The percentage difference between two datasets in MATLAB can be calculated using the formula:
\[ \text{Percentage Difference} = \frac{|A - B|}{\frac{(A + B)}{2}} \times 100 \]
where A and B are the data points from the two datasets, as demonstrated in the following MATLAB code snippet:
A = [10, 20, 30]; % First dataset
B = [8, 22, 28]; % Second dataset
percentageDifference = abs(A - B) ./ ((A + B) / 2) * 100;
disp(percentageDifference);
Understanding Percentage Difference
Mathematical Formula
To compute the MATLAB percentage difference between two datasets, we utilize the following formula:
\[ \text{Percentage Difference} = \left(\frac{|A - B|}{\frac{|A + B|}{2}}\right) \times 100 \]
This formula compares values in both datasets, providing a relative measure of difference:
- A and B represent the two datasets.
- The numerator captures the absolute difference between the two values.
- The denominator averages the two values, allowing the result to reflect a percentage difference relative to their average.
When to Use Percentage Difference
Percentage difference is especially valuable in scenarios where different datasets represent the same phenomenon, such as:
- Comparing experimental data with theoretical predictions.
- Analyzing results from before and after a treatment in scientific research.
- Evaluating performance metrics across different models in machine learning.
While absolute differences can tell us how much values vary, the percentage difference offers insights into the significance of that variation.
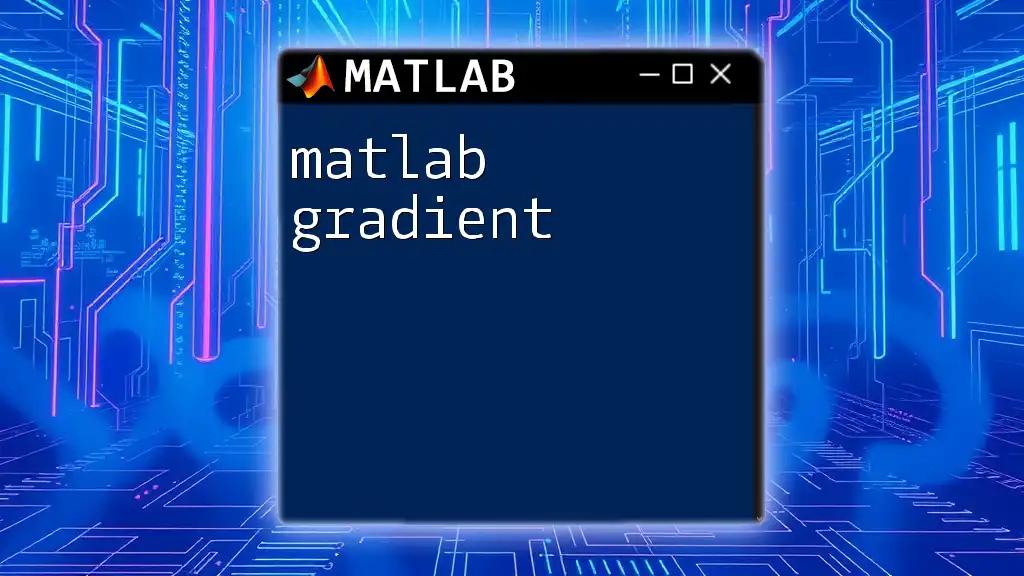
Preparing Your Datasets in MATLAB
Importing Data into MATLAB
The first step in calculating MATLAB percentage difference between two datasets is the proper importation of your datasets. MATLAB supports various file formats such as CSV and Excel.
To import data, you can use the following command:
dataA = readtable('datasetA.csv');
dataB = readtable('datasetB.csv');
Formatting Data for Analysis
Once imported, it’s critical that both datasets are of equal dimensions. This means that each corresponding data point in the two datasets can be directly compared. Additional preparations may be necessary to ensure data integrity:
- Remove any missing values or non-numeric data using `rmmissing`.
- Use the following example to clean your datasets:
dataA = rmmissing(dataA);
dataB = rmmissing(dataB);
By ensuring cleanliness and proper format, your calculations will be more robust.
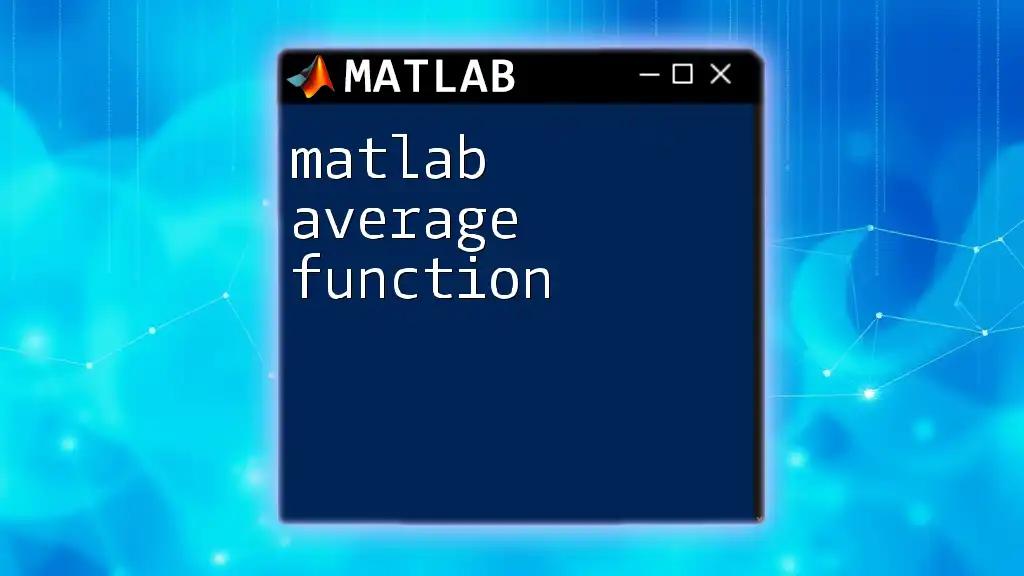
Calculating Percentage Difference in MATLAB
Basic Calculation
The calculation of percentage difference can be achieved directly using MATLAB. For vectorized datasets, you can compute the percentage difference with ease:
percentageDifference = abs(dataA - dataB) ./ mean([dataA, dataB], 2) * 100;
In this line:
- `abs(dataA - dataB)` computes the absolute difference.
- `mean([dataA, dataB], 2)` calculates the average of the corresponding elements from the two datasets.
Vectorized Operations for Efficiency
MATLAB excels in handling operations vectorized, which significantly boosts performance by minimizing the use of loops. This method is straightforward and ideal for large datasets. Here’s how you can implement it:
percentageDifference = arrayfun(@(x, y) abs(x - y) / mean([x, y]) * 100, dataA, dataB);
In this example, `arrayfun` processes each element from datasets `dataA` and `dataB`, applying the percentage difference calculation over the vectors efficiently.
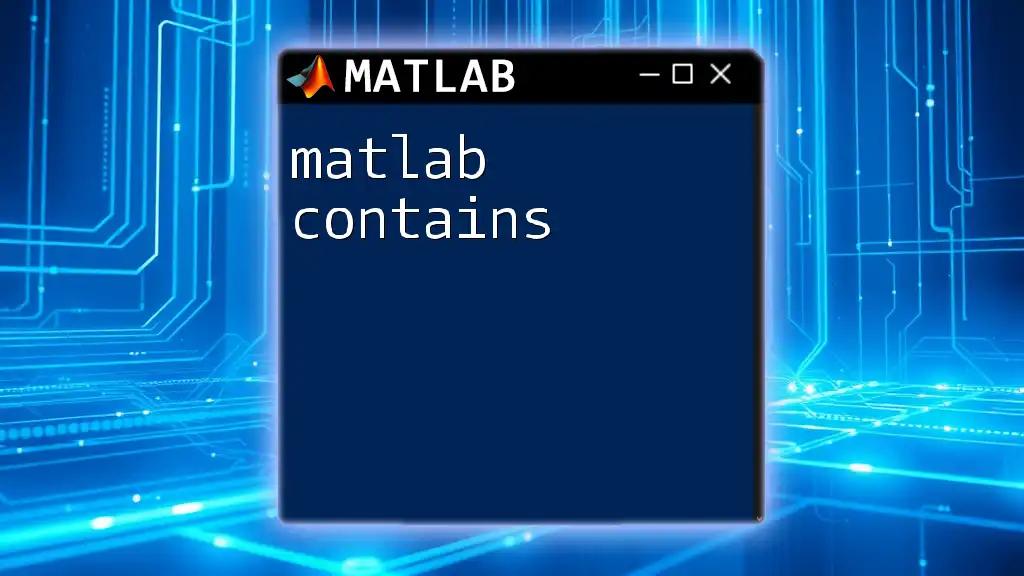
Visualizing Percentage Difference
Creating visual representations of your results is crucial for effective data analysis. MATLAB provides various plotting functions for this purpose.
To create a bar plot for the percentage differences, you can utilize the code:
bar(percentageDifference);
xlabel('Data Points');
ylabel('Percentage Difference (%)');
title('Percentage Difference Between Datasets A and B');
Visuals greatly enhance understanding and allow for insightful analyses at a glance.
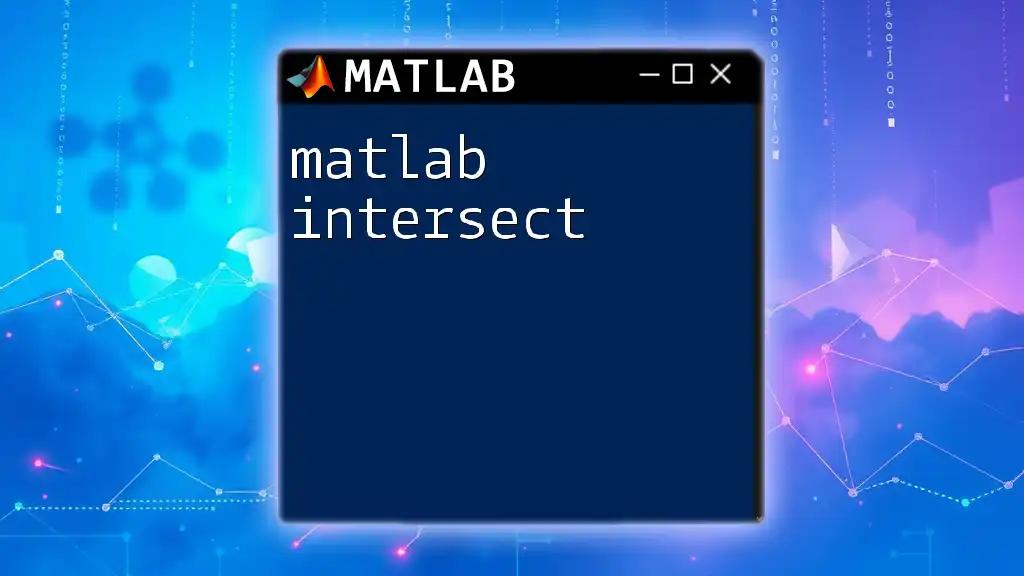
Interpreting Results
Once you have your percentage differences calculated and visualized, it’s essential to interpret these results accurately:
- A percentage difference of 0% indicates no difference.
- A higher percentage represents a greater disparity between datasets, which may be of significant concern in fields such as quality control or social sciences.
While low percentages may seem trivial, it's important to understand the context; sometimes a small numerical difference can have substantial implications in practical applications.
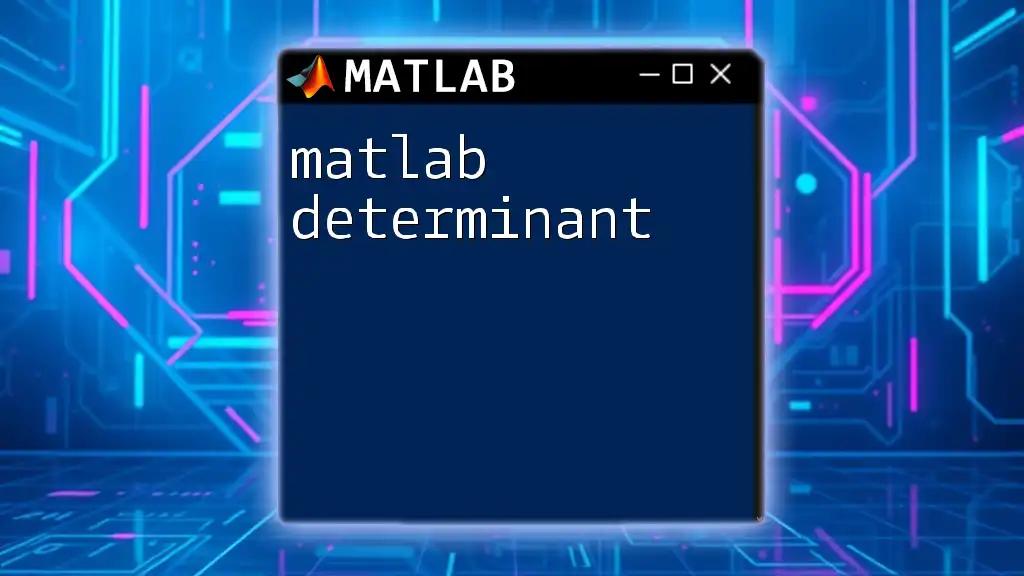
Common Pitfalls and Troubleshooting
When working with MATLAB percentage difference between two datasets, several common errors may arise:
- Mismatched dimensions can lead to calculation errors, causing MATLAB to throw dimension mismatch warnings.
- Neglecting data cleaning (like missing values) can skew outcomes dramatically.
To avoid these pitfalls:
- Always check the dimensions of your datasets using the `size` function.
- Troubleshoot your code by using breakpoints and the MATLAB debugger to pinpoint issues directly.
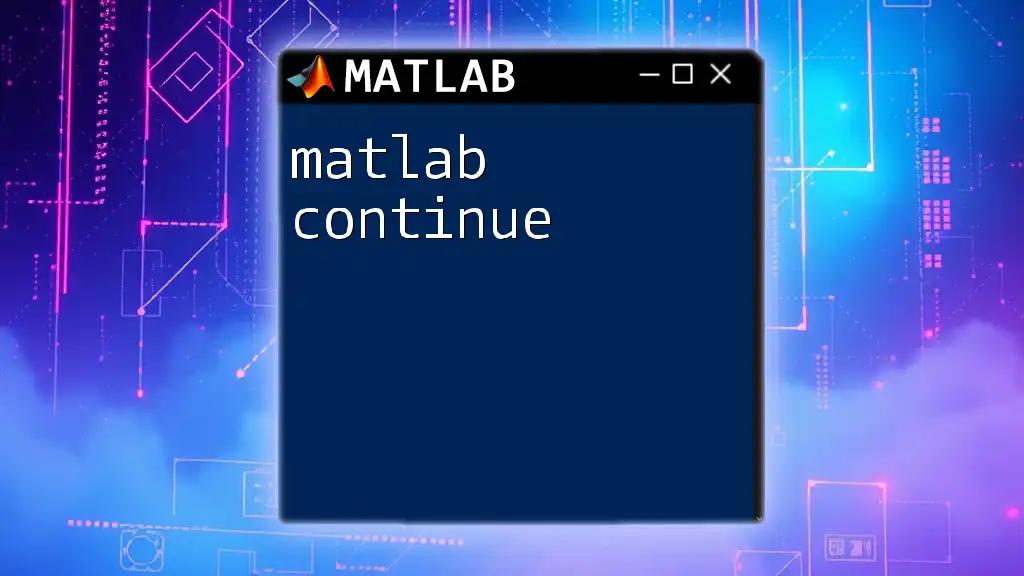
Conclusion
In summary, understanding how to compute and interpret the MATLAB percentage difference between two datasets is a powerful skill for any data analyst. By harnessing the capabilities of MATLAB, you can efficiently process datasets, calculate relevant differences, visualize results, and gain critical insights into your data.
To further enhance your MATLAB proficiency, explore additional resources, participate in workshops, and practice with diverse datasets. This will not only solidify your understanding but prepare you for real-world applications where data-driven decisions are essential.