In MATLAB, the `if` statement allows you to execute specific blocks of code based on whether a given condition is true or false. Here's a simple example:
x = 5;
if x > 0
disp('x is positive');
else
disp('x is not positive');
end
Understanding the Syntax of If Statements
When working with MATLAB for if statements, it is crucial to grasp the basic structure that allows for control flow in your scripts. The fundamental syntax to create an if statement in MATLAB is as follows:
if condition
% Code to execute if condition is true
end
In this structure, condition is the logical test that MATLAB evaluates. If this condition is true, the code within the block will execute. This simplicity makes if statements an essential tool for decision-making in MATLAB programming.
Elif and Else Constructs
To handle multiple conditions, you can extend the basic if statement by incorporating `elseif` and `else`. This means you can create a more robust control flow. The syntax looks like this:
if condition1
% Code for condition1
elseif condition2
% Code for condition2
else
% Code when none are true
end
This structure allows your program to check a sequence of conditions, leading to increased flexibility. It is especially useful in situations where multiple scenarios might fit.

Logical Conditions and Operators
Every if statement relies on logical conditions to determine execution paths. MATLAB supports various logical operators, including:
- Equality (`==`): Checks if two values are equal.
- Inequality (`~=`): Checks if two values are not equal.
- Greater than (`>`): Checks if the first value is greater than the second.
- Less than (`<`): Checks if the first value is less than the second.
- Greater than or equal (`>=`): Checks if one value is greater than or equal to another.
- Less than or equal (`<=`): Checks if one value is less than or equal to another.
You can combine these conditions using logical operators, enhancing the power of your if statements. The operators `&&` (AND), `||` (OR), and `~` (NOT) play crucial roles in compound logical expressions.
For instance, if you want to check if two conditions are true simultaneously, you could write:
if condition1 && condition2
% Code for both conditions true
end
This technique enables complex decision-making processes within your MATLAB script.
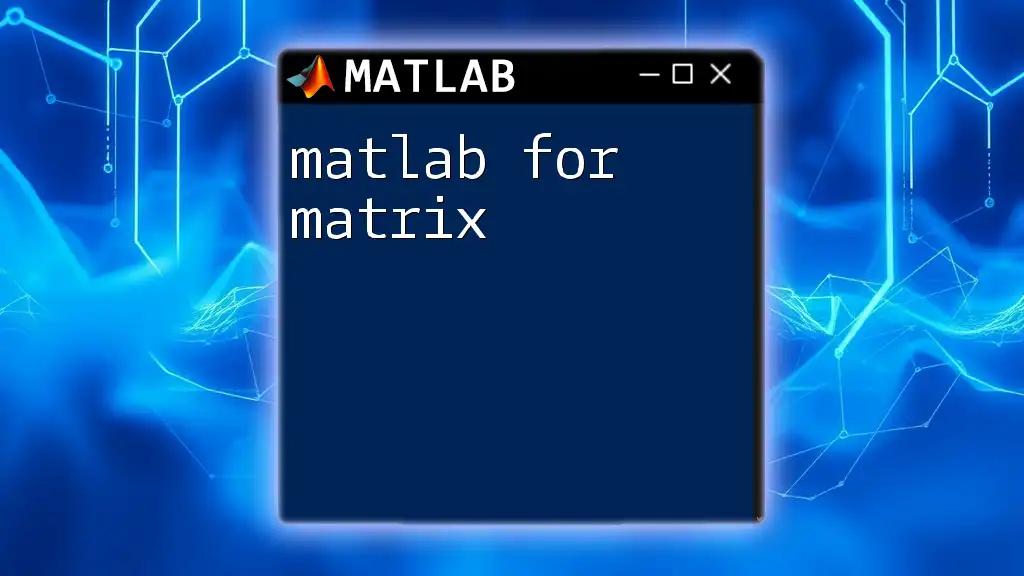
Practical Examples of Using If Statements
Example 1: Basic Numeric Comparison
Let’s consider a practical scenario to illustrate how to use if statements effectively. Imagine you want to assess students' grades. You can categorize grades into different letter grades using an if statement:
grade = 85;
if grade >= 90
fprintf('Grade: A\n');
elseif grade >= 80
fprintf('Grade: B\n');
else
fprintf('Grade: C or below\n');
end
In this example, the program checks the value of grade and prints the corresponding letter grade based on the conditions specified.
Example 2: Using If Statements with Functions
If statements also work seamlessly within functions. Consider a function that determines whether a number is even or odd:
function checkEvenOdd(num)
if mod(num, 2) == 0
fprintf('%d is even.\n', num);
else
fprintf('%d is odd.\n', num);
end
end
This function utilizes the `mod` operator to check if a number is divisible by 2. The appropriate message is displayed depending on the outcome of the condition.
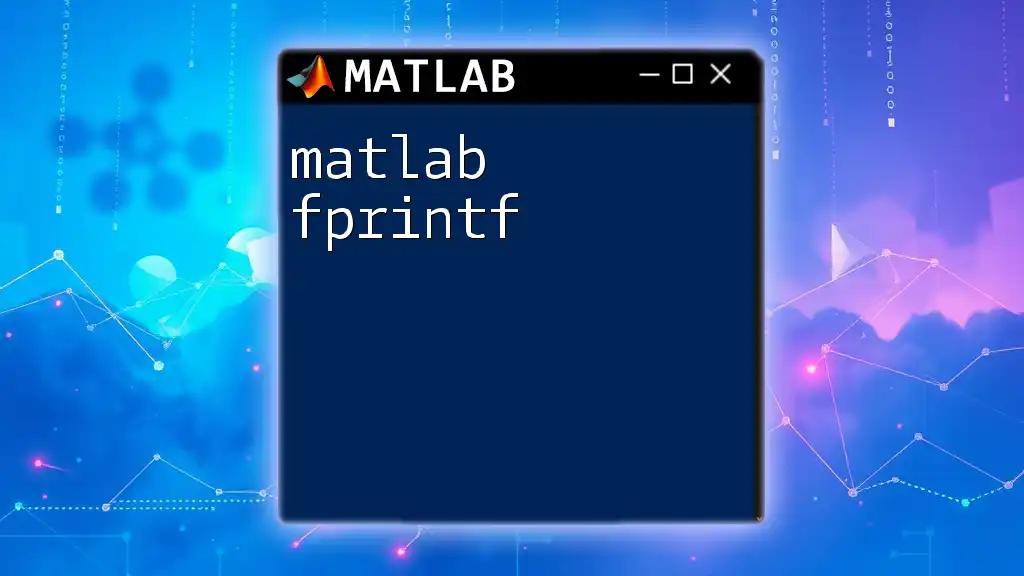
Nested If Statements
Nested if statements can create more complex decision trees. These allow you to check for additional conditions if an initial condition is met.
A basic structure for a nested if statement looks like this:
x = 10;
if x > 0
disp('Positive');
if mod(x, 2) == 0
disp('Even');
else
disp('Odd');
end
end
In this example, the first if statement checks if x is positive. If true, it proceeds to check if x is even or odd. While powerful, nested if statements can quickly become complicated, so they should be used judiciously to maintain code clarity.
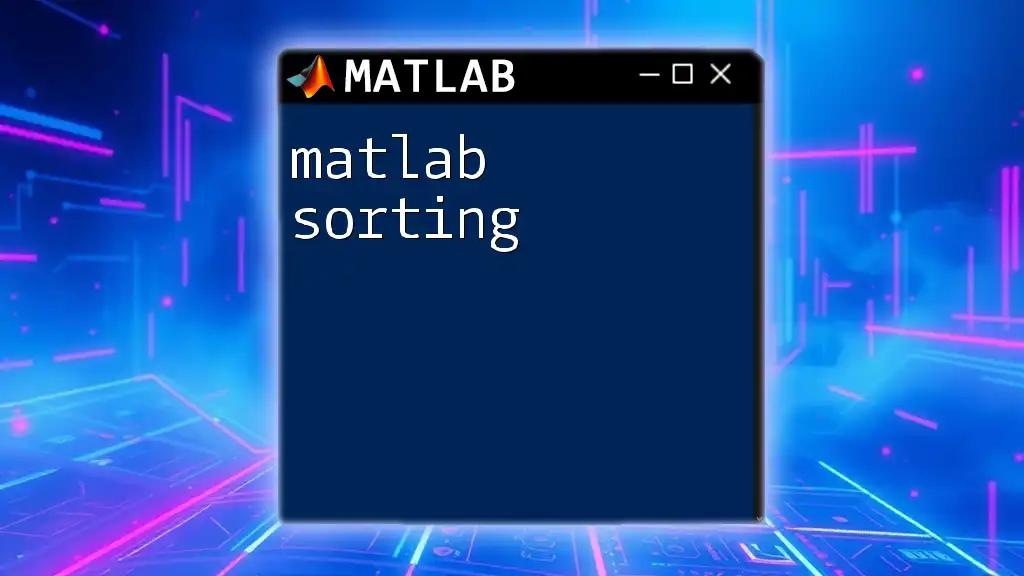
Best Practices for Using If Statements
When utilizing MATLAB for if, it’s essential to adhere to best practices to ensure your code remains readable and maintainable:
-
Keep If Statements Clear and Readable: Code should communicate its intent easily. Avoid convoluted expressions that can confuse readers.
-
Minimize Nested Structures: While they can be useful, over-nesting can lead to complications. Aim for a maximum of two or three levels of nesting if possible.
-
Comment for Clarity: Adequate commenting can significantly enhance the understandability of your code. Ever complex logic should be accompanied by explanations to aid future readers (including yourself).

Debugging Common Errors in If Statements
Even seasoned programmers occasionally run into problems with if statements. The common pitfalls often include logical errors (wrong conditions), syntax errors (missed keywords or parentheses), and runtime errors (trying to evaluate undefined variables).
For instance, if you mistakenly write:
if x = 10 % Incorrect usage
This will result in a syntax error, as a single equals `=` is an assignment operator, while `==` is required for comparison.
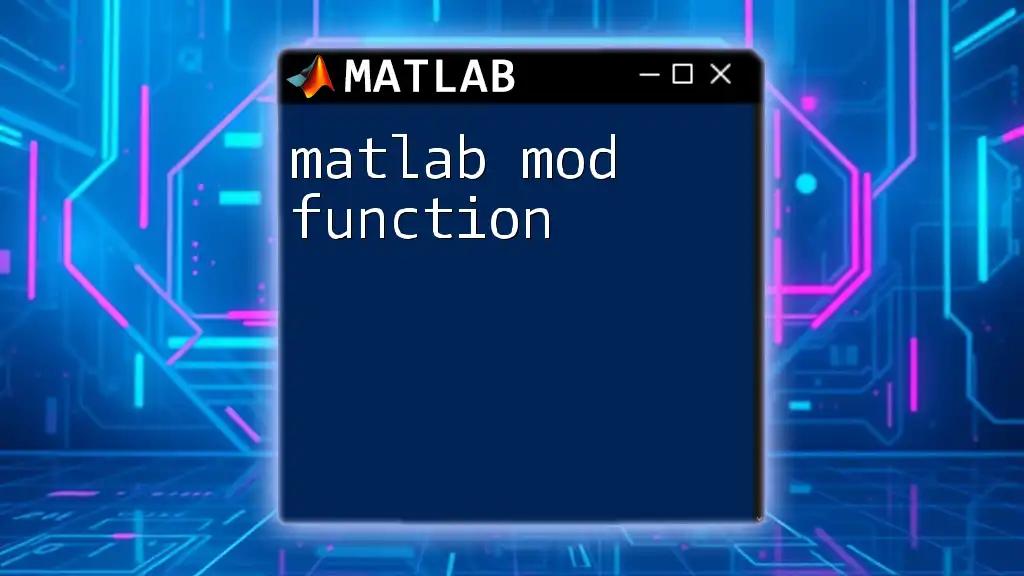
Conclusion
Understanding how to effectively implement if statements is fundamental to mastering MATLAB programming. This powerful tool for control flow can enhance your scripts' functionality and clarity. By practicing the techniques and examples presented, you'll be well-equipped to tackle a variety of challenges using MATLAB for if statements.
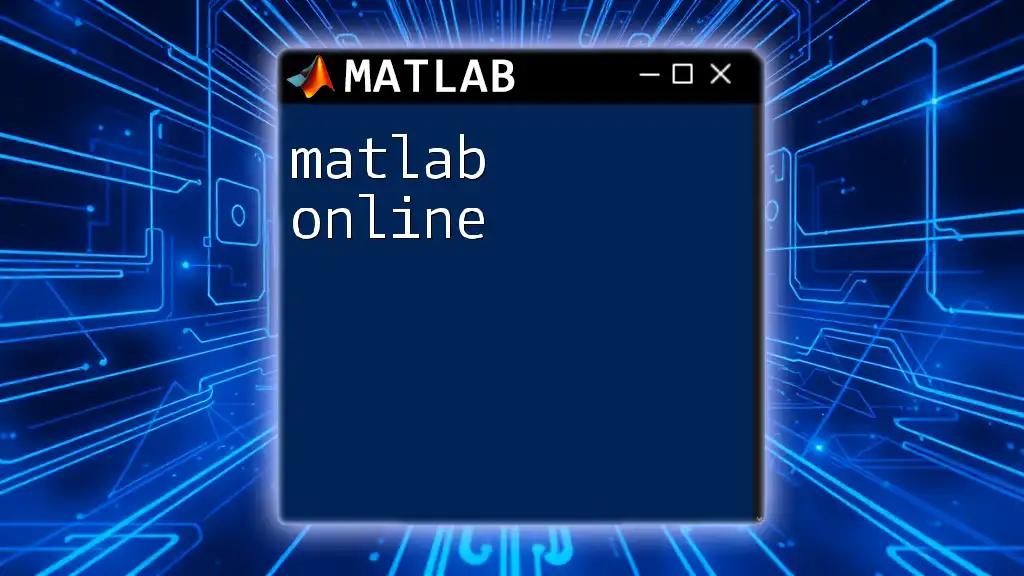
Additional Resources
For those looking to dive deeper into MATLAB for if, consider exploring additional resources such as books, online tutorials, and community forums specific to MATLAB. Engaging with other learners and seasoned programmers can provide invaluable insights and foster your growth in MATLAB proficiency.
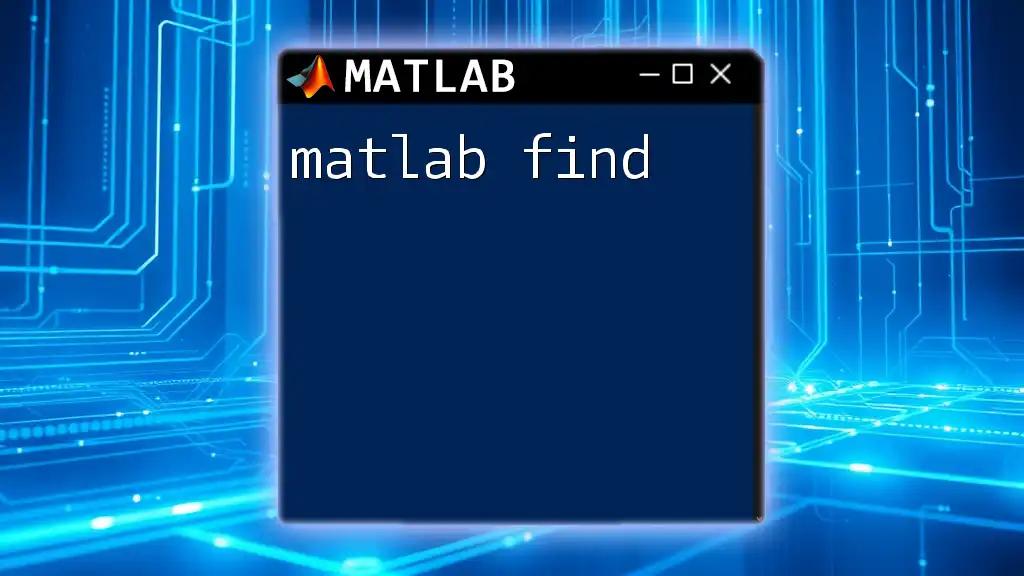
FAQs about MATLAB If Statements
What is the difference between if and switch statements?
An `if` statement evaluates conditions in sequence, whereas a `switch` statement allows you to compare a variable against multiple possible values, making it suitable for discrete value comparisons.
Can if statements be used with arrays?
Yes, if statements can be applied to arrays, though MATLAB will evaluate the condition for each element, behaving similarly to element-wise logical operations.
What is the maximum depth of nested if statements allowed?
Although MATLAB technically doesn't limit the number of nested if statements, excessive nesting can lead to code that is difficult to maintain and understand. Strive for clarity over complexity.