In this post, we explore the nuances between "MATLAB" as a programming language and its various applications, highlighting key commands to enhance your proficiency in using MATLAB effectively.
Here’s a simple example demonstrating how to create a 2D plot in MATLAB:
x = 0:0.1:10; % Create array from 0 to 10 with 0.1 increments
y = sin(x); % Compute sine of each x value
plot(x, y); % Plot the graph of y = sin(x)
title('Sine Wave'); % Set the title of the plot
xlabel('X-axis'); % Label for x-axis
ylabel('Y-axis'); % Label for y-axis
Understanding the Basics of MATLAB
What is MATLAB?
MATLAB is a high-performance language for technical computing, known for its versatility in mathematical modeling, data analysis, algorithm development, and visualization. It allows users to manipulate matrices easily, implement algorithms, and create user interfaces. Originating from the need to provide easy access to matrix software, MATLAB has evolved into a powerful platform used in various fields, including engineering, finance, biology, and data science.
Case Sensitivity in MATLAB
One fundamental aspect of programming in MATLAB is its case sensitivity. While both MATLAB and matlab refer to the same programming language, their case can have varying implications in other programming contexts. In MATLAB, commands are not case-sensitive, meaning that you can use uppercase or lowercase letters interchangeably when writing code. However, following a consistent naming convention is crucial for maintaining code clarity and readability. For example, variable names `myVariable`, `MyVariable`, and `MYVARIABLE` are treated as distinct identifiers. Being diligent in your approach will help others (and future you!) better understand your code.
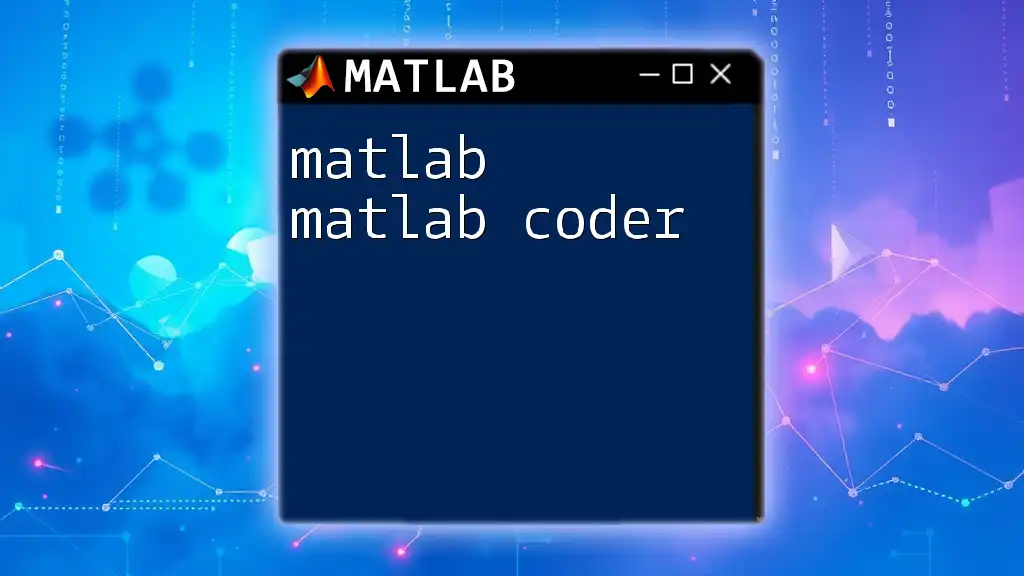
Key Features of MATLAB
Mathematics and Computation
MATLAB excels in mathematical computation thanks to its comprehensive built-in functions. It allows users to perform complex calculations effortlessly.
For instance, consider the need to compute the hypotenuse of a right triangle:
x = 3; % length of the first side
y = 4; % length of the second side
z = sqrt(x^2 + y^2); % Calculate the hypotenuse using Pythagorean theorem
disp(z); % Display the result
In this code snippet, we calculate the hypotenuse and display it. The simplicity of computing results using built-in functions is one of MATLAB’s major advantages.
Data Analysis and Visualization
MATLAB provides robust tools for data analysis and visualization, making it an ideal choice for interpreting complex datasets. Users can quickly plot graphs and visual representations of data, facilitating a clearer understanding of trends and patterns.
Here's an example of how to plot a simple sine wave:
x = 0:0.1:10; % Create a range of values from 0 to 10, incremented by 0.1
y = sin(x); % Calculate the sine of each x value
plot(x, y); % Create a 2D plot of x vs. y
title('Sine Wave'); % Title of the plot
xlabel('X-axis'); % Label for the x-axis
ylabel('Y-axis'); % Label for the y-axis
This example demonstrates how straightforward it is to visualize mathematical functions in MATLAB. With a few commands, users can generate dynamic visuals that help convey their data's story effectively.
Simulink Integration
Another significant feature of MATLAB is its integration with Simulink, a graphical programming environment for modeling, simulating, and analyzing dynamic systems. This integration allows users to design and test complex systems through a block diagram approach, facilitating faster prototyping and analysis. For engineers, this means they can work seamlessly between MATLAB scripts and Simulink models, leveraging the strengths of both environments.
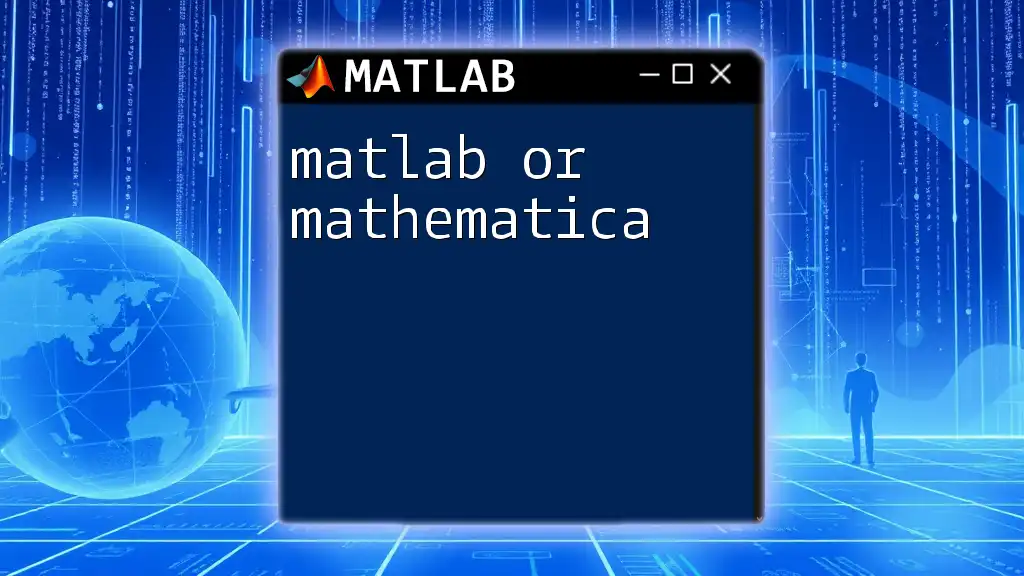
Markdown in MATLAB
Using Markdown for Documentation
In addition to programming, documenting your work using Markdown can enhance clarity when sharing your scripts. Markdown enables you to create structured, readable text that can easily accompany your MATLAB code on platforms like GitHub, within MATLAB itself, or other educational settings.
Writing Comments and Annotations in MATLAB
Comments are critical for crafting code that others can easily understand. By using `%` to begin comments, you can provide context and explanations without affecting the execution of your script.
For example:
% This script calculates the area of a circle
radius = 5; % Define the radius of the circle
area = pi * (radius^2); % Calculate the area using the formula
disp(area); % Display the calculated area
This code snippet illustrates how effective commenting can enhance the readability of your code, helping others (and yourself in the future) understand the purpose and function of each line.
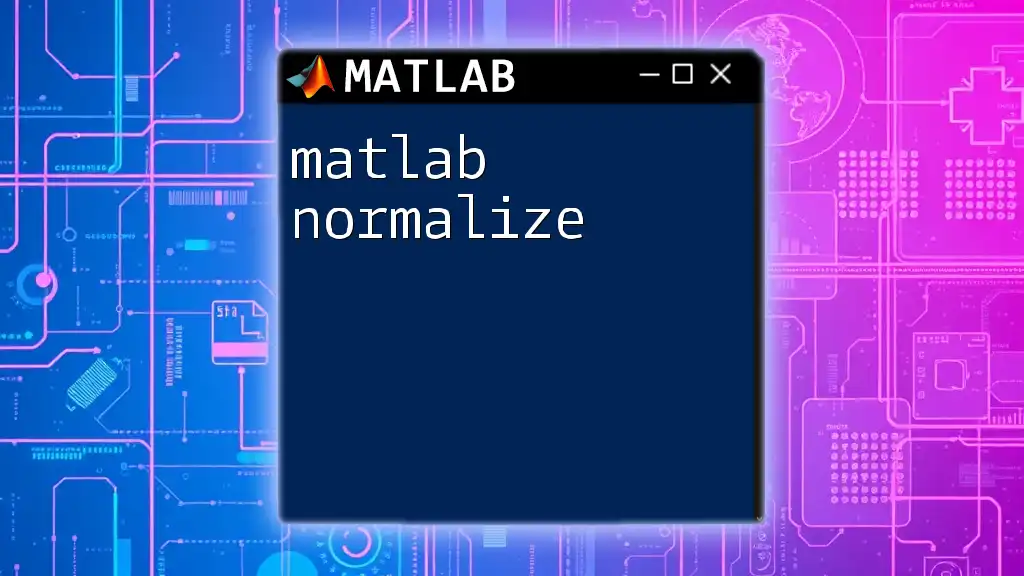
Common Commands - A Quick Reference
Basic Commands
MATLAB is built around matrix operations, and creating matrices is one of the foundational skills you will need. Whether you're initializing matrices filled with zeros, ones, or even constructing them manually, commands like `zeros`, `ones`, and array notation are essential.
For instance:
A = [1 2; 3 4]; % Creating a 2x2 matrix manually
In this example, the matrix `A` is defined explicitly, which can be used in various computations and analyses.
Data Manipulation Commands
Manipulating data is critical in programming, and MATLAB simplifies this with commands such as `sort`, `length`, and `size`. For instance, if you have a vector of numbers and want to sort them, you can do so easily:
vec = [5, 2, 8, 3]; % Define an unsorted vector
sortedVec = sort(vec); % Sort the vector in ascending order
disp(sortedVec); % Display the sorted vector
This straightforward operation demonstrates MATLAB's power in managing and processing datasets efficiently.
Control Flow Commands
Understanding control flow in MATLAB empowers you to operate conditionally and repetitively. Familiar structures include `if-else` statements and loops like `for` and `while`. Here’s a basic `for` loop example:
for i = 1:5
disp(['Current index: ', num2str(i)]); % Display the current index
end
This loop will output the current index five times, showcasing how to iterate over a sequence of numbers in MATLAB.
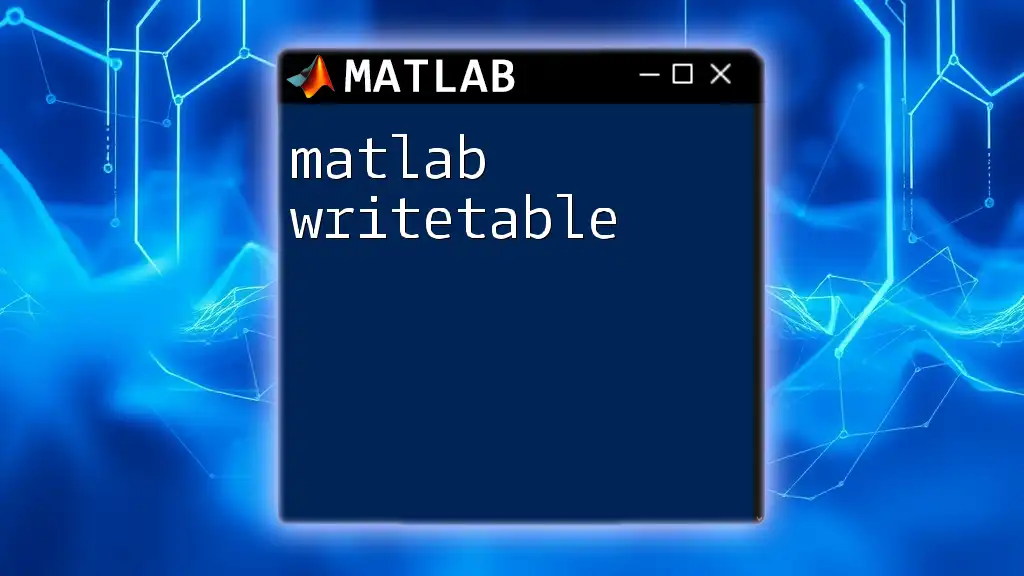
Best Practices for MATLAB Coding
Write Readable Code
When writing code, clarity is critical. Aim for readability by structuring your code with appropriate spacing, grouping related operations, and consistently naming your variables.
Leverage MATLAB Documentation
MATLAB comes with extensive built-in documentation. Harness this resource by using the `help` command to learn about functions, syntax, and toolboxes:
help plot % Displays information on the 'plot' function
Mastering the ability to use the help function can significantly enhance your coding efficiency and understanding.
Collaborate and Share Code
Collaboration is integral to programming, and MATLAB supports code sharing through platforms like GitHub. Familiarizing yourself with version control systems allows you to maintain your code and collaborate efficiently with others, enhancing the overall quality and productivity of your projects.
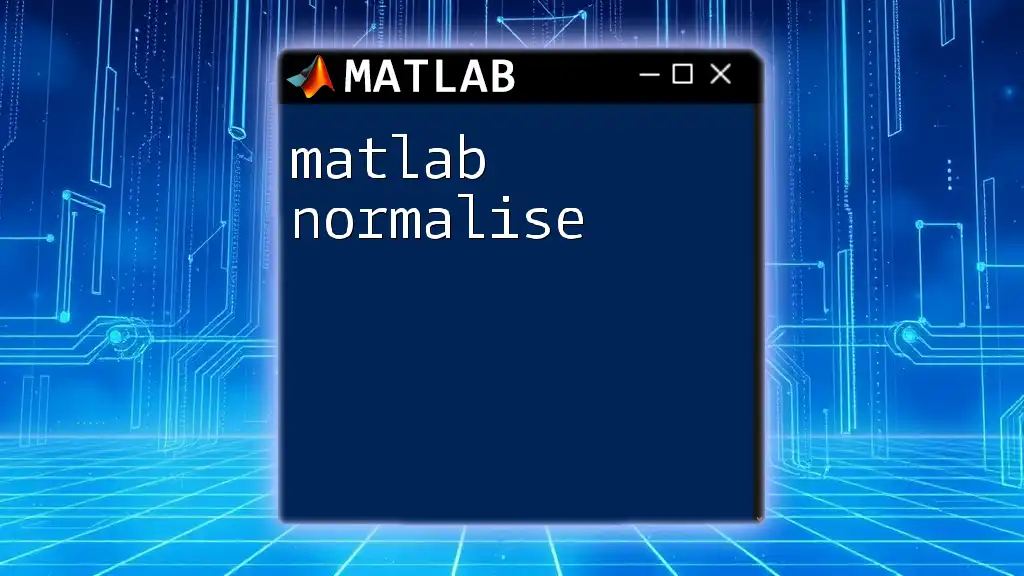
Conclusion
Understanding MATLAB or matlab is pivotal for anyone engaged in technical computing. By grasping its foundational features and adhering to best practices, users can unlock formidable capabilities in data analysis, visualization, and mathematical computation. With consistent practice and exploration of MATLAB's myriad features, you will elevate your programming journey.
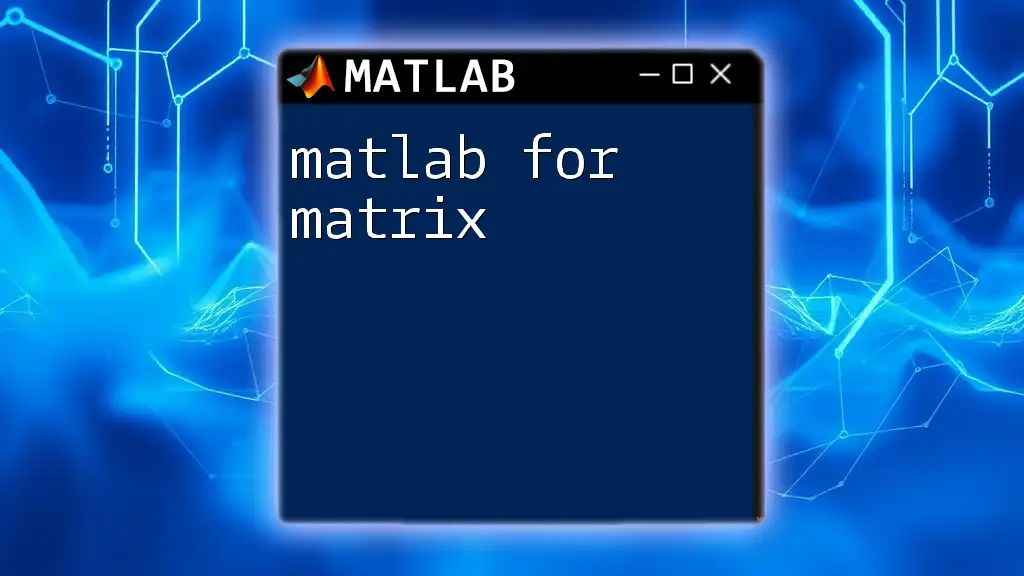
Call to Action
I encourage you to explore further and subscribe to our courses focused on quick MATLAB command usage, designed to enhance your proficiency efficiently. Join our community in the comments section, where we can all learn and grow together in our MATLAB journey.
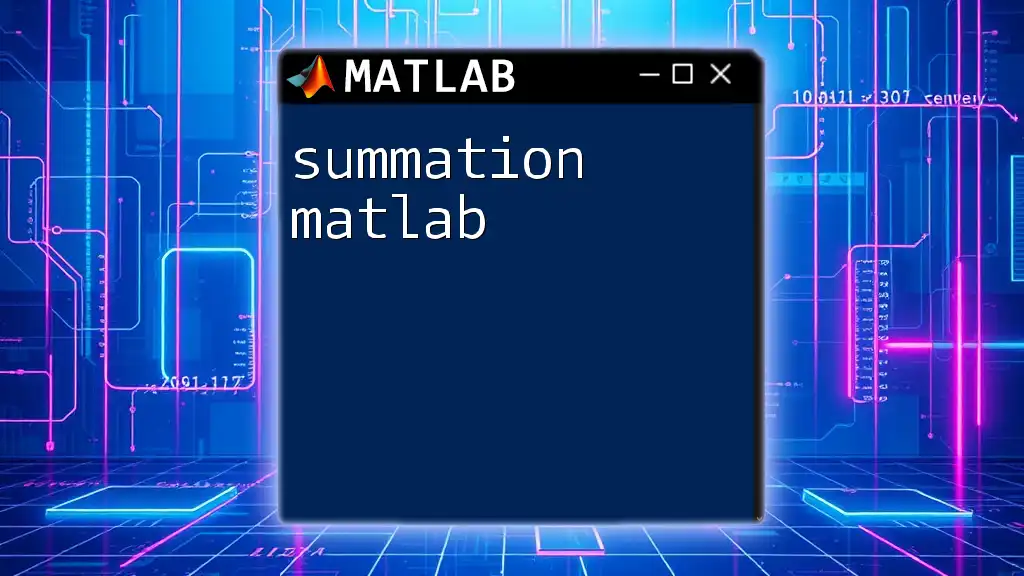
Additional Resources
For continued learning, consider exploring recommended textbooks and online courses that dive deep into MATLAB programming. Additionally, the comprehensive user guides and toolboxes available on the MathWorks website provide invaluable insights and support.