MATLAB is an essential tool for students, providing a powerful environment for numerical computing and data visualization through concise commands.
Here’s a simple example of how to plot a sine wave in MATLAB:
x = 0:0.1:2*pi; % Create a vector from 0 to 2*pi with increments of 0.1
y = sin(x); % Compute the sine of each value in x
plot(x, y); % Plot the sine wave
title('Sine Wave'); % Add a title
xlabel('x'); % Label x-axis
ylabel('sin(x)'); % Label y-axis
grid on; % Turn on the grid
What is MATLAB?
MATLAB, short for MATrix LABoratory, is a high-level programming language and interactive environment designed primarily for numerical computation, visualization, and programming. It is widely used in academia, especially in engineering and mathematics fields, due to its powerful tools for data manipulation, algorithm implementation, and graphical data representation.
Applications of MATLAB are diverse. Students frequently use it for:
- Engineering simulations to test and visualize designs before implementation.
- Data analysis, helping to make sense of complex datasets.
- Algorithm development, allowing for rapid prototyping and testing.
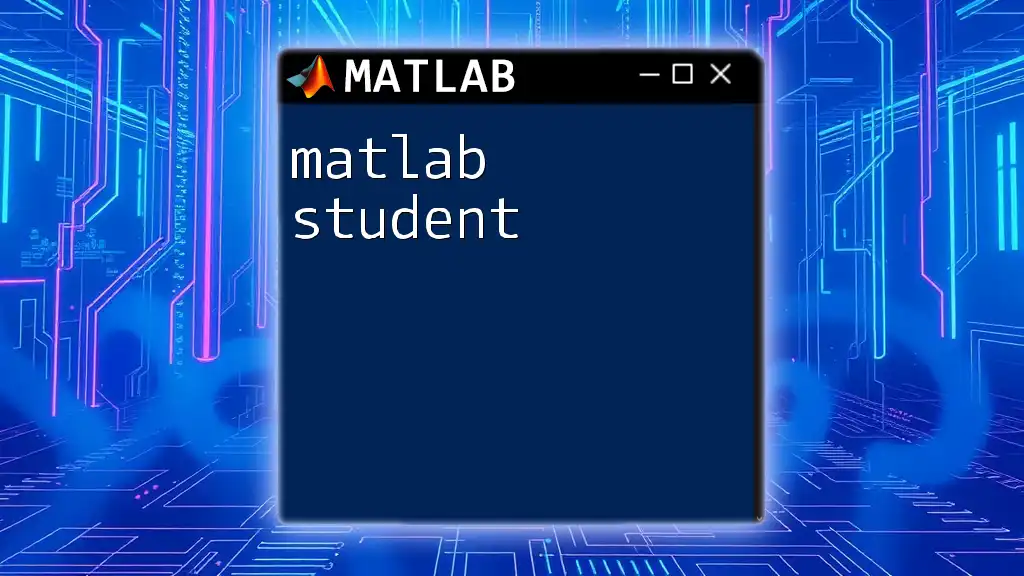
Getting Started with MATLAB
Installation and Setup
The first step in your MATLAB journey is to install and set up the software. To get started:
- Download the MATLAB installer from the official MathWorks website.
- Follow the installation instructions, ensuring your system meets the necessary requirements.
It's essential to note that MATLAB often requires a license, which many universities provide for students, either for free or at a reduced cost.
Navigating the MATLAB Environment
After installation, familiarize yourself with the MATLAB environment. The interface consists of several key components:
- Command Window: Where you enter commands and see outputs.
- Workspace: Displays all the variables currently in memory.
- Editor: A space to write, edit, and debug scripts.
Understanding the layout is crucial for efficient navigation and usage of the software, as it streamlines your workflow.
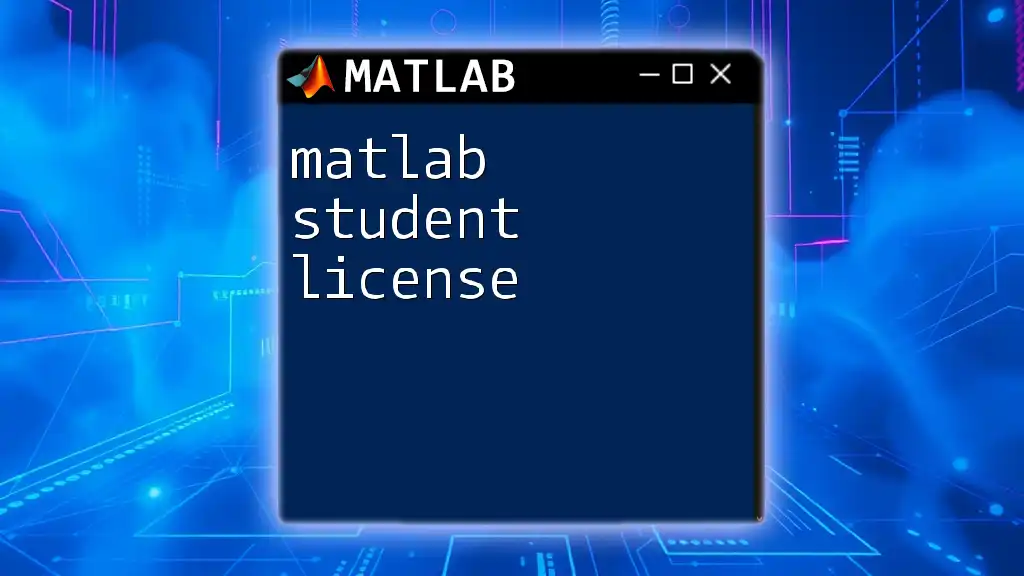
Basic MATLAB Commands
Essential Commands for Beginners
To harness the power of MATLAB, beginners should master a few fundamental commands:
- `clc`: Clears the Command Window.
- `clear`: Removes all variables from memory.
- `close all`: Closes all open figures.
These commands help maintain a neat workspace and reset your environment when starting new tasks.
Creating Variables and Data Types
In MATLAB, creating variables involves straightforward syntax. You can define variables of various data types, including:
- Scalar: A single value.
- Vector: A one-dimensional array of values.
- Matrix: A two-dimensional array.
For example, the following code demonstrates how to create different variable types:
a = 5; % Scalar
b = [1, 2, 3]; % Row vector
c = [1 2; 3 4]; % Matrix
Understanding data types is crucial, as MATLAB is optimized for matrix operations, making it exceptionally well-suited for numerical analysis.
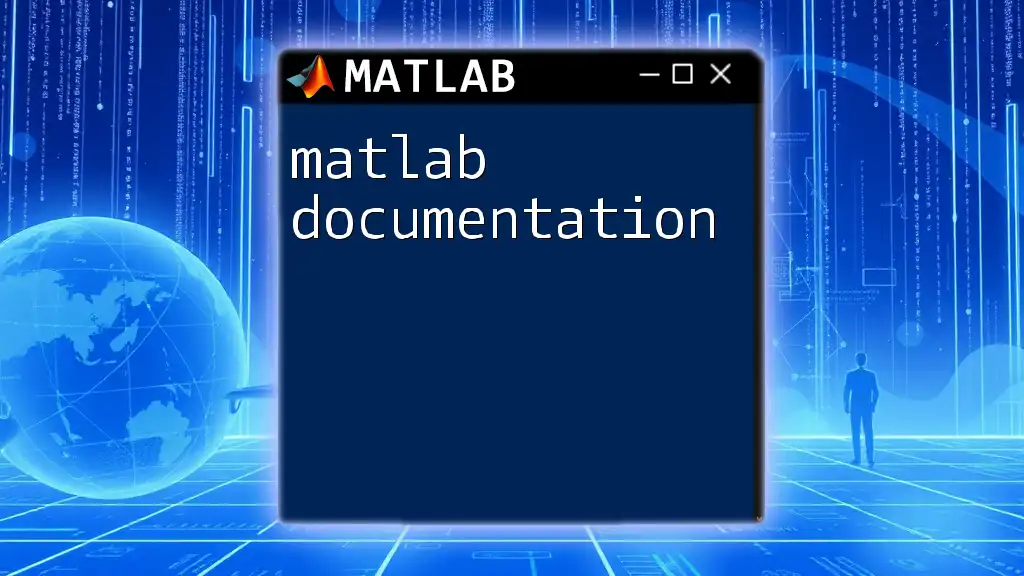
Working with Vectors and Matrices
Introduction to Arrays
Arrays form the backbone of MATLAB. Students must grasp how to manipulate arrays, as they are integral to most computations you'll perform.
Basic Array Operations
MATLAB supports a wide range of array operations, including element-wise operations, such as addition, subtraction, and multiplication. For instance:
A = [1 2; 3 4];
B = [5 6; 7 8];
C = A + B; % Matrix addition
Here, `C` becomes:
C = [6 8;
10 12]
This illustrates how matrix operations occur in MATLAB, emphasizing its powerful mathematical capabilities.
Indexing and Slicing
One of the key features of MATLAB is its indexing system. Students can access specific elements or subsets of arrays, allowing for sophisticated data manipulation. For example:
element = A(1,2); % Accesses the element in row 1, column 2
Indexing not only helps in retrieving data but also in performing operations on individual elements or a subset of an array.
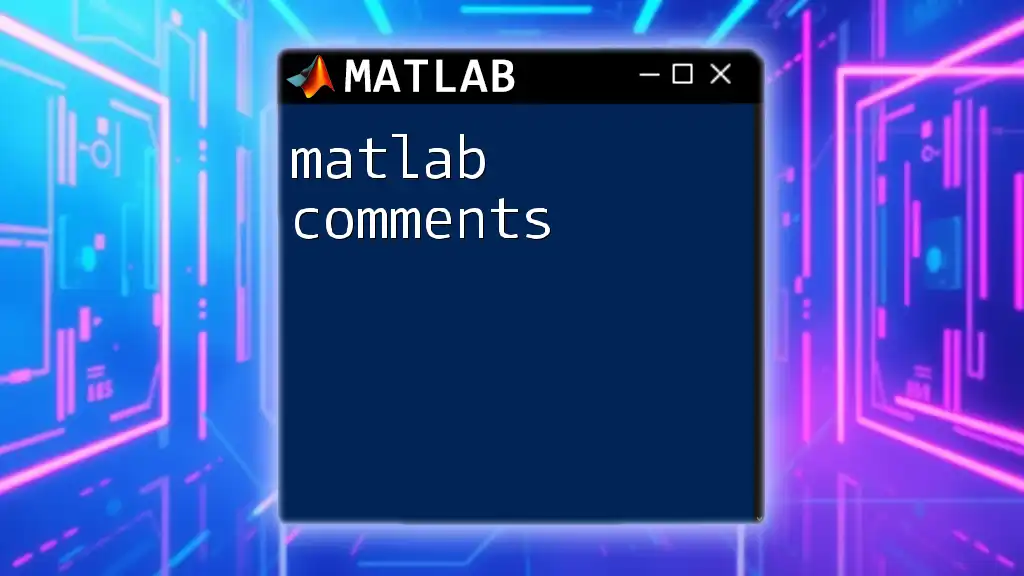
Understanding Functions in MATLAB
Defining Functions
Defining functions in MATLAB is essential for creating reusable code. The syntax for defining a function typically includes:
- The function name.
- The input arguments.
- The output values.
Here is a simple example:
function result = squareNumber(x)
result = x^2;
end
Functions encourage modular programming, making your code cleaner and easier to follow.
Using Built-in Functions
MATLAB offers a plethora of built-in functions for various tasks, including:
- Statistical analysis (e.g., `mean`, `median`, `std`).
- Mathematical computations (e.g., `sqrt`, `sin`, `cos`).
For instance, to compute the sum of an array, you can do:
data = [1, 2, 3, 4, 5];
total = sum(data); % Calculates the sum of elements
Utilizing built-in functions can save time and simplify code complexity.
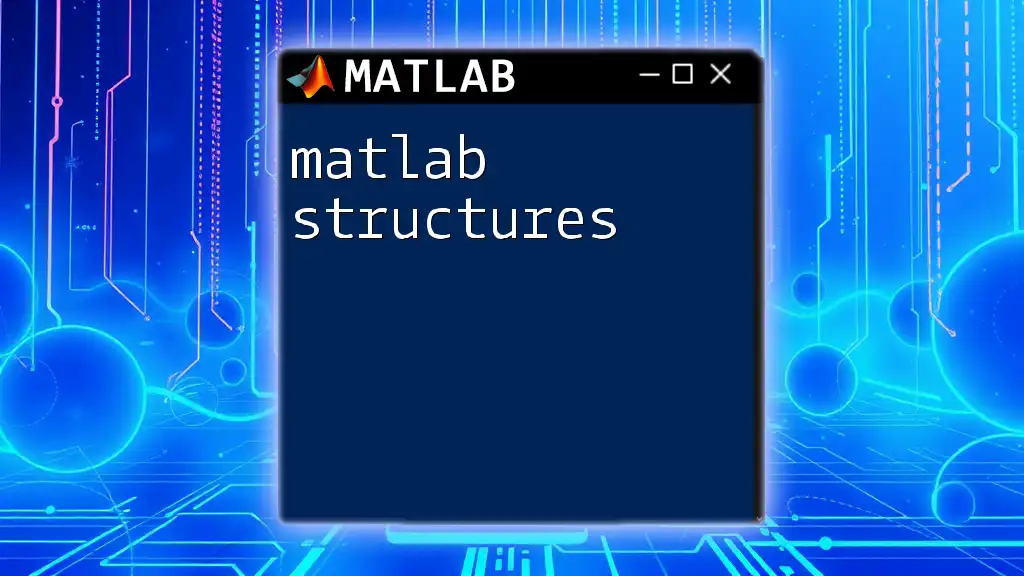
Data Visualization in MATLAB
Creating Plots
Data visualization is a vital skill for any student working with numerical data. MATLAB provides intuitive commands for creating a variety of plots.
Basic Plotting Commands
Beginning with the `plot` function allows students to visualize data effectively. Here’s an example:
x = 0:0.1:10; % Creating a vector from 0 to 10 with increments of 0.1
y = sin(x); % Calculating the sine of each x value
plot(x, y); % Plotting the sine function
This will display a simple sine wave graph, illustrating the relationship between the x and y values.
Customizing Plots
Customization of plots enhances their interpretability. Adding titles, labels, and legends can make plots more informative. Here’s how to customize a plot:
plot(x, y);
title('Sine Wave');
xlabel('X-axis');
ylabel('Y-axis');
legend('sin(x)');
grid on;
These enhancements are essential for creating professional-quality graphics that convey your data effectively.
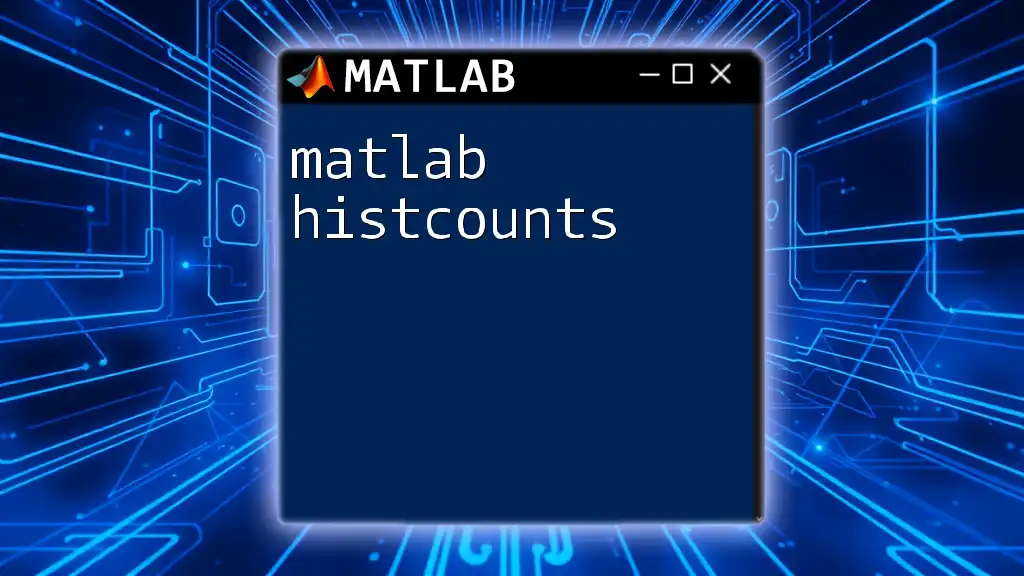
Common Issues and Troubleshooting
Debugging in MATLAB
Every student encounters errors during programming. Learning to debug is an essential skill. Common issues might include variable conflicts, syntax errors, or incorrect function calls. MATLAB’s built-in debugging tools, such as breakpoints and step execution, help track down errors efficiently.
Resources for Help
Whenever you need assistance, turn to:
- MATLAB documentation: It contains extensive resources, tutorials, and examples.
- Online forums and communities: Sites like Stack Overflow provide quick resolutions from peers and experts.
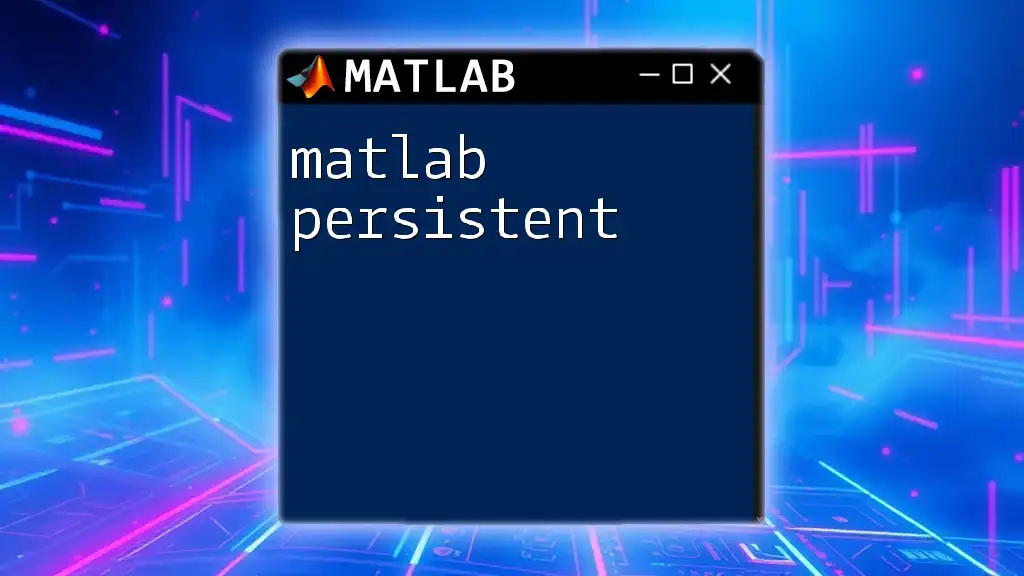
Advanced Topics for Students
Introduction to Simulink
As students advance, they may find Simulink a valuable tool. Simulink extends MATLAB capabilities for modeling and simulating dynamic systems, particularly in engineering and control systems.
Data Import and Export
Understanding how to import and export data is crucial for any data analysis task. MATLAB supports various file formats, and reading a table from a CSV file can be done using:
data = readtable('datafile.csv');
This capability enables students to analyze real-world data sets efficiently.
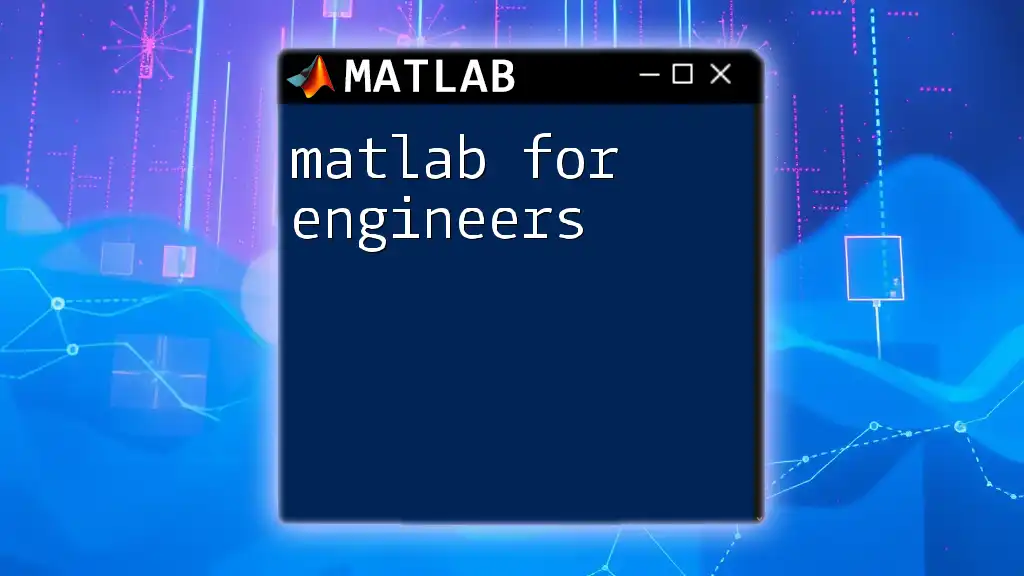
Conclusion
In summary, mastering MATLAB for students opens up a wealth of opportunities for academic and professional success. By understanding its commands, functions, and plotting methods, students can effectively solve complex mathematical problems, visualize data, and conduct simulations with confidence. Embrace the learning curve, explore advanced topics, and leverage the resources available to deepen your knowledge of MATLAB. Your journey in mastering this powerful tool is just beginning!
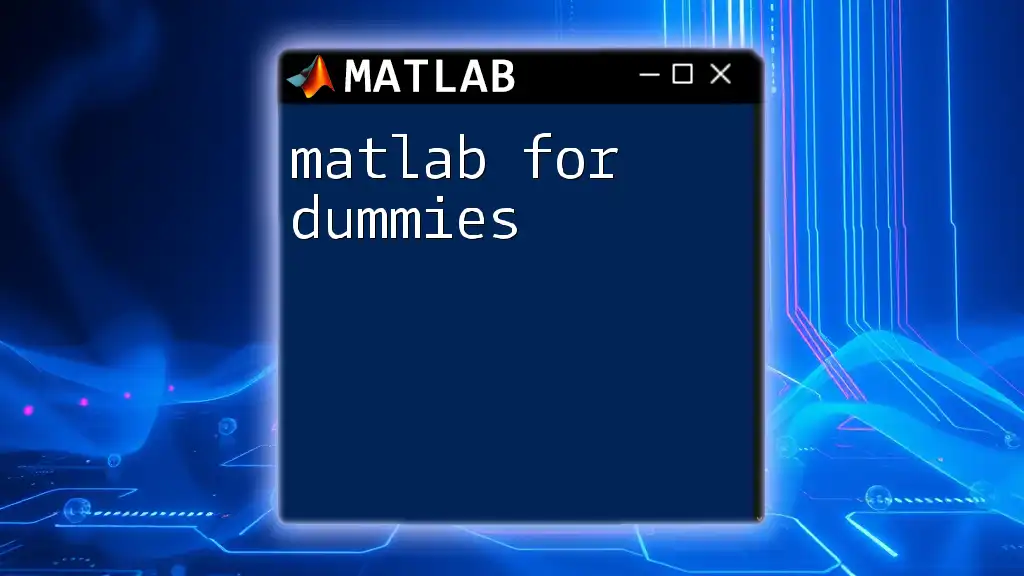
FAQ Section
As you navigate MATLAB, you might encounter common questions such as:
- How do I fix syntax errors? Reviewing the error message helps pinpoint the issue, often a missing parenthesis or a typo.
- Can I use MATLAB for statistical analysis? Absolutely! MATLAB comes equipped with many built-in statistical functions to support your analysis needs.
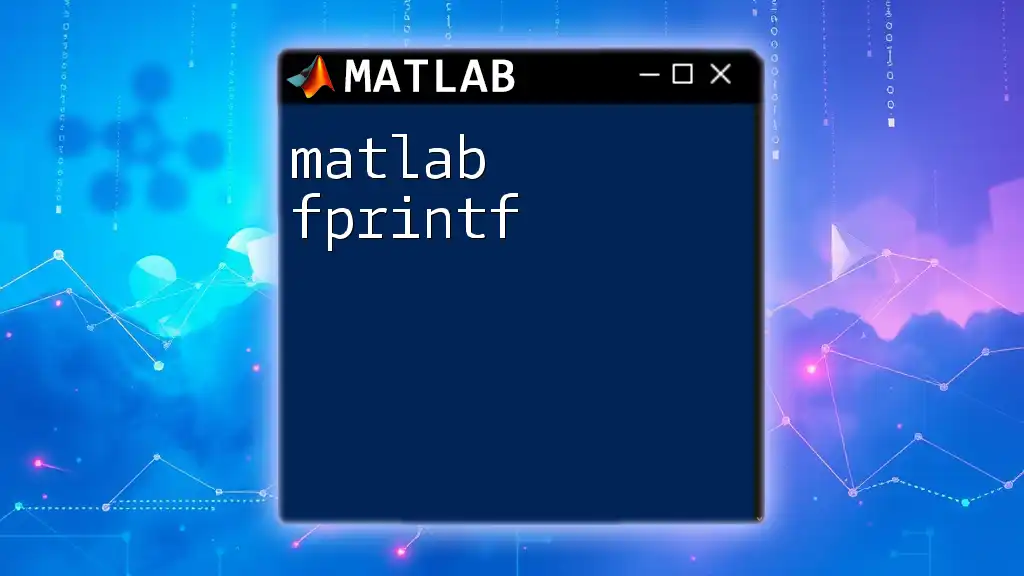
Additional Resources
Recommended Books and Online Courses
- Books on MATLAB programming cover both basic and advanced topics.
- Online courses often include video tutorials and practical exercises.
Useful Websites for MATLAB Learning
- Dive into platforms like MathWorks’ official site for resources and tutorials tailored for students.