In this MATLAB language tutorial, we will quickly demonstrate how to create and plot a simple sine wave using basic MATLAB commands.
x = 0:0.01:2*pi; % Generate an array from 0 to 2π
y = sin(x); % Compute the sine of each x value
plot(x, y); % Plot the sine wave
xlabel('x'); % Label x-axis
ylabel('sin(x)'); % Label y-axis
title('Sine Wave'); % Title of the plot
Introduction to MATLAB
What is MATLAB?
MATLAB, short for Matrix Laboratory, is a powerful programming and numerical computing environment used extensively in various fields such as engineering, data science, finance, and academic research. It excels in matrix computations and offers functional tools for algorithm development, data analysis, and visualization.
Benefits of Using MATLAB
Learning MATLAB offers multiple advantages. Its user-friendly interface simplifies complex numerical computations, and the vast libraries help users perform advanced data analysis and visualization without extensive programming knowledge. Additionally, a large community and extensive documentation are readily available to support your learning journey.
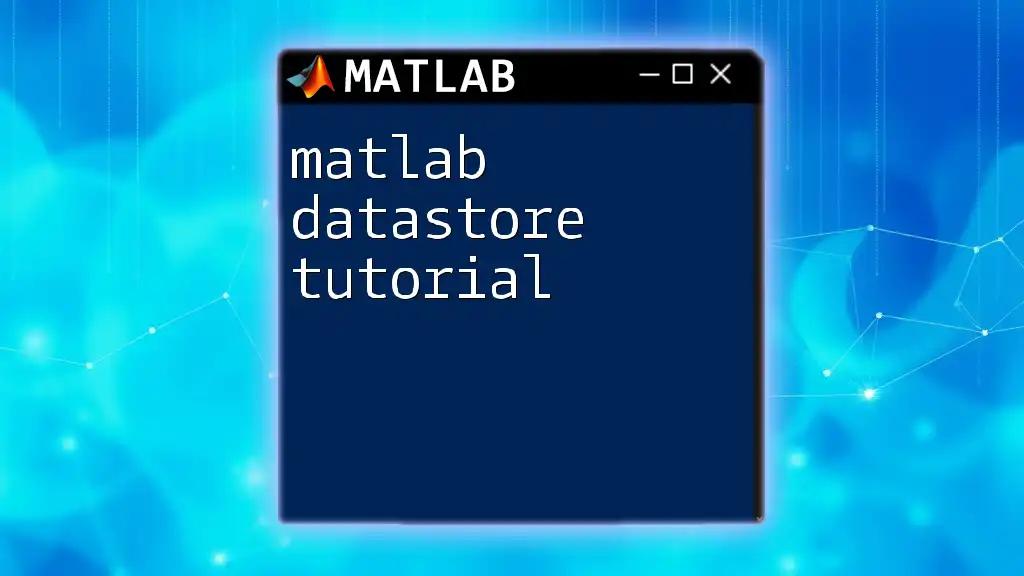
Getting Started with MATLAB
Installing MATLAB
To start using MATLAB, you need to download and install the software. The installation process typically involves creating a MathWorks account, choosing your operating system, and following the installation prompts. After installation, you'll need to activate your MATLAB license to start using the software.
Understanding the MATLAB Interface
Once installed, familiarize yourself with the MATLAB interface. The main components include:
- Command Window: Where you can enter commands and see the results immediately.
- Workspace: Displays the variables you have created during your session.
- Editor: For writing, saving, and editing scripts and functions.
You can customize the workspace layout to enhance your productivity, allowing you to arrange elements according to your preferences.
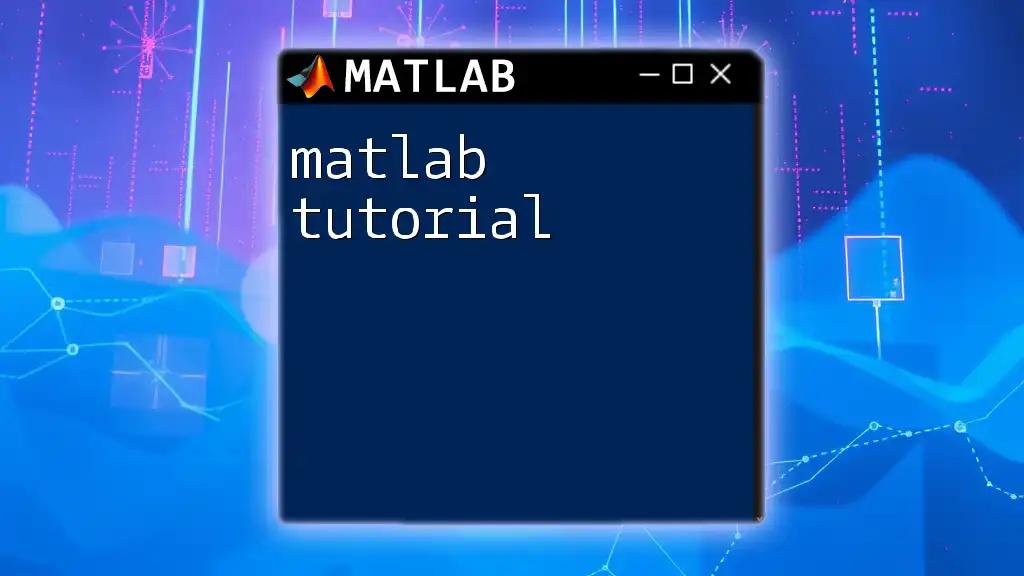
Basic MATLAB Commands
MATLAB Syntax Overview
MATLAB has a specific syntax you need to adhere to. Understanding this structure is crucial; remember that MATLAB is case-sensitive and treats commands as distinct based on capitalization. Also, special characters such as commas and semicolons play important roles in syntax.
Variables and Data Types
Creating variables in MATLAB is straightforward. You simply assign a value to a variable name. For example:
x = 10; % Integer
y = 20.5; % Floating point
MATLAB supports various data types, including scalars, vectors, and matrices. Understanding these data types is vital for effective computing.
Basic Math Operations
MATLAB allows you to perform common mathematical operations easily. Here are examples of basic operations:
a = 5 + 7; % Addition
b = 10 - 3; % Subtraction
c = 4 * 2; % Multiplication
d = 8 / 2; % Division
These operations follow a familiar mathematical syntax, making it easier for those with basic math skills to adapt.
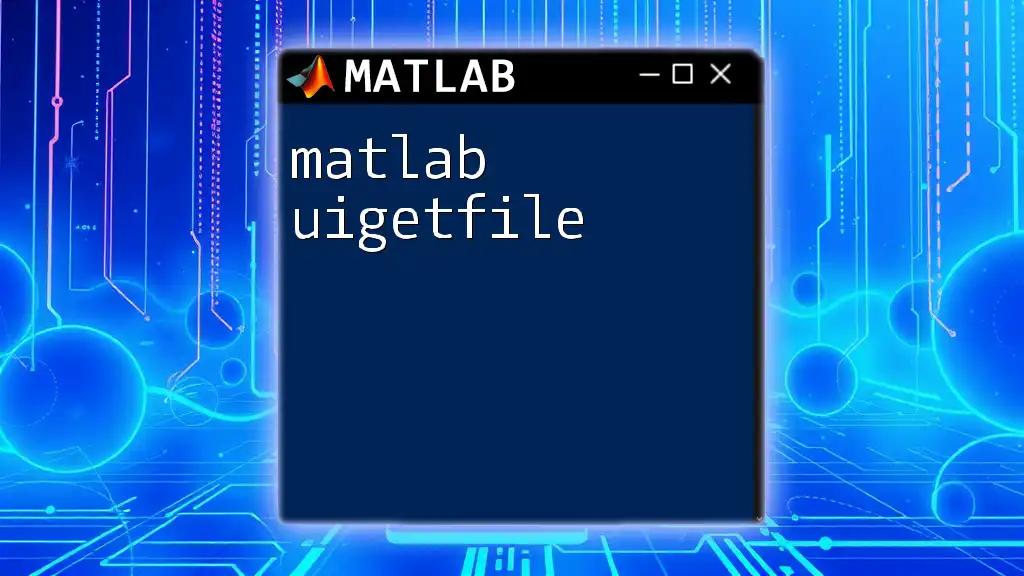
Advanced MATLAB Commands
Control Flow Statements
Control flow statements, such as conditional statements and loops, enable you to control the execution of your code based on certain conditions. For example, consider using an if statement:
if a > b
disp('a is greater than b');
else
disp('b is greater than or equal to a');
end
This allows MATLAB to execute specific code depending on whether `a` is greater than `b`.
When working with repeated tasks, loops become essential. For instance, a simple for loop looks like this:
for i = 1:5
disp(i);
end
This loop will display the numbers 1 to 5 sequentially.
Functions in MATLAB
Functions are self-contained modules that can be called to perform specific tasks. Writing functions promotes code reusability. Here is how to create a simple custom function in MATLAB:
function result = addNumbers(num1, num2)
result = num1 + num2;
end
This function takes two inputs and returns their sum, demonstrating how you can encapsulate functionality for ease of use.
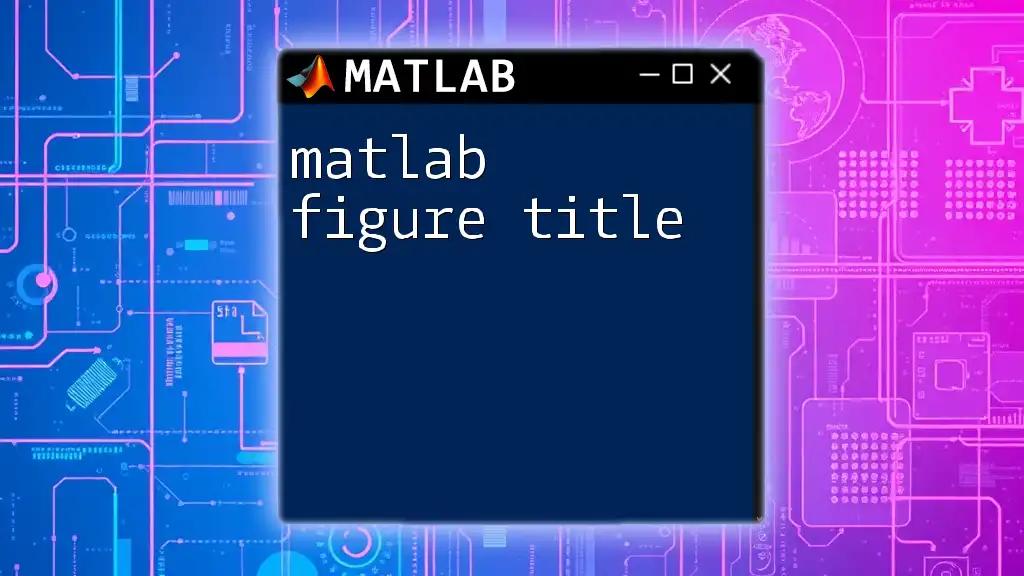
Working with Data in MATLAB
Importing and Exporting Data
MATLAB provides several methods for importing and exporting data. A common format for data files is CSV. Here’s how you can read a CSV file into MATLAB:
data = readtable('data.csv');
This command reads the contents of data.csv into a table format, allowing for easy manipulation and analysis.
Data Manipulation Techniques
Once data is imported, you can perform various operations such as sorting, filtering, and aggregating. Handling missing data is crucial, and MATLAB provides functions like `rmmissing()` to manage such scenarios. You can also convert data types using functions like `num2str()` or `str2double()` to ensure your analyses are accurate.
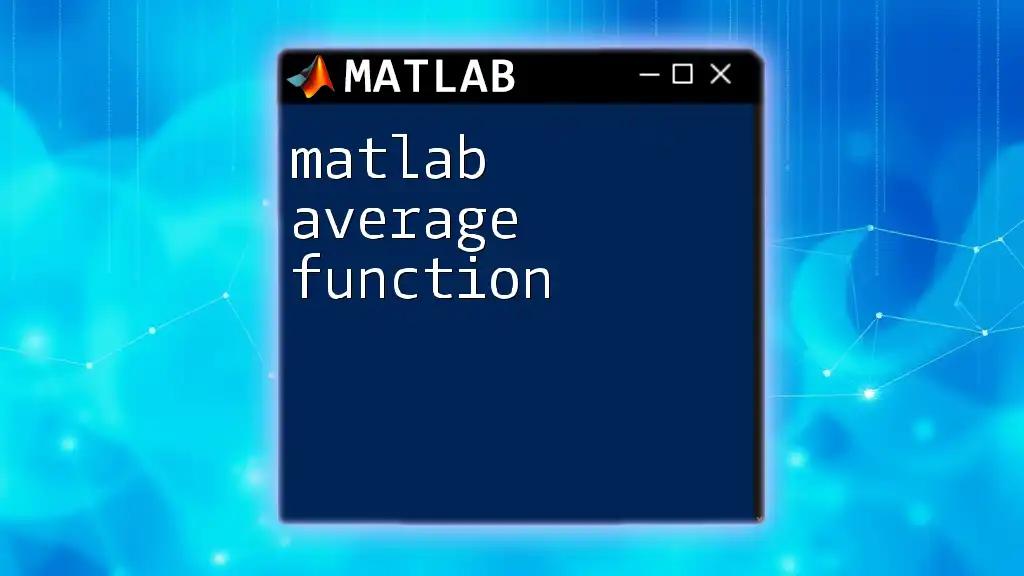
Visualizing Data
Plotting Functions
MATLAB's visualization capabilities are robust, making it easy to generate plots. You can create simple plots with the following code:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
title('Sine Function');
xlabel('x values');
ylabel('sin(x)');
This code will generate a plot of the sine function over the interval from 0 to 10.
Customizing Plots
To enhance the visual presentation of your plots, consider adding elements such as gridlines, legends, and annotations. Here’s an example enhancing the plot above:
grid on;
legend('sin(x)', 'Location', 'best');
By including these improvements, your plots become clearer and more informative to the audience.
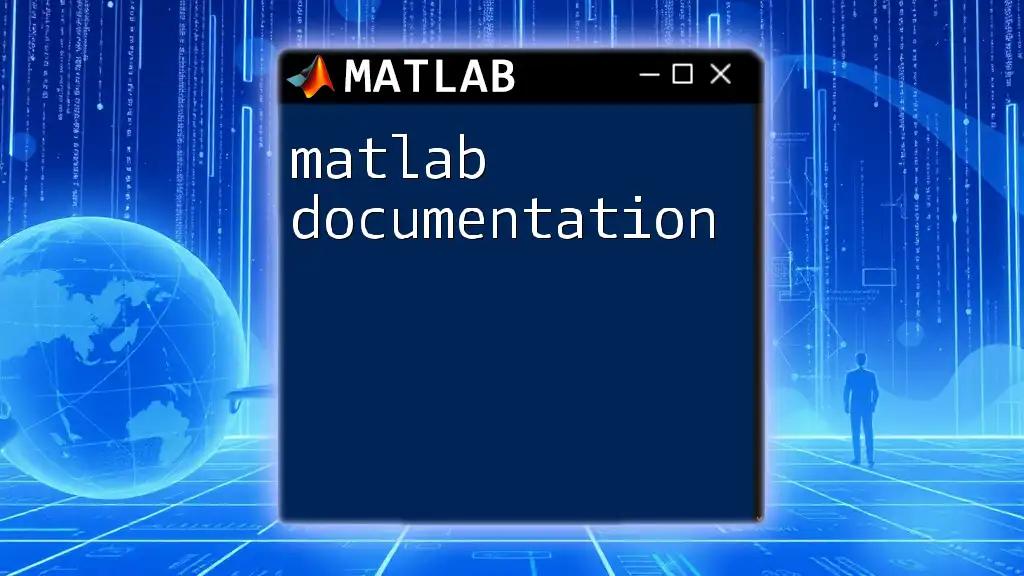
Debugging and Error Handling
Common Errors and Troubleshooting
As you write code, you'll encounter common errors like syntax errors or runtime errors. Understanding these errors and knowing how to address them is essential for effective debugging.
Using Breakpoints and the Debugger
MATLAB has built-in debugging tools that make it easier to track and fix errors. You can set breakpoints in your code by clicking on the margins of the editor, allowing you to pause execution and inspect variable values to better understand where errors may be occurring.
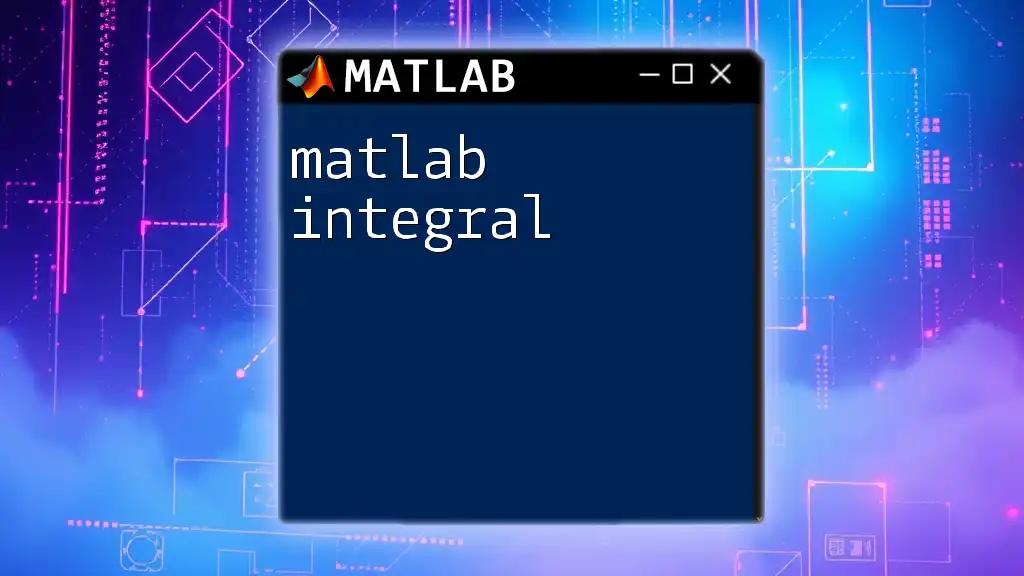
Conclusion
Recap of Key Concepts
This MATLAB language tutorial has covered fundamental concepts from installing MATLAB and understanding its interface to using basic and advanced commands, working with data, visualizing results, and troubleshooting errors.
Next Steps for Learning MATLAB
To continue your MATLAB learning journey, explore various online courses, resources, and community forums that provide further insights. The best way to solidify your MATLAB skills is through consistent practice and experimentation with the commands and techniques outlined in this tutorial.
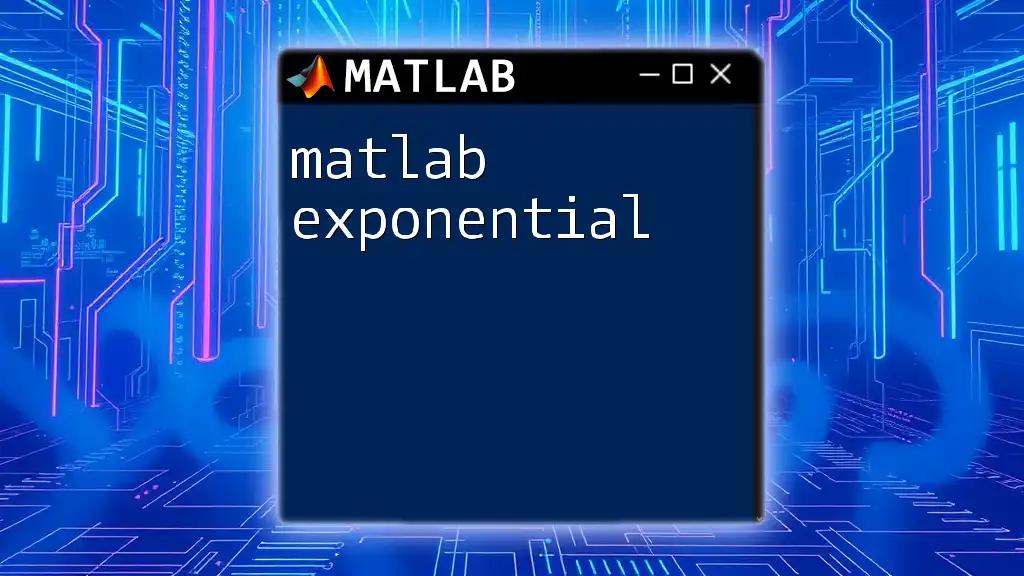
Additional Resources
Helpful MATLAB Toolboxes
MATLAB offers specialized toolboxes for diverse applications such as Signal Processing and Image Processing. Familiarizing yourself with these resources can open up new avenues for exploration and project development.
Online Communities and Forums
Engaging with online communities, forums, and user groups can facilitate knowledge sharing and provide solutions to problems you may encounter on your MATLAB journey. Don’t hesitate to seek assistance and contribute your findings.