In MATLAB, the "marker" property is used to specify the symbol that represents data points in a plot, allowing for customization of how data is visually represented.
x = 0:0.1:10;
y = sin(x);
plot(x, y, 'o', 'MarkerFaceColor', 'r'); % 'o' sets the marker to a circle and 'MarkerFaceColor' fills it with red
Understanding MATLAB Markers
What Are Markers?
Markers in MATLAB are graphical symbols used to represent data points in plots. They play a crucial role in enhancing the visibility of individual data values against a continuum, making it easier for users to interpret and analyze datasets. Markers provide clarity in visualizations, which is essential for tasks ranging from data analysis to presentation.
Types of Markers Available in MATLAB
When it comes to visualizing data using markers, MATLAB offers a variety of built-in options, including:
- Circle (`'o'`): Commonly used for scatter plots.
- Square (`'s'`): Provides a different perspective on data without overlapping points.
- Diamond (`'d'`): Good for distinguishing data points in closely packed visualizations.
- Plus (`'+'`) and Cross (`'x'`): Useful for indicating specific data features.
Additionally, MATLAB allows users to customize markers to fit the context of their data visualization needs.
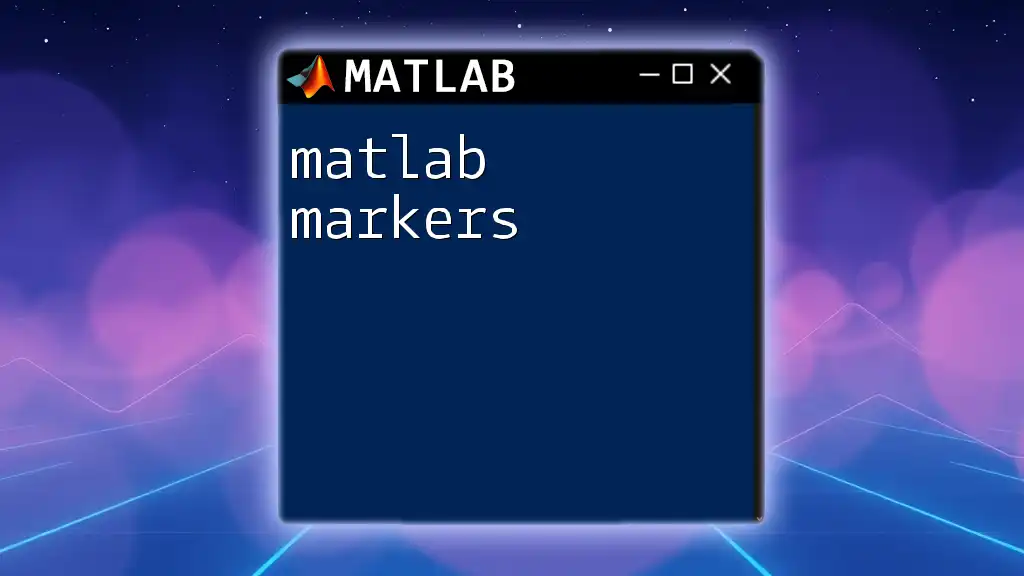
Basic Marker Syntax
Essential Syntax for Adding Markers
To add markers to your plots, you can use the `plot` function in MATLAB. The basic syntax involves specifying the data and the marker type. Here's an example:
x = 1:10;
y = rand(1,10);
plot(x, y, 'o') % Example using circles as markers
This code creates a simple plot with circular markers representing the data points. The single quotes around `'o'` indicate to MATLAB that you want to use circle markers.
Marker Properties
Markers in MATLAB come with several customizable properties that can enhance your data visualization. Some of the key properties include:
- Marker Size: Controls the size of the marker.
- Marker Color: Sets the color of the marker edges.
- Marker Face Color: Fills the interior of the marker.
You can easily customize these properties by including them as parameters in your plotting commands. For instance:
plot(x, y, 'Marker', 's', 'MarkerSize', 10, 'MarkerEdgeColor', 'r', 'MarkerFaceColor', 'g')
In this example, the plot displays square markers with specified sizes and colors, providing a more vibrant visual representation of the data.
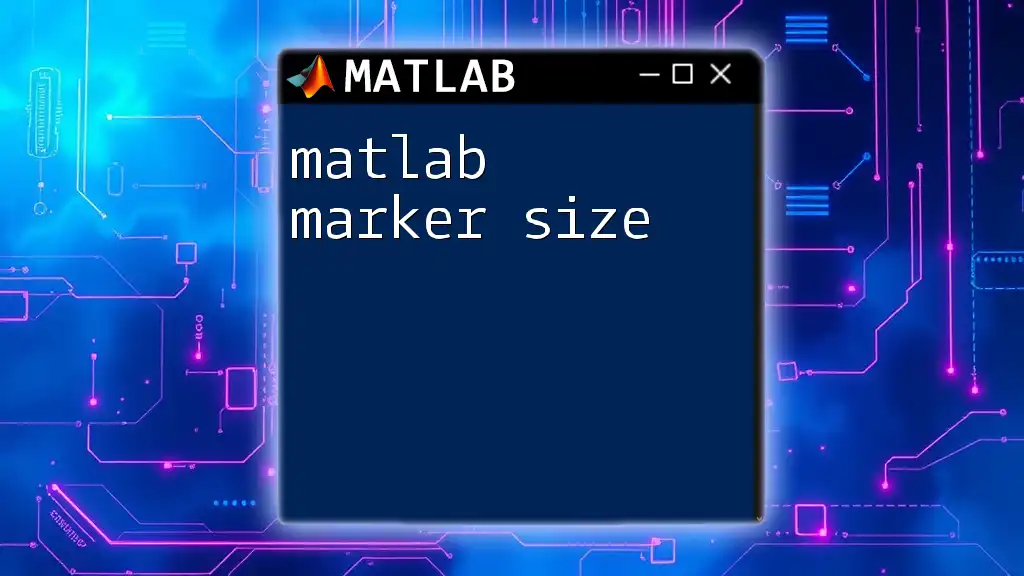
Customizing Markers
Changing Marker Size
In MATLAB, you can achieve dynamic visualizations by adjusting the size of your markers based on certain data points. For example, you might want to use varying sizes to represent different magnitudes of data:
sizes = [10, 20, 30, 40, 50];
scatter(x, y, sizes, 'filled')
The `scatter` function allows you to specify sizes for each data point, creating an informative and visually appealing plot.
Utilizing Different Colors in Markers
Different colors can significantly enhance the interpretability of your plots. MATLAB allows you to set the color of markers easily. For example:
scatter(x, y, 100, 'r', 'filled') % Red markers
Here, the `scatter` function creates a plot with red, filled circular markers, emphasizing data representation.
Combining Markers with Other Plot Elements
Combining markers with other plot types can improve overall readability. For example, you may wish to overlay markers on a line plot:
plot(x, y, 'b-'); % Line
hold on;
plot(x, y, 'ro'); % Red circles as markers
hold off;
In this snippet, blue lines represent the data trend, while red circles emphasize the individual data points, creating a clear and informative visualization.
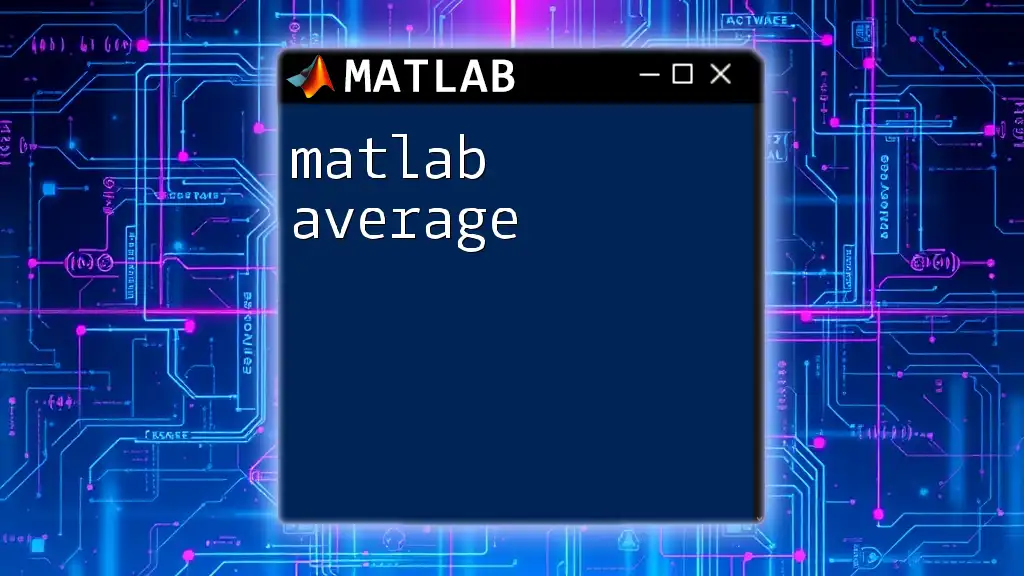
Advanced Marker Techniques
Using Marker Styles in 3D Plots
Markers can also be effectively used to enhance 3D visualizations. To create a 3D plot with custom markers:
z = rand(1,10); % Random z-coordinates
plot3(x, y, z, 'd'); % Diamond markers in 3D
Here, the `plot3` function allows you to visualize data points in three dimensions, using diamond-shaped markers for clarity.
Creating a Legend with Markers
Including a legend in your plots helps viewers understand what each marker represents. To create a legend including markers:
plot(x, y, 'o', 'DisplayName', 'Data Points');
legend show;
By assigning a `DisplayName`, you ensure that the legend accurately references the data points using markers, offering clarity to your plot.
Combining Markers with Annotations
Annotations can serve to highlight significant data points, providing additional context. You can annotate markers by adding textual descriptions:
text(x(5), y(5), 'Important Point', 'VerticalAlignment', 'bottom');
This line of code places a text label above a specific marker, drawing attention to particular data when necessary.
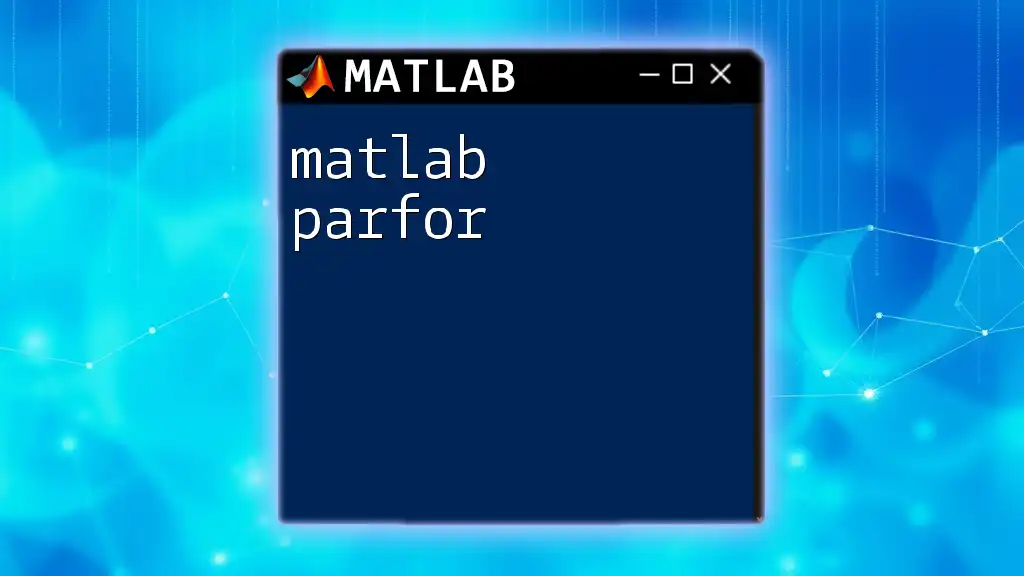
Practical Examples
Example One: Scatter Plot with Custom Markers
Let’s create a scatter plot using different marker types to impact data representation visually.
scatter(x, y, 100, 'b', 'filled','DisplayName','Data A');
hold on;
scatter(x, y + 0.5, 100, 'r', 'filled','DisplayName','Data B');
legend show;
hold off;
In this example, the blue markers represent one dataset while the red markers show another, clearly distinguishing between the two using color and position.
Example Two: Markers in Subplots
You can also utilize markers across multiple subplots for comparative analysis of different datasets. Here’s how:
figure;
subplot(2,1,1);
plot(x, y, 'o');
title('Plot 1: Data with Circles');
subplot(2,1,2);
plot(x, y + 0.5, 's');
title('Plot 2: Data with Squares');
Each subplot demonstrates a different marker type, facilitating easy comparison between datasets while keeping the visual structure orderly.
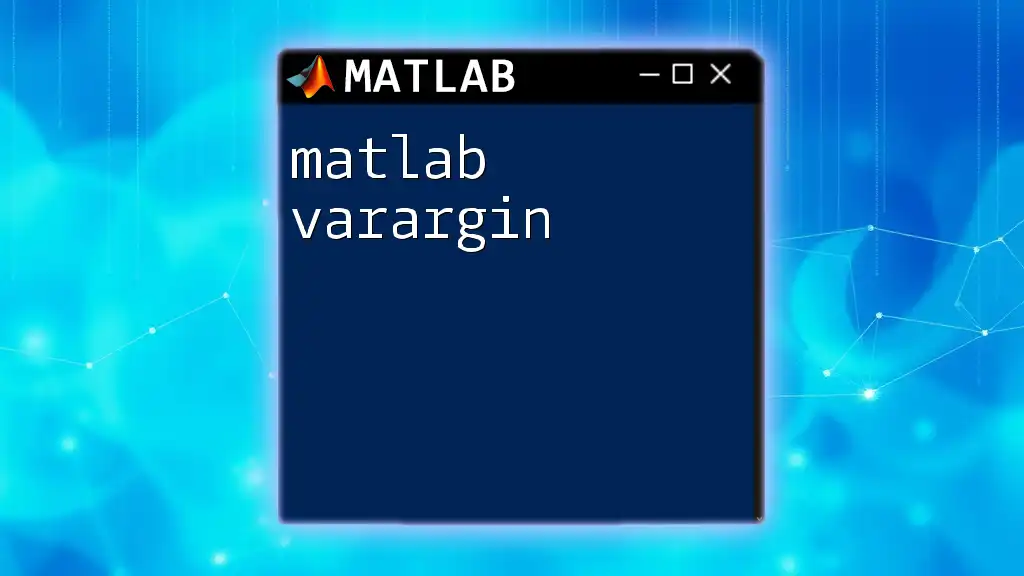
Conclusion
In summary, understanding MATLAB markers is fundamental to creating effective and informative plots. By leveraging various types of markers, adjusting their properties, and combining them with other plot elements, you can significantly enhance data visualizations. Experimenting with these techniques will deepen your data analysis and presentation skills.
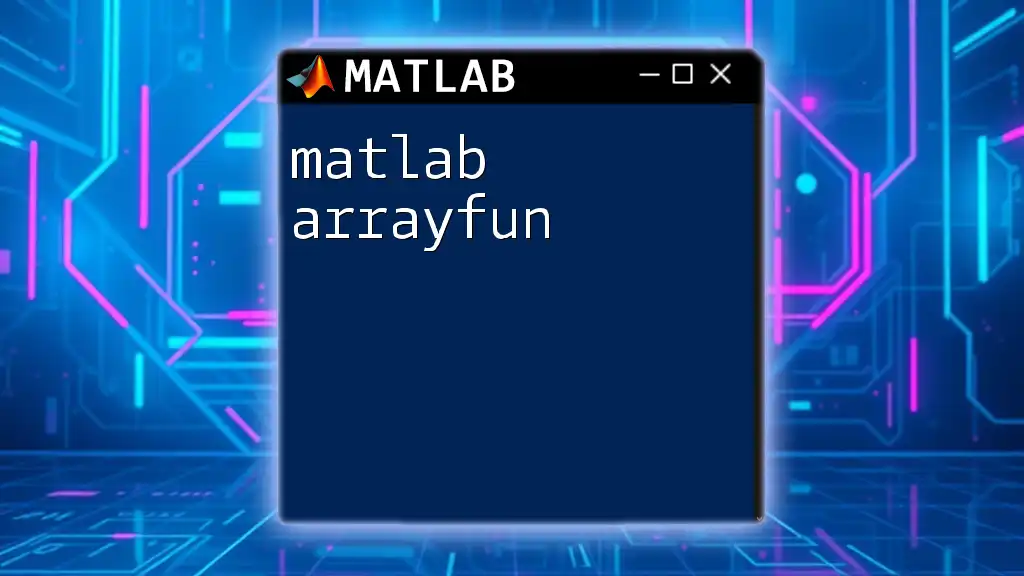
Call to Action
To delve further into the powerful capabilities of MATLAB, consider enrolling in our specialized MATLAB courses. Additionally, we encourage you to share your questions and insights in the comments section to foster community engagement. Happy plotting!