MATLAB offers various plotting options that allow users to visualize data effectively through simple commands, enabling quick customization of graphics, such as line styles, colors, and markers.
Here’s a basic example of how to create a plot with customized options:
x = 0:0.1:10;
y = sin(x);
plot(x, y, 'r--o', 'LineWidth', 2, 'MarkerSize', 6);
title('Sine Wave');
xlabel('X-axis');
ylabel('Y-axis');
grid on;
Understanding MATLAB Plotting Basics
What are MATLAB Plots?
MATLAB provides a powerful environment for creating various types of plots that help visualize data. Whether you are a data scientist, engineer, or researcher, grasping the concept of MATLAB plots is essential for effective data interpretation. In MATLAB, plotting is not just about representation; it’s about enabling insights through visibility.
Setting Up the Environment
Before diving into plotting commands, you need to ensure your MATLAB environment is ready.
- Installing MATLAB: Download MATLAB from the official MathWorks website and follow the installation instructions supplied.
- Opening MATLAB: Once installed, launch the application. You’ll see interfaces such as the command window where you can input commands directly, and the workspace that displays variables you’ve created.
- Setting Up Your Workspace: To make your work efficient, set the current folder to where you’ll save your scripts and output.
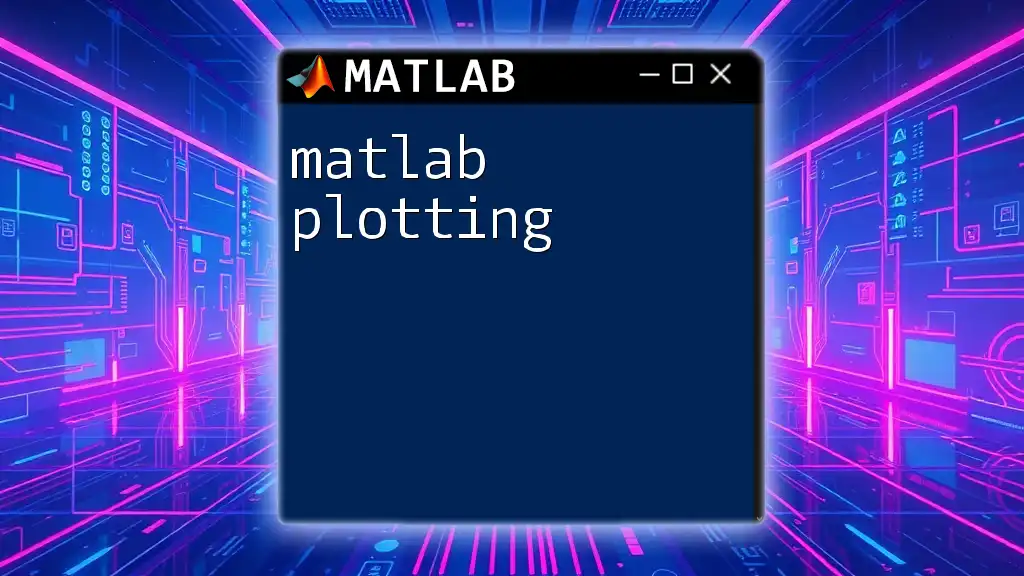
Basic Plotting Commands
The `plot` Function
The cornerstone of MATLAB plotting is the `plot` function, which creates 2D line graphs.
- Usage: The basic syntax is straightforward: `plot(X, Y)`, where X and Y are vectors of the same length.
Example Code Snippet:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
This code generates a sine wave from 0 to 10. It transforms numerical data into a graphical format, making trends and patterns easier to identify.
Adding Titles and Labels
To enhance your plots, adding titles and axis labels fosters a clearer understanding of what the graph conveys.
Adding Title
To insert a title to your plot, you can utilize the `title` function:
Code Example:
title('Sine Wave Plot');
This command provides context to the viewer and highlights the purpose of the graph.
Labeling Axes
Use `xlabel` and `ylabel` to label your axes, clarifying what the data represents.
Code Example:
xlabel('Time (s)');
ylabel('Amplitude');
With these labels, viewers can interpret the x-axis as time and the y-axis as amplitude, significantly enhancing the graph's informativeness.
Customizing the Plot
Customization is where MATLAB plotting becomes powerful.
Line Styles and Colors
The aesthetics of your plots can be adjusted using different line styles and colors.
- Available Line Styles: Solid (`'-'`), dashed (`'--'`), and dotted (`':'`) lines.
- Customizing Colors: You can specify colors using short color codes like `'r'` for red, `'g'` for green.
Example Code Snippet:
plot(x, y, 'r--'); % Red dashed line
This configuration makes the plot visually appealing and helps distinguish between multiple datasets.
Markers
Markers are symbols placed at data points which help highlight specific values in the data series.
Example Code Snippet:
plot(x, y, 'o'); % Circle marker at each data point
Integrating markers can significantly improve the interpretability of dense data plots.
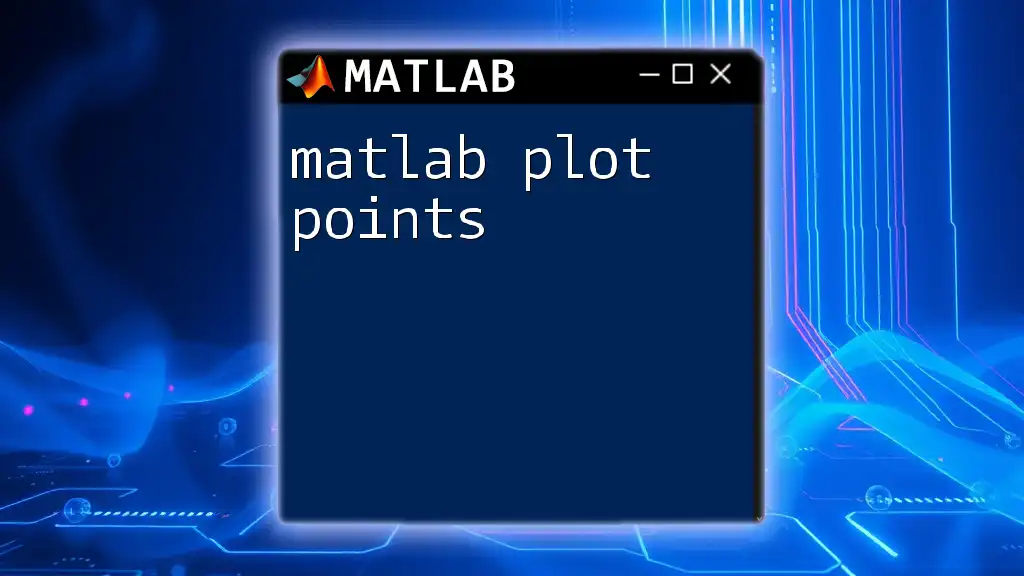
Advanced Plot Types
Bar and Histogram Plots
Different types of data visualization might require a different approach.
Creating Bar Graphs
Bar graphs are ideal for comparing different categories or groups.
Example Code Snippet:
categories = {'A', 'B', 'C'};
values = [10, 20, 15];
bar(values);
set(gca, 'XTickLabel', categories);
This demonstrates how to create a bar graph where categories 'A', 'B', and 'C' are clearly labeled on the x-axis with corresponding values.
Creating Histograms
Histograms provide insights into the distribution of data.
Example Code Snippet:
data = randn(1, 1000);
histogram(data);
This example generates a histogram from random data, showcasing its distribution.
3D Plots
3D plots offer a more dynamic representation of three-dimensional datasets.
Using `surf` for Surface Plots
The `surf` function creates surface plots, providing depth to your visualization.
Example Code Snippet:
[X, Y] = meshgrid(-5:0.5:5, -5:0.5:5);
Z = sin(sqrt(X.^2 + Y.^2));
surf(X, Y, Z);
This code snippet showcases a 3D surface plot that renders the sine function over a mesh grid.
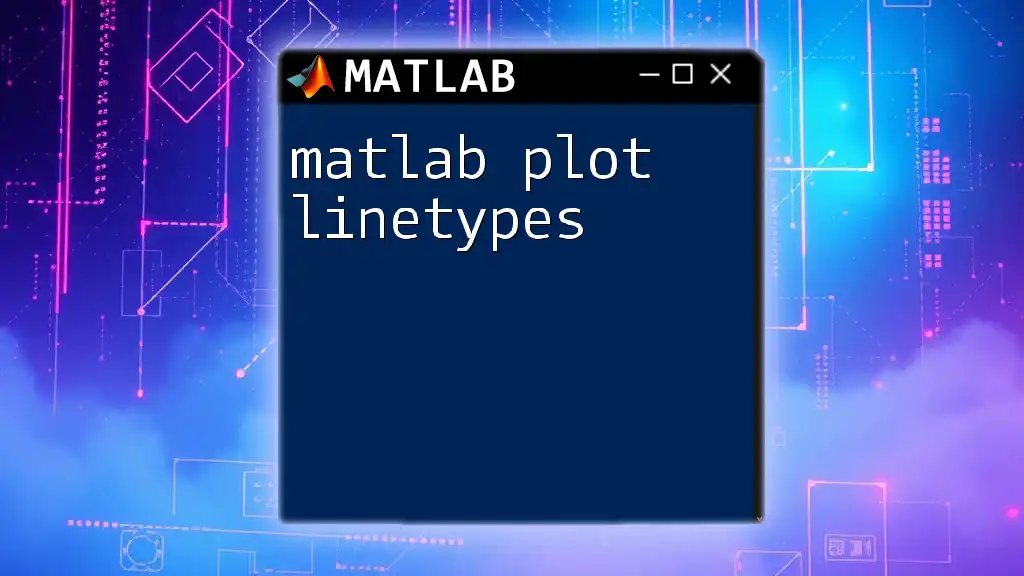
Customizing Appearance
Setting Axes Limits and Scale
Modify the limits of the axes to zoom in on data points of interest.
- Usage: Functions like `xlim`, `ylim`, and `zlim` allow explicit control over the displayed range.
Example Code Snippet:
xlim([-10, 10]);
ylim([-1, 1]);
Setting these limits can prevent the inclusion of irrelevant data, enhancing clarity.
Legends and Annotations
Adding legends and annotations provides context and details to the different data represented in your plots.
Adding Legends
Legends help explain which data series corresponds to which part of the graph.
Example Code Snippet:
legend('Sine Function');
Utilizing legends makes it easier for viewers to understand complex plots that feature multiple datasets.
Adding Annotations
Annotations can be used to add specific information directly onto the plot.
Example Code Snippet:
text(5, 0, 'Peak Point', 'FontSize', 12);
Annotations, such as this peak point marker, enhance the viewer's ability to quickly grasp key insights from the graph.
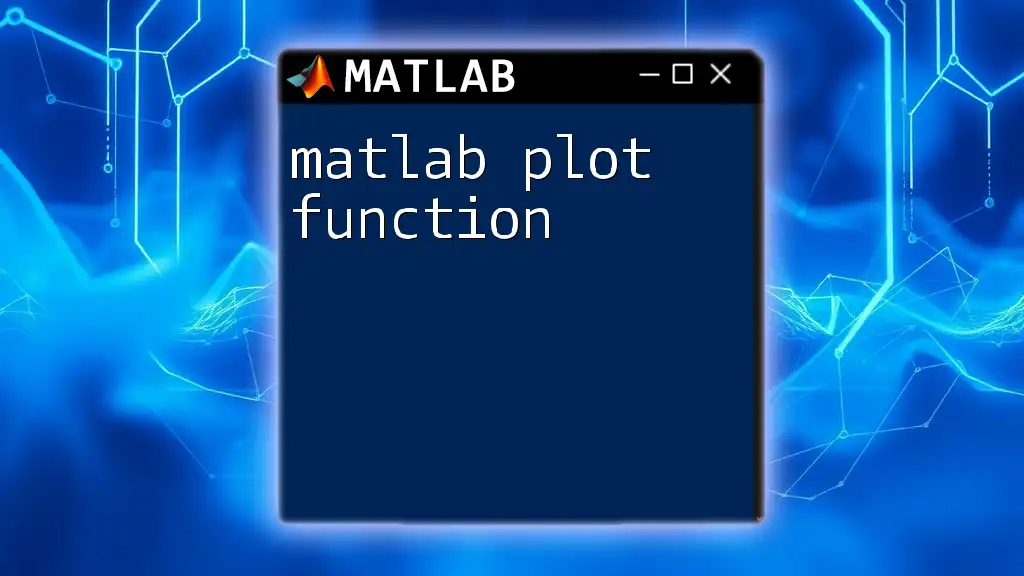
Exporting and Saving Plots
Saving Plots to Files
To share or use plots externally, you can easily save them in various formats.
- File Formats: Common formats include PNG, JPEG, and PDF.
Example Code Snippet:
saveas(gcf, 'myPlot.png');
This command saves the current figure as a PNG file, making it suitable for presentations or reports.
Using Print Command
For high-quality output, the `print` function offers precise control over plot output.
Example Code Snippet:
print('myPlot','-dpng');
Using `print`, you can specify the resolution and format, ensuring your plots maintain quality across various platforms.
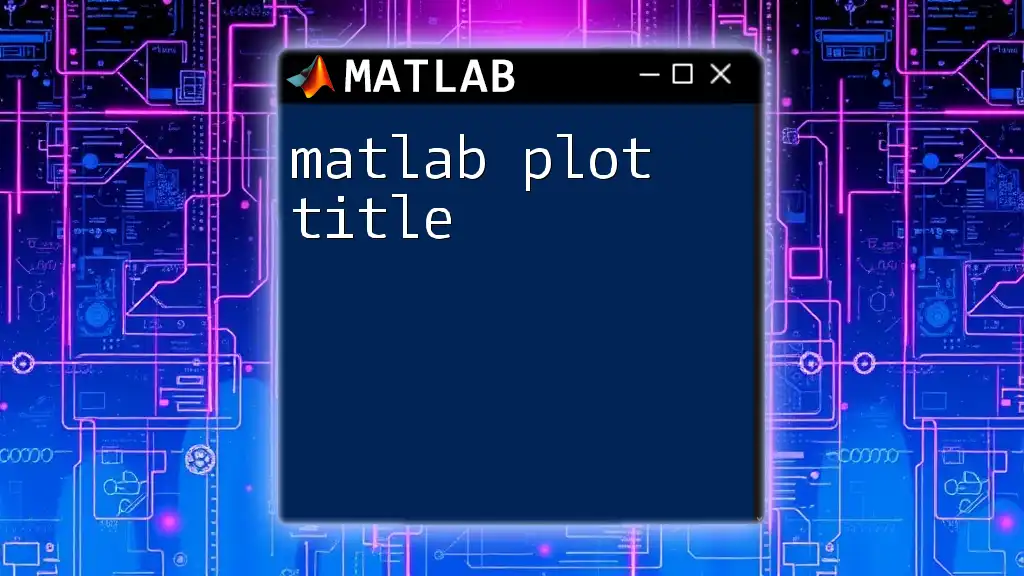
Conclusion
Understanding MATLAB plot options is crucial for transforming raw data into meaningful visuals. By mastering the commands and customizing elements, you can create compelling and informative plots. Effective data visualization is not just about representing numbers; it’s about telling a story that leads to insightful conclusions. Embrace these MATLAB features, practice, and explore further to elevate your data visualization skills.