In MATLAB, the command `null` computes an orthonormal basis for the null space of a matrix, effectively finding vectors that result in the zero vector when the matrix is multiplied by them.
Here’s an example of how to use the `null` function in MATLAB:
A = [1 2; 2 4];
N = null(A);
In this example, `N` will contain a vector that spans the null space of matrix `A`.
Understanding MATLAB Null
What is Null in MATLAB?
In the context of MATLAB, null refers to the absence of a value or a placeholder indicating that no data exists. It is crucial for various programming tasks, including data manipulation and the creation of functions. Recognizing and managing null values is essential for effective data analysis and programming in MATLAB.
MATLAB's Representation of Null
MATLAB represents null values primarily as empty arrays, `NaN` (Not a Number), and in certain contexts, as `[]`. Understanding the differences between these representations is vital for accurate data handling.
-
Empty Arrays: An empty array is created by using `[]`. This indicates that no data or elements are present, which is especially important when initializing variables or preparing for data collection.
-
NaN Values: The `NaN` represents a value that is technically numeric but signifies that the actual numeric value is undefined or unrepresentable. Common scenarios where `NaN` arises include divisions by zero or missing data in datasets. Unlike zero, which has a defined value, `NaN` explicitly conveys that something is absent or incorrect.
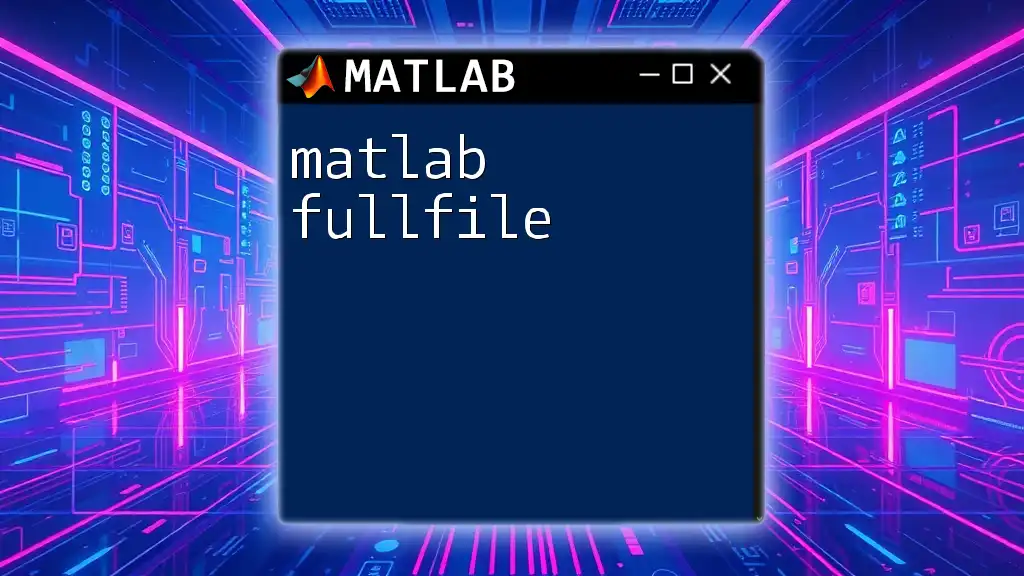
Working with MATLAB Null
Creating Null Arrays
Creating Empty Arrays
An empty array can be easily created in MATLAB, serving as a foundational element in many programming tasks.
emptyArray = [];
Using an empty array is beneficial when you do not yet know what data will be stored in a variable or when you’re initializing a container that will be filled later.
Using `nan` and `null`
The `nan()` function allows you to create an array filled with `NaN` values. This is particularly useful for initializing matrices for calculations where some data points may be missing.
nansArray = nan(3,2);
This creates a 3x2 matrix filled with `NaN` values. Using `NaN` ensures that calculations or analysis can proceed without assuming a default value like zero.
Checking for Null Values
Utilizing `isempty()` Function
To determine if an array is empty, MATLAB offers the `isempty()` function, which returns `true` if the target array has no elements.
if isempty(emptyArray)
disp('Array is empty.');
end
This function is critical for validating data before performing calculations or operations that require at least one data point.
Using `isnan()` Function
To check for `NaN` values in an array, the `isnan()` function comes into play. This function returns a logical array of the same size where each entry is `true` if the corresponding element is `NaN`.
if isnan(nansArray(1,1))
disp('Value is NaN.');
end
Employing the `isnan()` function assists in data validation when manipulating datasets, especially those collected from real-world observations.
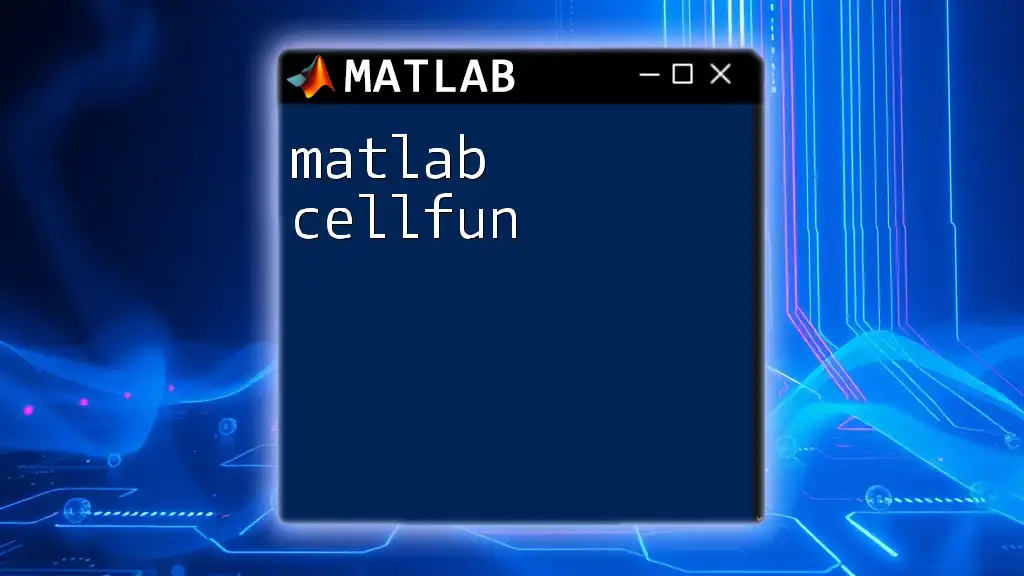
Practical Applications of Null
Handling Missing Data
Replacing Null Values
When processing datasets, you might encounter `NaN` values that represent missing data. These can be replaced with a specific value, such as zero, to allow further computation without interruption.
data = [1 2 NaN 4];
data(isnan(data)) = 0; % Replace NaN with 0
This technique ensures that your data remains useful by filling in gaps, which is a common practice in data preprocessing.
Removing Rows with Nulls
Sometimes, it’s essential to clean datasets by removing any rows containing `NaN` values. This approach can help improve data integrity during analysis.
data = [1 2 NaN; 3 4 5; NaN 6 7];
cleanData = data(~any(isnan(data), 2), :);
With this code, any row that contains `NaN` will be discarded, resulting in a cleaner dataset which supports better analytical outcomes.
Performance Considerations
While handling null values in MATLAB, it is essential to remain aware of the efficiency of operations involving these values. Performing operations over large datasets filled with nulls can introduce computational overhead.
To mitigate performance issues, structure your data efficiently. Instead of using a standard matrix with many `NaN` values spread across, consider preallocating your array to the exact size needed or using sparse matrices where applicable.
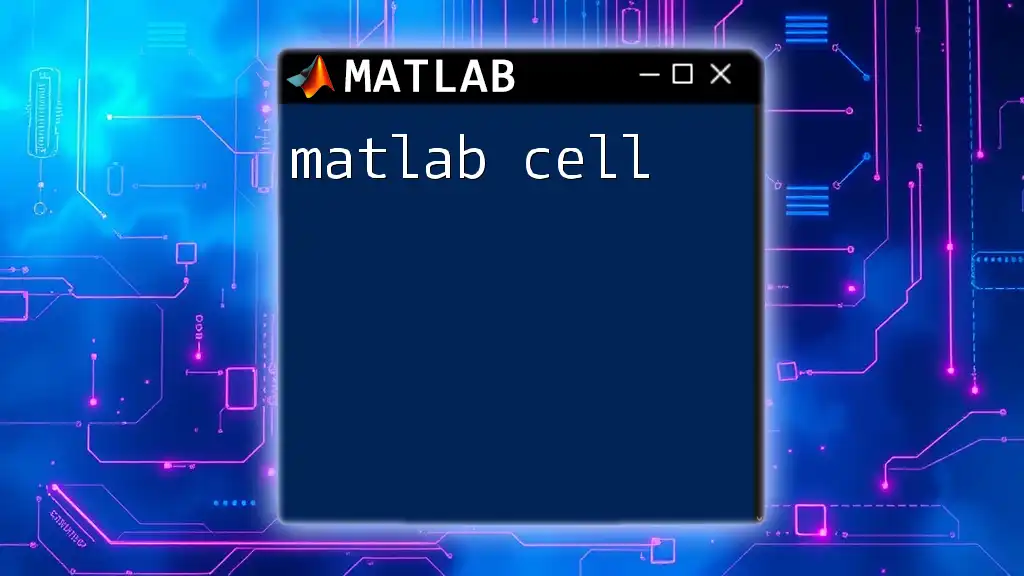
Advanced Concepts of Null in MATLAB
Null in Functions
When defining functions in MATLAB, you may find scenarios where you want to accept null arguments. This capability can make your functions more versatile and adaptable to various situations.
function myFunction(x)
if isempty(x)
disp('Input is null.');
end
end
In this example, the function checks if the input argument is null and takes corresponding action. This approach facilitates robust error handling within your functions.
Null versus Other Data Types
Understanding how null compares to other data types is beneficial for effective programming. While `null` represents the absence of data, other data types like numeric values, strings, or structures all convey distinct meanings in MATLAB.
Knowing when to use null over a specific data type depends on the context of your application. For example, when creating a matrix meant for subsequent calculations but currently has no applicable data, using `NaN` or an empty array is preferable to inserting arbitrary values.
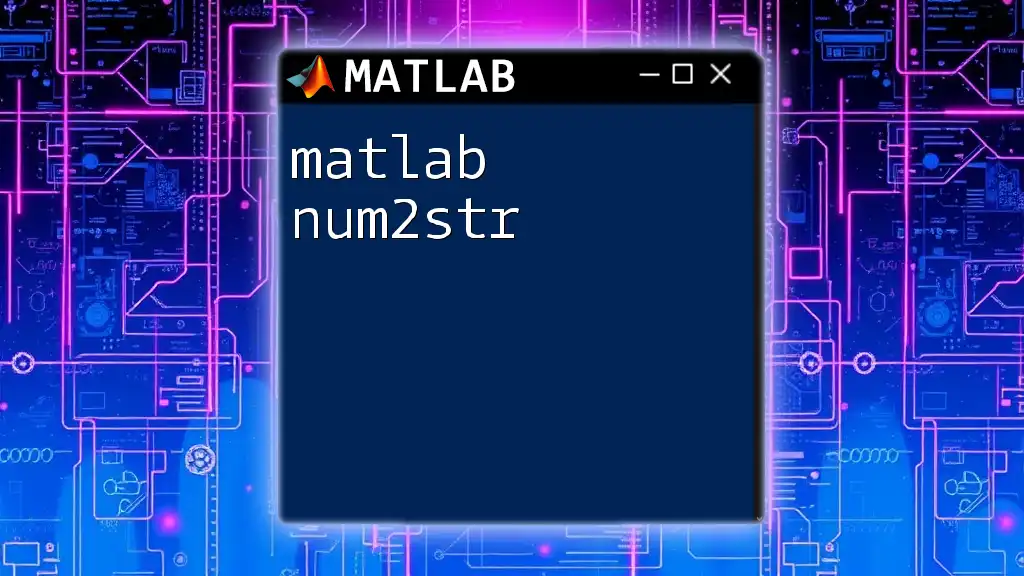
Conclusion
Understanding and effectively using `matlab null` is vital for robust data handling and analysis. By mastering concepts of null arrays, performing checks for emptiness and `NaN`, and navigating the intricacies of function arguments, you can enhance your MATLAB programming skills. Emphasize the importance of cleanliness in your datasets and utilize nulls thoughtfully to ensure that your analyses yield the most meaningful results.
Further Learning Resources
For those eager to expand their knowledge on MATLAB null and related topics, consider consulting recommended books, online courses, or video tutorials. Additionally, refer to the official MATLAB documentation for comprehensive guidance and best practices concerning the use of null in your programming journey.