
This snippet demonstrates that `ceil` rounds up to the nearest integer, resulting in 4.
Example 2: Array of Values
Now, let us explore the ceiling function applied to an array:
x = [1.5, 2.2, -3.7, -2.1];
y = ceil(x);
disp(y); % Output: [2, 3, -3, -2]
In this instance:
- Positive decimal values round up to the next integer.
- Negative values also round, but towards zero, showcasing a critical aspect of how ceiling works differently with negative numbers.
Practical Applications of the Ceiling Function
The ceiling function finds its utility in various domains:
- In data storage calculations, it ensures that rounding up guarantees sufficient space or resources.
- In programming algorithms that require integer results from floating-point calculations, ceiling can help prevent data type errors.
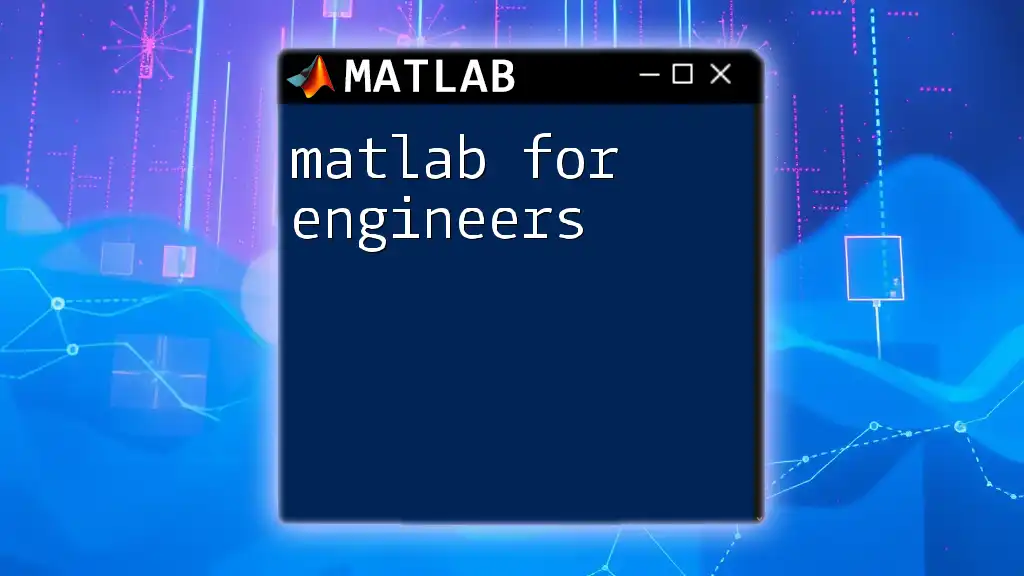
Differences Between Floor and Ceiling Functions
Mathematical Differences
Understanding the differences between these two functions is key:
- The floor function always rounds down towards negative infinity, regardless of the decimal part of the number.
- The ceiling function always rounds up towards positive infinity.
Practical Implications in Calculations
When deciding which function to utilize in calculations, consider the context:
- The floor function is often more valuable in cases where rounding to the nearest lower limit is essential.
- Conversely, the ceiling function should be used when it’s critical to round up, particularly in resource allocation problems or scenarios involving bounds.
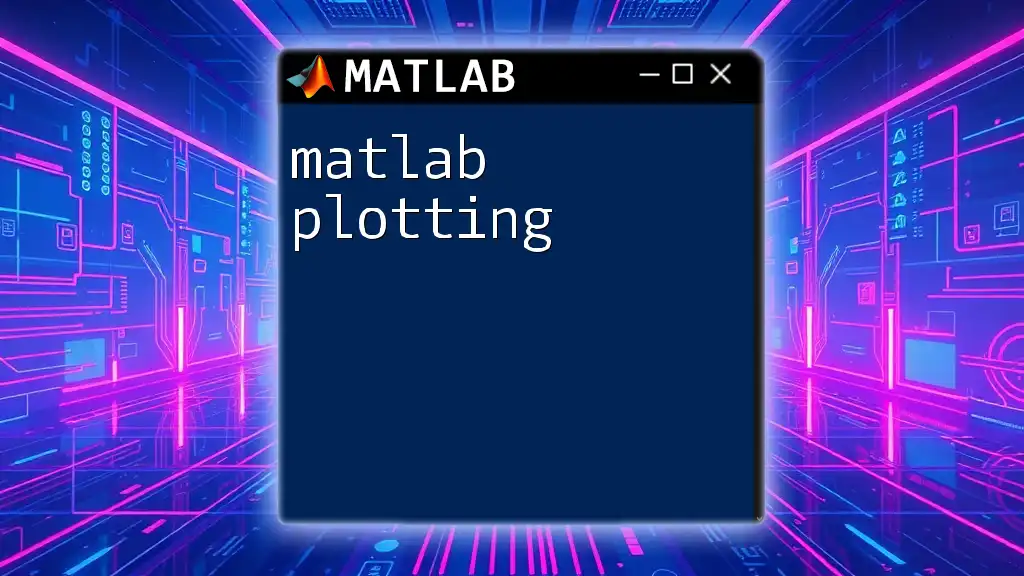
Combining Floor and Ceiling Functions
Using Both Functions Together
Sometimes you may need both functions for a single computation. For example:
x = [3.4, 4.6, -2.9, -1.1];
floor_vals = floor(x);
ceil_vals = ceil(x);
disp([floor_vals', ceil_vals']); % Outputs two columns; floors and ceilings
This provides a clear side-by-side comparison of both functions applied to the same vector.
Nested Use Cases
In more complex algorithms, you might find yourself using one function within the context of another:
result = ceil(floor(x)); % Explaining the redundancy
While this uses both functions, it highlights the interplay in certain scenarios, like ensuring that you never exceed a physical limit in calculations.
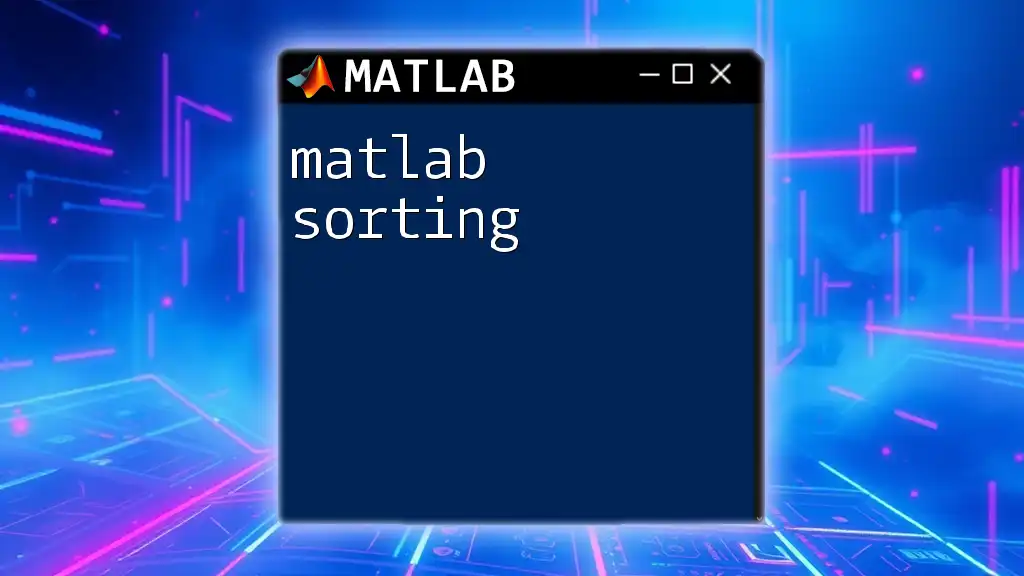
Conclusion
In summary, understanding MATLAB floor and ceiling functions can enhance your coding practices significantly. These functions serve as foundational tools not only in MATLAB programming but also in broader calculations across various fields. By incorporating these rounding techniques into your workflow, you can improve the accuracy and reliability of your results.
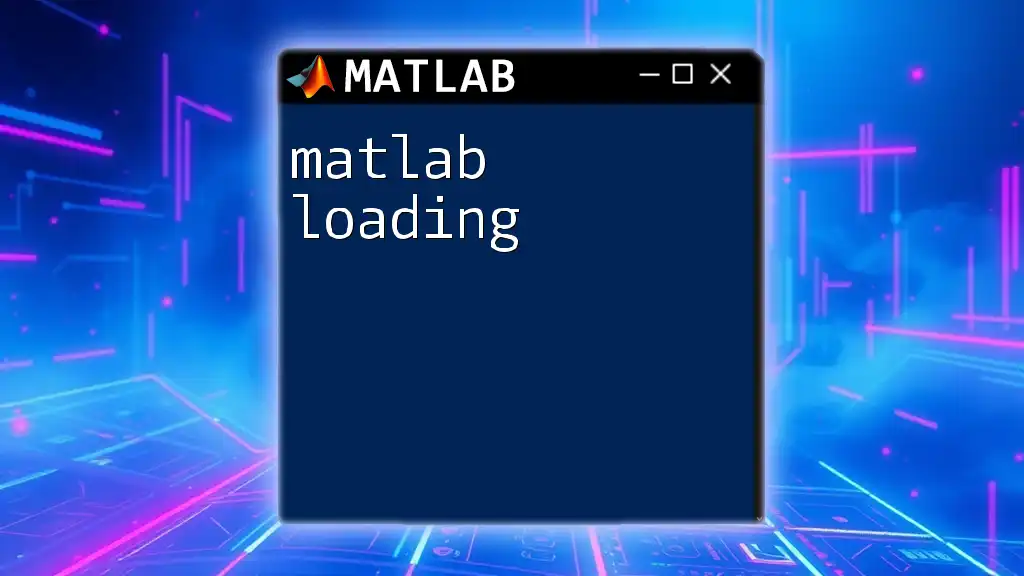
Additional Resources
For those looking to deepen their knowledge, consult the official MATLAB documentation on the `floor` and `ceil` functions. Additionally, explore readings on numerical methods to grasp better rounding techniques in programming.
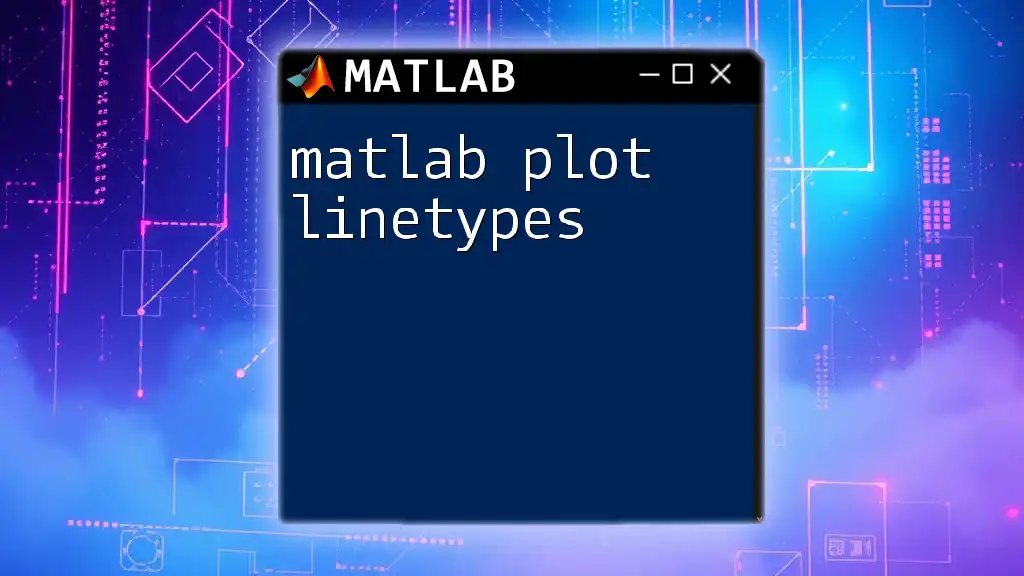
Call to Action
We invite you to share your experiences using these functions in MATLAB. Did you find a unique application? Feel free to comment below and share your insights while spreading the knowledge with others!