The MATLAB Image Processing Toolbox provides a comprehensive set of functions and tools that enable users to perform advanced image processing, analysis, and visualization tasks efficiently.
Here's a simple code snippet that demonstrates how to read an image, convert it to grayscale, and display both the original and the grayscale images:
% Read the image
originalImage = imread('image.jpg');
% Convert to grayscale
grayImage = rgb2gray(originalImage);
% Display the original and grayscale images
subplot(1, 2, 1), imshow(originalImage), title('Original Image');
subplot(1, 2, 2), imshow(grayImage), title('Grayscale Image');
What is the MATLAB Image Processing Toolbox?
The MATLAB Image Processing Toolbox is a powerful suite of functions and tools designed for processing and analyzing images. It extends MATLAB's capabilities, enabling users to perform a wide variety of image manipulation tasks. This toolbox is essential for developers, researchers, and engineers who work with graphics, segmentation algorithms, and image analysis techniques.
Key features include functionality for:
- Reading, writing, and displaying images.
- Transforming images through resizing, rotating, and filtering.
- Enhancing images using techniques like histogram equalization and edge detection.
- Analyzing image characteristics and properties.
The applications of the Image Processing Toolbox span numerous fields such as medical imaging, robotics, computer vision, and digital photography.
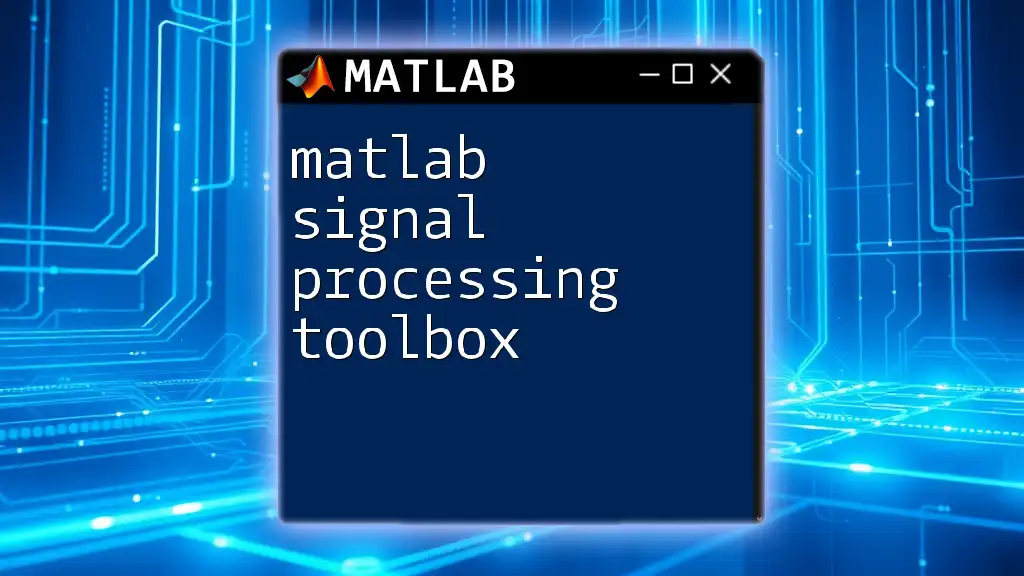
Getting Started with the Image Processing Toolbox
Installing the Toolbox
Before diving into image processing, ensure that your MATLAB environment has the Image Processing Toolbox installed. You can check this by executing the following command in the Command Window:
ver
This command lists all installed toolboxes. If the toolbox is missing, you can install it through the Add-Ons manager in MATLAB.
Overview of the MATLAB Environment
Familiarizing yourself with the MATLAB interface is crucial. The main components include:
- Command Window: Where you can test commands and functions interactively.
- Workspace: Displays all variables in memory, facilitating easy access and organization.
- Editor: Used for writing and editing MATLAB scripts and functions.
Additionally, MATLAB’s Help Documentation is invaluable, offering extensive examples and explanations that can guide you as you utilize the toolbox.
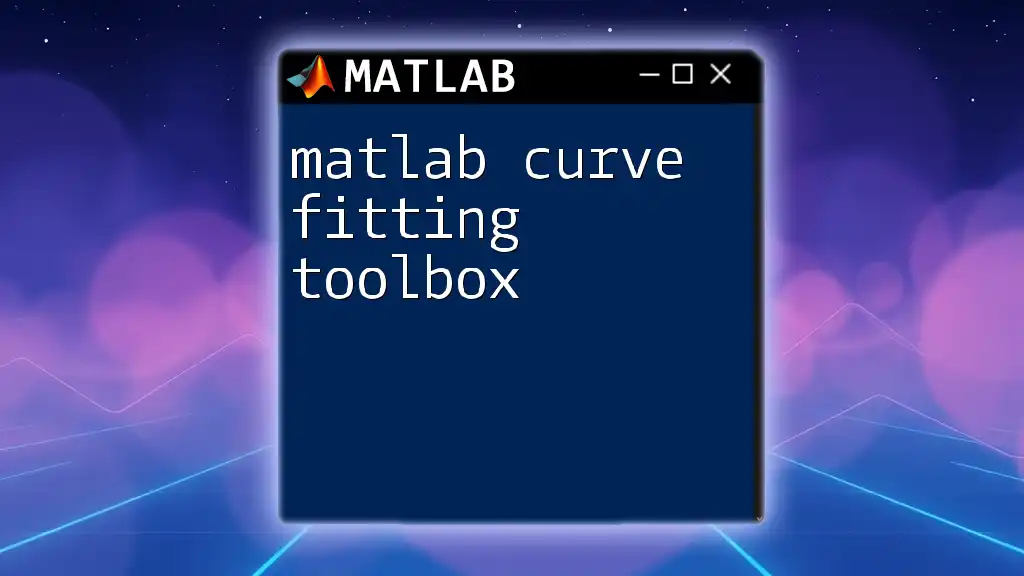
Core Functions of the Image Processing Toolbox
Reading and Displaying Images
The first step in any image processing task is to load the image into MATLAB. Use the `imread` function to read image files:
img = imread('image.jpg');
Once the image is read, you can display it using `imshow`:
imshow(img);
This will show the image in a new figure window. It’s important to remember that images in MATLAB are typically represented as matrices, with varying data types depending on the image format.
Image Transformation
Image Resizing
One common transformation is resizing an image. The `imresize` function allows you to change the dimensions of your image. In the example below, the image is resized to 50% of its original size:
resizedImg = imresize(img, 0.5);
When resizing, it is essential to select an interpolation method. MATLAB offers several, including nearest neighbor, bilinear, and bicubic, each impacting the quality and computational efficiency.
Image Rotation
Rotating images can also be done easily with the `imrotate` function. This function allows you to specify the angle of rotation:
rotatedImg = imrotate(img, 45);
This rotates the image 45 degrees counterclockwise. When rotating, take into consideration that this may change the image size and create empty areas that need to be filled.
Image Enhancement Techniques
Contrast Enhancement
To improve the visibility of features within an image, we often utilize contrast enhancement. The `histeq` function performs histogram equalization:
enhancedImg = histeq(img);
This technique enhances the contrast by effectively distributing the intensity values across the range of available values.
Noise Reduction
Images can often contain noise, which can obscure essential details. The `imfilter` function can apply a filter to smooth out noise:
filter = fspecial('average', [3, 3]);
noiseReducedImg = imfilter(img, filter);
In this example, an averaging filter is applied, reducing random noise while still preserving edges.
Image Analysis
Edge Detection
Edge detection is a critical technique used to identify the boundaries within images. The `edge` function leverages methods like Canny edge detection:
edges = edge(img, 'Canny');
This function can reveal the outlines of objects, making it invaluable for segmentation tasks.
Object Detection and Measurement
The `regionprops` function allows you to measure properties of detected objects in binary images. Below is a brief implementation:
stats = regionprops('table', labelImage, 'Area', 'Centroid');
This function yields a table containing the area and centroid for each detected object, which can be crucial for analysis in various applications, such as counting cells in biological images.
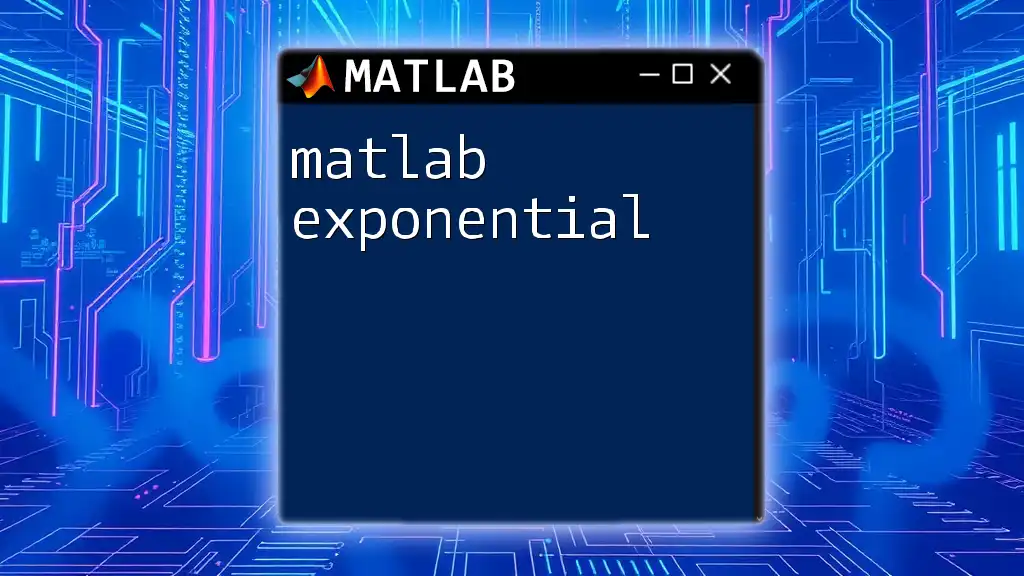
Advanced Image Processing Techniques
Image Segmentation
Image segmentation involves partitioning an image into multiple segments (sub-regions) to simplify or change the representation of an image. Techniques vary, including:
-
Thresholding: A straightforward method often used for separating objects from the background. For example:
bw = imbinarize(img);
-
K-means Clustering: Groups pixels into clusters based on color similarity.
-
Watershed Segmentation: A more complex approach that considers the topology of the image, effectively identifying regions based on gradient information.
Feature Extraction
Feature extraction is pivotal in tasks like image recognition and classification. The `extractHOGFeatures` function extracts Histogram of Oriented Gradients, which are useful descriptors for objects in images:
[features, visualization] = extractHOGFeatures(img);
Using these features, you can train models for recognizing objects based on their patterns and shapes.
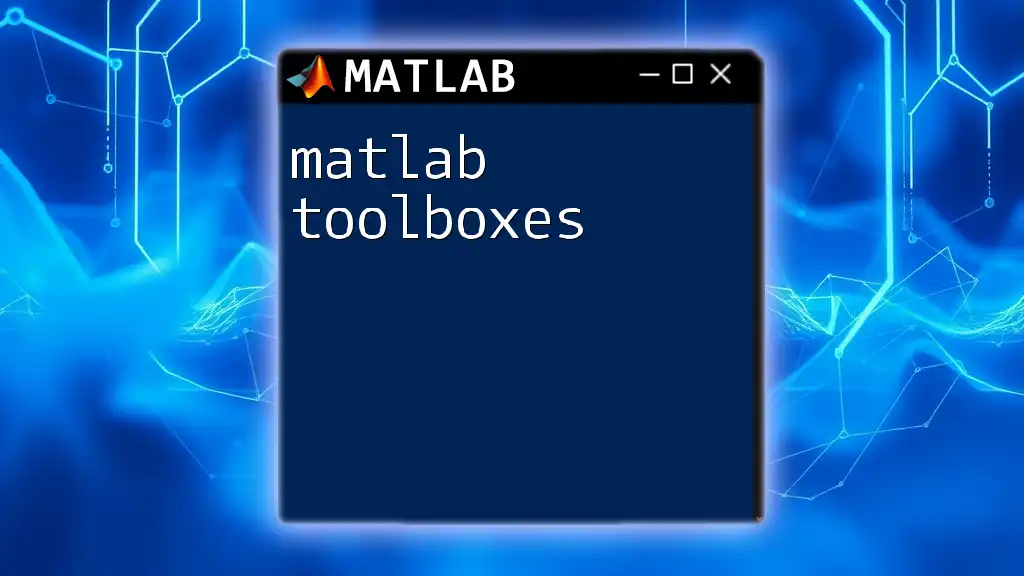
Practical Examples
Example 1: Image Enhancement Workflow
To demonstrate the use of the MATLAB Image Processing Toolbox, consider a complete workflow:
-
Read an Image:
img = imread('image.jpg');
-
Enhance Contrast:
enhancedImg = histeq(img);
-
Display Results:
imshow(enhancedImg);
Example 2: Object Detection Application
Applying segmentation and feature extraction can result in object detection. Here's how:
-
Read the Image:
img = imread('image.jpg');
-
Segment the Image:
bw = imbinarize(img);
-
Label Connected Components:
labelImage = bwlabel(bw);
-
Measure Properties:
stats = regionprops(labelImage, 'BoundingBox', 'Area');
-
Display Detected Objects:
imshow(img); hold on; for k = 1:numel(stats) rectangle('Position', stats(k).BoundingBox, 'EdgeColor', 'r', 'LineWidth', 1); end hold off;
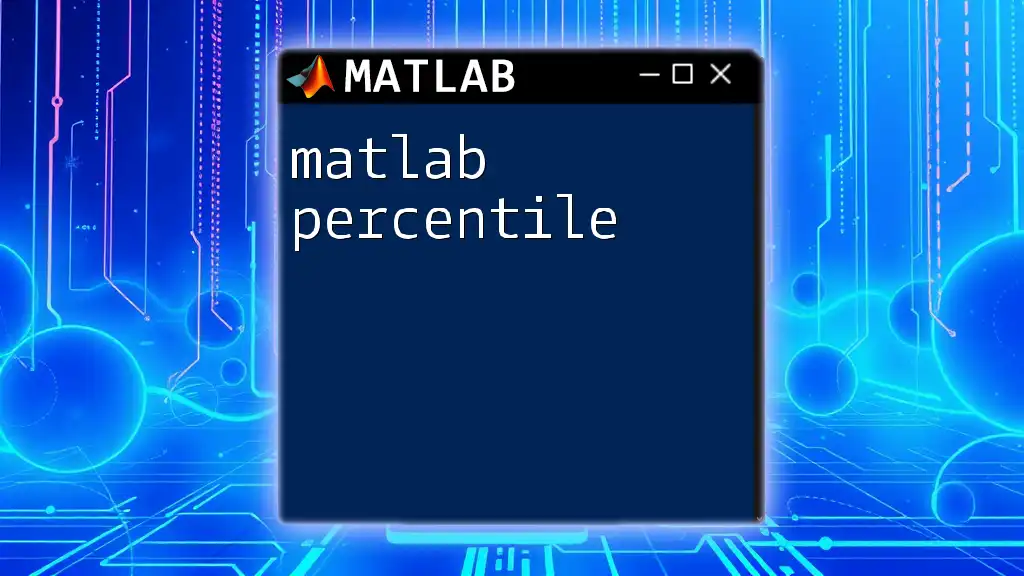
Conclusion
The MATLAB Image Processing Toolbox is an indispensable asset for anyone involved in image processing tasks. With its extensive array of functions and tools, users can manipulate, analyze, and enhance images effectively. As you explore this toolbox, don’t hesitate to experiment with different functions and techniques. Make the most of the resources available to you, and take your image processing skills to the next level!
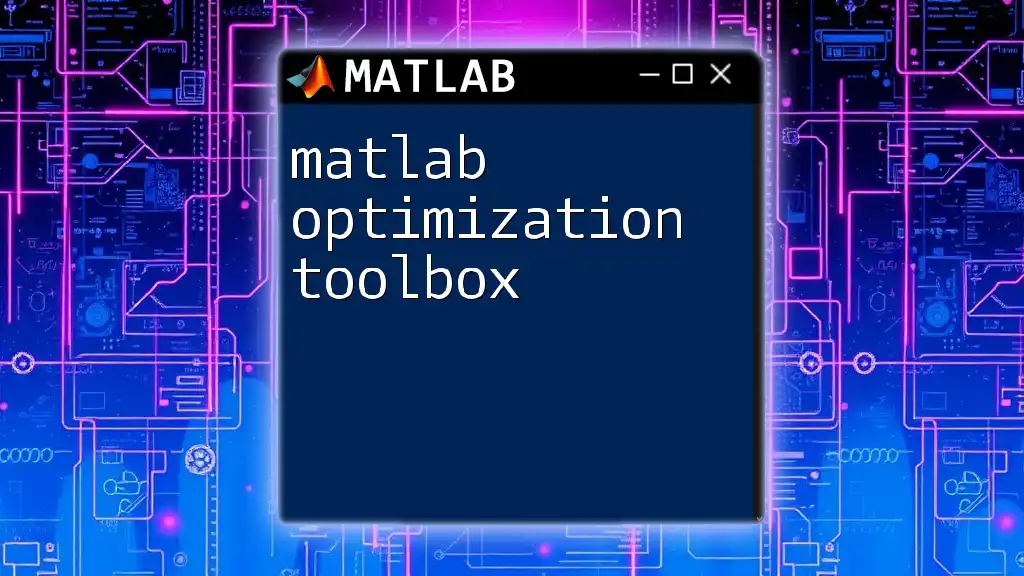
Additional Resources
For further learning and exploration, consider referring to the official MATLAB documentation for the Image Processing Toolbox. You can also engage with online courses and community forums to deepen your understanding and connect with other learners.